Ruby on Rails is a popular web development framework, and finding the right talent can be challenging. Asking the right interview questions is crucial to identify candidates with the necessary skills and experience.
This blog post provides a comprehensive list of Ruby on Rails interview questions and answers for different experience levels. From basic concepts to advanced topics, we cover questions related to processes, technical knowledge, and real-world scenarios.
Use these questions to effectively evaluate Ruby on Rails developers and make informed hiring decisions. Consider using a pre-employment assessment to shortlist candidates before conducting in-depth interviews.
Table of contents
18 basic Ruby on Rails interview questions and answers to assess candidates
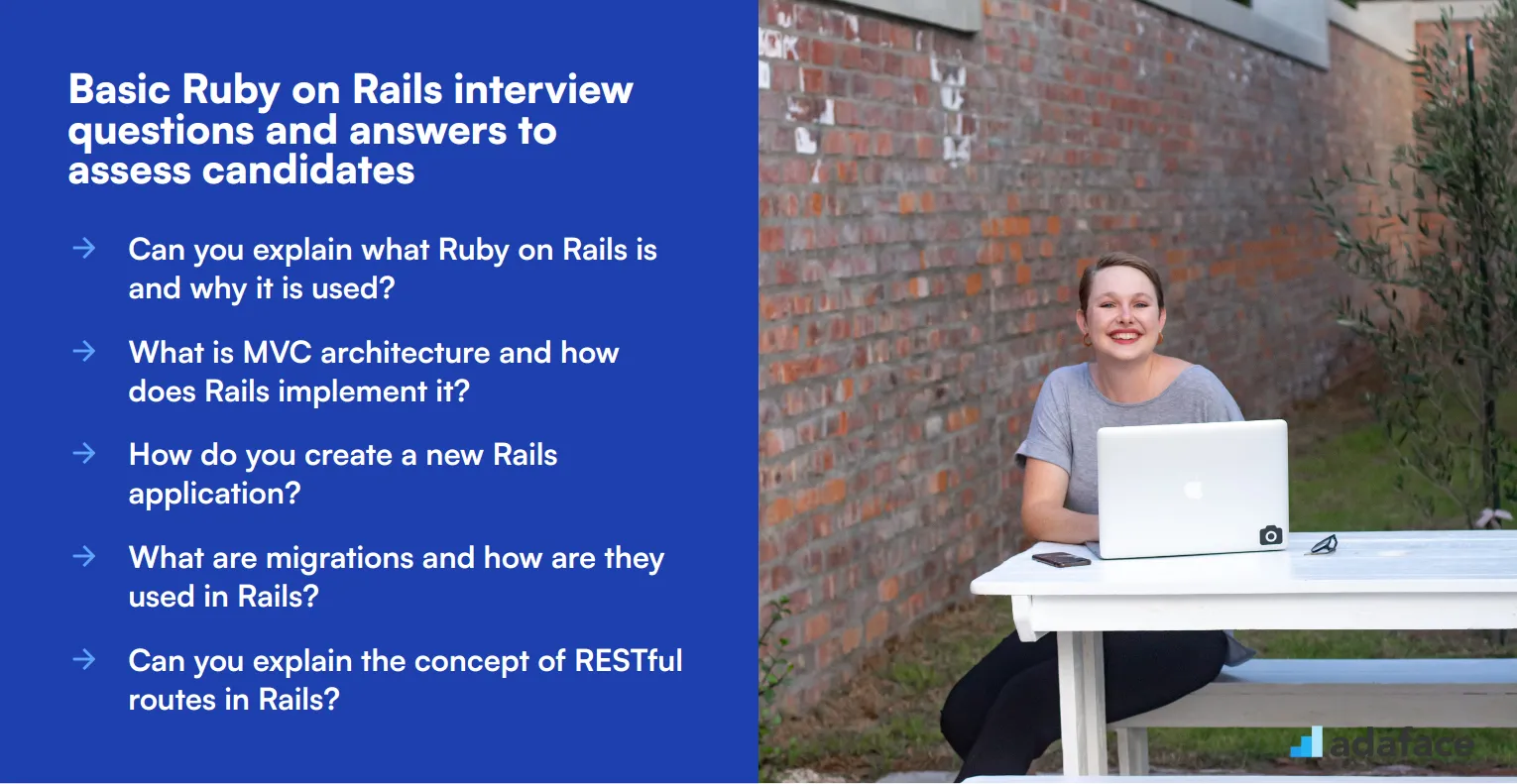
To determine whether your applicants have the right skills for a Ruby on Rails role, ask them some of these 18 basic interview questions. Use this list to assess their understanding of key concepts and their ability to apply them effectively in Ruby on Rails Developer positions.
- Can you explain what Ruby on Rails is and why it is used?
- What is MVC architecture and how does Rails implement it?
- How do you create a new Rails application?
- What are migrations and how are they used in Rails?
- Can you explain the concept of RESTful routes in Rails?
- What is Active Record and how does it work?
- How do you handle validations in Rails models?
- Can you describe the Rails asset pipeline?
- What are the main differences between `render` and `redirect_to` in Rails controllers?
- How do you manage dependencies in a Rails application?
- What is a partial and how do you use it in Rails?
- Can you explain the purpose and use of helper methods in Rails?
- How do you debug a Rails application?
- What is the difference between `find` and `find_by` methods in Active Record?
- Can you explain the Rails callback system?
- How do you handle errors and exceptions in Rails?
- What is the significance of the `Gemfile` in a Rails project?
- How do you implement authentication in a Rails application?
