Hiring the right Ruby developer can be a game-changer, but evaluating candidates can be tough without a clear set of questions. Well-crafted interview questions are vital to discern the depth of a candidate’s programming skills and problem-solving abilities, ensuring you pick the best fit for your team.
This blog post will provide a curated list of Ruby interview questions tailored for different expertise levels. From junior to intermediate developers, and covering areas like code optimization and error handling, you’ll find the questions you need to make informed hiring decisions.
Armed with this guide, you can ensure your Ruby interviews are thorough and insightful, resulting in better hiring outcomes. For an extra layer of assessment, consider using an online Ruby test before proceeding to interviews.
Table of contents
Top 9 Ruby questions to ask in interviews
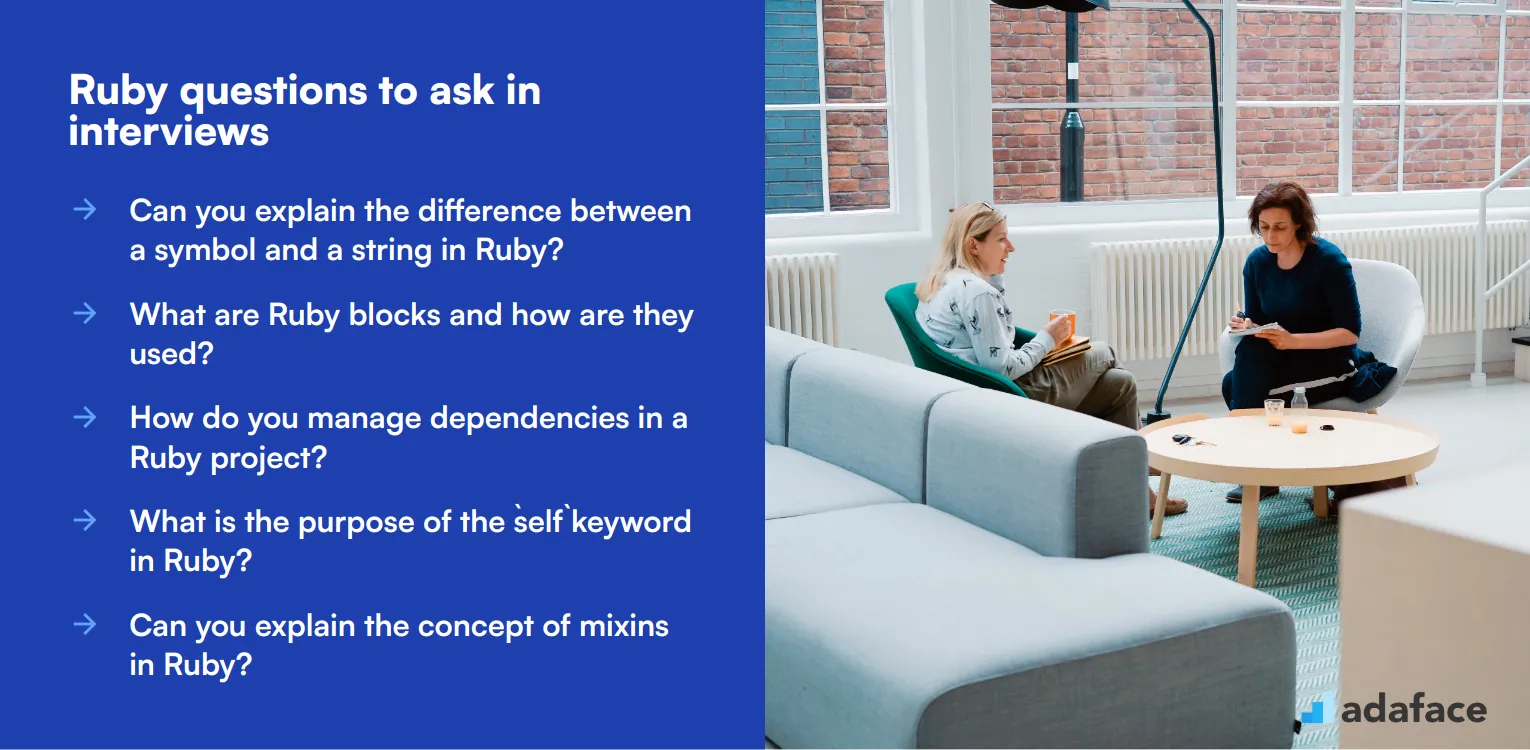
To help you identify top Ruby talent, we've compiled a list of essential Ruby interview questions. These questions will help you assess the candidate's understanding of core Ruby concepts, problem-solving skills, and practical experience. Use them during your technical interviews to ensure you hire the right fit for your team.
1. Can you explain the difference between a symbol and a string in Ruby?
A symbol in Ruby is an immutable, unique identifier often used for names and labels, while a string is a mutable sequence of characters.
Symbols are more memory-efficient for identifiers that do not change, as they are stored only once in memory. Strings, however, are better for text manipulation and data that needs to change.
Look for candidates who can clearly explain these differences and provide examples of when to use each. Ideal responses will mention memory efficiency and immutability for symbols versus mutability for strings.
2. What are Ruby blocks and how are they used?
Ruby blocks are anonymous pieces of code that can be passed to methods and executed. They are defined between do...end
or curly braces {...}
.
Blocks are often used for iteration, callbacks, and to defer execution of code until needed. They are a powerful feature that allows for more flexible and reusable code.
Ideal candidates should provide examples of block usage, such as with iterators like each
or custom method implementations. Look for their understanding of how blocks can make code more concise and readable.
3. How do you manage dependencies in a Ruby project?
In Ruby projects, dependencies are managed using Bundler. Bundler allows you to specify the gems your project needs in a Gemfile
and then install them using bundle install
.
It ensures that the correct versions of gems are used, and it maintains a Gemfile.lock
to keep track of installed versions, ensuring consistency across different environments.
Candidates should demonstrate familiarity with Bundler and discuss how they use it to manage dependencies effectively, including handling version conflicts and updating gems.
4. What is the purpose of the `self` keyword in Ruby?
The self
keyword in Ruby refers to the current object in context. It can be used to access the object's methods and variables within its own instance or class scope.
In instance methods, self
refers to the instance of the class, while in class methods, it refers to the class itself. It is also used for defining class-level methods.
Look for candidates who can explain different contexts where self
is used and provide examples. They should be able to articulate its role in both instance and class methods.
