Hiring skilled Visual Basic developers can be challenging without the right interview questions. A well-structured interview process helps you assess candidates' programming skills and find the best fit for your team.
This blog post provides a comprehensive list of Visual Basic interview questions categorized by difficulty level and topic. From basic concepts to advanced programming scenarios, we cover a wide range of questions to help you evaluate candidates at different experience levels.
By using these questions, you can effectively gauge a candidate's Visual Basic proficiency and problem-solving abilities. Consider combining these interview questions with a pre-employment VB.NET skills test for a more thorough evaluation of potential hires.
Table of contents
15 basic Visual Basic interview questions and answers to assess candidates
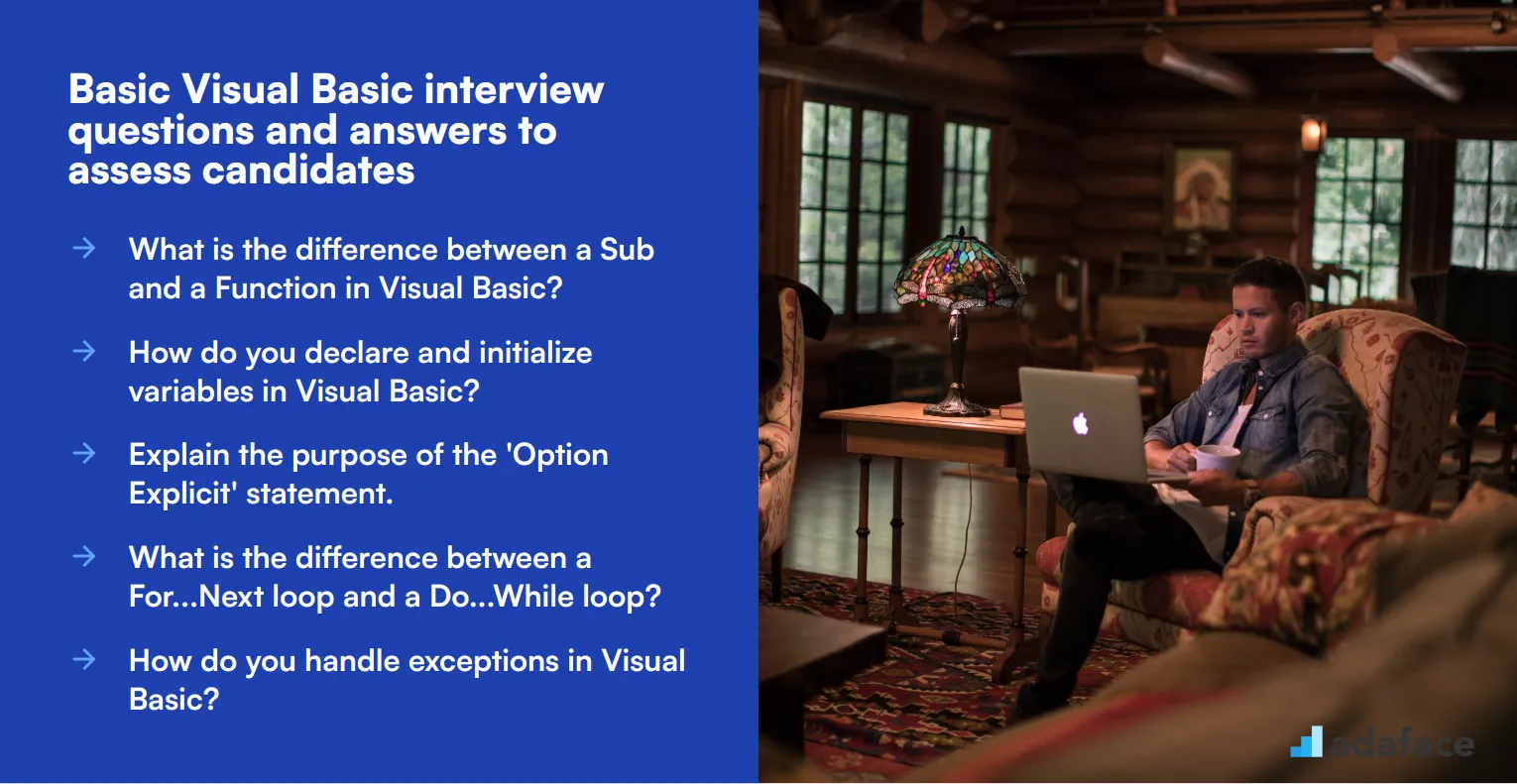
To assess candidates' foundational knowledge of Visual Basic, use these 15 basic interview questions. These questions will help you evaluate a software developer's understanding of VB syntax, core concepts, and common programming tasks.
- What is the difference between a Sub and a Function in Visual Basic?
- How do you declare and initialize variables in Visual Basic?
- Explain the purpose of the 'Option Explicit' statement.
- What is the difference between a For...Next loop and a Do...While loop?
- How do you handle exceptions in Visual Basic?
- What is the purpose of the 'With' statement in VB?
- How do you create and use arrays in Visual Basic?
- Explain the difference between 'ByVal' and 'ByRef' when passing parameters.
- What is the purpose of the 'MsgBox' function in Visual Basic?
- How do you connect to a database using Visual Basic?
- What is the difference between a class module and a standard module?
- How do you create and use custom events in Visual Basic?
- Explain the concept of inheritance in Visual Basic.
- What is the purpose of the 'ReDim' statement in VB?
- How do you implement error handling in Visual Basic?
