Hiring the right VB .NET developer can be a challenge, especially when you're not sure what questions to ask during the interview process. A well-structured set of interview questions can help you identify candidates with the right skills and experience for your team.
This blog post provides a comprehensive list of VB .NET interview questions, ranging from basic to advanced, to help you assess candidates at different experience levels. We've organized the questions into categories, covering fundamental concepts, debugging techniques, error handling, and even situational scenarios to evaluate problem-solving skills.
By using these questions, you can gain valuable insights into a candidate's VB .NET proficiency and make informed hiring decisions. Consider complementing your interview process with a VB .NET online test to get a more complete picture of a candidate's abilities.
Table of contents
10 basic VB .NET interview questions and answers to assess applicants
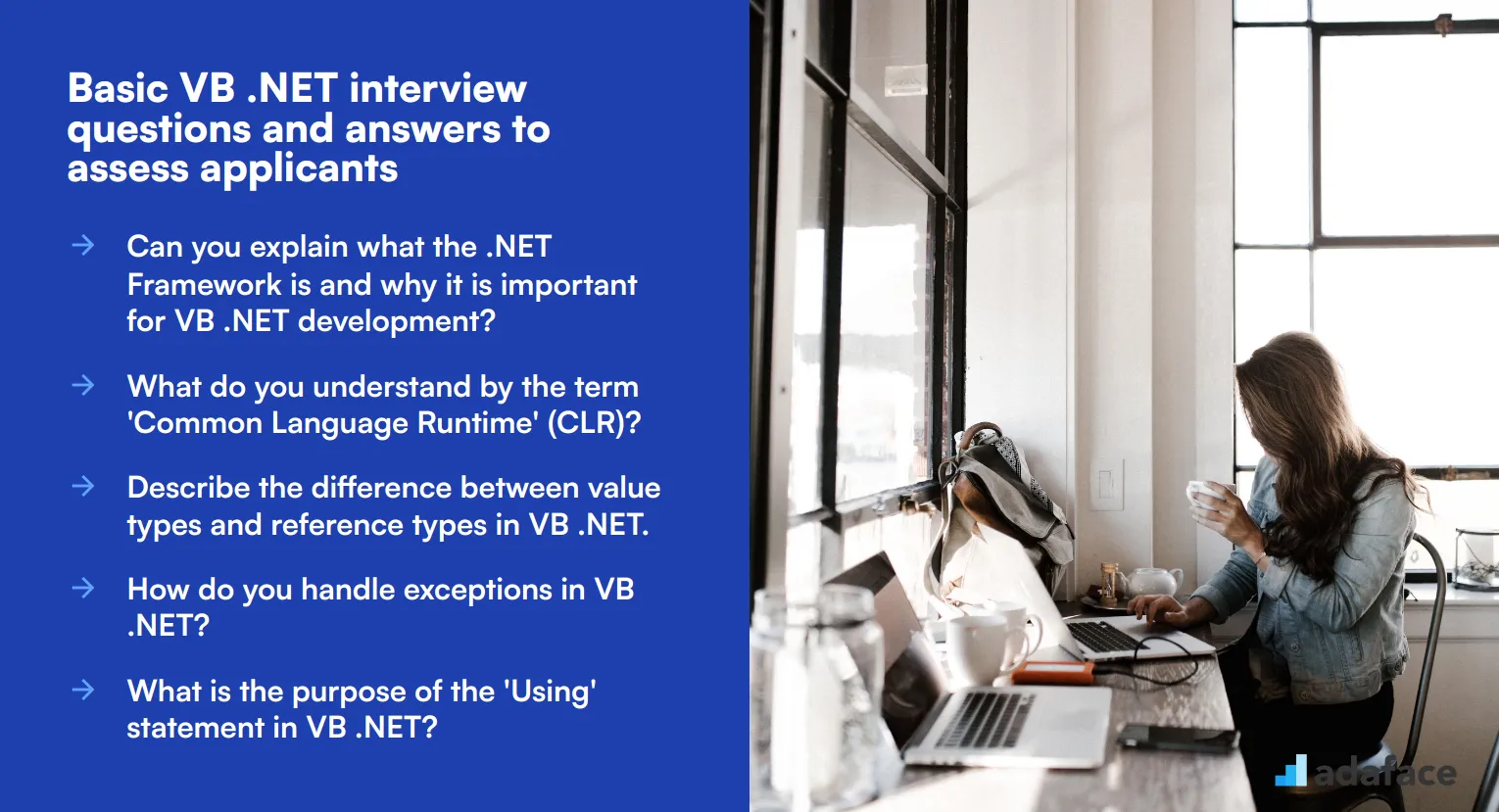
To determine whether your VB .NET applicants have the right foundational knowledge, use this list of 10 basic interview questions. They are perfect for assessing a candidate's understanding of fundamental concepts, ensuring you hire the right fit for your team.
1. Can you explain what the .NET Framework is and why it is important for VB .NET development?
The .NET Framework is a software development platform developed by Microsoft. It's crucial for VB .NET development because it provides a controlled environment for the execution of applications and includes a large library of pre-coded solutions to common programming tasks.
A strong candidate will highlight the significance of the .NET Framework in managing memory, improving security, and simplifying the development process. They might also mention how it supports various programming languages and promotes interoperability.
2. What do you understand by the term 'Common Language Runtime' (CLR)?
The Common Language Runtime (CLR) is the core runtime engine in the .NET Framework that manages the execution of .NET programs. It provides important services such as memory management, type safety, and exception handling.
An ideal response should reflect an understanding of how CLR improves performance and reliability in .NET applications. Look for explanations on its role in JIT compilation and garbage collection.
3. Describe the difference between value types and reference types in VB .NET.
In VB .NET, value types directly contain their data and are stored on the stack. Reference types, on the other hand, store references to their data, which is stored on the heap.
Candidates should articulate how value types are generally more efficient but limited in size, while reference types are more flexible but come with additional overhead. Look for examples to gauge their practical understanding.
4. How do you handle exceptions in VB .NET?
Exception handling in VB .NET is managed using Try, Catch, Finally, and Throw statements. The Try block contains code that might throw an exception, Catch handles the exception, Finally executes code regardless of whether an exception occurred, and Throw is used to manually throw an exception.
Strong candidates should emphasize the importance of proper exception handling to maintain application stability and user experience. They might also discuss best practices such as logging exceptions and providing user-friendly error messages.
5. What is the purpose of the 'Using' statement in VB .NET?
The 'Using' statement is used to ensure that IDisposable objects, such as file streams and database connections, are disposed of properly, releasing any unmanaged resources they hold.
Look for candidates who can explain how the 'Using' statement helps in managing resources efficiently and reducing the risk of memory leaks. They might also give examples of its use with specific classes.
6. Can you explain what a Delegate is and its use in VB .NET?
A Delegate in VB .NET is a type that represents references to methods with a specific parameter list and return type. Delegates are used to pass methods as arguments to other methods, which is essential for implementing event handling and callback methods.
Candidates should demonstrate an understanding of how delegates enable flexibility and decoupling in code. They might mention real-world scenarios such as using delegates for asynchronous programming or event-driven programming.
7. What is the difference between an Interface and an Abstract Class in VB .NET?
An Interface in VB .NET defines a contract that classes can implement. It only contains declarations of methods, properties, events, or indexers. An Abstract Class, on the other hand, can provide both complete and incomplete implementations, allowing some methods to be defined while others are left abstract.
A well-rounded answer would compare their use cases: interfaces for defining capabilities across different class hierarchies and abstract classes for shared base functionality. Candidates should discuss scenarios where one is preferred over the other.