6 Ruby on Rails interview questions and answers to evaluate junior developers
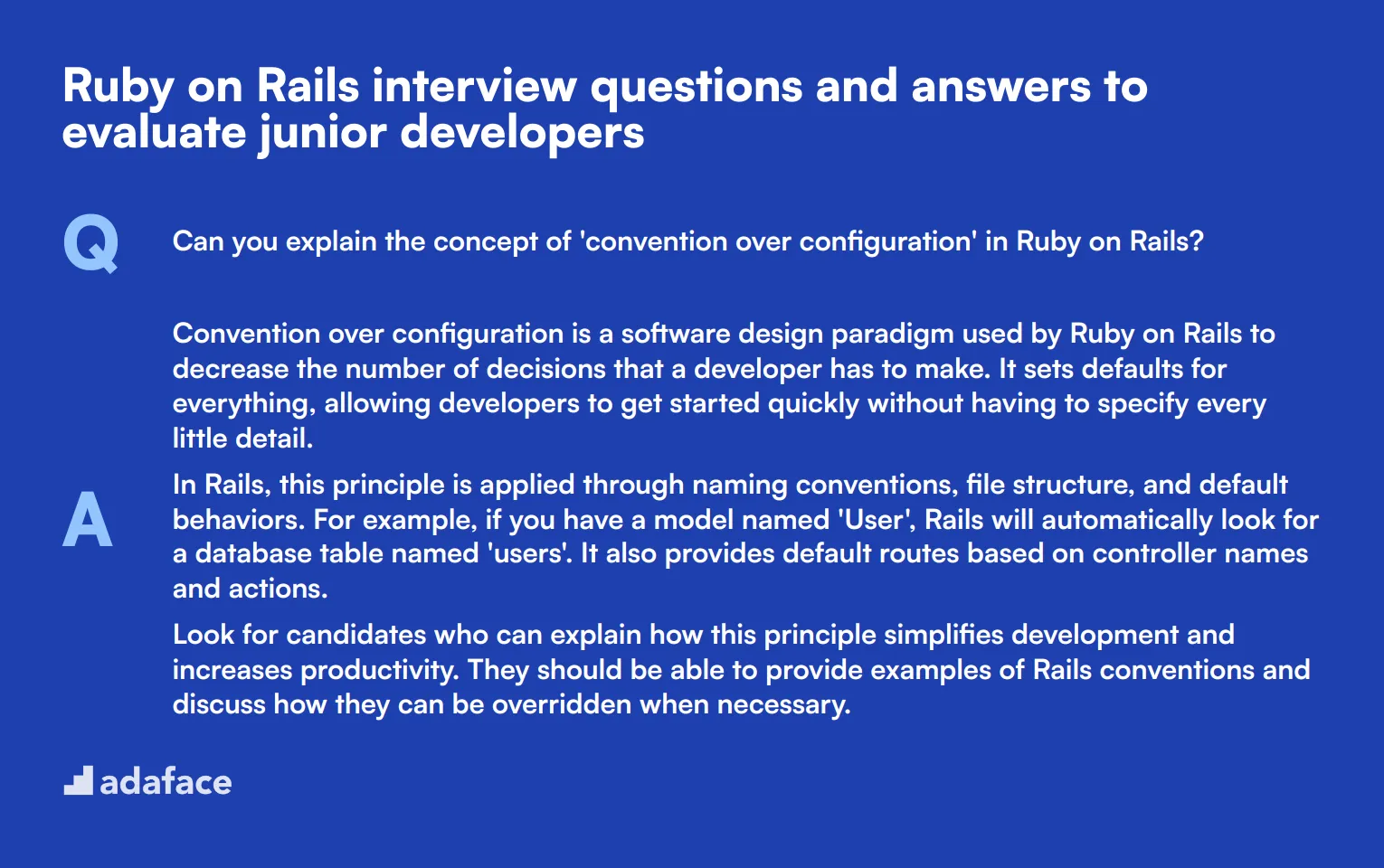
Ready to evaluate junior Ruby on Rails developers? This curated list of interview questions will help you assess candidates' fundamental understanding and practical knowledge. Use these questions to gauge their grasp of Rails concepts and their ability to apply them in real-world scenarios. Remember, the goal is to find developers who can hit the ground running and contribute to your team's success.
1. Can you explain the concept of 'convention over configuration' in Ruby on Rails?
Convention over configuration is a software design paradigm used by Ruby on Rails to decrease the number of decisions that a developer has to make. It sets defaults for everything, allowing developers to get started quickly without having to specify every little detail.
In Rails, this principle is applied through naming conventions, file structure, and default behaviors. For example, if you have a model named 'User', Rails will automatically look for a database table named 'users'. It also provides default routes based on controller names and actions.
Look for candidates who can explain how this principle simplifies development and increases productivity. They should be able to provide examples of Rails conventions and discuss how they can be overridden when necessary.
2. How would you implement caching in a Rails application?
Caching in Rails can be implemented at various levels to improve application performance. Some common caching strategies include:
- Page caching: Storing the entire HTML output of a page.
- Action caching: Similar to page caching but runs before filters.
- Fragment caching: Caching a fragment of a view, useful for dynamic pages.
- Low-level caching: Using Rails.cache to store arbitrary data.
- Russian Doll caching: Nesting cached fragments within each other.
A strong candidate should be able to explain these caching types and discuss when to use each. They should also mention cache expiration strategies and potential challenges in maintaining cache coherency. Look for full stack developers who understand the importance of performance optimization in web applications.
3. What are concerns in Rails and how do you use them?
Concerns in Rails are a way to extract common code from models, controllers, or any Ruby classes and share it across multiple classes. They are essentially modules that extend ActiveSupport::Concern and allow you to define methods, validations, callbacks, and other behavior that can be mixed into multiple classes.
To use a concern, you define it in the app/models/concerns or app/controllers/concerns directory (or any subdirectory of app that ends with concerns). Then, you can include the concern in any class using the 'include' keyword.
Look for candidates who can explain how concerns help in keeping code DRY (Don't Repeat Yourself) and maintainable. They should be able to give examples of when to use concerns and how they differ from regular modules. A good follow-up question might be to ask about potential drawbacks or when not to use concerns.
4. How do you handle file uploads in a Rails application?
File uploads in Rails can be handled using built-in functionality or third-party gems. The most common approaches include:
Using ActiveStorage (built into Rails 5.2+):
- Configures storage services (local, Amazon S3, Google Cloud Storage, etc.)
- Provides a simple way to attach files to Active Record objects
- Offers on-the-fly image transformations
Using third-party gems like CarrierWave or Paperclip:
- Provide more customization options
- Offer additional features like image processing
A strong candidate should be able to explain the basics of setting up file uploads, discuss security considerations (like validating file types and sizes), and mention how to handle file storage in different environments. Look for developers who understand the trade-offs between different approaches and can explain when to use each.
