Recruiting top Swift developers can be challenging, especially if you don't know what specific questions to ask. This guide will help you identify the skills and expertise necessary for different experience levels in Swift development.
In this blog post, we cover a comprehensive list of interview questions categorized into general, junior, intermediate, senior, memory management, protocols and delegates, and situational scenarios. Each category is tailored to assess Swift developers at varying stages of their careers.
Using these questions, you can streamline your interview process and pinpoint the best candidates for your team. Additionally, consider using our Swift online test to pre-qualify candidates before the interview stage.
Table of contents
7 general Swift interview questions and answers
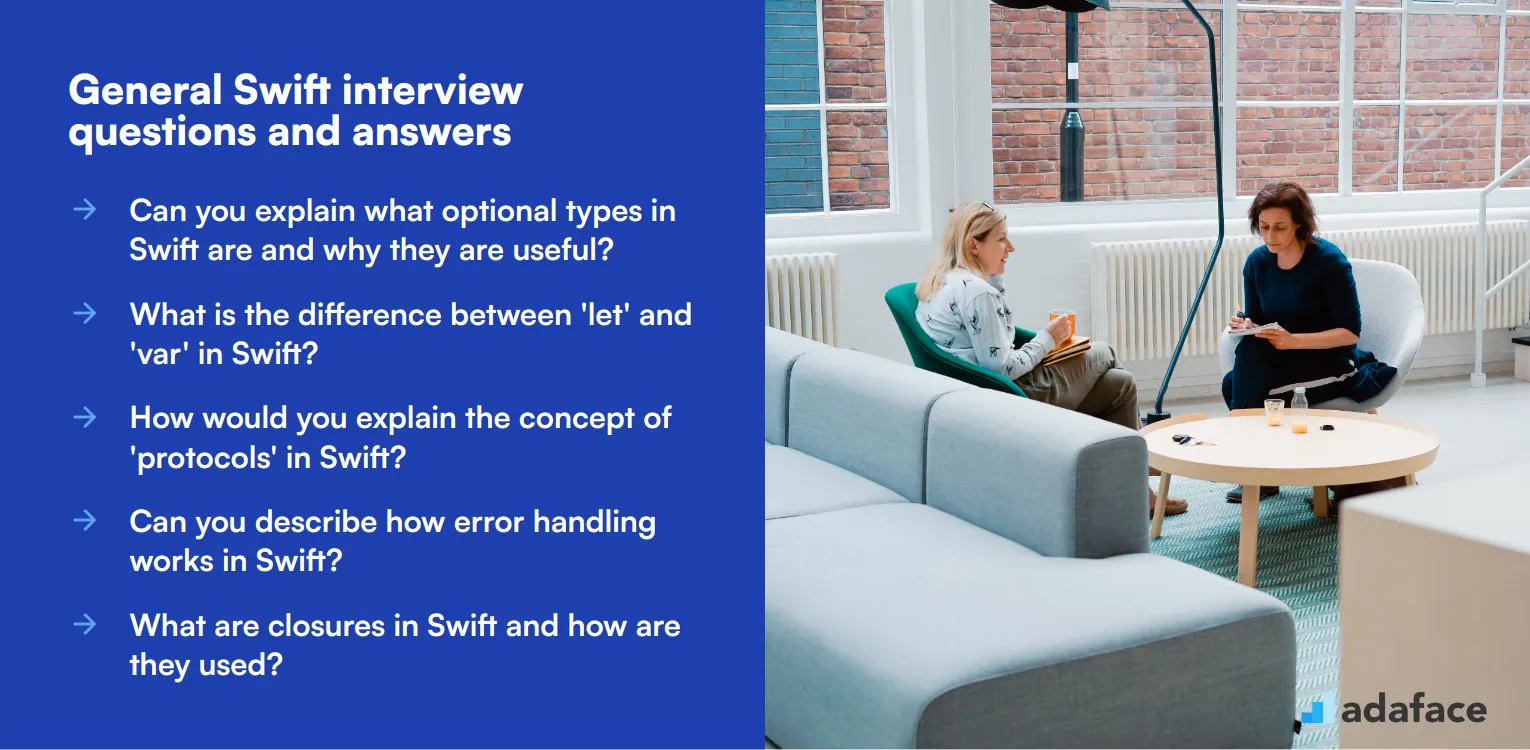
To ensure you’re hiring a developer with a solid understanding of Swift, we've compiled a list of essential interview questions. These questions are designed to gauge a candidate's grasp of fundamental concepts and their ability to apply them in real-world scenarios.
1. Can you explain what optional types in Swift are and why they are useful?
Optionals in Swift are a type that can hold either a value or no value at all (nil). They are used to handle the absence of a value in a type-safe way, ensuring that a variable is either assigned a value or clearly marked as having no value.
Optionals are particularly useful for avoiding errors that arise from uninitialized variables or null pointer exceptions, common in other programming languages. They make it clear when a value might be missing and force the developer to handle that case explicitly.
Look for candidates who can articulate the concept clearly and understand the practical implications of using optionals in code. Follow up by asking how they handle unwrapping optionals safely.
2. What is the difference between 'let' and 'var' in Swift?
In Swift, 'let' is used to declare constants, meaning the value cannot be changed once set. On the other hand, 'var' is used to declare variables, allowing the value to be modified after it has been initialized.
Using 'let' promotes immutability, which can help prevent bugs by ensuring the values remain consistent throughout the code. 'Var' should be used when the value needs to change, such as in loops or when tracking state.
An ideal candidate should demonstrate a good understanding of when to use 'let' versus 'var' and the importance of immutability for maintaining code stability and readability.
3. How would you explain the concept of 'protocols' in Swift?
Protocols in Swift define a blueprint of methods, properties, and other requirements that suit a particular task or piece of functionality. Classes, structs, and enums can adopt these protocols to ensure they conform to the blueprint.
Protocols are a powerful feature in Swift because they allow for a high degree of flexibility and reuse in code. They promote loose coupling and can be used to implement multiple inheritance in a way that avoids some of the pitfalls seen in other languages.
A strong answer should include an understanding of how protocols can be used to define clear and reusable interfaces. Look for examples from the candidate's past work where protocols helped in designing maintainable and scalable code.
4. Can you describe how error handling works in Swift?
Swift uses a combination of throwing, catching, and propagating errors to handle exceptions. Functions can be marked with the 'throws' keyword to indicate they can throw an error. Errors must be handled using 'do-catch' blocks, 'try?' for optional results, or 'try!' for force unwrapping.
Error handling in Swift is designed to be clear and concise, ensuring that errors are properly managed without cluttering the code. It forces developers to think about error scenarios and handle them appropriately.
Candidates should demonstrate a clear understanding of the different ways to handle errors and discuss scenarios where each method is appropriate. Follow up by asking about specific challenges they've faced with error handling in past projects.
5. What are closures in Swift and how are they used?
Closures in Swift are self-contained blocks of functionality that can be passed around and used in your code. They can capture and store references to variables and constants from the context in which they are defined.
Closures are often used for callback functions, event handlers, and to encapsulate functionality that will be executed at a later time. They promote code reuse and can lead to more readable and maintainable code.
Look for candidates who can clearly explain closures and provide examples of how they have used them in their previous work. Understanding the concept of capturing values and avoiding retain cycles is also crucial.
6. How do you manage memory in Swift?
Swift uses Automatic Reference Counting (ARC) to manage memory. ARC tracks and manages the memory usage of your app by keeping a count of references to each instance. When the reference count drops to zero, the instance is deallocated.
Developers need to be mindful of strong, weak, and unowned references to avoid retain cycles, which can lead to memory leaks. Weak references are used when one instance doesn't own the other, and unowned references are used when the reference will never be nil after initialization.
Candidates should demonstrate an understanding of how ARC works and discuss strategies they use to prevent memory leaks. Ask for specific examples of how they've debugged memory issues in the past.
7. What is the role of a delegate in Swift?
A delegate in Swift is a design pattern that allows one object to communicate with another object in a loosely coupled way. It involves a protocol that defines methods to be implemented by the delegate, which then handles or responds to specific events.
Delegates are commonly used in UI components to manage events like user interactions, data updates, or asynchronous tasks. They promote separation of concerns, making code more modular and easier to maintain.
