In a dynamic field like web development, finding the right Django developer can be challenging. Knowing the right Django interview questions to ask is crucial to identifying your ideal candidate.
In this blog post, we’ve compiled comprehensive lists of Django interview questions for different levels of expertise. From common questions to framework-specific ones, we cover what you need to evaluate junior to top-tier developers.
Using these questions will help you critically assess the capabilities of your candidates. For a streamlined approach, consider utilizing a Django online test before conducting interviews.
Table of contents
10 common Django interview questions to ask your candidates
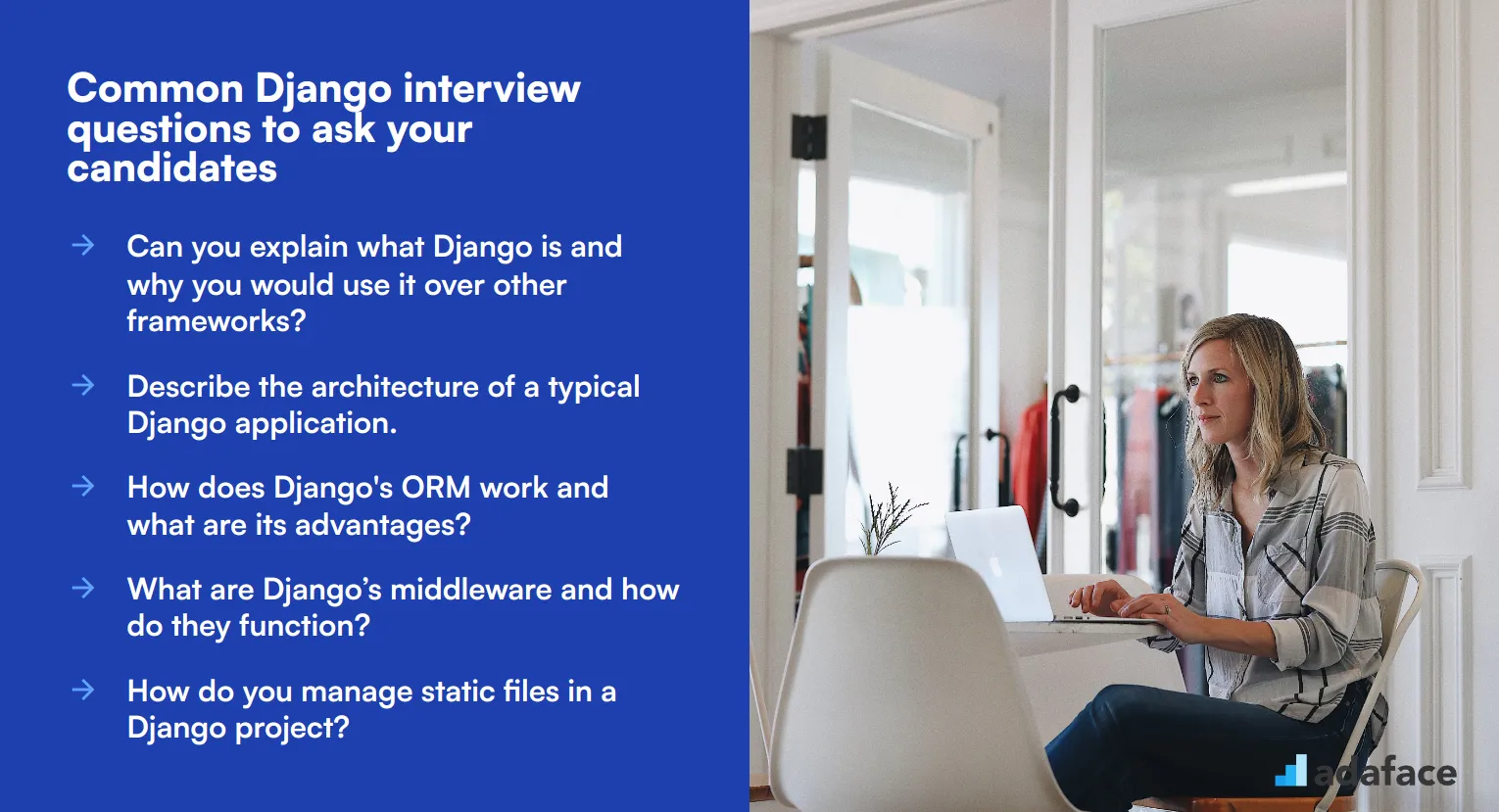
To determine whether your applicants have the right skills to excel as Django developers, use these common interview questions. They will help you gauge their technical understanding and problem-solving abilities. For a detailed job description, refer to this Django developer job description.
- Can you explain what Django is and why you would use it over other frameworks?
- Describe the architecture of a typical Django application.
- How does Django's ORM work and what are its advantages?
- What are Django’s middleware and how do they function?
- How do you manage static files in a Django project?
- Can you explain the purpose and use of Django’s settings.py file?
- What are Django signals and how do you use them?
- How would you implement user authentication in Django?
- Describe how you would optimize a Django application for better performance.
- What steps would you take to secure a Django application?