5. Can you explain the concept of mixins in Ruby?
Mixins in Ruby allow modules to be included in classes, providing a way to share code across multiple classes. They enable multiple inheritance and code reuse.
By including a module, a class gains access to the module's methods as if they were defined in the class itself. This helps in keeping the code DRY (Don't Repeat Yourself).
Candidates should provide examples of using modules for mixins and discuss scenarios where they are beneficial, such as adding functionality to several classes without duplicating code.
6. What are some common uses of metaprogramming in Ruby?
Metaprogramming in Ruby involves writing code that writes or modifies other code at runtime. It's commonly used for defining methods dynamically, creating DSLs (Domain-Specific Languages), and implementing design patterns.
Common uses include method_missing
for handling undefined methods, define_method
for creating methods dynamically, and using class_eval
or instance_eval
for altering class or instance behavior.
Look for explanations that highlight the benefits of metaprogramming, such as reducing boilerplate code and enhancing flexibility. Candidates should also mention potential pitfalls, like making code harder to understand and debug.
7. How do you handle exceptions in Ruby?
In Ruby, exceptions are handled using begin...rescue...ensure
blocks. The begin
block contains code that might raise an exception, rescue
handles the exception, and ensure
executes code regardless of whether an exception occurred.
You can also use raise
to explicitly raise exceptions and create custom exception classes for more granular error handling.
Candidates should demonstrate a clear understanding of exception handling and provide examples. They should discuss the importance of gracefully handling errors to ensure application stability and user experience.
8. What is the role of garbage collection in Ruby?
Garbage collection in Ruby is the process of automatically freeing up memory by removing objects that are no longer in use. This helps manage memory efficiently and prevents memory leaks.
Ruby's garbage collector uses a mark-and-sweep algorithm to identify and clean up unused objects. This allows developers to focus on writing code without worrying about manual memory management.
Ideal candidates should explain the benefits of garbage collection and discuss its impact on application performance. They should also mention any potential issues, such as performance overhead during garbage collection cycles.
9. How do you ensure the quality and reliability of your Ruby code?
Ensuring quality and reliability in Ruby code involves several practices: writing tests, using code linters, performing code reviews, and following best practices for coding standards.
Tests can be written using frameworks like RSpec or Minitest to cover various scenarios and edge cases. Continuous integration tools can automate testing and ensure code quality.
Look for candidates who emphasize the importance of testing and code reviews. They should provide examples of tools they use and discuss how they ensure their code is maintainable and reliable.
20 Ruby interview questions to ask junior developers
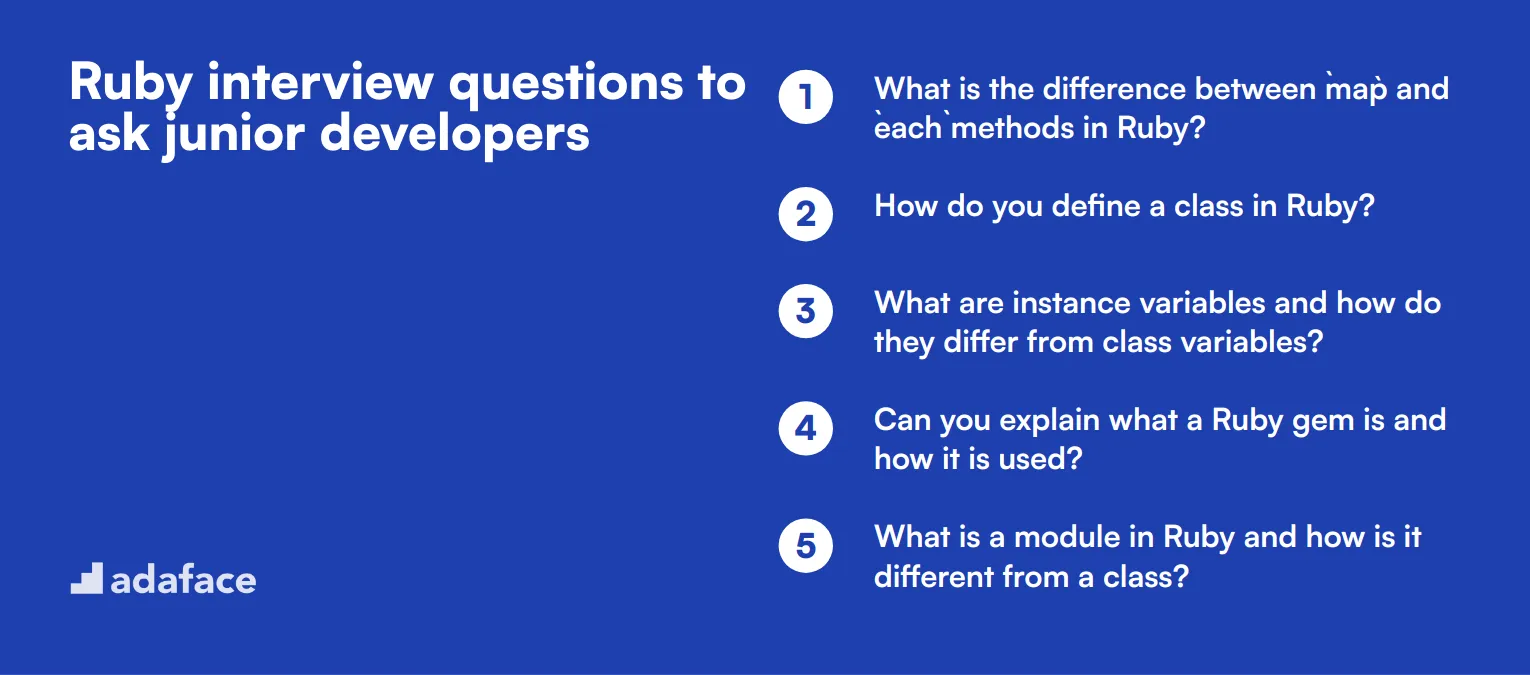
To determine whether your junior developers have a solid understanding of Ruby, use this list of 20 practical interview questions. These questions are designed to evaluate their fundamental knowledge and problem-solving skills, ensuring they can handle the key aspects of Ruby development.
- What is the difference between `map` and `each` methods in Ruby?
- How do you define a class in Ruby?
- What are instance variables and how do they differ from class variables?
- Can you explain what a Ruby gem is and how it is used?
- What is a module in Ruby and how is it different from a class?
- How do you create getter and setter methods in Ruby?
