REST APIs are the backbone of modern web applications, making it crucial for developers to have a strong grasp of this technology. As an interviewer, asking the right REST API questions can help you identify candidates who truly understand the concepts and can apply them effectively in real-world scenarios.
This blog post provides a comprehensive list of REST API interview questions, categorized by difficulty level and topic. From basic concepts to advanced implementations, we cover questions suitable for junior, mid-tier, and senior developers, as well as technical definitions and process-related inquiries.
By using these questions, you can effectively assess a candidate's REST API knowledge and problem-solving skills. Consider combining these interview questions with a REST API skills test to get a more complete picture of a candidate's abilities before making your hiring decision.
Table of contents
10 basic REST API interview questions and answers to assess candidates
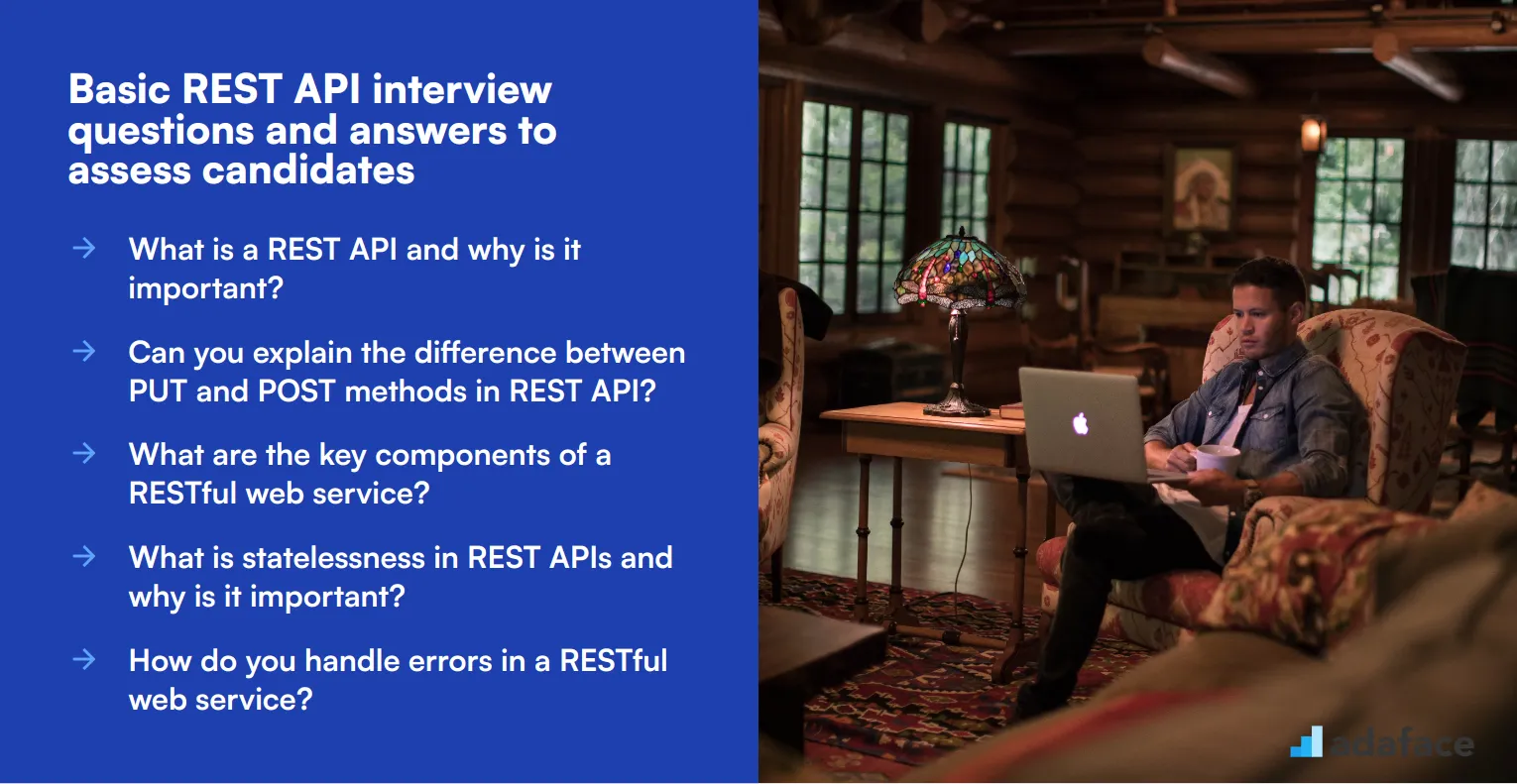
To evaluate whether your candidates have a solid grasp of REST API fundamentals, use these essential interview questions. They’ll help you gauge their understanding of core concepts and practical applications, ensuring you find the right fit for your team.
1. What is a REST API and why is it important?
A REST API (Representational State Transfer Application Programming Interface) is a set of rules and conventions for building and interacting with web services. It relies on stateless, client-server communication and uses HTTP methods such as GET, POST, PUT, and DELETE for operations.
REST APIs are important because they promote scalability, simplicity, and flexibility in web services development. They enable different systems to communicate and exchange data seamlessly, regardless of the underlying architecture.
Look for candidates who can explain the basic principles of REST and why it’s widely adopted. An ideal response should mention scalability, simplicity, and flexibility.
2. Can you explain the difference between PUT and POST methods in REST API?
The PUT method is used to update a resource or create a resource if it doesn’t exist. It’s idempotent, meaning multiple identical requests should have the same effect as a single request. Essentially, PUT replaces the resource at the given URL with the payload provided in the request.
On the other hand, the POST method is used to create a new resource. It’s not idempotent, meaning multiple identical requests may result in multiple new resources. POST typically adds a new item to a collection of resources.
Candidates should highlight the idempotent nature of PUT and the non-idempotent behavior of POST. This shows their understanding of how these methods are used effectively in RESTful services.
3. What are the key components of a RESTful web service?
The key components of a RESTful web service include:
- Resources: Entities or objects that the service manages, represented by URIs (Uniform Resource Identifiers).
- HTTP Methods: The actions that can be performed on resources, such as GET, POST, PUT, and DELETE.
- Representation: The format in which resources are represented, often using JSON or XML.
- Statelessness: Each request from a client contains all the information needed to process the request, with no reliance on previous interactions.
- Hypermedia: Links to related resources, allowing clients to navigate the API.
Look for candidates who can clearly identify and explain these components, demonstrating a comprehensive understanding of RESTful web services.
4. What is statelessness in REST APIs and why is it important?
Statelessness means that each request from a client to a server must contain all the information needed to understand and process the request. The server does not store any context or state information between requests.
Statelessness is important because it simplifies the server design, improves scalability, and makes it easier to handle and route requests. Each request is independent, which means that servers can be added or removed without affecting the overall system.
Candidates should emphasize the benefits of statelessness, such as simplification of server design and improved scalability. This shows their understanding of why REST APIs are built this way.
5. How do you handle errors in a RESTful web service?
Error handling in a RESTful web service typically involves using standard HTTP status codes to indicate the result of a request. Common status codes include 200 (OK), 201 (Created), 400 (Bad Request), 401 (Unauthorized), 404 (Not Found), and 500 (Internal Server Error).
In addition to status codes, the response body can include more detailed error messages or codes to provide additional context to the client. This helps clients understand what went wrong and how to address the issue.
Look for candidates who can explain the use of HTTP status codes and detailed error messages in response bodies. This indicates their ability to design robust and user-friendly APIs.
6. Can you explain the concept of resource representation in REST?
Resource representation in REST refers to the format in which resources are presented to clients. This is typically done using standard data formats like JSON or XML. The representation includes the data of the resource as well as metadata about the resource.
