Hiring the right Spring Boot developer can be a challenge for recruiters and hiring managers. Asking the right interview questions is key to identifying candidates with the necessary skills and experience.
This blog post provides a comprehensive list of Spring Boot interview questions, categorized for different experience levels and specific areas of expertise. We cover general questions, junior developer questions, microservices-related questions, and dependency injection topics.
By using these questions, you can effectively assess candidates' Spring Boot knowledge and make informed hiring decisions. Consider complementing your interviews with a Spring Boot skills assessment to get a complete picture of candidates' abilities.
Table of contents
8 general Spring Boot interview questions and answers to assess applicants
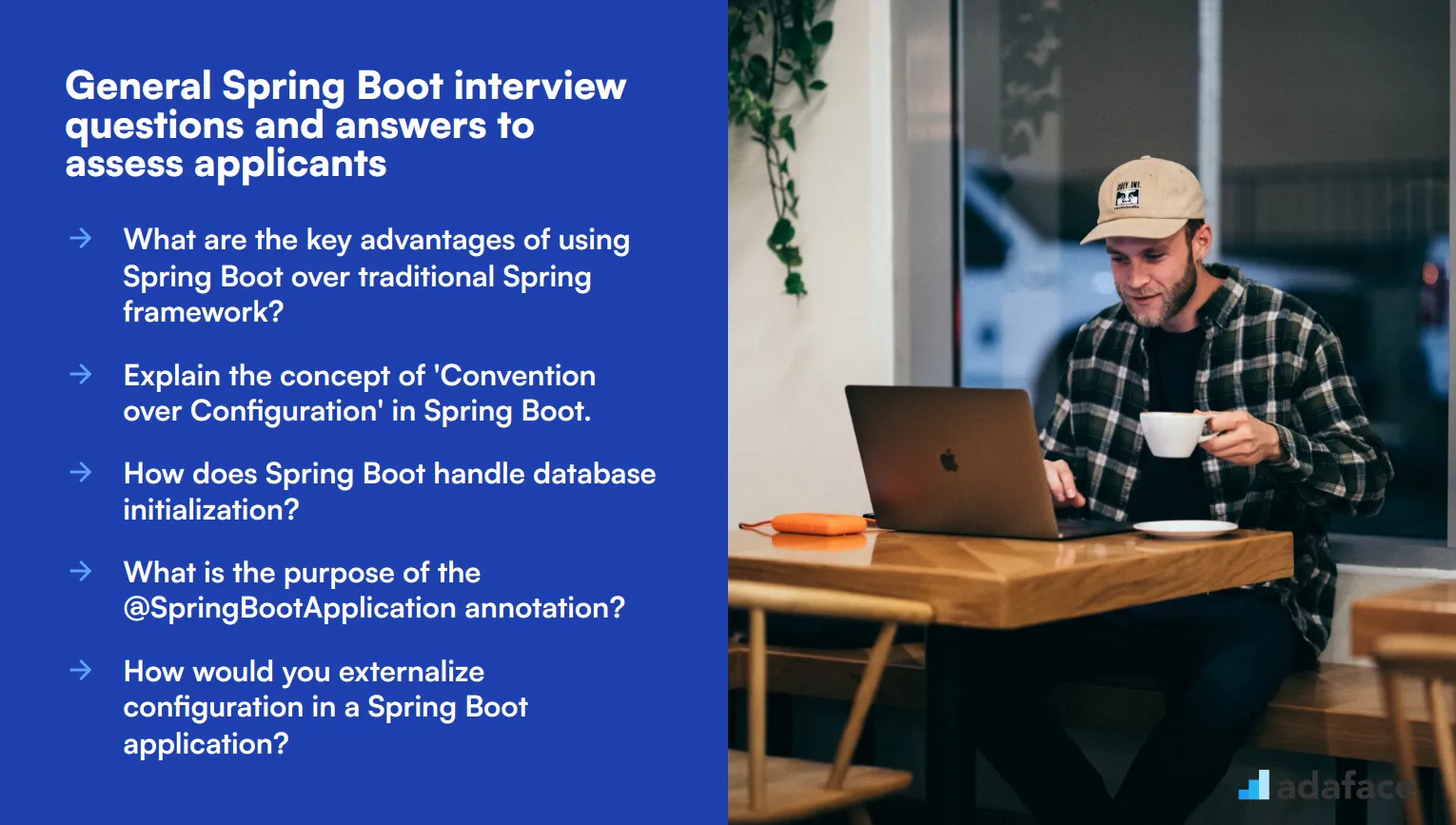
Ready to dive into the Spring Boot talent pool? These eight general interview questions will help you assess candidates' understanding of core concepts and their ability to apply them. Use this list to gauge applicants' knowledge and problem-solving skills, ensuring you find the right fit for your development team.
1. What are the key advantages of using Spring Boot over traditional Spring framework?
Spring Boot offers several advantages over the traditional Spring framework:
- Simplified configuration: Spring Boot uses auto-configuration to reduce the need for XML configurations and annotations.
- Embedded server: It comes with an embedded server, eliminating the need for external server setup.
- Starter dependencies: These pre-configured dependencies simplify the build configuration.
- Production-ready features: Spring Boot includes built-in features for metrics, health checks, and externalized configuration.
Look for candidates who can articulate these benefits and explain how they contribute to faster development and easier deployment. Strong answers may also touch on potential drawbacks or scenarios where traditional Spring might be preferred.
