Hiring the right NodeJS developer can be a make-or-break decision for your project's success. As an interviewer, having a well-curated list of NodeJS interview questions helps you assess candidates' skills effectively and make informed hiring decisions.
This blog post provides a comprehensive set of NodeJS interview questions, ranging from basic to advanced, tailored for different experience levels. We cover fundamental concepts, asynchronous programming, event-driven architecture, and include situational questions to evaluate problem-solving skills.
By using these questions, you can gain deeper insights into candidates' NodeJS expertise and identify top talent. Consider pairing these interview questions with a NodeJS skills assessment for a more thorough evaluation of potential hires.
Table of contents
16 basic NodeJS interview questions and answers to assess candidates
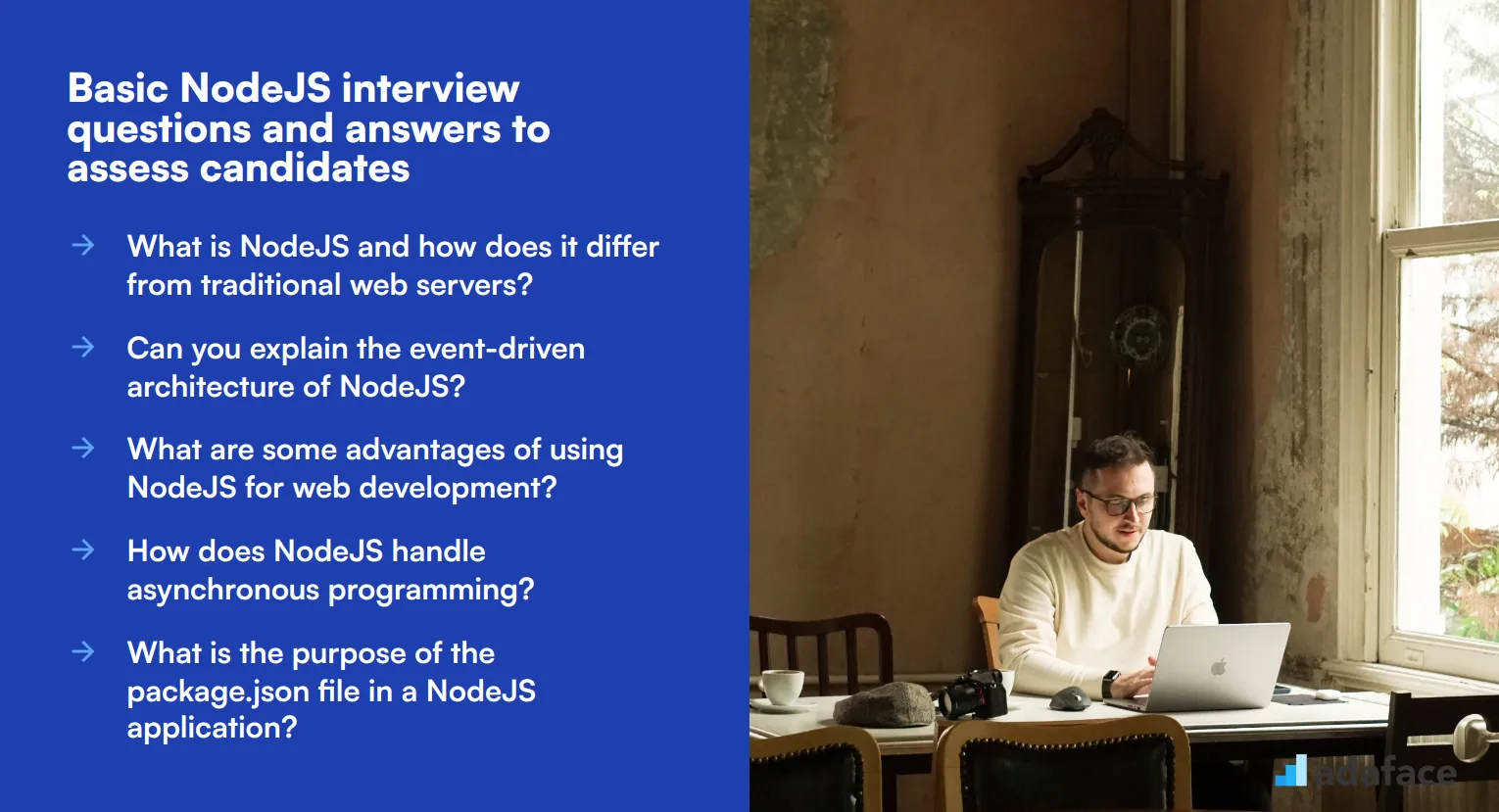
To evaluate if your candidates possess a solid understanding of NodeJS fundamentals, consider using this list of basic interview questions. These inquiries will help you assess their technical knowledge and practical skills essential for development roles. For more resources, check out our NodeJS interview guide.