The representation allows clients to understand the structure and properties of the resource. Clients can request specific representations through content negotiation by specifying the desired format in the Accept
header of the HTTP request.
Candidates should be able to explain the importance of resource representation and how content negotiation works. This shows their understanding of delivering data in a flexible and client-friendly manner.
7. What is HATEOAS, and why is it significant in RESTful APIs?
HATEOAS (Hypermedia As The Engine Of Application State) is a constraint of RESTful APIs that allows clients to interact with the application entirely through hypermedia provided dynamically by the server. This means that the server includes links in its responses to guide clients through the available actions.
HATEOAS is significant because it decouples clients from server implementation details, allowing for more flexible and maintainable client-server interactions. Clients do not need to know the URI structure upfront; they can discover available actions via the links provided.
Look for candidates who understand HATEOAS and can explain how it enhances the flexibility and maintainability of RESTful APIs. This indicates their knowledge of advanced REST concepts.
8. Describe the role of HTTP headers in RESTful web services.
HTTP headers play a crucial role in RESTful web services by providing meta-information about the request or response. They can include details such as content type, content length, authentication credentials, caching directives, and more.
For example, the Content-Type
header indicates the media type of the resource (e.g., JSON, XML), while the Authorization
header is used to pass authentication credentials. Headers like Cache-Control
and ETag
help manage caching and resource versioning.
Candidates should highlight the importance of HTTP headers in conveying essential information and controlling aspects of communication. This shows their understanding of how to effectively use headers in RESTful APIs.
9. What are some common security considerations for REST APIs?
Common security considerations for REST APIs include:
- Authentication and Authorization: Ensuring that only authenticated users can access the API and that they have the appropriate permissions.
- Encryption: Using HTTPS to encrypt data in transit, protecting it from eavesdropping and tampering.
- Rate Limiting: Preventing abuse by limiting the number of requests a client can make within a certain period.
- Input Validation: Validating and sanitizing input to prevent injection attacks and other malicious activities.
- Error Handling: Avoiding the exposure of sensitive information in error messages.
Look for candidates who can discuss these considerations and understand the importance of securing RESTful APIs. This indicates their awareness of potential vulnerabilities and their ability to implement best practices.
10. How do you version a REST API, and why is it necessary?
Versioning a REST API involves assigning version numbers to different iterations of the API to manage changes and ensure backward compatibility. Common methods include:
- URI Versioning: Including the version number in the URI (e.g.,
/api/v1/resource
).
- Header Versioning: Specifying the version number in a custom HTTP header (e.g.,
X-API-Version: 1
).
- Query Parameter Versioning: Adding the version number as a query parameter (e.g.,
/api/resource?version=1
).
Versioning is necessary to manage changes without disrupting existing clients. It allows developers to introduce new features and improvements while maintaining support for older versions.
Candidates should explain the different versioning strategies and the importance of backward compatibility. This shows their understanding of how to evolve an API without breaking existing integrations.
20 REST API interview questions to ask junior developers
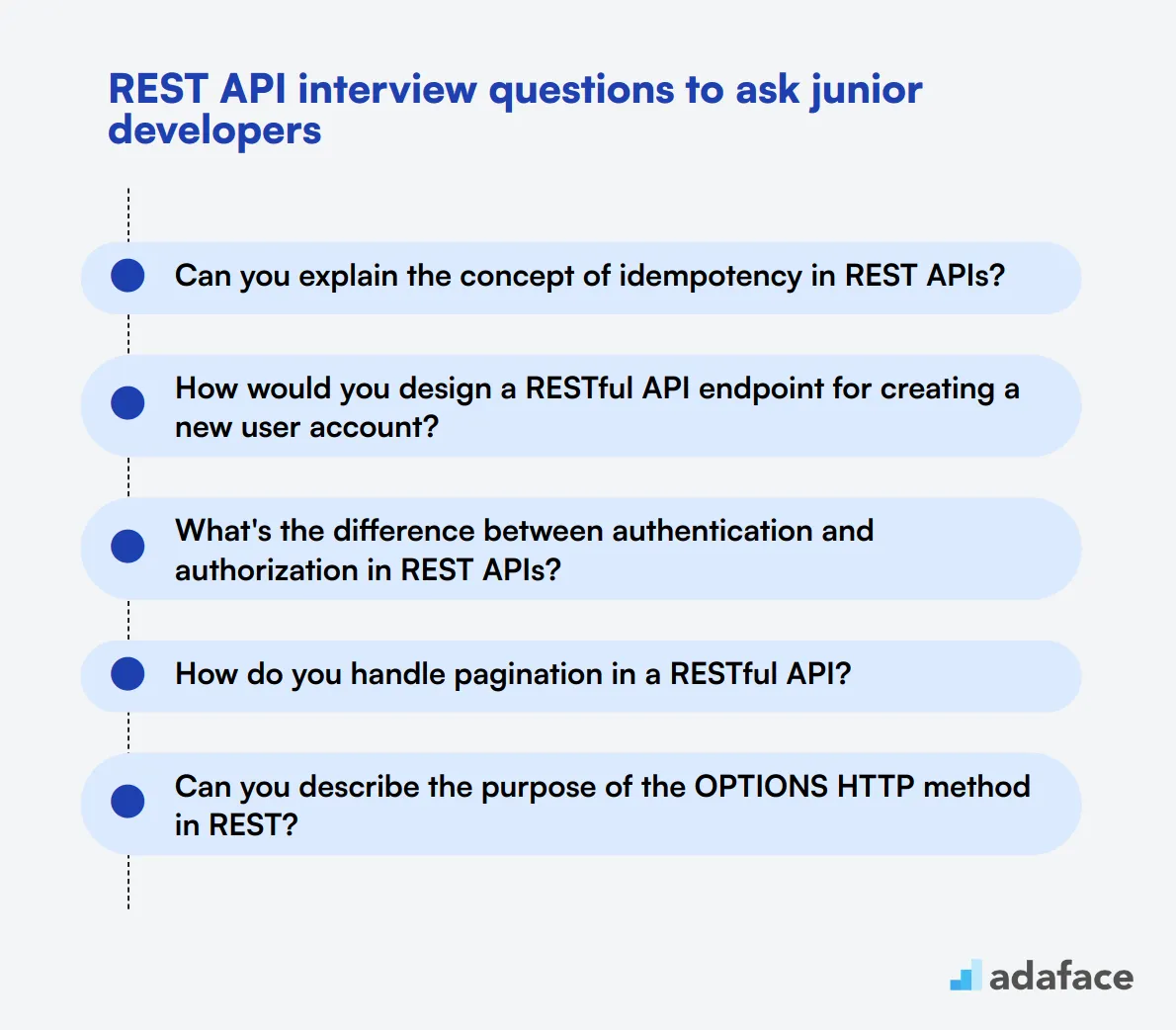
When interviewing junior software developers, it's crucial to assess their understanding of REST APIs. These questions will help you gauge their basic knowledge and practical skills. Use them to identify candidates who can contribute effectively to your API development projects.
- Can you explain the concept of idempotency in REST APIs?
- How would you design a RESTful API endpoint for creating a new user account?
- What's the difference between authentication and authorization in REST APIs?
- How do you handle pagination in a RESTful API?
- Can you describe the purpose of the OPTIONS HTTP method in REST?
- What are the benefits of using JSON Web Tokens (JWT) in REST APIs?
- How would you implement rate limiting in a REST API?
- Can you explain what CORS is and why it's important for REST APIs?
- What's the significance of the Accept and Content-Type headers in REST API requests?
- How would you design a RESTful API endpoint for updating a user's profile information?
- Can you describe the difference between synchronous and asynchronous API calls?
- What are some best practices for naming REST API endpoints?
- How would you handle file uploads in a RESTful API?