7 Django interview questions and answers to evaluate junior developers
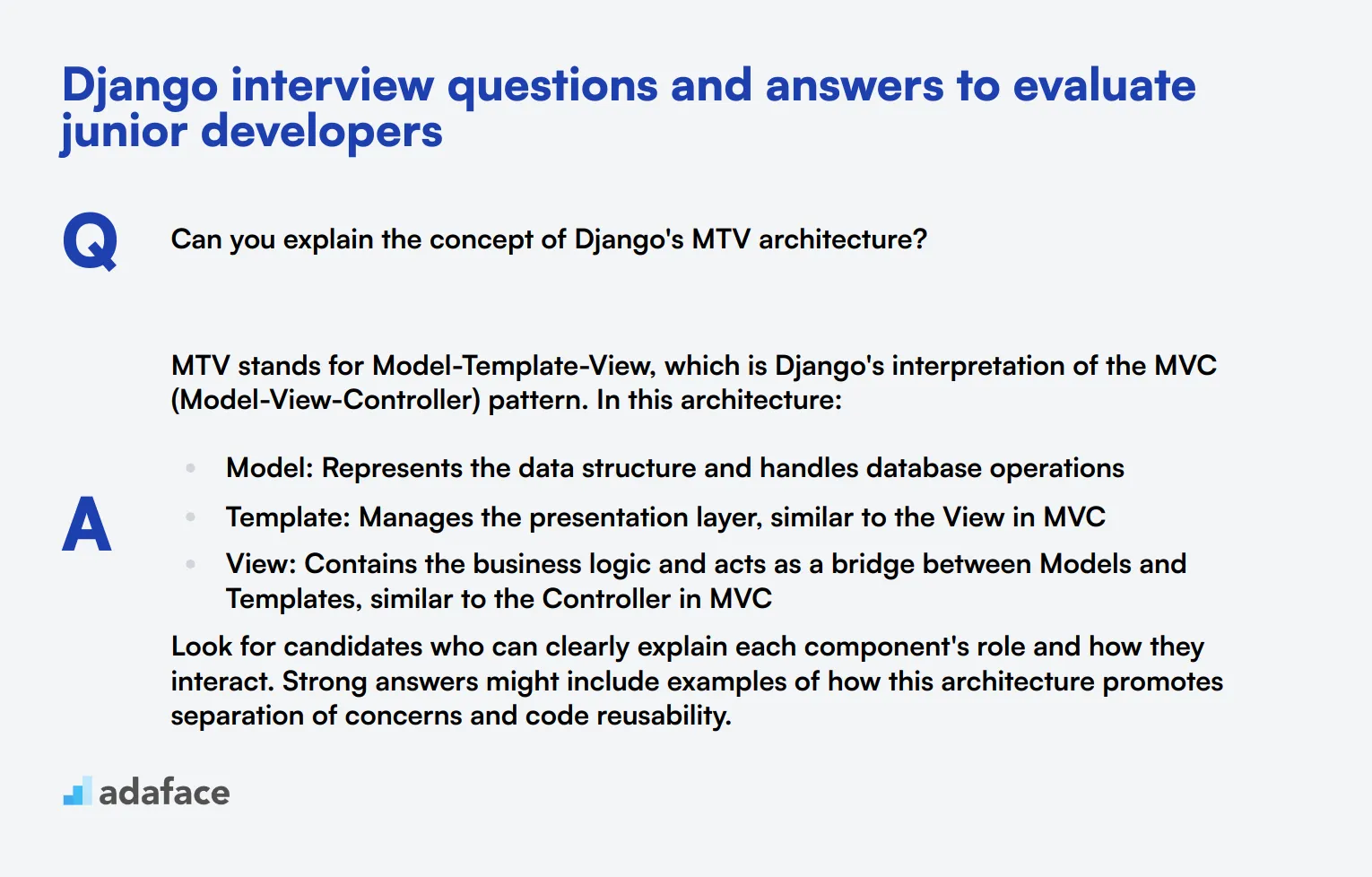
Ready to evaluate junior Django developers? These seven questions will help you assess their foundational knowledge and problem-solving skills. Use them to spark discussions about real-world scenarios and gauge how candidates approach Django development. Remember, the goal isn't just to test technical knowledge, but to understand how they think and apply their skills in practical situations.
1. Can you explain the concept of Django's MTV architecture?
MTV stands for Model-Template-View, which is Django's interpretation of the MVC (Model-View-Controller) pattern. In this architecture:
- Model: Represents the data structure and handles database operations
- Template: Manages the presentation layer, similar to the View in MVC
- View: Contains the business logic and acts as a bridge between Models and Templates, similar to the Controller in MVC
Look for candidates who can clearly explain each component's role and how they interact. Strong answers might include examples of how this architecture promotes separation of concerns and code reusability.
2. How would you handle form validation in Django?
Django provides built-in form validation through its Forms API. Candidates should mention:
- Using Django's Form class to define form fields and validation rules
- Implementing clean() methods for custom validation logic
- Utilizing built-in validators or creating custom validators
- Handling form errors in views and templates
Pay attention to candidates who discuss best practices like server-side validation, handling different types of form inputs, and providing user-friendly error messages. Follow up with questions about specific validation scenarios they've encountered in past projects.
3. What are Django migrations and why are they important?
Django migrations are a way to propagate changes made to models (adding a field, deleting a model, etc.) into the database schema. They're important because:
- They allow for version control of database schema
- They provide a way to share database changes with other developers
- They make it easier to reproduce the database state on different environments
Look for candidates who understand the migration workflow (makemigrations, migrate commands) and can explain scenarios where migrations are crucial, such as collaborative development or deploying changes to production databases.
4. How would you optimize database queries in a Django application?
Optimizing database queries is crucial for Django developer performance. Candidates should mention techniques like:
- Using select_related() and prefetch_related() to reduce the number of database queries
- Implementing database indexing on frequently queried fields
- Utilizing QuerySet methods like values() or values_list() when full model objects aren't needed
- Employing caching strategies to store frequently accessed data
Strong candidates might also discuss the importance of monitoring query performance using Django Debug Toolbar or similar tools, and knowing when to use raw SQL for complex queries that ORM can't handle efficiently.
5. Explain the purpose of Django's middleware and give an example of when you might create a custom middleware.
Django middleware is a framework of hooks into Django's request/response processing. Its main purposes are:
- To modify the request before it reaches the view
- To modify the response before it's sent to the client
- To perform actions that are common to all views (like authentication or logging)
For custom middleware, look for examples like implementing a site-wide cache, adding custom headers to all responses, or logging user activities. Assess the candidate's understanding of middleware's role in the request-response cycle and their ability to identify scenarios where custom middleware provides an elegant solution.
6. How do you ensure security in a Django application?
Security is a critical aspect of web development. Candidates should mention Django's built-in security features and additional measures:
- Using Django's authentication system and permission checks
- Implementing CSRF protection
- Enabling HTTPS
- Properly handling user input and using parameterized queries to prevent SQL injection
- Keeping Django and all dependencies up to date
- Utilizing Django's security middleware
Strong candidates might also discuss security best practices like regular security audits, implementing content security policies, or using tools like Django Security Check. Look for awareness of common web vulnerabilities and proactive approaches to security.
7. Describe a challenging problem you've faced in a Django project and how you solved it.
This open-ended question allows candidates to showcase their problem-solving skills and Django expertise. Look for responses that demonstrate:
- Clear problem identification
- Logical approach to troubleshooting
- Creative use of Django features or third-party libraries
- Consideration of performance, scalability, and maintainability in the solution
Pay attention to how candidates communicate technical concepts and their thought process. Strong answers will not only describe the solution but also explain why it was chosen over alternatives and what was learned from the experience.
