In the rapidly growing field of web development, hiring the right React developer can be challenging. Crafting the perfect set of interview questions to identify top talent is crucial for success.
This blog post provides a comprehensive list of React interview questions tailored for different levels, from juniors to experienced developers. It covers general questions, component-specific queries, and situational questions to help you thoroughly evaluate your candidates.
By leveraging this list, you can improve your hiring process and identify developers who match your needs. Additionally, consider using our ReactJS skills test for an even more thorough assessment before conducting interviews.
Table of contents
7 general React interview questions and answers
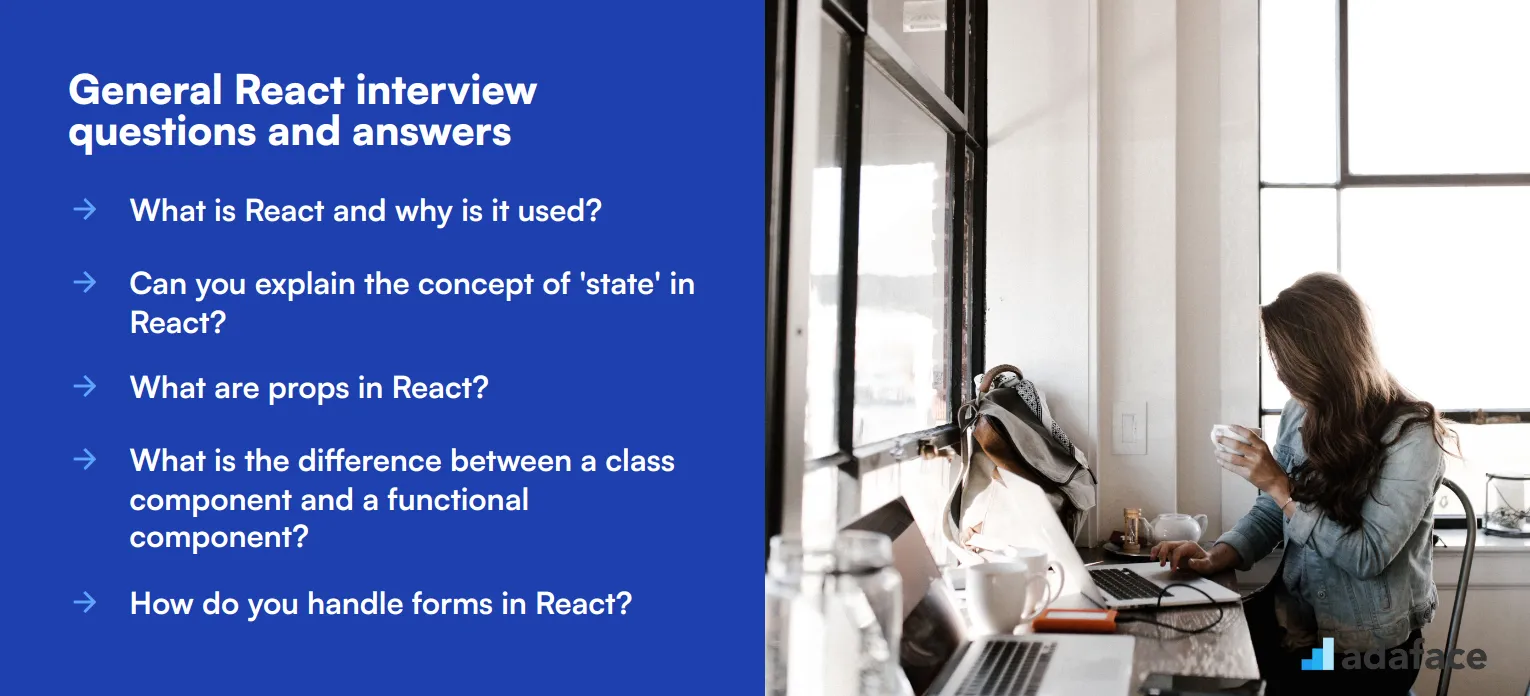
To gauge whether your candidates have a solid understanding of React, utilize these 7 general interview questions and answers. Perfect for identifying both foundational knowledge and practical insights, these questions can help you pinpoint the best fit for your front-end development team.
1. What is React and why is it used?
React is a JavaScript library developed by Facebook for building user interfaces, specifically for single-page applications. It's used for handling the view layer for web and mobile apps and allows developers to create reusable UI components.
When evaluating answers, look for candidates who understand that React's component-based architecture makes it easier to manage and scale large applications. An ideal answer should also touch on React's efficiency in updating and rendering the right components when data changes.
2. Can you explain the concept of 'state' in React?
In React, 'state' refers to an object that determines how a component renders and behaves. It is dynamic, meaning it can change over time, usually in response to user actions or network responses.
A strong candidate will highlight that state is managed within the component and can be passed down to child components as props. This helps in creating interactive and dynamic user interfaces. Look for an understanding of how state management impacts performance and user experience.
3. What are props in React?
Props, short for properties, are used to pass data from one component to another in React. They are read-only, meaning a component receiving props cannot modify them.
Candidates should mention that props help in making components reusable by allowing them to accept dynamic data. Look for an explanation that includes how props can enable communication between parent and child components, enhancing modularity and maintainability.
