Hiring the right Redux developer can be a challenge for recruiters and hiring managers. Asking the right interview questions is key to assessing a candidate's knowledge and skills effectively.
This blog post provides a comprehensive list of Redux interview questions, categorized by difficulty level and topic. From basic concepts to advanced state management techniques, we cover everything you need to evaluate candidates across different experience levels.
By using these questions, you can gain deeper insights into a candidate's Redux expertise and make informed hiring decisions. Consider complementing your interview process with a React Redux test to get a holistic view of candidates' skills.
Table of contents
10 basic Redux interview questions and answers to assess candidates
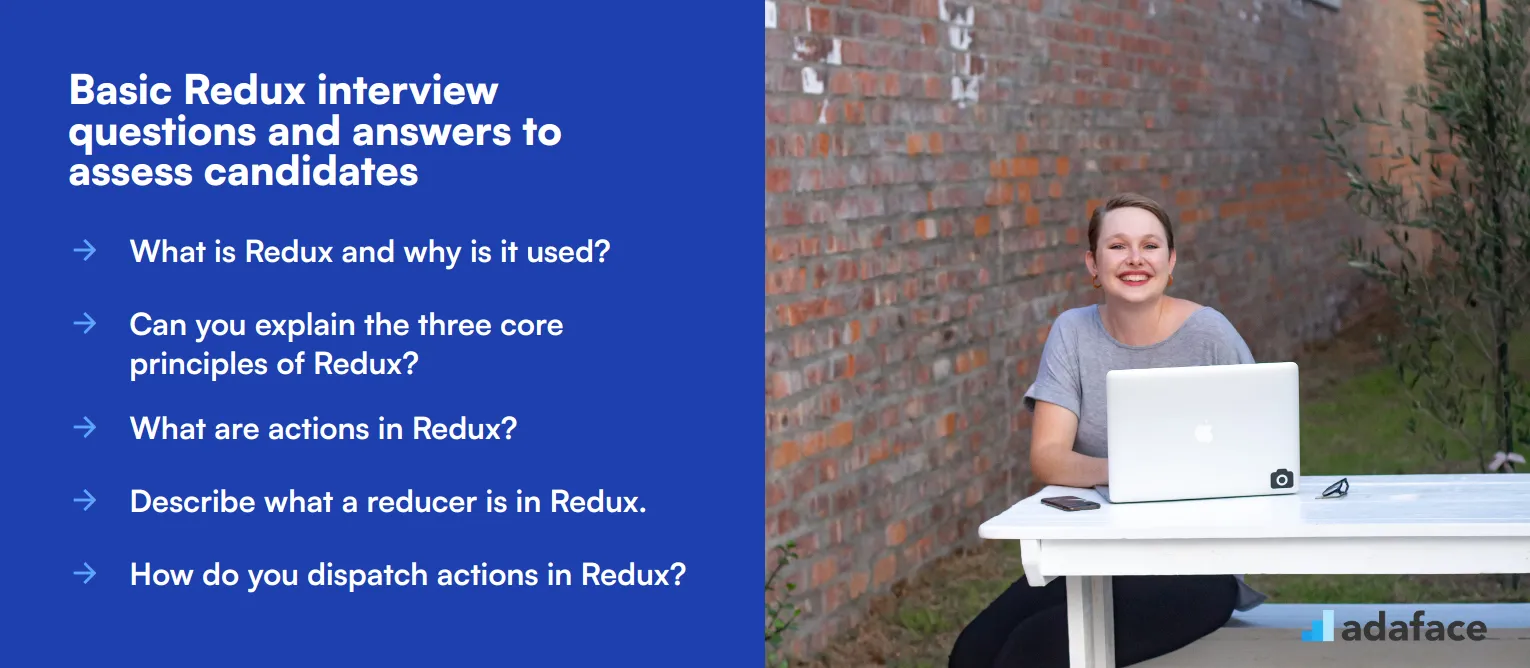
When you're hiring for a role that involves Redux, it’s crucial to assess candidates on their understanding of its basic concepts and applications. These interview questions will help you determine whether applicants have a solid grasp of Redux essentials, ensuring you bring the right talent on board.
1. What is Redux and why is it used?
Redux is a state management library for JavaScript applications. It helps manage the state of an application in a predictable way by using a single source of truth, which means that all the state is stored in a single, centralized location.
Redux is used to make state management predictable and easier to debug. It allows for more efficient state updates and helps in maintaining a more organized and scalable codebase. An ideal candidate should explain that Redux is particularly useful in larger applications where managing state can become complex.
2. Can you explain the three core principles of Redux?
The three core principles of Redux are:
- Single Source of Truth: The state of the entire application is stored in a single object called the store.
- State is Read-Only: The only way to change the state is to dispatch an action, which describes the change.
- Changes are Made with Pure Functions: To specify how the state tree is transformed by actions, you write pure reducers.
Look for candidates who can clearly articulate these principles and how they contribute to the predictability and stability of an application using Redux.
3. What are actions in Redux?
Actions are plain JavaScript objects that have a type
field and optionally other fields to describe what happened in the application. They are the only source of information for the store.
Actions must have a type
property that indicates the type of action being performed. They can also contain other data that payload the action. Strong answers should note that actions are dispatched to trigger state changes in the Redux store.
4. Describe what a reducer is in Redux.
Reducers are pure functions that take the current state and an action as arguments, and return a new state. They specify how the application's state changes in response to actions sent to the store.
The key characteristic of reducers is that they must be pure functions, meaning they do not produce side effects and return the same output given the same input. Look for candidates who emphasize the importance of reducers in maintaining the predictability of state changes.
5. How do you dispatch actions in Redux?
To dispatch actions in Redux, you call the dispatch
function with an action object. This function is provided by the Redux store and can be called from anywhere within the application.
Candidates should mention that dispatching actions is the only way to trigger state changes in Redux. They might also elaborate on how middleware can be used to handle asynchronous actions.
6. What is the purpose of middleware in Redux?
Middleware in Redux provides a third-party extension point between dispatching an action and the moment it reaches the reducer. It is often used for logging, crash reporting, and handling asynchronous actions.
Middleware like redux-thunk
or redux-saga
can be implemented to manage complex state logic or side effects. A good candidate will explain how middleware enhances the functionality of Redux by allowing for more complex workflows.
7. How do you handle asynchronous operations in Redux?
Handling asynchronous operations in Redux typically involves middleware like redux-thunk
or redux-saga
. These tools allow you to write action creators that return functions or generators instead of plain objects.
redux-thunk
is simpler and allows for functions that dispatch actions after asynchronous operations. redux-saga
uses generator functions to handle more complex side effects. Candidates should discuss their experience with either middleware and how it helps manage async operations in Redux.
8. What is the role of the store in Redux?
The store is the central piece of Redux that holds the state of the application. It is created using a root reducer and an optional initial state. The store provides methods to dispatch actions, subscribe to state changes, and access the current state.
Candidates should describe how the store acts as a single source of truth for the application's state and simplifies state management. They might also mention how to configure the store with middleware and enhancers.
9. Explain the concept of selectors in Redux.
Selectors are functions that extract specific pieces of state from the Redux store. They help keep components decoupled from the store structure by providing a consistent way to access state.
Selectors can be simple or complex, depending on the data they need to retrieve. Good candidates will explain how selectors improve code maintainability and performance by memoizing computed data.