15 intermediate Django interview questions and answers to ask mid-tier developers.
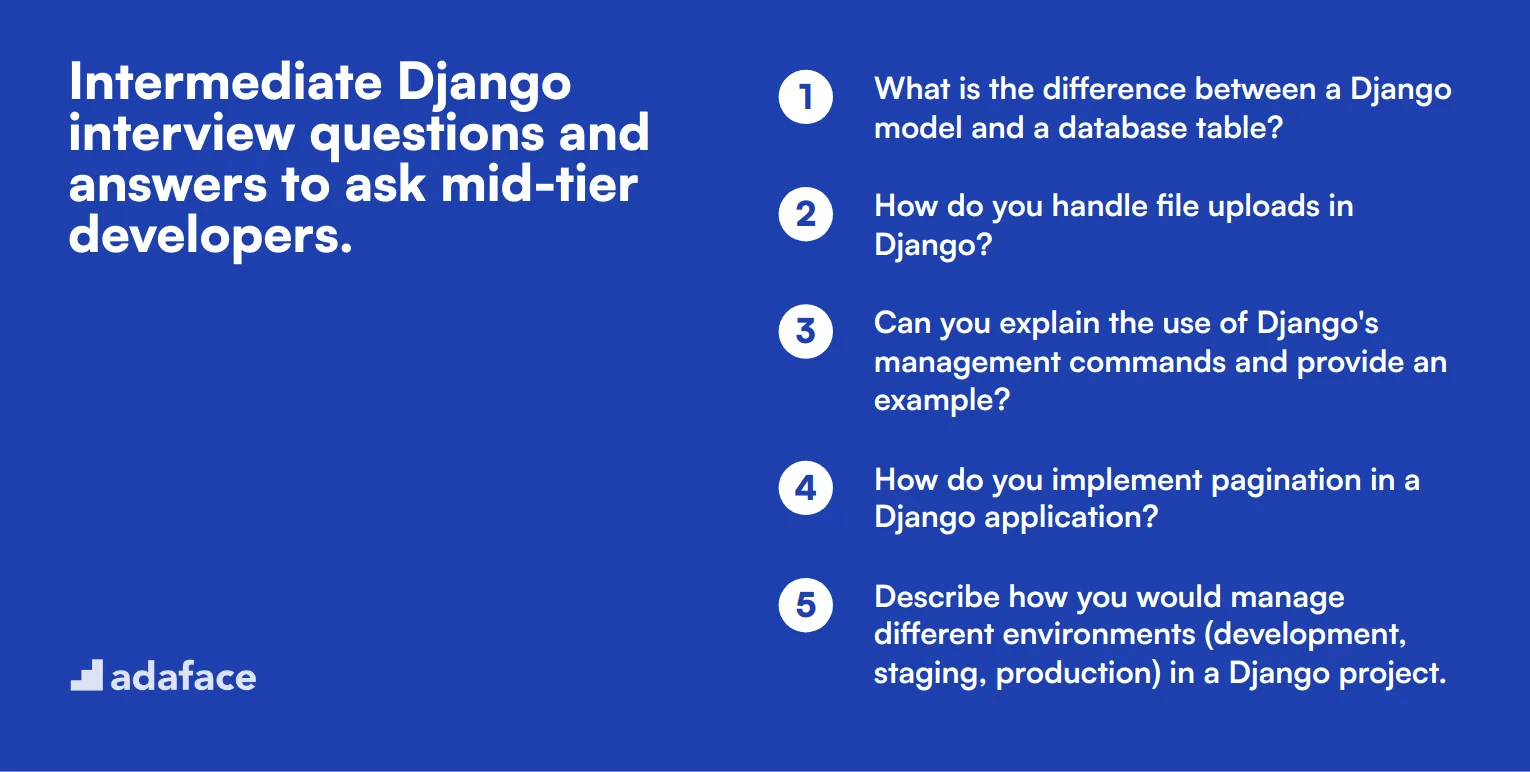
To determine whether your applicants have the right skills for mid-tier Django development, ask them some of these intermediate Django interview questions. This list will help you evaluate their understanding of more complex concepts and their ability to apply Django skills in real-world scenarios.
- What is the difference between a Django model and a database table?
- How do you handle file uploads in Django?
- Can you explain the use of Django's management commands and provide an example?
- How do you implement pagination in a Django application?
- Describe how you would manage different environments (development, staging, production) in a Django project.
- What is Django Rest Framework (DRF) and when would you use it?
- How do you handle internationalization (i18n) in a Django project?
- What are class-based views and how do they differ from function-based views in Django?
- Explain the use of Django's QuerySets and how they can be chained.
- How do you use Django's built-in user model to extend user profiles?
- What is the purpose of Django's 'apps' structure and how do you organize multiple apps within a project?
- How do you integrate third-party libraries or APIs into a Django project?
- What are custom template tags and filters in Django, and how do you create them?
- Explain how Django handles user sessions and cookies.
- How do you perform unit testing and integration testing in Django?