8. How do you manage state in a VB .NET web application?
State management in VB .NET web applications can be handled using various techniques such as ViewState, Session State, Cookie State, and Application State. Each method has its pros and cons depending on the scope and lifetime of the data.
Candidates should show a clear understanding of when to use each method. For example, ViewState for retaining user input on a single page, and Session State for user-specific data across pages. Look for emphasis on security and performance considerations.
9. What is LINQ and how is it used in VB .NET?
LINQ (Language Integrated Query) is a feature in VB .NET that allows querying of data collections using a syntax reminiscent of SQL. It can be used to query arrays, collections, XML, and databases.
Ideal candidates should describe the benefits of LINQ, such as improved readability and maintainability of code. They might also discuss its advantages over traditional loops and how it integrates seamlessly with different types of data sources.
10. Can you explain what Garbage Collection is and why it is important in VB .NET?
Garbage Collection in VB .NET is a process by which the CLR automatically manages the allocation and release of memory. It identifies objects that are no longer in use and reclaims their memory.
Candidates should emphasize the importance of garbage collection in preventing memory leaks and enhancing application performance. Look for explanations of how the garbage collector works, such as generations and the managed heap.
10 VB .NET interview questions to ask junior developers
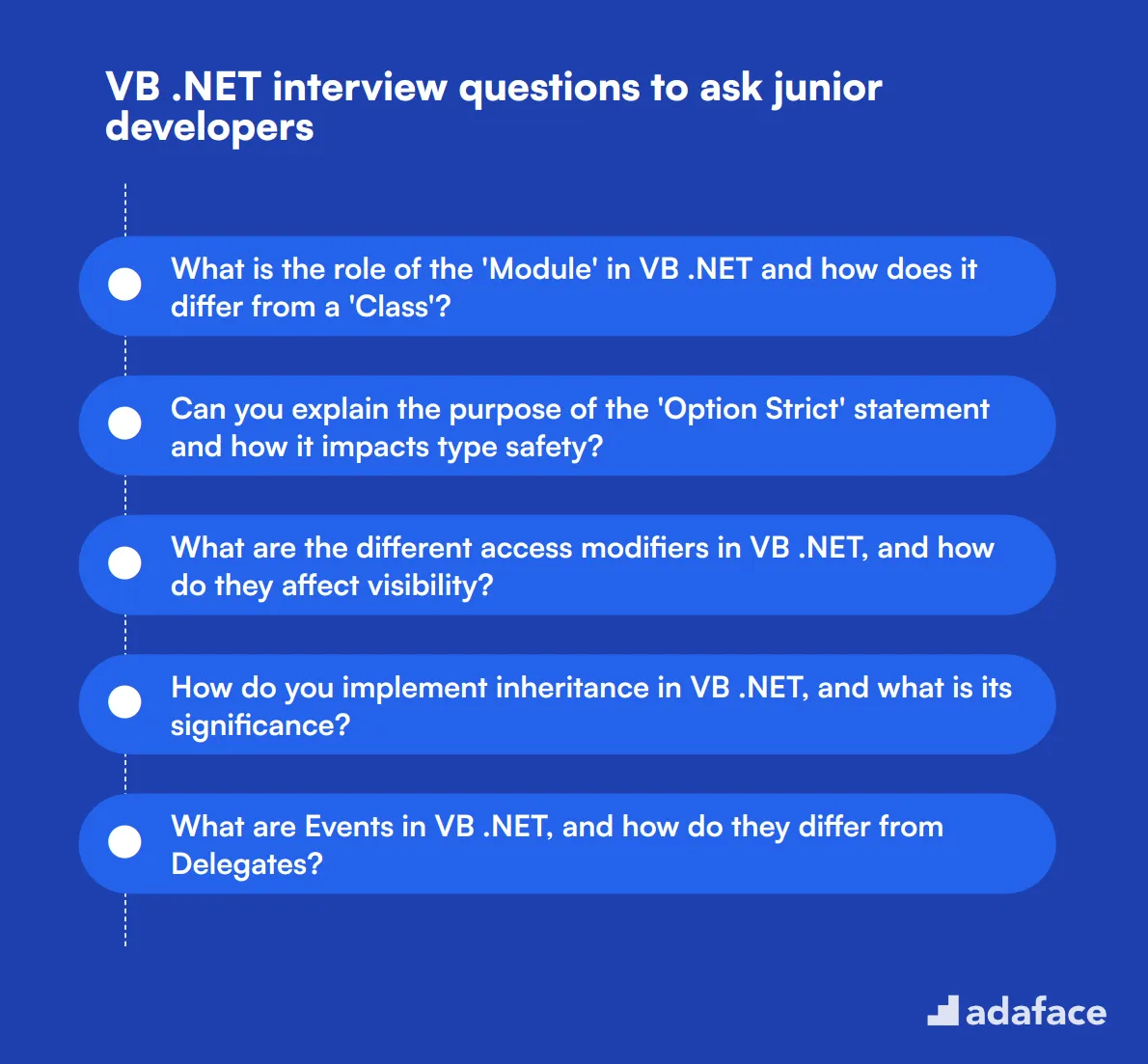
To gauge the foundational knowledge of junior VB .NET developers, utilize this list of tailored interview questions. These inquiries will help you assess their understanding of key concepts and their capability to apply them in real-world scenarios. For more insights into the role, consider reviewing our job description for a .NET developer.
- What is the role of the 'Module' in VB .NET and how does it differ from a 'Class'?
- Can you explain the purpose of the 'Option Strict' statement and how it impacts type safety?
- What are the different access modifiers in VB .NET, and how do they affect visibility?
- How do you implement inheritance in VB .NET, and what is its significance?
- What are Events in VB .NET, and how do they differ from Delegates?
- Can you explain the concept of Polymorphism in the context of VB .NET?
- What is the purpose of the 'Handles' keyword in VB .NET?
- Describe how to create a simple Windows Forms application in VB .NET.
- What is the significance of 'My' namespace in VB .NET?
- How can you perform file I/O operations in VB .NET?
