Recruiting the right Python developers requires not only finding candidates with technical expertise but also ensuring they have a strong grasp of Object-Oriented Programming (OOP) concepts. Understanding these concepts is crucial for developers to write clean, maintainable, and efficient Python code.
This blog post offers a comprehensive collection of Python OOPs interview questions tailored for various developer levels, from basic to intermediate, as well as design patterns and situational questions. Each section is designed to help you assess your candidate's understanding and application of OOP principles in Python effectively.
By using these questions, you can identify top Python OOP talent suited for your project requirements. Additionally, consider leveraging our Python online test to pre-screen candidates before diving into technical interviews.
Table of contents
15 basic Python OOPs interview questions and answers to assess candidates
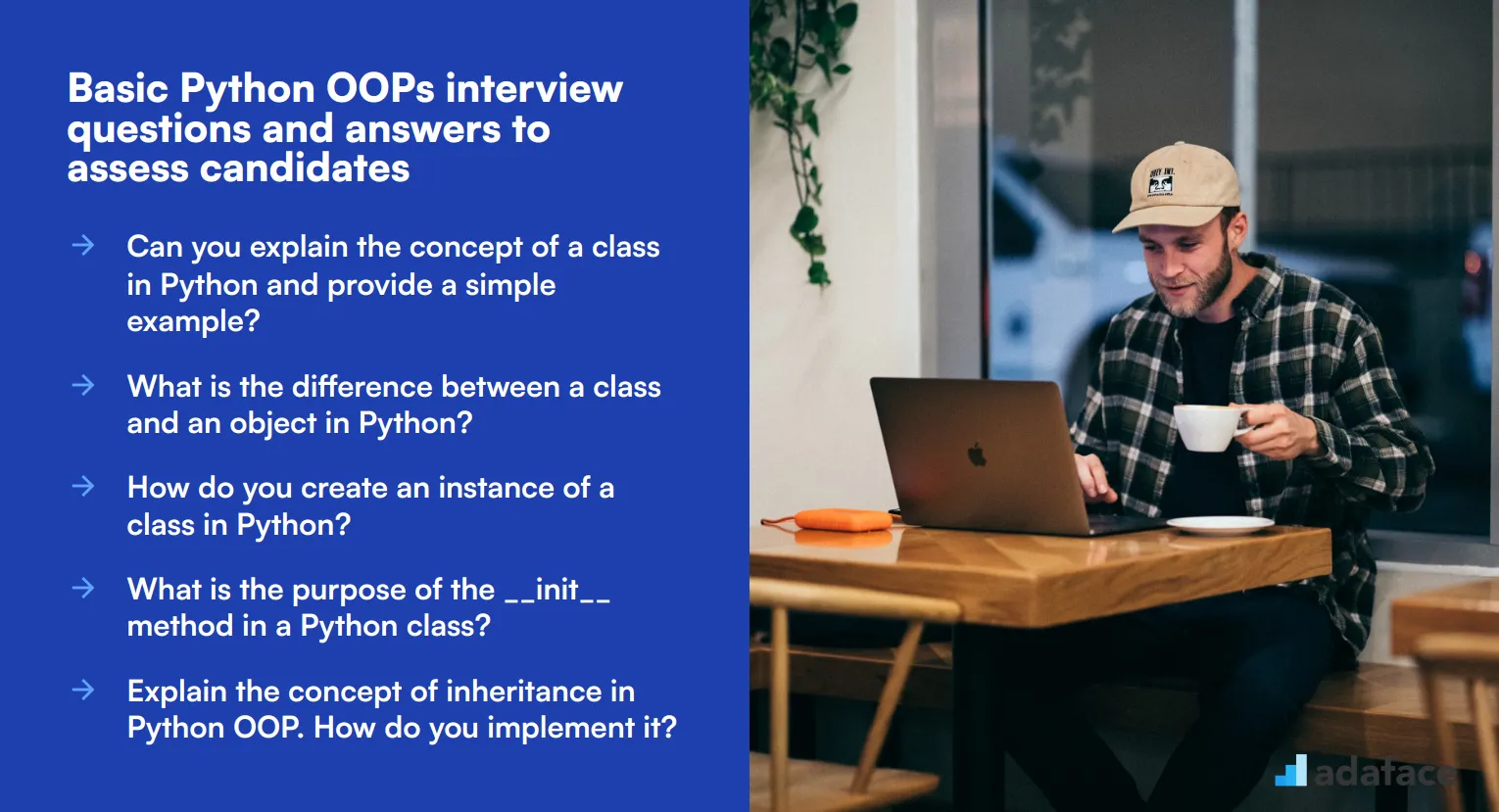
To assess candidates' understanding of Python's object-oriented programming concepts, use these 15 basic OOP interview questions. These questions will help you evaluate a Python developer's grasp of fundamental OOP principles and their ability to apply them in real-world scenarios.
- Can you explain the concept of a class in Python and provide a simple example?
- What is the difference between a class and an object in Python?
- How do you create an instance of a class in Python?
- What is the purpose of the __init__ method in a Python class?
- Explain the concept of inheritance in Python OOP. How do you implement it?
- What is method overriding in Python? Provide an example.
- How does Python support multiple inheritance?
- What are class variables and instance variables in Python?
- Explain the use of the 'self' keyword in Python classes.
- What is encapsulation in Python? How do you implement it?
- How do you define and use a static method in Python?
- What is polymorphism in Python? Can you provide a simple example?
- Explain the difference between public, protected, and private attributes in Python.
- What are Python decorators and how are they used in OOP?
- How do you implement abstraction in Python using abstract base classes?