8 Django interview questions and answers related to framework components
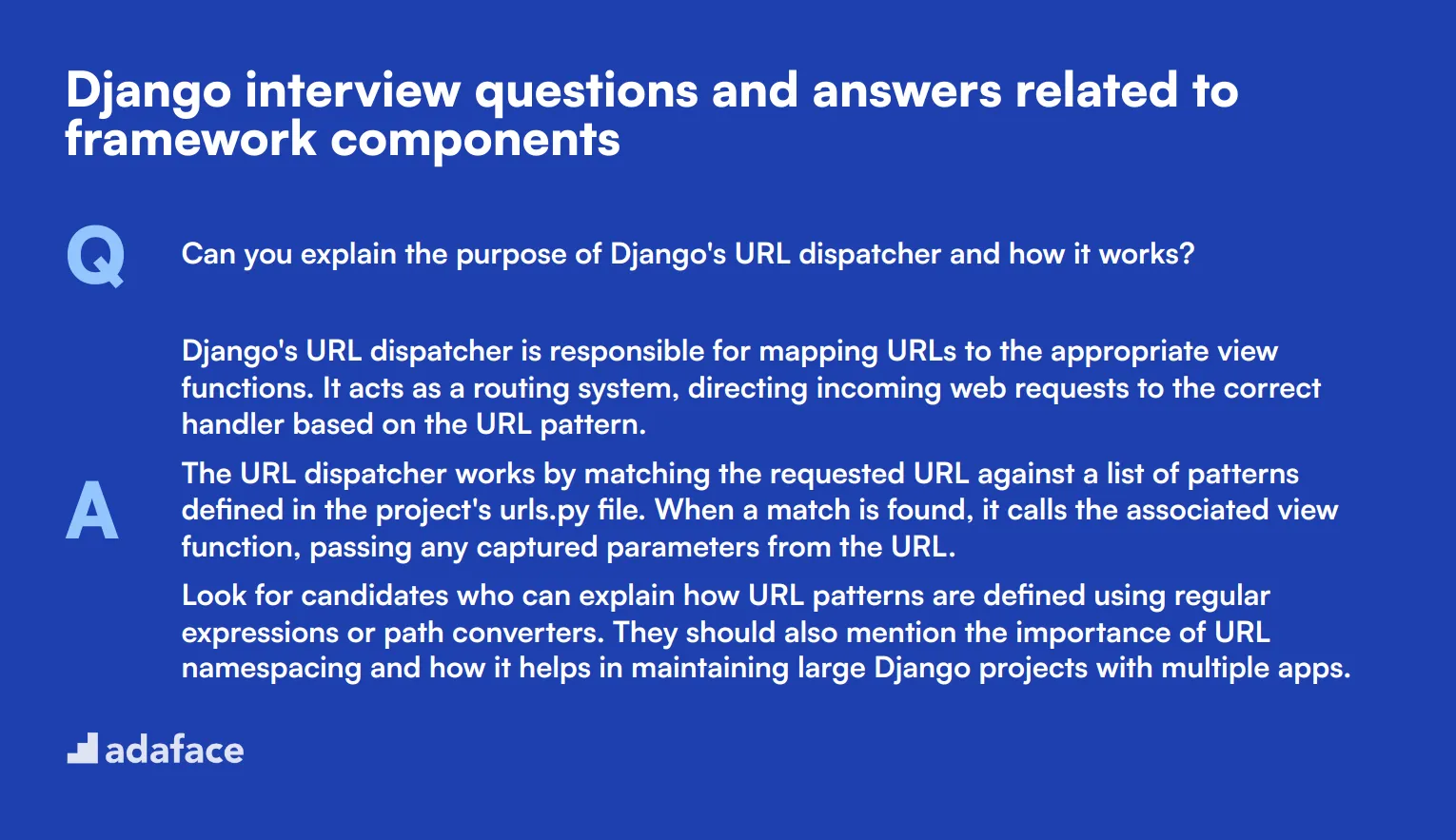
Ready to dive into the Django framework's components? These 8 interview questions will help you assess candidates' understanding of Django's core features. Use them to gauge how well applicants can leverage Django's power for efficient web development. Remember, the best responses will showcase both theoretical knowledge and practical application!
1. Can you explain the purpose of Django's URL dispatcher and how it works?
Django's URL dispatcher is responsible for mapping URLs to the appropriate view functions. It acts as a routing system, directing incoming web requests to the correct handler based on the URL pattern.
The URL dispatcher works by matching the requested URL against a list of patterns defined in the project's urls.py file. When a match is found, it calls the associated view function, passing any captured parameters from the URL.
Look for candidates who can explain how URL patterns are defined using regular expressions or path converters. They should also mention the importance of URL namespacing and how it helps in maintaining large Django projects with multiple apps.
2. What are Django forms and how do they differ from HTML forms?
Django forms are Python classes that provide a high-level abstraction for HTML forms. They handle form rendering, data validation, and processing, making it easier to work with user input in a secure and efficient manner.
Unlike plain HTML forms, Django forms offer several advantages:
- Automatic HTML generation
- Server-side validation
- Easy handling of form data in views
- Built-in CSRF protection
- Integration with Django's ORM for model-based forms
A strong candidate should be able to discuss how Django forms simplify form handling and improve security. They might also mention form widgets, custom validation, and the difference between bound and unbound forms.
3. How does Django's template inheritance work, and why is it useful?
Django's template inheritance is a powerful feature that allows developers to create a base template with common elements and then extend it in child templates. This promotes code reuse and maintains a consistent layout across multiple pages.
The process works by defining blocks in the base template that can be overridden or extended in child templates. Child templates use the {% extends %} tag to specify their parent template and then redefine or add to the blocks as needed.
Look for candidates who can explain the benefits of template inheritance, such as reducing code duplication and making site-wide changes easier. They should also be able to discuss practical examples of how they've used it in their projects.
4. What is the purpose of Django's context processors?
Django context processors are Python functions that add variables to the template context automatically. They provide a way to make certain data available to all templates without explicitly passing it in each view.
Common uses for context processors include:
- Adding user authentication information
- Providing site-wide settings
- Including debug information in development
- Adding custom variables that should be available globally
A good candidate should be able to explain how context processors are configured in settings.py and how they can create custom context processors. They might also discuss the performance implications of using context processors and when it's more appropriate to pass data directly from views.
5. Explain the concept of Django's generic views and when you would use them.
Django's generic views are pre-built class-based views that implement common web development patterns. They aim to reduce the amount of code developers need to write for standard operations like displaying lists and detail pages, or handling forms.
Some common types of generic views include:
- ListView for displaying a list of objects
- DetailView for showing details of a single object
- CreateView, UpdateView, and DeleteView for handling CRUD operations
- TemplateView for rendering a template with a simple context
Look for candidates who can explain the benefits of using generic views, such as faster development and adherence to DRY principles. They should also be able to discuss scenarios where custom views might be more appropriate and how to extend generic views for specific needs.
6. How does Django handle database migrations, and why are they important?
Django uses a migration system to manage database schema changes over time. Migrations are Python files that describe how to modify the database schema to match changes in your models.
The migration process typically involves:
- Making changes to your models
- Running 'python manage.py makemigrations' to create migration files
- Applying the migrations with 'python manage.py migrate'
A strong candidate should emphasize the importance of migrations for version control of database schemas, team collaboration, and maintaining data integrity during deployment. They might also discuss how to handle migration conflicts and the benefits of Django's migration system compared to manual schema management.