2. Explain the concept of 'Convention over Configuration' in Spring Boot.
'Convention over Configuration' is a software design paradigm that Spring Boot heavily relies on. It aims to decrease the number of decisions a developer has to make without losing flexibility.
In practice, this means Spring Boot:
- Provides sensible defaults for project setup and configuration
- Automatically configures application components based on the classpath
- Reduces boilerplate code and XML configuration
- Allows developers to override defaults when needed
A good candidate should be able to explain how this principle speeds up development and reduces errors. They might also discuss how it relates to the auto-configuration feature in Spring Boot.
3. How does Spring Boot handle database initialization?
Spring Boot offers multiple ways to initialize a database:
- Using schema.sql and data.sql files: Spring Boot automatically executes SQL files in the classpath.
- Hibernate's DDL auto: Properties can be set to create, update, or validate the schema.
- Spring Boot's DataSource initialization: This can be enabled or disabled using properties.
- Using database migration tools like Flyway or Liquibase, which Spring Boot supports out of the box.
Look for candidates who can explain these methods and discuss their pros and cons. They should also be able to recommend which method to use in different scenarios, such as development vs. production environments.
4. What is the purpose of the @SpringBootApplication annotation?
The @SpringBootApplication annotation is a convenience annotation that combines three other annotations:
- @Configuration: Tags the class as a source of bean definitions
- @EnableAutoConfiguration: Tells Spring Boot to start adding beans based on classpath settings, other beans, and various property settings
- @ComponentScan: Tells Spring to look for other components, configurations, and services in the current package
A strong candidate should explain that this annotation simplifies the initial setup of a Spring Boot application. They might also mention that while convenient, in larger applications it's sometimes better to use these annotations separately for more fine-grained control.
5. How would you externalize configuration in a Spring Boot application?
Spring Boot provides several ways to externalize configuration:
- Application properties file (application.properties or application.yml)
- Command-line arguments
- OS environment variables
- Cloud-based configuration (e.g., Spring Cloud Config)
- @Value annotation for injecting values into beans
- @ConfigurationProperties for binding entire classes to prefixed properties
Look for candidates who can explain these methods and discuss scenarios where each might be most appropriate. They should also be aware of the order of precedence when multiple sources define the same property.
6. Describe the role of actuator in Spring Boot applications.
Spring Boot Actuator is a sub-project of Spring Boot that adds several production-ready features to applications. Its main roles include:
- Monitoring: Provides endpoints to check application health, metrics, and more
- Management: Allows runtime modification of logging levels and viewing thread dumps
- Auditing: Enables tracking of security-related events
- HTTP tracing: Logs HTTP request-response exchanges
A good candidate should be able to explain how Actuator enhances application observability and manageability. They might also discuss security considerations when exposing Actuator endpoints and how to customize or extend its functionality.
7. How does Spring Boot simplify microservices development?
Spring Boot offers several features that simplify microservices development:
- Easy setup: Starter dependencies and auto-configuration reduce boilerplate code
- Embedded servers: Simplify deployment and testing
- Health check endpoints: Aid in service discovery and monitoring
- Externalized configuration: Supports different environments and cloud deployments
- Integration with Spring Cloud: Provides tools for service discovery, config management, and circuit breakers
Look for candidates who can explain these benefits and how they address common microservices challenges. They should also be able to discuss potential drawbacks and when a microservices architecture might not be suitable.
8. What strategies would you use to test a Spring Boot application?
Testing a Spring Boot application typically involves multiple strategies:
- Unit testing: Using JUnit or TestNG for individual components
- Integration testing: With @SpringBootTest to load the application context
- Slice testing: Using annotations like @WebMvcTest or @DataJpaTest for specific layers
- Mocking: Utilizing Mockito or EasyMock for isolating components
- API testing: With tools like RestAssured or TestRestTemplate
- Performance testing: Using tools like JMeter or Gatling
A strong candidate should be able to explain these strategies and when to apply each. They might also discuss Spring Boot's testing annotations and how they simplify the testing process. Look for awareness of test pyramid principles and the importance of balancing different types of tests.