10. Can you describe how to structure a Redux application?
Structuring a Redux application typically involves organizing it by features or domains, with separate folders for actions, reducers, and selectors. Each feature might have its own set of actions, reducers, and components.
The goal is to keep the code modular and easy to maintain. Ideal candidates will discuss their preferred structure and how it helps manage complex applications. They might also reference best practices or personal experiences with structuring Redux applications.
20 Redux interview questions to ask junior developers
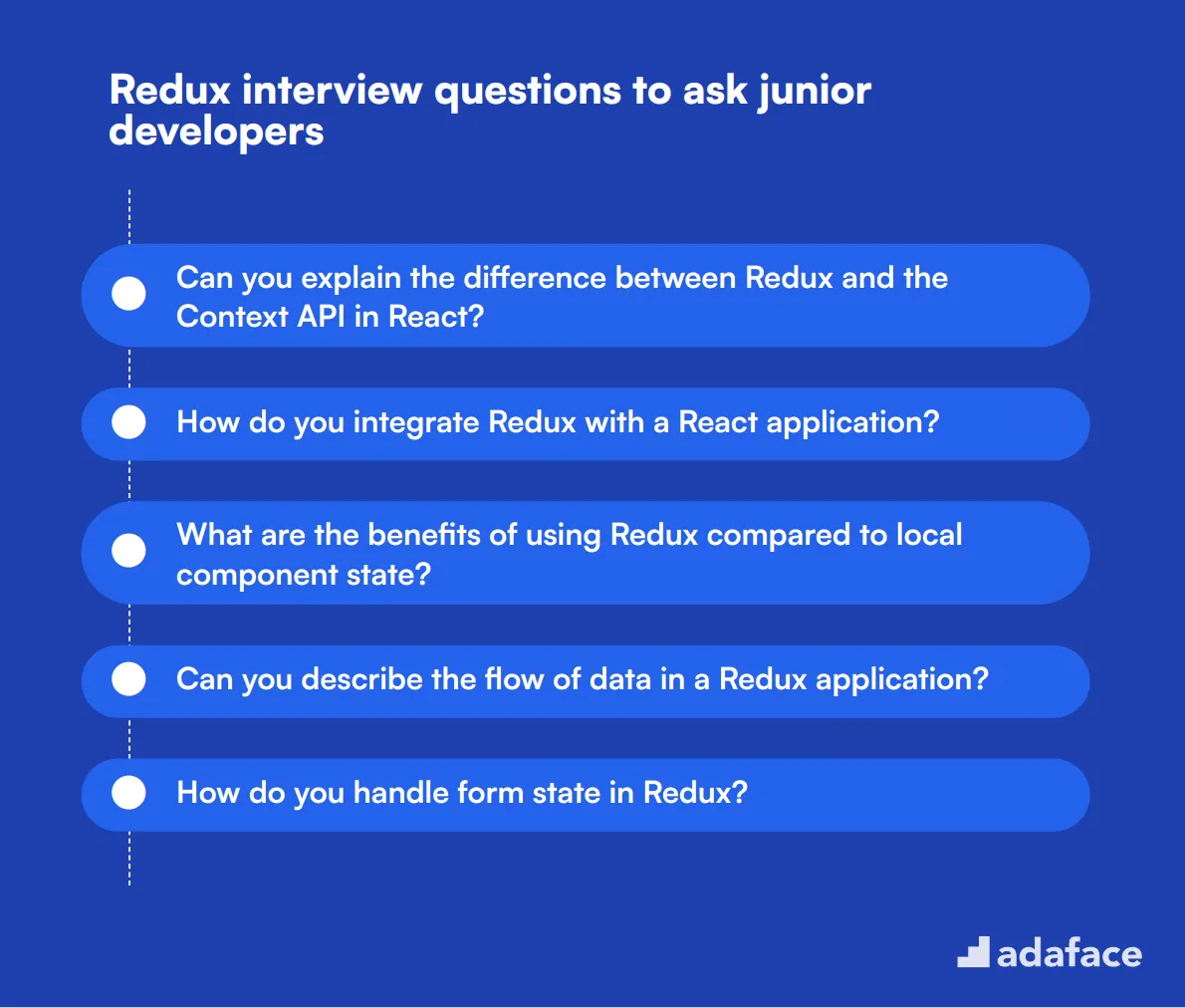
To ensure your junior developers have a solid grasp of Redux, consider using these targeted interview questions. These questions will help you assess their practical knowledge and understanding of key Redux concepts, ensuring they can effectively manage state in your applications. For more information on hiring developers, check out our job descriptions.
- Can you explain the difference between Redux and the Context API in React?
- How do you integrate Redux with a React application?
- What are the benefits of using Redux compared to local component state?
- Can you describe the flow of data in a Redux application?
- How do you handle form state in Redux?
- What is the significance of the initial state in a Redux reducer?
- How would you debug an issue in a Redux application?
- What are the benefits and drawbacks of using Redux Thunk?
- How can you optimize performance in a Redux application?
- Could you explain the concept of immutability and why it is important in Redux?
- What are some common patterns or best practices for writing reducers?
- How do you manage side effects in Redux?
- Can you explain what Redux DevTools are and how to use them?
- What is the purpose of combining reducers in Redux?
- How do you handle nested state in Redux?
- What are some strategies for testing Redux actions and reducers?
- How do you perform state normalization in Redux, and why is it important?
- Can you explain how to handle errors in Redux?
- What are some common pitfalls to avoid when using Redux?
- How do you handle large-scale state management with Redux?
10 intermediate Redux interview questions and answers to ask mid-tier developers.