7. What is the purpose of Django's admin interface, and how would you customize it?
Django's admin interface is an automatic, model-driven interface for managing a site's content. It provides a quick way to perform CRUD operations on your models without writing additional code.
The admin interface can be customized in several ways:
- Modifying the ModelAdmin class to change how models are displayed and edited
- Creating custom admin views for complex actions
- Overriding admin templates for design changes
- Adding custom filters and actions
Look for candidates who can explain the benefits of the admin interface for rapid prototyping and content management. They should also be able to discuss scenarios where they've customized the admin for specific project needs, demonstrating an understanding of its flexibility and limitations.
8. How does Django's caching system work, and when would you use it?
Django's caching system is designed to save dynamic content so that it doesn't need to be calculated for each request. This can significantly improve the performance of a Django application, especially for pages that require complex database queries or external API calls.
Django offers several caching options:
- Per-site caching
- Per-view caching
- Template fragment caching
- Low-level cache API
A good candidate should be able to explain different caching backends (e.g., Memcached, Redis) and discuss scenarios where caching is most beneficial. They might also mention potential pitfalls, such as stale data, and strategies for cache invalidation. Look for understanding of when and how to implement caching to optimize web application performance.
12 Django questions related to ORM
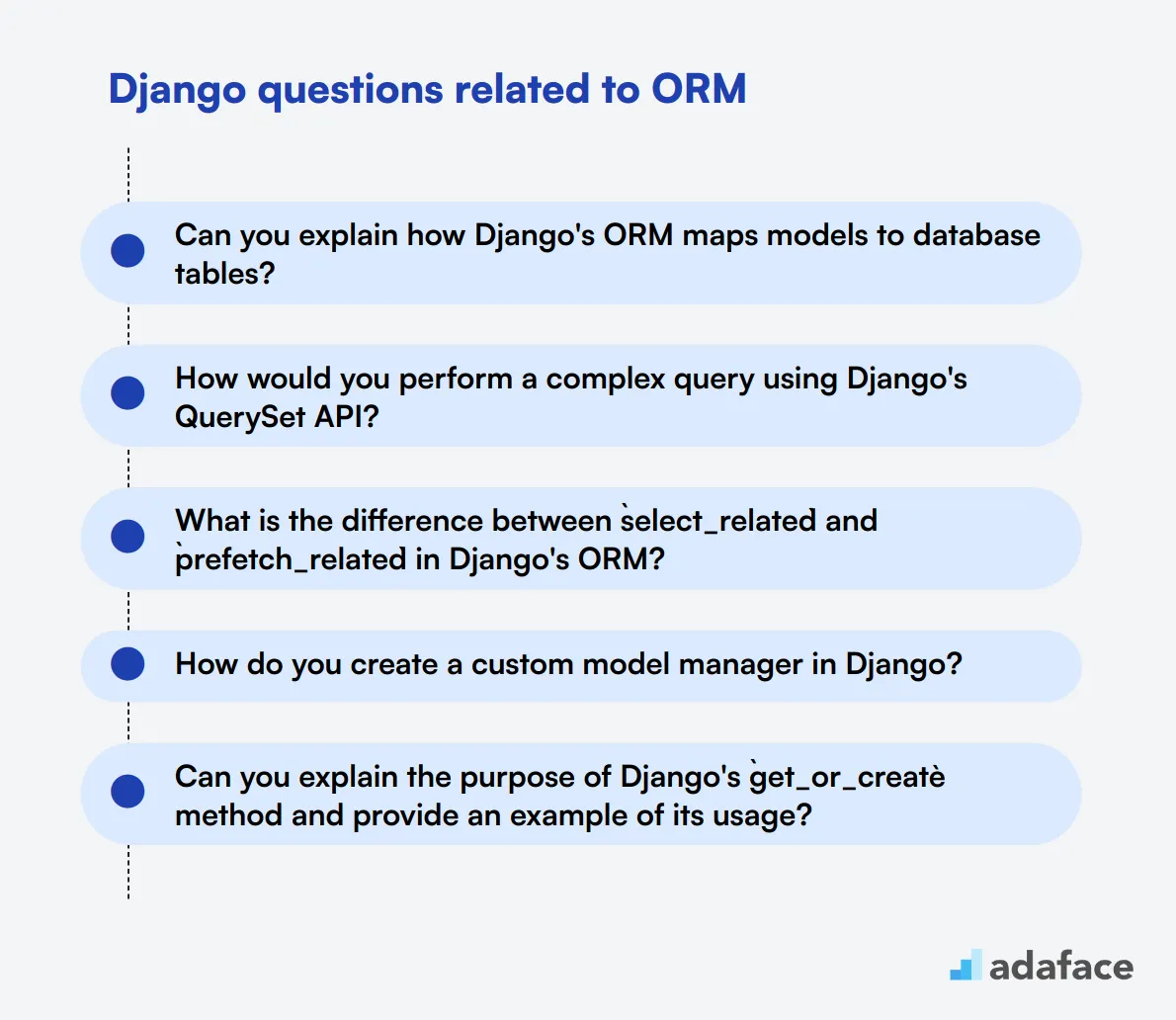
To evaluate whether candidates have a solid grasp of Django's ORM capabilities, consider using some of these targeted questions during interviews. These questions will help you determine if applicants possess the necessary skills to handle database operations effectively within a Django project, ensuring they are well-suited for roles such as Django Developer.
- Can you explain how Django's ORM maps models to database tables?
- How would you perform a complex query using Django's QuerySet API?
- What is the difference between `select_related` and `prefetch_related` in Django's ORM?
- How do you create a custom model manager in Django?
- Can you explain the purpose of Django's `get_or_create` method and provide an example of its usage?
- How do you perform bulk operations (inserts, updates) using Django's ORM?
- What are the implications of using Django's `save` method with the `update_fields` parameter?
- How do you handle many-to-many relationships in Django models?
- Can you explain how to use Django's ORM for database transactions?
- What is the purpose of the `Meta` class in Django models, and how would you use it?
- How do you handle database migrations in a multi-developer environment using Django?
- Explain how you would use Django's ORM to filter and order query results based on specific criteria.