4. What is the difference between a class component and a functional component?
Class components are ES6 classes that extend from React.Component and have a render method to return JSX. They can hold and manage their own state and lifecycle methods.
Functional components are simpler and are typically written as plain JavaScript functions. Initially, they were stateless, but with the introduction of hooks in React 16.8, they can now manage state and side-effects.
Candidates should demonstrate an understanding of when to use each type of component. An ideal answer will touch on the simplicity and performance benefits of functional components, especially with hooks.
5. How do you handle forms in React?
Handling forms in React involves managing state to track the form's inputs and handling events like onChange and onSubmit to update state and process form data.
Look for candidates who mention controlled components, where form data is handled by the state of the component, ensuring that the input elements' values are derived from the state. This makes form handling predictable and easier to debug.
6. What is JSX and why is it used in React?
JSX stands for JavaScript XML. It is a syntax extension for JavaScript that allows developers to write HTML-like code within JavaScript. JSX makes it easier to create React components and templates.
Strong candidates should explain that JSX provides a more intuitive way to structure component render functions, making code more readable and maintainable. They should also mention that under the hood, JSX is transformed into React.createElement calls.
7. What are React hooks?
React hooks are functions that let you use state and lifecycle features in functional components. Hooks were introduced in React 16.8 to allow functional components to manage state and side-effects, features that were previously only available in class components.
Candidates should be able to name and describe some common hooks like useState, useEffect, and useContext. Look for an explanation that covers how hooks promote cleaner and more concise code by allowing logic to be reused and shared across components.
10 React interview questions to ask junior developers
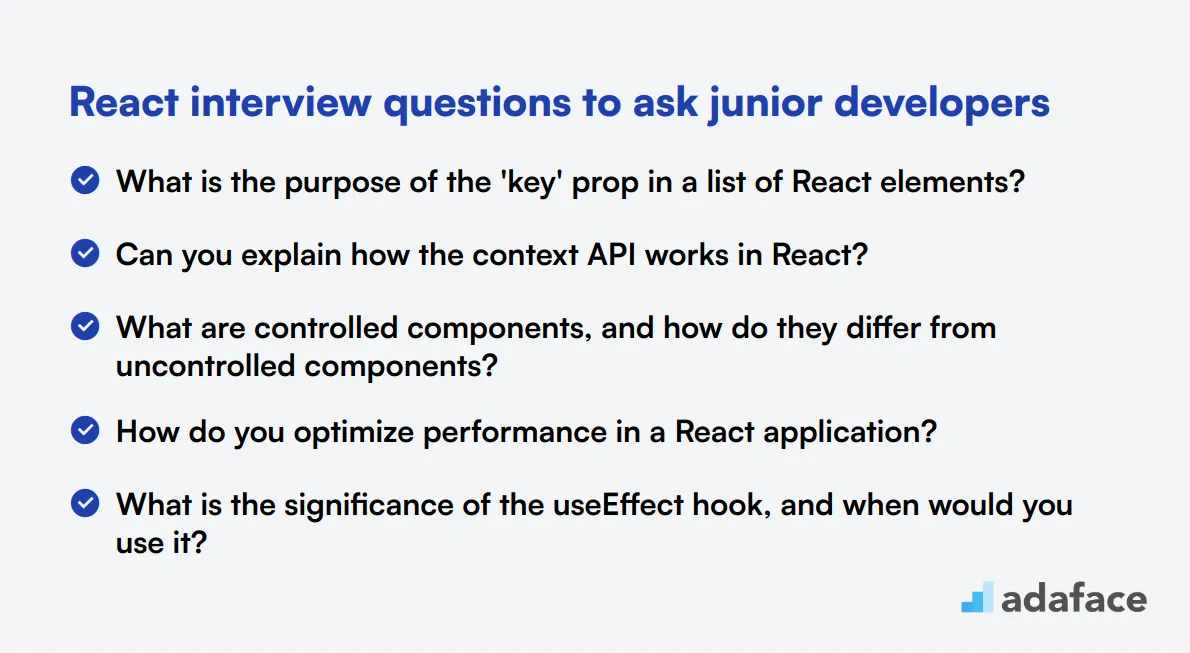
To effectively gauge a junior developer's understanding of React, consider using this curated list of questions. These inquiries will help you assess their familiarity with essential React concepts and their ability to apply them in real-world scenarios. For more insights on hiring, check out our React JS Developer Job Description.
- What is the purpose of the 'key' prop in a list of React elements?
- Can you explain how the context API works in React?
- What are controlled components, and how do they differ from uncontrolled components?
- How do you optimize performance in a React application?
- What is the significance of the useEffect hook, and when would you use it?
- Can you explain what a higher-order component is?
- What is React Router, and how does it help in building single-page applications?
- How do you manage global state in a React application?
- What are the benefits of using TypeScript with React?
- How would you handle error boundaries in a React application?