Strong candidates will be able to explain the delegate pattern clearly and provide examples of how they’ve used it in their projects. Look for an understanding of how delegation improves code architecture and decouples components.
20 Swift interview questions to ask junior developers
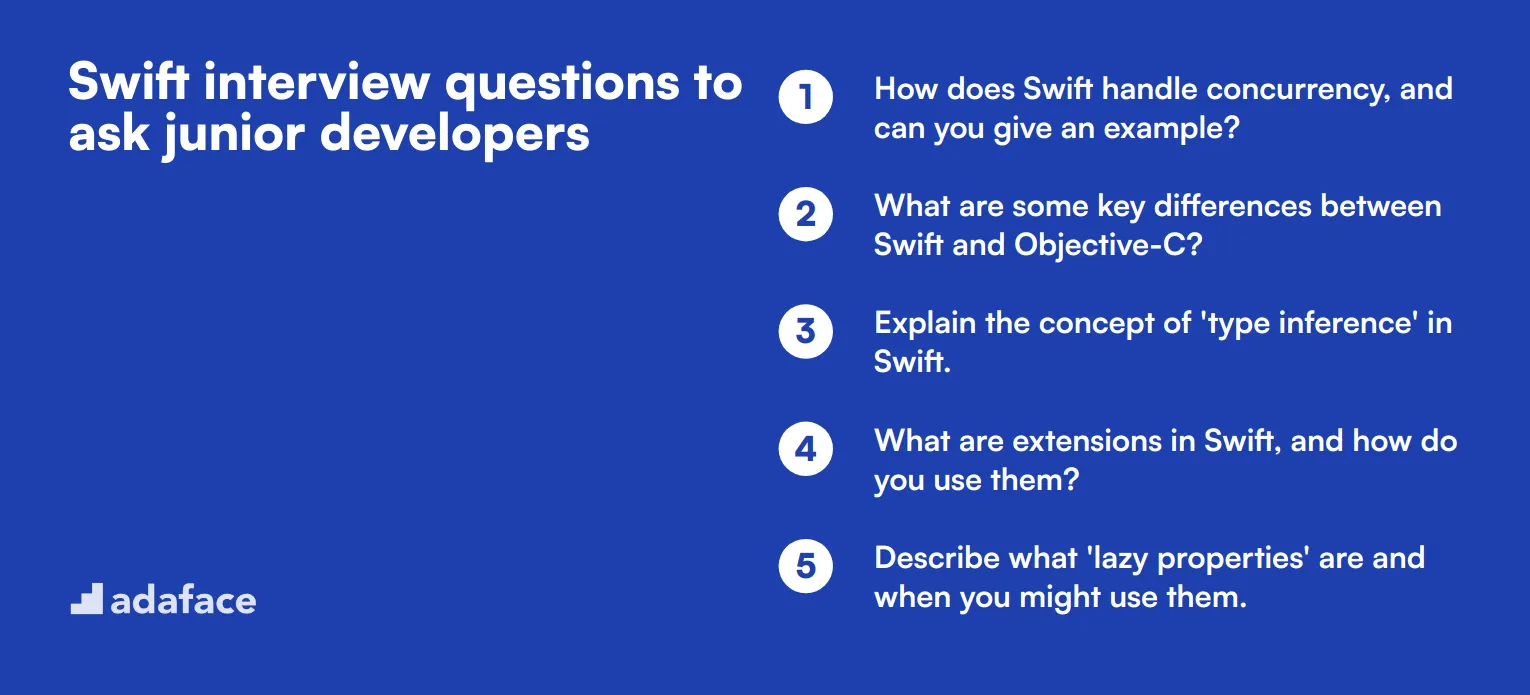
To ensure you hire the right junior developer for your team, use these 20 Swift interview questions. These questions are designed to help you evaluate the candidate's understanding of essential Swift concepts and their ability to apply them in real-world scenarios. For a deeper dive into the skills required for a Swift developer, check out this guide.
- How does Swift handle concurrency, and can you give an example?
- What are some key differences between Swift and Objective-C?
- Explain the concept of 'type inference' in Swift.
- What are extensions in Swift, and how do you use them?
- Describe what 'lazy properties' are and when you might use them.
- How do you handle JSON data in Swift?
- Can you explain what 'guard' statements do in Swift?
- What is the role of 'didSet' and 'willSet' in Swift?
- How would you implement a singleton pattern in Swift?
- Describe the model-view-controller (MVC) pattern and its use in iOS development.
- What are generics in Swift? Can you provide an example?
- How do you achieve thread safety in Swift?
- Explain what 'enum' is in Swift and provide an example use case.
- What is the difference between a class and a struct in Swift?
- Can you explain the concept of 'mutability' in Swift?
- What are higher-order functions in Swift? Can you give an example?
- How would you debug a Swift application?
- What is the purpose of 'defer' in Swift?
- Explain what 'weak' and 'unowned' references are and when you would use them.
- What are Swift Playgrounds, and how can they be useful?
10 intermediate Swift interview questions and answers to ask mid-tier developers
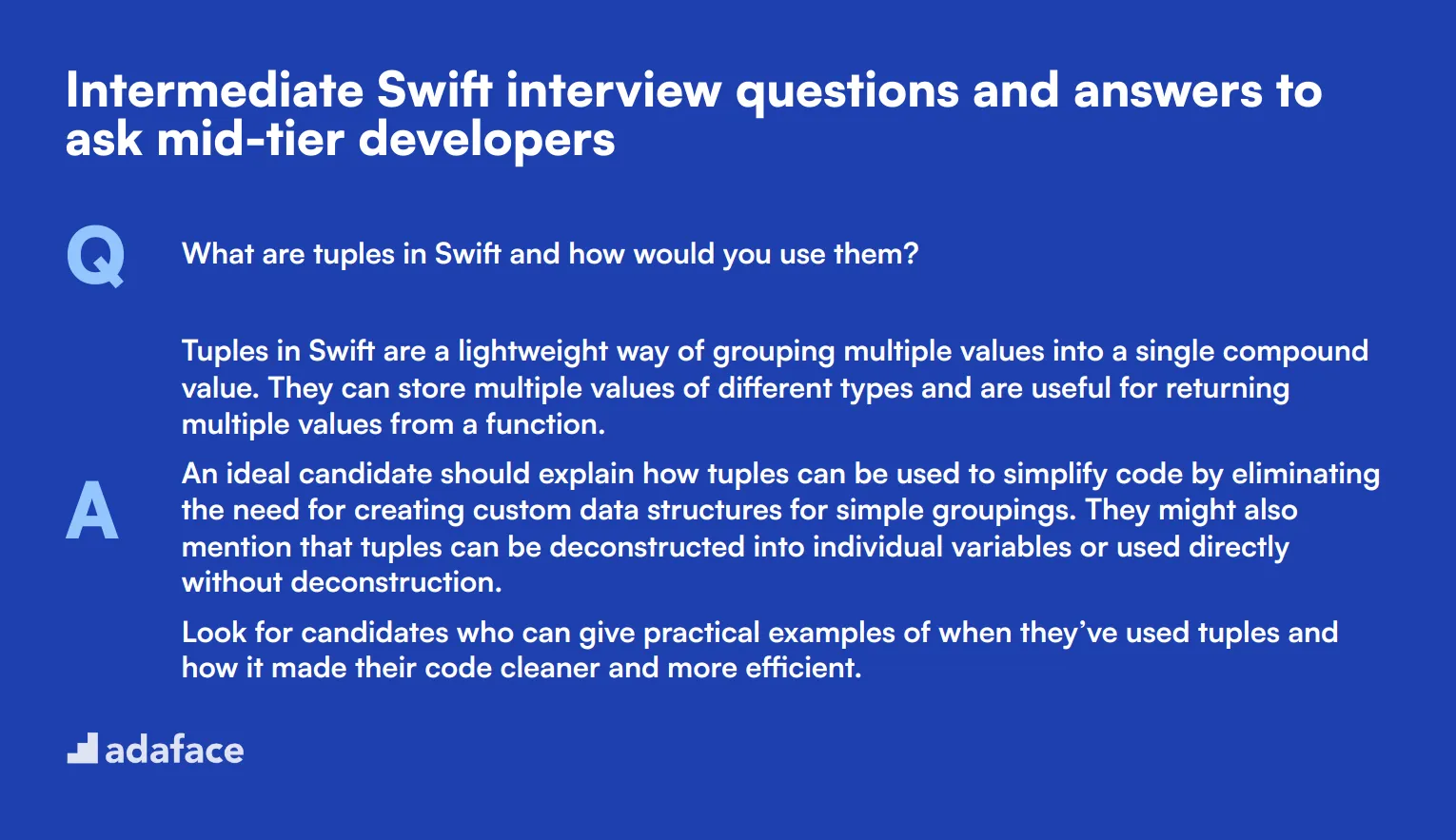
To find out if your candidates have a solid grasp of Swift's intermediate concepts, ask them some of these questions. This list is designed to help you gauge their deeper understanding and practical knowledge of Swift, ensuring they can tackle more complex tasks in your projects.