10 situational Django interview questions for hiring top developers
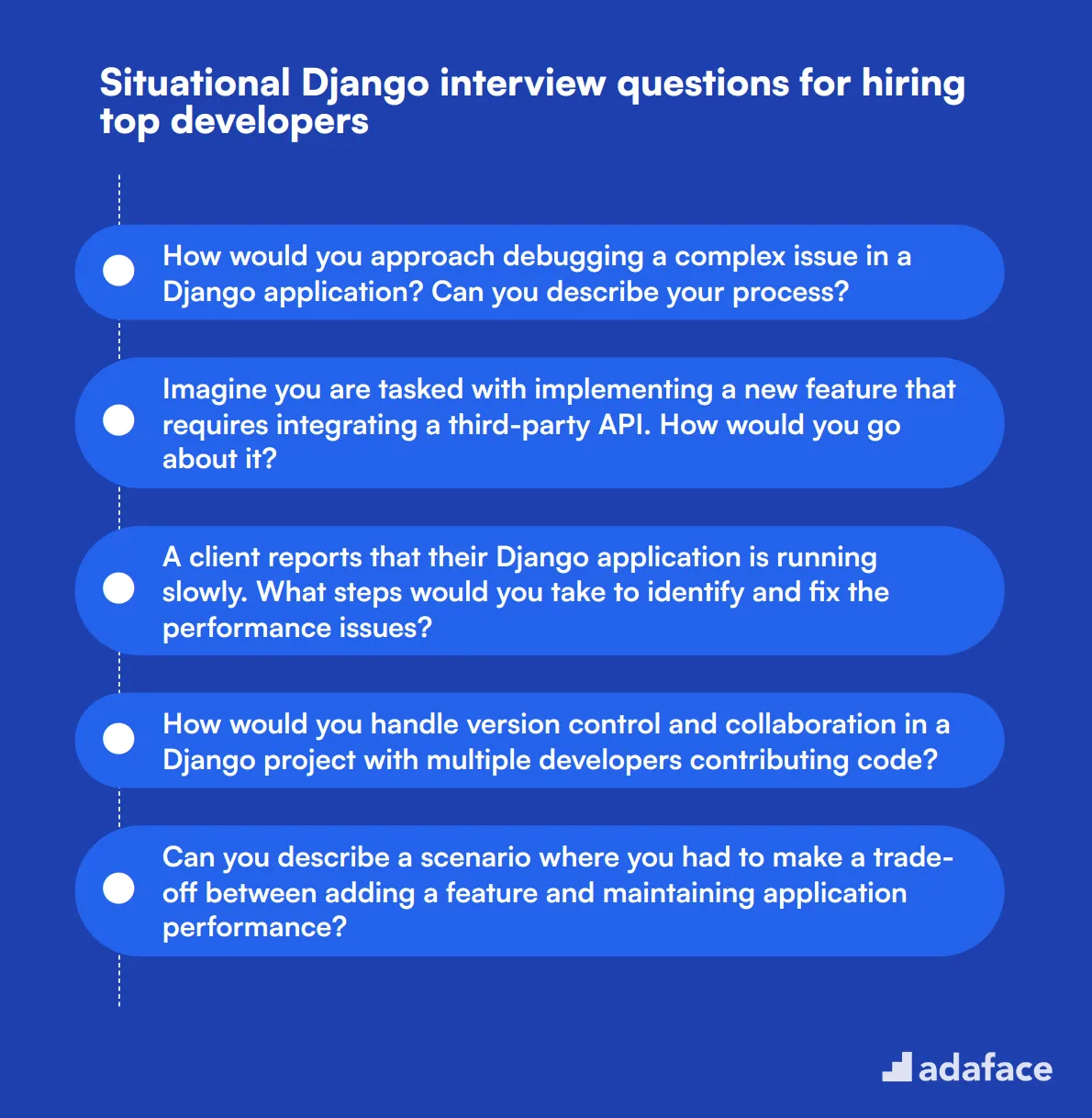
To ensure you find the best Django developers, use these situational questions during interviews. They will help gauge how candidates approach real-world challenges in Django development. This approach can reveal their problem-solving skills and technical expertise tailored for Django developer roles.
- How would you approach debugging a complex issue in a Django application? Can you describe your process?
- Imagine you are tasked with implementing a new feature that requires integrating a third-party API. How would you go about it?
- A client reports that their Django application is running slowly. What steps would you take to identify and fix the performance issues?
- How would you handle version control and collaboration in a Django project with multiple developers contributing code?
- Can you describe a scenario where you had to make a trade-off between adding a feature and maintaining application performance?
- If you had to migrate a large legacy application to Django, what would be your strategy to ensure a smooth transition?
- Suppose you need to maintain two different versions of a Django application. How would you manage this without causing disruptions?
- You discover a security vulnerability in your Django app. What immediate actions would you take to mitigate the risk?
- How would you implement a caching strategy in a Django project to improve response times for users?
- Imagine your team is adopting Django Rest Framework for a project. What best practices would you suggest to ensure a clean and maintainable codebase?
Which Django skills should you evaluate during the interview phase?
While it is impossible to assess every aspect of a candidate's skillset in a single interview, there are specific core competencies in Django that are essential to evaluate. These skills provide a foundational understanding of a candidate's ability to work effectively with the Django framework and deliver robust web applications.