10 intermediate VB .NET interview questions and answers to ask mid-tier developers
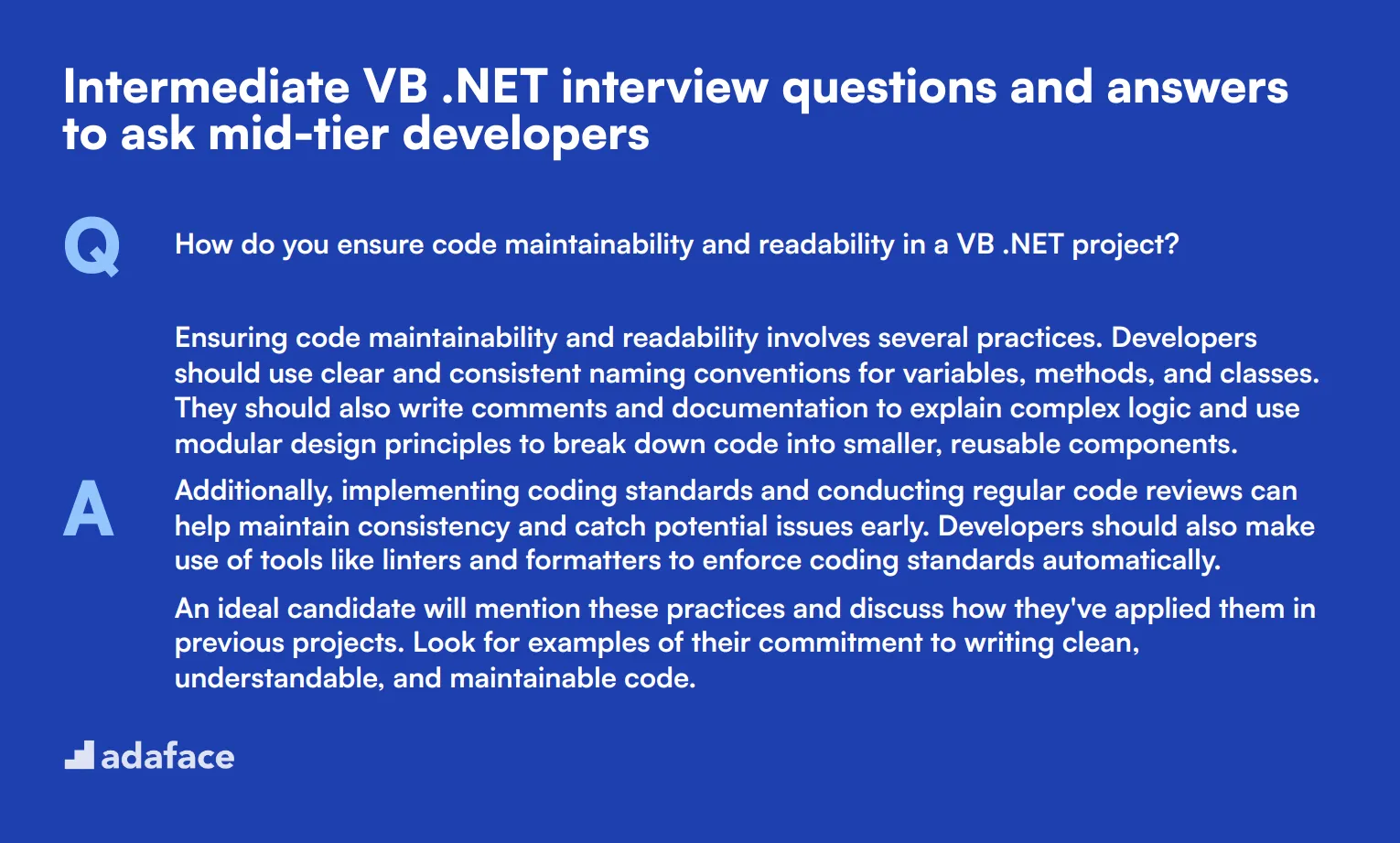
To assess whether your mid-tier VB .NET developers have the depth of knowledge and practical skills necessary for your projects, use these intermediate interview questions. These questions are designed to help you uncover their problem-solving abilities and familiarity with essential VB .NET concepts.
1. How do you ensure code maintainability and readability in a VB .NET project?
Ensuring code maintainability and readability involves several practices. Developers should use clear and consistent naming conventions for variables, methods, and classes. They should also write comments and documentation to explain complex logic and use modular design principles to break down code into smaller, reusable components.
Additionally, implementing coding standards and conducting regular code reviews can help maintain consistency and catch potential issues early. Developers should also make use of tools like linters and formatters to enforce coding standards automatically.
An ideal candidate will mention these practices and discuss how they've applied them in previous projects. Look for examples of their commitment to writing clean, understandable, and maintainable code.
2. What strategies do you use for debugging and troubleshooting in VB .NET?
Effective debugging and troubleshooting involve a combination of using the built-in debugging tools in Visual Studio and following a systematic approach. Developers should know how to set breakpoints, inspect variables, and step through code to identify where issues occur.
They should also be familiar with logging and exception handling to capture and analyze runtime errors. Additionally, writing unit tests can help identify issues early in the development process.
Look for candidates who can explain these strategies clearly and provide examples of how they've successfully debugged complex issues in the past.
3. How do you handle version control and collaboration in a VB .NET project?
Version control is essential for managing changes and collaboration in software projects. Developers typically use Git for version control, along with platforms like GitHub or GitLab. They should be familiar with branching strategies, pull requests, and code reviews to ensure smooth collaboration.
Effective communication with team members and maintaining clear commit messages are also crucial for tracking changes and understanding the history of the project.
Ideal candidates will demonstrate a solid understanding of version control best practices and share their experiences collaborating with teams using these tools.
4. Can you discuss the importance of unit testing in VB .NET and how you implement it?
Unit testing is crucial for ensuring code quality and reliability. It involves writing tests for individual units or components of the application to verify that they work as intended. In VB .NET, developers can use frameworks like MSTest or NUnit for writing unit tests.
Implementing unit tests helps catch bugs early, simplifies refactoring, and provides documentation for the expected behavior of the code. It also facilitates continuous integration and delivery by ensuring that changes don't introduce new issues.
Candidates should explain their approach to writing unit tests and provide examples of how unit testing has benefited their previous projects. Look for a strong understanding of the principles and benefits of unit testing.
5. How do you optimize the performance of a VB .NET application?
Optimizing performance involves several strategies, such as efficient use of data structures, minimizing resource usage, and avoiding unnecessary computations. Developers should also consider caching frequently accessed data and optimizing database queries.
Profiling tools can help identify performance bottlenecks, and code reviews can ensure that performance optimizations are implemented correctly. Additionally, following best practices for asynchronous programming can improve responsiveness and scalability.
Look for candidates who can discuss these strategies in detail and share examples of how they've optimized performance in their previous projects.
6. What approach do you take to ensure security in a VB .NET application?
Ensuring security involves following best practices such as input validation, secure authentication, and authorization mechanisms. Developers should use encryption for sensitive data and follow secure coding guidelines to prevent common vulnerabilities like SQL injection and cross-site scripting (XSS).
Regular security audits and code reviews can help identify and address potential security issues. Additionally, staying updated with the latest security patches and updates is crucial for maintaining a secure application.
Candidates should demonstrate a strong understanding of security principles and provide examples of how they've implemented security measures in their previous projects.
7. How do you approach refactoring existing code in a VB .NET project?
Refactoring involves improving the structure and readability of existing code without changing its functionality. Developers should start by identifying areas of code that are hard to understand or maintain. They can then break down large methods into smaller, reusable functions and eliminate duplicate code.
Automated tests can ensure that refactoring doesn't introduce new issues. It's also important to refactor incrementally and commit changes frequently to track progress and revert if necessary.
Ideal candidates will discuss their strategies for refactoring and share examples of successful refactoring efforts from their previous projects.
8. Can you explain the concept of asynchronous programming and its benefits in VB .NET?
Asynchronous programming allows developers to perform tasks without blocking the main thread, improving the responsiveness of applications. In VB .NET, asynchronous programming is achieved using the Async
and Await
keywords.
The main benefit of asynchronous programming is that it keeps the user interface responsive while performing time-consuming operations, such as file I/O or network requests. It also improves scalability by allowing multiple operations to run concurrently.
Look for candidates who can explain these concepts clearly and provide examples of how they've used asynchronous programming to enhance the performance and responsiveness of their applications.
9. What is your experience with deploying VB .NET applications, and what challenges have you faced?
Deploying VB .NET applications involves several steps, including packaging the application, configuring the environment, and ensuring that dependencies are correctly managed. Developers should be familiar with deployment tools and platforms, such as IIS for web applications or ClickOnce for desktop applications.
Challenges may include managing configuration settings, handling environment-specific issues, and ensuring that the deployment process is automated and repeatable. Developers should also be prepared to troubleshoot deployment issues and roll back changes if necessary.