8 Visual Basic interview questions and answers to evaluate junior developers
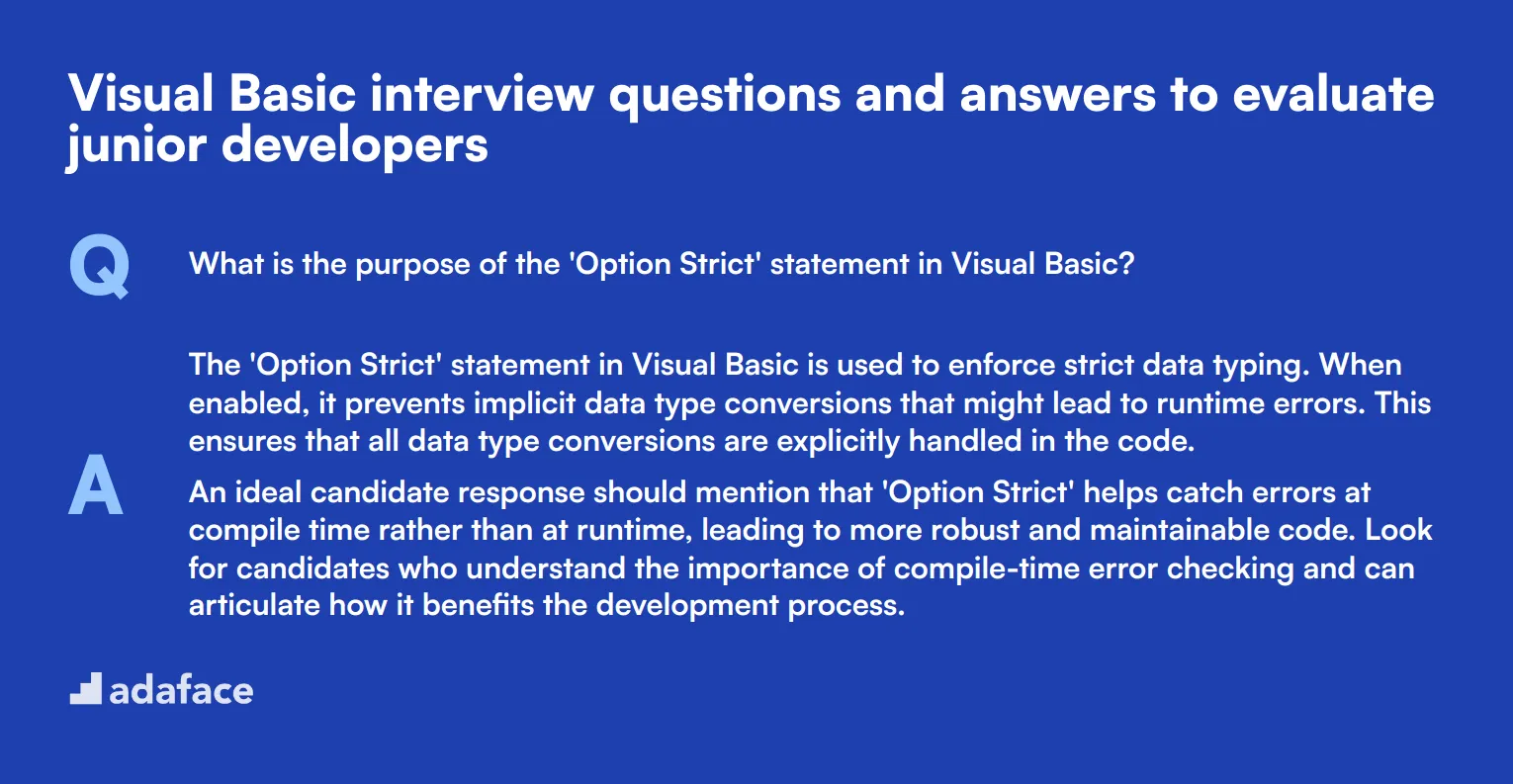
To gauge whether your junior developers have a solid grasp on Visual Basic essentials, these 8 interview questions and answers can be a great starting point. Use them to identify candidates who possess the foundational knowledge needed to grow and excel in your team.
1. What is the purpose of the 'Option Strict' statement in Visual Basic?
The 'Option Strict' statement in Visual Basic is used to enforce strict data typing. When enabled, it prevents implicit data type conversions that might lead to runtime errors. This ensures that all data type conversions are explicitly handled in the code.
An ideal candidate response should mention that 'Option Strict' helps catch errors at compile time rather than at runtime, leading to more robust and maintainable code. Look for candidates who understand the importance of compile-time error checking and can articulate how it benefits the development process.
2. Can you explain what properties are in Visual Basic and how they are used?
Properties in Visual Basic are special methods that provide a flexible mechanism to read, write, or compute the values of private fields. They are used to encapsulate a field, offering controlled access to it. Properties can have 'Get' and 'Set' accessors to define how values are retrieved or modified.
Candidates should highlight that properties are essential for data encapsulation and validation in object-oriented programming. An ideal response will also touch on the benefits of using properties over public fields, such as increased control over how data is accessed and modified.
3. How do you use the 'Select Case' statement in Visual Basic?
The 'Select Case' statement in Visual Basic is used to run one of several groups of statements, depending on the value of an expression. It's a cleaner alternative to multiple 'If...Then...Else' statements when there are multiple conditions to check.
Strong candidates will explain that 'Select Case' enhances code readability and maintainability by reducing the complexity of nested 'If' statements. Look for candidates who can provide examples of when and why they would use 'Select Case' in a real-world scenario.
4. What is a module in Visual Basic and how is it different from a class?
A module in Visual Basic is a container for procedures, functions, variables, and properties that can be accessed globally within an application. Unlike classes, modules cannot be instantiated and do not support inheritance.
An ideal answer should mention that modules are useful for grouping related procedures and variables that don't need to maintain state. Candidates who understand the differences between modules and classes, and can explain when to use each, demonstrate a strong foundational knowledge of Visual Basic.
5. How do you handle multiple forms in a Visual Basic application?
Handling multiple forms in a Visual Basic application typically involves creating instances of the forms and using methods like 'Show' and 'ShowDialog' to display them. 'Show' displays a form modelessly, allowing the user to interact with other forms, while 'ShowDialog' displays a form modally, blocking interaction with other forms until it is closed.
Candidates should highlight the importance of managing form state and navigation in a multi-form application. Look for responses that demonstrate an understanding of modal vs. modeless forms and the scenarios in which each type should be used.
6. What is the purpose of the 'Imports' statement in Visual Basic?
The 'Imports' statement in Visual Basic simplifies code by allowing you to reference namespaces without having to specify the full namespace path each time. It brings all the members of a namespace into scope, making the code cleaner and easier to read.