- Can you explain the concept of idempotency in REST APIs?
- What's the purpose of using status codes in REST API responses?
- How would you implement search functionality in a RESTful API?
- Can you describe the difference between REST and GraphQL?
- What are some common tools or libraries you've used for testing REST APIs?
- How would you handle long-running operations in a RESTful API?
- Can you explain the concept of API throttling and when it's useful?
10 intermediate REST API interview questions and answers to ask mid-tier developers
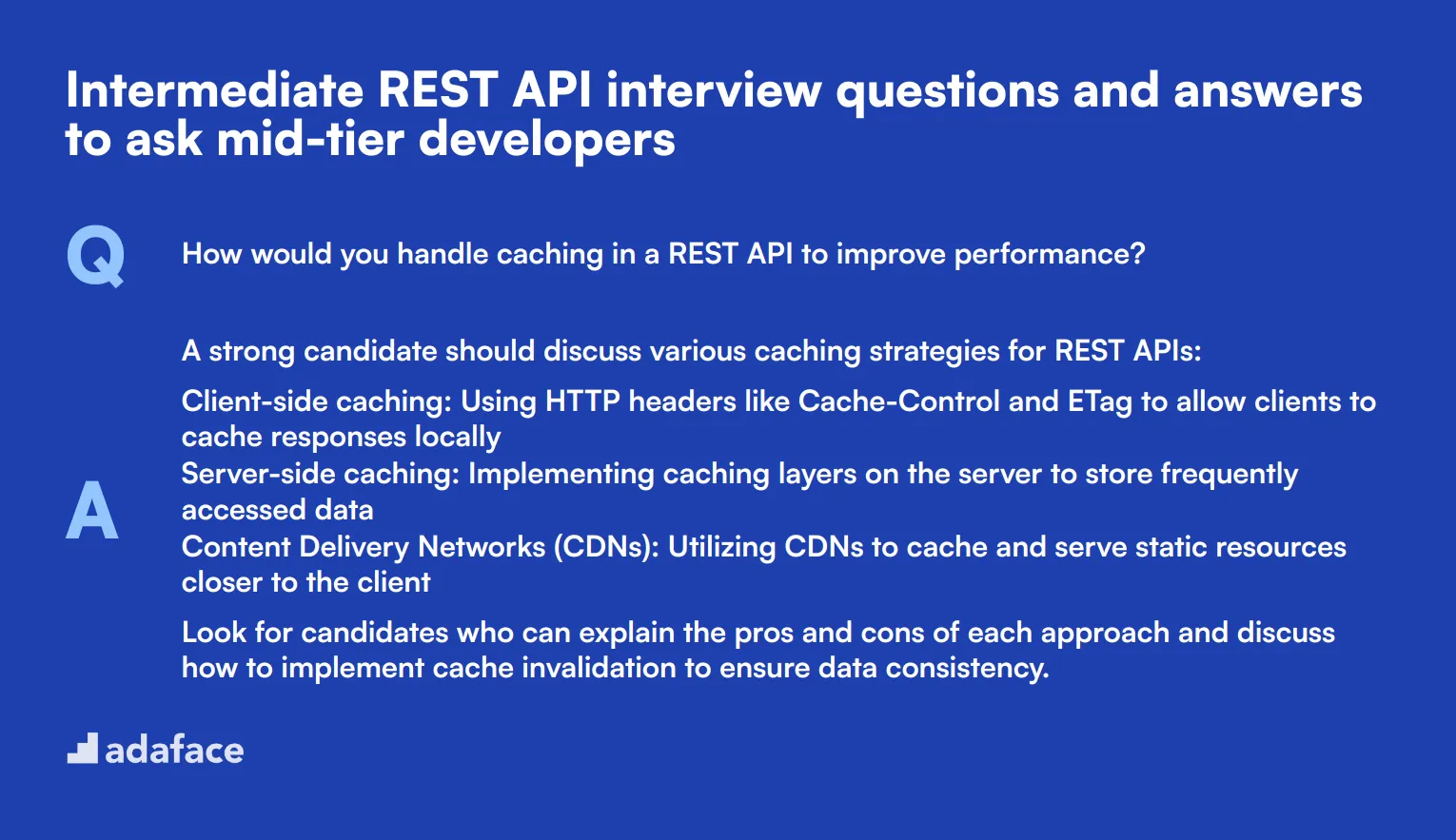
Ready to level up your REST API interviews? These 10 intermediate questions are perfect for assessing mid-tier developers. They'll help you gauge candidates' understanding of REST principles and their ability to apply them in real-world scenarios. Use these questions to spark discussions and uncover valuable insights about your potential hires.
1. How would you handle caching in a REST API to improve performance?
A strong candidate should discuss various caching strategies for REST APIs:
- Client-side caching: Using HTTP headers like Cache-Control and ETag to allow clients to cache responses locally
- Server-side caching: Implementing caching layers on the server to store frequently accessed data
- Content Delivery Networks (CDNs): Utilizing CDNs to cache and serve static resources closer to the client
Look for candidates who can explain the pros and cons of each approach and discuss how to implement cache invalidation to ensure data consistency.
2. Can you explain the concept of hypermedia-driven REST APIs?
Hypermedia-driven REST APIs, also known as HATEOAS (Hypertext As The Engine Of Application State), is an approach where the API provides links to related resources in its responses. This allows clients to navigate the API dynamically without prior knowledge of the entire API structure.
Candidates should be able to explain:
- How hypermedia links are included in API responses
- The benefits of this approach, such as improved discoverability and flexibility
- Potential challenges in implementing HATEOAS, like increased response size and client complexity
Look for answers that demonstrate an understanding of how HATEOAS can make APIs more self-descriptive and easier to evolve over time.
3. How would you implement API versioning, and what are the trade-offs of different approaches?
Candidates should discuss common API versioning strategies:
- URL versioning: Including the version in the URL (e.g., /api/v1/users)
- Header versioning: Using custom headers to specify the version
- Query parameter versioning: Adding a version parameter to the query string
- Content negotiation: Using Accept headers to request specific versions
Strong answers will include pros and cons of each approach. For example, URL versioning is simple but can lead to duplication, while header versioning is cleaner but may be less visible.
Look for candidates who can explain how versioning impacts API maintenance and backward compatibility, and who can recommend an approach based on specific project needs.
4. Explain the concept of idempotency in REST APIs and why it's important.
Idempotency in REST APIs means that making multiple identical requests should have the same effect as making a single request. This property is crucial for ensuring the reliability and consistency of API operations, especially in distributed systems where network issues may cause retries.
Candidates should explain:
- Which HTTP methods are typically idempotent (GET, PUT, DELETE) and which are not (POST)
- How idempotency helps in handling network failures and retries
- Strategies for implementing idempotency, such as using unique request identifiers
Look for answers that demonstrate an understanding of how idempotency impacts API design and error handling, and how it contributes to building robust and fault-tolerant systems.
5. How would you design a RESTful API for a social media platform's newsfeed feature?
This question tests a candidate's ability to apply REST principles to a real-world scenario. A good answer might include:
- Endpoint design: /api/v1/users/{userId}/feed for fetching a user's newsfeed
- Query parameters for pagination, filtering, and sorting
- Resource representations: JSON structure for newsfeed items, including content, author, timestamp, etc.
- Proper use of HTTP methods: GET for fetching, POST for creating new posts, etc.
- Considerations for performance, such as pagination and caching strategies
Look for candidates who can explain their design choices, discuss potential scalability challenges, and consider features like real-time updates or personalization in their API design.