8 Python OOPs interview questions and answers to evaluate junior developers
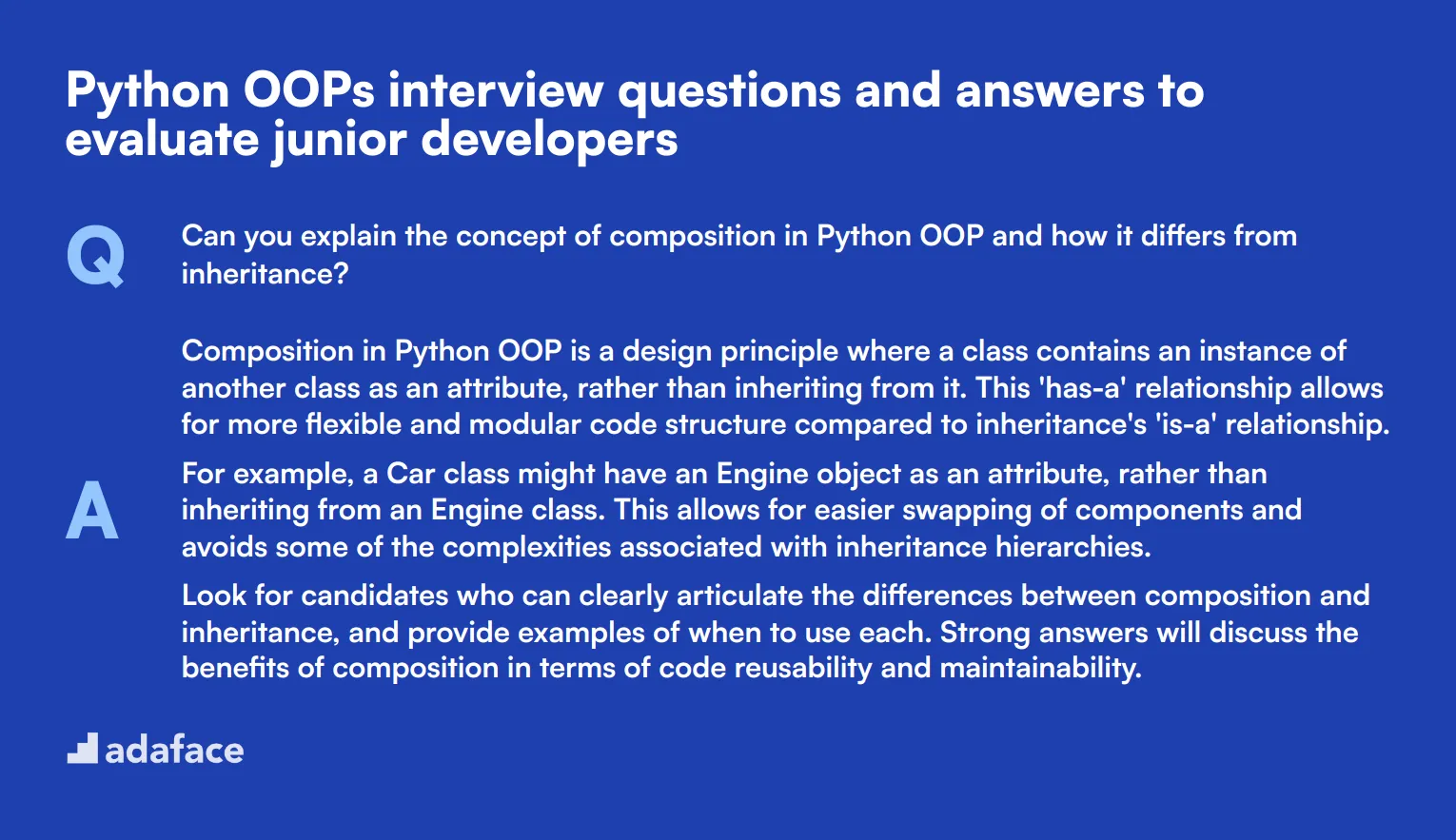
Ready to evaluate junior developers for their Python OOP skills? These 8 questions will help you assess their understanding of object-oriented programming concepts in a practical, interview-friendly format. Use this list to gauge candidates' knowledge and problem-solving abilities, ensuring you find the right Python developer for your team.
1. Can you explain the concept of composition in Python OOP and how it differs from inheritance?
Composition in Python OOP is a design principle where a class contains an instance of another class as an attribute, rather than inheriting from it. This 'has-a' relationship allows for more flexible and modular code structure compared to inheritance's 'is-a' relationship.
For example, a Car class might have an Engine object as an attribute, rather than inheriting from an Engine class. This allows for easier swapping of components and avoids some of the complexities associated with inheritance hierarchies.
Look for candidates who can clearly articulate the differences between composition and inheritance, and provide examples of when to use each. Strong answers will discuss the benefits of composition in terms of code reusability and maintainability.
2. How would you implement a singleton pattern in Python?
A singleton pattern ensures that a class has only one instance and provides a global point of access to it. In Python, this can be implemented using class methods, decorators, or metaclasses.
One common approach is to use a class method as a constructor. This method checks if an instance already exists and returns it if so, or creates a new instance if not. The new method can also be overridden to achieve this.
Listen for candidates who can explain at least one method of implementing a singleton, and why it might be useful. Strong answers will discuss potential drawbacks of singletons and alternative patterns that might be more appropriate in certain situations.
3. What are magic methods in Python and can you give an example of how you might use one?
Magic methods, also known as dunder methods, are special methods in Python that have double underscores before and after their names. They allow classes to emulate the behavior of built-in types or implement operator overloading.
For example, the str method is used to define a string representation of an object, which is returned when str() is called on the object or when it's printed. Another common magic method is len, which allows an object to be used with the len() function.
Look for candidates who can explain the purpose of magic methods and provide examples beyond just init. Strong answers will demonstrate an understanding of how magic methods can make classes more Pythonic and integrate seamlessly with built-in functions and operators.
4. How does the property decorator work in Python and why is it useful?
The @property decorator in Python is used to define methods that act like attributes, allowing for more control over attribute access. It's a way to implement getters, setters, and deleters for class attributes without explicitly calling them as methods.
This is useful for several reasons:
- It allows you to add logic (like validation) when getting or setting attributes
- It maintains backward compatibility if you need to change an attribute to a computed value
- It provides a clean, Pythonic way to encapsulate private attribute access
Evaluate candidates based on their ability to explain how @property works and provide practical examples of its use. Strong answers will discuss how it relates to the principle of encapsulation in OOP and how it can improve code readability and maintainability.
5. Explain the concept of method resolution order (MRO) in Python and why it's important in multiple inheritance scenarios.
Method Resolution Order (MRO) in Python determines the order in which base classes are searched when looking for a method in inheritance hierarchies. It's particularly important in multiple inheritance scenarios to resolve the 'diamond problem' where a class inherits from two classes that have a common ancestor.
Python uses the C3 linearization algorithm to determine the MRO. This ensures a consistent and predictable order for method resolution, avoiding ambiguities that can arise in complex inheritance structures.
Look for candidates who can explain the basics of MRO and its importance in multiple inheritance. Strong answers will mention the C3 linearization algorithm and possibly demonstrate how to view a class's MRO using the mro attribute or the mro() method.