Ideal candidates should explain that 'Imports' helps in organizing and managing code, especially in larger projects with multiple namespaces. They should also be able to discuss how it contributes to better code readability and maintainability.
7. How do you manage user input validation in a Visual Basic application?
User input validation in a Visual Basic application can be managed using various techniques such as input validation methods, regular expressions, and error handling mechanisms. The goal is to ensure that the input meets the required format and constraints before processing it.
Strong answers will include examples of common validation techniques and explain how to provide feedback to users when inputs are invalid. Look for candidates who understand the importance of robust input validation to prevent errors and improve user experience.
8. What is the role of 'My' namespace in Visual Basic?
The 'My' namespace in Visual Basic provides easy access to various .NET Framework classes, properties, and methods that are commonly used in applications. It simplifies tasks such as file I/O, network operations, and accessing application settings.
An ideal response will highlight that 'My' namespace is designed to improve productivity by reducing the amount of code developers need to write. Candidates who can provide examples of how they've used 'My' in their projects demonstrate practical knowledge and experience.
10 intermediate Visual Basic interview questions and answers to ask mid-tier developers
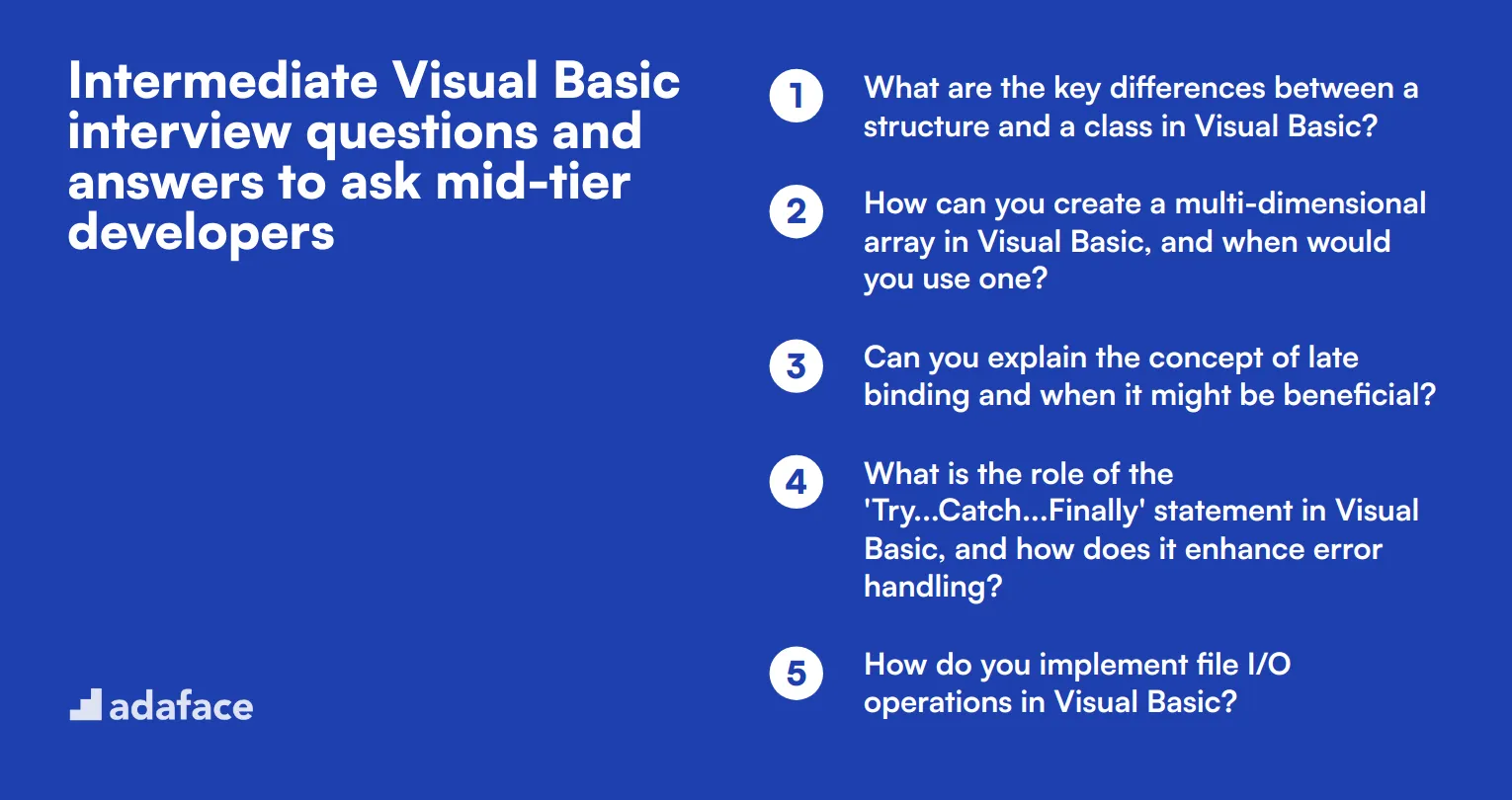
To assess whether candidates possess the necessary technical skills for mid-tier Visual Basic positions, utilize this list of intermediate interview questions. These questions will help gauge their practical knowledge and problem-solving abilities in real-world scenarios, ensuring you find the right fit for your team. For more insights on hiring developers, check out our job description resources.
- What are the key differences between a structure and a class in Visual Basic?
- How can you create a multi-dimensional array in Visual Basic, and when would you use one?
- Can you explain the concept of late binding and when it might be beneficial?
- What is the role of the 'Try...Catch...Finally' statement in Visual Basic, and how does it enhance error handling?
- How do you implement file I/O operations in Visual Basic?
- What are delegates and events, and how do they facilitate communication between objects?
- Can you describe how to use LINQ with Visual Basic for data manipulation?
- What is the significance of the 'Using' statement in resource management?
- How do you implement asynchronous programming in Visual Basic, and why is it important?
- Explain how to create and handle custom exceptions in Visual Basic.