9 React interview questions and answers related to components and lifecycle methods
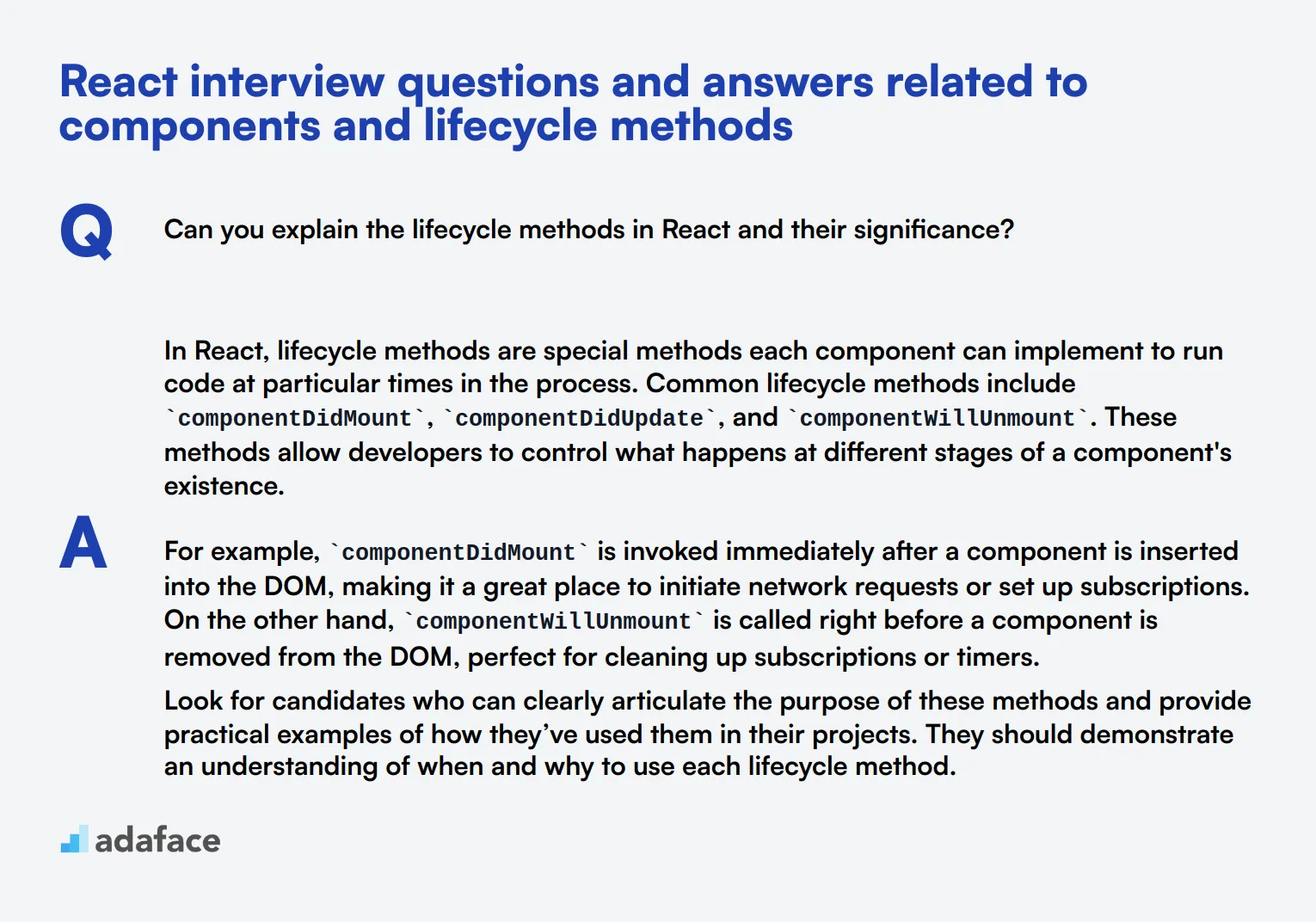
To determine whether your candidates have a strong grasp of React components and lifecycle methods, ask them these targeted interview questions. These questions will help uncover their understanding of React's core concepts without diving too deep into technical jargon.
1. Can you explain the lifecycle methods in React and their significance?
In React, lifecycle methods are special methods each component can implement to run code at particular times in the process. Common lifecycle methods include componentDidMount
, componentDidUpdate
, and componentWillUnmount
. These methods allow developers to control what happens at different stages of a component's existence.
For example, componentDidMount
is invoked immediately after a component is inserted into the DOM, making it a great place to initiate network requests or set up subscriptions. On the other hand, componentWillUnmount
is called right before a component is removed from the DOM, perfect for cleaning up subscriptions or timers.
Look for candidates who can clearly articulate the purpose of these methods and provide practical examples of how they’ve used them in their projects. They should demonstrate an understanding of when and why to use each lifecycle method.
2. Describe the difference between mounting and updating in React.
Mounting and updating are two phases in the React component lifecycle. Mounting refers to the phase when a component is being created and inserted into the DOM for the first time. Key lifecycle methods during mounting include constructor
, componentDidMount
, and render
.
Updating, on the other hand, occurs when a component is being re-rendered due to changes in props or state. During this phase, lifecycle methods like shouldComponentUpdate
, componentDidUpdate
, and render
come into play.
An ideal candidate should understand these phases and be able to explain the importance of these lifecycle events in maintaining and optimizing component performance.
3. What is the purpose of the `render` method in React components?
The render
method is a crucial part of React components, responsible for returning the JSX that makes up the component's structure. It is called during the mounting and updating phases of a component's lifecycle.
This method ensures that the component's appearance matches its current state and props. It is a pure function, meaning it should only depend on the component's props and state and should not cause side effects.