20 Spring Boot interview questions to ask junior developers
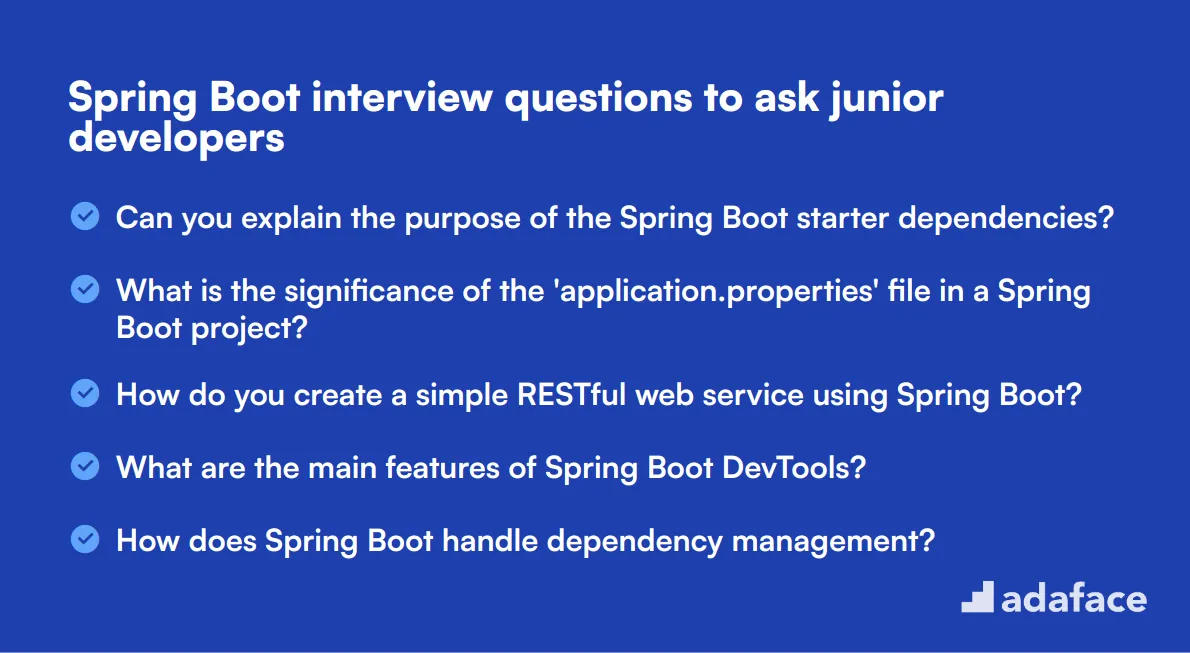
To assess whether your junior developer candidates have the foundational knowledge needed for Spring Boot applications, consider these essential interview questions. These questions are designed to provide insights into their grasp on basic concepts, ensuring they have the right skills to contribute effectively. For more information on what to look for, check out this Spring Boot developer job description.
- Can you explain the purpose of the Spring Boot starter dependencies?
- What is the significance of the 'application.properties' file in a Spring Boot project?
- How do you create a simple RESTful web service using Spring Boot?
- What are the main features of Spring Boot DevTools?
- How does Spring Boot handle dependency management?
- Describe how to configure logging in a Spring Boot application.
- What is Spring Boot CLI and what are its advantages?
- How would you enable HTTPS in a Spring Boot application?
- Explain the concept of profiles in Spring Boot and how to use them.
- Can you describe the process of creating a custom Spring Boot starter?
- What are the different ways to run a Spring Boot application?
- How do you handle exception management in Spring Boot?
- What is the role of Spring Boot annotations such as @Controller, @Service, and @Repository?
- How does Spring Boot integrate with Spring Security for authentication and authorization?
- What are the different ways to deploy a Spring Boot application?
- How do you configure a Spring Boot application to connect to an external database?
- Explain the concept of auto-configuration in Spring Boot.
- How would you implement pagination and sorting in a Spring Boot application?
- Can you describe how to use Spring Boot with JPA for data access?
- How do you handle internationalization (i18n) in a Spring Boot application?
7 Spring Boot interview questions and answers related to microservices
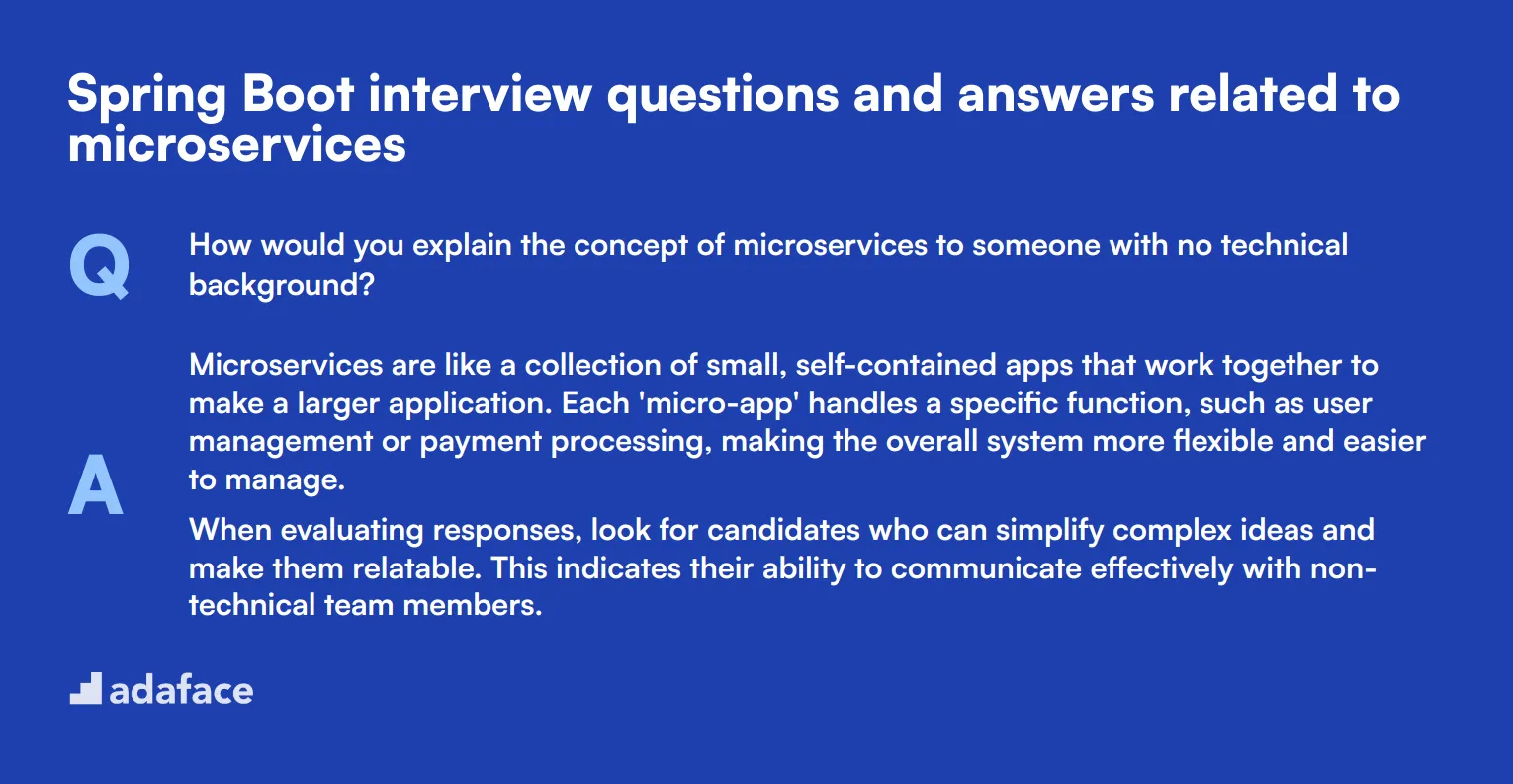
To determine if your candidates have a solid understanding of microservices with Spring Boot, use these focused interview questions. These questions are designed to help you assess their conceptual grasp and practical experience without diving too deep into the technical weeds.
1. How would you explain the concept of microservices to someone with no technical background?
Microservices are like a collection of small, self-contained apps that work together to make a larger application. Each 'micro-app' handles a specific function, such as user management or payment processing, making the overall system more flexible and easier to manage.
When evaluating responses, look for candidates who can simplify complex ideas and make them relatable. This indicates their ability to communicate effectively with non-technical team members.