6 Visual Basic interview questions and answers related to technical definitions
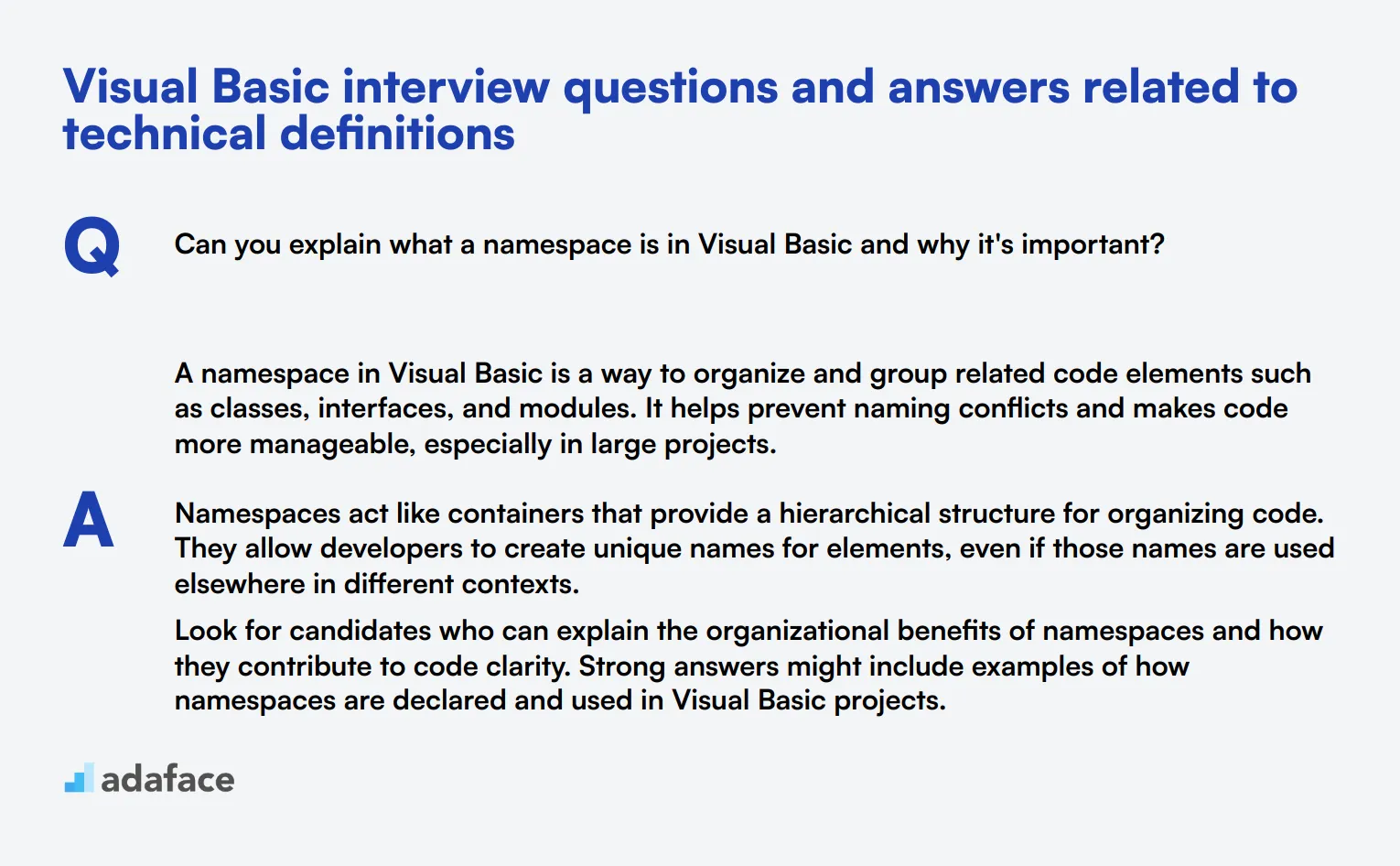
When interviewing for Visual Basic positions, it's crucial to assess candidates' understanding of technical definitions. These questions will help you gauge applicants' grasp of VB concepts without delving too deep into code. Use this list to evaluate candidates effectively and identify those with a solid foundation in Visual Basic terminology.
1. Can you explain what a namespace is in Visual Basic and why it's important?
A namespace in Visual Basic is a way to organize and group related code elements such as classes, interfaces, and modules. It helps prevent naming conflicts and makes code more manageable, especially in large projects.
Namespaces act like containers that provide a hierarchical structure for organizing code. They allow developers to create unique names for elements, even if those names are used elsewhere in different contexts.
Look for candidates who can explain the organizational benefits of namespaces and how they contribute to code clarity. Strong answers might include examples of how namespaces are declared and used in Visual Basic projects.
2. What is the difference between Early Binding and Late Binding in Visual Basic?
Early binding occurs at compile-time when the compiler knows the specific object type and can perform type checking. This results in faster code execution and better performance. Late binding, on the other hand, occurs at runtime and allows for more flexibility but at the cost of performance.
With early binding, IntelliSense is available, making development easier. Late binding is useful when working with objects that aren't known at compile-time, such as when using COM objects or when writing more dynamic code.
Ideal candidates should be able to discuss the trade-offs between performance and flexibility. Look for answers that demonstrate understanding of when to use each binding type in real-world scenarios.
3. Explain the concept of a constructor in Visual Basic.
A constructor in Visual Basic is a special method within a class that is automatically called when an instance of the class is created. Its primary purpose is to initialize the object's state, setting initial values for fields or performing any necessary setup.
In Visual Basic, constructors are defined using the 'New' keyword. They can be parameterless (default constructor) or parameterized, allowing for different initialization scenarios. Constructors don't have a return type, not even 'void'.
Strong candidates should be able to explain how constructors contribute to object-oriented design and encapsulation. Look for mentions of overloading constructors and how they can be used to provide flexibility in object creation.
4. What is the purpose of the 'Friend' keyword in Visual Basic?
The 'Friend' keyword in Visual Basic is an access modifier that allows a member (method, property, or variable) to be accessed from within the same project, but not from outside the project. It provides a level of accessibility between 'Private' and 'Public'.
