Python debugging skills are critical for developers to efficiently identify and fix issues in their code. Assessing these skills during the hiring process helps ensure you bring on board Python developers who can maintain and improve your codebase effectively.
This blog post provides a comprehensive set of Python debugging interview questions for different experience levels, from junior to senior developers. We cover general debugging concepts, specific techniques, processes, and situational questions to help you evaluate candidates thoroughly.
By using these questions, you can gauge a candidate's problem-solving abilities and their familiarity with Python's debugging tools and best practices. Consider pairing these interview questions with a Python skills assessment to get a well-rounded view of each applicant's capabilities.
Table of contents
8 general Python Debugging interview questions and answers to assess applicants
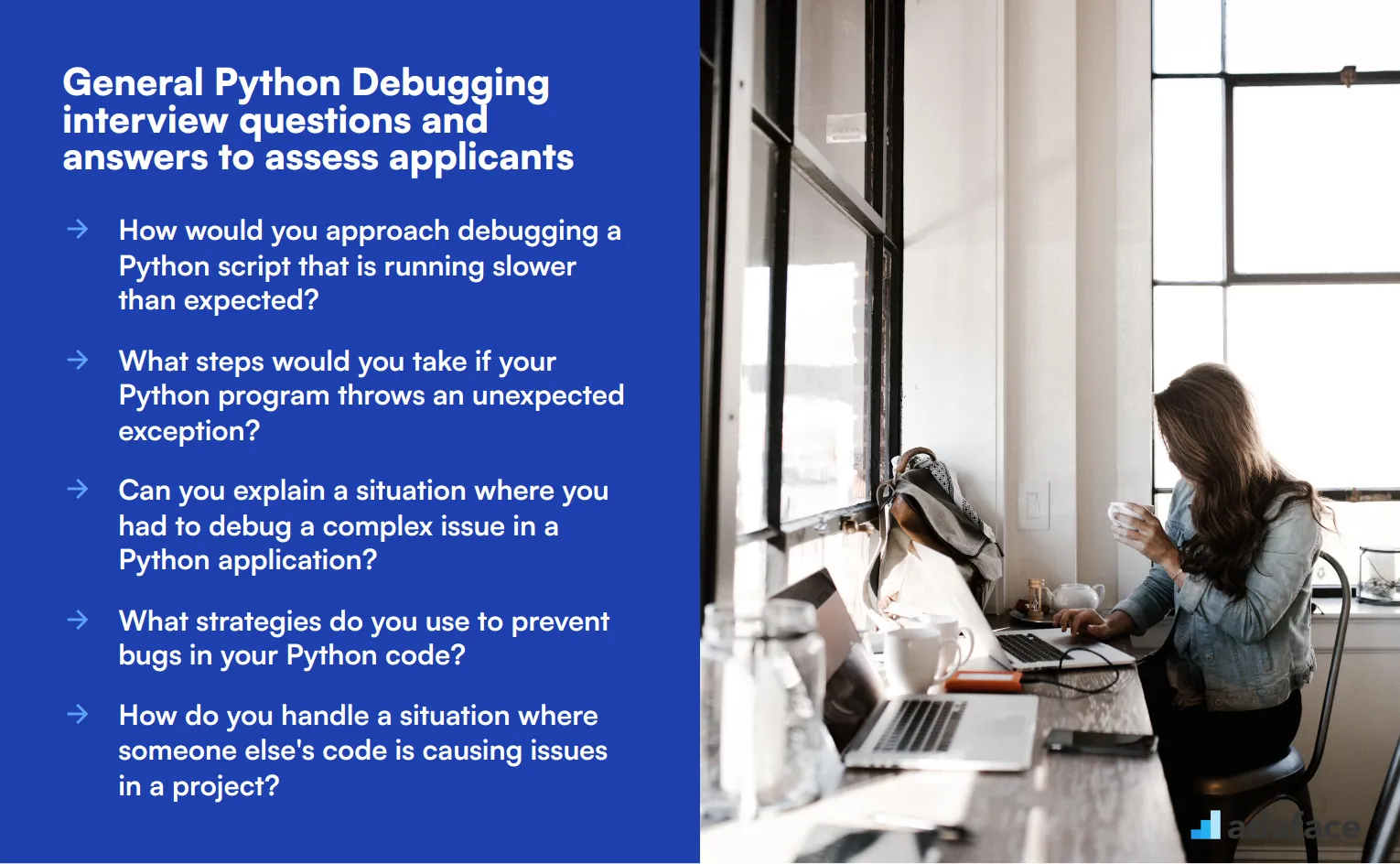
To determine whether your applicants have the right skills to troubleshoot and debug Python programs effectively, ask them these 8 well-rounded Python debugging interview questions. These questions will help you gauge their practical understanding and problem-solving abilities.
1. How would you approach debugging a Python script that is running slower than expected?
An effective candidate will start by explaining the importance of identifying the root cause of the slowdown. They may mention using profiling tools to measure where the time is being spent in the code.
They might also talk about checking for common performance bottlenecks like inefficient loops, unnecessary computations, or blocking I/O operations.
Look for candidates who can articulate a systematic approach to debugging, such as isolating different parts of the code and testing them independently. This shows a structured problem-solving mindset.
2. What steps would you take if your Python program throws an unexpected exception?
A strong candidate would likely describe first understanding the exception message to identify what kind of error it is and where it occurred in the code.
They might also discuss using debugging tools like breakpoints to examine the state of the program at the point where the exception is thrown.
Ideal responses should include checking the stack trace, isolating the problematic part of the code, and writing test cases to ensure the issue is resolved and doesn't recur.
3. Can you explain a situation where you had to debug a complex issue in a Python application?
Here, candidates should share a specific example from their experience, detailing the issue, their debugging process, and the solution they implemented.
They should highlight steps they took to diagnose the problem, such as logging, using debugging tools, or consulting documentation.
An ideal answer will show their ability to handle complex scenarios, demonstrating persistence and a methodical approach to problem-solving.
4. What strategies do you use to prevent bugs in your Python code?
Candidates should mention practices like writing unit tests and integrating continuous testing into the development process.
They might also discuss code reviews, adhering to coding standards, and using linters to catch common mistakes early.
Look for responses that emphasize proactive measures and a commitment to writing clean, maintainable code. This shows foresight and professionalism.
5. How do you handle a situation where someone else's code is causing issues in a project?
A good candidate will describe the importance of clear communication and collaboration. They might mention starting with a code review to understand the problematic code.
They might also discuss strategies like pair programming, where they work together with the original author to solve the issue.
Look for answers that highlight their ability to work effectively in a team, resolve conflicts diplomatically, and contribute to a positive work environment.
6. What tools and techniques do you find most useful for debugging Python applications?
Candidates should mention standard tools like Python's built-in debugger (pdb), as well as other tools like PyCharm, VSCode, or logging libraries.
They might also discuss techniques like using breakpoints, step execution, and inspecting variables and stack traces.
Ideal candidates will have a comprehensive toolkit and can explain why they prefer certain tools over others, showing both experience and critical thinking.
7. Explain how you would debug a memory leak in a Python application.
A strong candidate will discuss identifying memory usage patterns and possibly using tools like tracemalloc
to track memory allocations.
They might also talk about looking for common culprits like circular references or objects that are not being garbage collected.
Look for answers that demonstrate a clear strategy for isolating and fixing memory leaks, as well as an understanding of Python's memory management.
8. How do you ensure your Python code is easy to debug and maintain?
Candidates might mention writing clear, readable code with meaningful variable names and comments.
They could also discuss structuring their programs in a modular way, making it easier to isolate and test individual components.
Look for responses that emphasize best practices in software engineering, such as adhering to coding standards and writing comprehensive documentation. This shows a commitment to quality and maintainability.