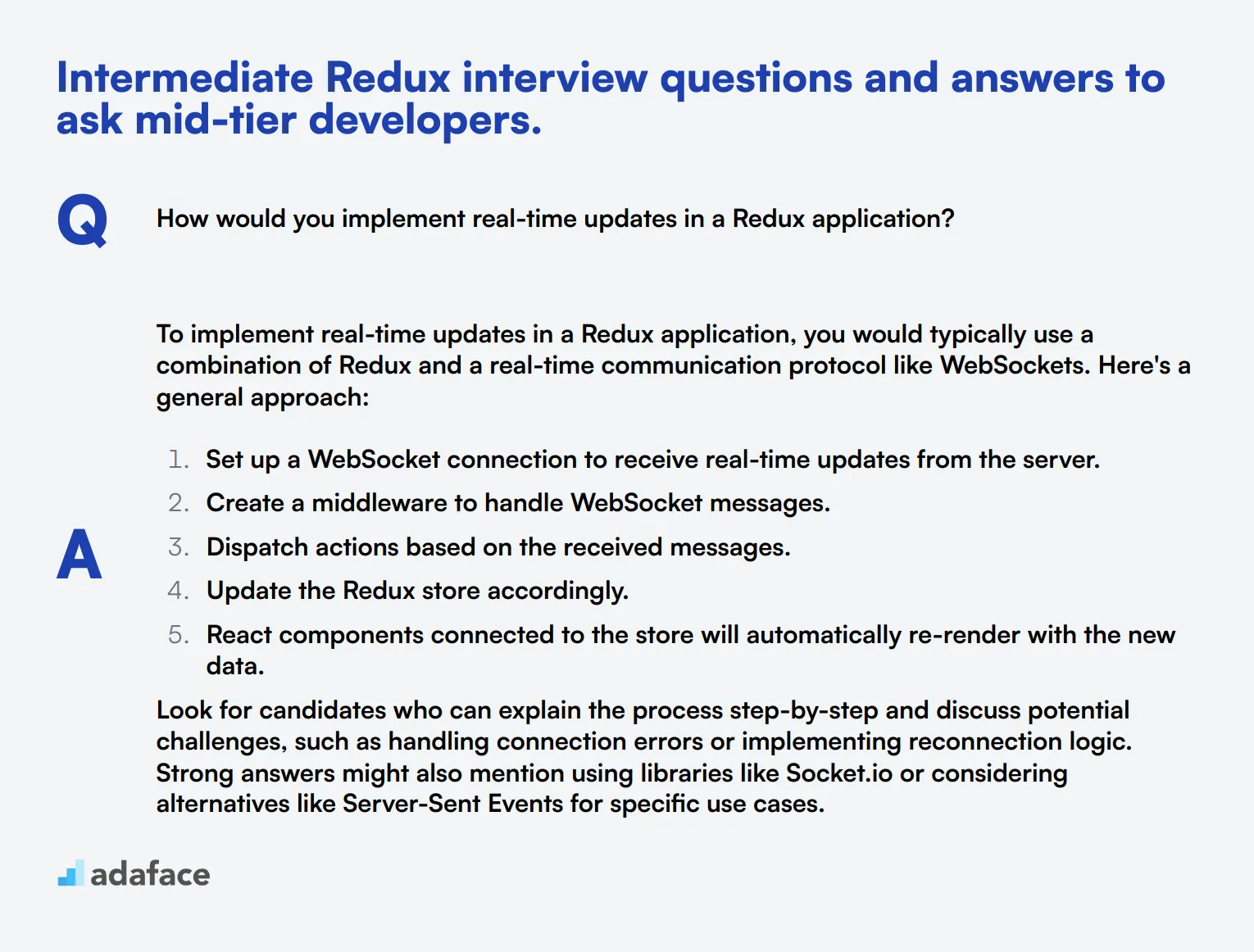
Ready to dive deeper into Redux? These 10 intermediate questions are perfect for assessing mid-tier developers' understanding of Redux concepts and best practices. Use them to gauge candidates' ability to handle complexity and implement Redux effectively in real-world scenarios. Remember, the goal is to spark insightful discussions, not just get textbook answers!
1. How would you implement real-time updates in a Redux application?
To implement real-time updates in a Redux application, you would typically use a combination of Redux and a real-time communication protocol like WebSockets. Here's a general approach:
- Set up a WebSocket connection to receive real-time updates from the server.
- Create a middleware to handle WebSocket messages.
- Dispatch actions based on the received messages.
- Update the Redux store accordingly.
- React components connected to the store will automatically re-render with the new data.
Look for candidates who can explain the process step-by-step and discuss potential challenges, such as handling connection errors or implementing reconnection logic. Strong answers might also mention using libraries like Socket.io or considering alternatives like Server-Sent Events for specific use cases.
2. Can you explain the concept of 'time travel debugging' in Redux and how it's achieved?
Time travel debugging in Redux refers to the ability to move back and forth through the history of state changes in an application. This powerful feature is made possible by Redux's predictable state management and the immutability of its state updates.
It's achieved through the following mechanisms:
- Each action that modifies the state is recorded.
- The state at each point in time is preserved.
- Developer tools (like Redux DevTools) allow you to 'jump' to any previous state.
- The application re-renders based on the selected historical state.
A strong candidate should be able to explain not just the concept, but also its practical benefits, such as easier bug reproduction and understanding of state changes over time. They might also mention potential performance considerations for apps with complex state or frequent updates.
3. How would you optimize performance in a Redux application with a large state tree?
Optimizing performance in a Redux application with a large state tree involves several strategies:
- Use normalization to flatten nested data structures.
- Implement memoized selectors (e.g., with Reselect) to compute derived data.
- Avoid unnecessary re-renders by using
React.memo
orshouldComponentUpdate
. - Use immutable update patterns to enable shallow equality checks.
- Consider using Redux Toolkit for built-in performance optimizations.
- Implement code splitting to reduce initial bundle size.
- Use lazy loading for components and reducers.
Look for candidates who can explain these concepts and provide examples of when they've applied them. Strong answers might also include discussion of profiling tools and techniques to identify performance bottlenecks in Redux applications.
4. Explain the concept of 'middleware' in Redux and give an example of when you might use it.
Middleware in Redux is a way to extend Redux's capabilities by adding custom functionality between dispatching an action and the moment it reaches the reducer. It provides a third-party extension point between dispatching an action and the moment it reaches the reducer.
Common use cases for middleware include:
- Logging actions and state changes
- Crash reporting
- Handling asynchronous actions (e.g., API calls)
- Routing
- Modifying actions or dispatching additional actions
A strong candidate should be able to explain the concept clearly and provide specific examples. They might mention popular middleware like Redux Thunk or Redux Saga for handling asynchronous operations. Look for answers that demonstrate an understanding of how middleware can enhance functionality without cluttering reducer logic.
5. How would you handle complex form state in Redux?
Handling complex form state in Redux requires balancing between local component state and Redux store. Here's a general approach:
- For simple, single-use forms, use local React state.
- For complex forms or when form data is needed across components:
- Store form data in Redux
- Use action creators for form field updates
- Create a dedicated reducer for form state
- Use selectors to access form data in components
- Consider using libraries like Redux Form or Formik for advanced form handling.
- Implement form validation logic, either in the reducer or using middleware.
Look for candidates who can discuss trade-offs between different approaches and explain how they've handled form state in previous projects. Strong answers might include strategies for performance optimization, such as debouncing updates for fields that change frequently.
6. What are some strategies for organizing action creators and reducers in a large Redux application?
Organizing action creators and reducers in a large Redux application is crucial for maintainability. Some effective strategies include:
- Feature-based organization: Group actions, reducers, and selectors by feature or domain.
- Ducks pattern: Combine actions and reducers into a single file for each feature.
- Use Redux Toolkit to simplify boilerplate and enforce best practices.
- Implement action creator factories for common action patterns.
- Use higher-order reducers to add shared functionality across reducers.
- Utilize combineReducers to split the root reducer into smaller, manageable pieces.
- Create a clear naming convention for actions and action creators.
A strong candidate should be able to explain the benefits and potential drawbacks of different organizational strategies. Look for answers that demonstrate experience with large-scale applications and an understanding of how code organization impacts development efficiency and application performance.
7. How would you implement undo/redo functionality in a Redux application?
Implementing undo/redo functionality in a Redux application leverages the predictable state management of Redux. Here's a general approach:
- Maintain a history stack in the Redux store to keep track of past states.
- Create 'UNDO' and 'REDO' actions.
- Implement a higher-order reducer that wraps the main reducer:
- For normal actions, push the current state to the history stack before reducing.
- For UNDO, pop the last state from the history and set it as the current state.
- For REDO, push the current state to history and apply the next state in the redo stack.
- Optionally, limit the history size to prevent memory issues.
Look for candidates who can explain this process clearly and discuss potential challenges, such as handling complex state or optimizing for performance. Strong answers might also mention libraries like Redux Undo or discuss how they've implemented similar functionality in past projects.
8. Explain the concept of 'selector memoization' and why it's important in Redux applications.
Selector memoization is an optimization technique used in Redux applications to improve performance by caching the results of expensive computations. It's typically implemented using libraries like Reselect.