1. What are tuples in Swift and how would you use them?
Tuples in Swift are a lightweight way of grouping multiple values into a single compound value. They can store multiple values of different types and are useful for returning multiple values from a function.
An ideal candidate should explain how tuples can be used to simplify code by eliminating the need for creating custom data structures for simple groupings. They might also mention that tuples can be deconstructed into individual variables or used directly without deconstruction.
Look for candidates who can give practical examples of when they’ve used tuples and how it made their code cleaner and more efficient.
2. How does Swift ensure type safety?
Swift is a type-safe language, which means it helps you be clear about the types of values your code can work with. If a part of your code expects a String
, you can’t pass it an Int
by mistake.
The type safety feature helps in catching and fixing errors early in the development process. Swift's compiler performs type checks and ensures that mismatched types can't interact with each other, reducing the risk of runtime crashes.
Candidates should demonstrate an understanding of type safety by discussing how it helps prevent bugs and makes code more predictable and easier to maintain.
3. What is the difference between weak and strong references in Swift?
In Swift, a strong reference is the default and keeps a firm hold on the object it references, preventing it from being deallocated. A weak reference, on the other hand, does not keep a strong hold and allows the object to be deallocated.
Weak references are often used to avoid retain cycles, which can lead to memory leaks. They are typically used in delegate patterns where the delegate does not need to own the object it references.
Look for candidates who understand when and why to use weak references, and can provide scenarios where using weak references helped them maintain better memory management in their projects.
4. What is the purpose of using 'lazy' properties in Swift?
'Lazy' properties in Swift are not initialized until they are first accessed. This can be useful for properties that require significant resources to initialize or when their initial value depends on external factors that are not available during initialization.
Using 'lazy' properties can improve performance by deferring the creation of an object until it's really needed. This can be especially beneficial in cases where the property might not be used at all during the lifecycle of the object.
An ideal candidate should explain practical scenarios where they have used 'lazy' properties and how it benefited their application's performance or resource management.
5. How does Swift handle version compatibility when updating to a new version?
Swift uses a mechanism called Swift versions to manage compatibility. When a new version of Swift is released, it may include changes that are not backward compatible. Swift provides migration tools to help developers update their code to the latest version.
Developers can specify which Swift version their code should use by configuring their project settings. This ensures that code remains stable and predictable, even as new language features and improvements are introduced.
Candidates should show an understanding of the importance of version compatibility and discuss how they manage updates in their projects, ensuring smooth transitions and minimal disruption.
6. What are optionals in Swift and how do they enhance safety in your code?
Optionals in Swift represent a variable that can hold either a value or no value at all. They are a powerful feature that helps prevent runtime errors by making it clear when variables might be nil
.
By forcing developers to handle the nil
case explicitly, optionals make the code safer and more predictable. This reduces the risk of unexpected crashes due to unhandled nil
values.
An ideal candidate should discuss practical examples of using optionals, such as safely unwrapping optionals with if let
or guard let
, and how it has improved the robustness of their code.
7. How do you handle asynchronous tasks in Swift?
In Swift, asynchronous tasks can be handled using various techniques such as closures, delegate methods, and more recently, the Combine framework or Swift's native concurrency model using async
and await
.
Handling asynchronous tasks properly is crucial for maintaining a responsive user interface. Candidates should discuss how they manage tasks that run in the background and update the UI on the main thread.
Look for candidates who can provide examples of using these techniques in real-world applications, ensuring smooth and efficient user experiences.
8. What is the significance of 'Protocol-Oriented Programming' in Swift?
Protocol-Oriented Programming (POP) in Swift emphasizes the use of protocols to define methods and properties that can be adopted by classes, structs, and enums. This approach promotes code reusability and flexibility.
POP helps in reducing code duplication and enhancing the modularity of code by defining behavior in protocols that can be shared across different types. It encourages composition over inheritance.
An ideal candidate should explain how they have used POP in their projects and the benefits it brought in terms of code maintainability and scalability.
9. How do you go about designing a responsive and efficient user interface in Swift?
Designing a responsive and efficient user interface in Swift involves using Auto Layout for dynamic and adaptive layouts, optimizing performance by avoiding heavy computations on the main thread, and utilizing efficient data structures.
Candidates should discuss their approach to handling different screen sizes and orientations, ensuring that the UI remains consistent and user-friendly across various devices.
Look for candidates who can provide examples of their work on UI design, particularly how they addressed challenges related to performance and responsiveness, and their familiarity with iOS developer job description.
10. How does Swift's memory management work, and what are some common pitfalls?
Swift uses Automatic Reference Counting (ARC) for memory management, which tracks and manages the app’s memory usage by counting the number of references to each object. When the reference count of an object drops to zero, the memory used by the object is automatically deallocated.
Common pitfalls include retain cycles, where two objects hold strong references to each other, preventing ARC from deallocating them. This can lead to memory leaks.
Candidates should discuss strategies for avoiding retain cycles, such as using weak or unowned references, and provide examples of how they have managed memory in their projects to ensure efficient resource usage.
15 advanced Swift interview questions to ask senior developers
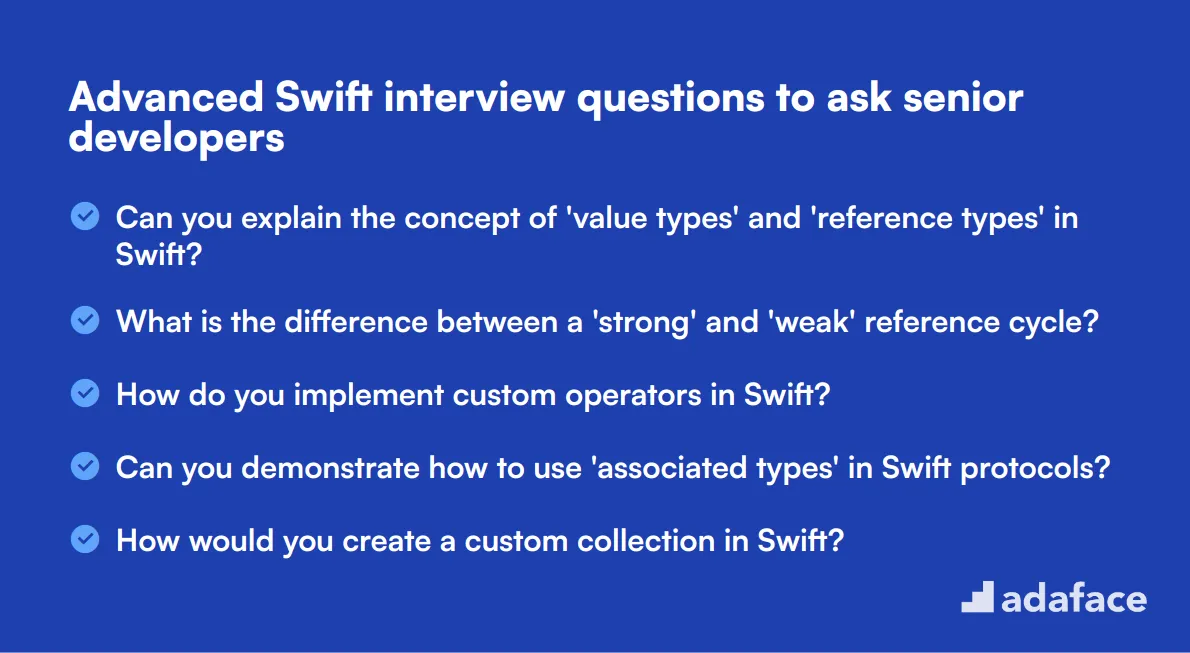
To ensure you find the right talent for your team, use these advanced Swift interview questions when assessing senior developers. These questions will help you gauge their in-depth knowledge of Swift and their ability to solve complex problems. For more details on the skills required for a Swift developer, check out this guide.