2. What are some advantages of using microservices over a monolithic architecture?
Microservices offer several advantages, including improved scalability, easier maintenance, and better fault isolation. Since each service is independent, you can scale individual components as needed without affecting the entire system. It also allows for continuous deployment and integration.
Expect candidates to highlight these benefits and explain how they can lead to more efficient project management and reduced downtime.
3. How do you handle communication between microservices in a Spring Boot application?
In a Spring Boot microservices architecture, communication is typically handled using REST APIs or messaging queues. REST APIs are straightforward for request-response interactions, while messaging queues like RabbitMQ or Kafka are used for more complex, asynchronous communication.
Look for candidates who can discuss the pros and cons of each method and explain scenarios where one might be preferred over the other.
4. Can you describe a situation where implementing microservices improved a project's outcome?
A strong candidate might describe a scenario where microservices allowed for better scalability or fault tolerance. For instance, they might talk about how breaking down a large, monolithic application into separate services led to easier updates and faster deployment cycles.
Listen for specific examples and outcomes, which can provide insights into their practical experience and problem-solving skills.
5. What are some challenges you might face when implementing microservices with Spring Boot?
Common challenges include managing distributed data, ensuring consistent communication between services, and handling security across multiple endpoints. Additionally, monitoring and logging can become complex as the number of services grows.
Ideal candidates will acknowledge these challenges and offer potential solutions or strategies they've used in the past to address them.
6. How do you ensure data consistency in a microservices architecture?
Data consistency can be maintained using strategies like event sourcing and the Saga pattern. Event sourcing ensures that all changes to the application state are stored as a sequence of events, while the Saga pattern helps manage distributed transactions across multiple services.
Look for candidates who can explain these concepts clearly and discuss how they've implemented them in real-world projects.
7. What role does Spring Cloud play in a microservices architecture?
Spring Cloud provides tools for managing configuration, discovery, and communication between microservices. It offers features like Config Server for centralized configuration management, Eureka for service discovery, and Zuul for API gateway management.
A good answer will include specific features and how they contribute to making microservices more manageable and resilient.