20 Python Debugging interview questions to ask junior developers
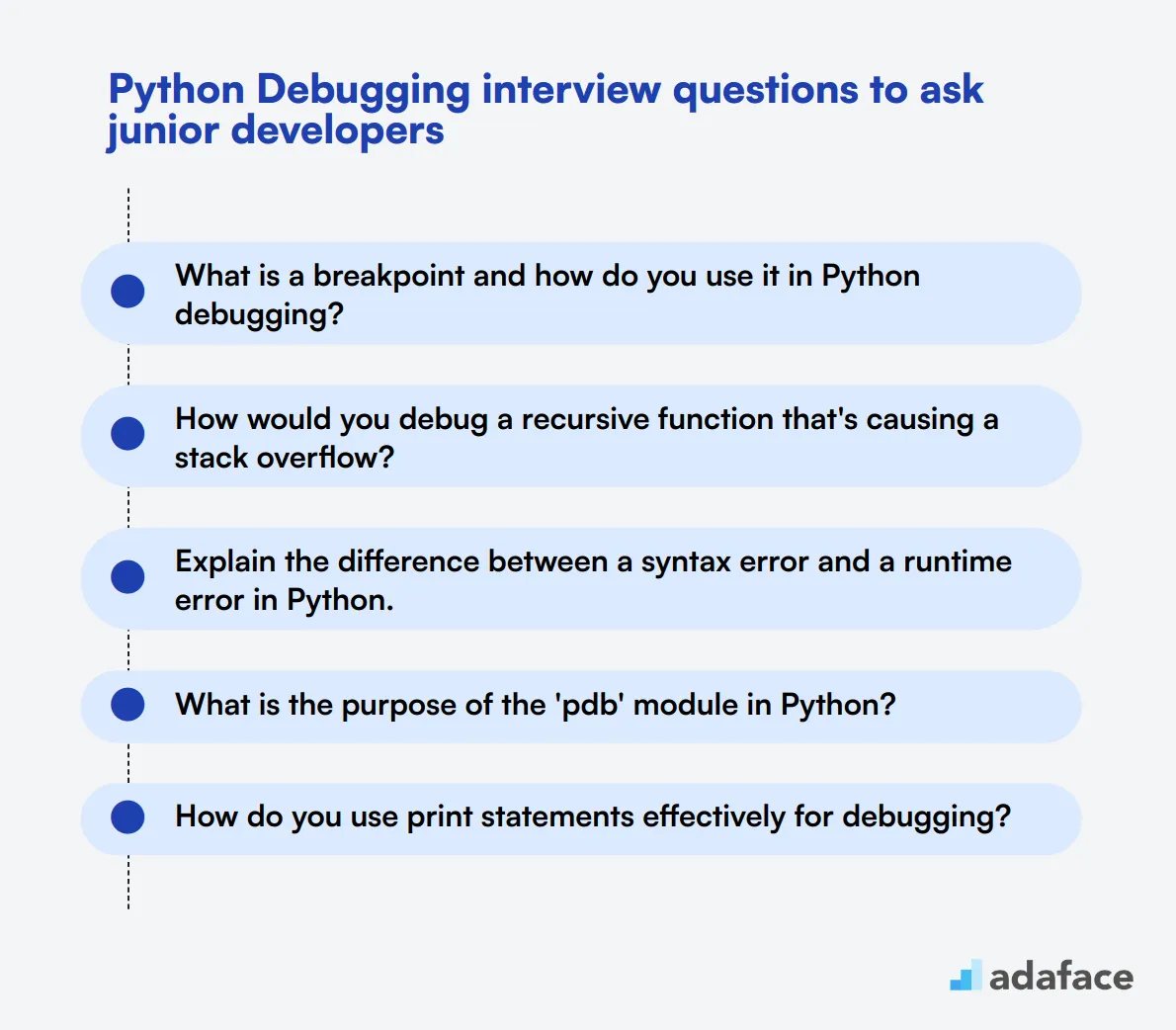
To assess junior Python developers' debugging skills effectively, use these 20 interview questions. They help evaluate candidates' ability to identify, troubleshoot, and resolve common coding issues. These questions are designed to be practical and reveal real-world problem-solving capabilities.
- What is a breakpoint and how do you use it in Python debugging?
- How would you debug a recursive function that's causing a stack overflow?
- Explain the difference between a syntax error and a runtime error in Python.
- What is the purpose of the 'pdb' module in Python?
- How do you use print statements effectively for debugging?
- What is a logical error and how would you approach debugging it?
- Explain how you would use logging instead of print statements for debugging.
- How do you debug a Python script that's producing incorrect output?
- What is the significance of the 'traceback' in Python error messages?
- How would you debug a Python script that's hanging or not terminating?
- Explain the concept of step-through debugging in Python.
- How do you use assertions in Python for debugging?
- What tools would you use to profile a Python script for performance issues?
- How do you debug race conditions in multi-threaded Python programs?
- Explain how you would use a debugger to inspect variable values during runtime.
- What strategies do you use to debug import errors in Python?
- How would you approach debugging a Python script that works locally but fails in production?
- Explain the use of the 'try-except' block in debugging Python code.
- How do you debug issues related to Python's indentation?
- What techniques would you use to debug memory usage in a large Python application?
10 intermediate Python Debugging interview questions and answers to ask mid-tier developers.
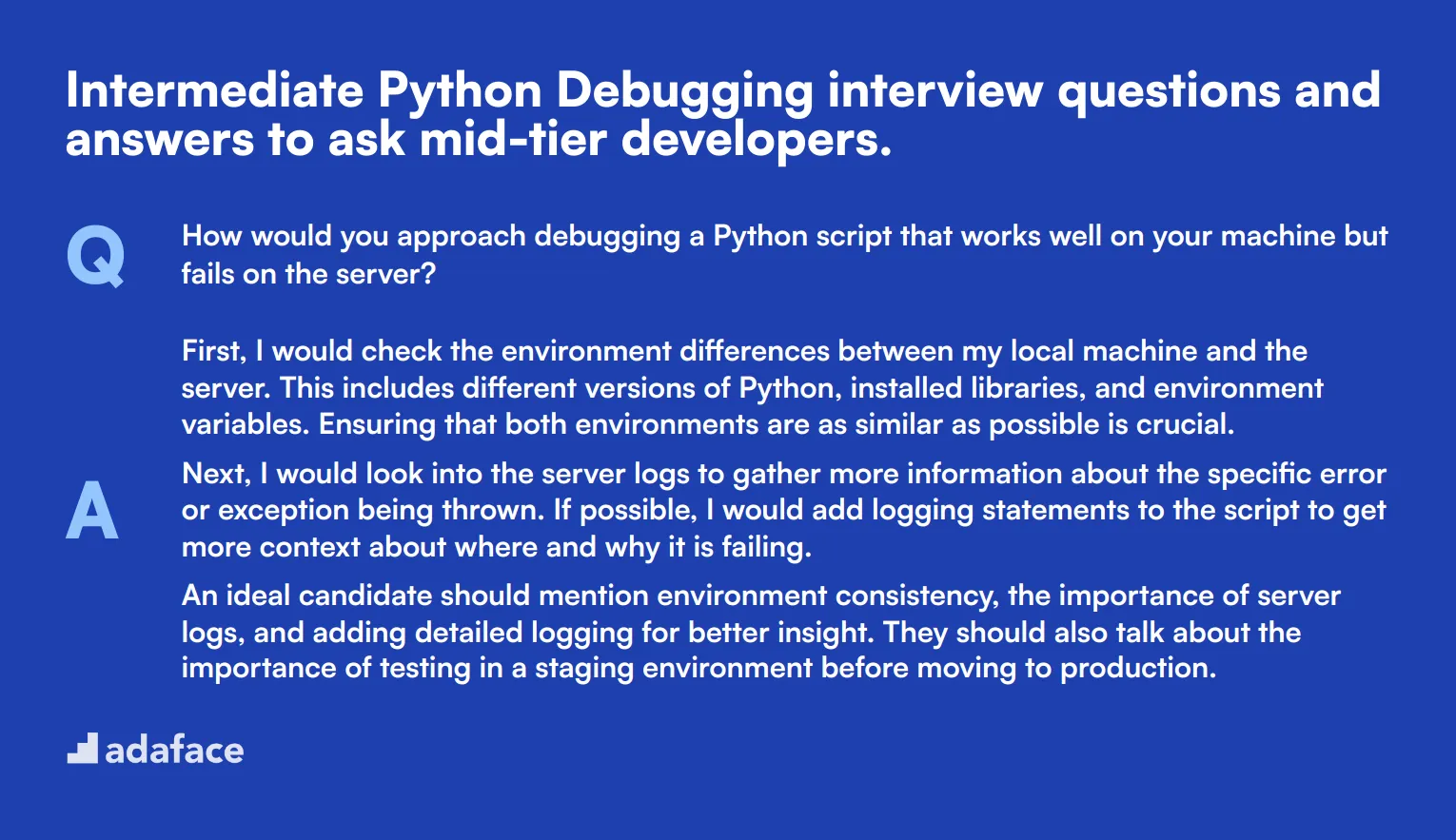
To identify mid-tier developers who are adept at debugging Python, refer to this list of intermediate debugging questions. These questions will help you gauge the candidates' problem-solving skills and their ability to troubleshoot effectively in real-world scenarios.