Candidates should discuss their experience with deployment and share examples of how they've overcome challenges in previous projects. Look for a solid understanding of the deployment process and best practices.
10. How do you stay updated with the latest developments in VB .NET and software development in general?
Staying updated involves following reputable sources such as official documentation, blogs, and forums. Developers should also participate in online communities and attend conferences or webinars to learn about new features and best practices.
Continuous learning through courses and certifications can help developers stay current with the latest trends and technologies. Experimenting with new tools and techniques in side projects can also be beneficial.
Candidates should demonstrate a commitment to continuous learning and share their strategies for staying updated. Look for evidence of their proactive approach to professional development and keeping their skills relevant.
12 VB .NET interview questions about debugging techniques and code optimization
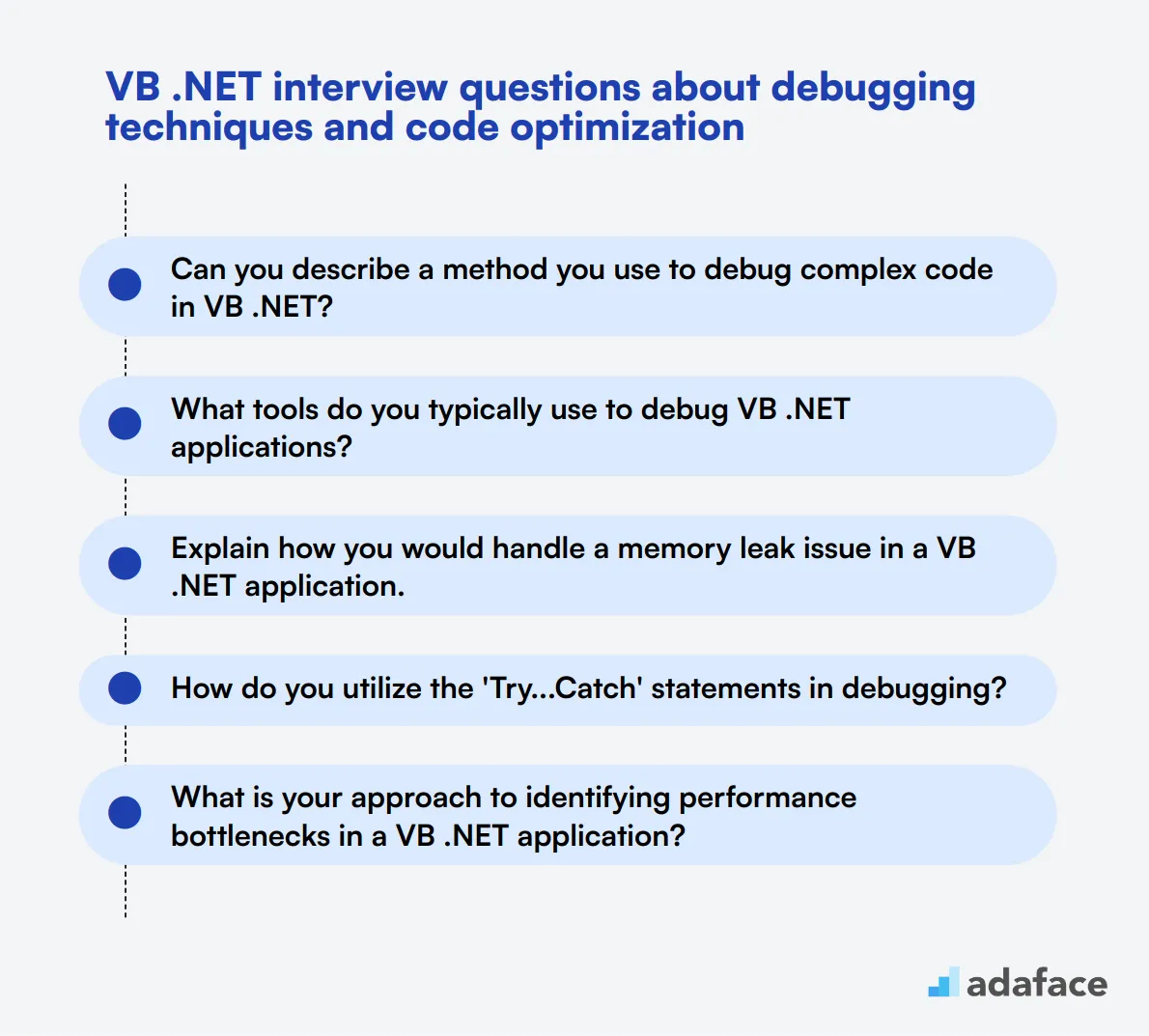
To ensure candidates have the necessary skills for debugging and optimizing VB .NET code, refer to some of these 12 VB .NET interview questions about debugging techniques and code optimization. This focused set of questions will help you identify developers who can efficiently troubleshoot and enhance performance, an essential trait for roles detailed in our software developer job description.
- Can you describe a method you use to debug complex code in VB .NET?
- What tools do you typically use to debug VB .NET applications?
- Explain how you would handle a memory leak issue in a VB .NET application.
- How do you utilize the 'Try...Catch' statements in debugging?
- What is your approach to identifying performance bottlenecks in a VB .NET application?
- How do you optimize database queries within a VB .NET application?
- Describe a time when you used profiling tools to optimize your code.
- What strategies do you use to reduce the load time of a VB .NET application?
- How do you ensure your code is thread-safe?
- Can you explain how to use conditional breakpoints in VB .NET?
- How do you handle and log exceptions for better debugging?
- Describe your approach to optimizing the rendering performance of a Windows Forms application.