When evaluating responses, look for candidates who emphasize the importance of keeping the render
method pure and efficient, as well as those who can discuss how it fits into the broader lifecycle of a React component.
4. How would you explain the concept of 'lifting state up' in React?
Lifting state up is a technique used in React to manage shared state between multiple components. When two or more components need to share data, the state is moved to the closest common ancestor component. This ancestor component then passes the state down as props to its children.
For example, if two child components need to interact with the same piece of state, that state should be managed by their parent component. The parent controls the state and passes it down to the children via props, ensuring consistency.
Candidates should demonstrate an understanding of why lifting state up is essential for avoiding duplicate state and ensuring a single source of truth. They should also discuss scenarios where this technique is beneficial.
5. What is the purpose of default props in React?
Default props in React allow you to set default values for a component's props. This is useful when you want to ensure that your component has all the necessary data it needs to render properly, even if some props are not provided.
You can define default props by adding a defaultProps
property to your component. This ensures that any missing props are assigned default values, preventing potential errors and providing a fallback.
Look for candidates who understand the practical applications of default props, such as providing default values for optional props, and who can explain how default props contribute to more robust and error-resistant components.
6. Can you explain the difference between a controlled and uncontrolled component in React?
Controlled components are components where React controls the form data. In these components, the form data is handled by the component's state, and the state is updated via setState
on every change. This ensures that the form data is always in sync with the component's state.
Uncontrolled components, on the other hand, allow the DOM to handle the form data. The input elements maintain their own state, and you use refs to access the current values when necessary. This approach can be simpler but offers less control over the form data.
Candidates should be able to discuss scenarios where one approach might be preferred over the other and demonstrate a clear understanding of the trade-offs involved in using controlled versus uncontrolled components.
7. How would you handle component cleanup in React?
Component cleanup in React is typically handled within the componentWillUnmount
lifecycle method or the cleanup function returned by the useEffect
hook. This is where you can perform any necessary cleanup tasks, such as cancelling network requests, removing event listeners, or clearing timers.
For example, if a component sets up an interval timer in componentDidMount
, the corresponding componentWillUnmount
method should clear that interval. Similarly, if using hooks, the useEffect
hook can return a cleanup function that performs these tasks when the component unmounts.
An ideal candidate should highlight the importance of proper cleanup to prevent memory leaks and ensure that resources are properly released. They should also provide examples of how they’ve implemented cleanup in their own projects.
8. What is the significance of keys in React lists?
Keys in React lists are essential for helping React identify which items have changed, been added, or removed. They provide a stable identity for each element, allowing React to optimize updates and avoid unnecessary re-renders.
A key should be unique among siblings and consistent across renders. Using keys ensures that React can efficiently update only the elements that have changed, improving performance and providing a smoother user experience.
When evaluating answers, look for candidates who understand the importance of using stable and unique keys, such as unique IDs, and who can explain how improper key usage can lead to performance issues and bugs.
9. Why is it important to use `setState` instead of modifying the state directly in React?
Using setState
is crucial in React because it schedules an update to a component's state and tells React to re-render the component with the updated state. Directly modifying the state does not trigger a re-render, leading to inconsistent UI and unexpected behavior.
When you use setState
, React can batch multiple state updates for performance optimization and ensure that the component re-renders with the most recent state. This method also maintains the integrity of state updates, as React can manage and reconcile changes efficiently.
Candidates should demonstrate an understanding of the React state management philosophy and explain the risks and pitfalls of direct state manipulation. They should also provide examples of issues they have encountered when not using setState
properly.
13 React questions related to state and props
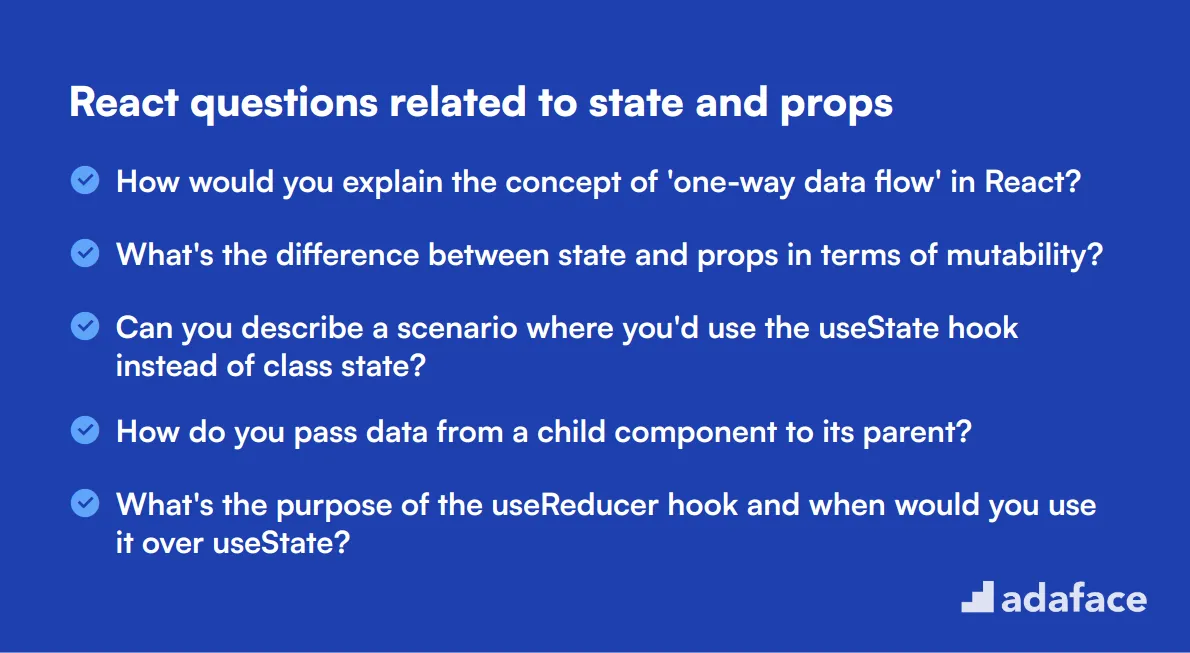
To assess a candidate's understanding of React's core concepts, use these questions about state and props. These inquiries will help you evaluate the React skills of potential hires, focusing on their grasp of data management within components.