- Can you explain the concept of 'value types' and 'reference types' in Swift?
- What is the difference between a 'strong' and 'weak' reference cycle?
- How do you implement custom operators in Swift?
- Can you demonstrate how to use 'associated types' in Swift protocols?
- How would you create a custom collection in Swift?
- What are property wrappers in Swift and when would you use them?
- How do you handle multi-threading in Swift using GCD (Grand Central Dispatch)?
- Can you explain the difference between synchronous and asynchronous tasks in Swift?
- Describe how you would handle dependency injection in a Swift application.
- How do you implement and use result types in Swift?
- What are context-sensitive keywords in Swift and how are they used?
- Can you explain the purpose and use of 'dynamic' in Swift?
- How would you optimize a Swift application for performance?
- Describe the process of handling large data sets in Swift using Core Data.
- How do you manage code modularity and organization in Swift projects?
8 Swift interview questions and answers related to memory management
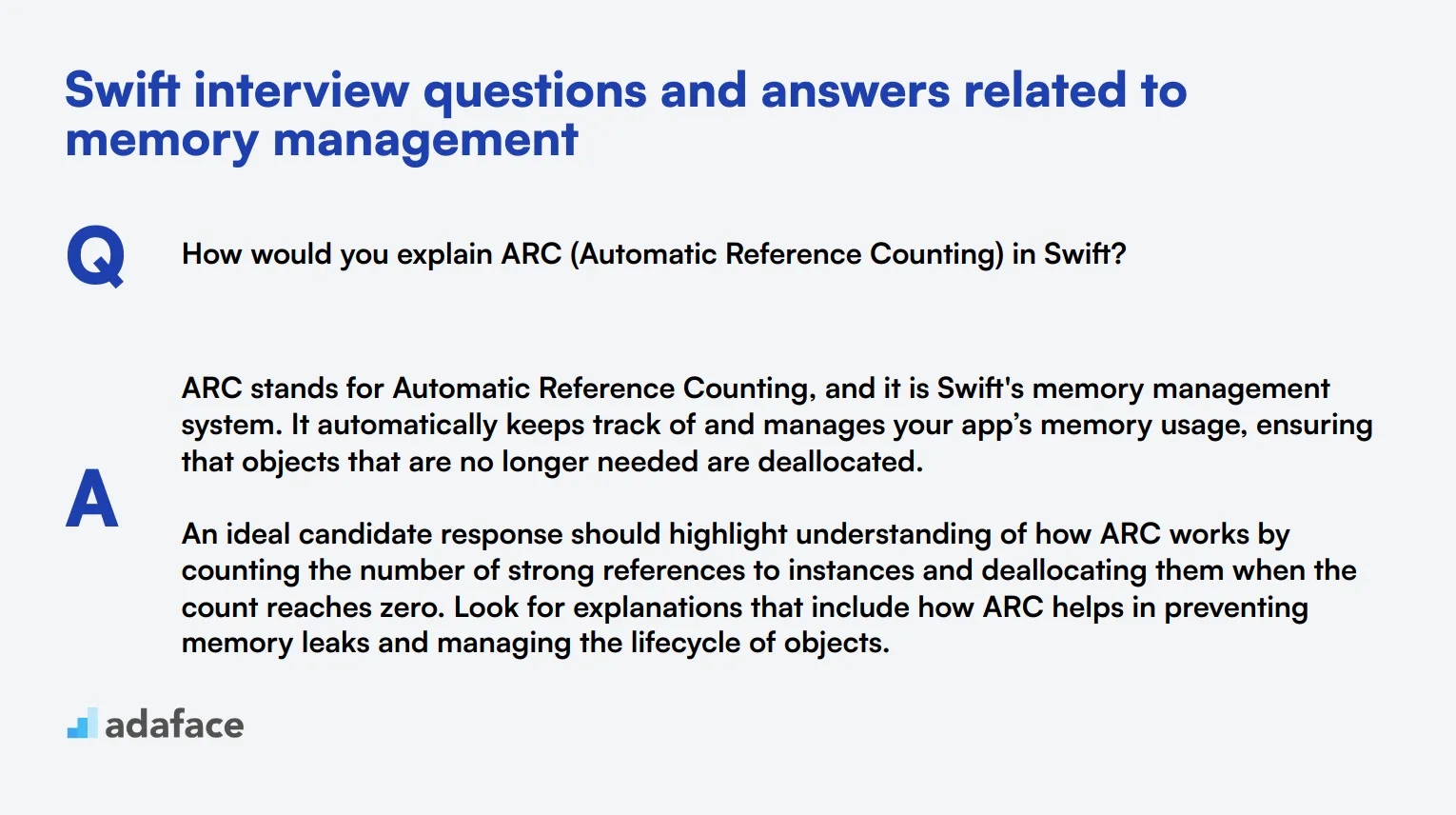
To assess whether candidates have a solid understanding of memory management in Swift, use these essential interview questions. These questions are designed to help you evaluate their knowledge and practical skills in managing resources effectively in Swift applications.
1. How would you explain ARC (Automatic Reference Counting) in Swift?
ARC stands for Automatic Reference Counting, and it is Swift's memory management system. It automatically keeps track of and manages your app’s memory usage, ensuring that objects that are no longer needed are deallocated.
An ideal candidate response should highlight understanding of how ARC works by counting the number of strong references to instances and deallocating them when the count reaches zero. Look for explanations that include how ARC helps in preventing memory leaks and managing the lifecycle of objects.
2. What are strong, weak, and unowned references in Swift, and when would you use each?
In Swift, references to instances can be strong, weak, or unowned. A strong reference means that as long as the reference exists, the instance it points to is kept in memory. Weak references are used to prevent strong reference cycles; they do not keep the instance in memory if there are no other strong references. Unowned references are similar to weak references but are used when you know the reference will always point to an instance that has not yet been deallocated.
Candidates should explain that strong references are the default and are used when you need to ensure an instance stays in memory. Weak references are typically used for properties that can become nil, such as delegate properties. Unowned references are used when you have a non-optional reference that should not create a strong reference cycle but is guaranteed to always have a value. Look for understanding of practical use cases and how these references prevent memory leaks.
3. Can you describe what a retain cycle is and how to avoid it in Swift?
A retain cycle occurs when two or more objects hold strong references to each other, causing a memory leak since neither object can be deallocated. This can happen, for example, between a parent and child object, or between delegates and their owners.
To avoid retain cycles, Swift developers use weak and unowned references. A weak reference is used when the referenced object can become nil in the future, and an unowned reference is used when the reference will never become nil but should not cause a retain cycle. Ideal answers should include practical scenarios where retain cycles might occur and how to identify and resolve them.
4. What is a memory leak, and how can you detect and fix it in a Swift application?
A memory leak occurs when allocated memory is not properly deallocated, leading to wasted memory resources and potentially causing the application to crash. Memory leaks often happen due to retain cycles or improper memory management.
To detect and fix memory leaks in Swift, developers typically use tools like Xcode’s Instruments, especially the Leaks and Allocations instruments. Candidates should discuss using these tools to profile and track memory usage, identify leaks, and fix them by breaking retain cycles or correctly managing object lifecycles. Look for practical examples and familiarity with debugging tools.
5. How does Swift handle memory management for value types vs. reference types?
In Swift, value types (like structs and enums) are copied when they are assigned to a new variable or passed to a function, which means each instance keeps its own copy of data. Reference types (like classes) share a single instance of data, and when assigned or passed, they create references to the same instance.
Candidates should explain that value types are generally safer and simpler for memory management because they do not involve reference counting. Reference types, however, require careful management using ARC to avoid memory leaks and retain cycles. Look for an understanding of when to use value vs. reference types based on the needs of the application.
6. Can you explain the concept of 'deinit' in Swift and when it should be used?
The 'deinit' method in Swift is called just before an instance of a class is deallocated. It is the place where you can perform cleanup tasks, such as removing observers, closing files, or releasing resources that the instance was holding.