6. What are metaclasses in Python and how might you use them?
Metaclasses in Python are classes for classes. They define how classes behave, allowing you to customize the class creation process. When a class is created, Python uses its metaclass to determine its behavior.
Metaclasses can be used for various purposes, such as:
- Automatically registering classes in a system
- Implementing singleton patterns
- Adding methods or attributes to classes
- Enforcing coding standards or design patterns
Assess candidates based on their understanding of metaclasses and their ability to provide practical use cases. Strong answers will demonstrate an awareness that metaclasses are an advanced feature and should be used judiciously, as they can make code harder to understand and maintain.
7. How would you implement a custom iterator in Python?
To implement a custom iterator in Python, you need to define a class with two special methods: iter() and next(). The iter() method returns the iterator object itself, usually just 'return self'. The next() method returns the next value in the sequence or raises a StopIteration exception when there are no more items.
For example, you might create a custom iterator for a range of numbers with a step value, or for traversing a custom data structure in a specific order.
Look for candidates who can explain the basic structure of an iterator class and the purpose of iter() and next(). Strong answers will include an example implementation and discuss the benefits of custom iterators, such as memory efficiency for large datasets or complex traversal patterns.
8. Can you explain the concept of duck typing in Python and how it relates to OOP?
Duck typing is a concept in Python where the type or class of an object is less important than the methods it defines. It's based on the idea that 'if it walks like a duck and quacks like a duck, then it must be a duck.' In practice, this means that Python doesn't care about the type of an object, only whether it has the attributes and methods you're trying to use.
This concept is closely related to OOP in Python because it allows for more flexible and dynamic code. Instead of checking an object's type, you simply try to use its methods. If the methods exist and work correctly, the object is considered suitable for the task at hand.
Evaluate candidates based on their ability to explain duck typing and its implications for software development. Strong answers will discuss how duck typing promotes polymorphism and can lead to more maintainable and extensible code, while also mentioning potential drawbacks like reduced type safety.
18 intermediate Python OOPs interview questions and answers to ask mid-tier developers
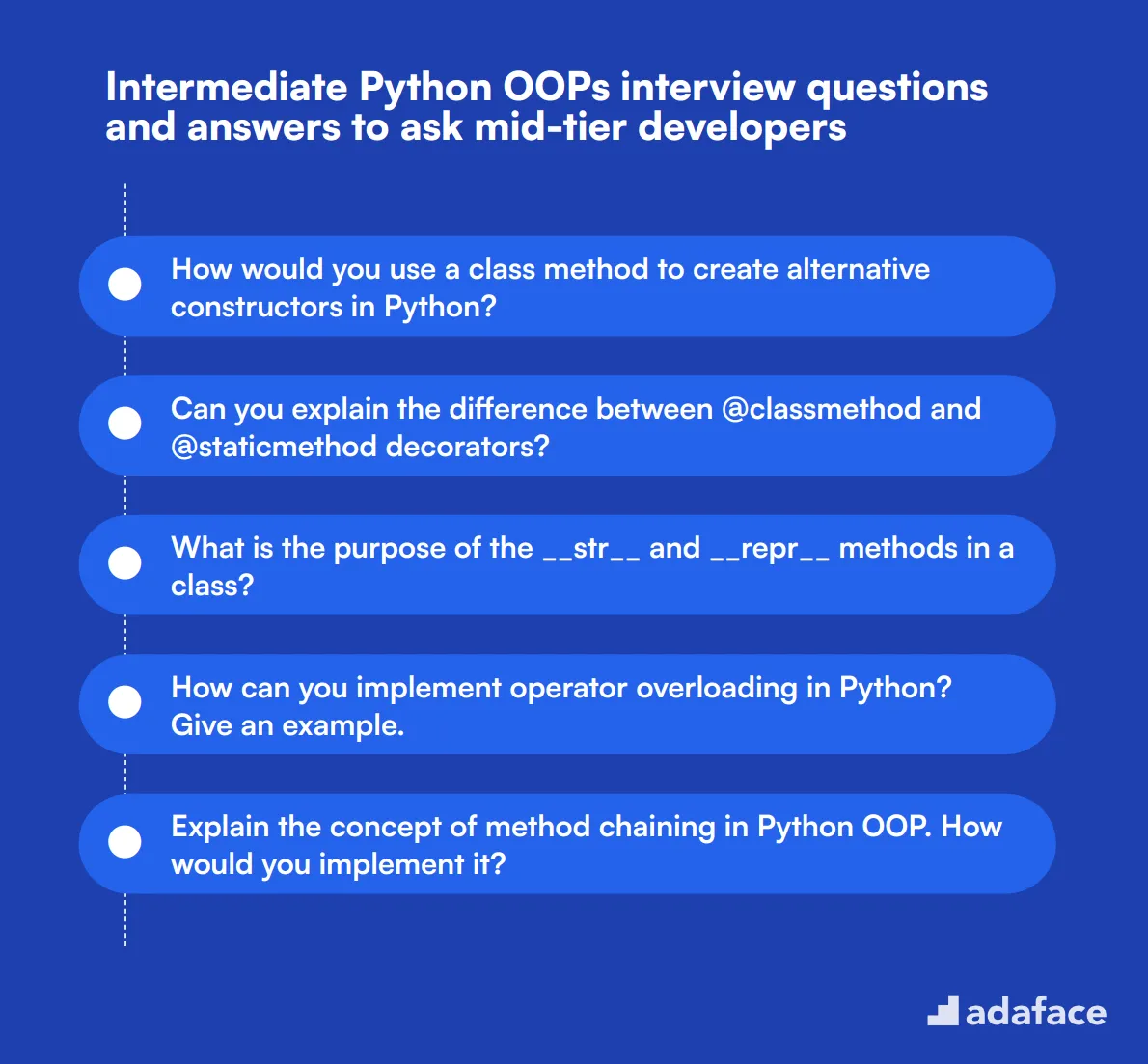
To assess the Python skills of mid-tier developers, use these 18 intermediate Python OOPs interview questions. These questions will help you evaluate candidates' understanding of more complex object-oriented programming concepts and their ability to apply them in real-world scenarios.
- How would you use a class method to create alternative constructors in Python?
- Can you explain the difference between @classmethod and @staticmethod decorators?
- What is the purpose of the __str__ and __repr__ methods in a class?