8 VB .NET interview questions and answers related to error handling and exception management
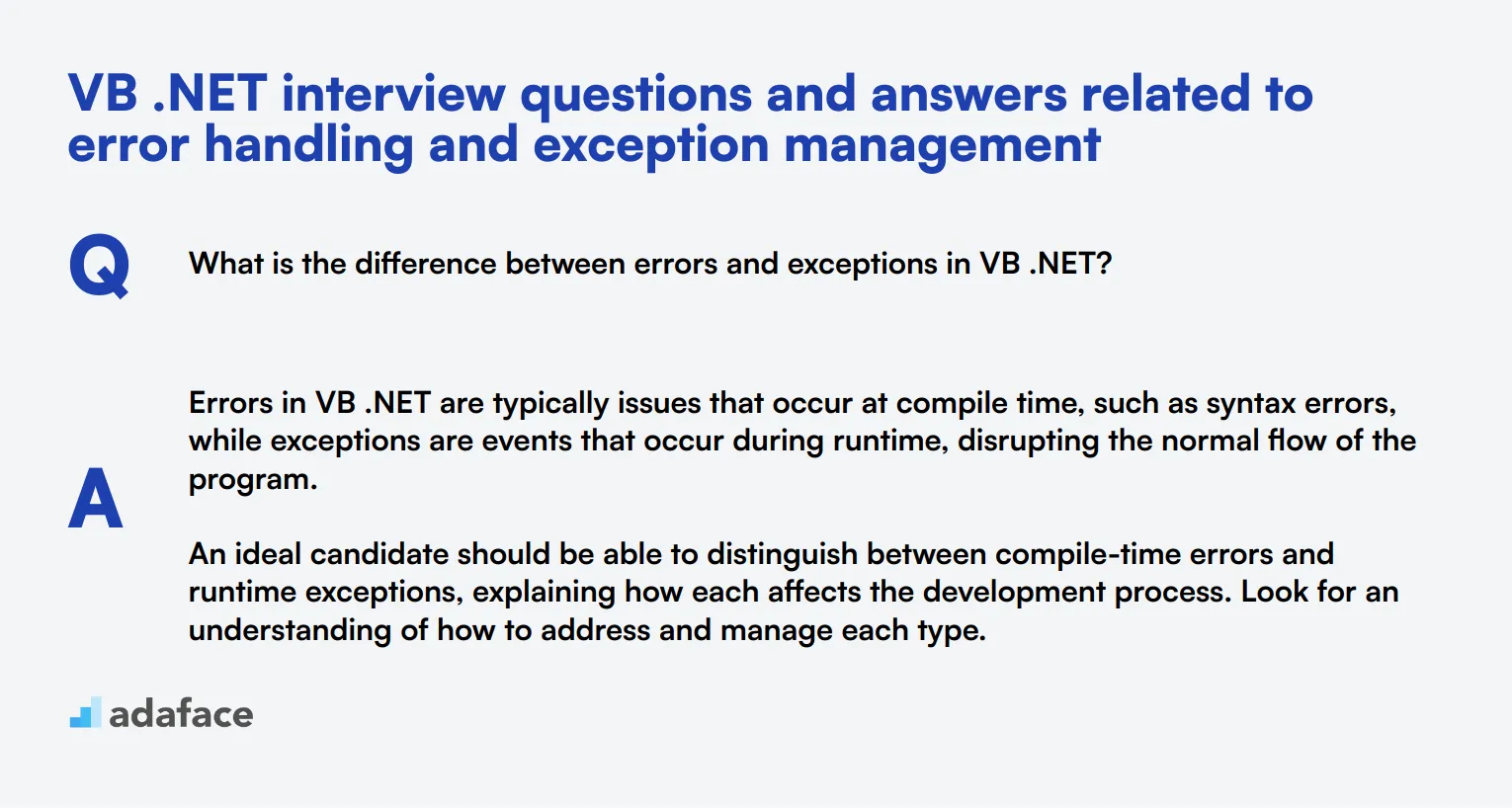
To evaluate candidates on their ability to manage and debug runtime errors effectively, use these VB .NET interview questions focused on error handling and exception management. These queries will help you gauge their problem-solving skills and ensure they can maintain smooth application performance.
1. What is the difference between errors and exceptions in VB .NET?
Errors in VB .NET are typically issues that occur at compile time, such as syntax errors, while exceptions are events that occur during runtime, disrupting the normal flow of the program.
An ideal candidate should be able to distinguish between compile-time errors and runtime exceptions, explaining how each affects the development process. Look for an understanding of how to address and manage each type.
2. How do you decide when to use 'Try...Catch' blocks in your code?
'Try...Catch' blocks are used to handle exceptions that may occur during the execution of a program. A candidate might explain that they use these blocks to catch exceptions that they anticipate could happen, such as file I/O errors or network issues, to ensure the application remains robust and user-friendly.
Look for responses that demonstrate a balanced approach to exception handling, where 'Try...Catch' blocks are neither overused nor underutilized. The candidate should understand the importance of handling exceptions gracefully to maintain application stability.
3. What is a finally block and when would you use it?
A 'finally' block in VB .NET is used to execute code after a 'Try...Catch' block, regardless of whether an exception was thrown or not. It's typically used for cleanup activities, such as closing files, releasing resources, or resetting variables.
A strong candidate will highlight the importance of ensuring resources are released properly and might give examples of scenarios where a 'finally' block is essential. Look for an understanding of how this contributes to resource management and application stability.
4. Can you explain the concept of custom exceptions and why you might create one?
Custom exceptions are user-defined exceptions that inherit from the base Exception class. They are used to create specific error-handling scenarios tailored to the application's needs.
Candidates should explain the benefits of custom exceptions, such as providing more detailed error information and improving the readability and maintainability of the code. Look for examples where custom exceptions helped in solving complex error-handling requirements.
5. What are the best practices for logging exceptions in VB .NET?
Best practices for logging exceptions include capturing detailed information about the exception, such as the message, stack trace, and any relevant context data. It's also important to use a consistent logging framework and ensure that logs are stored securely and are easily accessible for debugging.
The candidate should discuss the importance of logging for diagnosing issues and improving application reliability. They may mention tools or frameworks they have used for logging. Look for an understanding of how effective logging supports ongoing maintenance and troubleshooting.
6. How do you handle unhandled exceptions in a VB .NET application?
Unhandled exceptions can be managed using global exception handlers, such as the Application.ThreadException event in Windows Forms or the AppDomain.UnhandledException event. These handlers catch exceptions that weren't caught by specific 'Try...Catch' blocks.
A good response will include the importance of logging these exceptions and providing user-friendly error messages to maintain a positive user experience. Look for an understanding of how to prevent unhandled exceptions from causing application crashes.
7. What is your approach to debugging a VB .NET application that frequently throws exceptions?
Debugging frequent exceptions involves identifying the root cause by examining the exception details, using debugging tools, and analyzing the code flow. The process may include setting breakpoints, reviewing logs, and using a step-by-step approach to isolate the issue.
Candidates should demonstrate a systematic approach to debugging and mention specific tools or techniques they use. Look for a problem-solving mindset and the ability to remain patient and thorough during the debugging process.
8. How do you ensure that exceptions do not leak sensitive information in your application?
To prevent exceptions from leaking sensitive information, it's important to sanitize error messages, manage log access carefully, and avoid displaying raw exception details to end users. Instead, user-friendly and generic error messages should be shown while detailed logs are kept secure.
An ideal candidate will emphasize the significance of security best practices in error handling. Look for awareness of how to balance transparency for debugging with the need to protect sensitive information.