Candidates should mention that 'deinit' is only available for classes since value types are automatically managed and do not need explicit cleanup. Look for examples of how 'deinit' can be used effectively and an understanding of its importance in resource management.
7. What is the difference between 'copy-on-write' and manual copying, and how does Swift implement 'copy-on-write'?
Copy-on-write is a technique where a copy of an object is only made when it is modified. In Swift, this is implemented for certain value types like arrays and dictionaries to optimize performance and memory usage by delaying the copy operation until it is necessary.
Candidates should explain that manual copying involves explicitly creating a new instance, which can be less efficient. They should demonstrate understanding of how 'copy-on-write' works in Swift by discussing scenarios where it’s beneficial and how it helps in managing memory efficiently. Look for clarity on the performance benefits and practical examples.
8. How do you manage large data sets in memory within a Swift application?
Managing large data sets in Swift requires strategies to optimize memory usage and performance. Techniques include using lazy properties to load data only when needed, employing pagination to load data in chunks, and leveraging background threads to handle data processing without blocking the main thread.
Candidates should discuss specific practices like using Core Data for efficient data storage and retrieval, leveraging Swift's built-in memory management tools, and ensuring efficient data structures. Look for knowledge of tools and libraries that assist in managing large datasets, and practical examples of how they've handled such scenarios in past projects.
9 Swift interview questions and answers related to protocols and delegates
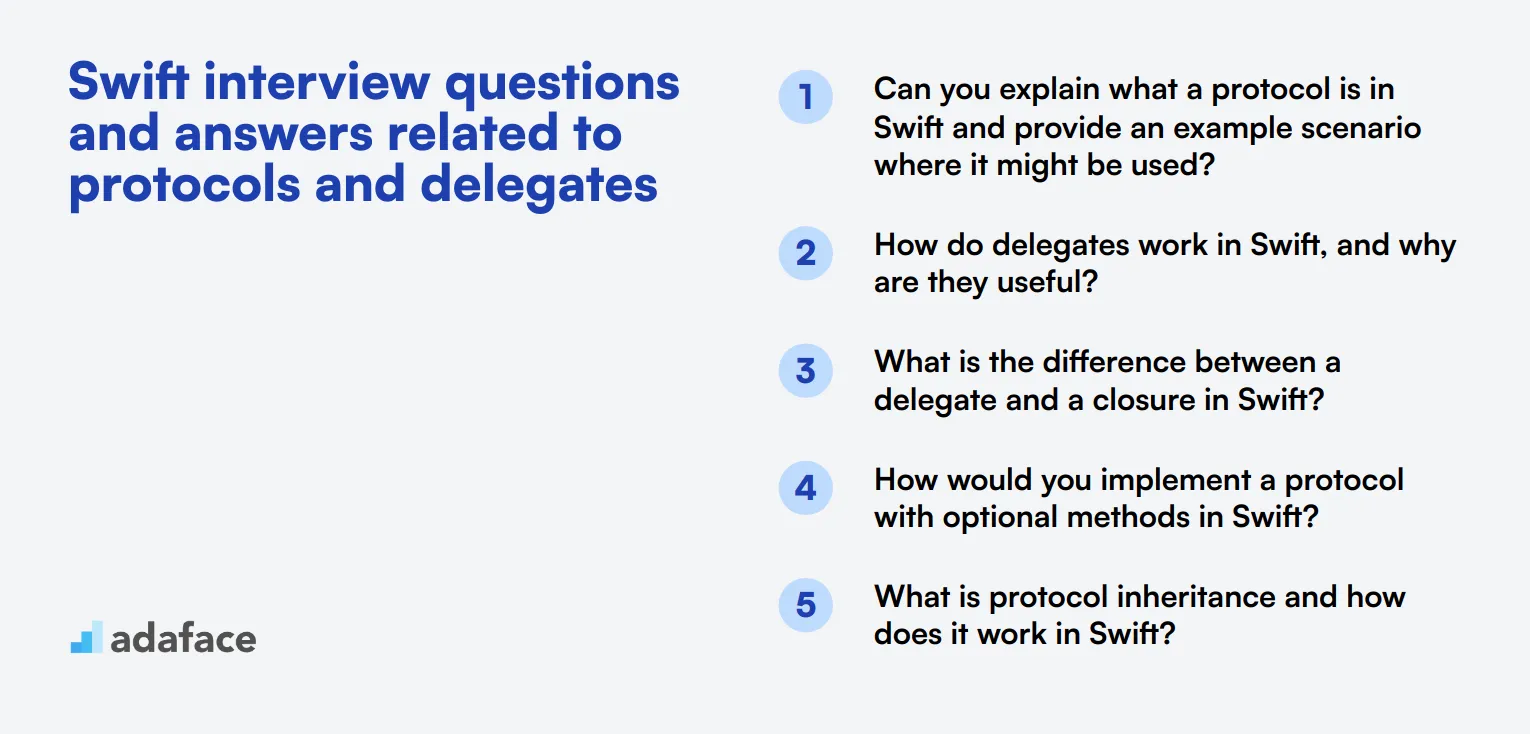
To evaluate whether candidates grasp the intricacies of protocols and delegates in Swift, dive into these essential interview questions. Use this list to identify applicants who can effectively utilize these concepts to create clean, modular code.
1. Can you explain what a protocol is in Swift and provide an example scenario where it might be used?
A protocol in Swift is a blueprint of methods, properties, and other requirements that suit a particular task or piece of functionality. Protocols can be adopted by classes, structs, and enums to provide actual implementations of these requirements.
For instance, a protocol might be used to define a common interface for different types of data sources in an app. Instead of tightly coupling your code to specific data sources, you can use protocols to interact with them in a more flexible and decoupled manner.
Look for candidates who understand the versatility of protocols and can articulate scenarios where protocols enhance code flexibility and maintainability.
2. How do delegates work in Swift, and why are they useful?
Delegates in Swift are a design pattern that allows one object to communicate with another object in a decoupled way. The delegate pattern involves defining a protocol and having another object implement that protocol. The delegating object holds a reference to the delegate, usually defined as a weak property to avoid retain cycles.
Delegates are particularly useful in scenarios where an object needs to pass information or notify another object about changes or events. For example, a table view might use a delegate to notify its controller when a user selects a row.
Candidates should demonstrate an understanding of the delegate pattern and how it helps in creating modular, reusable code. They should provide practical examples that showcase their experience with this pattern.
3. What is the difference between a delegate and a closure in Swift?
Delegates and closures are both used for passing information or actions between objects, but they do so in different ways. Delegates involve a formal protocol and a reference to an object that adopts the protocol, allowing for more structured and potentially multi-method communication.
Closures, on the other hand, are blocks of code that can be passed around and executed at a later time. They are often used for single-task callbacks and make the code more concise and easier to read for simple scenarios.
Look for candidates who can clearly articulate these differences and provide examples of when they would use one over the other. An ideal answer will highlight the structured nature of delegates versus the simplicity and flexibility of closures.
4. How would you implement a protocol with optional methods in Swift?
In Swift, protocols themselves do not support optional methods directly. However, you can achieve optional behavior by using protocol extensions to provide default implementations.
Another approach is to use the @objc
attribute and the optional
keyword, but this requires the protocol to be compatible with Objective-C and limits its use to classes.
Candidates should mention both methods and explain their considerations, such as when default implementations might be more suitable than using @objc
protocols.
5. What is protocol inheritance and how does it work in Swift?
Protocol inheritance allows one protocol to inherit the requirements of another protocol. This means a protocol can add its own requirements on top of the requirements it inherits, enabling more complex and hierarchical designs.
For example, you might have a base protocol called Vehicle
with methods like start
and stop
, and a derived protocol called ElectricVehicle
that adds methods specific to electric vehicles.
A strong response will showcase the candidate's ability to use protocol inheritance to create clean, modular, and extendable code. They should be able to discuss scenarios where this inheritance is beneficial.
6. How can protocols be used to achieve polymorphism in Swift?
Protocols enable polymorphism by allowing different types to conform to the same protocol and be treated interchangeably. This is particularly useful in scenarios where you want to write generic code that can operate on a variety of types.