- How can you implement operator overloading in Python? Give an example.
- Explain the concept of method chaining in Python OOP. How would you implement it?
- What are mixins in Python and how are they useful in multiple inheritance scenarios?
- How would you use the @property decorator to create a read-only attribute?
- Can you explain the difference between shallow copy and deep copy in Python objects?
- What is the purpose of the __slots__ attribute in a Python class?
- How would you implement a context manager using a class in Python?
- Explain the concept of descriptors in Python and provide a use case.
- What is the difference between __getattr__ and __getattribute__ methods?
- How can you use the @dataclass decorator in Python? What are its benefits?
- Explain the concept of method resolution order (MRO) in Python's multiple inheritance.
- How would you implement a custom exception class in Python?
- What is the purpose of the __call__ method in a Python class?
- How can you use abstract base classes to define interfaces in Python?
- Explain the concept of metaclasses and provide a practical example of their use.
7 Python OOPs interview questions and answers related to design patterns
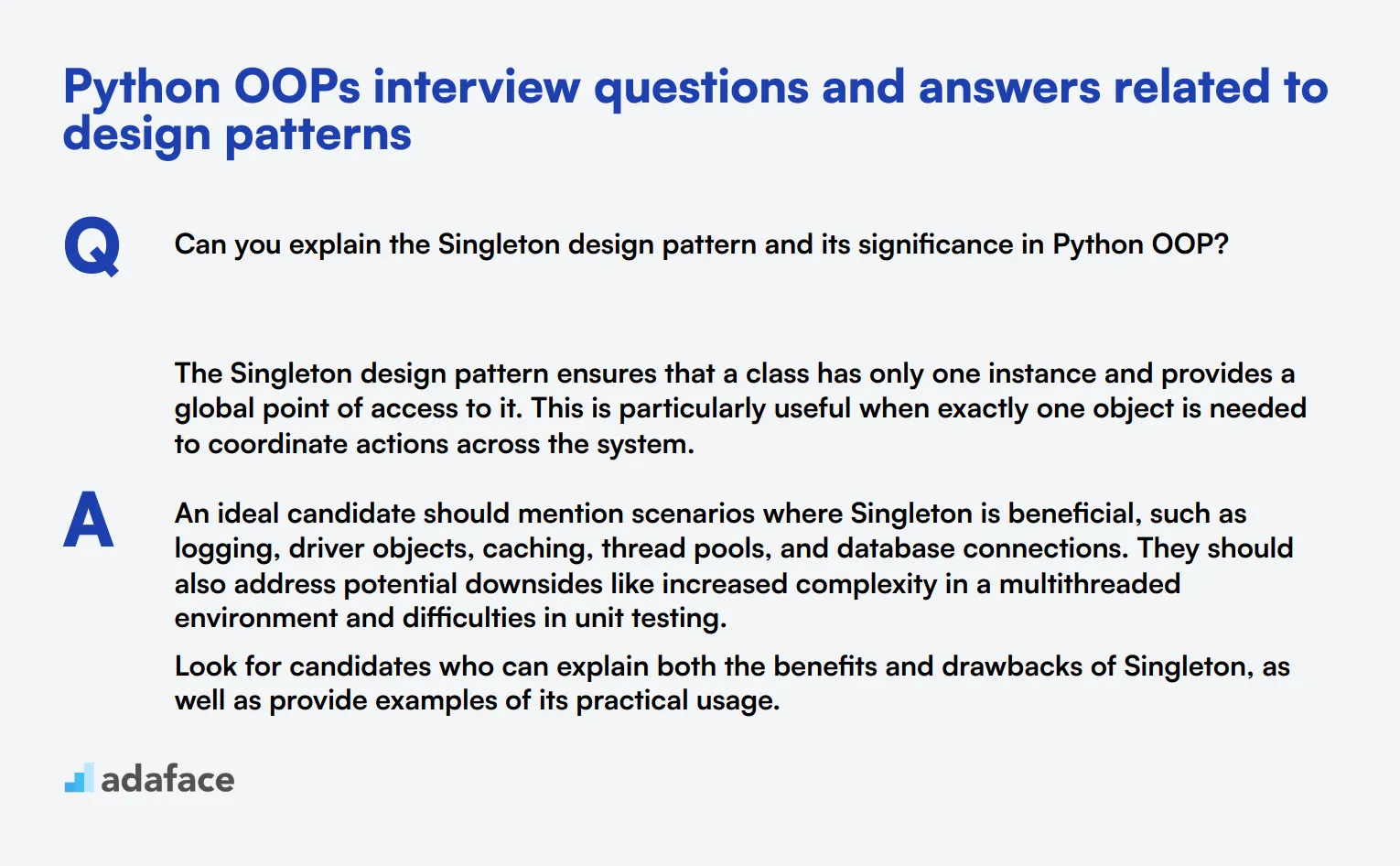
To determine whether your candidates have a solid understanding of design patterns in Python OOP, ask them some of these 7 interview questions. These questions will help you gauge their ability to apply design patterns effectively in real-world scenarios, ensuring they can tackle complex software design challenges with ease.
1. Can you explain the Singleton design pattern and its significance in Python OOP?
The Singleton design pattern ensures that a class has only one instance and provides a global point of access to it. This is particularly useful when exactly one object is needed to coordinate actions across the system.
An ideal candidate should mention scenarios where Singleton is beneficial, such as logging, driver objects, caching, thread pools, and database connections. They should also address potential downsides like increased complexity in a multithreaded environment and difficulties in unit testing.
Look for candidates who can explain both the benefits and drawbacks of Singleton, as well as provide examples of its practical usage.
2. What is the Factory Method design pattern and when would you use it?
The Factory Method design pattern defines an interface for creating an object but allows subclasses to alter the type of objects that will be created. It provides a way to delegate the instantiation logic to child classes.
Candidates should highlight scenarios where Factory Method is useful, such as when the exact type of object to be created isn't known until runtime. They might also mention that it promotes loose coupling by reducing the dependency on concrete classes.
Strong responses will include situations where candidates have used the Factory Method in past projects and the benefits they observed, such as improved code maintainability and scalability.
3. Can you describe the Observer design pattern and give an example of its application in Python?
The Observer design pattern defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified and updated automatically. This pattern is commonly used in event handling systems.
Candidates should discuss real-world applications of the Observer pattern, such as in GUI frameworks where changes in the state of a model need to be reflected in all dependent views. They might also mention its use in implementing event listeners or pub-sub systems.