5. Explain the concept of polymorphic associations in Rails.
Polymorphic associations in Rails allow a model to belong to more than one other model on a single association. This is useful when you have a model that can be associated with multiple other models.
For example, you might have a Comment model that can belong to either a Post or an Image. Instead of creating separate associations for each (comments_on_posts, comments_on_images), you can use a polymorphic association to create a single 'commentable' association.
Look for candidates who can explain how to set up polymorphic associations, including the necessary database columns (typically a type column alongside the id column) and the model declarations. They should also be able to discuss scenarios where polymorphic associations are beneficial and potential drawbacks, such as increased complexity in querying.
6. How would you optimize a slow Rails application?
Optimizing a slow Rails application involves identifying and addressing performance bottlenecks. Some common optimization techniques include:
- Database optimization: Adding indexes, optimizing queries, using eager loading (includes, preload, joins)
- Caching: Implementing various caching strategies (page, fragment, Russian doll caching)
- Background jobs: Moving time-consuming tasks to background processes
- Code optimization: Refactoring inefficient code, using faster algorithms
- Server-side improvements: Upgrading hardware, using a faster web server, load balancing
A strong candidate should be able to discuss various performance monitoring tools (like New Relic, Scout, or Rails' built-in instrumentation) and explain how to identify performance issues. They should also understand the importance of measuring performance before and after optimizations to ensure improvements. Look for developers who take a systematic approach to optimization and understand that premature optimization can lead to unnecessary complexity.
14 Ruby on Rails questions related to processes and tasks
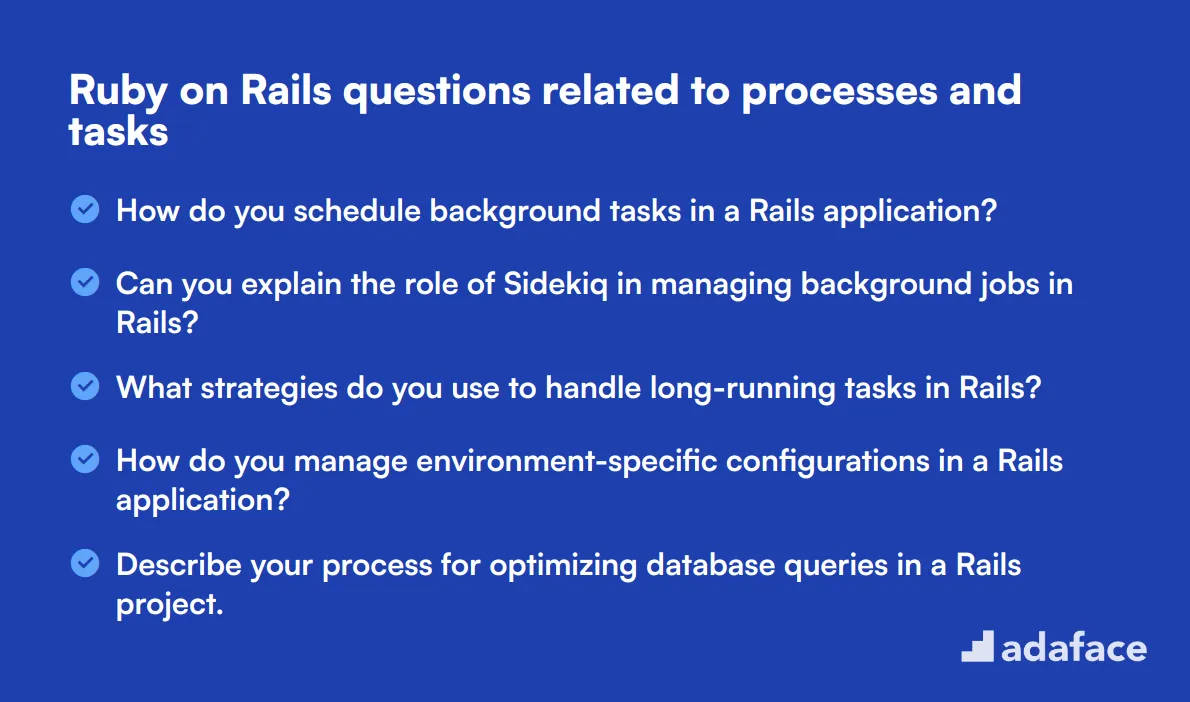
To evaluate whether your candidates possess the necessary capabilities to handle processes and tasks within a Ruby on Rails environment, refer to these 14 Ruby on Rails interview questions. These questions will help you discern their practical knowledge and problem-solving skills, ensuring you select the right fit for your Ruby on Rails developer job description.
- How do you schedule background tasks in a Rails application?
- Can you explain the role of Sidekiq in managing background jobs in Rails?
- What strategies do you use to handle long-running tasks in Rails?
- How do you manage environment-specific configurations in a Rails application?
- Describe your process for optimizing database queries in a Rails project.
- How do you handle data seeding in Rails?
- What is the role of Active Job in Rails, and how do you use it?
- How do you handle concurrent processing in a Rails application?
- Can you describe the process of setting up and using Rake tasks in Rails?
- What steps do you take to ensure your Rails application is scalable?
- How do you handle multi-tenant applications in Rails?
- Can you explain how you would implement a search feature in a Rails application?
- What methods do you use to maintain code quality in a Rails project?
- How do you handle time zones and localization in a Rails application?