1. How would you approach debugging a Python script that works well on your machine but fails on the server?
First, I would check the environment differences between my local machine and the server. This includes different versions of Python, installed libraries, and environment variables. Ensuring that both environments are as similar as possible is crucial.
Next, I would look into the server logs to gather more information about the specific error or exception being thrown. If possible, I would add logging statements to the script to get more context about where and why it is failing.
An ideal candidate should mention environment consistency, the importance of server logs, and adding detailed logging for better insight. They should also talk about the importance of testing in a staging environment before moving to production.
2. Can you explain how you would debug an issue where a Python script is consuming too much memory?
I would start by using memory profiling tools to identify which parts of the script are consuming the most memory. Tools like memory_profiler
can be very useful for this purpose.
Next, I would look into optimizing the usage of data structures and algorithms. Sometimes, inefficient use of lists, dictionaries, or other data structures can lead to excessive memory consumption.
A strong response should highlight the use of profiling tools and the importance of optimizing data structures. They should also mention the significance of understanding memory allocation and deallocation in Python.
3. What steps would you take to debug a Python application that crashes without any error messages?
First, I would run the application in a controlled environment to see if I can reproduce the crash. Running in a debug mode or using a debugger can help capture more information.
I would then add extensive logging throughout the application to pinpoint where the crash is happening. Logs can provide insights that may not be obvious from just running the application.
Ideal candidates should focus on reproducing the issue, the importance of detailed logging, and perhaps using a debugger to step through the code. They should also talk about checking system-level logs if the issue persists.
4. How do you handle debugging a Python script that interacts with an API, but the API is returning unexpected results?
First, I would verify the API endpoint, parameters, and headers being used in the script to ensure they match the API documentation. Sometimes, a minor typo can lead to unexpected results.
Next, I would use tools like Postman to manually test the API endpoints and compare the results with what my script is getting. This helps isolate whether the issue is with the API or the script itself.
Candidates should demonstrate their ability to verify API parameters and use tools like Postman for manual testing. They should also discuss the importance of understanding the API documentation thoroughly.
5. What methods do you use to debug permission issues in a Python script running on a different system?
I would start by checking the file and directory permissions to ensure the script has the necessary access rights. This includes checking read, write, and execute permissions.
Next, I would review user permissions and roles, especially if the script interacts with databases or other services. Ensuring the user running the script has the appropriate permissions is crucial.
A good candidate should mention checking file and directory permissions, user roles, and perhaps using tools to diagnose permission issues. They should also consider the system's security policies.
6. Can you describe how you would debug a Python application that intermittently fails to connect to a database?
First, I would check the database server's status and logs to see if there are any connectivity issues or configuration problems. Intermittent failures often point to issues on the server side.
Next, I would add retry logic in the application to handle intermittent failures gracefully. This includes adding delays between retries and logging each attempt.
Ideal answers should include checking the database server status, adding retry logic, and ensuring proper logging to capture any intermittent failures. Candidates should focus on robustness and error handling.
7. How would you debug a Python application that suddenly starts throwing a lot of exceptions?
I would first locate the part of the application where the exceptions are being thrown. Adding logging or using a debugger can help pinpoint the issue.
Next, I would analyze the specific exceptions being thrown to understand their root cause. This might involve reviewing recent changes to the codebase or dependencies.
Candidates should emphasize locating the source of the exceptions, understanding their root cause, and reviewing recent changes that might have introduced the issue. They should also mention the importance of thorough testing.
8. What would you do if you suspect a third-party library is causing issues in your Python script?
I would first check the library's documentation and recent updates to see if there are any known issues or changes that could be causing the problem.
Next, I would try to isolate the issue by creating a minimal script that reproduces the problem. This helps confirm whether the third-party library is indeed the cause.
A good response should include checking the library's documentation, isolating the issue with a minimal script, and possibly looking for alternative libraries if the issue persists.
9. How do you handle debugging a Python script that interacts with a large dataset and is running out of memory?
First, I would look into optimizing the data processing logic to work in smaller chunks rather than loading the entire dataset into memory at once. Using generators and iterators can be very helpful.
Next, I would consider using external storage solutions like databases or data lakes to handle large datasets more efficiently.
Candidates should mention optimizing data processing, using generators and iterators, and considering external storage solutions. They should demonstrate a good understanding of handling large datasets efficiently.
10. Can you explain how you would debug a Python script that is supposed to run as a scheduled task but never starts?
First, I would check the scheduler's logs to see if there are any errors or issues preventing the task from starting. This includes checking the task's configuration and permissions.
Next, I would try to run the script manually to ensure it works as expected. This helps isolate whether the issue is with the script itself or the scheduler.
Ideal candidates should focus on checking scheduler logs, verifying task configuration, and running the script manually to isolate the issue. They should also consider any system-level restrictions or policies.
12 Python Debugging interview questions about debugging techniques
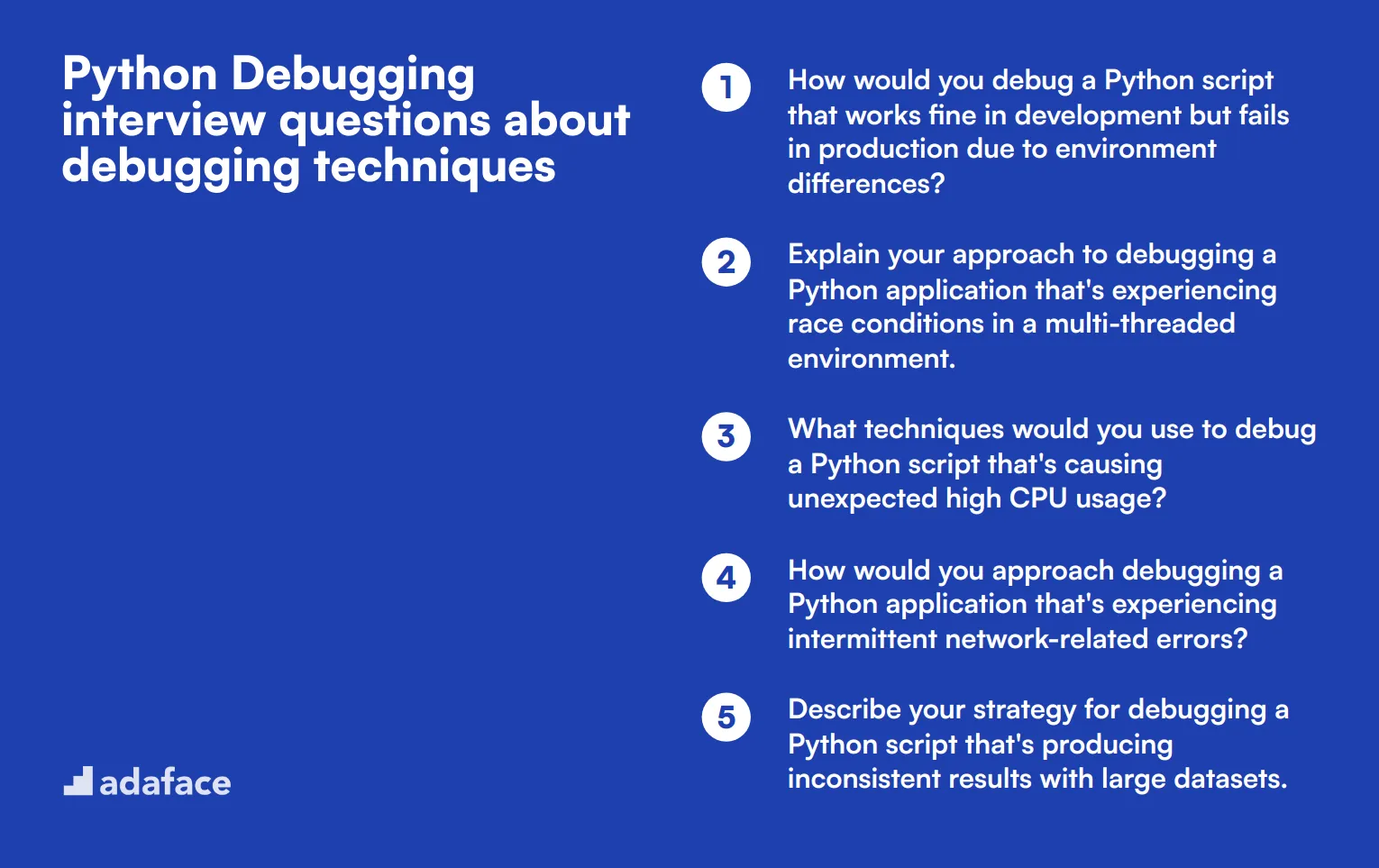
To assess a candidate's proficiency in Python debugging techniques, consider using these 12 interview questions. These questions are designed to evaluate the applicant's ability to identify, analyze, and resolve complex issues in Python code, helping you find the right talent for your team.