Look for candidates who can articulate the benefits of using the Observer pattern for decoupling and managing dependencies, as well as potential challenges like managing updates efficiently.
4. What is the Strategy design pattern and how does it improve code flexibility?
The Strategy design pattern defines a family of algorithms, encapsulates each one, and makes them interchangeable. This pattern allows the algorithm to vary independently from clients that use it.
Candidates should explain how the Strategy pattern can improve code flexibility by promoting the use of composition over inheritance to allow swapping of algorithms at runtime. They might also mention examples like sorting algorithms or different behaviors in a game character.
An ideal candidate will discuss both the advantages, such as increased flexibility and easier maintenance, and any potential trade-offs, such as the overhead of managing multiple strategy classes.
5. How does the Decorator design pattern work and when would you apply it?
The Decorator design pattern allows behavior to be added to individual objects, either statically or dynamically, without affecting the behavior of other objects from the same class. It’s like a flexible alternative to subclassing.
Candidates should mention use cases such as adding responsibilities to objects in layers, like UI components or adding logging, authentication, or caching behaviors. They might also highlight the pattern's ability to adhere to the Open/Closed Principle by allowing behavior to be added without modifying existing code.
Look for candidates who can provide practical examples of using the Decorator pattern and discuss its benefits in terms of flexibility and code reuse.
6. What is the Adapter design pattern and how can it be used in Python?
The Adapter design pattern allows incompatible interfaces to work together. It acts as a bridge between two incompatible interfaces by converting the interface of a class into another interface clients expect.
Candidates should discuss scenarios where Adapter is useful, such as integrating new components into an existing system without modifying the existing code. They might also mention examples like adapting a third-party library to fit the application’s interface.
An ideal candidate will provide real-world examples of using the Adapter pattern and explain how it helps in achieving software integration.
7. Can you explain the Builder design pattern and its advantages in object creation?
The Builder design pattern separates the construction of a complex object from its representation so that the same construction process can create different representations. It is particularly useful for creating objects with multiple optional parameters.
Candidates should highlight the advantages of using the Builder pattern, such as improved readability and maintainability of the code by abstracting the complex construction process. They might also mention its use in scenarios like constructing complex configurations or assembling objects from multiple parts.
Look for candidates who can provide examples of using the Builder pattern in real projects and discuss the benefits they observed, such as reduced code duplication and improved clarity.
12 Python OOPs interview questions about inheritance
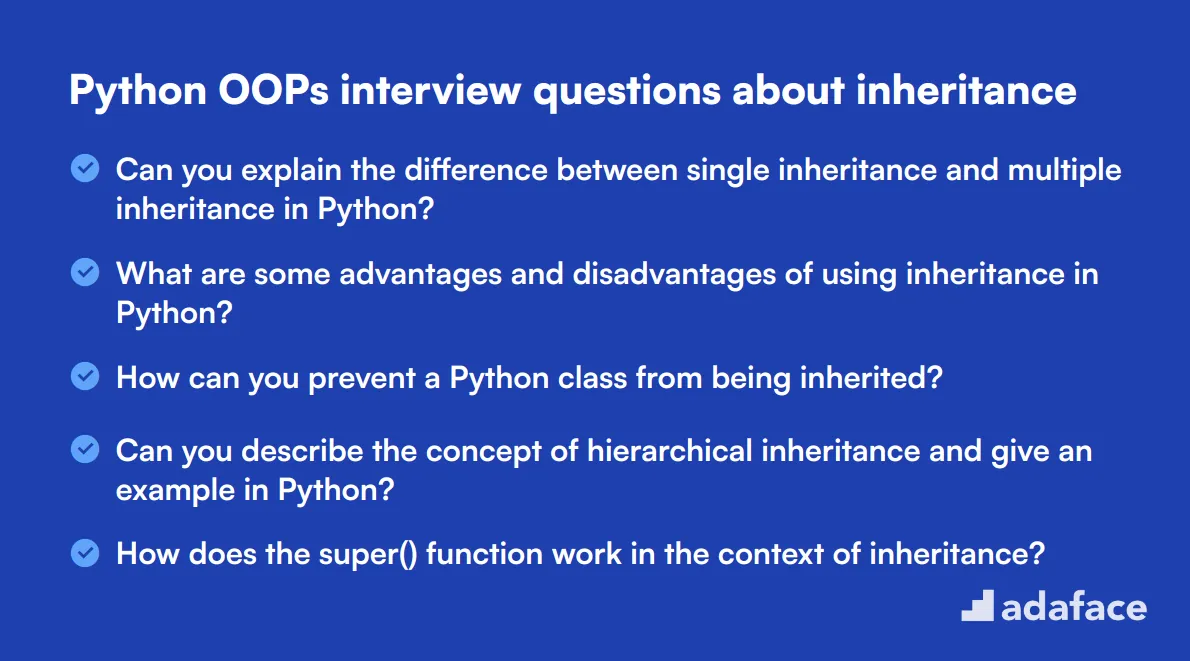
To determine whether your applicants have the right understanding of Python inheritance concepts, ask them some of these Python OOPs interview questions about inheritance. These questions are designed to help recruiters and hiring managers gauge candidates' proficiency and practical knowledge in inheritance, a key pillar in object-oriented programming. For more information on what makes a well-rounded Python developer, refer to this job description.
- Can you explain the difference between single inheritance and multiple inheritance in Python?
- What are some advantages and disadvantages of using inheritance in Python?
- How can you prevent a Python class from being inherited?
- Can you describe the concept of hierarchical inheritance and give an example in Python?
- How does the super() function work in the context of inheritance?
- What is the difference between method overriding and method overloading in Python?
- How would you handle diamond problem when using multiple inheritance in Python?
- Can you provide an example of how to use the `issubclass()` function in Python?
- Explain the concept of hybrid inheritance and its usage with an example in Python.
- How does inheritance impact the performance of a Python program?
- What role does the __mro__ attribute play in inheritance?
- How can you use inheritance to achieve code reusability in Python?