12 Spring Boot questions related to dependency injection
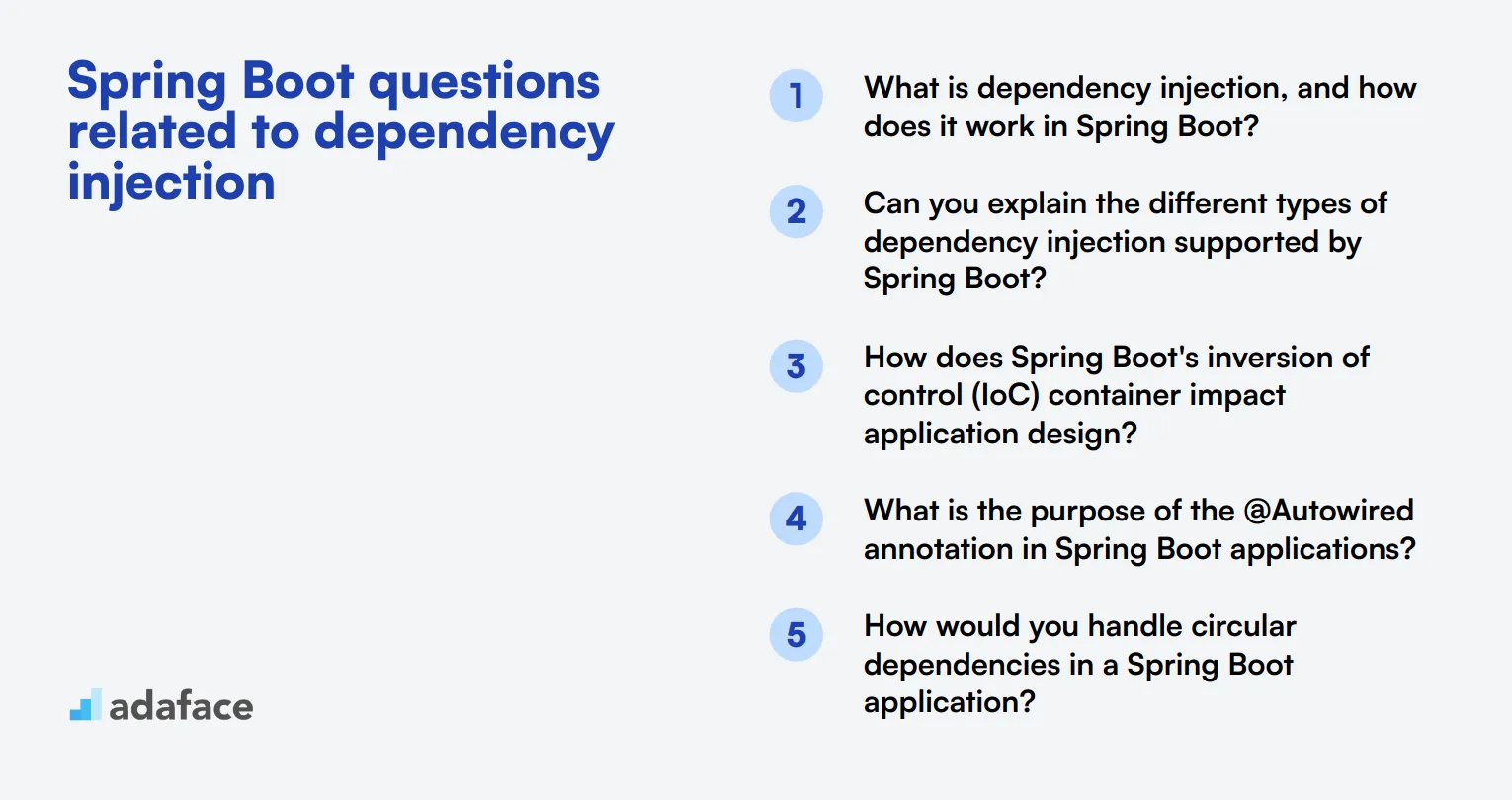
To assess candidates' understanding of dependency injection in Spring Boot, utilize these focused interview questions. They will help you gauge whether applicants possess the necessary skills to thrive in a Spring Boot development role, such as a Spring Boot Developer.
- What is dependency injection, and how does it work in Spring Boot?
- Can you explain the different types of dependency injection supported by Spring Boot?
- How does Spring Boot's inversion of control (IoC) container impact application design?
- What is the purpose of the @Autowired annotation in Spring Boot applications?
- How would you handle circular dependencies in a Spring Boot application?
- What are the advantages of using constructor-based dependency injection over setter-based injection?
- Can you describe the role of the ApplicationContext in Spring Boot?
- What is the difference between prototype and singleton scopes in the context of Spring Boot beans?
- How can you create a custom bean and manage its lifecycle in Spring Boot?
- What are qualifiers, and how do you use them in Spring Boot for dependency injection?
- How does Spring Boot facilitate testing with mock dependencies using annotations?
- Can you explain how to use profiles for defining different beans in Spring Boot?
Which Spring Boot skills should you evaluate during the interview phase?
Conducting a single interview to assess a candidate's Spring Boot skills is challenging. However, by focusing on core skills, you can gain valuable insights into their proficiency and fit for your team. Here are the essential Spring Boot skills to evaluate during the interview phase.