For example, you can define a protocol Shape
with a method area
, and then have different shapes like Circle
and Rectangle
conform to this protocol. You can then write functions that take a Shape
parameter and operate on any shape without knowing its specific type.
Look for candidates who can discuss the benefits of using protocols to achieve polymorphism and provide clear examples that demonstrate their understanding.
7. Can you explain how protocol extensions work in Swift?
Protocol extensions in Swift allow you to provide default implementations for the methods and properties defined in a protocol. This means that conforming types can automatically inherit these implementations without having to provide their own.
Protocol extensions are powerful because they let you add functionality to many types at once and share common behavior. For instance, you might extend a protocol with utility methods that all conforming types can use.
Ideal candidates will understand the significance of protocol extensions in reducing code duplication and enhancing maintainability. They should provide examples of how they have used this feature in their projects.
8. What is the role of associated types in Swift protocols?
Associated types in Swift protocols allow you to define a placeholder type that is used as part of the protocol's definition. This makes protocols more flexible and enables them to work with generics.
For instance, you might define a protocol Container
with an associated type ItemType
. This allows various types of containers, like arrays or sets, to conform to the protocol while specifying what kind of items they hold.
Candidates should demonstrate an understanding of how associated types increase the flexibility of protocols and provide examples of scenarios where they have implemented this feature.
9. How do you conform to multiple protocols in Swift, and what are the benefits?
In Swift, a single type can conform to multiple protocols by listing them in the type's declaration. This means a class, struct, or enum can adopt multiple sets of methods and properties, making the type more versatile.
Conforming to multiple protocols allows you to create types that fulfill various roles and responsibilities, promoting code reuse and separation of concerns. For example, a ViewController
might conform to both UITableViewDelegate
and UITableViewDataSource
to handle table view behavior.
Look for candidates who can discuss the practical benefits of adopting multiple protocols and provide clear examples demonstrating their experience with this practice.
7 situational Swift interview questions with answers for hiring top developers
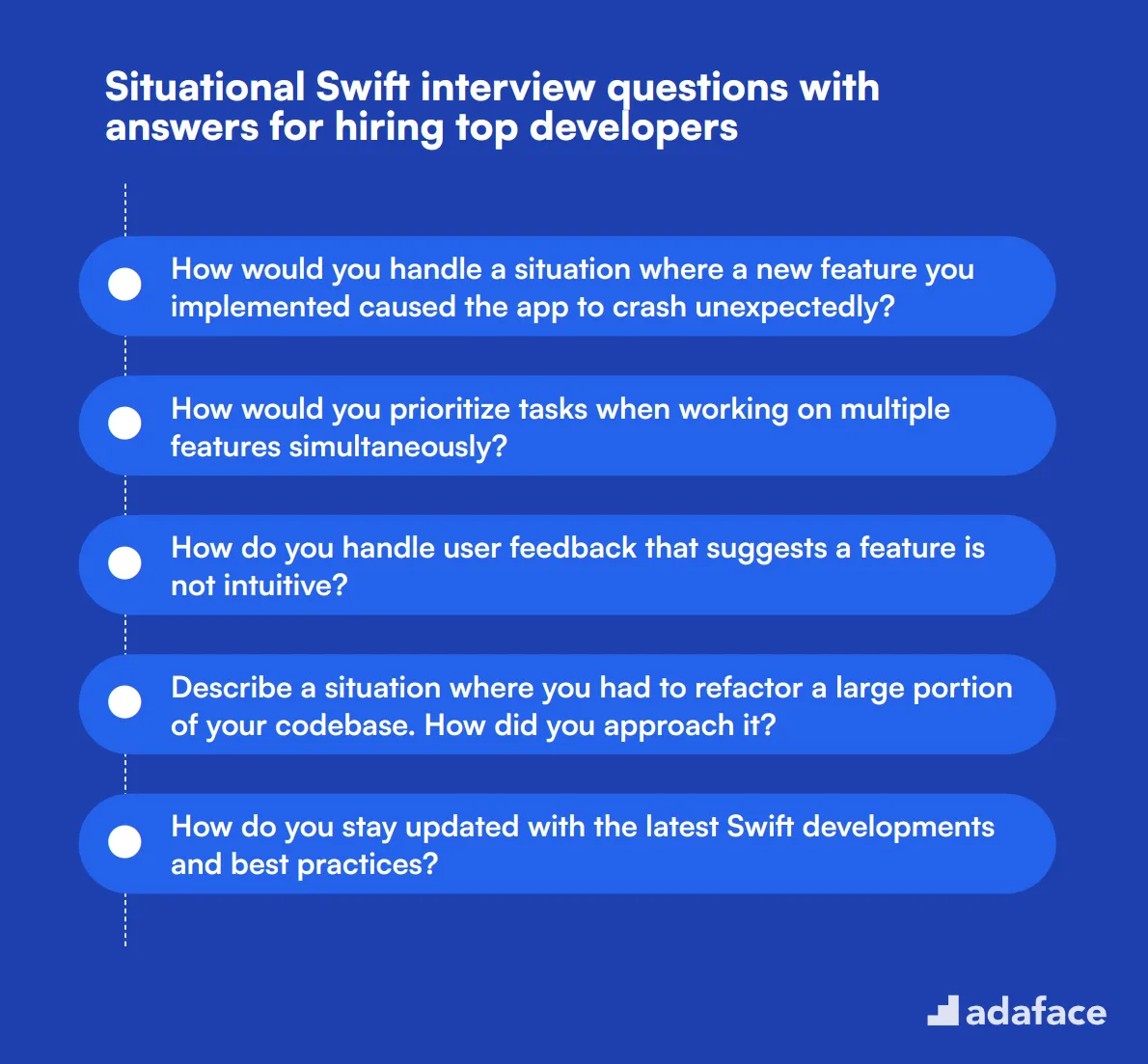
To assess if candidates can handle real-world scenarios with Swift, use these situational questions in your interviews. They offer a glimpse into how applicants think and solve problems, ensuring you find the best developers for your team.
1. How would you handle a situation where a new feature you implemented caused the app to crash unexpectedly?
An ideal candidate would start by explaining their debugging process. They might mention using tools like Xcode to trace the crash logs and identify the root cause. Once the issue is pinpointed, they'd discuss rolling back the feature or applying a quick fix while working on a long-term solution.
Look for candidates who demonstrate a systematic approach to problem-solving and an understanding of the importance of maintaining app stability. Follow up by asking how they would prevent similar issues in the future, which should include rigorous testing and code reviews.
2. How would you prioritize tasks when working on multiple features simultaneously?
Candidates should describe their approach to prioritization, which might include assessing the impact and urgency of each feature. They could mention using project management tools to keep track of deadlines and dependencies, ensuring that critical features are completed first.
An ideal answer will show the candidate’s ability to balance multiple responsibilities effectively. Look for mentions of communication with team members and stakeholders to manage expectations and ensure alignment on priorities.
3. How do you handle user feedback that suggests a feature is not intuitive?
A strong candidate would explain how they gather and analyze user feedback, possibly through analytics tools and user testing sessions. They should discuss how they use this feedback to iterate on the design and functionality to improve the user experience.
Look for a response that highlights the importance of user-centered design and continuous improvement. Candidates should demonstrate a willingness to engage with users and adapt based on their needs. Follow up by asking for examples of how they have implemented user feedback in past projects.
4. Describe a situation where you had to refactor a large portion of your codebase. How did you approach it?
Candidates might discuss the need for refactoring due to code complexity or performance issues. They should detail their approach, such as breaking the task into smaller, manageable parts, writing tests to ensure functionality before and after changes, and documenting their process.
An ideal candidate will show a strategic and methodical approach to refactoring. Look for mentions of how they ensured minimal disruption to the existing system and collaborated with team members throughout the process. Follow up with questions about specific challenges they faced and how they overcame them.
5. How do you stay updated with the latest Swift developments and best practices?
Candidates should mention resources like official documentation, Swift forums, and developer conferences. They might also discuss participating in coding communities or contributing to open-source projects to keep their skills sharp.
Look for a genuine interest in continuous learning and professional growth. A proactive approach to staying updated indicates a candidate’s commitment to delivering high-quality code and adopting industry best practices. You can subtly reference skills required for Swift developers here.