Key points about selector memoization:
- Selectors are functions that compute derived data from the Redux store.
- Memoized selectors only recompute when their inputs change.
- This reduces unnecessary re-renders and improves application performance.
- Particularly useful for complex calculations or when dealing with large datasets.
A strong candidate should be able to explain how memoization works and provide examples of when it's beneficial. Look for answers that demonstrate an understanding of performance optimization in Redux and the ability to identify scenarios where memoization can significantly improve application responsiveness.
9. How would you handle authentication in a Redux application?
Handling authentication in a Redux application typically involves several components working together. Here's a general approach:
- Create actions for login, logout, and checking authentication status.
- Implement a reducer to manage authentication state (e.g., isAuthenticated, user info, token).
- Use middleware to handle authentication-related side effects (e.g., API calls, token storage).
- Create selectors to access authentication state in components.
- Implement protected routes that check authentication status before rendering.
- Store authentication tokens securely (e.g., in HttpOnly cookies or secure local storage).
- Handle token expiration and refresh mechanisms.
Look for candidates who can explain this process and discuss security considerations. Strong answers might include strategies for handling different authentication methods (e.g., JWT, OAuth) or experience with libraries like Redux Auth Wrapper. Pay attention to how they address common challenges like persisting auth state across page reloads.
10. How would you approach testing a Redux application?
Testing a Redux application involves testing various parts of the Redux architecture separately and then integrating them. A comprehensive testing approach might include:
- Unit testing:
- Test individual reducers
- Test action creators
- Test selectors
- Integration testing:
- Test the interaction between actions, reducers, and the store
- Use mock stores to test connected components
- End-to-end testing:
- Test the entire application flow
- Snapshot testing for UI components
- Middleware testing
- Asynchronous action testing (e.g., for API calls)
Look for candidates who can explain different testing strategies and tools (like Jest, Enzyme, or React Testing Library). Strong answers might include discussion of test-driven development practices, strategies for mocking external dependencies, or experience with setting up continuous integration for Redux applications.
12 Redux interview questions about concepts and best practices
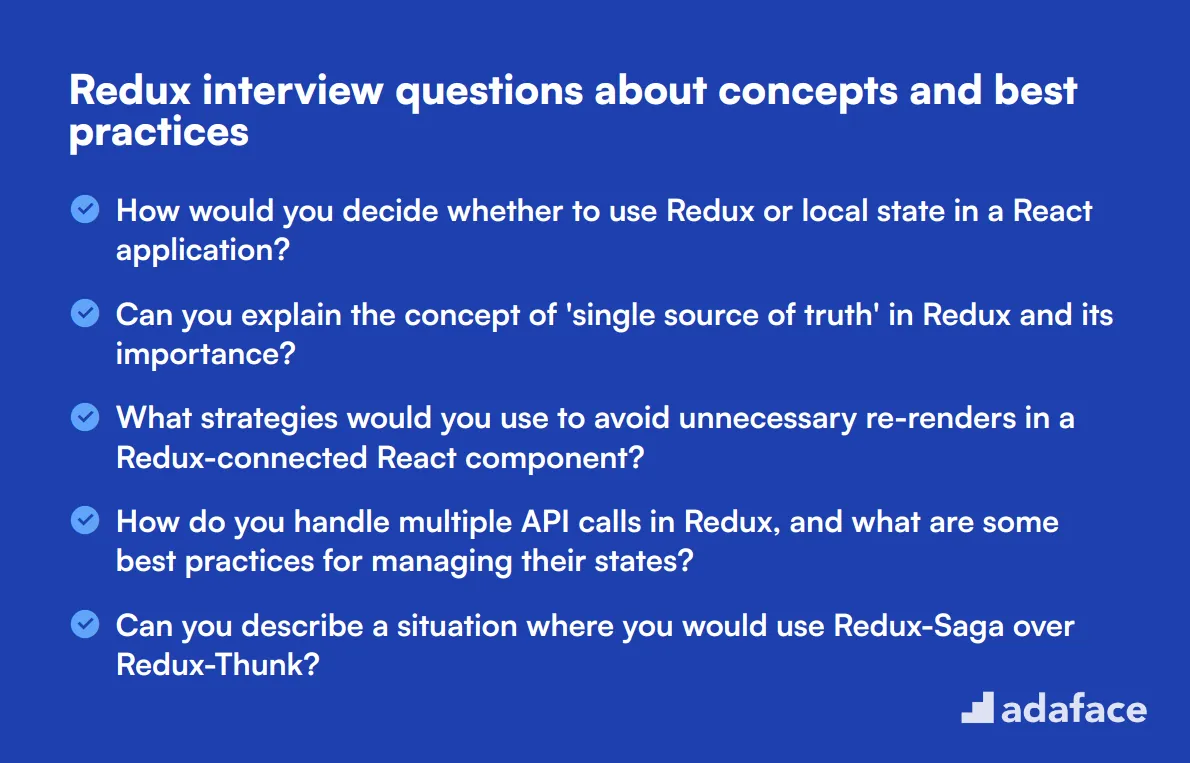
To assess candidates' understanding of Redux concepts and best practices, consider using these 12 interview questions. These questions are designed to probe deeper into a React developer's knowledge of Redux, helping you identify those who truly grasp its intricacies and can apply them effectively in real-world scenarios.
- How would you decide whether to use Redux or local state in a React application?
- Can you explain the concept of 'single source of truth' in Redux and its importance?
- What strategies would you use to avoid unnecessary re-renders in a Redux-connected React component?
- How do you handle multiple API calls in Redux, and what are some best practices for managing their states?
- Can you describe a situation where you would use Redux-Saga over Redux-Thunk?
- How would you implement a feature flag system using Redux?
- What are some strategies for splitting a large Redux store into smaller, more manageable pieces?
- How do you handle optimistic updates in a Redux application?
- Can you explain the concept of 'ducks' pattern in Redux and when you might use it?
- How would you implement a caching mechanism for API responses using Redux?
- What are some best practices for handling deeply nested state updates in Redux?
- How would you approach migrating a large application from Redux to React's Context API, and what factors would you consider?