6 Ruby on Rails interview questions and answers related to technical knowledge and definitions
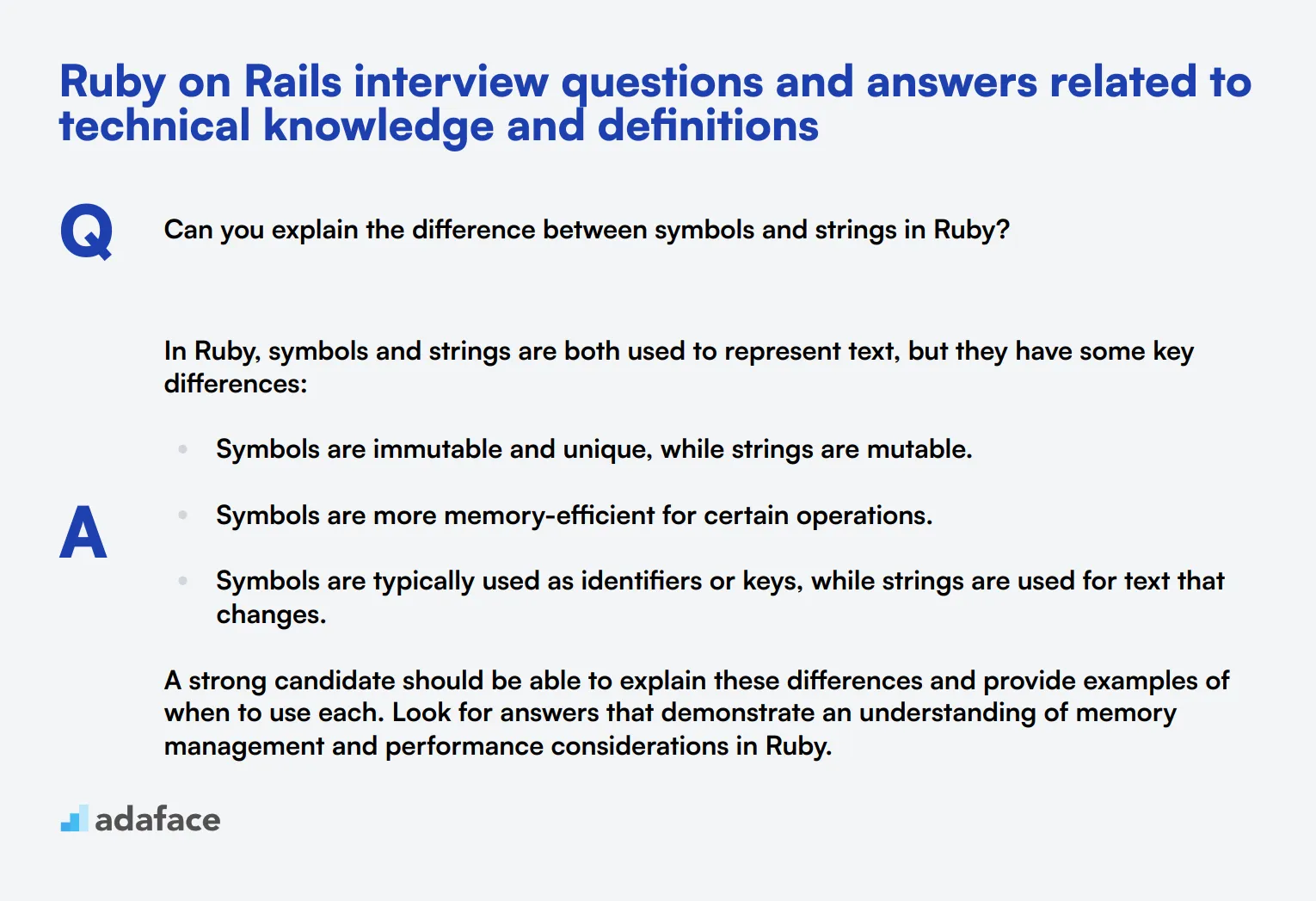
Ready to dive into the world of Ruby on Rails? These technical questions will help you assess a candidate's knowledge of key concepts and definitions. Use them to gauge whether your applicants have the right skills required for the job. Remember, the best answers will demonstrate both theoretical understanding and practical application.
1. Can you explain the difference between symbols and strings in Ruby?
In Ruby, symbols and strings are both used to represent text, but they have some key differences:
- Symbols are immutable and unique, while strings are mutable.
- Symbols are more memory-efficient for certain operations.
- Symbols are typically used as identifiers or keys, while strings are used for text that changes.
A strong candidate should be able to explain these differences and provide examples of when to use each. Look for answers that demonstrate an understanding of memory management and performance considerations in Ruby.
2. What is the purpose of the 'yield' keyword in Ruby?
The 'yield' keyword in Ruby is used to transfer control from a method to a block of code. It allows a method to receive a block and execute it at a specific point within the method.
Some key points about 'yield':
- It enables more flexible and reusable code.
- It's commonly used in iterators and custom control structures.
- It can pass arguments to the block.
Listen for explanations that show how 'yield' enhances code flexibility and reusability. A good candidate might provide an example of using 'yield' in a custom iterator or explain how it's used in Rails, such as in view rendering.
3. How does Ruby on Rails handle database migrations?
Ruby on Rails uses database migrations to manage changes to the database schema over time. Migrations are Ruby classes that define a set of changes to be applied to the database.
Key aspects of migrations in Rails:
- They're version-controlled and can be rolled back if needed.
- They use a DSL (Domain Specific Language) to define schema changes.
- Rails keeps track of which migrations have been run using a schema_migrations table.
Look for answers that demonstrate understanding of the migration process, including creating, running, and rolling back migrations. A strong candidate might also mention best practices like keeping migrations reversible and avoiding data migrations in schema migrations.
4. What is the difference between 'include' and 'extend' in Ruby modules?
'Include' and 'extend' are both used to add functionality from modules in Ruby, but they work differently:
- 'Include' adds methods as instance methods to a class.
- 'Extend' adds methods as class methods.
- 'Include' is typically used for adding behavior to instances.
- 'Extend' is used for adding behavior to the class itself.
A good answer should clearly explain these differences and provide examples of when to use each. Listen for mentions of how these concepts relate to Ruby's object model and inheritance system. Candidates might also discuss the use of 'extend self' within a module to create both instance and class methods.
5. Can you explain what Rack is and its role in Ruby on Rails?
Rack is a modular Ruby webserver interface that provides a minimal API for connecting web servers and web frameworks. It's a crucial part of the Ruby on Rails ecosystem.
Key points about Rack:
- It standardizes the interface between web servers and Ruby web frameworks.
- It allows for easy use of middleware to extend functionality.
- Rails uses Rack under the hood to handle HTTP requests and responses.