- What is the use of the `initialize` method in a Ruby class?
- Can you explain the difference between `include` and `extend` in Ruby?
- How do you iterate over a hash in Ruby?
- What is the purpose of the `require` statement in Ruby?
- How does the `respond_to?` method work?
- What are the different types of variables in Ruby?
- How can you create a new instance of a class in Ruby?
- What is the purpose of the `attr_accessor` method?
- How do you write a conditional statement in Ruby?
- What is a Proc in Ruby and how does it differ from a lambda?
- How do you check if an object is an instance of a certain class?
- Can you explain what a mixin is used for in Ruby?
- What does the `super` keyword do in Ruby?
- How do you handle method overloading in Ruby?
10 intermediate Ruby interview questions and answers to ask mid-tier developers.
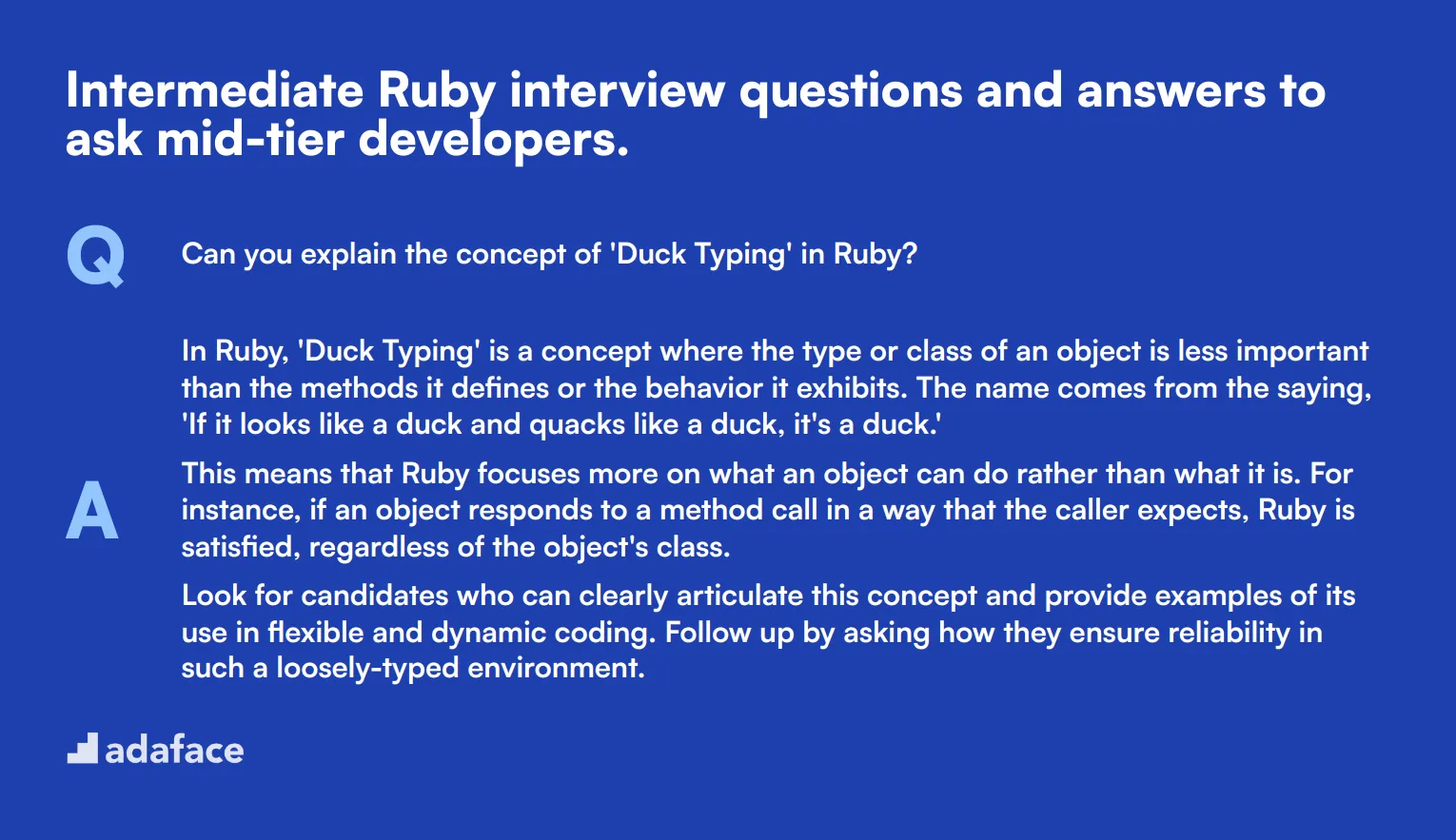
To gauge whether your mid-tier Ruby developers have the right mix of technical know-how and practical experience, here is a list of 10 intermediate Ruby interview questions. These questions will help you assess their understanding of more complex aspects of Ruby without getting overly technical.
1. Can you explain the concept of 'Duck Typing' in Ruby?
In Ruby, 'Duck Typing' is a concept where the type or class of an object is less important than the methods it defines or the behavior it exhibits. The name comes from the saying, 'If it looks like a duck and quacks like a duck, it's a duck.'
This means that Ruby focuses more on what an object can do rather than what it is. For instance, if an object responds to a method call in a way that the caller expects, Ruby is satisfied, regardless of the object's class.
Look for candidates who can clearly articulate this concept and provide examples of its use in flexible and dynamic coding. Follow up by asking how they ensure reliability in such a loosely-typed environment.
2. What is the significance of 'yield' in Ruby?
The 'yield' keyword in Ruby is used to transfer control from a method to a block given to that method. It allows the block to execute within the context of the method, often used for defining methods that take a block of code as an argument.
An example would be iterating over collections, where 'yield' can be used to execute a block for each element. This makes the code more concise and readable.
An ideal response should highlight practical uses of 'yield' and demonstrate an understanding of when to use it over other constructs like Procs and lambdas. You might also want to ask about any pitfalls they've encountered while using 'yield'.
3. How do you handle thread safety in Ruby?
Thread safety in Ruby involves ensuring that data is protected from concurrent access issues. This is crucial when multiple threads are working with shared data. One common approach is using mutexes (mutual exclusions) to lock data structures.
Another approach could involve using thread-safe data structures provided by Ruby libraries or employing functional programming techniques to avoid shared state.
Look for candidates who can explain these techniques and provide scenarios where they've successfully implemented thread safety. Follow up by asking how they balance performance with thread safety.