6 Redux interview questions and answers related to state management
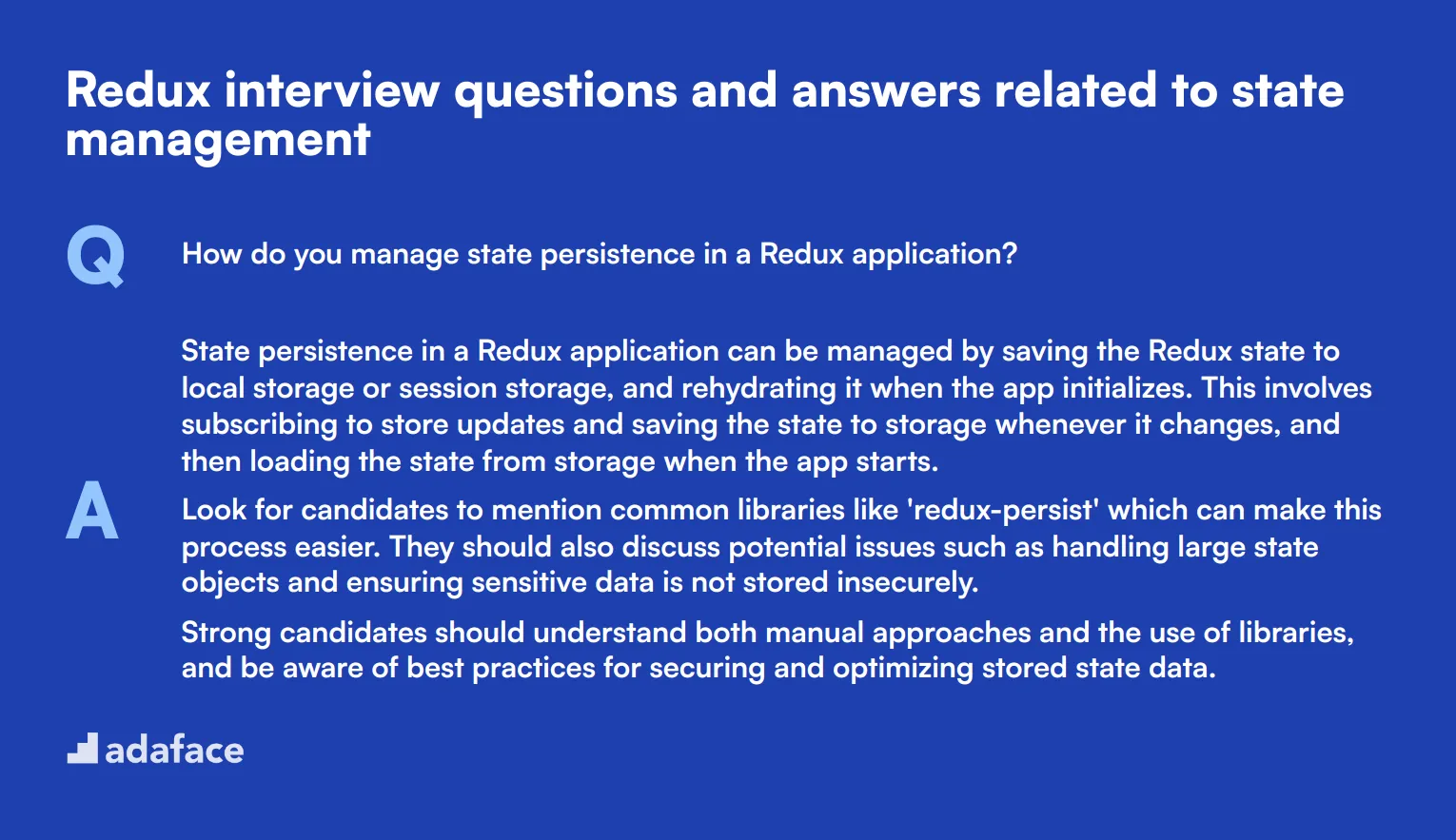
To determine whether candidates have the right grasp of state management in Redux, use these questions. They will help you assess not only their theoretical knowledge but also their practical understanding of managing state in a complex application.
1. How do you manage state persistence in a Redux application?
State persistence in a Redux application can be managed by saving the Redux state to local storage or session storage, and rehydrating it when the app initializes. This involves subscribing to store updates and saving the state to storage whenever it changes, and then loading the state from storage when the app starts.
Look for candidates to mention common libraries like 'redux-persist' which can make this process easier. They should also discuss potential issues such as handling large state objects and ensuring sensitive data is not stored insecurely.
Strong candidates should understand both manual approaches and the use of libraries, and be aware of best practices for securing and optimizing stored state data.
2. How would you handle state updates that need to be synchronized across multiple components?
To synchronize state updates across multiple components, you can use actions and reducers to centralize state changes in Redux. By dispatching actions that represent the state change, all components that are connected to the Redux store will receive the updated state.
Candidates should mention the importance of structuring the state properly to avoid unnecessary re-renders and ensuring that only the components that need the state are connected to the store. Techniques like memoization with selectors can be helpful in optimizing performance.
An ideal response would demonstrate an understanding of both the architectural considerations and performance implications of managing shared state across components.
3. What strategies would you use to avoid common pitfalls in state management with Redux?
To avoid common pitfalls in Redux state management, it's essential to follow best practices such as keeping the state flat and normalized, avoiding mutations by using immutable data structures, and using middleware for handling asynchronous operations.
Candidates should also mention the importance of clear and consistent action types, thorough testing of reducers and actions, and leveraging tools like Redux DevTools for debugging and tracking state changes.
A strong answer will include both the identification of common pitfalls and practical strategies or tools to mitigate them. Look for a balanced approach that combines theoretical knowledge with practical experience.
4. How do you handle form state in a Redux application?
Form state in a Redux application can be managed by creating a dedicated slice of the state for form data and handling form-related actions with specific reducers. Actions for form updates, submission, and validation can be dispatched to update the Redux state accordingly.
Candidates may also mention libraries like 'redux-form' or 'formik' that simplify form state management by providing built-in utilities for handling form events, validation, and submission.
Look for a response that covers both manual management and the use of libraries, and check for an understanding of how to handle validation and error states within the Redux paradigm.
5. What are some strategies for optimizing performance in a Redux application?
Optimizing performance in a Redux application can be achieved by using techniques like memoized selectors with libraries such as 'reselect', avoiding unnecessary re-renders by connecting only the essential components to the Redux store, and splitting the state into smaller, more manageable slices.
Candidates should also mention the importance of using middleware effectively to handle side effects, and leveraging tools like Redux DevTools to identify and address performance bottlenecks.
An ideal candidate response will include both high-level strategies and specific tools or techniques they have used in past projects. Look for practical examples that demonstrate their ability to apply these optimizations effectively.
6. How do you handle error states in a Redux application?
Handling error states in a Redux application involves creating dedicated actions and reducers for managing error state. These actions should be dispatched whenever an error occurs, and the reducers should update the state to reflect the error condition.
Candidates might also mention the importance of displaying error messages to users, logging errors for debugging, and potentially integrating with external error monitoring services.
Look for a comprehensive approach that includes both state management and user experience considerations. Strong candidates should demonstrate an understanding of how to manage and recover from errors gracefully.