Friend members are often used when you want to allow access to certain parts of your code within a project but restrict access from external code. This is particularly useful in creating internal APIs or when working on large projects with multiple assemblies.
Look for candidates who can explain how 'Friend' contributes to encapsulation and modular design. They should be able to contrast it with other access modifiers and provide scenarios where using 'Friend' is beneficial for code organization and security.
5. Can you explain what a delegate is in Visual Basic?
A delegate in Visual Basic is a type-safe function pointer. It's a reference type that can hold a reference to a method with a specific signature. Delegates allow methods to be passed as parameters, enabling flexible and extensible code designs.
Delegates are commonly used for implementing event handling, callback mechanisms, and defining abstract behaviors that can be customized. They provide a way to achieve loose coupling between components in an application.
Strong candidates should be able to explain how delegates contribute to software development patterns like the Observer pattern. Look for answers that include examples of declaring and using delegates, and how they differ from direct method calls.
6. What is the difference between a structure and an enumeration in Visual Basic?
A structure in Visual Basic is a value type that can contain multiple data members of different types. It's used to create custom data types and is stored on the stack. Structures are useful for small, data-centric objects that don't require inheritance.
An enumeration, on the other hand, is a distinct type that consists of a set of named constants. Enumerations are typically used to represent a fixed set of values, such as days of the week or card suits.
Look for candidates who can explain when to use structures versus enumerations. Strong answers might include discussions on memory usage, performance implications, and appropriate use cases for each. Candidates should also be able to provide simple examples of how to declare and use both structures and enumerations.
10 Visual Basic interview questions about programming concepts
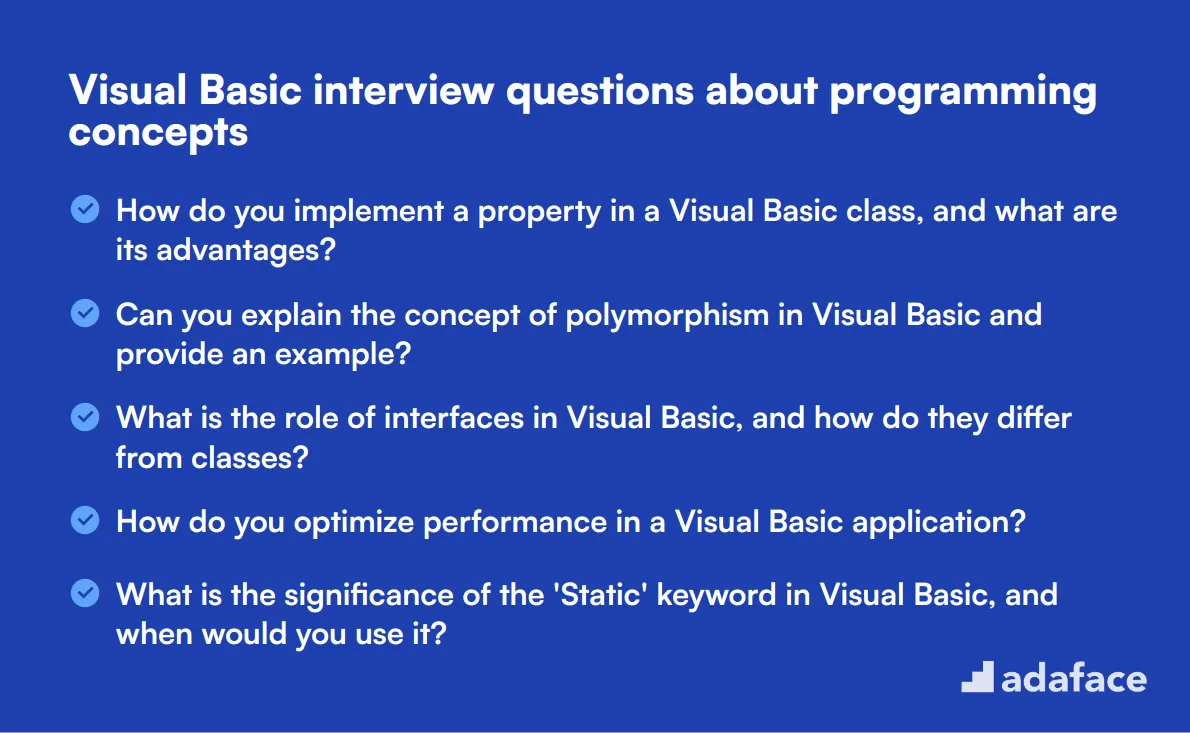
To assess a candidate's grasp of essential programming concepts in Visual Basic, utilize these targeted interview questions. They can help you gauge whether applicants possess the technical skills necessary for roles like a Software Developer.
- How do you implement a property in a Visual Basic class, and what are its advantages?
- Can you explain the concept of polymorphism in Visual Basic and provide an example?
- What is the role of interfaces in Visual Basic, and how do they differ from classes?
- How do you optimize performance in a Visual Basic application?
- What is the significance of the 'Static' keyword in Visual Basic, and when would you use it?
- Can you explain the difference between synchronous and asynchronous programming in Visual Basic?
- What are the key features of the Visual Basic .NET framework?
- How does garbage collection work in Visual Basic, and why is it important?
- Can you describe what a lambda expression is in Visual Basic and give an example of its use?
- What is the importance of comments in your Visual Basic code, and how do you effectively use them?