6. What are the key considerations when designing a RESTful API for mobile applications?
When designing APIs for mobile apps, candidates should consider:
- Bandwidth efficiency: Minimizing data transfer to conserve mobile data
- Battery impact: Optimizing requests to reduce battery drain
- Offline support: Designing the API to support offline-first architectures
- Versioning: Ensuring backward compatibility for users who haven't updated their apps
- Security: Implementing proper authentication and encryption for sensitive data
Strong answers will also discuss strategies like response compression, efficient pagination, and optimizing payload size. Look for candidates who understand the unique challenges of mobile environments and can propose solutions to address them.
7. How would you implement rate limiting in a REST API, and why is it important?
Rate limiting is crucial for protecting APIs from abuse and ensuring fair usage. Candidates should explain:
- Implementation strategies: Token bucket, fixed window, or sliding window algorithms
- Where to apply rate limits: By IP address, API key, or user account
- How to communicate limits to clients: Using HTTP headers like X-RateLimit-Limit and X-RateLimit-Remaining
- Handling limit exceedance: Returning 429 Too Many Requests status code with retry-after information
Look for answers that discuss the balance between protecting the API and providing a good user experience. Candidates should also mention considerations like distributed rate limiting for scalable systems and how to handle rate limiting in microservices architectures.
8. Explain the concept of content negotiation in REST APIs and how it's implemented.
Content negotiation allows clients to request specific data formats from the API. Candidates should explain:
- The use of Accept and Content-Type headers for negotiation
- Common formats like JSON, XML, and how to support multiple formats
- Server-driven vs. agent-driven negotiation
- How content negotiation affects API versioning and backward compatibility
Strong answers will include examples of how to implement content negotiation in popular frameworks and discuss best practices for handling unsupported formats. Look for candidates who understand how content negotiation enhances API flexibility and interoperability.
9. How would you design a RESTful API to handle long-running operations?
Handling long-running operations in REST APIs requires careful design. Candidates should discuss:
- Asynchronous processing: Accepting the request and returning a 202 Accepted status
- Job resources: Creating a separate endpoint to check the status of long-running jobs
- Webhooks: Allowing clients to register callbacks for job completion notifications
- Polling: Providing an endpoint for clients to periodically check job status
Look for answers that consider the trade-offs between different approaches, such as the simplicity of polling vs. the efficiency of webhooks. Candidates should also mention error handling, timeout considerations, and how to design the API to be resilient to client or server failures during long-running operations.
10. What are the best practices for error handling and communicating errors in REST APIs?
Effective error handling is crucial for API usability. Candidates should discuss:
- Using appropriate HTTP status codes (e.g., 400 for client errors, 500 for server errors)
- Providing detailed error messages in the response body
- Consistent error response format across the API
- Including error codes or identifiers for easier troubleshooting
- Logging errors on the server side for debugging
Strong answers will also mention the importance of not exposing sensitive information in error messages and how to handle unexpected errors gracefully. Look for candidates who understand the balance between providing helpful information to API consumers and maintaining security and simplicity in the API design.
10 advanced REST API interview questions to ask senior developers
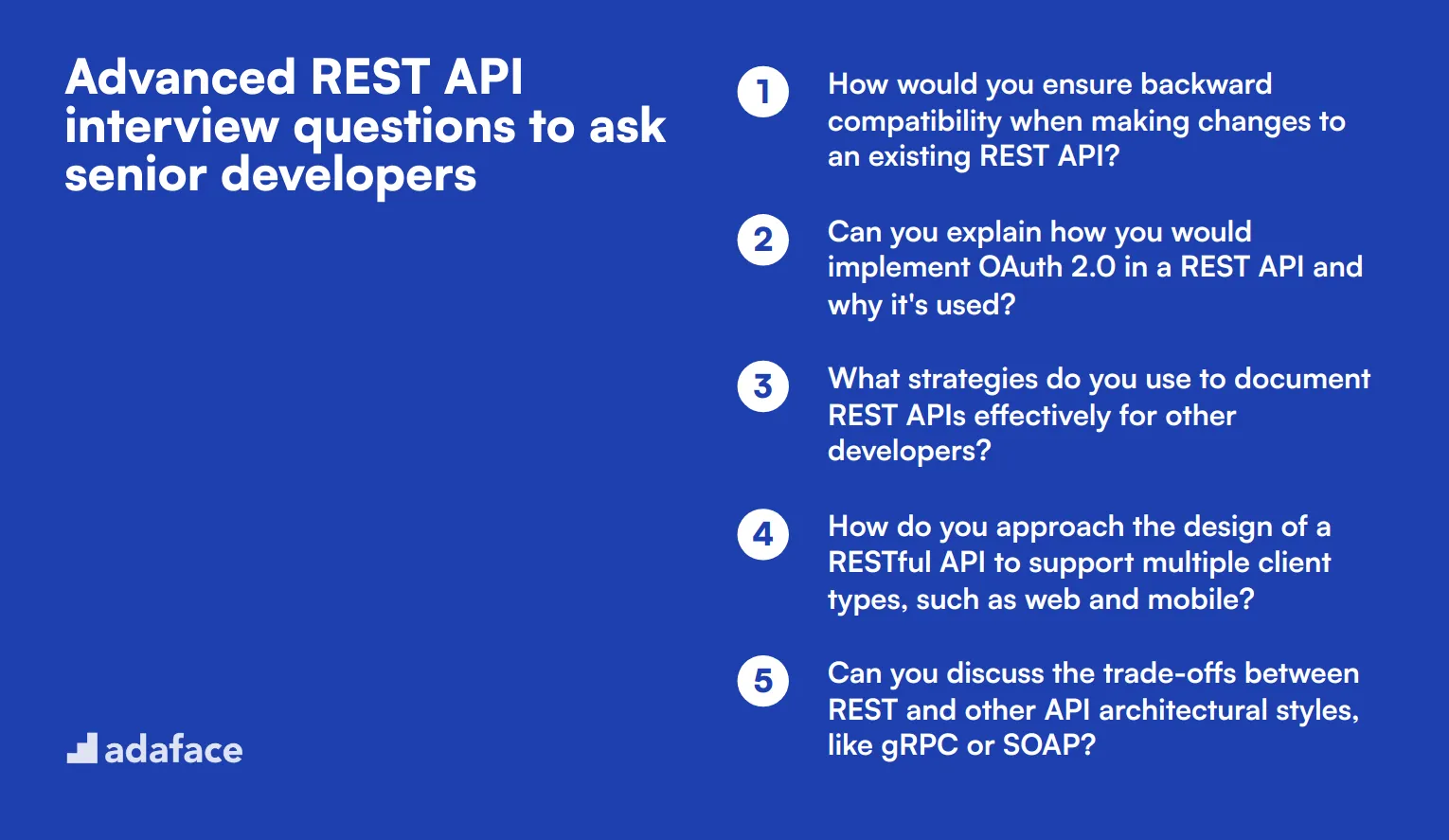
To assess if senior candidates possess the necessary expertise in REST API design and implementation, utilize these advanced questions during interviews. Tailor your approach based on the specific role, like a software developer, to gauge their technical understanding effectively.
- How would you ensure backward compatibility when making changes to an existing REST API?
- Can you explain how you would implement OAuth 2.0 in a REST API and why it's used?
- What strategies do you use to document REST APIs effectively for other developers?
- How do you approach the design of a RESTful API to support multiple client types, such as web and mobile?
- Can you discuss the trade-offs between REST and other API architectural styles, like gRPC or SOAP?
- How do you handle API deprecation, and what communication strategies do you use to inform your users?
- What are some techniques you can implement to enhance the performance of a REST API?
- Can you explain how you would implement a search filter in a RESTful service?
- What is the role of API gateways in a microservices architecture, particularly for REST APIs?
- How would you manage database transactions in a RESTful API to ensure data integrity?