- How would you debug a Python script that works fine in development but fails in production due to environment differences?
- Explain your approach to debugging a Python application that's experiencing race conditions in a multi-threaded environment.
- What techniques would you use to debug a Python script that's causing unexpected high CPU usage?
- How would you approach debugging a Python application that's experiencing intermittent network-related errors?
- Describe your strategy for debugging a Python script that's producing inconsistent results with large datasets.
- How would you debug a Python application where a specific function is occasionally returning None unexpectedly?
- What steps would you take to debug a Python script that's experiencing unexpected behavior due to circular imports?
- Explain your approach to debugging a Python application where certain API calls are timing out sporadically.
- How would you debug a Python script that's exhibiting different behavior on different operating systems?
- What techniques would you use to debug a Python application that's experiencing subtle floating-point calculation errors?
- How would you approach debugging a Python script that's failing silently without raising exceptions?
- Describe your strategy for debugging a Python application where a specific module is occasionally not being imported correctly.
7 Python Debugging interview questions and answers related to debugging processes
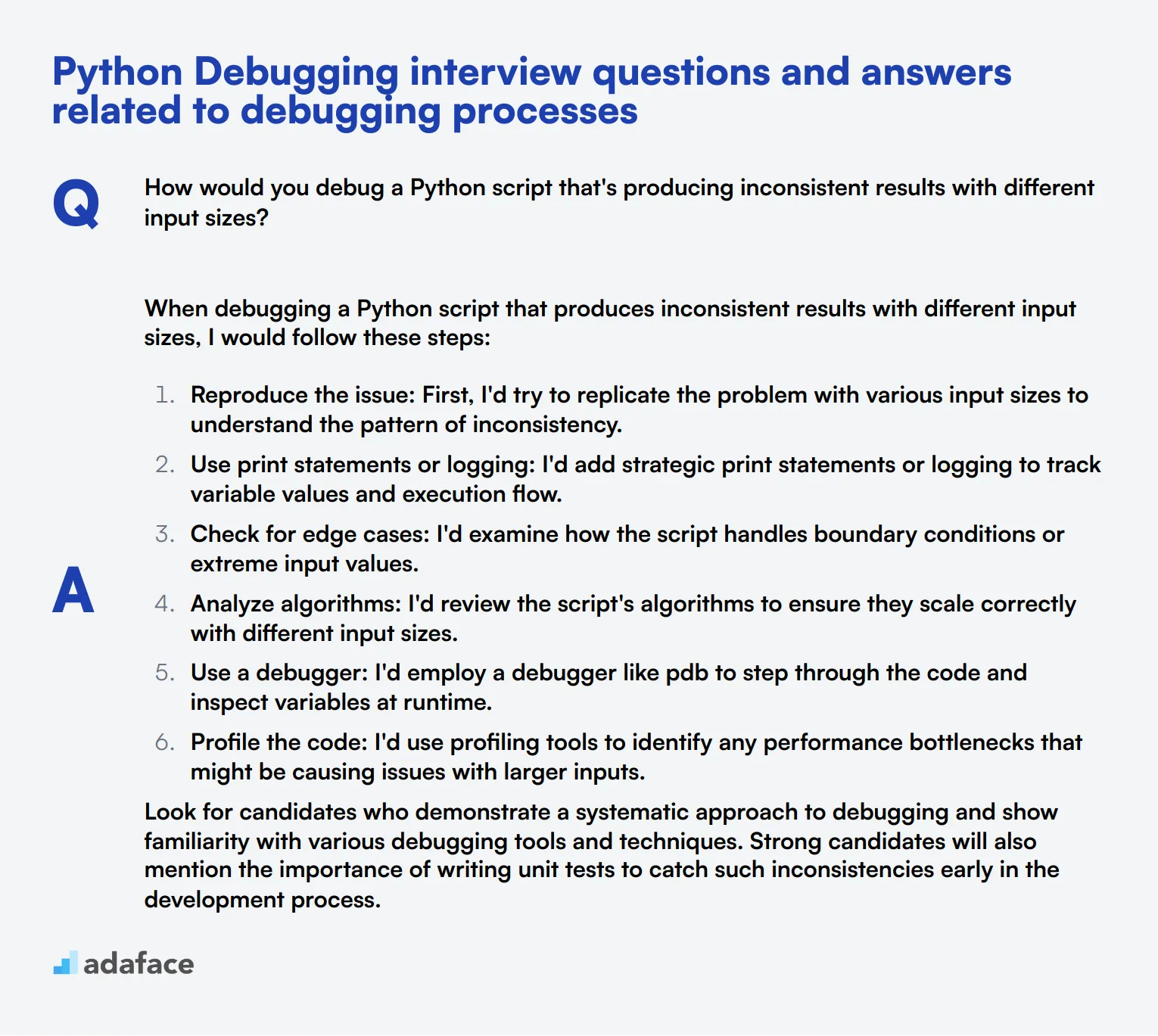
To gauge a candidate's proficiency in Python debugging, consider using these 7 interview questions. These questions are designed to assess a candidate's problem-solving skills and their ability to handle real-world debugging scenarios. By incorporating these questions into your interview process, you can gain valuable insights into how potential hires approach and resolve coding issues.
1. How would you debug a Python script that's producing inconsistent results with different input sizes?
When debugging a Python script that produces inconsistent results with different input sizes, I would follow these steps:
- Reproduce the issue: First, I'd try to replicate the problem with various input sizes to understand the pattern of inconsistency.
- Use print statements or logging: I'd add strategic print statements or logging to track variable values and execution flow.
- Check for edge cases: I'd examine how the script handles boundary conditions or extreme input values.
- Analyze algorithms: I'd review the script's algorithms to ensure they scale correctly with different input sizes.
- Use a debugger: I'd employ a debugger like pdb to step through the code and inspect variables at runtime.
- Profile the code: I'd use profiling tools to identify any performance bottlenecks that might be causing issues with larger inputs.
Look for candidates who demonstrate a systematic approach to debugging and show familiarity with various debugging tools and techniques. Strong candidates will also mention the importance of writing unit tests to catch such inconsistencies early in the development process.
2. Explain how you would debug a Python application where certain API calls are failing intermittently.
To debug intermittently failing API calls in a Python application, I would take the following approach:
- Implement robust logging: Add detailed logging for all API calls, including request parameters, headers, and response data.
- Use timeouts: Ensure all API calls have appropriate timeouts to handle unresponsive endpoints.
- Implement retry logic: Add a retry mechanism with exponential backoff for failed API calls.
- Monitor network conditions: Check for any network issues or limitations that might be causing intermittent failures.