7 situational VB .NET interview questions with answers for hiring top developers
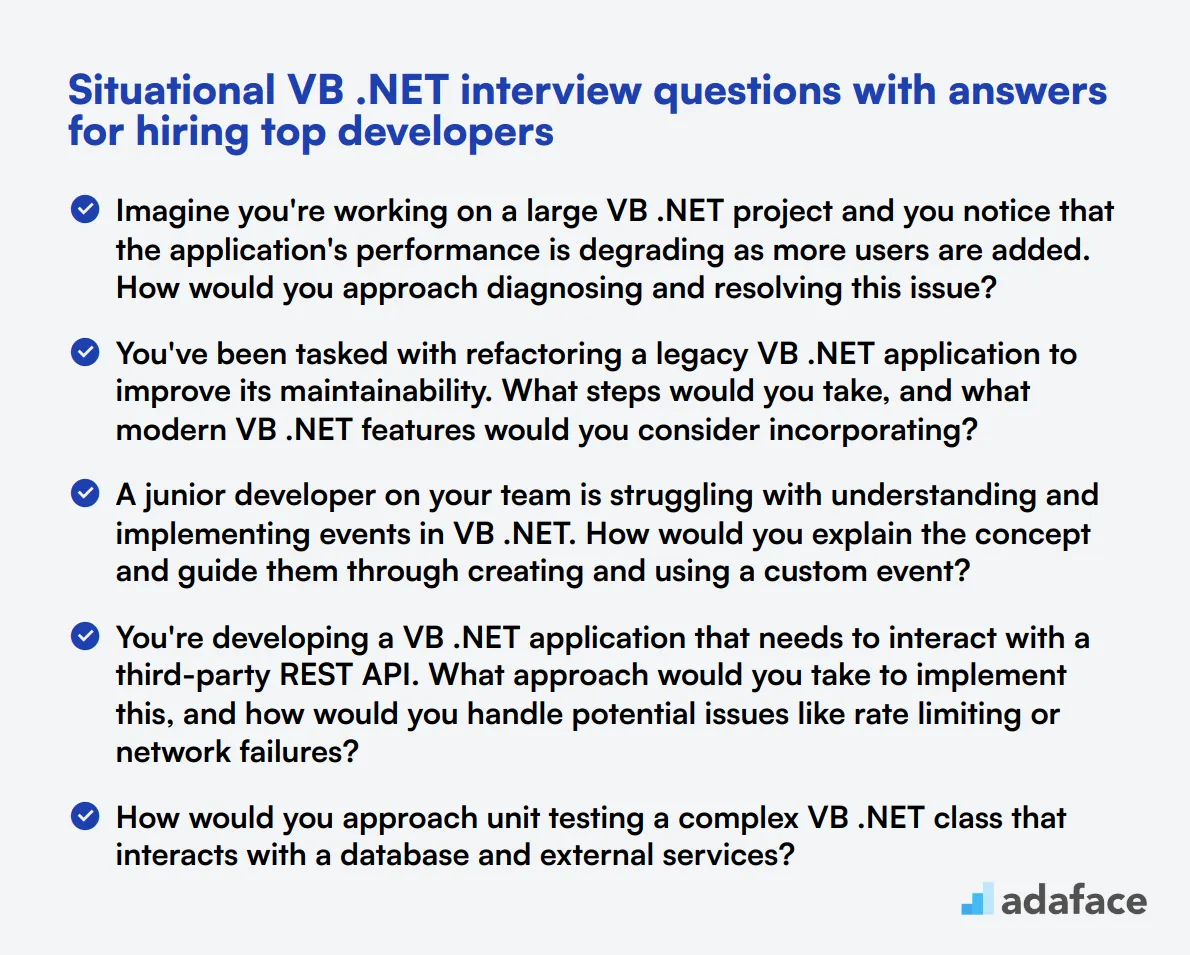
Ready to uncover the true potential of your VB .NET candidates? These situational interview questions are designed to help you dig deeper into a candidate's problem-solving skills and practical experience. Use them to assess how well applicants can apply their knowledge to real-world scenarios, and gain valuable insights into their thought process and decision-making abilities.
1. Imagine you're working on a large VB .NET project and you notice that the application's performance is degrading as more users are added. How would you approach diagnosing and resolving this issue?
A strong candidate should outline a systematic approach to performance optimization. They might mention the following steps:
- Profiling the application to identify bottlenecks
- Analyzing database queries and optimizing them if necessary
- Reviewing and optimizing any loops or resource-intensive operations
- Considering caching strategies to reduce database load
- Investigating potential memory leaks
- Exploring options for scaling the application horizontally or vertically
Look for candidates who demonstrate a methodical approach and mention specific tools or techniques they've used in similar situations. Follow up by asking about a specific performance issue they've resolved in the past and the impact of their solution.
2. You've been tasked with refactoring a legacy VB .NET application to improve its maintainability. What steps would you take, and what modern VB .NET features would you consider incorporating?
An experienced developer should discuss a structured approach to refactoring, potentially including:
- Analyzing the current codebase and identifying areas for improvement
- Breaking down large, monolithic functions into smaller, more manageable ones
- Implementing proper error handling and logging
- Utilizing object-oriented principles to improve code organization
- Considering the use of newer VB .NET features like LINQ, async/await for better performance, and generic collections for type safety
- Implementing unit tests to ensure the refactored code maintains the original functionality
Pay attention to candidates who emphasize the importance of maintaining the application's functionality while improving its structure. Ask them about their experience with specific refactoring techniques or tools they've found helpful in similar projects.
3. A junior developer on your team is struggling with understanding and implementing events in VB .NET. How would you explain the concept and guide them through creating and using a custom event?
A good candidate should be able to break down the concept of events into simple terms and provide a step-by-step explanation. They might explain events as:
- A way for objects to notify other objects when something of interest occurs
- Similar to a notification system where objects can subscribe to be notified when certain actions happen
- Implemented using delegates, which are like type-safe function pointers
Look for candidates who can provide a clear, concise explanation and demonstrate patience in teaching complex concepts. They should also be able to outline the basic steps for creating and using a custom event, such as declaring the event, raising the event, and subscribing to the event. Consider asking how they would handle common pitfalls or misconceptions about events to gauge their depth of understanding.
4. You're developing a VB .NET application that needs to interact with a third-party REST API. What approach would you take to implement this, and how would you handle potential issues like rate limiting or network failures?
A competent candidate should discuss a structured approach to API integration, possibly including:
- Using HttpClient for making HTTP requests
- Implementing proper error handling and logging
- Utilizing async/await for non-blocking API calls
- Serializing and deserializing JSON responses
- Implementing retry logic for handling temporary network issues
- Considering the use of a circuit breaker pattern for handling persistent failures
Pay attention to candidates who mention best practices like API rate limiting adherence, caching responses to reduce API calls, and proper exception handling. Ask them about their experience with specific libraries or frameworks they've used for API integration in VB .NET projects.
5. How would you approach unit testing a complex VB .NET class that interacts with a database and external services?
A thorough answer should cover several key aspects of unit testing complex classes:
- Using dependency injection to make the class testable
- Mocking external dependencies like database connections and service calls
- Breaking down complex methods into smaller, more testable units
- Using a testing framework like NUnit or MSTest
- Writing tests for different scenarios, including edge cases and error conditions
- Considering the use of test data builders or object mothers for creating test data
Look for candidates who emphasize the importance of isolating the unit under test and maintaining test independence. Ask about their experience with specific mocking frameworks or how they've handled particularly challenging testing scenarios in the past.
6. You've been asked to implement a new feature in a VB .NET application that requires storing sensitive user data. How would you ensure the security of this data both at rest and in transit?
A security-conscious candidate should discuss multiple layers of data protection:
- Using HTTPS for all network communications
- Encrypting sensitive data before storing it in the database
- Using secure hashing algorithms for passwords
- Implementing proper authentication and authorization mechanisms
- Utilizing parameterized queries to prevent SQL injection attacks
- Considering the use of key management services for handling encryption keys
Evaluate the candidate's understanding of security best practices and their ability to apply them in a VB .NET context. Ask about their experience with specific encryption libraries or security frameworks they've used in previous projects.
7. Describe a situation where you had to optimize the memory usage of a VB .NET application. What techniques did you use, and what was the outcome?
A strong answer should demonstrate practical experience with memory optimization techniques such as:
- Proper disposal of unmanaged resources using the IDisposable interface
- Avoiding memory leaks by unsubscribing from events
- Using weak references for caching scenarios
- Optimizing LINQ queries to reduce memory overhead
- Implementing lazy loading for resource-intensive objects
- Utilizing memory-efficient data structures when appropriate
Look for candidates who can provide specific examples from their past experience, including the tools they used to diagnose memory issues (like memory profilers) and the measurable impact of their optimizations. Ask them how they would approach identifying memory leaks in a large VB .NET application.
Which VB .NET skills should you evaluate during the interview phase?
While a single interview cannot encapsulate the entire depth of a candidate’s expertise, it is crucial to focus on the core VB .NET skills that will determine their potential and capability in real-world scenarios. This ensures that time is spent evaluating the skills that are most indicative of success in the role.