6 REST API interview questions and answers related to technical definitions
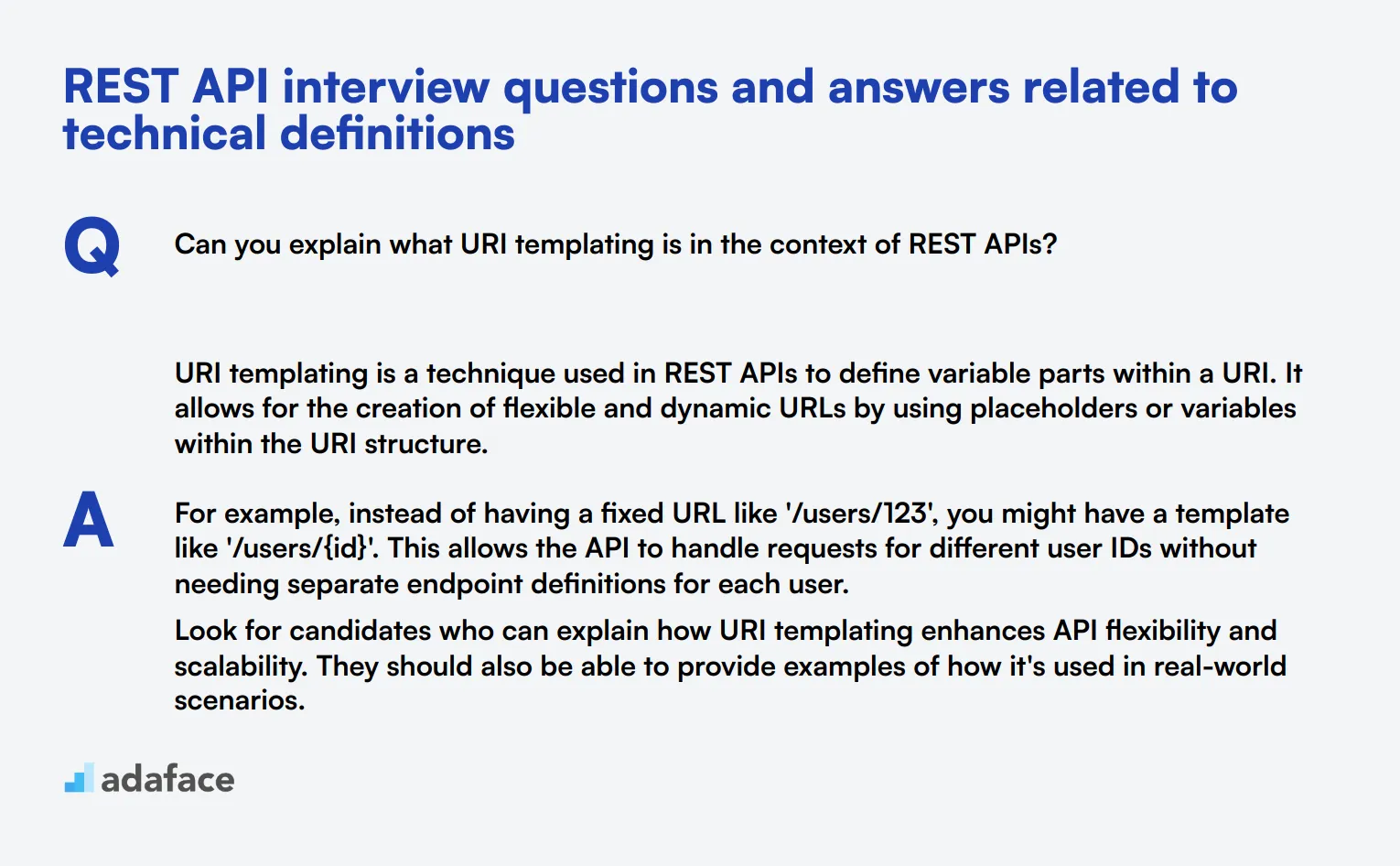
When interviewing for REST API developers, it's crucial to assess their understanding of technical definitions. These questions will help you gauge candidates' knowledge of key REST API concepts, allowing you to identify those who can effectively design and implement robust APIs. Use this list to delve into the technical aspects of REST without getting bogged down in code specifics.
1. Can you explain what URI templating is in the context of REST APIs?
URI templating is a technique used in REST APIs to define variable parts within a URI. It allows for the creation of flexible and dynamic URLs by using placeholders or variables within the URI structure.
For example, instead of having a fixed URL like '/users/123', you might have a template like '/users/{id}'. This allows the API to handle requests for different user IDs without needing separate endpoint definitions for each user.
Look for candidates who can explain how URI templating enhances API flexibility and scalability. They should also be able to provide examples of how it's used in real-world scenarios.
2. What is the difference between URI and URL in the context of REST APIs?
URI (Uniform Resource Identifier) is a broader term that includes both URLs and URNs. It's used to identify a resource, whether by location, name, or both. URL (Uniform Resource Locator), on the other hand, is a specific type of URI that provides the means to locate and retrieve a resource on a network.
In the context of REST APIs, URIs are often used to identify resources, while URLs are used to locate and access those resources. For example, 'http://api.example.com/users/123' is both a URI and a URL, as it identifies a specific user resource and provides the location to access it.
Strong candidates should be able to explain this distinction clearly and provide examples of how URIs and URLs are used in API design. They might also mention that in modern web development, the terms are often used interchangeably, though technically they have different meanings.
3. Can you explain the concept of idempotency in REST APIs and provide an example?
Idempotency in REST APIs refers to the property where making multiple identical requests has the same effect as making a single request. This means that repeating an operation multiple times will not change the result beyond the initial application.
For example, a DELETE request to remove a specific resource should be idempotent. If you send a DELETE request to '/users/123' multiple times, the first request will delete the user, and subsequent requests will have no additional effect (assuming the user isn't recreated between requests).
Look for candidates who can explain why idempotency is important for API reliability and error handling. They should be able to differentiate between idempotent methods (like GET, PUT, DELETE) and non-idempotent methods (like POST). Ask for additional examples to gauge their understanding of how idempotency applies in various API operations.
4. What is content negotiation in REST APIs and why is it important?
Content negotiation is a mechanism in REST APIs that allows clients and servers to agree on the most appropriate format for exchanging data. It enables the same resource to be represented in different formats (such as JSON, XML, or HTML) based on the client's preferences or capabilities.
This process typically involves the client sending headers like 'Accept' to specify preferred formats, and the server responding with the 'Content-Type' header to indicate the format of the returned data. For example, a client might send 'Accept: application/json' to request JSON data, and the server would respond with 'Content-Type: application/json' along with the JSON-formatted data.
Strong candidates should emphasize the importance of content negotiation in creating flexible and client-friendly APIs. They might discuss how it enhances interoperability, allows for backward compatibility, and improves the overall user experience. Ask them about potential challenges in implementing content negotiation and how they would address them.
5. Explain the concept of resource expansion in REST APIs.
Resource expansion, also known as embedded resources or compound documents, is a technique used in REST APIs to include related resources in the response of a single API call. This approach can reduce the number of requests a client needs to make to gather related data.
For example, when requesting information about a user, the API might allow you to expand the response to include details about the user's orders. Instead of making separate calls for '/users/123' and '/users/123/orders', you might use a query parameter like '/users/123?expand=orders' to get all the information in one request.
Look for candidates who can discuss the benefits of resource expansion, such as improved performance and reduced network overhead. They should also be aware of potential drawbacks, like increased response size and complexity in API design. Ask how they would implement this feature while maintaining API simplicity and flexibility.
6. What is the difference between statelessness and statefulness in the context of REST APIs?
Statelessness is a key principle of REST APIs, meaning that each request from a client to a server must contain all the information needed to understand and process the request. The server doesn't store any client context between requests. Each request is treated as an independent transaction, unrelated to any previous requests.