8 situational Redux interview questions with answers for hiring top developers
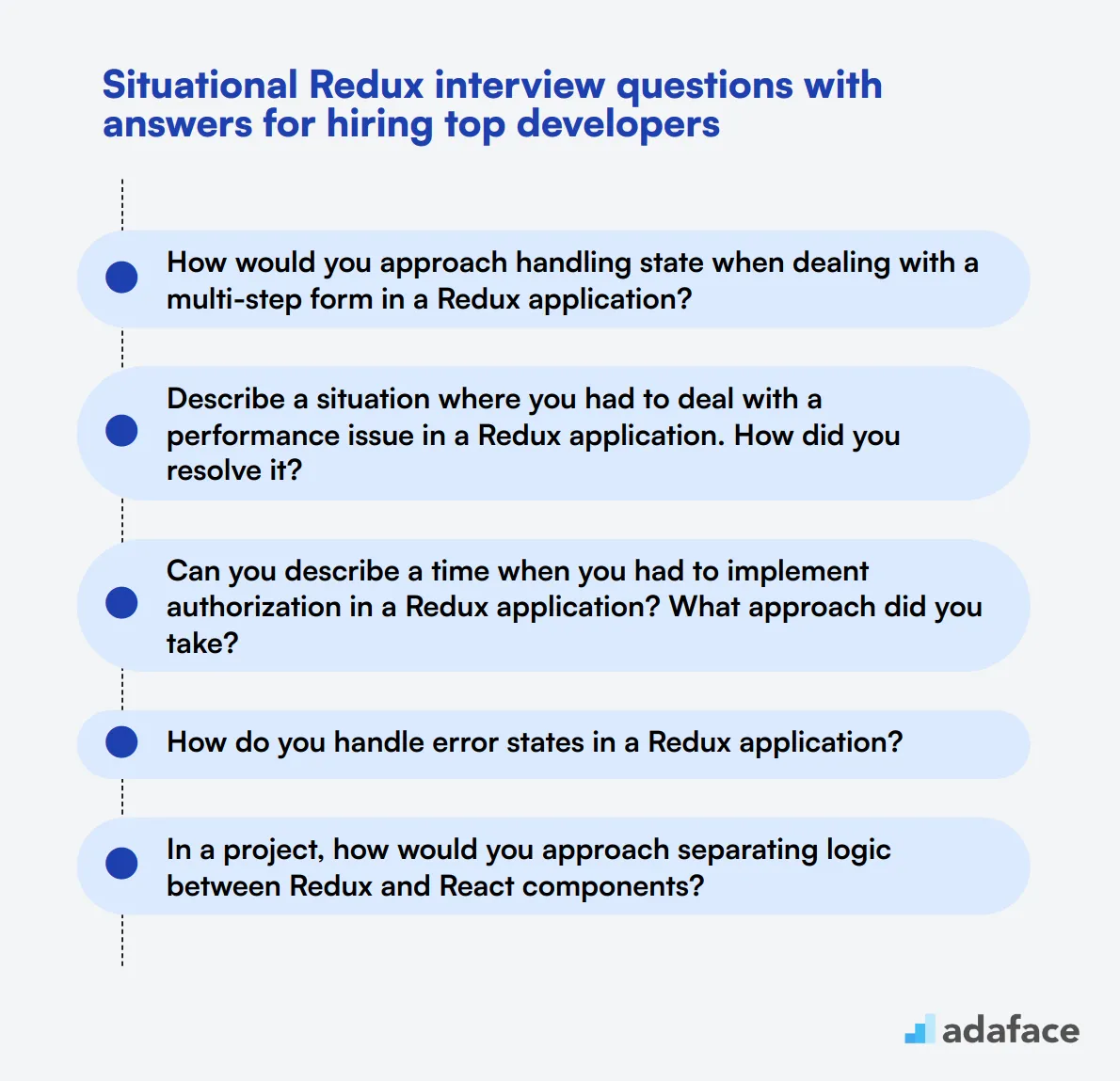
To identify top Redux developers, you need more than just basic questions. These situational Redux interview questions are designed to gauge how candidates think on their feet and solve real-world problems. Use these questions to uncover the depth of their practical knowledge and problem-solving skills.
1. How would you approach handling state when dealing with a multi-step form in a Redux application?
Candidates should explain that handling state in a multi-step form can be managed by creating a slice of state for each step of the form. Each step's state can be merged into a single form state when the user progresses through the steps.
They might suggest using individual reducers for each form step and combining them to a single reducer to manage the global form state. This approach allows for clear separation of concerns and easier debugging.
Look for candidates who emphasize the importance of maintaining immutability and making use of Redux middleware, like Redux Thunk or Redux Saga, for handling any asynchronous operations or side effects.
2. Describe a situation where you had to deal with a performance issue in a Redux application. How did you resolve it?
A strong candidate might describe identifying performance bottlenecks using tools like Redux DevTools and React Profiler. They would analyze the state updates and component re-renders to pinpoint inefficiencies.
They could mention optimizing selectors with memoization to prevent unnecessary re-renders and restructuring the state to make it flatter, reducing the complexity of state updates.
Ideal responses should highlight specific actions taken to improve performance and the measurable outcomes achieved. Look for a clear understanding of both the problem and the solution.
3. Can you describe a time when you had to implement authorization in a Redux application? What approach did you take?
Candidates should describe using a combination of actions, reducers, and middleware to handle authorization. They might mention setting up an auth reducer to manage authentication states like logged in, logged out, or failed login.
They may talk about dispatching actions to check user credentials and storing tokens securely. Additionally, they could mention integrating middleware to intercept actions and check for valid tokens before proceeding.
Look for candidates who demonstrate a thorough understanding of security best practices and can articulate how they ensured the application remained secure while maintaining a smooth user experience.
4. How do you handle error states in a Redux application?
Candidates should explain that error handling can be managed by creating specific actions and reducers for error states. They might describe dispatching an error action when an operation fails and updating the state accordingly.
They could mention showing error messages to the users through the UI and logging errors for further analysis. Additionally, they might talk about using middleware to handle asynchronous errors and ensure they are propagated correctly.
An ideal answer demonstrates a proactive approach to error handling, including user experience considerations and a robust strategy for logging and resolving errors.
5. In a project, how would you approach separating logic between Redux and React components?
Candidates should explain that business logic should be kept within Redux, while UI logic should reside within React components. They might suggest creating action creators and reducers to handle the state and side effects in Redux.
They could mention using React components to dispatch actions and subscribe to state changes, ensuring the components remain presentation-focused and reusable.
Look for candidates who understand the importance of separating concerns and can explain how this approach improves maintainability and scalability of the application.
6. Can you describe a challenging bug you encountered in a Redux application and how you resolved it?
Strong candidates might describe a specific bug, such as a state mutation issue, and the steps they took to diagnose it using tools like Redux DevTools and console logs.
They could explain how they identified and fixed the bug by ensuring immutability, refactoring reducers, or improving action handling. They might also mention writing tests to prevent similar issues in the future.
Look for detailed explanations that show a methodical approach to debugging and a commitment to code quality and testing.
7. How would you handle state persistence in a Redux application?
Candidates should explain that state persistence can be managed by using libraries like Redux Persist. They might describe configuring Redux Persist to save the state to local storage or session storage and rehydrate it on app load.
They could also mention selectively persisting parts of the state to avoid unnecessary storage overhead and ensure sensitive data is not persisted insecurely.
An ideal response shows a clear understanding of the trade-offs involved in state persistence and demonstrates experience with tools and best practices for implementing it securely and efficiently.
8. Describe your approach to testing Redux actions and reducers.
Candidates should explain that testing Redux actions and reducers involves using testing frameworks like Jest or Mocha. They might describe writing unit tests for actions to ensure they create the correct action objects and for reducers to verify they return the expected state.
They could mention using mock stores for integration tests to check how actions and reducers work together. Additionally, they might discuss testing asynchronous actions with tools like Redux Thunk or Redux Saga.
Look for candidates who emphasize the importance of testing and can detail their approach to ensuring reliable and maintainable Redux code.
Which Redux skills should you evaluate during the interview phase?
While a single interview cannot capture the entirety of a candidate's capabilities, evaluating specific skills related to Redux can be instrumental in gauging their proficiency in this state management library. The following skills are essential to focus on during the interview process to ensure candidates possess the necessary expertise to successfully utilize Redux in their projects.