10 situational Python OOPs interview questions for hiring top developers
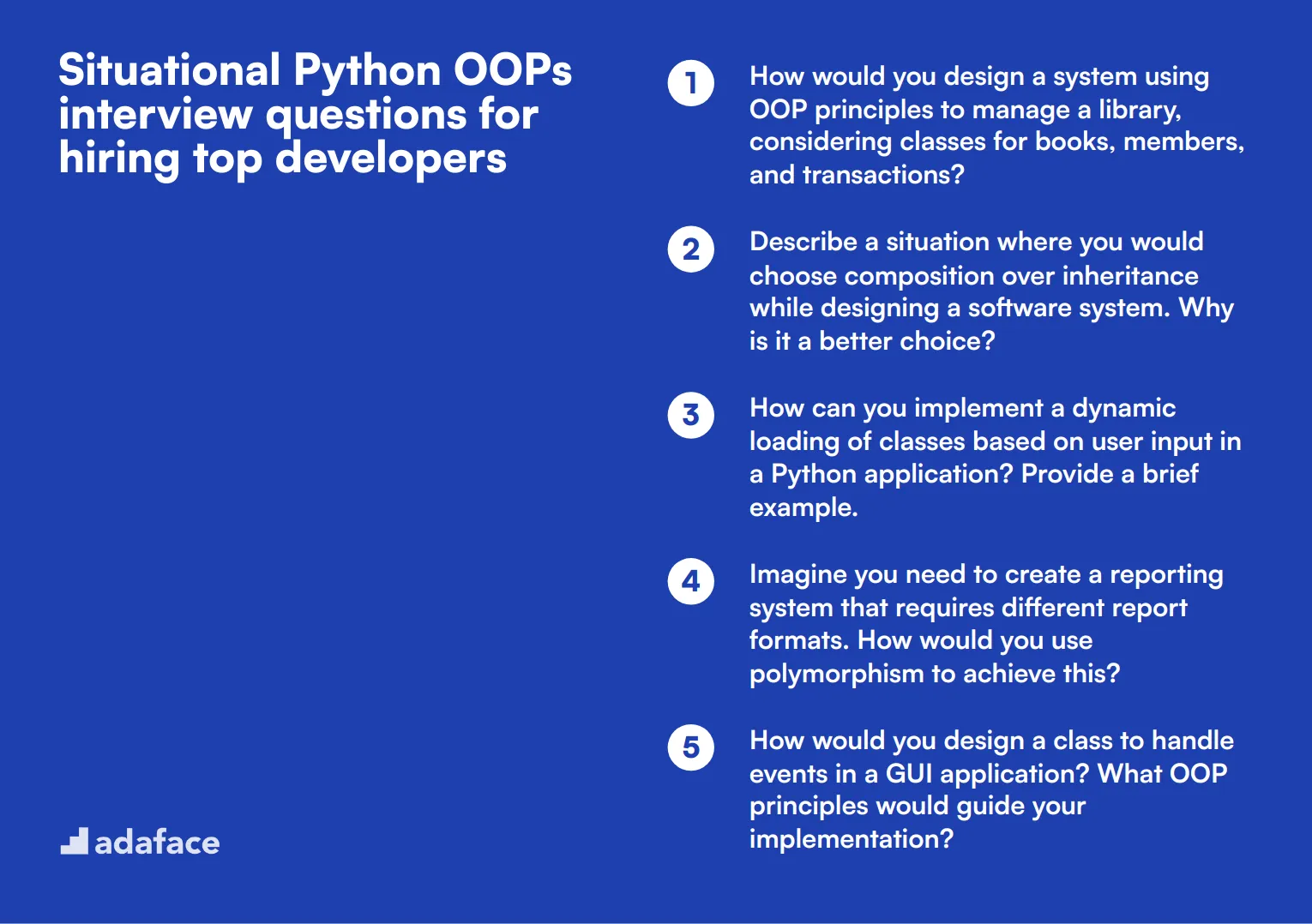
To assess candidates' understanding of advanced Python concepts in OOP, leverage these situational interview questions. They allow you to gauge practical knowledge and problem-solving skills essential for roles like a Python Developer. Use them to spark insightful discussions and evaluate technical expertise.
- How would you design a system using OOP principles to manage a library, considering classes for books, members, and transactions?
- Describe a situation where you would choose composition over inheritance while designing a software system. Why is it a better choice?
- How can you implement a dynamic loading of classes based on user input in a Python application? Provide a brief example.
- Imagine you need to create a reporting system that requires different report formats. How would you use polymorphism to achieve this?
- How would you design a class to handle events in a GUI application? What OOP principles would guide your implementation?
- Can you outline your approach to creating a plugin system using OOP concepts in Python?
- If you were to create an application that connects to different databases, how would you apply the Strategy design pattern?
- How would you handle versioning in an API that uses OOP principles? What strategies would you implement?
- In a game development context, how would you utilize inheritance and polymorphism to manage different types of characters?
- What steps would you take to ensure that your classes are easily testable and maintainable through the principles of OOP?
Which Python OOPs skills should you evaluate during the interview phase?
While it's impossible to assess every aspect of a candidate's Python OOPs knowledge in a single interview, focusing on core skills can provide valuable insights. The following key areas are particularly important when evaluating a candidate's proficiency in Python OOPs.