Statefulness, on the other hand, involves the server keeping track of the client's state across multiple requests. This might involve storing session information or remembering the client's previous actions.
Candidates should explain that REST APIs are designed to be stateless for scalability and reliability. They might discuss how statelessness simplifies server-side architecture, enables better load balancing, and improves fault tolerance. Ask them to provide examples of how to handle scenarios that seem to require state (like authentication) in a stateless manner, such as using tokens or client-side storage.
5 REST API interview questions and answers related to processes
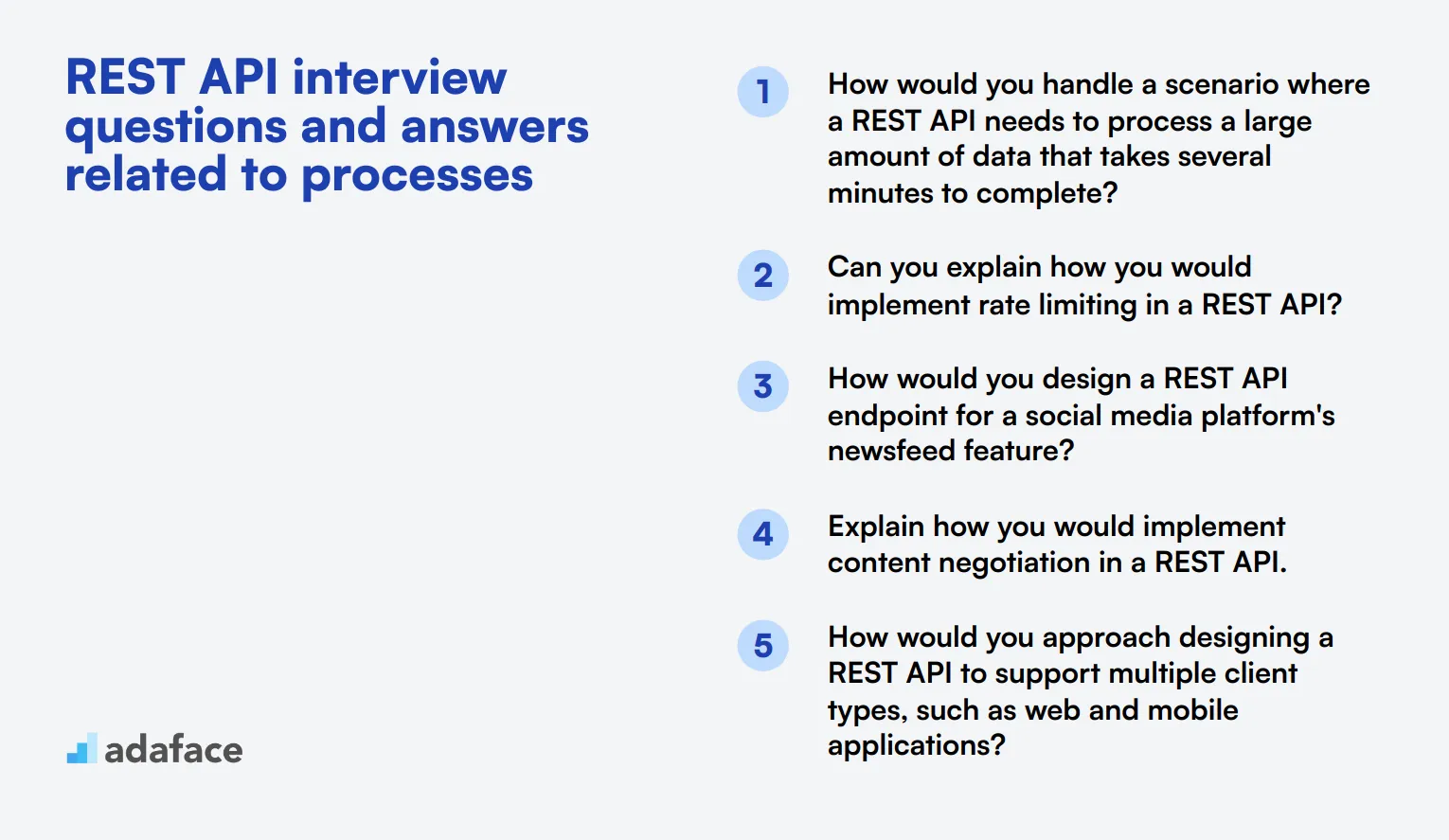
To assess whether your candidates truly understand the intricacies of REST API processes, consider using these carefully crafted interview questions. These questions will help you gauge a candidate's practical knowledge and problem-solving skills related to REST API implementation and management. Remember, the goal is to spark meaningful discussions and gain insights into the candidate's thought process.
1. How would you handle a scenario where a REST API needs to process a large amount of data that takes several minutes to complete?
When dealing with long-running processes in REST APIs, there are several approaches a candidate might suggest:
- Asynchronous processing: Implement a job queue system where the API immediately returns a job ID or status URL. The client can then poll this endpoint to check the progress.
- Webhooks: Once the process is complete, the server can send a callback to a pre-defined URL provided by the client.
- Streaming: For data that can be processed in chunks, consider implementing a streaming API to send partial results as they become available.
Look for candidates who can explain the trade-offs between these approaches and discuss factors like scalability, client experience, and error handling. A strong candidate might also mention the importance of clear documentation for these long-running operations to ensure proper client implementation.
2. Can you explain how you would implement rate limiting in a REST API?
Implementing rate limiting in a REST API involves several key steps:
- Define limits: Determine the number of requests allowed per time period (e.g., 100 requests per minute).
- Track requests: Use a database or in-memory store to keep track of client requests.
- Implement limiting logic: Check the request count against the defined limit before processing each request.
- Return appropriate headers: Include headers like 'X-RateLimit-Limit', 'X-RateLimit-Remaining', and 'X-RateLimit-Reset' in API responses.
- Handle limit exceeded: Return a 429 (Too Many Requests) status code when the limit is exceeded, along with information on when the limit will reset.
A strong candidate should be able to discuss different rate limiting strategies (e.g., fixed window, sliding window, token bucket) and their pros and cons. They might also mention considerations for distributed systems and how to handle rate limiting across multiple API servers.
3. How would you design a REST API endpoint for a social media platform's newsfeed feature?
When designing a REST API endpoint for a social media platform's newsfeed, candidates should consider the following aspects:
- Endpoint structure:
/api/v1/newsfeed
or/api/v1/users/{userId}/feed
- Query parameters: Include options for pagination, filtering (e.g., by date, post type), and sorting.
- Response format: Return a JSON object containing an array of feed items, each with properties like post content, author, timestamp, and engagement metrics.
- Pagination: Implement cursor-based pagination for efficient scrolling through large datasets.
- Caching: Utilize appropriate caching strategies to improve performance and reduce server load.
Look for candidates who discuss scalability concerns, such as handling high request volumes and optimizing database queries. They should also mention considerations for real-time updates, perhaps suggesting WebSocket integration for live feed updates. A strong candidate might also bring up privacy controls and how to efficiently filter content based on user relationships and preferences.
4. Explain how you would implement content negotiation in a REST API.
Content negotiation in REST APIs allows clients to request specific data formats. Here's how it can be implemented:
- Accept header: Use the 'Accept' header in client requests to specify desired content types (e.g., 'application/json', 'application/xml').
- Server-side logic: Implement logic to check the 'Accept' header and format the response accordingly.
- Content-Type header: Set the 'Content-Type' header in the response to indicate the format of the returned data.
- Default format: Provide a default format (usually JSON) if no 'Accept' header is specified or if the requested format is not supported.
- Error handling: Return a 406 (Not Acceptable) status code if the requested format cannot be provided.