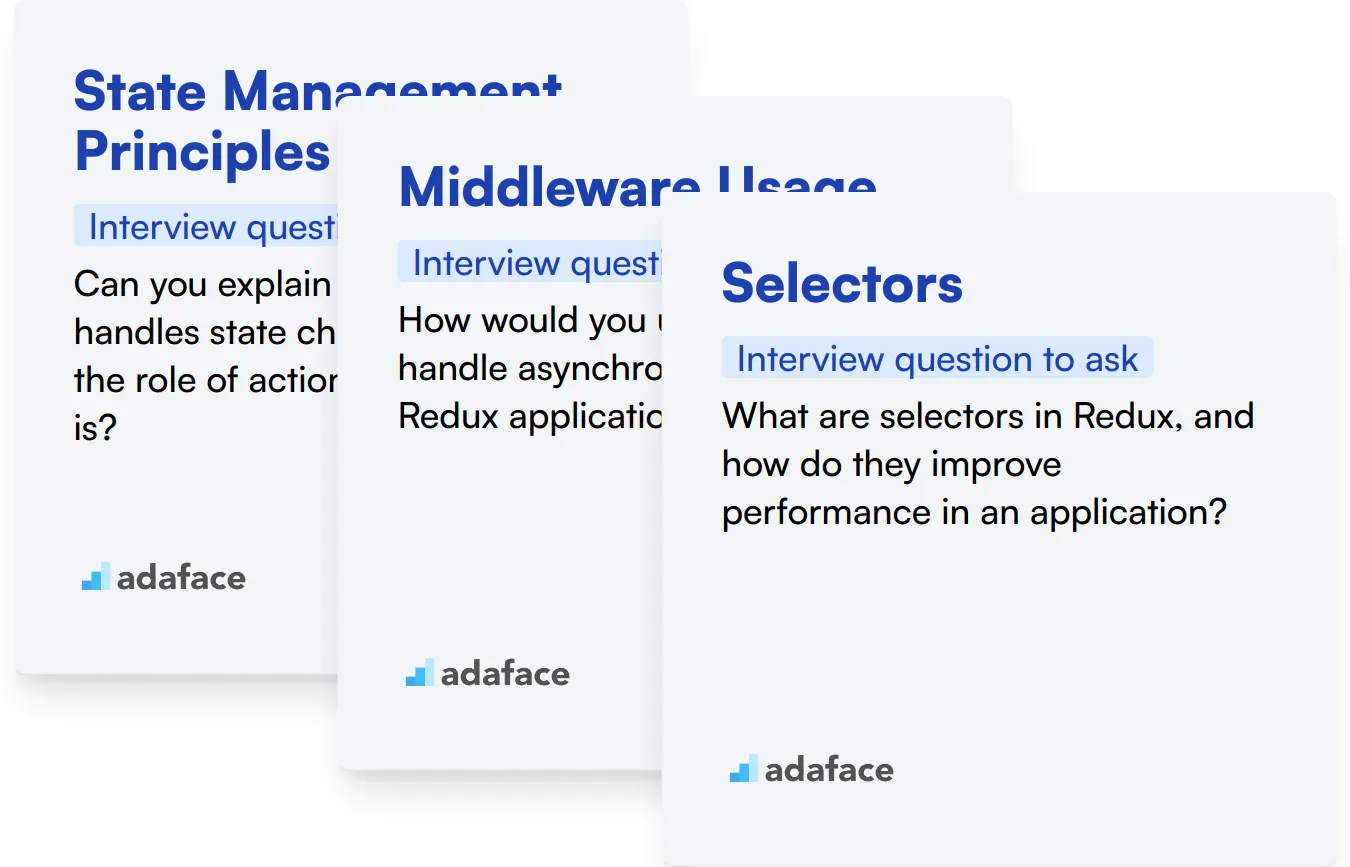
State Management Principles
To assess this skill, consider using an assessment test that includes relevant multiple-choice questions. You can explore our library for a suitable test on Redux to help filter candidates based on their understanding of state management.
To further evaluate this skill during the interview, you might ask targeted questions that require candidates to explain their approach to state management.
Can you explain how Redux handles state changes and what the role of actions and reducers is?
When you ask this question, look for candidates to articulate the flow of data in Redux, detailing how actions are dispatched and how reducers process these actions to update the state. A solid answer will demonstrate their grasp of the core concepts.
Middleware Usage
To test this skill, consider administering a multiple-choice assessment that includes questions on middleware concepts and implementations. For instance, our Redux assessment could help you identify candidates familiar with middleware applications.
Another effective way to evaluate this skill is to ask a question that dives into practical middleware usage within a Redux application.
How would you use middleware to handle asynchronous actions in a Redux application?
When asking this, pay attention to candidates’ ability to explain the use of middleware like Redux Thunk or Redux Saga and how they facilitate asynchronous operations. A strong candidate will provide clear examples of scenarios where middleware is beneficial.
Selectors
Consider utilizing a multiple-choice assessment that tests knowledge of selectors in Redux, as this can help clarify a candidate's ability to optimally retrieve and manipulate state data.
You can also assess their understanding of selectors by posing a specific question about their implementation.
What are selectors in Redux, and how do they improve performance in an application?
Look for candidates to explain that selectors are functions that derive data from the store and can be memoized for performance improvements. Their response should reflect an understanding of how wrapping state access in selectors can minimize unnecessary re-renders.
3 tips for using Redux interview questions
Before you start putting what you've learned into use, here are our top tips to make your Redux interview process more effective.
1. Incorporate skill tests before interviews
Using skill tests before interviews helps assess each candidate's expertise, ensuring only qualified candidates proceed. This saves time and enables you to focus the interview on deeper evaluation.
Consider tests like the React Redux Test to assess Redux skills, and the JavaScript HTML React Test to check foundational knowledge. These tests help filter out unqualified candidates early.
Performing skill tests post-candidate sourcing but pre-interview allows you to zero in on candidates with the right technical skills. This ensures a more productive and focused interview process.
2. Carefully compile your interview questions
It's essential to curate a concise set of interview questions to maximize the evaluation process. Avoid overloading with too many questions and focus on those that gauge important skills and knowledge.
Consider including questions from related areas such as JavaScript, TypeScript, and React Native. These can provide a holistic view of the candidate's capabilities.
Mix in questions related to soft skills like communication and culture fit to ensure the candidate aligns well with your team dynamics.
3. Ask follow-up questions
Using interview questions alone isn't enough; follow-up questions are necessary to gauge the depth of a candidate's knowledge and prevent surface-level answers.
For example, if you ask a candidate about how they handle state management in Redux, follow up with 'Can you walk me through a specific scenario where you optimized state management?' This helps you understand their practical experience and problem-solving abilities.
Leverage Redux Interview Questions and Skills Tests for Effective Hiring
When it comes to hiring developers with Redux expertise, accurately assessing their skills is key. The most straightforward way to do this is by utilizing skills tests. We recommend exploring our React-Redux Test which is specifically designed to evaluate candidates on this framework.
After implementing the skills test, you'll be able to efficiently shortlist the top candidates. To further streamline your hiring process, consider directing your efforts towards setting up interviews. Get started by signing up here or learn more about our online assessment tools on our Online Assessment Platform.
React & Redux Online Test
Download Redux interview questions template in multiple formats
Redux Interview Questions FAQs
The questions cover basic, junior, intermediate, and advanced levels, suitable for assessing candidates across different experience ranges.
Use them to assess technical knowledge, problem-solving skills, and real-world application of Redux concepts during the interview process.
Yes, the list includes situational questions to evaluate how candidates apply Redux knowledge in real-world scenarios.
Absolutely. The advanced and situational questions are designed to challenge and assess experienced Redux developers.