- How would you explain the concept of 'one-way data flow' in React?
- What's the difference between state and props in terms of mutability?
- Can you describe a scenario where you'd use the useState hook instead of class state?
- How do you pass data from a child component to its parent?
- What's the purpose of the useReducer hook and when would you use it over useState?
- How does prop drilling occur and what are some ways to avoid it?
- Can you explain the concept of derived state in React?
- What are the potential pitfalls of using the setState method in class components?
- How do you handle asynchronous state updates in React?
- What's the difference between state colocation and lifting state up?
- How do you decide whether a piece of data should be state or props?
- Can you explain how to use the useCallback hook to optimize prop passing?
- What are the benefits and drawbacks of using global state management libraries like Redux?
14 situational React interview questions for hiring top developers
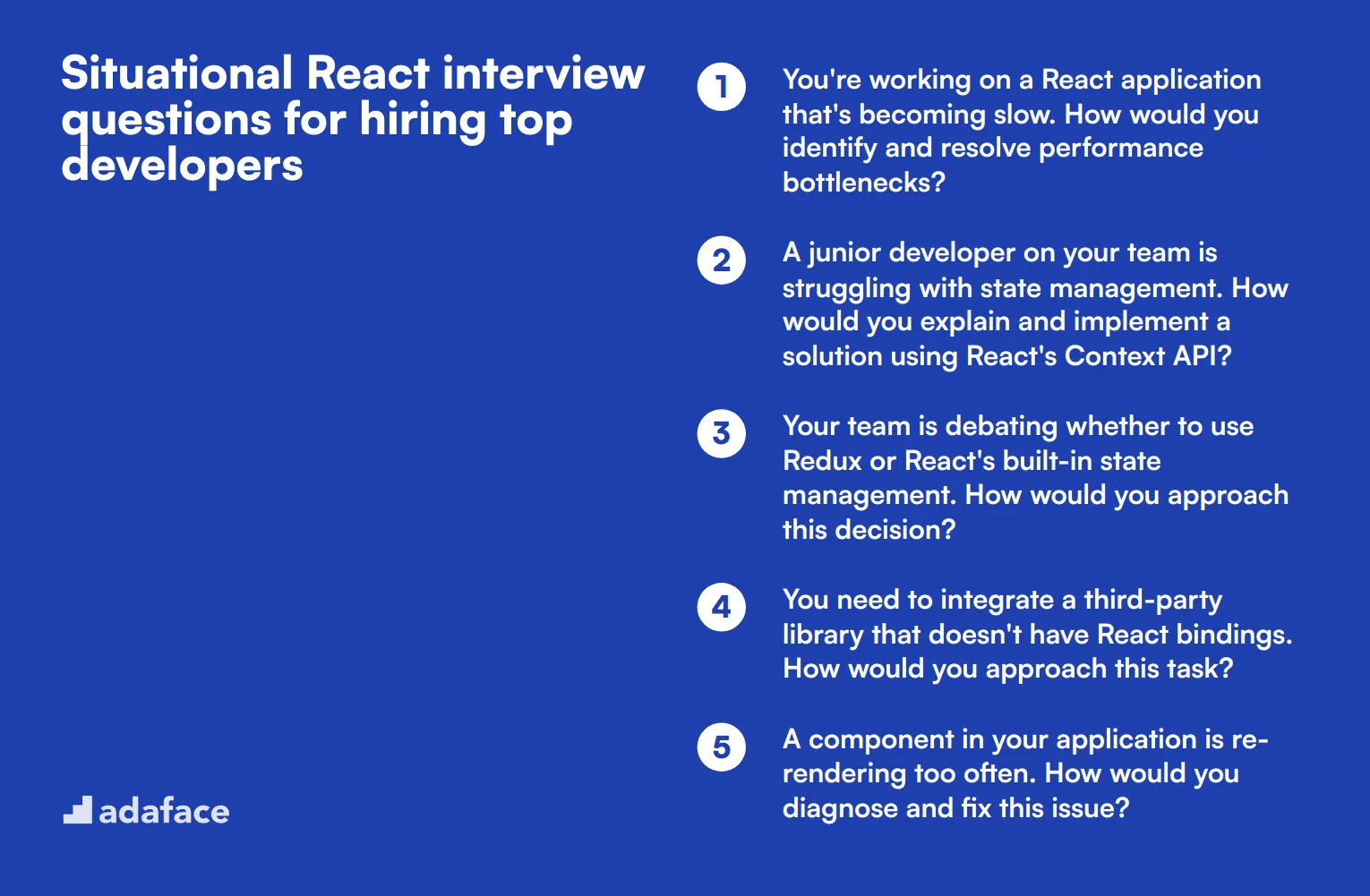
To assess a candidate's practical React skills and problem-solving abilities, use these situational interview questions. They help evaluate how applicants handle real-world scenarios and challenges typically faced by React developers.