6. How do you handle performance issues in a Swift application?
Candidates should discuss their methods for identifying and diagnosing performance bottlenecks, such as using profiling tools like Instruments. They might mention optimizing code, reducing memory usage, or improving the efficiency of algorithms to enhance app performance.
Look for a structured approach to performance optimization and an understanding of common performance issues in Swift. Follow up by asking for examples of specific performance challenges they have addressed in previous projects.
7. Describe a time when you had to work with a difficult team member on a Swift project. How did you handle it?
Candidates might describe a scenario where they had to navigate conflicts or differing opinions. They should emphasize communication and collaboration, discussing how they sought to understand the team member’s perspective and worked towards a mutually beneficial solution.
An ideal candidate will demonstrate strong interpersonal skills and the ability to maintain a positive working environment. Look for evidence of conflict resolution skills and a commitment to team cohesion. Follow up by asking how the experience influenced their approach to teamwork in subsequent projects.
Which Swift skills should you evaluate during the interview phase?
In the interview process, it's important to recognize that a single conversation cannot encompass every aspect of a candidate's capabilities. However, focusing on core Swift skills can provide insight into their potential as a developer. Evaluating these key skills will help you determine if the candidate is a good fit for your team.
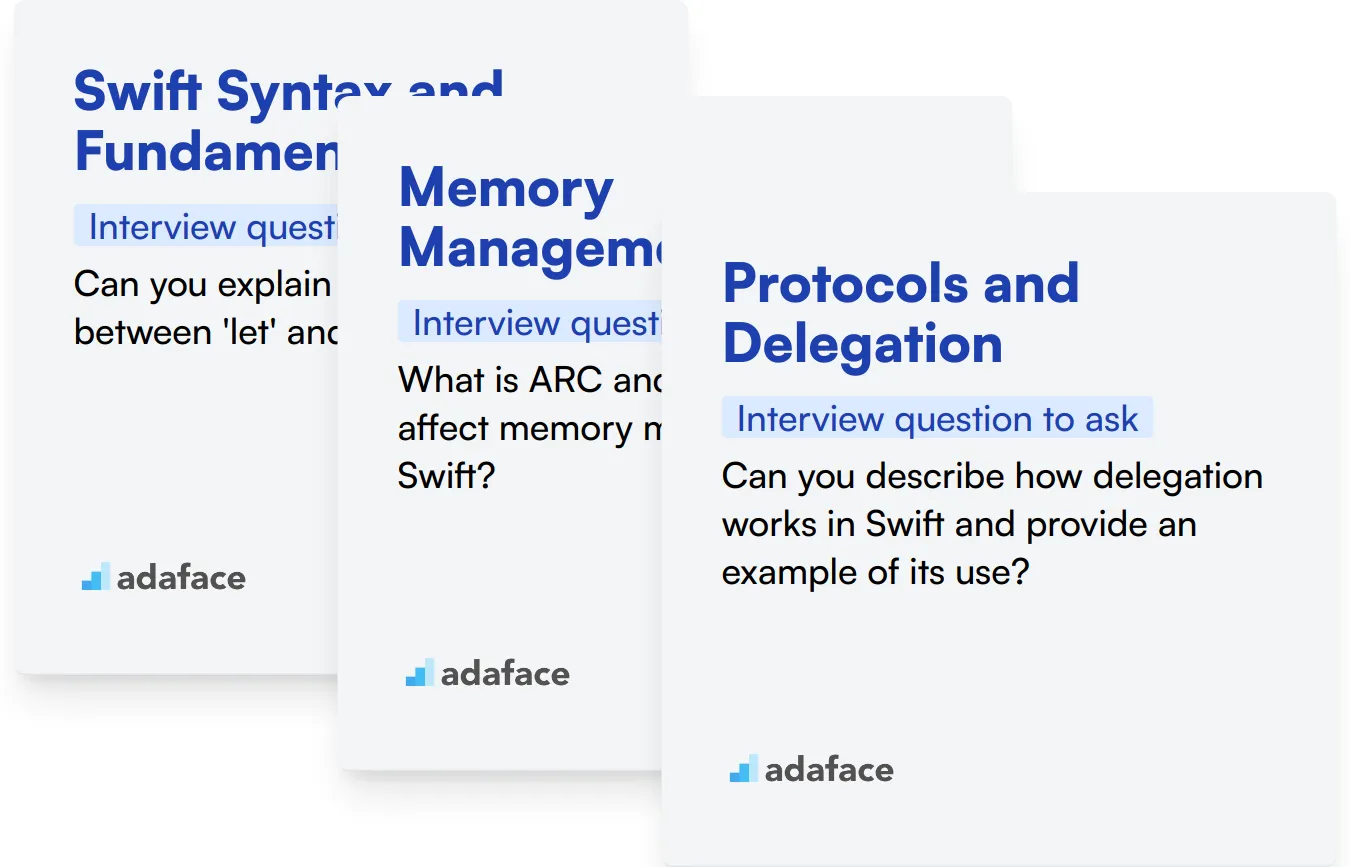
Swift Syntax and Fundamentals
To filter candidates on this skill, consider using an assessment test that includes multiple-choice questions focused on Swift syntax and fundamentals. This can quickly identify those who have a solid understanding of the language. You can explore our Swift Online Test for a structured evaluation.
Alongside testing, asking targeted interview questions can help assess a candidate's understanding of Swift syntax. One such question to consider is:
Can you explain the difference between 'let' and 'var' in Swift?
When asking this question, look for a clear explanation of the immutability associated with 'let' and how 'var' allows for mutable variables. Candidates should provide examples that illustrate their understanding of the implications of using each keyword, showcasing their familiarity with Swift's memory management.
Memory Management
To evaluate memory management knowledge, consider implementing an assessment with multiple-choice questions related to ARC and memory best practices. You might find our iOS Online Test useful for this purpose, as it includes relevant topics.
You can also ask candidates specific questions about memory management in Swift. A suitable question is:
What is ARC and how does it affect memory management in Swift?
As candidates respond, pay attention to their understanding of ARC's role in managing memory automatically, as well as its impact on performance. Look for mentions of strong and weak references and how they relate to avoiding retain cycles.
Protocols and Delegation
To assess knowledge in this area, consider including multiple-choice questions related to protocols and delegation in your evaluation process. While our current library may not have a dedicated test for this skill, structuring your own questions can be very effective.
An insightful question to gauge a candidate's understanding is:
Can you describe how delegation works in Swift and provide an example of its use?
When candidates answer, look for clarity in their explanation of how delegation allows one object to communicate with another. Strong candidates will provide examples of real-world scenarios, showcasing their ability to implement delegation in practical applications.
3 Tips for Effectively Using Swift Interview Questions
Before you put your newfound knowledge to practice, consider these tips to enhance your Swift interview process and ensure you assess candidates effectively.
1. Incorporate Skills Tests Before the Interview
Using skills tests before interviews can streamline your selection process and ensure candidates possess the required technical abilities. For Swift developers, consider using the Swift online test to evaluate their programming skills.
These tests not only save time but also provide measurable insights into a candidate's competencies. By assessing their skills upfront, you can focus your interview on discussing their strengths and areas for growth, allowing for a more meaningful conversation.
Ultimately, this approach enhances your ability to identify the right fit for your team while reducing the chances of making a bad hire.
2. Curate Relevant Interview Questions
Time is limited during interviews, so ask the right questions that target essential skills and knowledge areas. Rather than asking numerous questions, focus on a select few that allow you to assess candidates' capabilities in key areas of Swift development.
You may also want to incorporate questions from related topics, such as memory management, protocols, or problem-solving skills. For instance, you could explore questions on memory management or protocols and delegates.
Selecting relevant questions ensures a more efficient evaluation process and provides insights into the candidates' technical aptitude and interpersonal skills.
3. Utilize Follow-Up Questions
While asking initial interview questions is important, follow-up questions are necessary to gauge a candidate's depth of understanding. Candidates may provide surface-level answers that don't reveal their true capabilities or experiences.
For example, if you ask a candidate about their experience with closures in Swift, a relevant follow-up could be, 'Can you provide an example of a scenario where you used closures effectively?' This follow-up encourages candidates to elaborate and gives you a clearer picture of their practical skills.