10 situational Visual Basic interview questions for hiring top developers
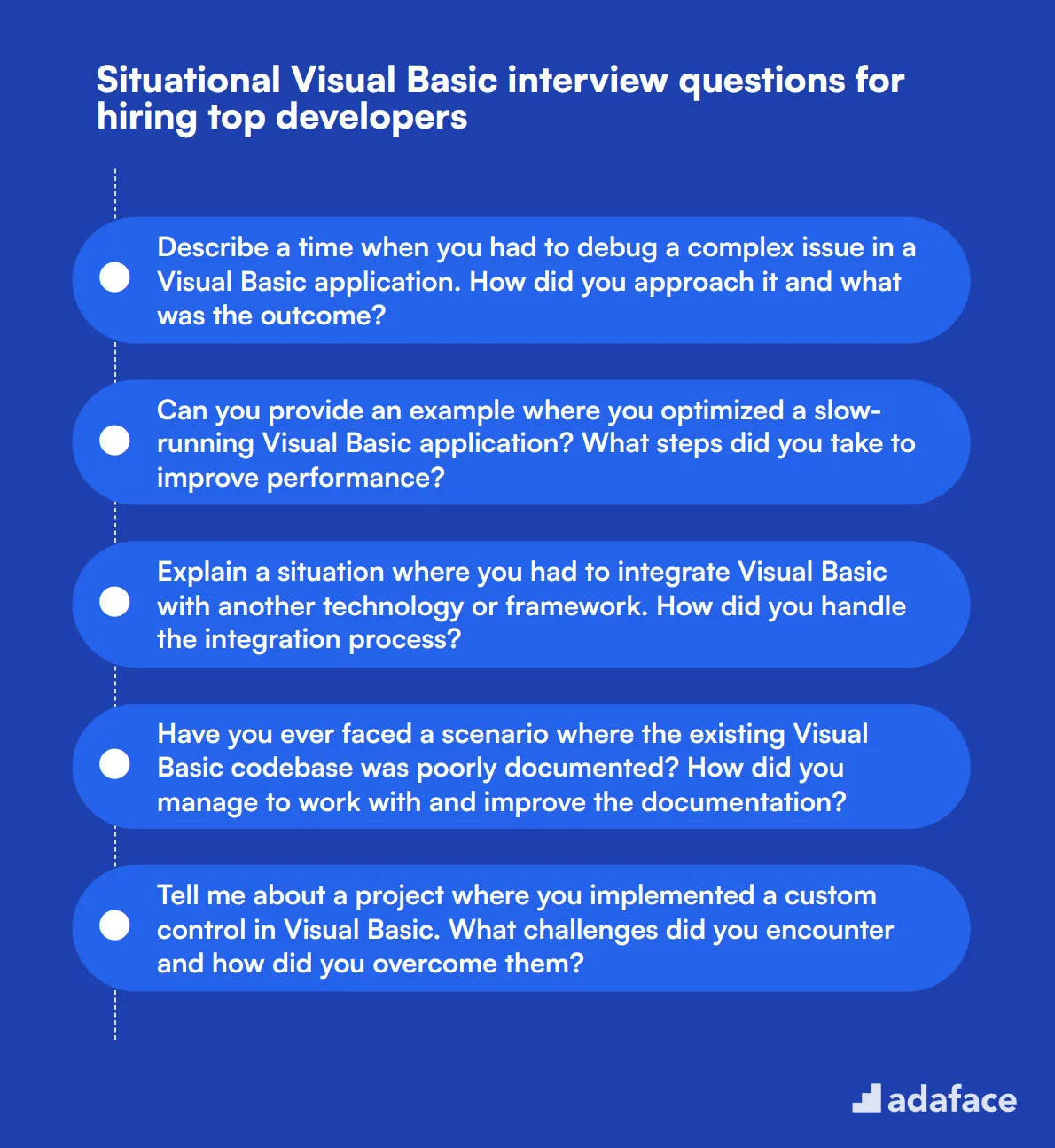
To identify top-tier Visual Basic developers, it's crucial to ask situational questions that reveal their problem-solving skills and practical knowledge. These 10 questions will help you understand how candidates handle real-world scenarios, ensuring they possess the expertise required for the role. For more specific job descriptions, you can check this application developer job description.
- Describe a time when you had to debug a complex issue in a Visual Basic application. How did you approach it and what was the outcome?
- Can you provide an example where you optimized a slow-running Visual Basic application? What steps did you take to improve performance?
- Explain a situation where you had to integrate Visual Basic with another technology or framework. How did you handle the integration process?
- Have you ever faced a scenario where the existing Visual Basic codebase was poorly documented? How did you manage to work with and improve the documentation?
- Tell me about a project where you implemented a custom control in Visual Basic. What challenges did you encounter and how did you overcome them?
- Can you discuss an instance where you had to refactor legacy Visual Basic code? What were the key improvements you made?
- Describe a situation where you had to handle concurrency in a Visual Basic application. What techniques did you use to manage simultaneous operations effectively?
- Have you ever had to migrate a Visual Basic application to a newer version or platform? What was your strategy and what challenges did you face?
- Can you explain a time when you had to ensure high security in a Visual Basic application? What measures did you implement to secure the application?
- Describe a scenario where you collaborated with a team on a Visual Basic project. How did you ensure effective communication and project success?
Which Visual Basic skills should you evaluate during the interview phase?
Conducting an interview to assess a candidate's proficiency in Visual Basic can be challenging. While a single interview might not cover every aspect of their skills, focusing on key areas can provide a solid understanding of their capabilities. Here are the fundamental skills you should evaluate during the interview phase.