4. What is the purpose of the 'freeze' method in Ruby?
The 'freeze' method in Ruby is used to make an object immutable. Once an object is frozen, it cannot be modified: its state is locked, and any attempts to alter it will result in an error.
This is particularly useful for protecting objects from unintended changes, ensuring data integrity, and improving performance in some cases by avoiding unnecessary object modifications.
Look for candidates to discuss scenarios where immutability is beneficial, such as in constants or shared resources. You might also want to ask about any limitations or drawbacks they've encountered with 'freeze'.
5. Can you explain the difference between 'require' and 'load' in Ruby?
'require' and 'load' are used to include external files in Ruby, but they work differently. 'require' loads a file only once per Ruby runtime, preventing multiple inclusions. It's commonly used for including libraries and gems.
'load', on the other hand, includes the specified file every time it is called, which can be useful for reloading code during development but is generally avoided in production for performance reasons.
Candidates should highlight the specific use cases for each and demonstrate a clear understanding of when to use 'require' versus 'load'. Follow up by asking about any situations where they've had to troubleshoot issues related to these methods.
6. What is the purpose of a 'singleton method' in Ruby?
A singleton method is a method that is defined on a single object rather than on all instances of a class. This means that only one specific object can use this method, making it unique to that object.
Singleton methods are often used for defining object-specific behavior or for creating class-level methods on the singleton class of the object.
Look for candidates who can provide practical examples of using singleton methods and discuss the scenarios where they found them particularly useful. You can follow up by asking about any limitations they've encountered.
7. How do you ensure your Ruby application is scalable?
Scalability in a Ruby application can be achieved through several approaches, such as optimizing database queries, using background jobs, and employing caching mechanisms. Additionally, using a microservices architecture can distribute the load more efficiently.
Another strategy is to use scalable cloud services and deploying the application in a way that can handle increased traffic without degrading performance, such as containerization with Docker and orchestration with Kubernetes.
Ideal candidates should demonstrate a comprehensive understanding of these techniques and provide examples of how they've implemented them. Follow up by asking for details on any specific challenges they've faced in scaling Ruby applications and how they overcame them.
8. What are Ruby's enumerable methods, and how do you use them?
Enumerable methods in Ruby are a set of methods provided by the Enumerable module, which can be included in Ruby classes to provide collection traversal and searching capabilities. These methods include map, select, reject, and find, among others.
These methods are particularly useful for iterating over collections like arrays and hashes, allowing for concise and readable code when performing operations on these collections.
Look for candidates who can discuss the various enumerable methods and provide examples of their use. You might also want to ask them to explain the benefits of using these methods over traditional looping constructs.
9. What is the role of 'monkey patching' in Ruby?
Monkey patching in Ruby refers to the practice of adding methods or altering existing methods in built-in classes or modules. This allows developers to modify or extend the behavior of libraries or classes without modifying their source code.
While monkey patching can be powerful, it should be used cautiously as it can lead to unexpected behavior and maintenance challenges if not done carefully.
Candidates should demonstrate an understanding of the risks and benefits of monkey patching and provide examples of when they've used this technique. Follow up by asking about any strategies they use to mitigate the risks associated with monkey patching.
10. How do you handle performance optimization in a Ruby application?
Performance optimization in a Ruby application can involve various strategies such as profiling the code to identify bottlenecks, optimizing database queries, and using caching mechanisms to reduce redundant computations.
Other techniques include optimizing algorithms, minimizing memory usage, and employing asynchronous processing for tasks that can be performed in parallel.
Look for candidates who can discuss these strategies in detail and provide examples of how they've successfully optimized Ruby applications. You might also want to ask about any performance monitoring tools they use and how they identify areas for improvement.
14 Ruby interview questions about code optimization
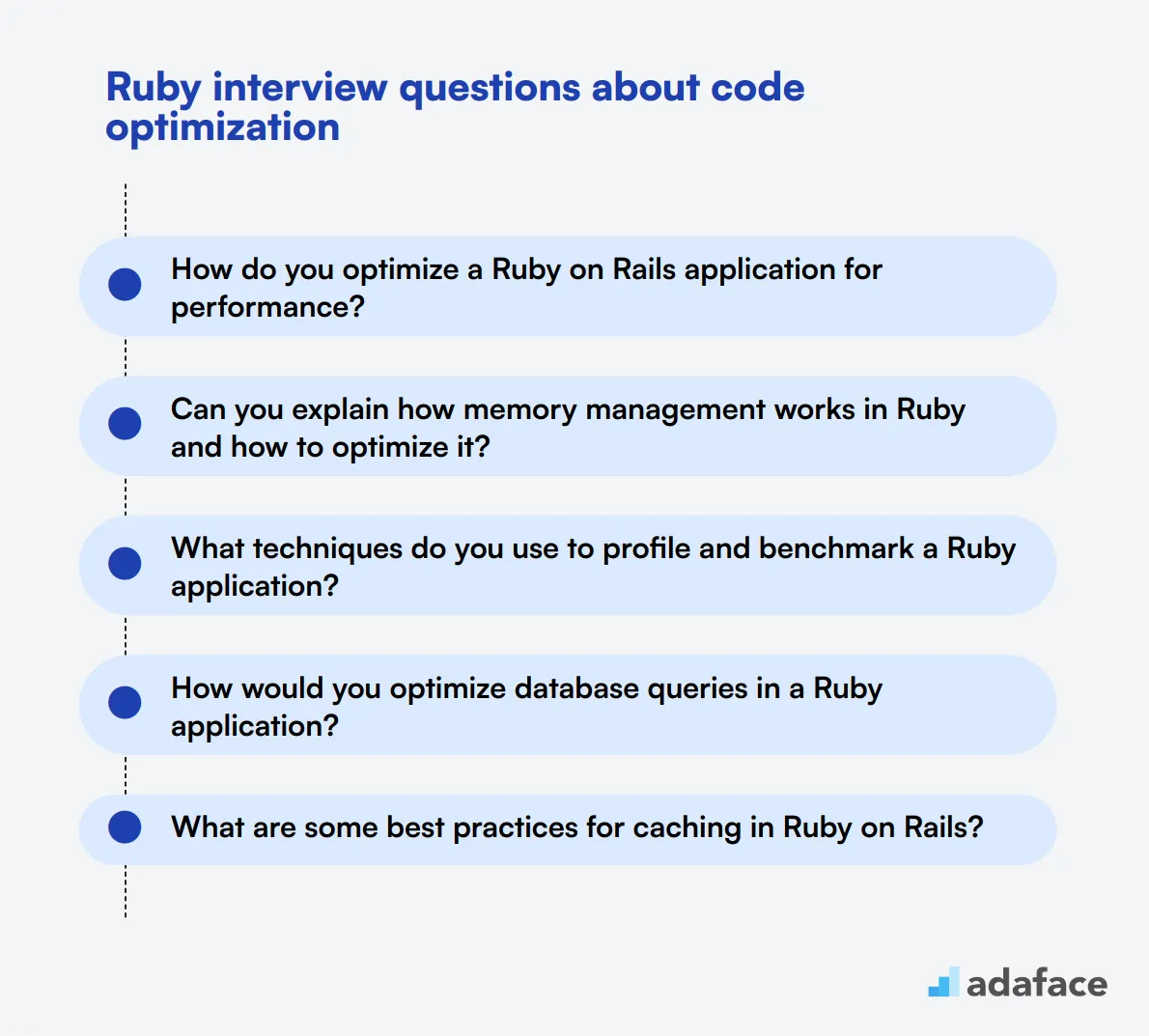
To assess whether your candidates possess the necessary skills for efficient and effective code optimization in Ruby, use these focused interview questions. They will help you evaluate a candidate's ability to write high-performing and maintainable code, a crucial aspect for any Ruby on Rails Developer.