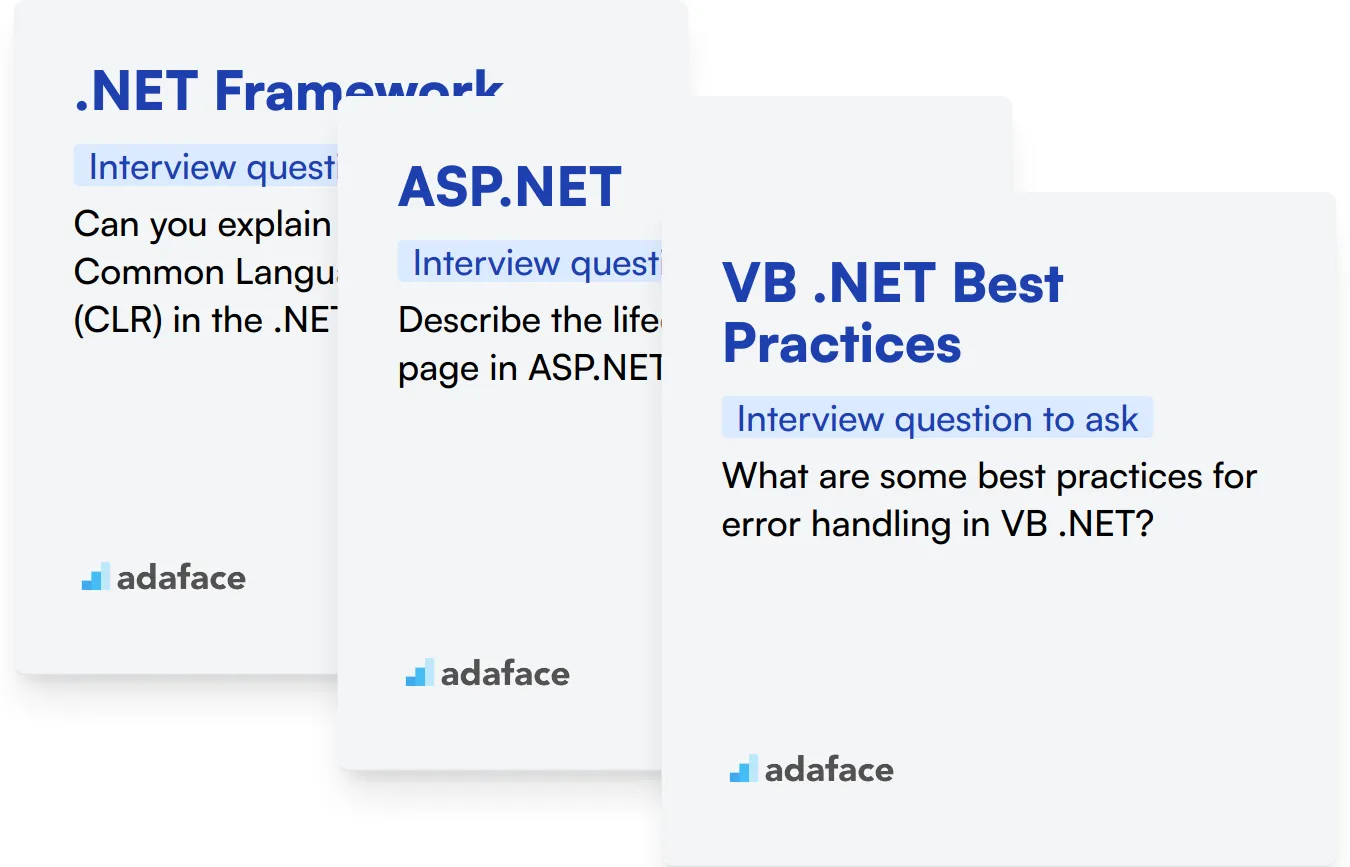
.NET Framework
Understanding the .NET Framework is essential for VB .NET developers as it provides the environment for executing .NET applications. A strong grasp of .NET Framework indicates a developer's capability to use VB .NET effectively within the platform's ecosystem.
To assess this skill, consider utilizing a structured assessment that includes relevant MCQs. Adaface offers a .NET Framework test that can help filter candidates proficient in core .NET concepts.
Additionally, you might consider asking targeted interview questions to gauge their depth of understanding in .NET Framework.
Can you explain the role of Common Language Runtime (CLR) in the .NET Framework?
Listen for details on how CLR manages memory, executes code, and handles security. A thorough explanation will demonstrate the candidate’s comprehensive understanding of the .NET Framework’s operational aspects.
ASP.NET
ASP.NET is crucial for building robust web applications using VB .NET. Knowledge of ASP.NET indicates the candidate's ability to effectively leverage the web application framework to develop, deploy, and manage apps.
Using an MCQ-based assessment, such as Adaface's ASP.NET test, can help verify the candidate’s proficiency in ASP.NET.
To further explore their expertise in ASP.NET, consider asking them the following practical question during the interview.
Describe the lifecycle of a web page in ASP.NET.
This question evaluates the candidate's understanding of critical ASP.NET processes like initialization, load, validation, and rendering. Effective answers should clearly articulate these steps.
VB .NET Best Practices
Adherence to VB .NET best practices is vital for writing clean, maintainable, and efficient code. It’s a demonstration of a candidate’s professional maturity and dedication to quality.
To test their familiarity with best practices in VB .NET, you could ask:
What are some best practices for error handling in VB .NET?
Look for responses that include using Try, Catch, Finally blocks, and handling specific exceptions. This shows their approach to robust and reliable coding.
3 Tips for Using VB .NET Interview Questions
Before you start putting what you've learned into practice, here are three tips to help you use VB .NET interview questions effectively.
1. Utilize Skill Tests Before Interviews
Using skill tests before interviews is a great way to filter out candidates who may not meet the basic requirements for the position. This saves time and ensures that only the most qualified candidates make it to the interview stage.
Consider using tests that evaluate different aspects of VB .NET and .NET framework skills. For instance, our VB .NET online test is designed to assess foundational knowledge, while the C# .NET test can be used to measure proficiency in C# programming.
By incorporating these skill tests, you can streamline the hiring process, making it easier to identify and focus on candidates who are truly prepared for the role.
2. Curate Relevant Interview Questions for Maximum Effectiveness
Given the limited time available for interviews, it's important to select the right amount and type of questions. Focusing on key areas will maximize the success of evaluating candidates effectively.
In addition to VB .NET questions, consider incorporating related technical questions such as those from our C# interview questions or ASP.NET MVC interview questions. Including questions about soft skills like communication or culture fit can also be valuable.
3. Always Ask Follow-Up Questions
Simply asking interview questions may not be enough. Candidates can sometimes provide rehearsed answers, so follow-up questions help you gauge their true understanding and depth of knowledge.
For example, if you ask a candidate how they handle exceptions in VB .NET, a good follow-up question could be: 'Can you describe a specific scenario where you implemented exception handling and what the outcome was?' This helps you understand their practical experience and problem-solving skills.
Use VB .NET interview questions and skills tests to hire talented developers
If you are looking to hire someone with VB .NET skills, you need to ensure they have those skills accurately. The best way to do this would be to use skill tests like the VB .NET Online Test or the ASP.NET MVC Online Test.
Once you use these tests, you can shortlist the best applicants and call them for interviews. To get started, you can sign up or visit our test library for more options.
VB.NET Online Test
Download VB .NET interview questions template in multiple formats
VB .NET Interview Questions FAQs
The questions cover basic, junior, intermediate, and advanced levels, suitable for assessing various developer skill ranges.
Use them alongside skills tests, tailor questions to the role, and focus on the candidate's problem-solving approach and coding practices.
Yes, the post includes 7 situational VB .NET interview questions to assess how candidates apply their knowledge in real-world scenarios.