A strong candidate should discuss the benefits of content negotiation, such as improved API flexibility and client satisfaction. They might also mention versioning considerations and how content negotiation can be used alongside or as an alternative to URL-based versioning. Look for candidates who understand the importance of clear documentation for supported content types and how to handle edge cases in content negotiation.
5. How would you approach designing a REST API to support multiple client types, such as web and mobile applications?
Designing a REST API for multiple client types requires careful consideration of various factors:
- Consistent data model: Create a unified data model that works across all client types.
- Flexible endpoints: Design endpoints that can serve both web and mobile needs, possibly using query parameters to customize responses.
- Versioning: Implement API versioning to support different client versions and allow for future changes.
- Performance optimization: Consider implementing techniques like response compression and partial responses to accommodate mobile network limitations.
- Authentication: Use a flexible authentication system (e.g., OAuth 2.0) that works well for both web and mobile clients.
Look for candidates who discuss the importance of thorough documentation and providing SDKs or client libraries for different platforms. They should also mention considerations for offline functionality in mobile apps and how to handle data synchronization. A strong candidate might bring up the concept of API-first design and how it can help create a more robust and flexible API that serves multiple client types effectively.
Which REST API skills should you evaluate during the interview phase?
It's impossible to gauge every aspect of a candidate’s skill set in a single interview, but for assessing REST API skills, focusing on core competencies can provide a clear picture of their abilities. These key skills are essential for understanding how well a candidate can design, develop, and maintain robust RESTful services.
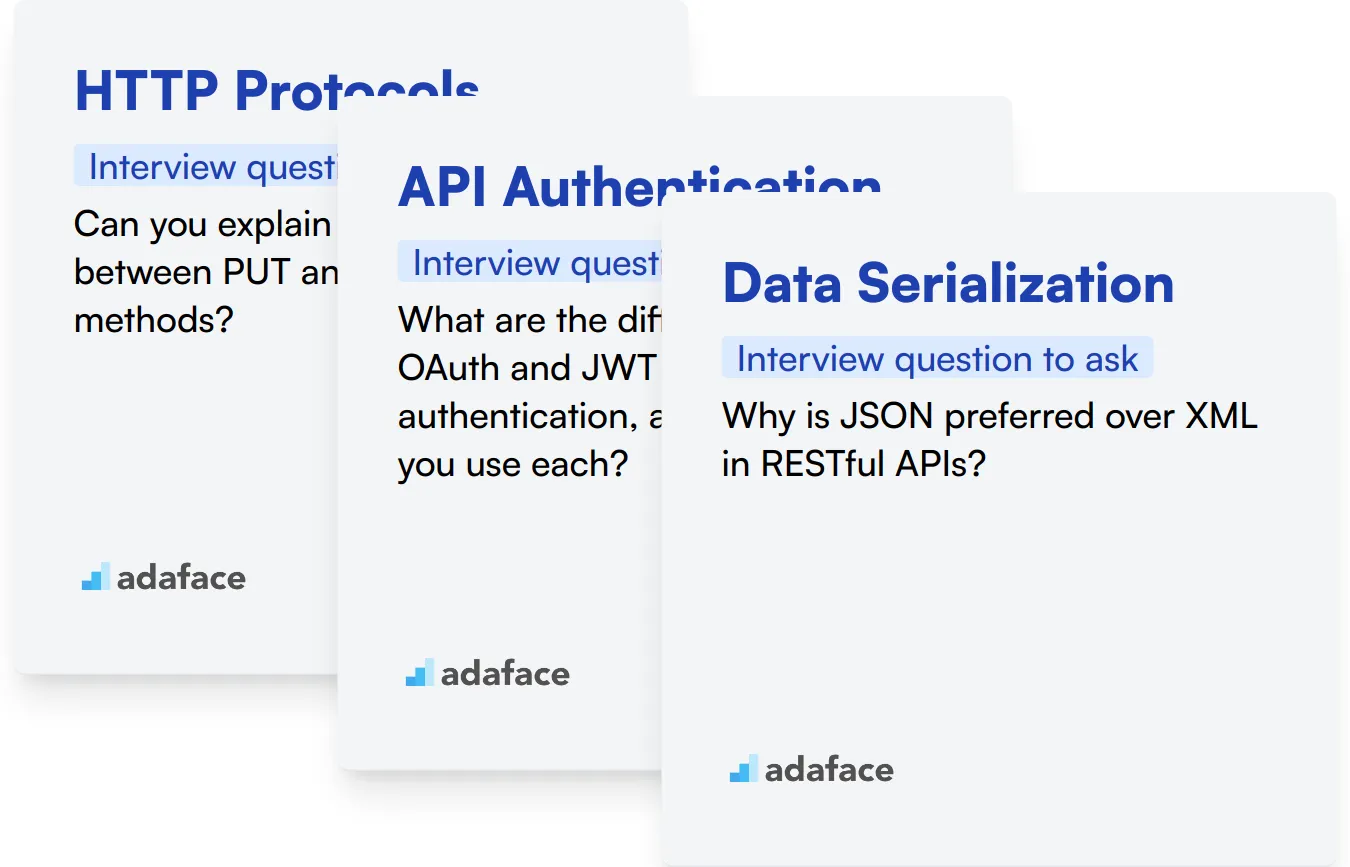
HTTP Protocols
Understanding HTTP protocols is essential for designing efficient REST APIs. HTTP methods such as GET, POST, PUT, and DELETE are the building blocks of RESTful services.
You can use an assessment test that asks relevant MCQs to filter out this skill. For instance, our REST API test covers various questions on HTTP methods.
Additionally, you can ask targeted interview questions to gauge their understanding of HTTP protocols.
Can you explain the difference between PUT and POST HTTP methods?
Look for a candidate who can explain the idempotent nature of PUT versus the non-idempotent nature of POST, highlighting their nuanced understanding.
API Authentication
API Authentication is crucial for securing REST APIs. Methods like OAuth, JWT, and API keys ensure that only authorized users can access the services.
You can use an assessment test that includes MCQs about different authentication methods. Our backend engineer test features questions on API security mechanisms.
Consider asking questions specifically about authentication to assess their grasp on securing APIs.
What are the differences between OAuth and JWT for API authentication, and when would you use each?
A strong candidate will discuss the use cases for both OAuth (for delegated access) and JWT (for stateless authentication), indicating their practical knowledge.
Data Serialization
Data serialization formats like JSON and XML play a critical role in data interchange in REST APIs. Proficiency in these formats ensures smooth communication between client and server.
You can use an assessment test that evaluates their knowledge of serialization. Our REST API test includes questions on JSON and XML.
Interview questions can also help evaluate their understanding of data serialization.
Why is JSON preferred over XML in RESTful APIs?
Expect the candidate to mention JSON's lightweight nature, ease of parsing, and compatibility with JavaScript, demonstrating their familiarity with current practices.
Hire top talent with REST API skills tests and the right interview questions
When hiring for REST API skills, it's important to ensure candidates possess the necessary expertise. A thorough assessment can help you determine if they truly have the skills required for your team.
The most accurate way to evaluate these skills is by using skill tests. Consider utilizing our REST API Test to effectively gauge candidates' knowledge and capabilities.
After conducting the test, you can shortlist the best applicants based on their performance and invite them for interviews. This process streamlines your hiring efforts and helps identify top candidates.
To get started, visit our test library to explore various assessments and sign up today. This will set you on the right path to hiring the best REST API talent.
REST API Test
Download REST API interview questions template in multiple formats
REST API Interview Questions FAQs
For junior developers, focus on basic concepts like HTTP methods, status codes, and API design principles. Our post includes 20 questions tailored for junior candidates.