- What is NodeJS and how does it differ from traditional web servers?
- Can you explain the event-driven architecture of NodeJS?
- What are some advantages of using NodeJS for web development?
- How does NodeJS handle asynchronous programming?
- What is the purpose of the package.json file in a NodeJS application?
- Can you describe what middleware is in the context of Express.js?
- What is npm and how does it relate to NodeJS?
- How do you manage dependencies in a NodeJS project?
- What is the role of the event loop in NodeJS?
- Can you explain how to create a simple HTTP server in NodeJS?
- What are streams in NodeJS and why are they useful?
- How do you handle errors in a NodeJS application?
- Can you differentiate between process.nextTick() and setImmediate()?
- What are environment variables, and how do you use them in NodeJS?
- How can you improve the performance of a NodeJS application?
- What are some common security concerns when developing with NodeJS?
8 NodeJS interview questions and answers to evaluate junior developers
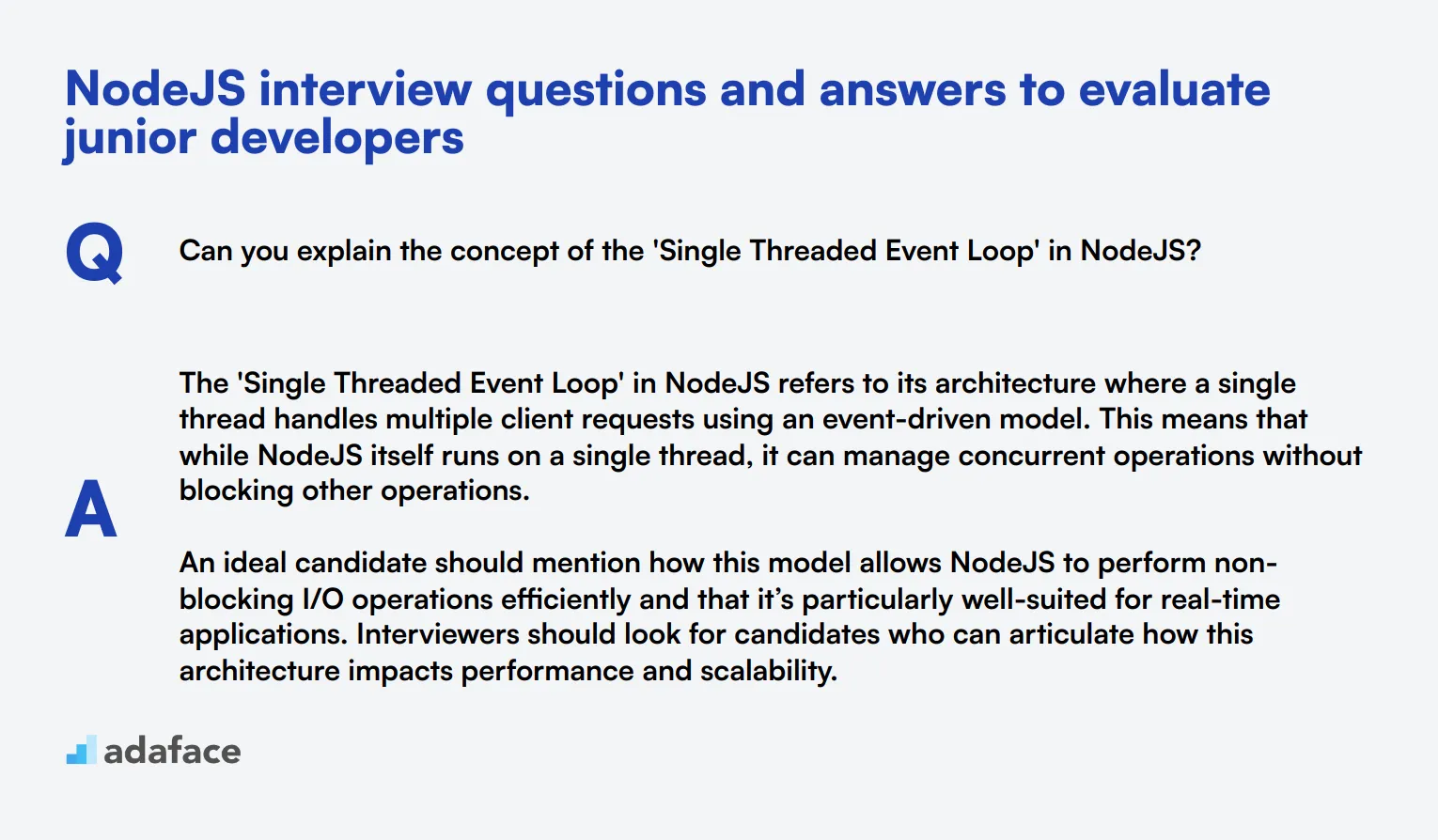
To determine whether your applicants have the right skills and understanding of NodeJS, ask them some of these key interview questions tailored specifically for junior developers.
1. Can you explain the concept of the 'Single Threaded Event Loop' in NodeJS?
The 'Single Threaded Event Loop' in NodeJS refers to its architecture where a single thread handles multiple client requests using an event-driven model. This means that while NodeJS itself runs on a single thread, it can manage concurrent operations without blocking other operations.
An ideal candidate should mention how this model allows NodeJS to perform non-blocking I/O operations efficiently and that it’s particularly well-suited for real-time applications. Interviewers should look for candidates who can articulate how this architecture impacts performance and scalability.
2. How do you handle file operations in NodeJS?
File operations in NodeJS, such as reading, writing, and deleting files, are handled using the built-in 'fs' (file system) module. This module provides both synchronous and asynchronous methods for file operations, allowing developers to choose based on their needs for performance and blocking behavior.
Candidates should demonstrate an understanding of the difference between synchronous and asynchronous file operations and explain the scenarios where each is appropriate. Look for responses that highlight awareness of potential performance impacts and error handling strategies.