Absolutely. There are dedicated sections for debugging techniques, code optimization, and error handling in VB .NET.
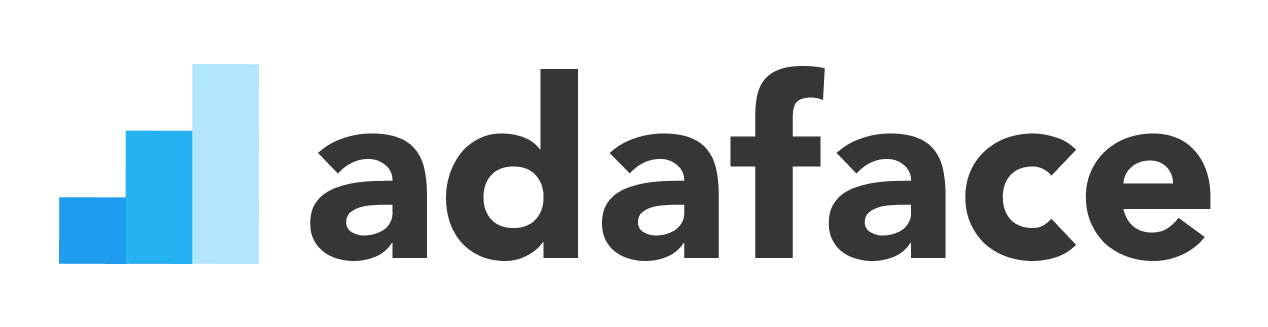
40 min skill tests.
No trick questions.
Accurate shortlisting.
We make it easy for you to find the best candidates in your pipeline with a 40 min skills test.
Try for freeRelated posts
Free resources
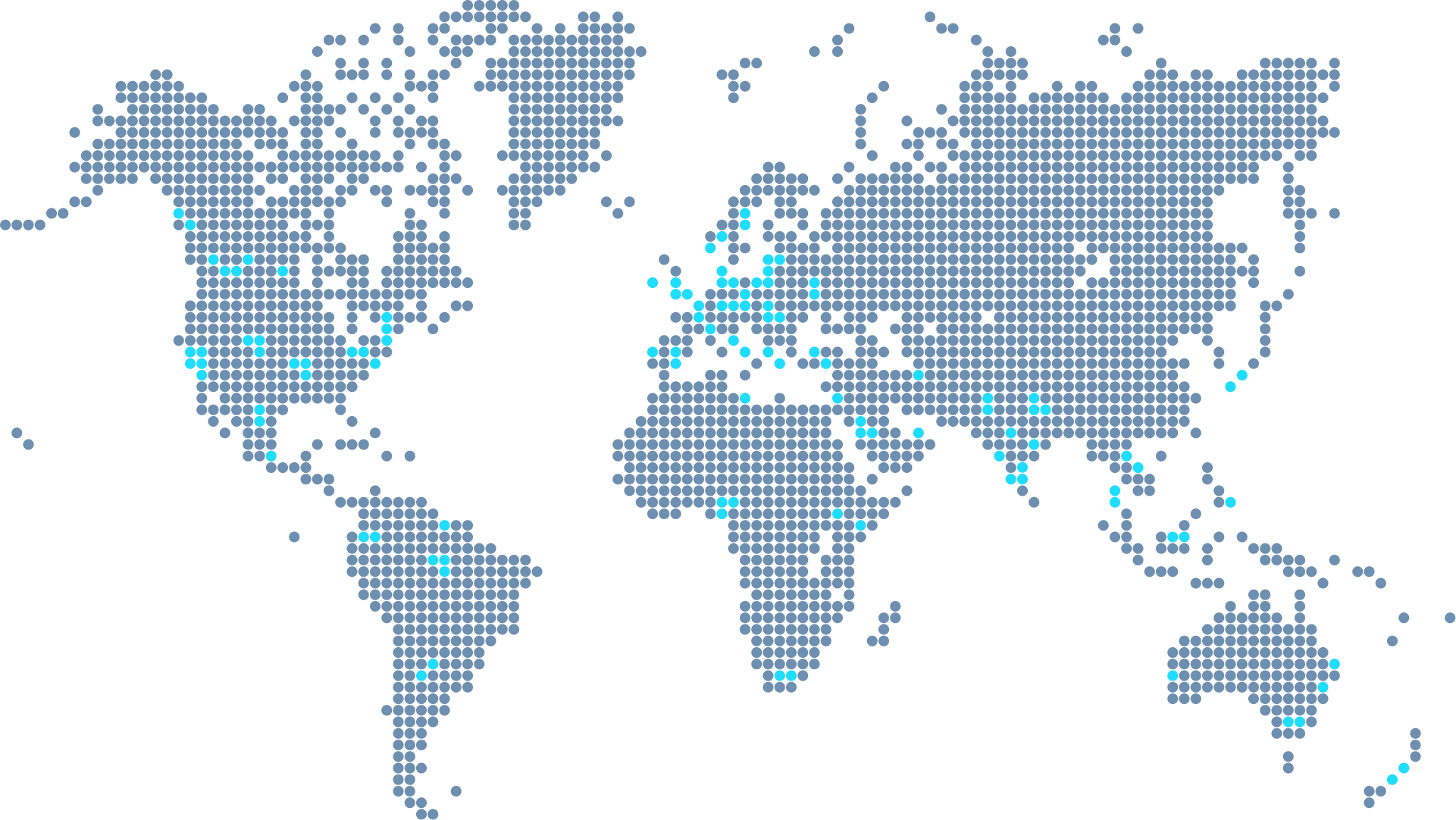
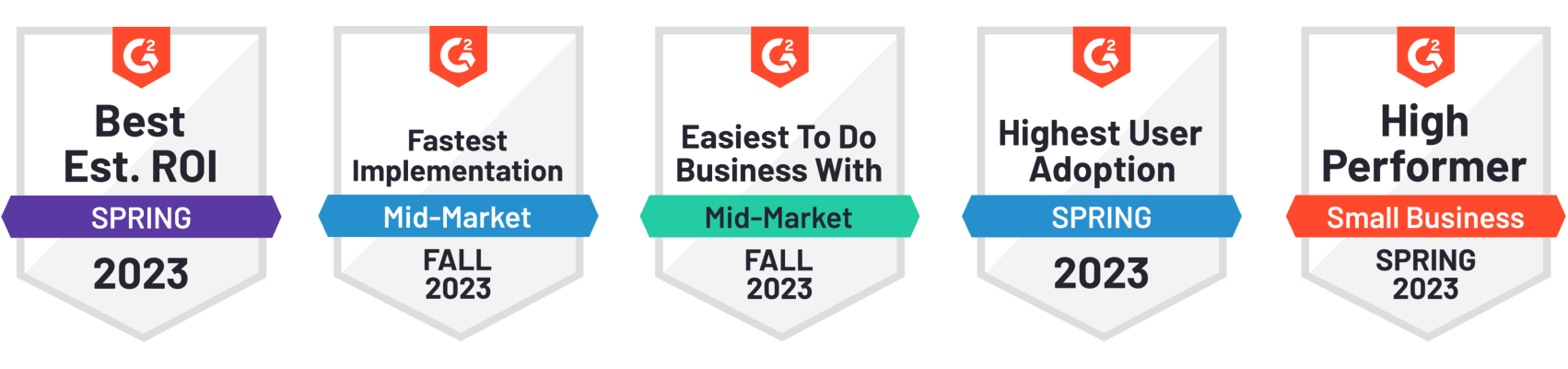