- You're working on a React application that's becoming slow. How would you identify and resolve performance bottlenecks?
- A junior developer on your team is struggling with state management. How would you explain and implement a solution using React's Context API?
- Your team is debating whether to use Redux or React's built-in state management. How would you approach this decision?
- You need to integrate a third-party library that doesn't have React bindings. How would you approach this task?
- A component in your application is re-rendering too often. How would you diagnose and fix this issue?
- Your team is transitioning from class components to functional components with hooks. How would you plan and execute this refactoring?
- You're tasked with improving the accessibility of a React application. What steps would you take?
- How would you implement a custom hook for handling form validation in a React application?
- You're working on a large-scale React application. How would you structure your components and organize your code?
- A new team member is unfamiliar with React's synthetic events. How would you explain them and their importance?
- You need to implement a feature that requires sharing state between unrelated components. How would you approach this?
- How would you handle authentication in a React application, considering both the UI and API interactions?
- You're tasked with optimizing the bundle size of a React application. What strategies would you employ?
- How would you implement a search functionality with autocomplete in a React application?
Which React skills should you evaluate during the interview phase?
While it's impossible to assess every aspect of a candidate's React proficiency in a single interview, focusing on core skills can provide valuable insights. The following are key React skills that interviewers should evaluate to gauge a candidate's expertise effectively.
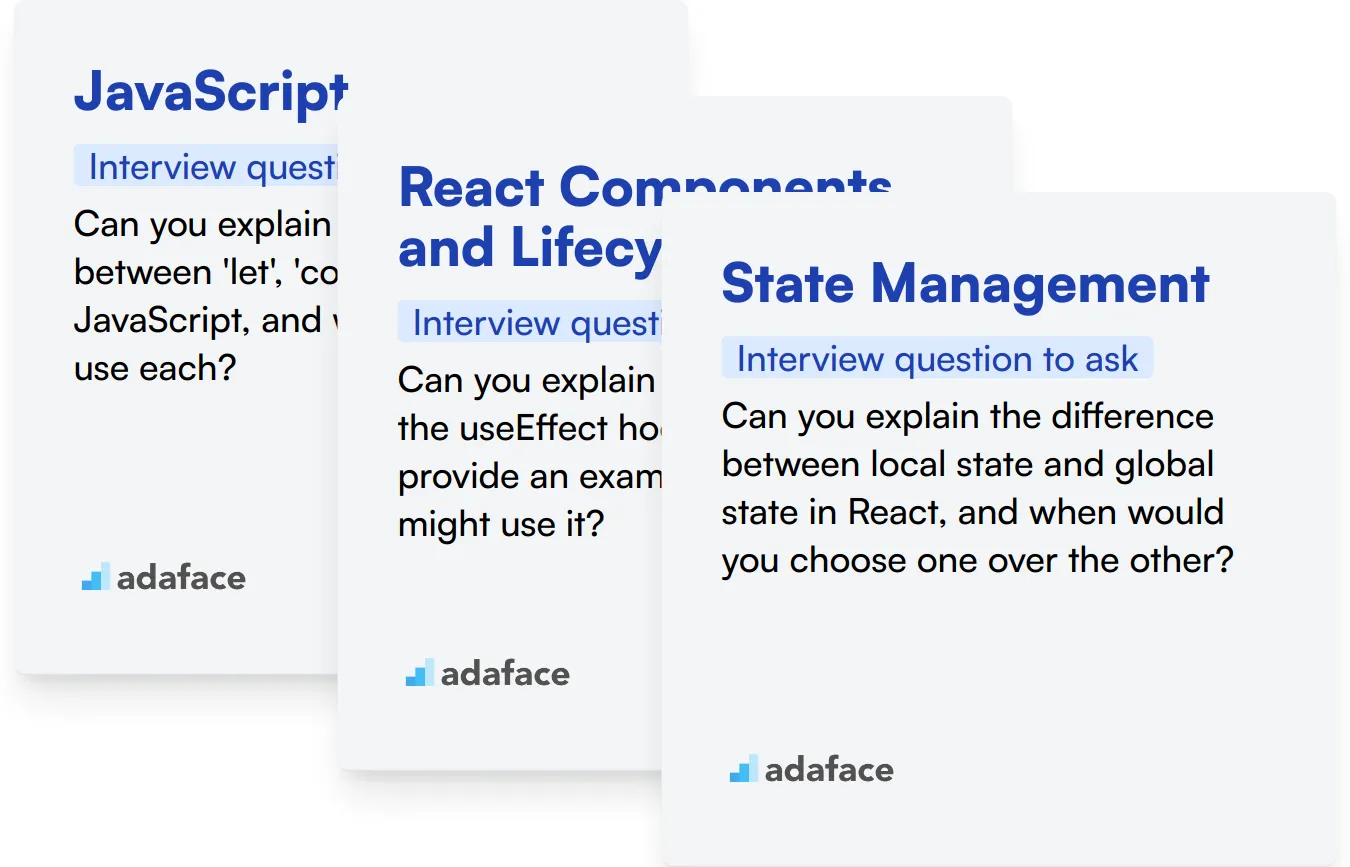
JavaScript
A strong foundation in JavaScript is essential for React development. React heavily relies on JavaScript, and proficiency in this language enables developers to write efficient and maintainable code.