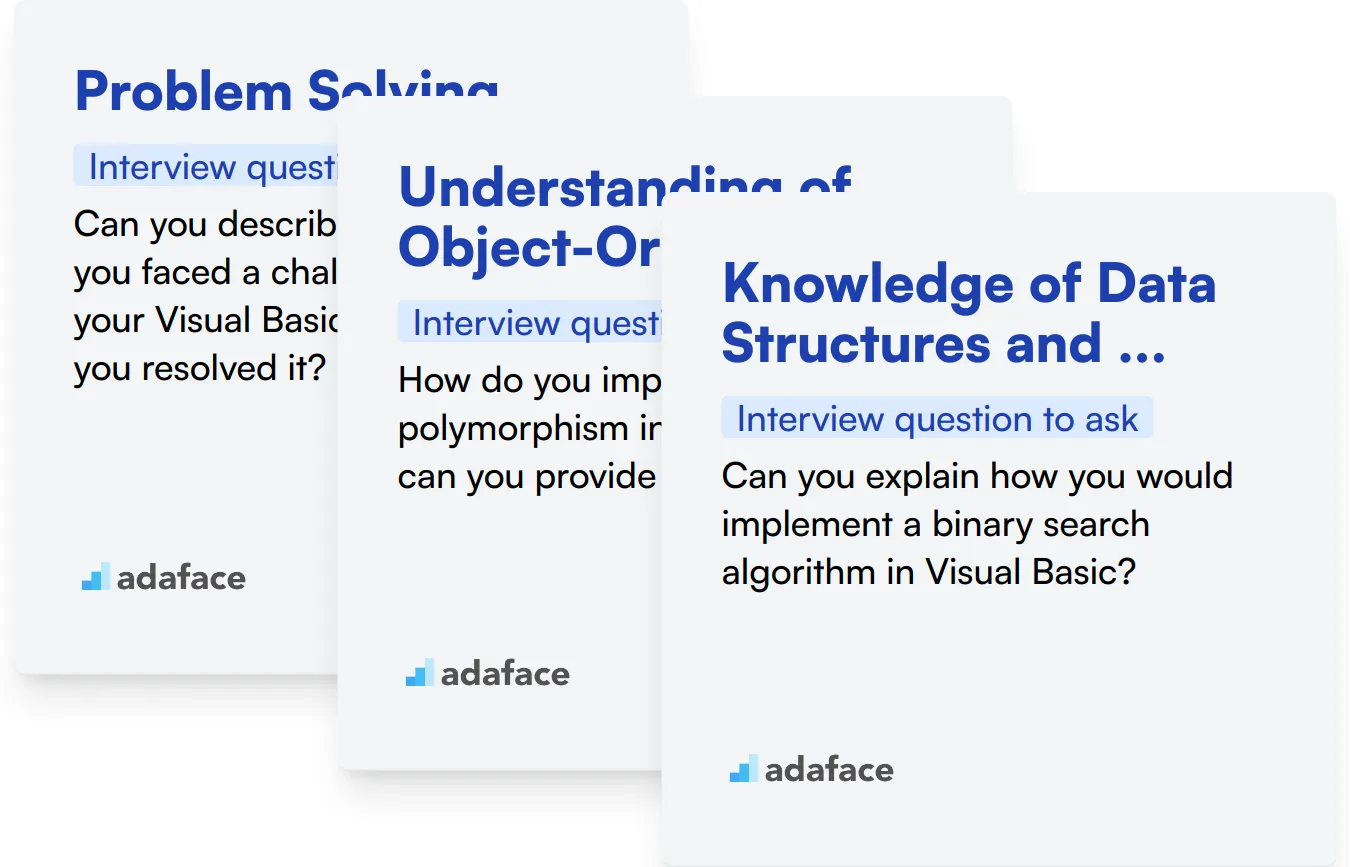
Problem Solving
You can use an assessment test that asks relevant MCQs to filter out strong problem-solvers. Our Visual Basic test includes questions designed to evaluate this skill.
In addition to MCQs, targeted interview questions can provide deeper insights into a candidate's problem-solving abilities.
Can you describe a time when you faced a challenging bug in your Visual Basic code and how you resolved it?
Look for a structured approach to identifying and resolving the bug. Pay attention to their problem-solving methodology, creativity, and ability to stay calm under pressure.
Understanding of Object-Oriented Programming
Consider an assessment test with relevant MCQs to gauge their grasp of OOP principles. Our Visual Basic test covers essential OOP concepts and principles.
To further probe their understanding, you can ask targeted questions on specific OOP concepts.
How do you implement polymorphism in Visual Basic, and can you provide an example?
Listen for a clear explanation of polymorphism, including practical examples. Assess their ability to apply theoretical knowledge to real-world scenarios.
Knowledge of Data Structures and Algorithms
Utilizing an assessment test with MCQs on data structures and algorithms can help filter candidates with strong foundational knowledge. Our Visual Basic test includes questions on these topics.
Ask specific questions during the interview to gauge their practical application of data structures and algorithms.
Can you explain how you would implement a binary search algorithm in Visual Basic?
Focus on their explanation of the binary search algorithm and their ability to code it in Visual Basic. Assess their understanding of algorithm efficiency and complexity.
3 Practical Tips for Using Visual Basic Interview Questions
Before you start applying what you've learned, consider these tips to enhance your interview process with Visual Basic candidates.
1. Utilize Skills Assessments Prior to Interviews
Using skills tests before interviews helps filter candidates based on their actual abilities, allowing you to focus on those who are truly qualified. Incorporating tests like the VB.NET Online Test can effectively evaluate essential programming skills.
By assessing candidates' abilities before the interview, you streamline the process and enhance your chances of selecting the right fit. This approach ensures that you engage only with candidates who have demonstrated the required skills, saving valuable time in the interview stage.
Integrating skills assessments into your hiring strategy provides a solid foundation for understanding a candidate's strengths and areas for development, which can lead to more informed decisions moving forward.
2. Select Relevant Interview Questions
Time is of the essence during interviews, so it's important to choose questions that effectively evaluate key competencies. Narrowing down your questions can help you gauge a candidate's expertise in Visual Basic without overwhelming them.
Consider including questions that touch on not just Visual Basic, but also relevant areas like SQL Server or C#. This will help assess their overall programming knowledge and adaptability.
Focusing on strategic questions will maximize your evaluation effectiveness, allowing you to explore critical soft skills such as communication and teamwork as well.
3. Incorporate Follow-Up Questions
Simply asking prepared interview questions is not enough; probing deeper with follow-up questions is essential. This approach helps uncover any discrepancies or surface-level answers candidates may provide, ensuring you find the right match for your role.
For instance, if a candidate states they have experience with Visual Basic error handling, a good follow-up question could be, 'Can you describe a specific instance where you handled a complex error?' This encourages candidates to elaborate on their experience, allowing you to gauge their depth of knowledge.
Use Visual Basic interview questions and skills tests to hire talented developers
If you’re looking to hire someone with Visual Basic skills, it's important to ensure they possess those skills accurately. The most effective way to do this is by using skill tests like the Visual Basic online test.