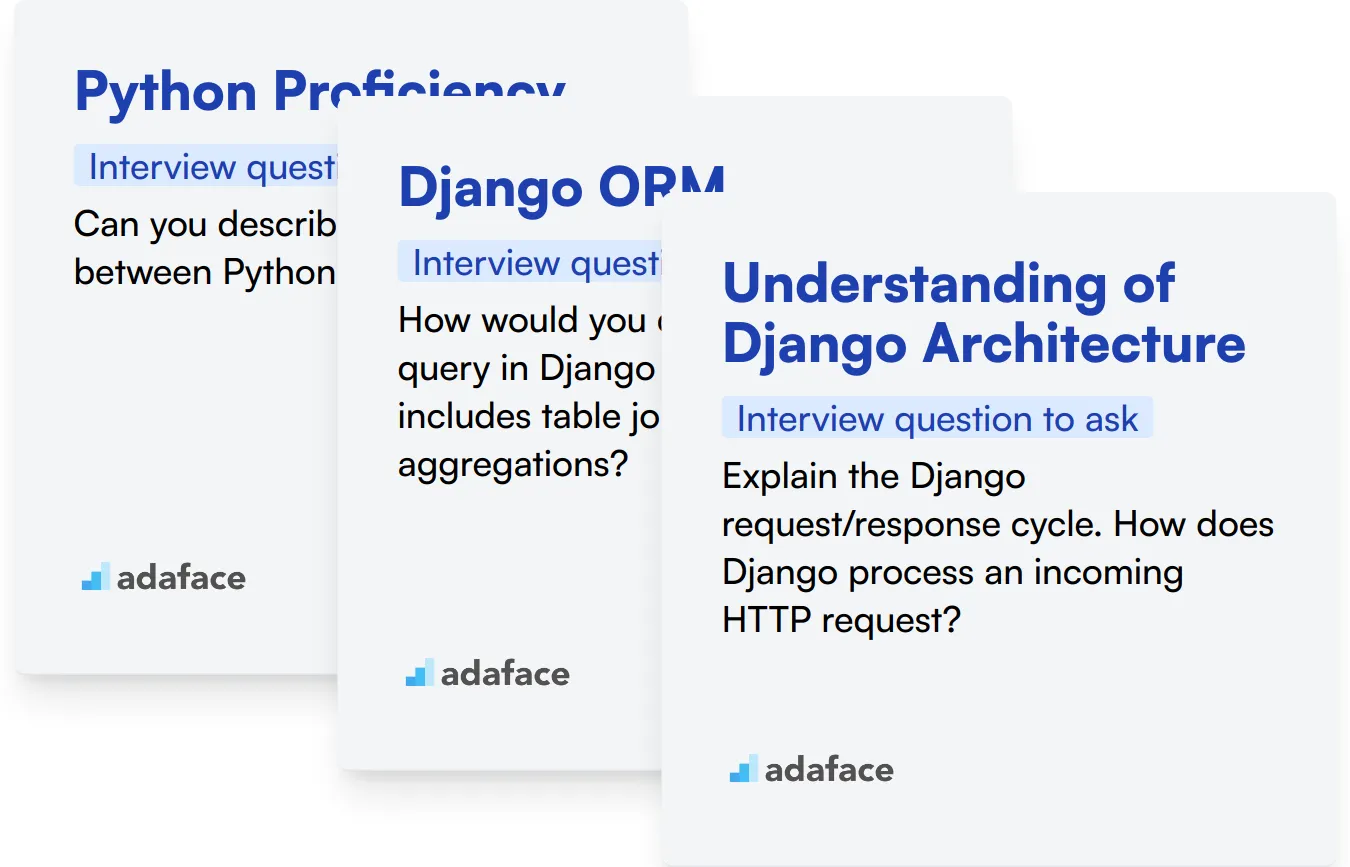
Python Proficiency
Python is the underlying language of Django, making it crucial for candidates to have strong Python skills. A proficient Python developer can easily grasp Django concepts and write efficient code.
To gauge a candidate's Python proficiency, you can use an assessment test that asks relevant MCQs. You might consider using the Adaface Python online test.
Apart from using assessment tests, you can also ask targeted interview questions to evaluate this skill.
Can you describe the differences between Python lists and tuples?
When asking this question, look for understanding of mutability, use cases, and performance differences between lists and tuples.
Django ORM
The Django ORM is a powerful tool that bridges the gap between Django models and the database. It is essential for candidates to understand how to effectively use the ORM to perform database operations.
You can assess a candidate's understanding of Django ORM with an Adaface Django online test.
Interview questions can also help you evaluate a candidate’s proficiency with Django ORM.
How would you create a complex query in Django ORM that includes table joins and aggregations?
Look for the candidate’s knowledge of Django ORM methods like select_related
, prefetch_related
, and aggregation functions.
Understanding of Django Architecture
Django's architecture is a critical aspect that developers need to grasp to build scalable and maintainable applications. This includes understanding of the MVT (Model-View-Template) pattern, middleware, and the request/response cycle.
You can evaluate a candidate’s understanding of Django architecture using the Adaface Django online test.
To further assess their knowledge, you can pose specific interview questions.
Explain the Django request/response cycle. How does Django process an incoming HTTP request?
Expect the candidate to outline the journey of a request from URL resolution through middleware to the view, and finally, the rendering of the response.
Hire top talent with Django skills tests and the right interview questions
If you're looking to hire someone with Django skills, it's important to ensure that they possess those skills accurately. A candidate’s experience and knowledge in Django can significantly impact your project's success.
The most effective way to verify a candidate's Django proficiency is through skill tests. You can explore our relevant assessments like the Python Django Test to gauge their capabilities.
Once you've administered the test, you'll be able to effectively shortlist the best applicants and invite them for interviews. This process will help you focus on candidates who not only meet the technical requirements but also align with your team's needs.
To get started, you can sign up and explore our assessment library. For more tailored options, visit our Django Online Test page.
Python, Django & SQL Online Test
Download Django interview questions template in multiple formats
Django Interview Questions FAQs
Django-specific questions help evaluate a candidate's proficiency with the framework and their ability to solve real-world problems using it.
Look for clarity, depth of knowledge, problem-solving skills, and practical experience with the Django framework.
Ask situational questions that require candidates to describe how they've used Django in past projects and the challenges they faced.
Yes, understanding Django’s ORM is crucial for effective database management in Django applications.
Yes, they help gauge how candidates apply their knowledge and skills to real-world problems.
Skill tests provide a practical assessment of a candidate’s capabilities, ensuring they can apply their knowledge effectively.
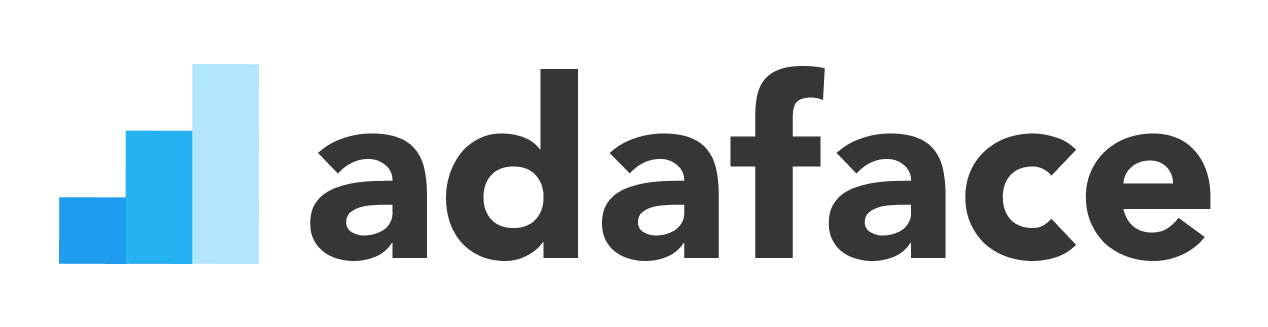
40 min skill tests.