- How do you optimize a Ruby on Rails application for performance?
- Can you explain how memory management works in Ruby and how to optimize it?
- What techniques do you use to profile and benchmark a Ruby application?
- How would you optimize database queries in a Ruby application?
- What are some best practices for caching in Ruby on Rails?
- How can you reduce the memory footprint of a Ruby application?
- What are the common performance bottlenecks in Ruby applications and how do you address them?
- How do you handle slow tests in a Ruby test suite?
- What tools do you use for profiling Ruby applications?
- How can you minimize the load time of a Ruby on Rails application?
- What strategies do you use for optimizing Ruby code for concurrency?
- How do you ensure efficient garbage collection in a Ruby application?
- What are the benefits and drawbacks of using just-in-time (JIT) compilation in Ruby?
- Can you explain how to use memoization to optimize performance in Ruby?
8 Ruby interview questions and answers related to error handling
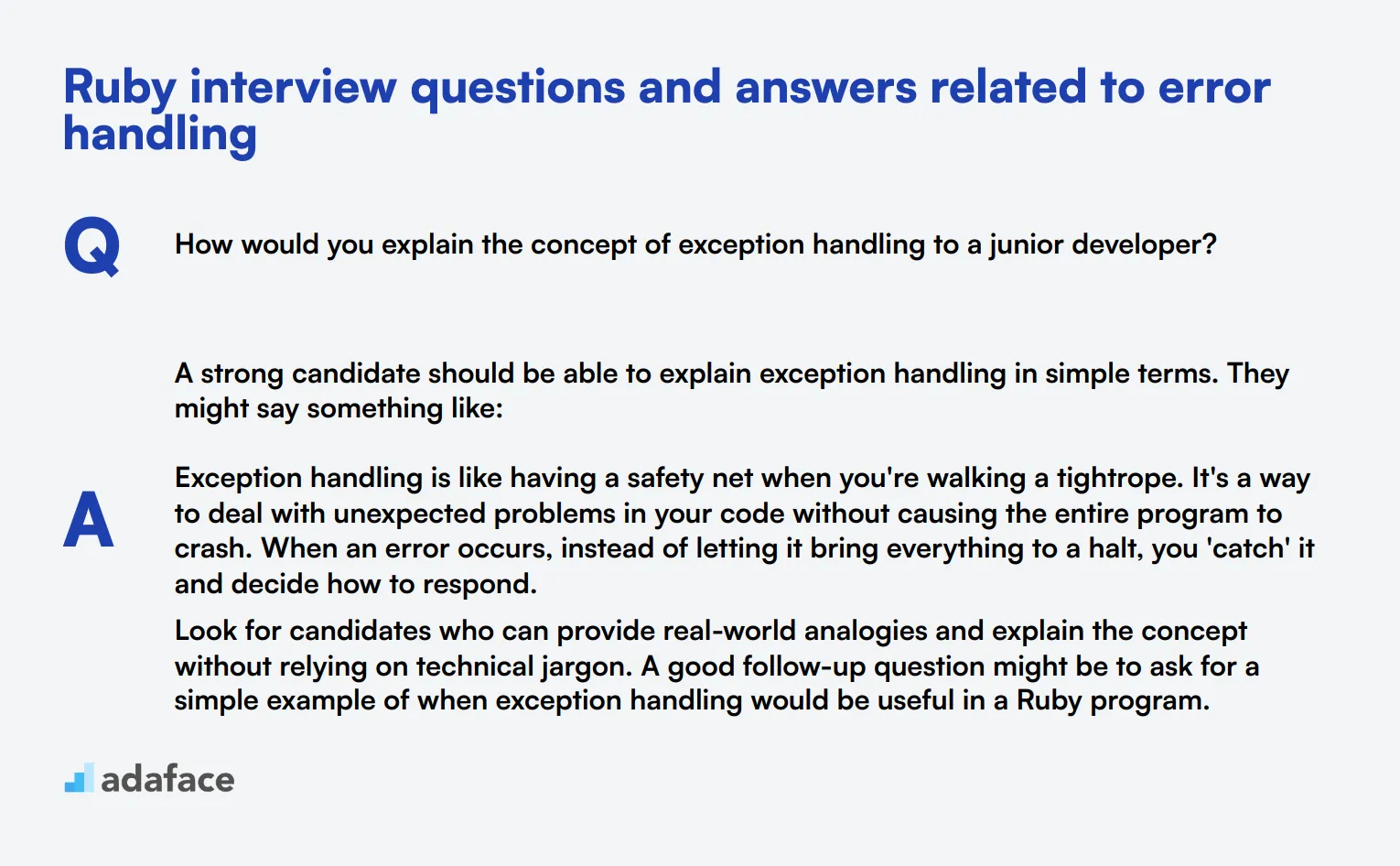
When it comes to hiring Ruby developers, understanding their grasp of error handling is crucial. These 8 Ruby interview questions will help you assess a candidate's ability to manage unexpected situations and write robust code. Use them to gauge how well potential hires can handle the bumps in the Ruby road!