Look for answers that demonstrate understanding of Rack's role in the request/response cycle and its importance in Rails' modularity. A strong candidate might discuss how Rack middleware can be used to add functionality to a Rails application or explain the basic structure of a Rack application.
6. What is the purpose of the 'config/initializers' directory in a Rails application?
The 'config/initializers' directory in a Rails application is used to store Ruby code that needs to be run when the Rails application starts up. This is where you put configuration code that sets up the application environment.
Common uses of initializers include:
- Configuring third-party libraries
- Setting global constants
- Extending or modifying Rails framework classes
- Setting up custom logging or error handling
A good answer should explain that files in this directory are executed during the Rails boot process. Look for candidates who can provide examples of when to use initializers and understand their role in the application lifecycle. They might also mention best practices like keeping initializers small and focused.
7 situational Ruby on Rails interview questions with answers for hiring top developers
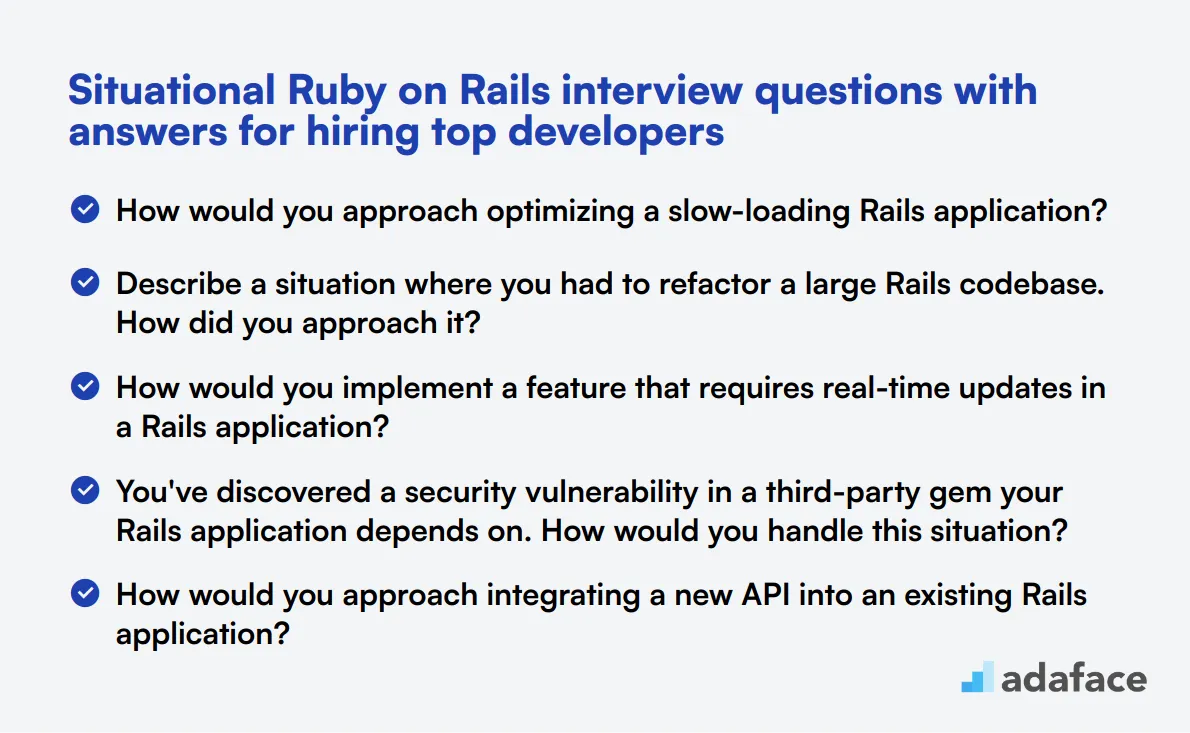
Ready to uncover the true potential of your Ruby on Rails candidates? These situational interview questions will help you dive deeper into a candidate's problem-solving skills and real-world experience. Use them to gauge how well applicants can apply their Ruby knowledge to practical scenarios, and get a better feel for their fit within your development team.
1. How would you approach optimizing a slow-loading Rails application?
A strong candidate should outline a systematic approach to performance optimization. They might mention:
- Identifying bottlenecks using tools like New Relic or Scout
- Optimizing database queries and adding appropriate indexes
- Implementing caching strategies (fragment caching, Russian Doll caching)
- Minimizing N+1 queries using eager loading
- Optimizing asset delivery through the asset pipeline
- Considering background job processing for time-consuming tasks
Look for candidates who demonstrate a methodical approach and familiarity with various optimization techniques. Follow up by asking about specific experiences they've had optimizing Rails applications in the past.
2. Describe a situation where you had to refactor a large Rails codebase. How did you approach it?
An experienced candidate should be able to describe a structured approach to refactoring:
- Starting with identifying pain points and areas for improvement
- Breaking down the refactoring into manageable chunks
- Ensuring proper test coverage before making changes
- Utilizing design patterns and principles like SOLID
- Gradually introducing changes while maintaining functionality
- Collaborating with team members and conducting code reviews
Pay attention to how the candidate balances the need for improvement with maintaining a working application. Look for mentions of testing, version control, and communication with team members throughout the process.
3. How would you implement a feature that requires real-time updates in a Rails application?
A knowledgeable candidate should discuss several options for implementing real-time features:
- Using Action Cable for WebSocket communication
- Implementing Server-Sent Events (SSE) for one-way real-time updates
- Utilizing polling as a simpler but less efficient alternative
- Considering third-party services like Pusher for easier implementation
- Discussing trade-offs between different approaches based on the specific use case
Look for candidates who can explain the pros and cons of different approaches and demonstrate an understanding of when to use each method. Ask follow-up questions about their experience with WebSockets or real-time features in previous projects.
4. You've discovered a security vulnerability in a third-party gem your Rails application depends on. How would you handle this situation?