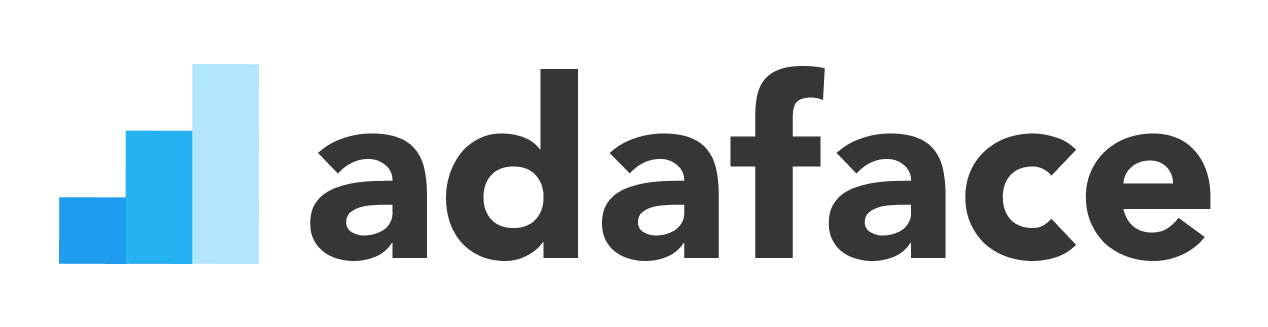
40 min skill tests.
No trick questions.
Accurate shortlisting.
We make it easy for you to find the best candidates in your pipeline with a 40 min skills test.
Try for freeRelated posts
Free resources
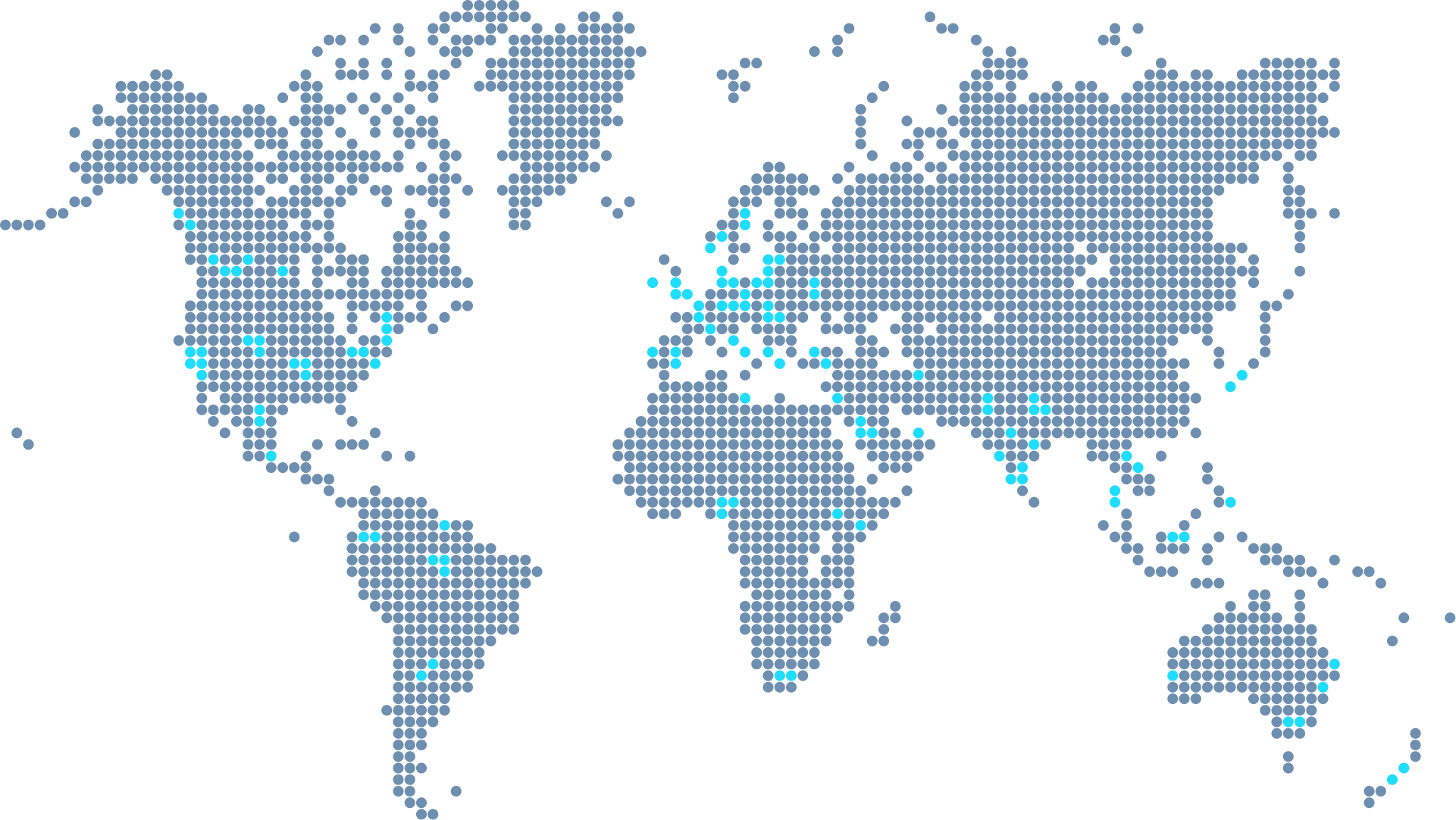
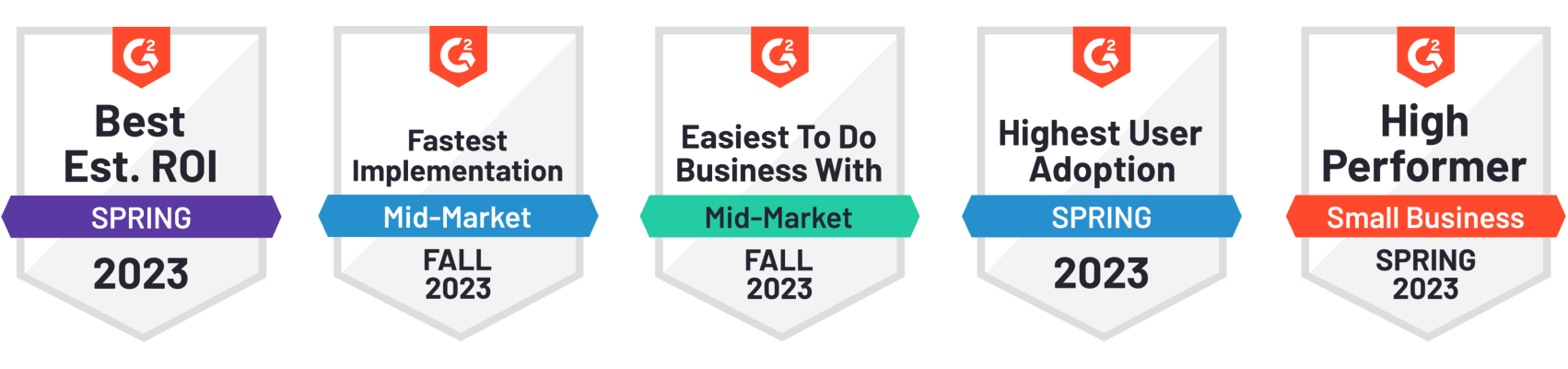