To assess JavaScript skills, consider using an assessment test with relevant multiple-choice questions. This can help filter candidates based on their JavaScript knowledge.
During the interview, ask targeted questions to evaluate the candidate's JavaScript proficiency. Here's an example:
Can you explain the difference between 'let', 'const', and 'var' in JavaScript, and when would you use each?
Look for answers that demonstrate understanding of block scope, function scope, and the immutability of 'const'. A good response should include examples of appropriate use cases for each keyword.
React Components and Lifecycle
Understanding React components and their lifecycle is crucial for building efficient React applications. This knowledge allows developers to create reusable UI elements and manage component behavior effectively.
To evaluate this skill, consider using a React assessment test that includes questions about components and lifecycle methods.
During the interview, ask a question to assess the candidate's understanding of React components and lifecycle:
Can you explain the purpose of the useEffect hook in React and provide an example of when you might use it?
Look for answers that demonstrate understanding of side effects in functional components, dependency arrays, and cleanup functions. A good response should include a practical example of useEffect implementation.
State Management
Effective state management is key to building scalable React applications. Candidates should be familiar with React's built-in state management as well as popular state management libraries.
Consider using an assessment test that includes questions about React state management and popular libraries like Redux.
To evaluate this skill during the interview, ask a question like:
Can you explain the difference between local state and global state in React, and when would you choose one over the other?
Look for answers that demonstrate understanding of component-level state versus application-wide state. A good response should include examples of scenarios where each type of state management is appropriate.
Hire top talent with React skills tests and the right interview questions
When looking to hire someone with React skills, it's important to ensure they possess the necessary expertise. This accuracy can significantly impact the effectiveness of your team in developing high-quality applications.
The most effective way to verify a candidate's skills is through dedicated skill tests. Consider using our React.js test to assess their knowledge and capabilities.
After administering the test, you'll be able to shortlist the best applicants based on their performance. This will streamline your interview process and help you focus on candidates who truly meet your requirements.
To get started, sign up on our online assessment platform and explore our library of tests. This will provide you with the tools needed to make informed hiring decisions.
ReactJS Online Test
Download React interview questions template in multiple formats
React Interview Questions FAQs
Ask a mix of general, junior-level, component-related, state and props, and situational questions to assess a candidate's React knowledge and problem-solving skills.
The number depends on the interview length, but aim for a diverse selection from our list of 63 questions to cover various React concepts and practical scenarios.
Yes, tailor your questions based on the candidate's experience level. Our list includes specific questions for junior developers and more advanced topics for senior roles.
Use situational questions and ask candidates to explain their approach to real-world React scenarios to evaluate their problem-solving abilities and hands-on experience.
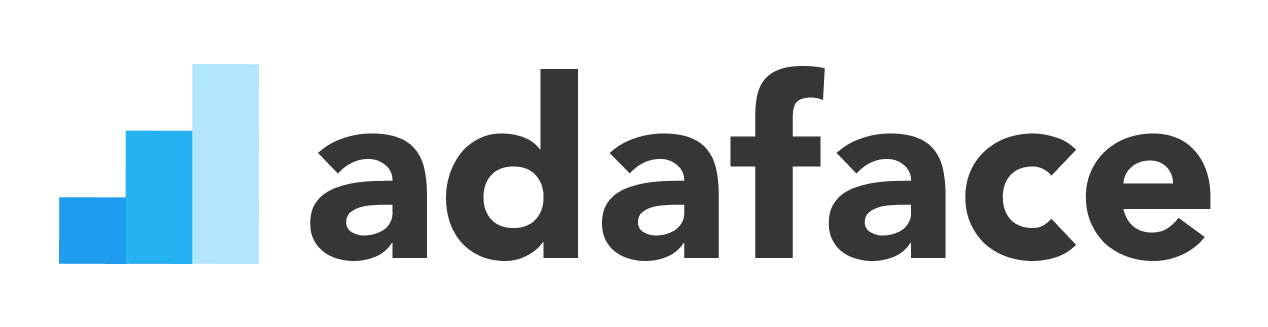
40 min skill tests.
No trick questions.
Accurate shortlisting.
We make it easy for you to find the best candidates in your pipeline with a 40 min skills test.