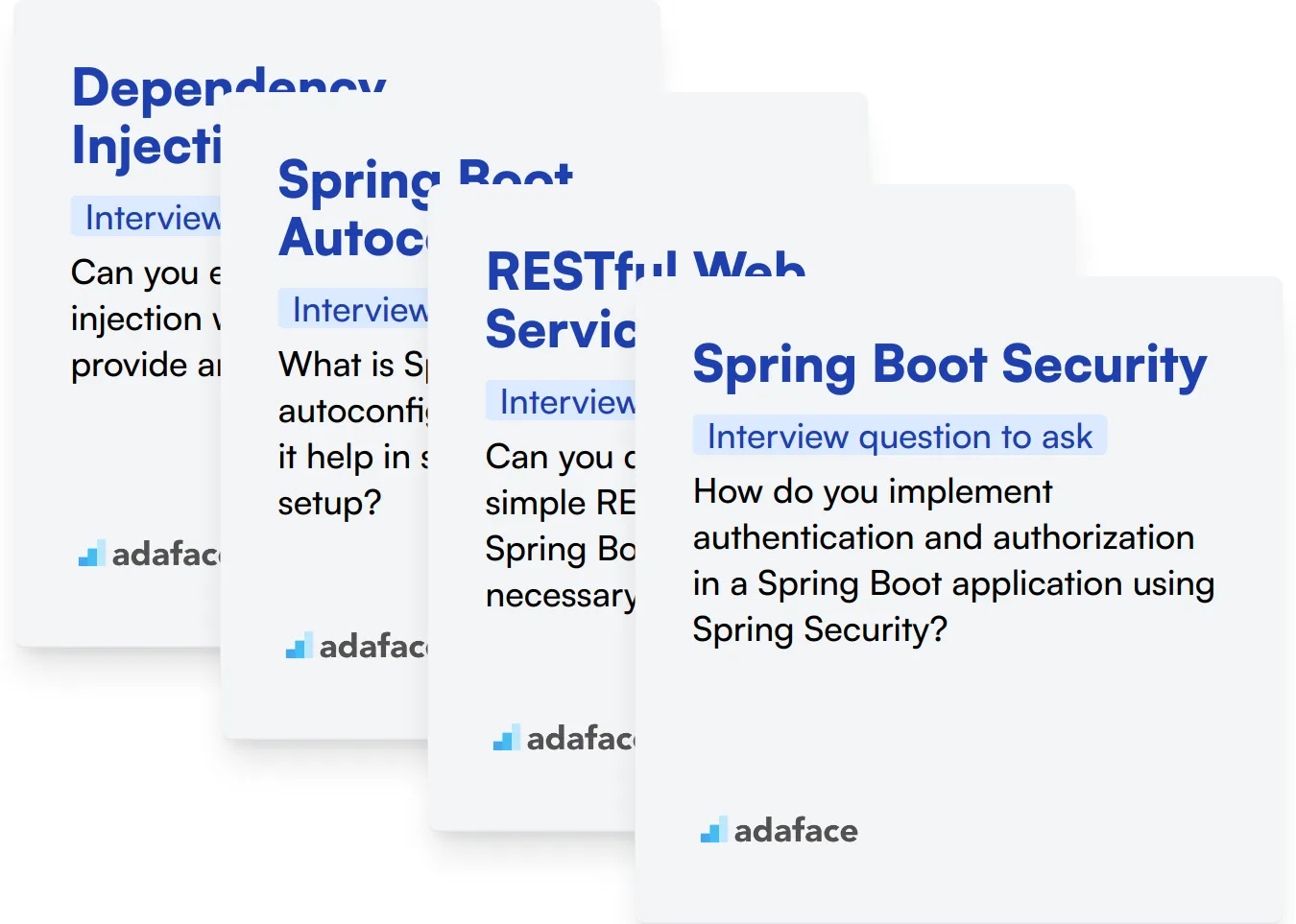
Dependency Injection
Dependency Injection (DI) is a key feature of the Spring framework, enabling developers to create loosely coupled, testable, and maintainable code. Understanding DI is crucial for leveraging Spring Boot's capabilities effectively.
You can use an assessment test that includes relevant MCQs to gauge a candidate's understanding of dependency injection concepts. Our Spring Boot test covers these topics comprehensively.
During the interview, ask targeted questions to assess their practical understanding of dependency injection.
Can you explain how dependency injection works in Spring Boot and provide an example?
Look for clear explanations of DI principles and an ability to provide practical examples using Spring Boot. Assess their understanding of different types of DI (constructor, setter, field injection) and the use of Spring annotations.
Spring Boot Autoconfiguration
Autoconfiguration is a fundamental feature that reduces the need for explicit configuration in Spring Boot applications. It automates the setup process, making it easier for developers to get started quickly.
Using our Spring Boot test that includes MCQs on autoconfiguration can help identify candidates who are familiar with this feature.
Ask specific questions to determine their understanding of Spring Boot's autoconfiguration capabilities.
What is Spring Boot autoconfiguration, and how does it help in simplifying application setup?
Look for responses that explain the purpose of autoconfiguration, how it works, and its impact on reducing boilerplate code. Candidates should mention Spring Boot's ability to automatically configure beans based on classpath settings, other beans, and various property settings.
RESTful Web Services
Creating RESTful web services is a common use case for Spring Boot. Understanding how to build and consume REST APIs is essential for developing scalable and maintainable applications.
You can use an assessment test with MCQs focusing on RESTful web services to evaluate this skill. Our Spring Boot test includes questions on REST API development.
Ask questions that focus on their experience and knowledge in developing RESTful APIs using Spring Boot.
Can you describe how to create a simple RESTful web service in Spring Boot, including any necessary annotations?
Listen for a step-by-step explanation of creating RESTful endpoints, including the use of annotations like @RestController, @RequestMapping, and @PathVariable. The candidate should demonstrate a clear understanding of HTTP methods (GET, POST, PUT, DELETE) and how to handle different request/response types.
Spring Boot Security
Security is a critical aspect of any application. Spring Boot Security provides robust authentication and authorization mechanisms to protect applications from common security threats.
An assessment test with MCQs on Spring Boot Security can help you identify candidates who are knowledgeable in this area. Our Spring Boot test includes relevant questions.
To evaluate their expertise in securing Spring Boot applications, ask targeted questions about Spring Boot Security.
How do you implement authentication and authorization in a Spring Boot application using Spring Security?
Expect a detailed explanation of setting up Spring Security, including configuring security filters, user roles, and permissions. The candidate should mention key concepts like the SecurityConfig class, @EnableWebSecurity annotation, and methods to secure REST endpoints.