- Analyze error patterns: Look for patterns in the failures, such as specific times of day or types of requests.
- Mock API responses: Use mocking to simulate various API responses and test the application's behavior.
- Use API monitoring tools: Implement tools to continuously monitor API performance and availability.
A strong candidate should emphasize the importance of thorough logging and monitoring in debugging intermittent issues. They should also discuss strategies for making the application more resilient to API failures, such as implementing circuit breakers or fallback mechanisms.
3. How would you approach debugging a Python script that's causing unexpected high CPU usage?
When debugging a Python script with unexpectedly high CPU usage, I would follow these steps:
- Profile the code: Use profiling tools like cProfile or line_profiler to identify which parts of the code are consuming the most CPU time.
- Analyze algorithms: Review the script's algorithms and data structures to identify any inefficient implementations.
- Check for infinite loops: Look for potential infinite loops or recursion that might be causing excessive CPU usage.
- Monitor system resources: Use system monitoring tools to observe CPU, memory, and I/O usage during script execution.
- Optimize database queries: If the script interacts with a database, ensure that queries are optimized and indexes are properly used.
- Review third-party libraries: Check if any third-party libraries are contributing to the high CPU usage and consider alternatives if necessary.
- Use multiprocessing: Consider using Python's multiprocessing module to distribute CPU-intensive tasks across multiple cores.
Look for candidates who demonstrate a methodical approach to performance optimization and show familiarity with profiling tools. Strong candidates might also mention the importance of establishing performance baselines and setting up continuous performance monitoring for Python applications.
4. How would you debug a Python application where a specific function is occasionally returning None unexpectedly?
To debug a Python application where a function is occasionally returning None unexpectedly, I would take the following steps:
- Add assertions: Place assertions at key points in the function to catch unexpected None values early.
- Implement extensive logging: Add detailed logging statements to track the function's input, intermediate steps, and output.
- Use exception handling: Wrap critical parts of the function in try-except blocks to catch and log any exceptions that might lead to None returns.
- Analyze input data: Examine the input data for patterns or edge cases that might be causing the unexpected None returns.
- Use debugger breakpoints: Set conditional breakpoints in the debugger to pause execution when None is about to be returned.
- Implement unit tests: Create comprehensive unit tests to cover various scenarios, including edge cases.
- Use type hinting: Implement type hinting to catch type-related issues that might lead to None returns.
A strong candidate should emphasize the importance of reproducing the issue consistently and using a combination of logging, debugging, and testing techniques. They should also discuss strategies for making the function more robust, such as implementing default return values or using the Optional type hint.
5. How would you debug a Python script that works fine in development but fails in production due to environment differences?
When debugging a Python script that works in development but fails in production due to environment differences, I would follow these steps:
- Compare environments: Carefully compare the development and production environments, including Python versions, installed packages, and system configurations.
- Use virtual environments: Ensure that both development and production use isolated virtual environments with identical package versions.
- Check environment variables: Verify that all necessary environment variables are set correctly in the production environment.
- Review file paths: Ensure that file paths used in the script are correct and accessible in the production environment.
- Implement logging: Add comprehensive logging to track the script's execution flow and any environment-specific information.
- Use feature flags: Implement feature flags to easily enable or disable certain functionalities based on the environment.
- Simulate production: Try to replicate the production environment locally or in a staging area for easier debugging.
Look for candidates who emphasize the importance of maintaining consistency between development and production environments. Strong candidates might also discuss strategies like containerization (e.g., Docker) to ensure environment parity and the use of configuration management tools to handle environment-specific settings.
6. How would you approach debugging a Python application that's experiencing race conditions in a multi-threaded environment?
To debug a Python application experiencing race conditions in a multi-threaded environment, I would take the following approach:
- Use thread-safe logging: Implement thread-safe logging to capture the sequence of events without interfering with the application's behavior.
- Employ thread sanitizers: Use tools like ThreadSanitizer to detect race conditions automatically.
- Implement locks and synchronization: Review the use of locks, semaphores, and other synchronization primitives to ensure proper thread synchronization.
- Use atomic operations: Replace non-atomic operations with their atomic counterparts where possible.
- Simplify the code: Temporarily simplify the multi-threaded code to isolate the race condition.
- Increase thread sleep times: Add strategic sleep() calls to exaggerate the race condition and make it more reproducible.
- Use concurrent.futures: Consider using higher-level concurrency abstractions like concurrent.futures to simplify thread management.
A strong candidate should demonstrate a deep understanding of concurrency issues and familiarity with Python's threading module. They should also discuss the challenges of reproducing and debugging race conditions, and mention techniques like code reviews and static analysis tools to prevent such issues in the first place.
7. How would you debug a Python script that's failing silently without raising exceptions?
When debugging a Python script that's failing silently without raising exceptions, I would follow these steps:
- Enable verbose logging: Implement detailed logging throughout the script to track its execution flow and identify where it might be failing.
- Use assertions: Add strategic assertions to catch unexpected conditions that might be causing silent failures.
- Implement error handling: Add try-except blocks with broad exception catching to identify any suppressed exceptions.
- Check return values: Verify that all function return values are being properly checked and handled.
- Use debugger: Step through the code using a debugger to identify where the execution path deviates from the expected behavior.
- Monitor system resources: Check for resource exhaustion (e.g., memory leaks, file descriptor limits) that might cause silent failures.
- Implement timeout mechanisms: Add timeouts to long-running operations to prevent indefinite hanging.
Look for candidates who emphasize the importance of defensive programming and thorough error handling. Strong candidates might also discuss the use of static analysis tools to identify potential sources of silent failures, and the importance of writing comprehensive unit tests to catch such issues early in the development process.
9 situational Python Debugging interview questions with answers for hiring top developers
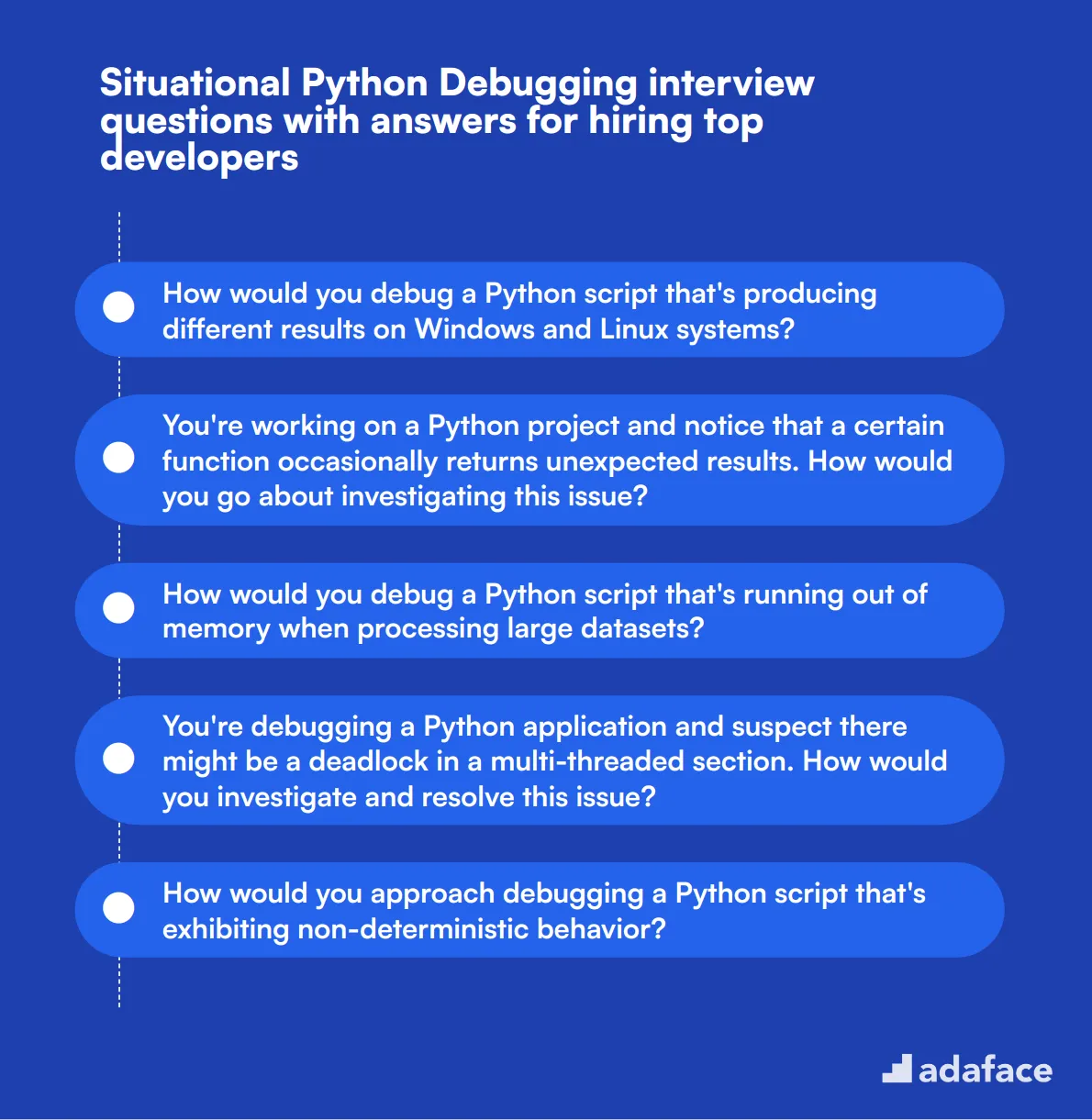
Ready to dive into the nitty-gritty of Python debugging? These 9 situational questions will help you assess a candidate's real-world problem-solving skills. Use them to uncover how potential hires approach tricky scenarios and debug effectively in Python. Remember, it's not just about the answer, but the thought process behind it!