A security-conscious candidate should outline a careful approach:
- Assess the severity and potential impact of the vulnerability
- Check if there's an updated version of the gem that addresses the issue
- If available, update the gem and thoroughly test the application
- If no fix is available, consider temporarily removing or disabling the gem
- Look for alternative gems that provide similar functionality
- Communicate the issue to the development team and stakeholders
- If appropriate, report the vulnerability to the gem maintainers
Pay attention to how the candidate balances security concerns with maintaining application functionality. Look for mentions of testing after updates and clear communication throughout the process.
5. How would you approach integrating a new API into an existing Rails application?
A methodical candidate should describe a step-by-step process:
- Review the API documentation thoroughly
- Choose an appropriate Ruby gem for API interaction (e.g., Faraday, HTTParty)
- Create a service object or module to encapsulate API calls
- Implement error handling and logging for API interactions
- Use environment variables for API keys and endpoints
- Write comprehensive tests for the integration
- Consider rate limiting and caching strategies if necessary
- Document the integration for other developers
Look for candidates who emphasize clean code organization, error handling, and testing. Ask follow-up questions about their experience with specific APIs or challenges they've faced in past integrations.
6. Describe how you would implement a multi-tenant architecture in a Rails application.
A knowledgeable candidate should discuss various approaches to multi-tenancy:
- Separate databases: Each tenant has its own database
- Shared database with separate schemas: Tenants share a database but have isolated schemas
- Shared database with a tenant identifier column: All data is in shared tables with a tenant ID
- Discussing pros and cons of each approach (scalability, data isolation, complexity)
- Mentioning gems like Apartment for easier implementation
- Considering authentication and authorization in a multi-tenant context
Look for candidates who can explain the trade-offs between different approaches and demonstrate understanding of data isolation and security concerns in multi-tenant systems. Ask about any experience they have with implementing or maintaining multi-tenant applications.
7. How would you handle database migrations in a Rails application with zero downtime?
A candidate with experience in continuous deployment should outline a strategy like:
- Use reversible migrations whenever possible
- Add new tables or columns without modifying existing structures first
- Use background jobs to gradually migrate data if large data movements are necessary
- Employ feature flags to control access to new functionality
- Use database views to abstract schema changes from the application
- Consider using gems like Strong Migrations to catch unsafe migrations
- Have a rollback plan ready in case of unexpected issues
Look for candidates who emphasize the importance of careful planning and testing. Ask about their experience with large-scale migrations or any challenges they've faced ensuring zero downtime during deployments.
Which Ruby on Rails skills should you evaluate during the interview phase?
While it's challenging to assess every aspect of a candidate's Ruby on Rails expertise in a single interview, focusing on core skills can provide valuable insights. The following key areas are particularly important to evaluate during the interview process for Ruby on Rails developers.
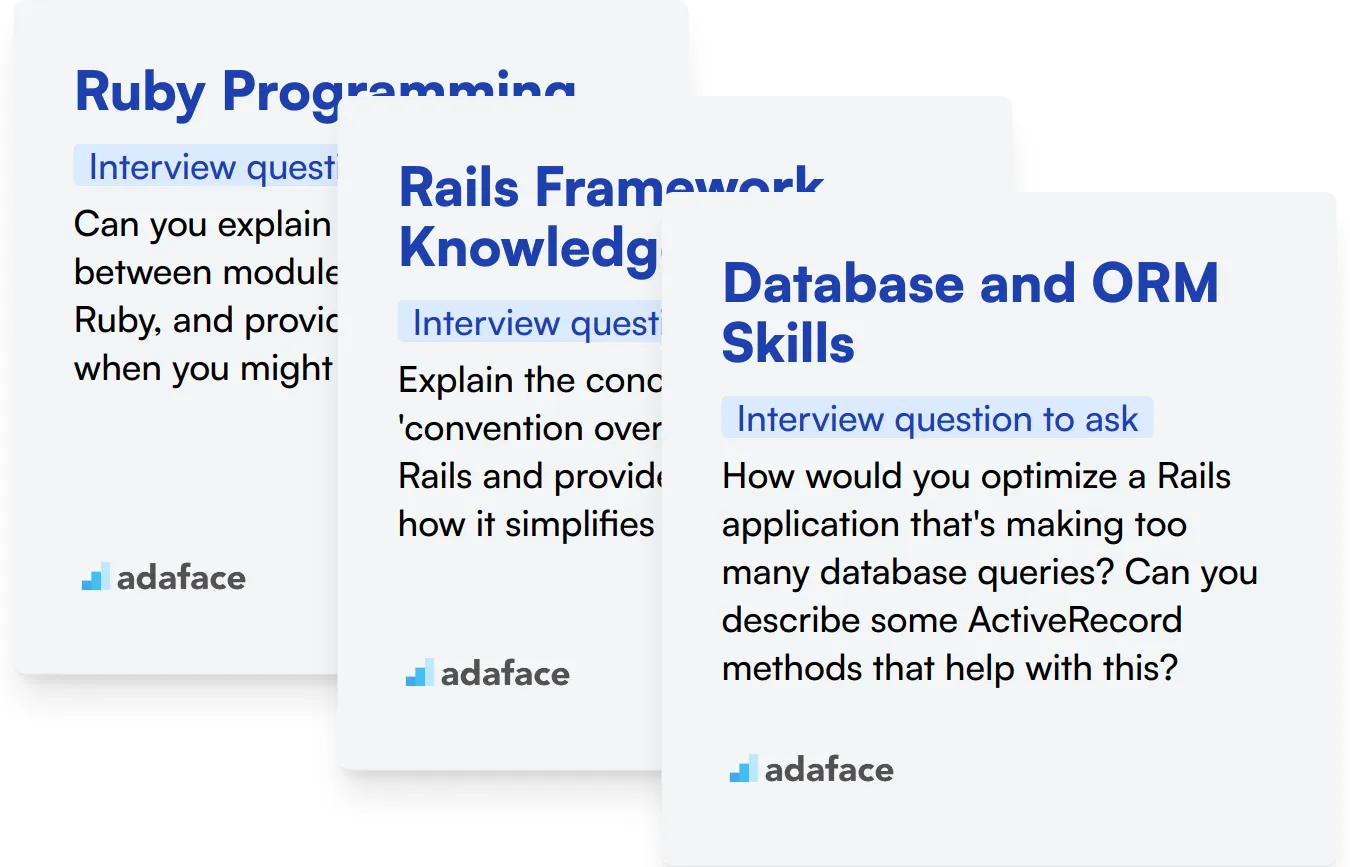
Ruby Programming
Ruby is the foundation of Ruby on Rails. A strong grasp of Ruby syntax, object-oriented concepts, and Ruby-specific features is essential for effective Rails development.