Effective Strategies for Utilizing Spring Boot Interview Questions
Before you begin implementing the insights from this guide, here are a few strategic tips to ensure you maximize the impact of your Spring Boot interview questions.
1. Incorporate Skill Tests Early in the Hiring Process
Introducing skill assessments prior to the interview phase helps in filtering candidates effectively based on their technical abilities. This step ensures that only those with proven competencies in Spring Boot and related technologies advance to the interview stage, optimizing your recruitment process.
For a role requiring Spring Boot proficiency, consider utilizing Adaface's Spring Test or the Java Spring Test. These targeted assessments provide a hands-on evaluation of each candidate’s skills, giving you a clear picture of their capabilities.
The benefits of utilizing these pre-interview assessments include saving time during the interview process and increasing the likelihood of finding the best fit for the role. This strategic filtering step ensures that your interviews are more focused and productive, allowing deeper exploration into each candidate's expertise and fit for your team.
2. Strategically Select and Compile Interview Questions
Considering the limited time in interviews, it's important to strategically select questions that cover essential aspects of Spring Boot. Focus on questions that assess critical thinking and problem-solving skills in real-world scenarios, thus providing insight into the candidate’s practical knowledge and approach.
Explore a variety of questions by incorporating different areas related to Java and Spring Boot. For instance, questions from the Hibernate Test or Java SQL Test can complement your interviews by covering related technologies that are often used in conjunction with Spring Boot.
This approach helps in evaluating candidates on different fronts, ensuring a comprehensive assessment of their capabilities and how they handle complex software development challenges.
3. Emphasize the Importance of Follow-Up Questions
Using prepared interview questions is a good starting point, but the true depth of a candidate's knowledge and suitability for the role often comes through in follow-up questions. These questions help in uncovering more about the candidate’s thought process and adaptability.
For example, if a candidate mentions experience in optimizing Spring Boot applications, a good follow-up could be, 'Can you explain a specific scenario where you improved performance? What challenges did you face and how did you overcome them?' This allows you to gauge the practical implications of their skills and their problem-solving approach.
Evaluate Spring Boot skills with targeted assessments and interviews
To hire developers with Spring Boot skills, you need to assess their abilities accurately. A quick and effective way to do this is by using skill tests. Consider using our Spring Boot test or Java Spring test to gauge candidates' proficiency.
After using these tests to shortlist top applicants, you can invite them for interviews. To streamline your hiring process and find the best talent, explore our online assessment platform. It offers a range of tools to help you make informed hiring decisions for Spring Boot developers.
Spring Online Test
Download Spring Boot interview questions template in multiple formats
Spring Boot Interview Questions FAQs
Focus on general Spring Boot concepts, junior-level knowledge, microservices implementation, and dependency injection understanding.
Ask about specific projects they've worked on, challenges faced, and solutions implemented using Spring Boot.
Microservices are a key aspect of modern Spring Boot applications, so understanding a candidate's knowledge in this area is valuable.
For juniors, focus on basic concepts and simple implementations. For seniors, delve into complex scenarios and architectural decisions.
Yes, practical coding exercises can help assess a candidate's ability to apply Spring Boot concepts in real-world scenarios.
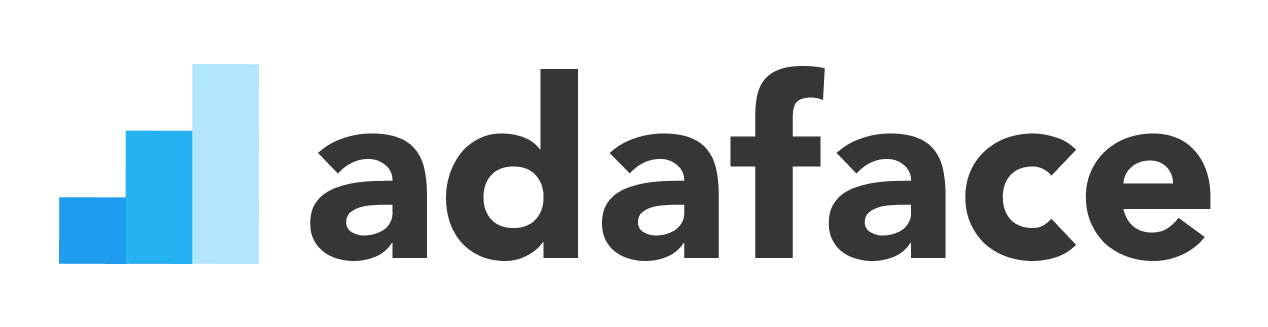
40 min skill tests.
No trick questions.
Accurate shortlisting.
We make it easy for you to find the best candidates in your pipeline with a 40 min skills test.