No trick questions.
Accurate shortlisting.
We make it easy for you to find the best candidates in your pipeline with a 40 min skills test.
Try for freeRelated posts
Free resources
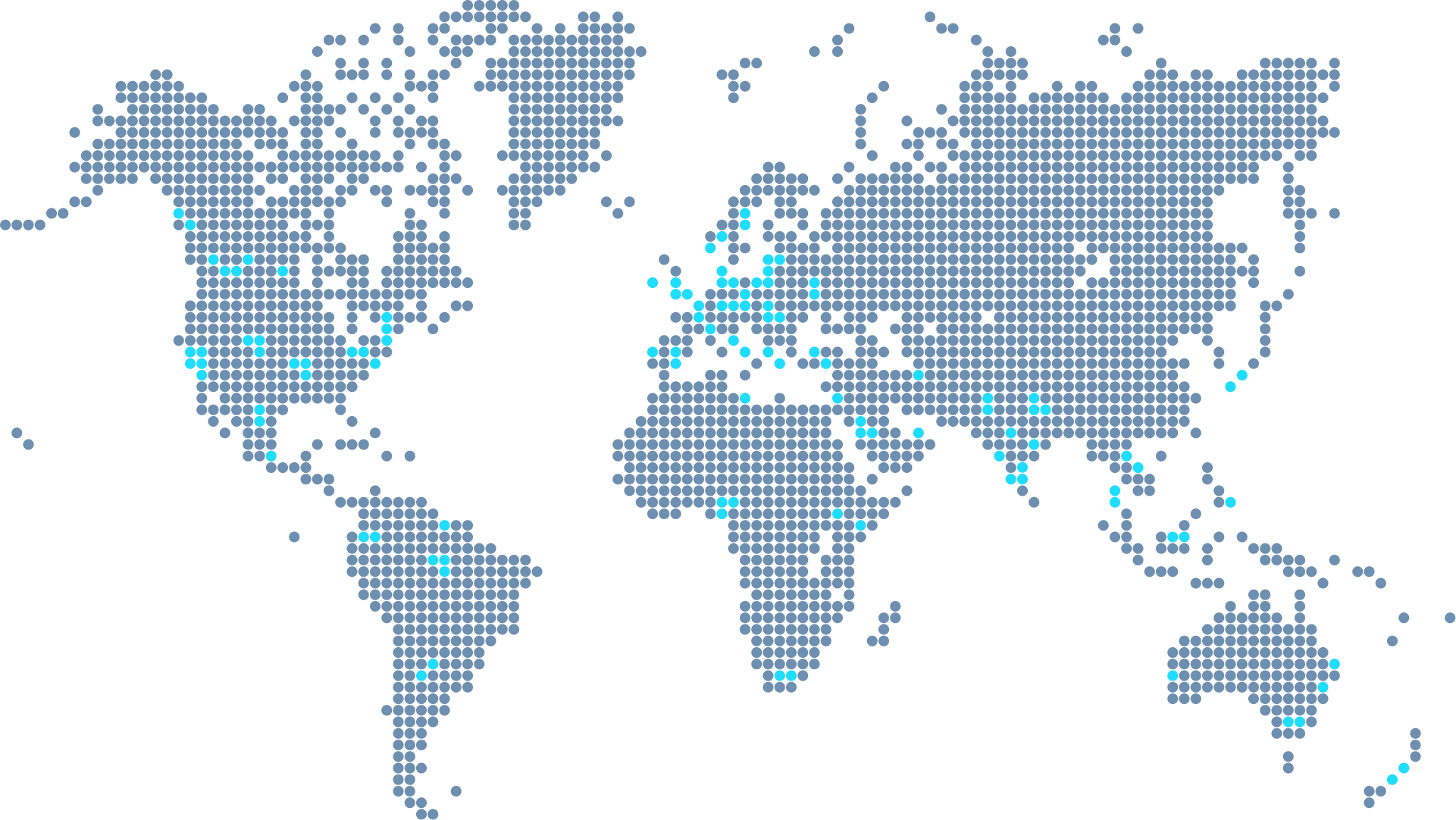
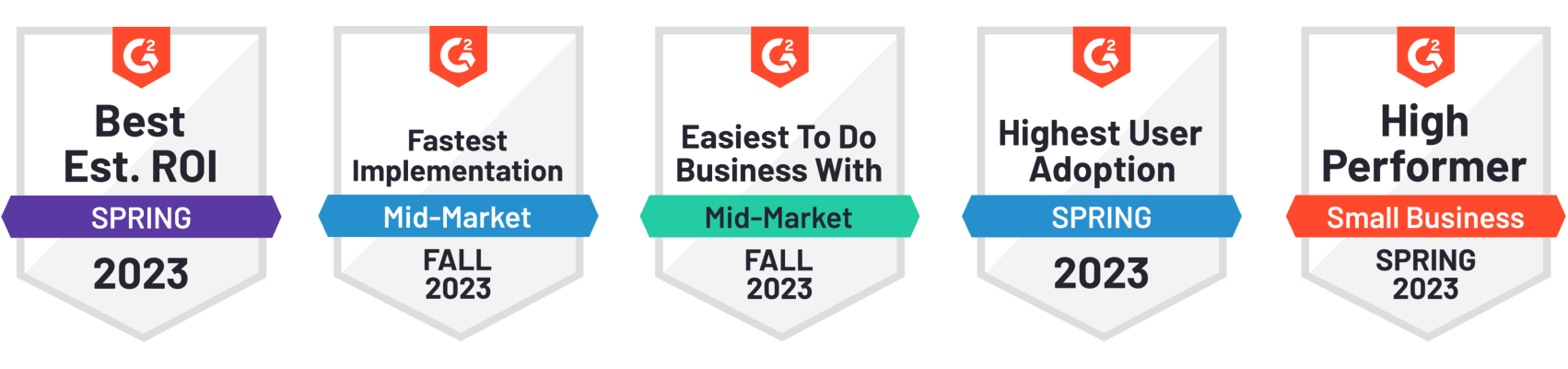