To assess Ruby skills, consider using a Ruby online test that includes relevant multiple-choice questions. This can help filter candidates based on their Ruby proficiency.
During the interview, you can ask targeted questions to gauge the candidate's Ruby knowledge. Here's an example question:
Can you explain the difference between modules and classes in Ruby, and provide an example of when you might use each?
Look for answers that demonstrate understanding of Ruby's object model, inheritance, and code organization. A good response should explain that modules are used for namespacing and mixins, while classes are used for creating objects.
Rails Framework Knowledge
Understanding the Rails framework, its conventions, and best practices is crucial for building efficient and maintainable web applications. This includes knowledge of MVC architecture, routing, and ActiveRecord.
To evaluate Rails-specific skills, you might use a Ruby on Rails assessment test that covers framework concepts and practical usage scenarios.
To assess Rails knowledge during the interview, consider asking a question like this:
Explain the concept of 'convention over configuration' in Rails and provide an example of how it simplifies development.
Look for answers that show understanding of Rails conventions, such as naming conventions for models, controllers, and database tables. A good response might include examples of how these conventions reduce the need for explicit configuration.
Database and ORM Skills
Proficiency with databases and ActiveRecord (Rails' ORM) is key for developing data-driven applications. This includes understanding database design, querying, and optimization.
To assess database skills, you might include database-related questions in your Ruby on Rails assessment or use a separate SQL online test to evaluate general SQL knowledge.
During the interview, you can ask a question to gauge the candidate's understanding of ActiveRecord and database interactions:
How would you optimize a Rails application that's making too many database queries? Can you describe some ActiveRecord methods that help with this?
Look for answers that mention techniques like eager loading (includes, preload, eager_load), indexing, and caching. A strong candidate might also discuss N+1 query problems and how to avoid them using ActiveRecord methods.
3 Tips for Using Ruby on Rails Interview Questions
Before you start putting what you've learned to use, here are our tips to maximize your interview process.
1. Utilize Skills Tests Before Interviews
Using skills tests before interviews helps in filtering candidates who possess the required technical expertise. This ensures that only qualified candidates move forward in the hiring process.
Consider using our Ruby on Rails online test to assess a candidate's technical proficiency. Other recommended tests include the Ruby Rails SQL test and the Ruby online test. These tests cover a range of skills from basic to advanced levels.
The benefits of skills tests include saving time and resources, and ensuring a more objective assessment. By pre-filtering candidates, you can focus your interviews on those who already demonstrate the necessary technical skills.
2. Outline and Compile Interview Questions Effectively
Time is limited in interviews, so compiling a concise list of questions is crucial for evaluating candidates thoroughly. Choose questions that effectively assess technical skills, problem-solving abilities, and cultural fit.
Consider including questions from other relevant domains. For instance, including soft skills like communication and teamwork can provide a holistic view of the candidate’s capabilities. Refer to our communication interview questions for more ideas.
3. Ask Follow-Up Questions to Gauge Depth
Simply asking interview questions isn't enough. Follow-up questions are necessary to understand the candidate's depth of knowledge and to verify their claims.
For example, if you ask a candidate about their experience with Ruby on Rails, a good follow-up question could be: 'Can you walk me through how you implemented a specific feature in your last project?' This helps you gauge their practical experience and problem-solving approach.
Hire top Ruby on Rails developers with targeted assessments and interviews
To hire skilled Ruby on Rails developers, it's important to verify their abilities accurately. Using Ruby on Rails skills tests is an effective way to evaluate candidates' proficiency. These tests help you gauge their knowledge and practical skills objectively.
After using the tests to shortlist the best applicants, you can invite them for interviews. To streamline your hiring process and find the right talent, consider exploring our online assessment platform for a comprehensive solution.
Ruby on Rails Online Test
Download Ruby on Rails interview questions template in multiple formats
Ruby on Rails Interview Questions FAQs
Ruby on Rails, often called Rails, is a server-side web application framework written in Ruby.
Key features include MVC architecture, database migrations, RESTful design, and the Rails API.
Assess proficiency by asking about their experience with Ruby, Rails, MVC architecture, and specific projects they've worked on.
Common pitfalls include focusing too much on theoretical knowledge and not enough on practical experience and problem-solving ability.
Look for a strong understanding of Ruby basics, familiarity with the Rails framework, and a willingness to learn and grow.
Understanding processes is crucial for efficient workflow, code quality, and to ensure that the developer can work well within your team’s practices.
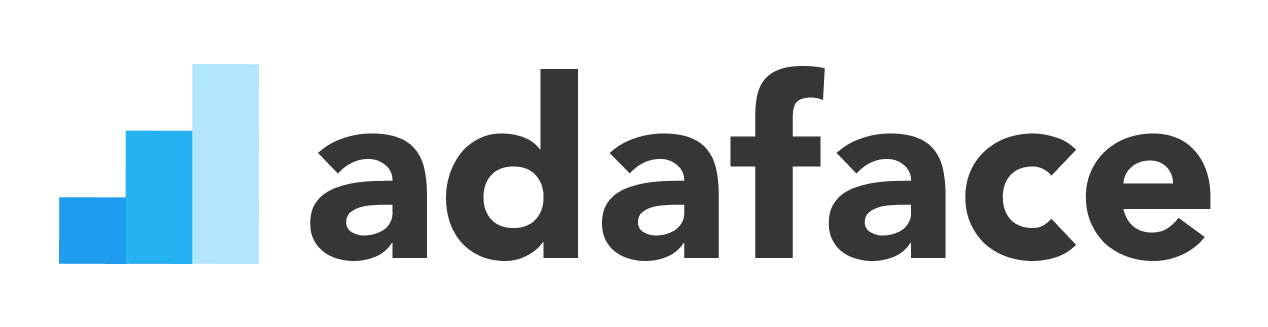
40 min skill tests.