Try for freeRelated posts
Free resources
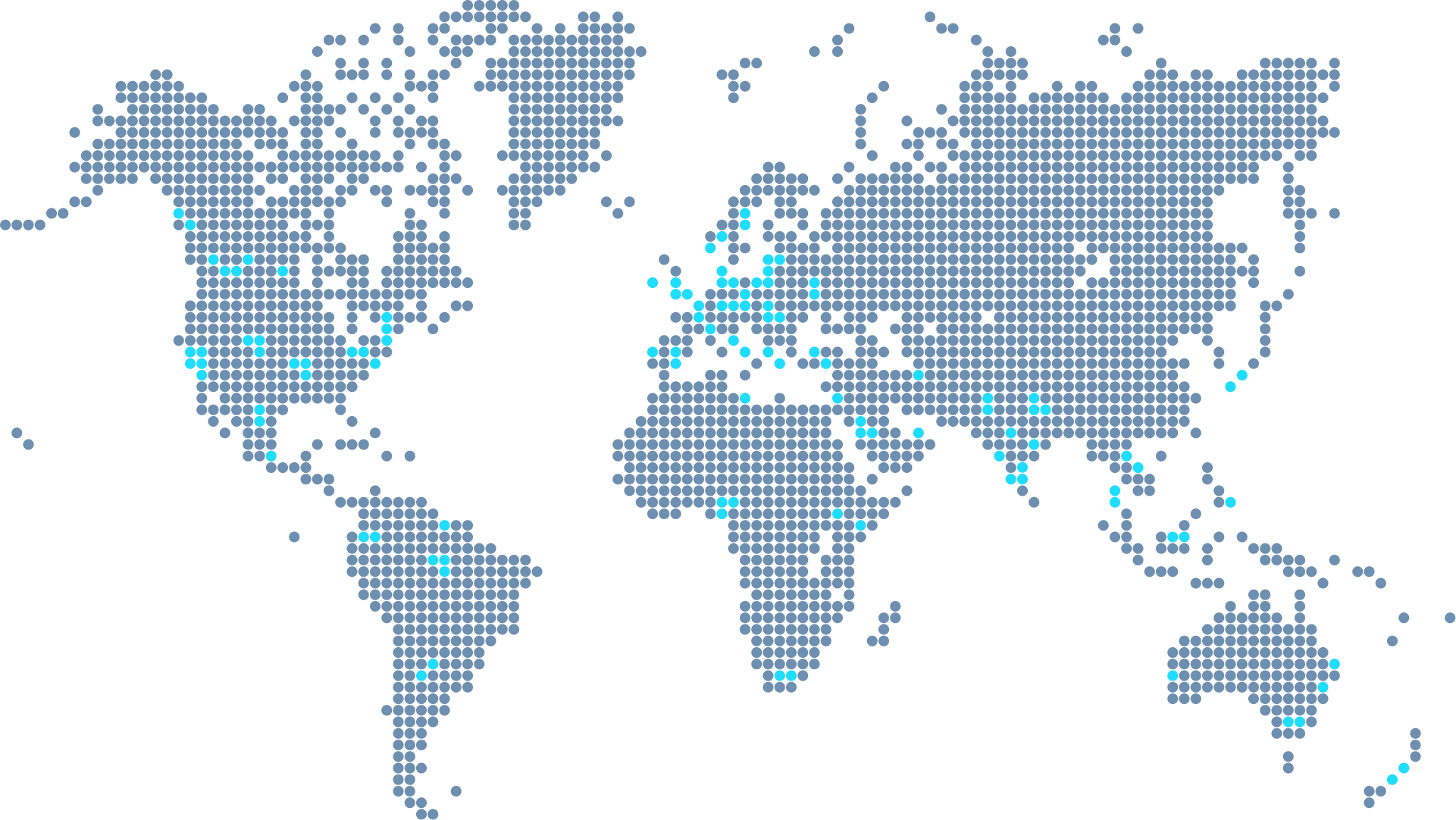
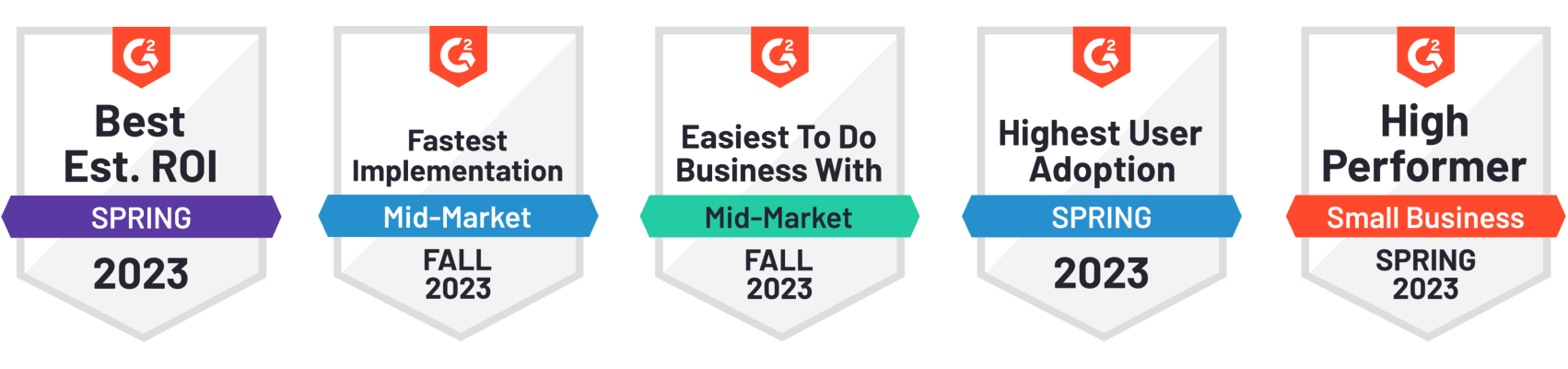