1. How would you debug a Python script that's producing different results on Windows and Linux systems?
A strong candidate should approach this problem systematically. They might suggest the following steps:
- Check for platform-specific code: Look for any OS-dependent functions or libraries being used.
- Verify file path handling: Ensure proper use of os.path for cross-platform compatibility.
- Check for environment variables: Confirm if any system-specific environment variables are affecting the script.
- Examine line endings: Verify if different line endings (CRLF vs LF) are causing issues.
- Compare Python versions: Ensure both systems are running the same Python version.
Look for candidates who demonstrate a methodical approach and understand the nuances of cross-platform Python development. They should also mention the importance of setting up a consistent development environment across different platforms.
2. You're working on a Python project and notice that a certain function occasionally returns unexpected results. How would you go about investigating this issue?
An experienced Python developer would likely suggest a multi-step approach to tackle this problem:
- Add logging: Implement detailed logging to track function inputs, outputs, and intermediate steps.
- Use assertions: Place strategic assertions to catch unexpected conditions.
- Implement unit tests: Create comprehensive unit tests to cover various scenarios.
- Employ debugging tools: Utilize tools like pdb or an IDE debugger to step through the function.
- Check for race conditions: If it's a multi-threaded application, investigate potential race conditions.
- Review input data: Examine if certain input patterns are causing the unexpected results.
A strong candidate should emphasize the importance of reproducibility and isolating the problem. They might also suggest creating a minimal reproducible example to better understand and potentially share the issue.
3. How would you debug a Python script that's running out of memory when processing large datasets?
A proficient Python developer should propose a structured approach to this memory issue:
- Profile memory usage: Use tools like memory_profiler to identify memory-intensive parts of the code.
- Implement lazy evaluation: Suggest using generators or iterators to process data in chunks.
- Optimize data structures: Recommend using more memory-efficient data structures where applicable.
- Use external storage: For very large datasets, suggest using databases or disk-based solutions.
- Implement garbage collection: Ensure proper garbage collection and consider manual memory management where necessary.
- Check for memory leaks: Look for potential memory leaks, especially in long-running processes.
Look for candidates who not only provide technical solutions but also demonstrate an understanding of Python's memory management and the trade-offs between memory usage and processing speed. They should also mention the importance of benchmarking before and after optimizations.
4. You're debugging a Python application and suspect there might be a deadlock in a multi-threaded section. How would you investigate and resolve this issue?
An adept Python developer should outline a systematic approach to diagnosing and resolving deadlocks:
- Use threading.enumerate(): To list all active threads and their states.
- Implement logging: Add detailed logging to track thread activities and lock acquisitions.
- Utilize debugging tools: Suggest using tools like faulthandler to generate tracebacks for deadlocked threads.
- Analyze lock order: Check for potential circular dependencies in lock acquisition.
- Consider using higher-level synchronization primitives: Recommend using threading.Condition or queue.Queue instead of low-level locks when appropriate.
- Implement timeouts: Suggest adding timeouts to lock acquisitions to prevent indefinite waiting.
A strong candidate should also mention the importance of reproducing the issue consistently and potentially simplifying the code to isolate the problem. They might also discuss strategies for designing thread-safe code to prevent deadlocks in the first place.
5. How would you approach debugging a Python script that's exhibiting non-deterministic behavior?
A skilled Python developer should propose a methodical approach to tackle non-deterministic behavior:
- Identify sources of randomness: Check for use of random number generators, time-dependent functions, or external inputs.
- Control the environment: Suggest fixing random seeds, mocking time-dependent functions, and controlling external inputs.
- Increase logging: Implement extensive logging to track program flow and variable states.
- Use debugging tools: Recommend tools like pdb or remote debugging for step-by-step execution.
- Implement assertions: Add strategic assertions to catch unexpected states.
- Increase test coverage: Suggest writing more unit tests and running them multiple times.
Look for candidates who emphasize the importance of reproducibility in debugging. They should also mention techniques like data scientist approaches to analyzing logs for patterns, and potentially using statistical methods to characterize the non-deterministic behavior.
6. You're working on a Python project where certain API calls are failing intermittently. How would you go about debugging this issue?
An experienced Python developer should suggest a comprehensive approach to debug intermittent API failures:
- Implement robust logging: Log all API calls, responses, and relevant metadata (timestamps, request parameters, etc.).
- Use request/response mocking: Suggest tools like responses or unittest.mock to simulate various API behaviors.
- Implement retry mechanisms: Recommend using libraries like tenacity for intelligent retrying of failed requests.
- Monitor network conditions: Suggest tools to monitor network stability and latency.
- Analyze patterns: Look for correlations between failures and factors like time of day, server load, or specific request parameters.
- Implement circuit breakers: Suggest using the circuit breaker pattern to handle temporary service unavailability.
A strong candidate should also mention the importance of error handling and graceful degradation in the face of API failures. They might discuss strategies for designing resilient systems that can handle intermittent failures without compromising overall functionality.
7. How would you debug a Python script that's producing floating-point calculation errors?
A proficient Python developer should outline a structured approach to debugging floating-point calculation errors:
- Understand floating-point representation: Explain the limitations of binary floating-point representation.
- Use the decimal module: Suggest using the decimal module for precise decimal representations when needed.
- Implement rounding: Recommend strategic rounding to avoid accumulation of small errors.
- Compare with exact fractions: Suggest using the fractions module to compare results with exact calculations.
- Analyze the algorithm: Review the calculation algorithm for potential sources of error accumulation.
- Use appropriate tolerances: Implement appropriate tolerance levels when comparing floating-point numbers.
Look for candidates who demonstrate a deep understanding of floating-point arithmetic in computers. They should also mention the importance of writing unit tests that account for floating-point imprecision and potentially suggest alternative algorithms that might be less susceptible to these issues.
8. You're debugging a Python application where certain operations are much slower than expected. How would you investigate and optimize this?
An adept Python developer should propose a systematic approach to performance debugging:
- Profile the code: Use tools like cProfile or line_profiler to identify bottlenecks.
- Use time complexity analysis: Analyze algorithms for potential inefficiencies.
- Optimize data structures: Suggest more efficient data structures where applicable.
- Implement caching: Recommend caching strategies for expensive computations.
- Consider concurrency: Suggest using multiprocessing or asyncio for parallelizable tasks.
- Use appropriate libraries: Recommend using optimized libraries like NumPy for numerical operations.