Senior developers should be asked about advanced topics like security, scalability, and performance optimization. We provide 10 advanced questions for senior candidates.
Yes, our post includes 6 questions specifically related to REST API technical definitions to help you gauge candidates' theoretical knowledge.
The number of questions depends on the interview duration and candidate level. Our post offers 61 questions across different experience levels, allowing you to choose the most relevant ones.
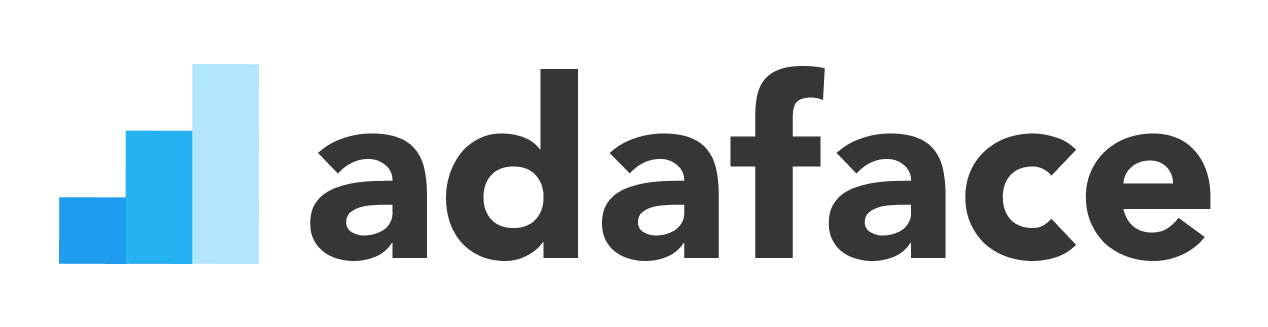
40 min skill tests.
No trick questions.
Accurate shortlisting.
We make it easy for you to find the best candidates in your pipeline with a 40 min skills test.
Try for freeRelated posts
Free resources
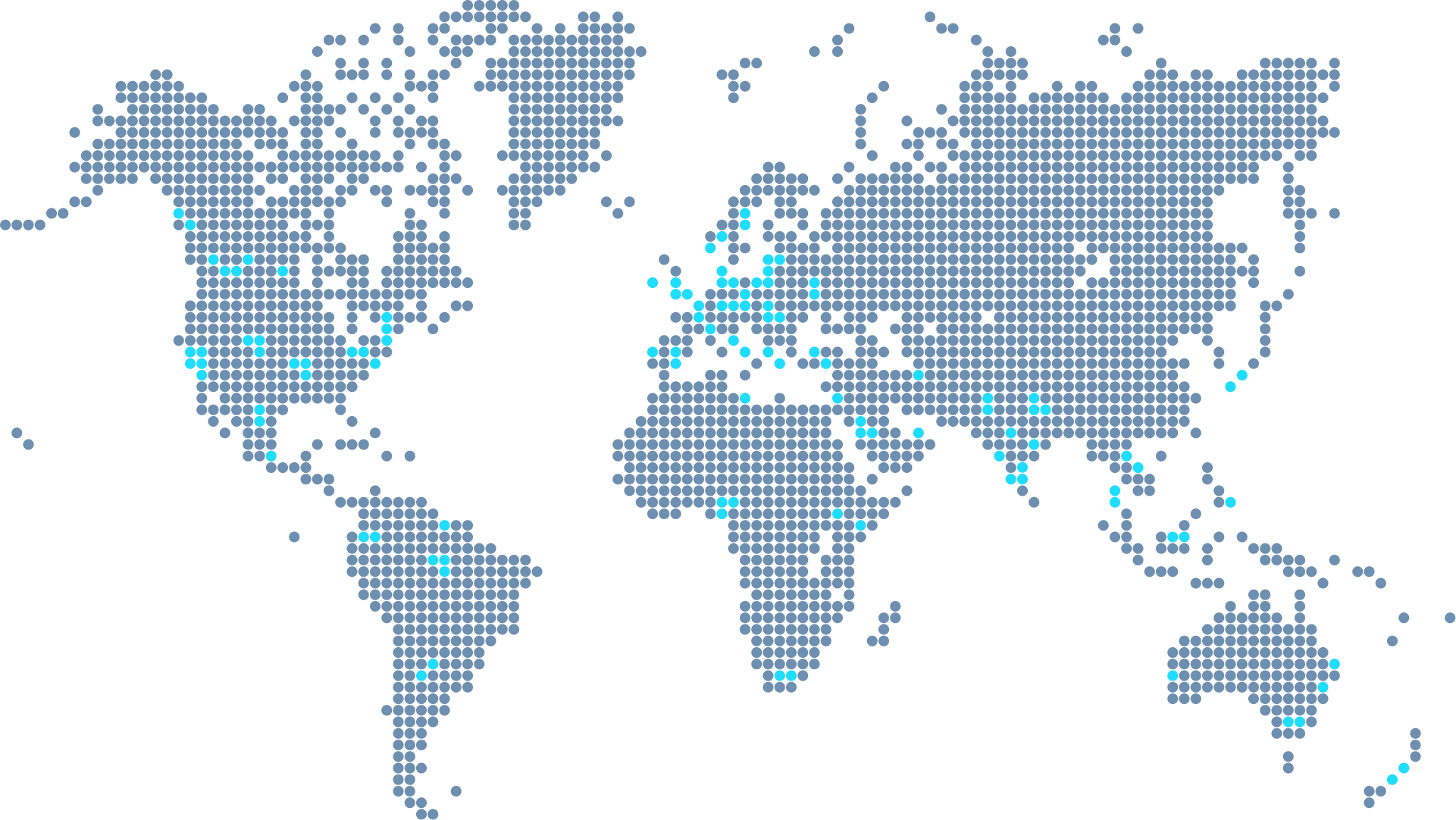
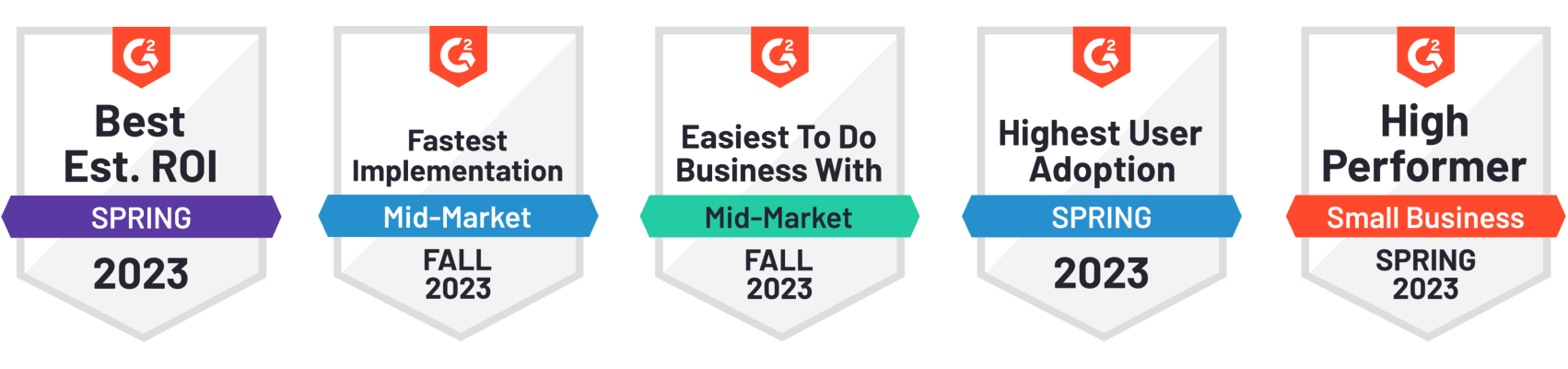