Once you have administered the test, you can shortlist the best applicants and invite them for interviews. To get started, sign up for our assessment platform here and explore our test library.
VB.NET Online Test
Download Visual Basic interview questions template in multiple formats
Visual Basic Interview Questions FAQs
The questions cover basic, junior, intermediate, and advanced skill levels, suitable for assessing various developer roles.
Combine these questions with skills tests, tailor them to your specific needs, and use them to evaluate candidates' problem-solving abilities.
Yes, the article includes situational and practical questions to assess candidates' hands-on Visual Basic programming skills.
Regularly review and update your questions to keep up with the latest Visual Basic developments and industry trends.
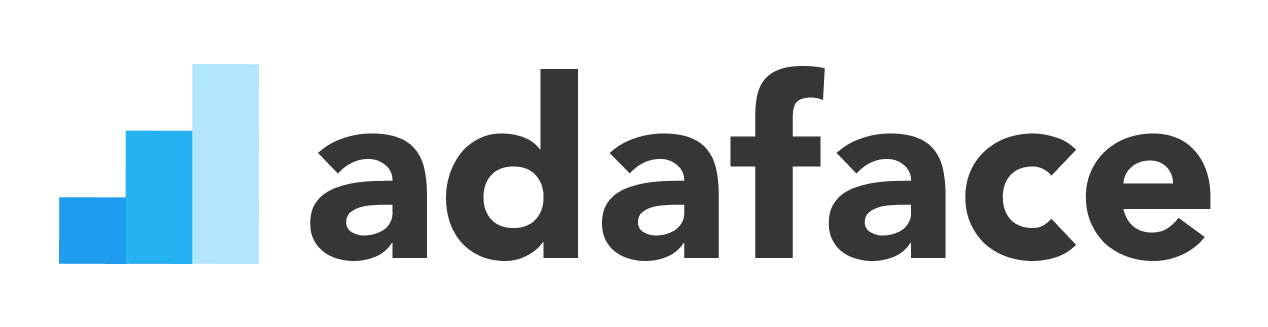
40 min skill tests.
No trick questions.
Accurate shortlisting.
We make it easy for you to find the best candidates in your pipeline with a 40 min skills test.
Try for freeRelated posts
Free resources
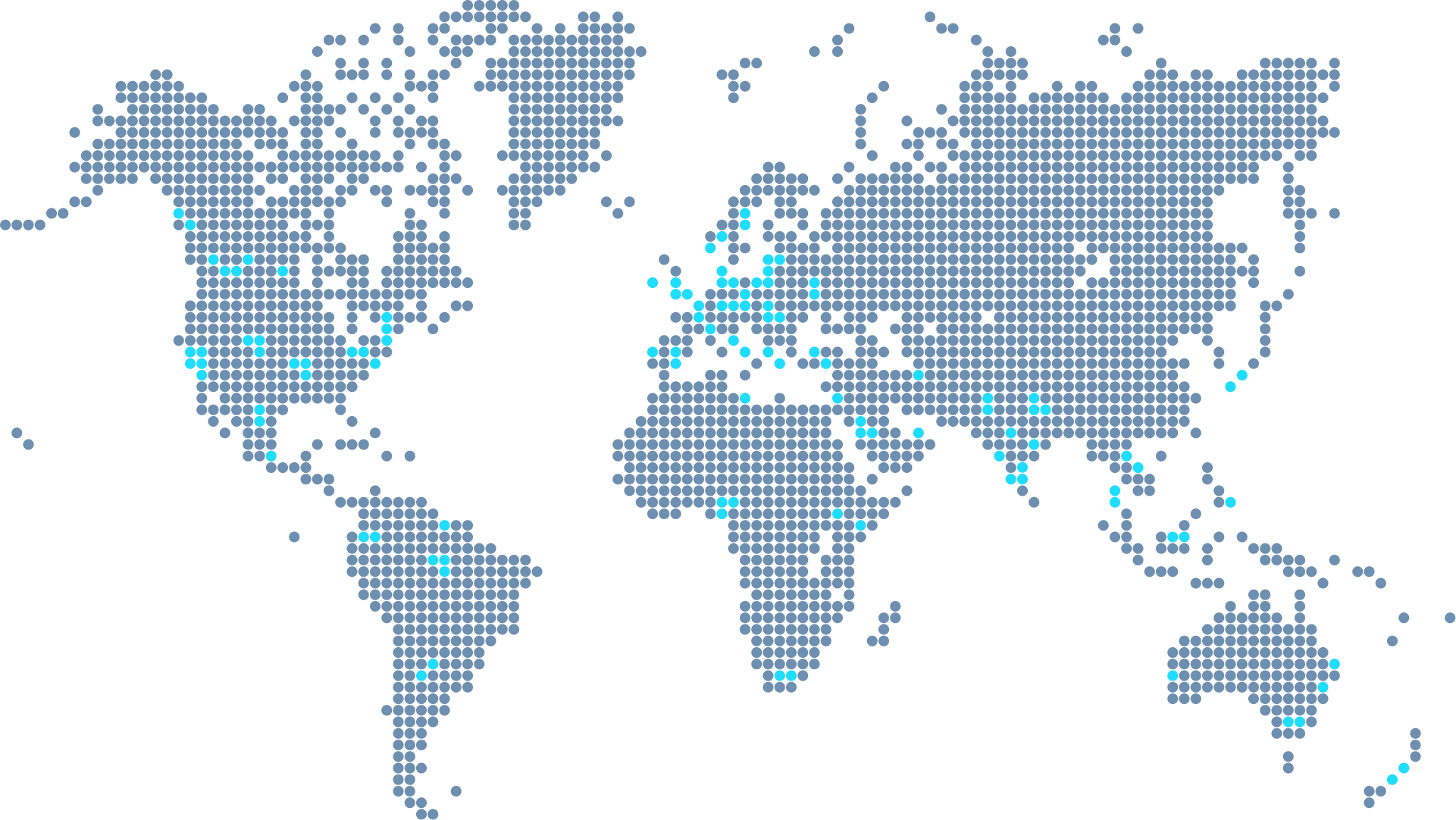