No trick questions.
Accurate shortlisting.
We make it easy for you to find the best candidates in your pipeline with a 40 min skills test.
Try for freeRelated posts
Free resources
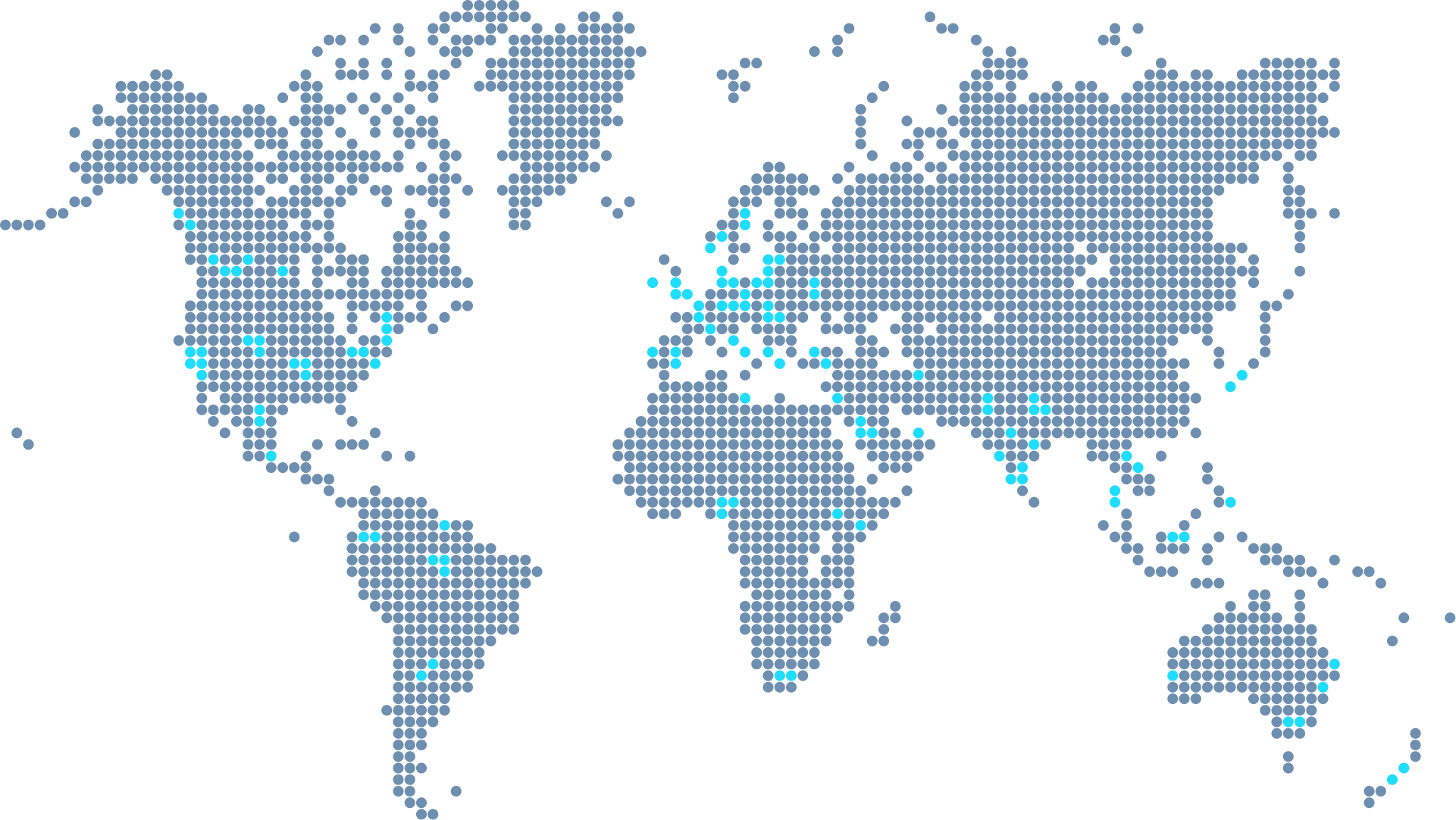
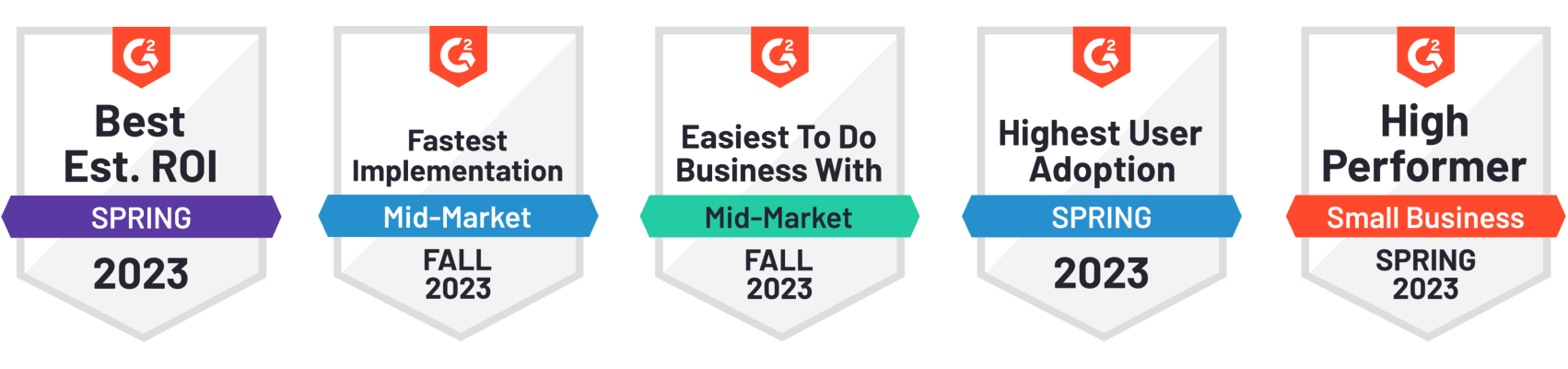