1. How would you explain the concept of exception handling to a junior developer?
A strong candidate should be able to explain exception handling in simple terms. They might say something like:
Exception handling is like having a safety net when you're walking a tightrope. It's a way to deal with unexpected problems in your code without causing the entire program to crash. When an error occurs, instead of letting it bring everything to a halt, you 'catch' it and decide how to respond.
Look for candidates who can provide real-world analogies and explain the concept without relying on technical jargon. A good follow-up question might be to ask for a simple example of when exception handling would be useful in a Ruby program.
2. Can you describe a situation where you've used custom exceptions in Ruby?
An experienced Ruby developer should be able to provide a specific example from their past projects. They might describe a scenario like:
I once worked on an e-commerce application where we created a custom InventoryError
exception. We used it when a customer tried to purchase an item that was out of stock. This allowed us to handle this specific scenario differently from other errors, providing a more user-friendly message and suggesting alternative products.
Look for answers that demonstrate the candidate's understanding of when and why to use custom exceptions. Their response should show how custom exceptions can improve code readability and error handling specificity. Consider asking how they decided between using a custom exception versus a standard Ruby exception in their example.
3. How do you decide between using 'rescue' at the method level versus wrapping a specific line of code?
A thoughtful answer might include:
The decision depends on the granularity of error handling needed. Method-level rescue is useful when you want to handle all exceptions in a method the same way, or when you're not sure where an exception might occur. Wrapping specific lines is better when you want to handle errors from a particular operation differently, or when you want to ensure that other parts of the method still execute even if one part fails.
Look for candidates who can articulate the trade-offs between these approaches. They should mention considerations like code readability, performance, and the principle of least surprise. A good follow-up might be to ask for an example of when they chose one approach over the other in a real project.
4. What's the difference between 'raise' and 'throw' in Ruby, and when would you use each?
A knowledgeable Ruby developer should explain:
raise
is used for typical exception handling and is part of Ruby's exception system. It's used to raise exceptions that will be caught by rescue
blocks. throw
, on the other hand, is not part of the exception system. It's used with catch
for flow control, allowing you to exit from deeply nested structures.
Look for answers that demonstrate understanding of these subtle differences. Candidates should be able to provide examples of when they'd use raise
(like for error conditions) versus throw
(like for breaking out of nested loops). Consider asking how error handling practices might differ in a Ruby on Rails application compared to a plain Ruby script.
5. How would you debug a rescued exception that doesn't provide enough information about the error?
A proficient Ruby developer might suggest several strategies:
- Use
begin...rescue => e
to capture the exception object and inspect its properties likee.message
ande.backtrace
- Employ logging libraries to record more detailed information about the application state when the error occurs
- Utilize debugging tools like
pry
orbyebug
to set breakpoints and step through the code - Implement more granular exception handling to pinpoint where exactly the error is occurring
- Add custom attributes to exceptions to carry more context about the error
Look for candidates who demonstrate a systematic approach to problem-solving and familiarity with Ruby's debugging tools. A good follow-up question might be to ask about their preferred logging practices or how they balance informative error messages with security concerns.
6. Can you explain the concept of 'ensure' in Ruby's exception handling? When would you use it?
A solid answer should cover the following points:
ensure
is a block used in exception handling that always executes, whether an exception was raised or not. It's typically used for cleanup operations that need to happen regardless of whether the code executed successfully or encountered an error. Common use cases include closing file handles, releasing database connections, or cleaning up temporary resources.
Look for candidates who can provide practical examples of using ensure
. They should understand its importance in maintaining application stability and preventing resource leaks. Consider asking how they might use ensure
in conjunction with begin...rescue
blocks in a real-world scenario.
7. How do you handle errors in Ruby that occur in background jobs or separate threads?
An experienced developer might describe strategies like:
- Implementing a global exception handler for background jobs
- Using logging mechanisms to record errors in separate files or services
- Implementing retry mechanisms for transient failures
- Setting up monitoring and alerting systems to notify developers of errors in background processes
- Using gems like Sidekiq's error handlers for job-specific error management
Look for answers that show an understanding of the challenges of debugging asynchronous processes. Candidates should be aware of the importance of comprehensive logging and monitoring in distributed systems. You might follow up by asking how they would prioritize which background job errors need immediate attention versus those that can be addressed later.
8. What's your approach to balancing detailed error messages for debugging with security concerns in a production environment?
A thoughtful response might include:
- Using different logging levels for development and production environments
- Implementing custom error pages that provide generic messages to users while logging detailed information for developers
- Utilizing error monitoring services that securely store and analyze error data
- Sanitizing sensitive information from error messages and stack traces
- Implementing role-based access to detailed error information
Look for candidates who understand the importance of both debugging capabilities and security. They should be able to discuss how they've handled this balance in previous projects. A good follow-up question might be to ask about specific tools or practices they've used to achieve this balance in Ruby on Rails applications.
Which Ruby skills should you evaluate during the interview phase?
While it's impossible to gauge every aspect of a candidate's capabilities in a single interview, focusing on key skills can provide significant insights. For Ruby developers, certain core skills are crucial for assessing their proficiency and fit for the role.