A strong candidate should emphasize the importance of benchmarking before and after optimizations. They might also discuss the trade-offs between readability, maintainability, and performance, and suggest profiling in production-like environments for realistic results.
9. How would you debug a Python script that's failing only when run as a scheduled task?
A skilled Python developer should outline a methodical approach to this scenario:
- Check environment differences: Compare the environment variables and Python path between interactive and scheduled runs.
- Verify file paths: Ensure all file paths are absolute in the script.
- Check permissions: Verify that the scheduled task has necessary permissions to access required resources.
- Implement logging: Add comprehensive logging to track script execution and potential error points.
- Simulate scheduled environment: Try to replicate the scheduled environment in an interactive session.
- Review task scheduler logs: Check the task scheduler's logs for any relevant information.
Look for candidates who emphasize the importance of replicating the issue consistently. They should also mention strategies for designing scripts to be more robust when run in different environments, such as using configuration files or environment variables for flexible setups.
Which Python Debugging skills should you evaluate during the interview phase?
While it's impossible to assess every aspect of a candidate's Python debugging skills in a single interview, focusing on core competencies can provide valuable insights. The following skills are particularly important to evaluate during the interview phase for Python debugging roles.
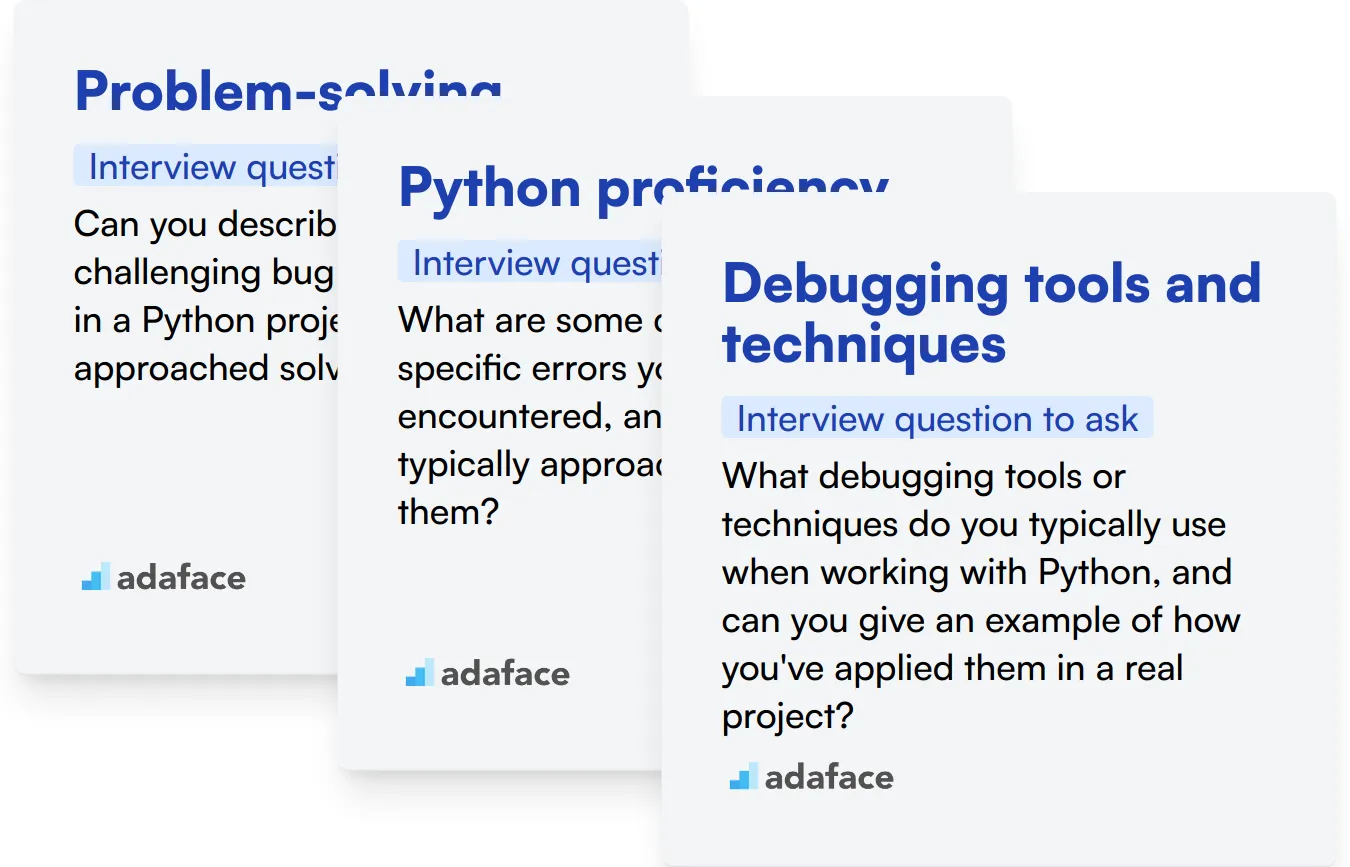
Problem-solving
Problem-solving is at the heart of debugging. It involves analyzing complex issues, breaking them down into manageable parts, and developing effective solutions.
To assess problem-solving skills, consider using an assessment test with relevant multiple-choice questions. These can help filter candidates based on their analytical abilities.
You can also ask targeted interview questions to gauge problem-solving skills in a Python debugging context. Here's an example:
Can you describe a particularly challenging bug you encountered in a Python project and how you approached solving it?
Look for candidates who can articulate their thought process, describe the steps they took to isolate the issue, and explain how they arrived at a solution. Pay attention to their ability to break down complex problems and use logical reasoning.
Python proficiency
A strong foundation in Python is essential for effective debugging. This includes understanding Python syntax, data structures, and common programming patterns.
To evaluate Python proficiency, you might consider using a Python online test that covers various aspects of the language.
In addition to assessment tests, you can ask specific questions to gauge Python knowledge. Here's an example:
What are some common Python-specific errors you've encountered, and how do you typically approach debugging them?
Look for answers that demonstrate familiarity with Python-specific concepts like indentation errors, type errors, or module import issues. Candidates should be able to explain debugging techniques tailored to Python's characteristics.
Debugging tools and techniques
Familiarity with debugging tools and techniques is crucial for efficient problem-solving in Python. This includes using debuggers, logging, and other diagnostic tools.
Consider incorporating questions about debugging tools in your assessment test to evaluate candidates' knowledge in this area.
You can also ask targeted questions during the interview to assess familiarity with debugging tools and techniques. Here's an example:
What debugging tools or techniques do you typically use when working with Python, and can you give an example of how you've applied them in a real project?
Look for answers that mention specific tools like pdb, logging modules, or IDE debuggers. Candidates should be able to explain how they've used these tools to identify and resolve issues in their Python projects.
3 Tips for Effectively Using Python Debugging Interview Questions
Before you start putting what you've learned to use, here are some tips to enhance your interview process and ensure you select the best candidates.
1. Incorporate Skills Tests Before Interviews
Using skill tests prior to interviews can help you accurately gauge a candidate's technical abilities. Implementing tests such as the Python Online Test or the Python Pandas Online Test provides insights into their proficiency.
These tests can reveal strengths and weaknesses, ensuring that you focus on the most relevant skills during the interview. By identifying areas for deeper questioning, you can streamline your interview process and concentrate on the candidate's potential fit for the role.
2. Craft Relevant Interview Questions
Time is limited during interviews, so it's essential to choose the right questions that assess core competencies effectively. Focusing on a select few, impactful questions will maximize your ability to evaluate candidates.
Moreover, consider integrating related interview questions from different skill areas. For example, asking about communication skills or teamwork dynamics can complement your Python Debugging questions, enhancing your overall assessment.
3. Utilize Follow-Up Questions
Simply asking interview questions may not provide the full picture of a candidate's abilities. Follow-up questions are necessary to probe deeper into their responses and assess their actual understanding of the concepts.
For instance, if a candidate explains their debugging process in Python, you might ask, 'Can you give an example of a debugging challenge you've faced and how you resolved it?' This approach helps illuminate their problem-solving skills and offers valuable insights into their practical experience.