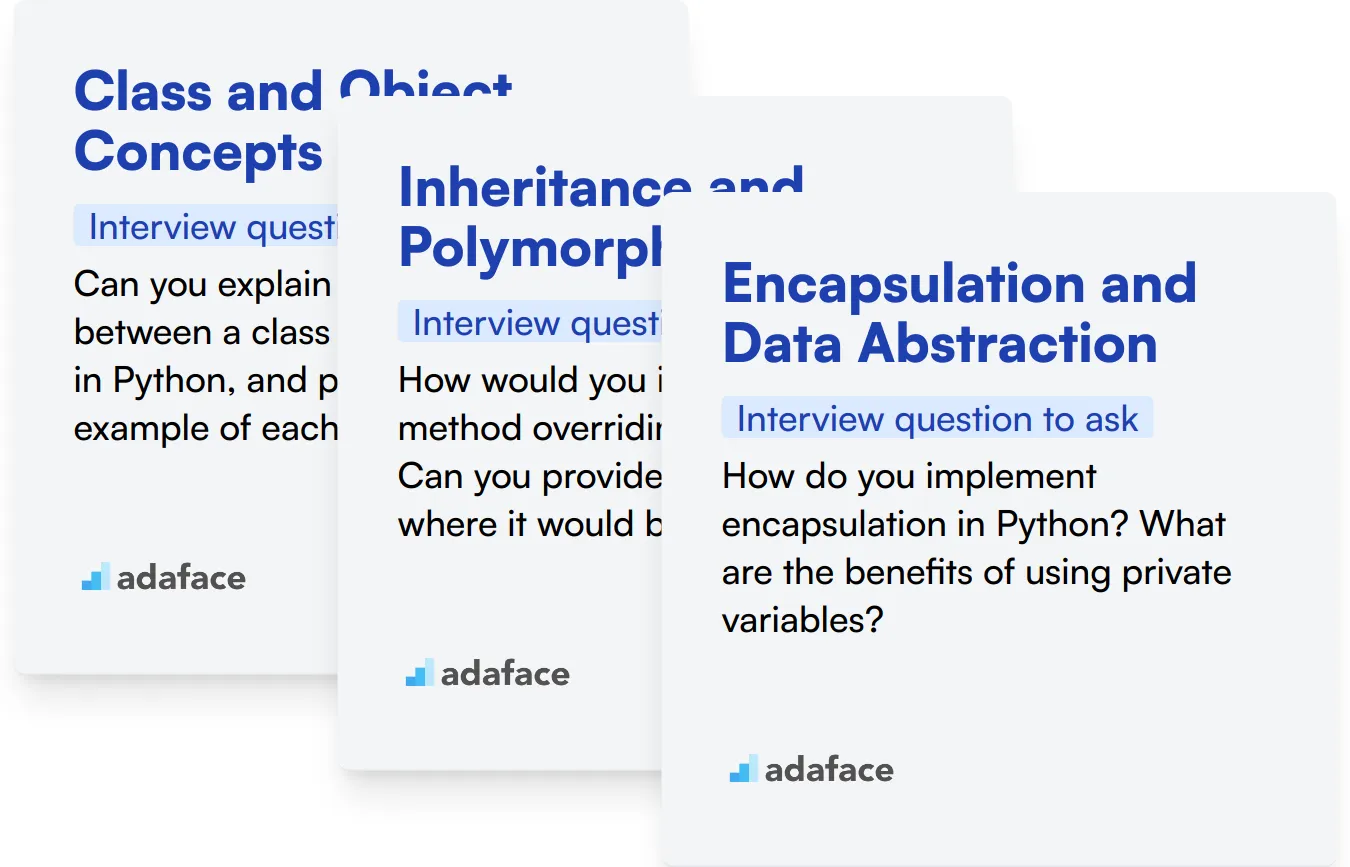
Class and Object Concepts
Understanding classes and objects is fundamental to OOP in Python. This skill forms the basis for creating reusable and modular code, which is essential for efficient software development.
To assess this skill, consider using an assessment test with relevant MCQs. Our Python online test includes questions targeting class and object concepts.
You can also ask targeted interview questions to evaluate this skill. Here's an example question to consider:
Can you explain the difference between a class and an instance in Python, and provide a simple example of each?
Look for answers that clearly differentiate between a class as a blueprint and an instance as a concrete object. The candidate should be able to provide a simple example, demonstrating their practical understanding of these concepts.
Inheritance and Polymorphism
Inheritance and polymorphism are key OOP principles that enable code reuse and flexibility. Proficiency in these areas indicates a deeper understanding of OOP design patterns and best practices.
An assessment test with MCQs focusing on inheritance and polymorphism can be helpful. Our Python test includes questions that evaluate these concepts.
To further assess this skill, consider asking a question like:
How would you implement method overriding in Python? Can you provide an example where it would be useful?
Look for answers that demonstrate understanding of method overriding in child classes. The candidate should be able to explain a practical scenario where method overriding enhances code functionality or readability.
Encapsulation and Data Abstraction
Encapsulation and data abstraction are crucial for creating secure and maintainable code. These concepts are essential for effective software design and are widely used in professional Python development.
To evaluate this skill, you might ask:
How do you implement encapsulation in Python? What are the benefits of using private variables?
Look for answers that mention using double underscores for private variables and explain the benefits of encapsulation, such as data protection and reducing dependencies between different parts of the code.
Elevate Your Python Team: Combine OOPs Interview Questions with Skills Tests
Hiring skilled Python developers with strong OOPs knowledge is key for building robust applications. To ensure candidates possess these skills, a thorough evaluation process is necessary. This helps in finding the right fit for your team and project needs.
The most effective way to assess Python OOPs skills is through a combination of skills tests and targeted interview questions. Consider using a Python online test to objectively evaluate candidates' coding abilities and OOPs concepts.
After candidates complete the skills test, you can shortlist the top performers for interviews. During these interviews, use the OOPs questions discussed in this post to dive deeper into their understanding and problem-solving approach. This two-step process helps in identifying truly talented Python developers.
Ready to streamline your Python hiring process? Sign up for Adaface to access our Python skills tests and start building your dream team. Our coding tests are designed to help you find the best tech talent efficiently.
Python Online Test
Download Python OOPs interview questions template in multiple formats
Python OOPs Interview Questions FAQs
The questions cover basic, junior, intermediate, and advanced levels, including design patterns and situational scenarios.
Use them to assess candidates' Python OOPs knowledge during interviews, complementing technical assessments and coding tests.
Yes, the post includes sections for basic, junior, intermediate, and advanced developers, allowing targeted assessment.
Absolutely. The post includes situational questions to evaluate how candidates apply OOPs concepts in real-world scenarios.
Combine these interview questions with practical coding tests and projects to get a well-rounded assessment of candidates' abilities.
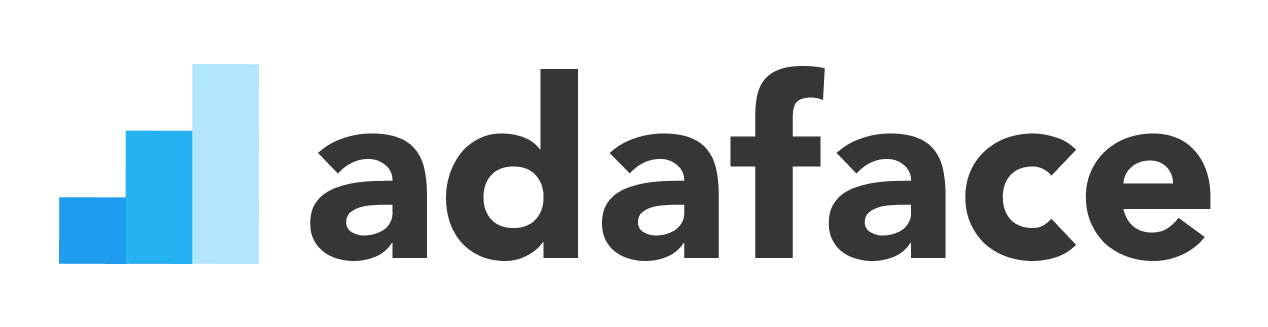
40 min skill tests.