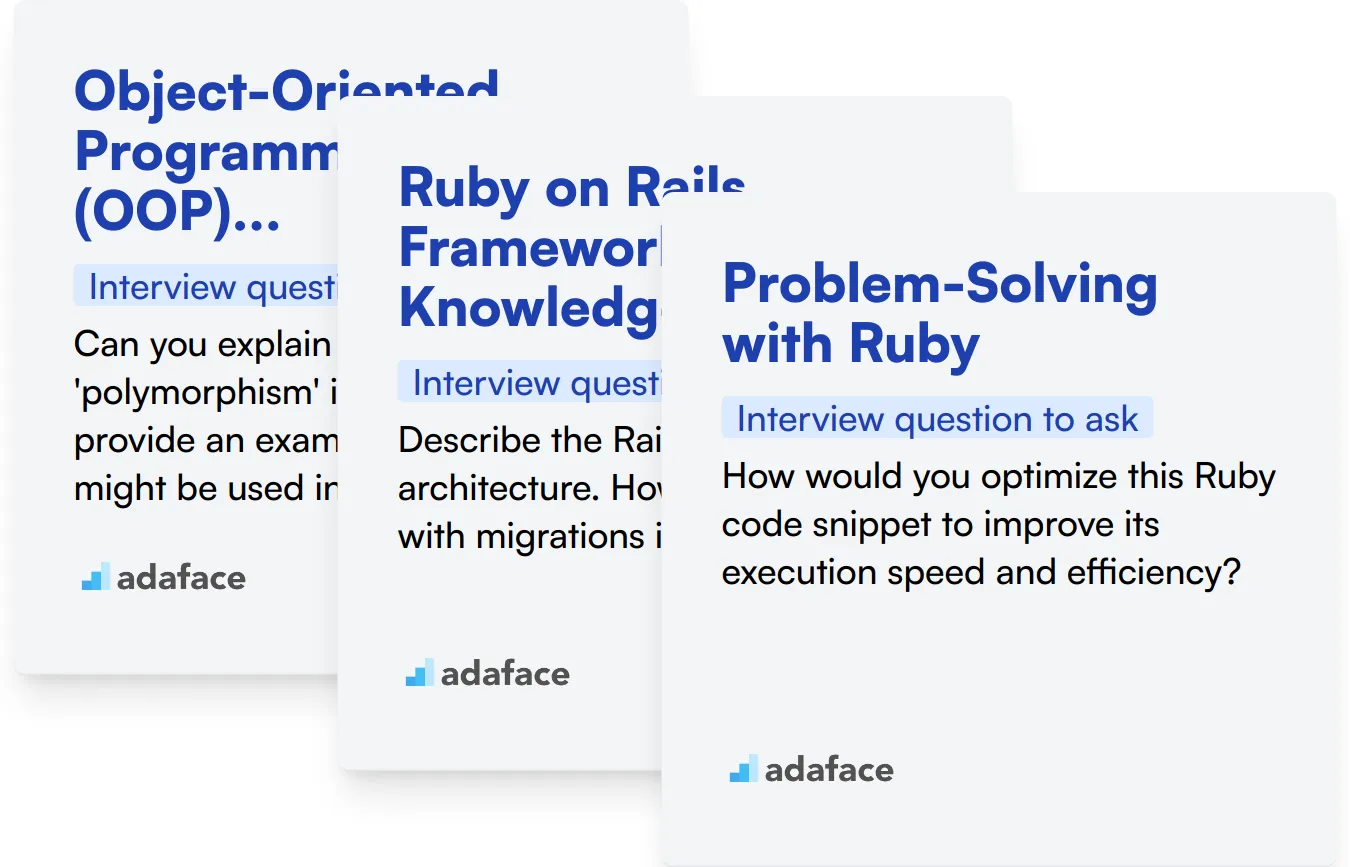
Object-Oriented Programming (OOP) Principles
Understanding OOP is fundamental for any Ruby developer, given Ruby's strong emphasis on object-oriented concepts. Mastery of this skill ensures the developer can effectively structure and organize code, which is critical for building scalable and maintainable applications.
To pre-screen candidates on their understanding of OOP principles, consider utilizing a structured assessment test. Adaface offers a Ruby Online Test which includes relevant MCQs to help identify candidates well-versed in OOP.
During the interview, you can delve deeper into their understanding by asking specific questions about OOP.
Can you explain the concept of 'polymorphism' in Ruby and provide an example of how it might be used in an application?
Look for detailed explanations that include correct terminology and practical examples, showing an in-depth understanding of different OOP features in Ruby.
Ruby on Rails Framework Knowledge
Proficiency in Ruby on Rails is often necessary for Ruby developers, as it's one of the most popular frameworks for web development. This skill is indicative of a developer's ability to build efficient, database-backed web applications quickly.
Evaluate their Rails proficiency early in the process using the Rails Assessment Test from Adaface, which screens for a comprehensive understanding of the Rails framework through carefully crafted MCQs.
Complement this test with interview questions that assess practical Rails knowledge.
Describe the Rails MVC architecture. How do you work with migrations in Rails?
Candidates should clearly outline each component of the MVC structure and demonstrate familiarity with Rails migrations, showcasing their practical Rails experience.
Problem-Solving with Ruby
The ability to solve problems effectively using Ruby is crucial. It reflects a candidate's logical thinking and fluency in using Ruby to devise solutions to complex challenges.
To explore their problem-solving skills, present real-world scenarios that require thoughtful solutions using Ruby.
How would you optimize this Ruby code snippet to improve its execution speed and efficiency?
Expect detailed reasoning about the changes made to the code, demonstrating both Ruby proficiency and a strong problem-solving approach.
Use Ruby interview questions and skills tests to hire talented developers
If you are looking to hire someone with Ruby skills, it is important to ensure that they possess the necessary expertise accurately.
The best way to do this is by using a skill test. Consider our Ruby Online Test or Ruby on Rails Test to evaluate candidates' capabilities.
Once you have utilized these tests, you can shortlist the top candidates and invite them for interviews.
To get started, head over to our test library or sign up to begin assessing your candidates.
Ruby Online Test
Download Ruby interview questions template in multiple formats
Ruby Interview Questions FAQs
Ask a mix of questions covering basics, OOP concepts, error handling, code optimization, and practical scenarios to assess candidates' Ruby skills comprehensively.
The number of questions depends on the interview duration and candidate level. For a thorough assessment, aim for 10-15 questions across different skill areas.
Yes, tailor your questions to the experience level. Ask simpler concepts for juniors and more advanced topics for seniors to accurately gauge their expertise.
Include coding challenges or ask candidates to explain their approach to solving complex problems using Ruby. This helps evaluate their logical thinking and coding abilities.
Yes, combining interview questions with coding tests provides a more complete picture of a candidate's Ruby skills and practical coding abilities.
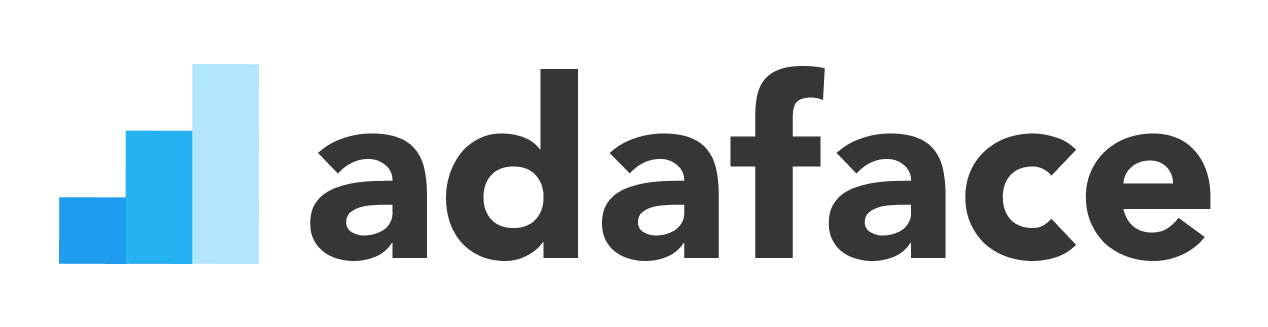
40 min skill tests.