Try for freeRelated posts
Free resources
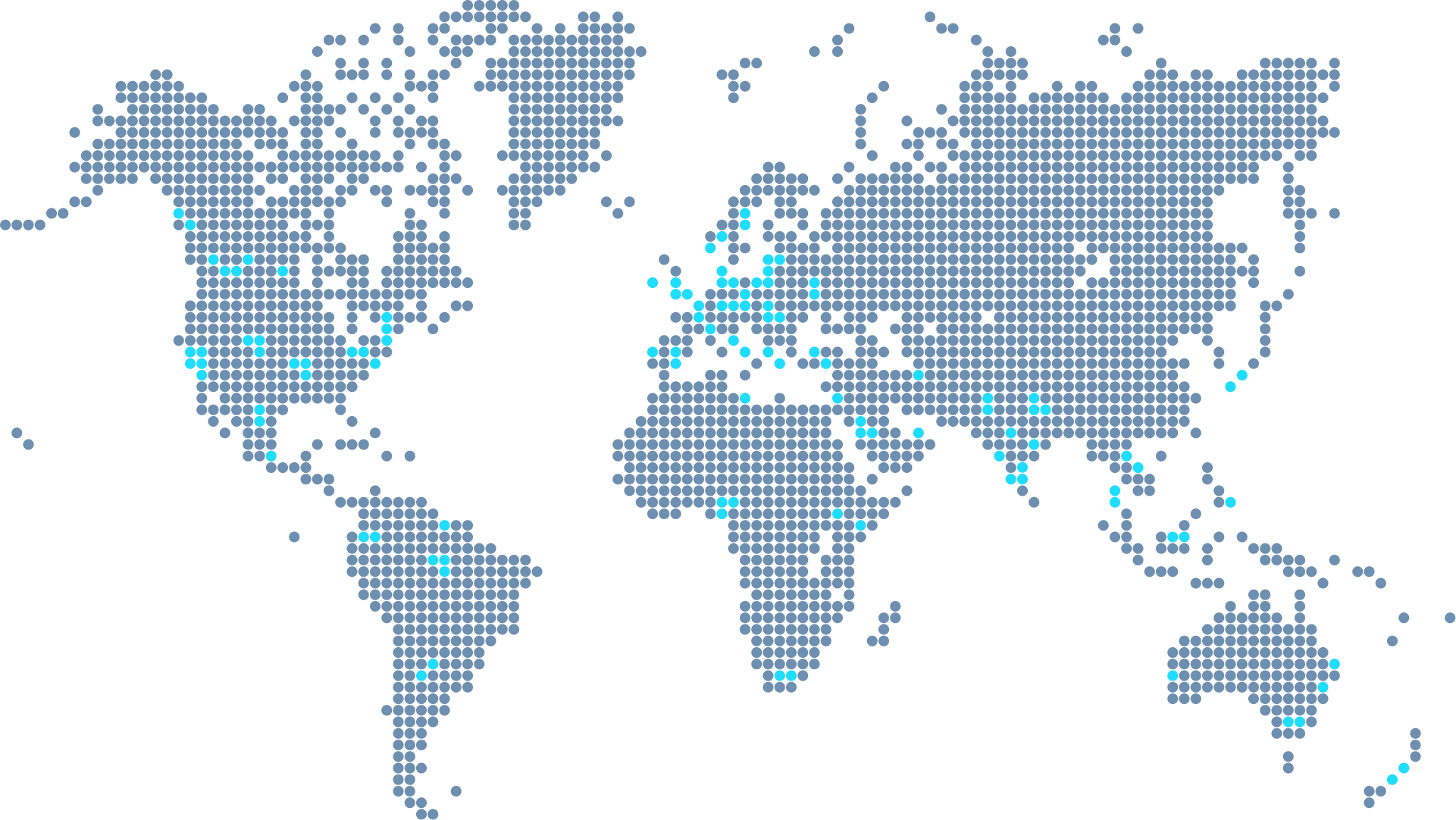
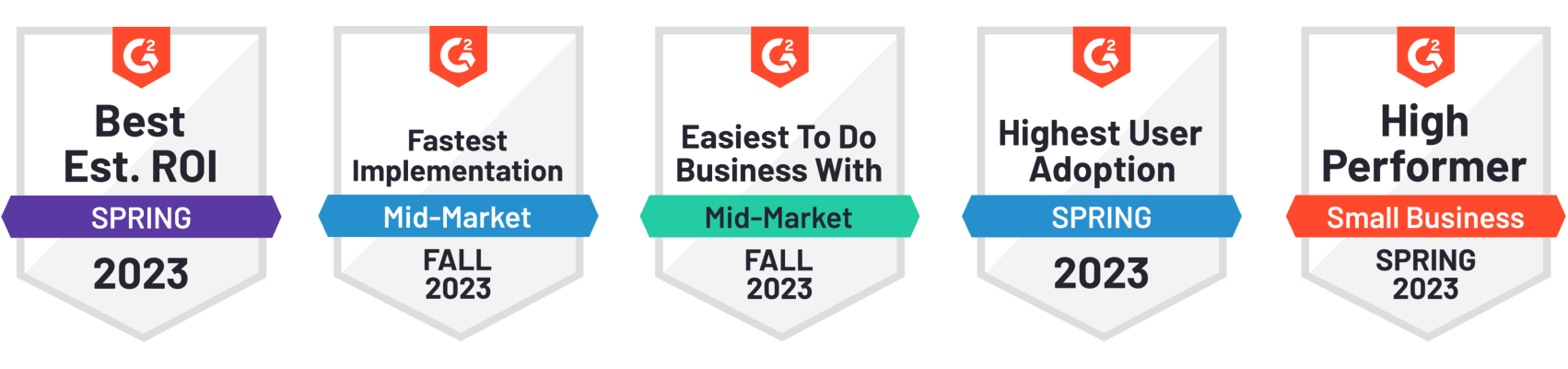