Use Swift interview questions and skills tests to hire talented developers
If you're looking to hire someone with Swift skills, it’s important to ensure they possess the right abilities. The most accurate way to assess these skills is by using specific skill tests, such as our Swift online test.
Once you've administered the test, you can effectively shortlist the best applicants and invite them for interviews. To get started, sign up via our online assessment platform and streamline your hiring process.
Swift Online Test
Download Swift interview questions template in multiple formats
Swift Interview Questions FAQs
This post covers general, junior, intermediate, advanced, memory management, protocols and delegates, and situational Swift interview questions.
The post includes 20 Swift interview questions specifically tailored for junior developers.
Yes, the post provides 3 tips for effectively using Swift interview questions during the hiring process.
Yes, the post includes questions for junior, mid-tier, and senior developers, covering a range of experience levels.
Yes, the post includes sections on memory management, protocols, and delegates in Swift.
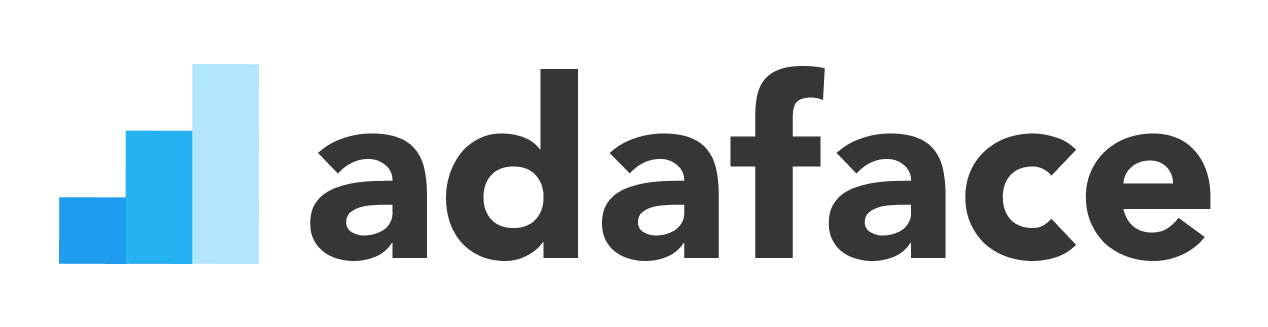
40 min skill tests.
No trick questions.
Accurate shortlisting.
We make it easy for you to find the best candidates in your pipeline with a 40 min skills test.
Try for freeRelated posts
Free resources
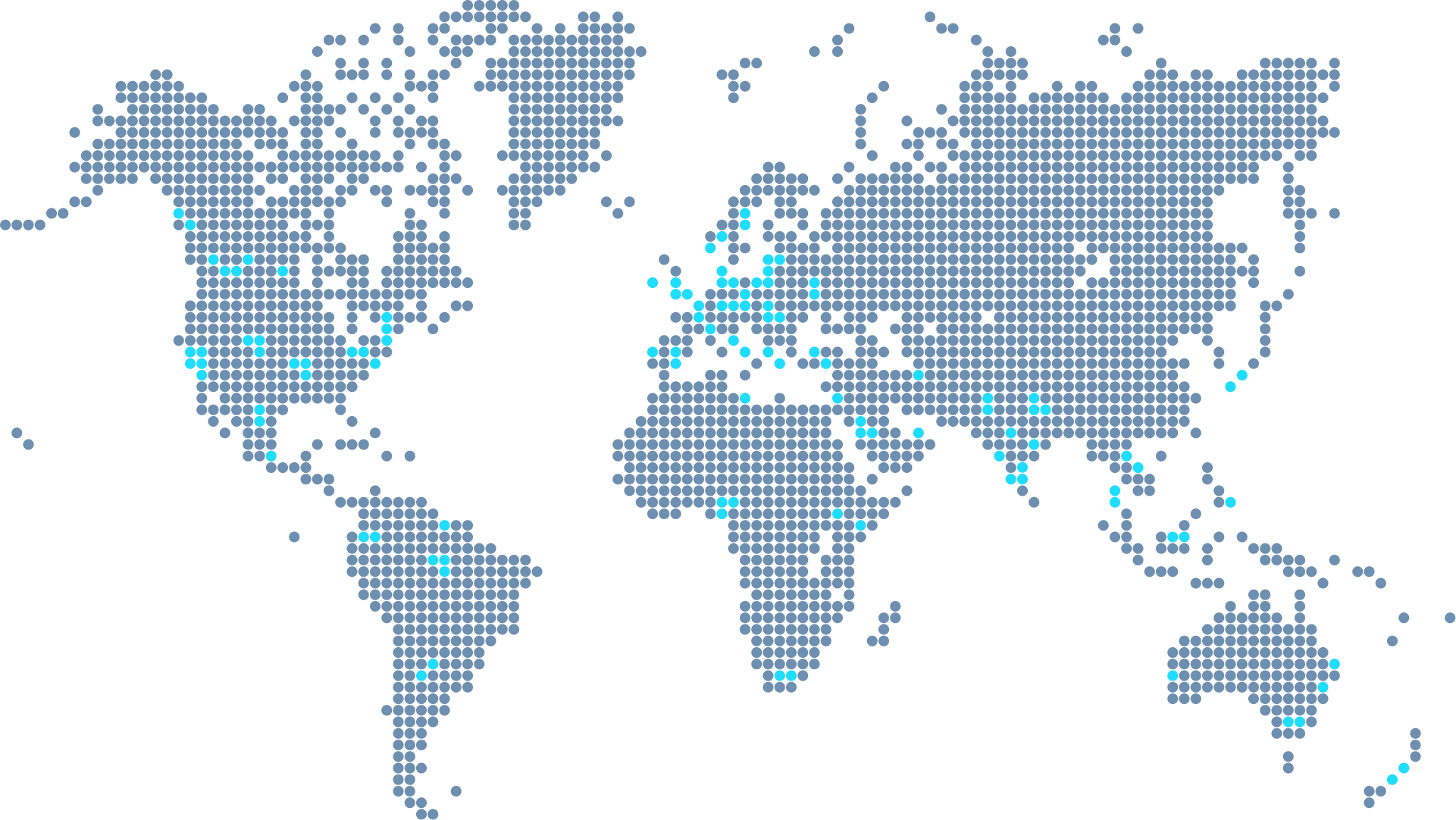
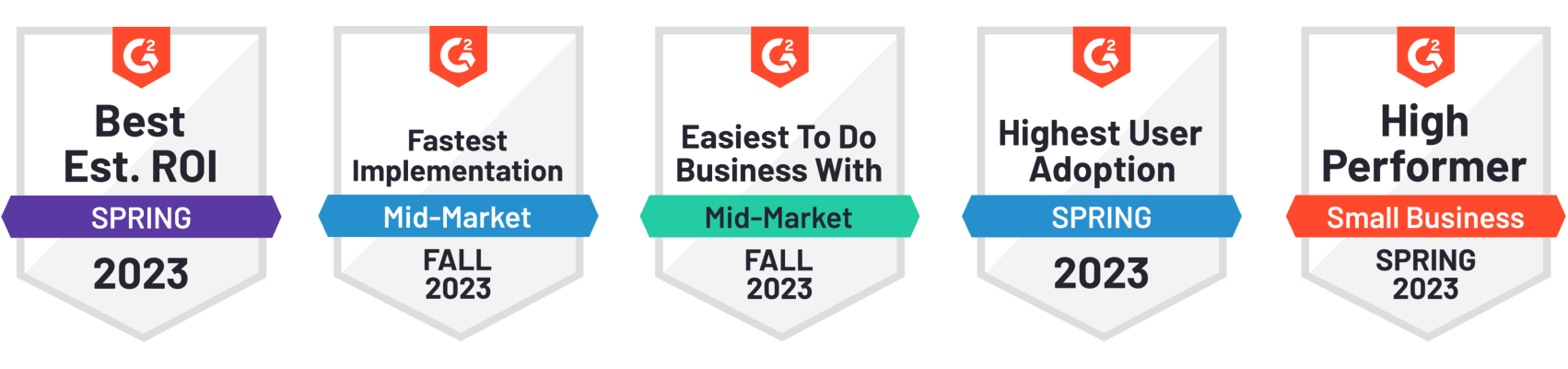