No trick questions.
Accurate shortlisting.
We make it easy for you to find the best candidates in your pipeline with a 40 min skills test.
Try for freeRelated posts
Free resources
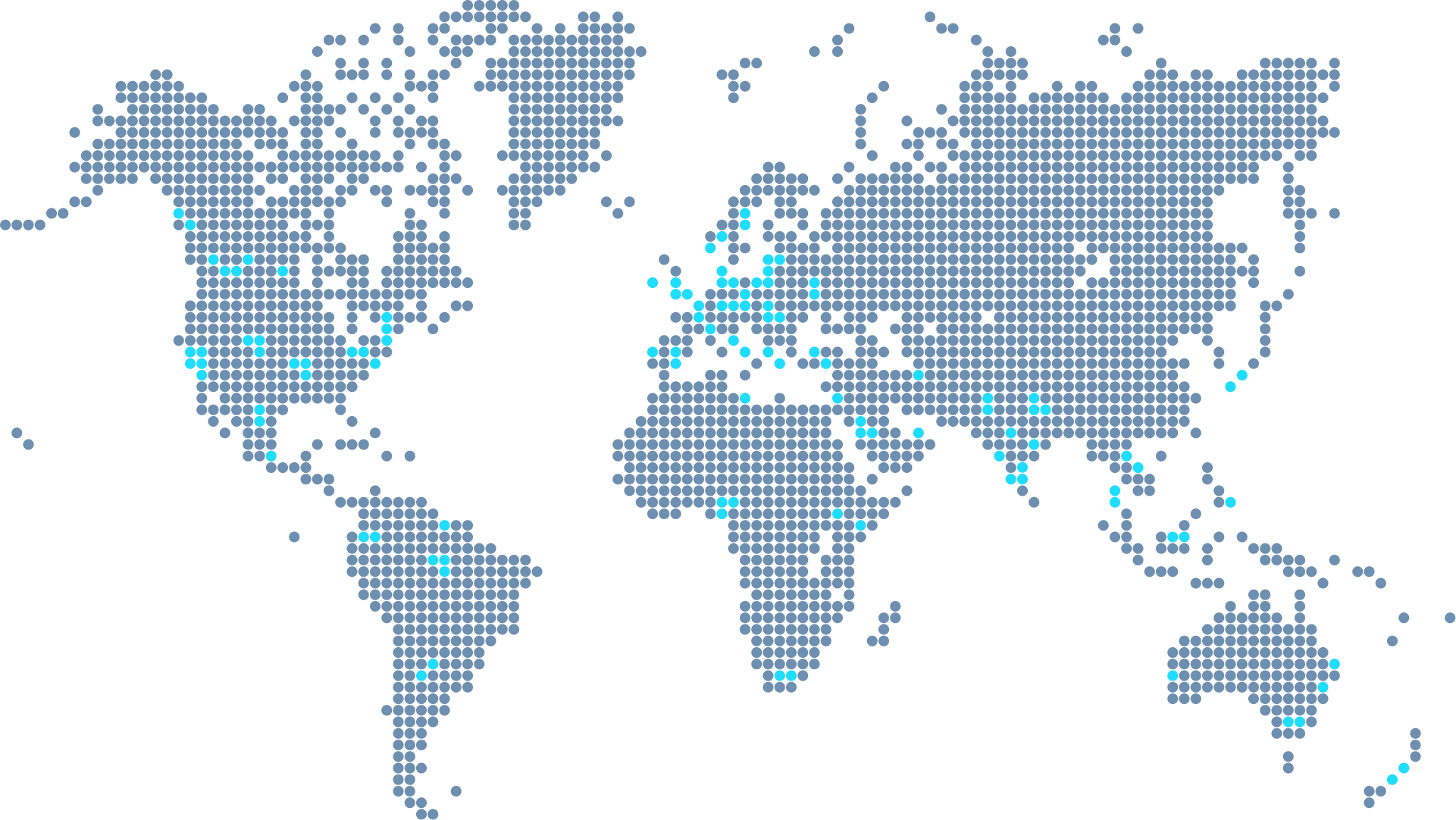
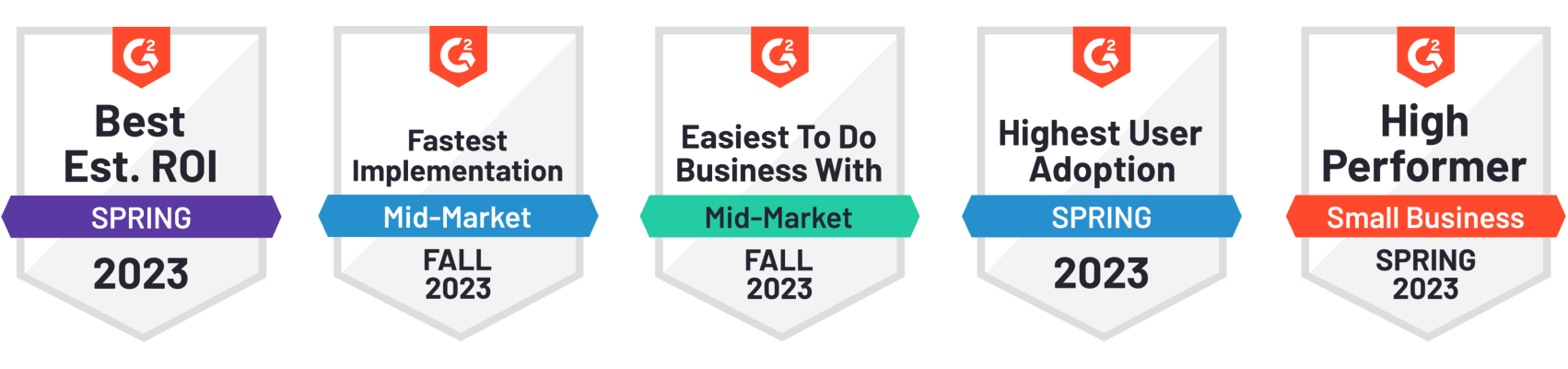