No trick questions.
Accurate shortlisting.
We make it easy for you to find the best candidates in your pipeline with a 40 min skills test.
Try for freeRelated posts
Free resources
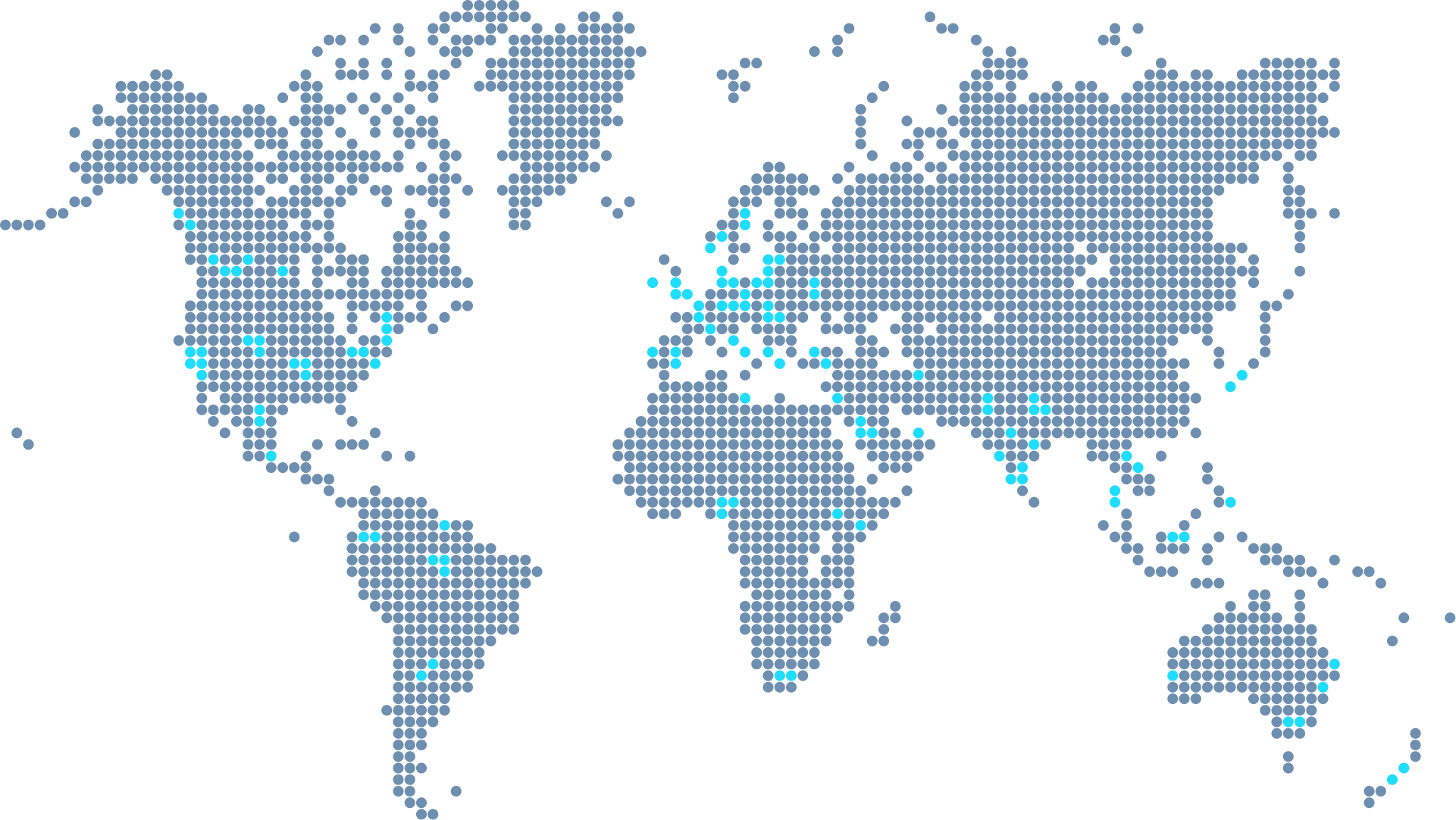
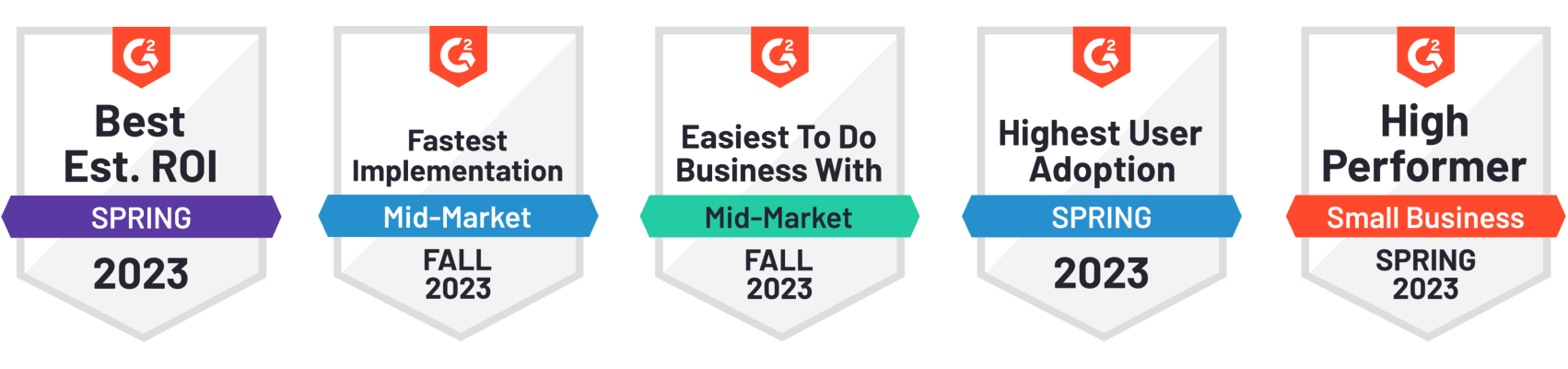