Utilize Python Debugging interview questions and skills tests to hire talented developers
When hiring for Python debugging roles, it's important to accurately assess candidates' skills. The best way to achieve this is through targeted skills tests, such as our Python online test.
After utilizing these tests, you can effectively shortlist the best applicants for interviews. To get started, consider signing up on our assessment platform for a streamlined hiring process.
Python Online Test
Download Python Debugging interview questions template in multiple formats
Python Debugging Interview Questions FAQs
The post covers general, junior, intermediate, and advanced Python debugging questions, as well as questions about debugging techniques and processes.
The post provides tips for using the questions effectively and suggests combining them with skills tests to assess candidates comprehensively.
Yes, the post includes a section with situational Python debugging questions and answers for hiring top developers.
The post includes 20 Python debugging interview questions specifically tailored for junior developers.
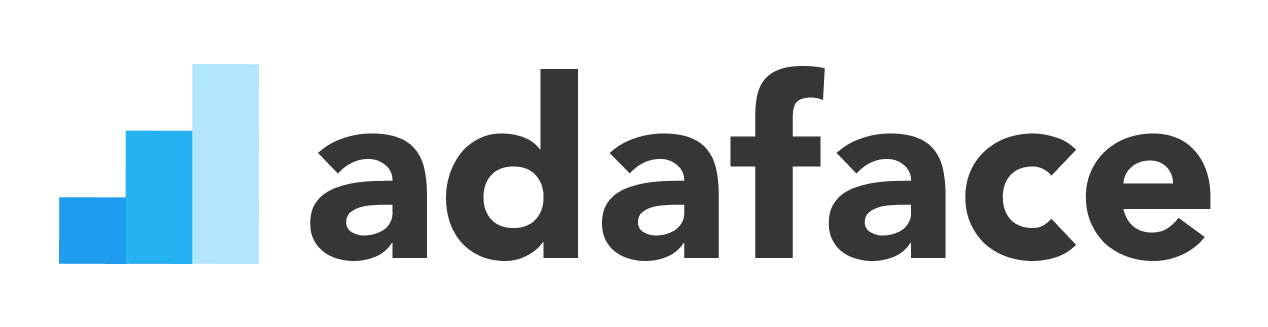
40 min skill tests.
No trick questions.
Accurate shortlisting.
We make it easy for you to find the best candidates in your pipeline with a 40 min skills test.
Try for freeRelated posts
Free resources
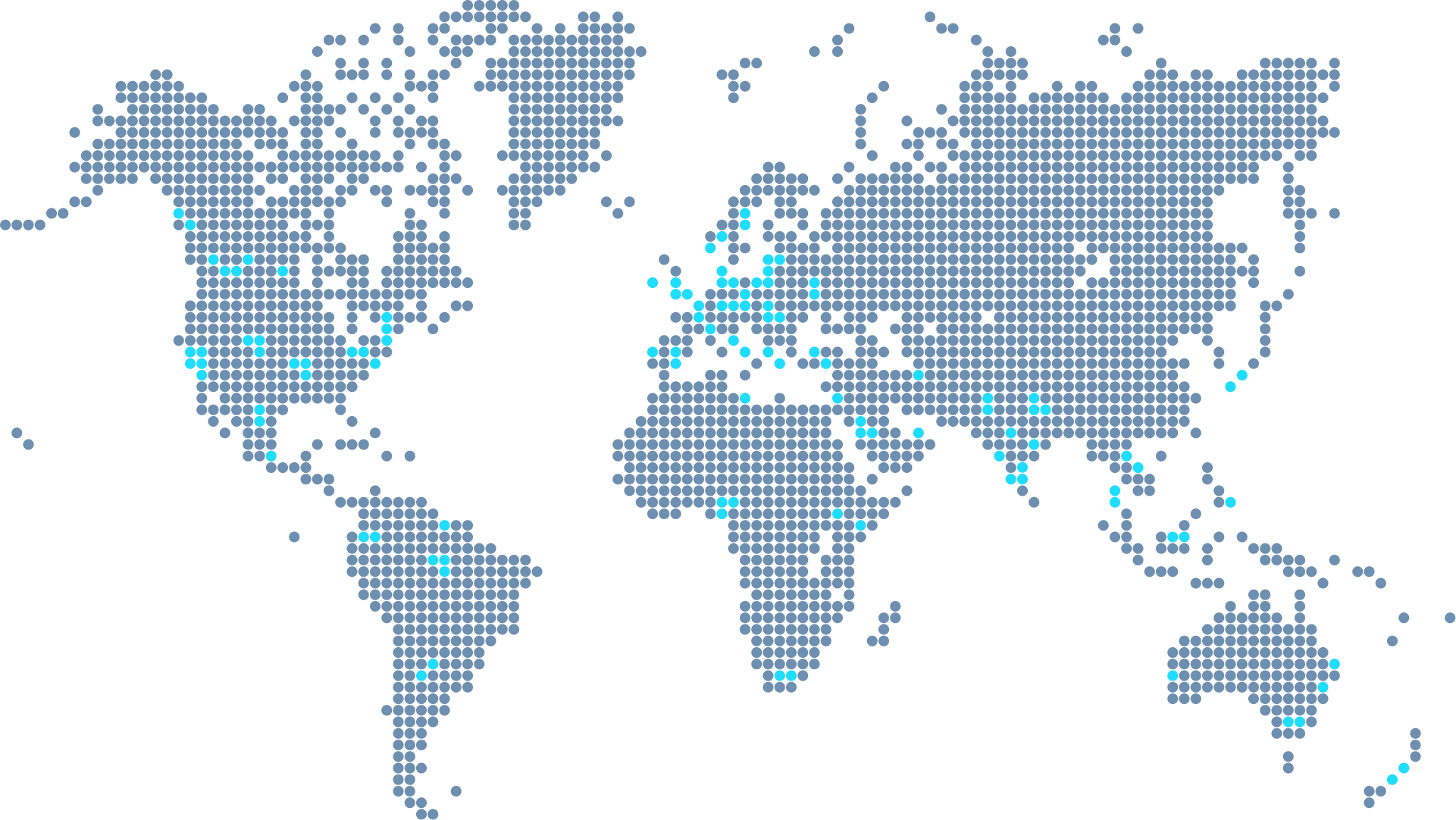
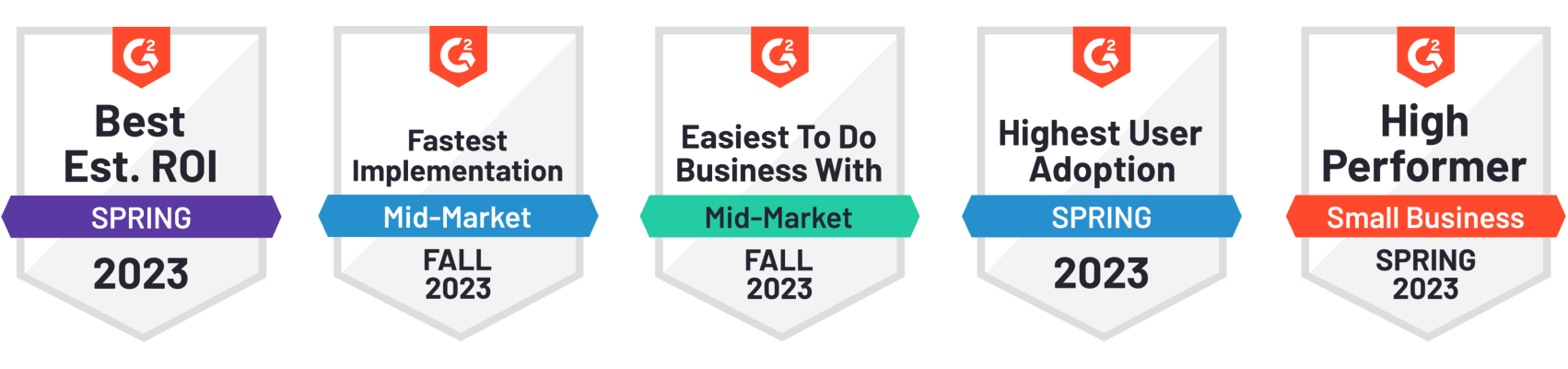