3. What is the purpose of the 'require' function in NodeJS?
The 'require' function in NodeJS is used to import modules, JSON, and local files into a NodeJS application. This function reads a JavaScript file, executes it, and then returns the exports object to be used in the application.
An ideal candidate should explain how 'require' helps in modularizing code, making it easier to manage and reuse. They should also touch upon how it enables dependency management within a NodeJS project. Interviewers should look for candidates who understand the importance of modular design in software development.
4. Can you explain what a callback function is and how it's used in NodeJS?
A callback function is a function that is passed as an argument to another function and is executed after the initial function has completed. In NodeJS, callbacks are primarily used for handling asynchronous operations, such as reading files or making HTTP requests.
Candidates should be able to discuss how callbacks help in managing asynchronous code execution and mention common issues like callback hell. Look for responses that include strategies to mitigate callback hell, such as using promises or async/await syntax.
5. What are Promises in NodeJS and why are they useful?
Promises in NodeJS are objects that represent the eventual completion (or failure) of an asynchronous operation, and their resulting value. They provide a cleaner, more manageable way to handle asynchronous operations compared to traditional callback functions.
An ideal answer would touch on how promises can be chained to handle multiple asynchronous operations in a more readable manner. Candidates should also mention error handling with promises and how they simplify the code by avoiding callback hell. Look for an understanding of promise states: pending, fulfilled, and rejected.
6. How do you manage different environments in a NodeJS application?
Managing different environments in a NodeJS application involves setting environment-specific configurations, typically using environment variables. These variables can be stored in a .env file and loaded using libraries like dotenv. This allows for different settings for development, testing, and production environments.
Candidates should mention best practices like not hardcoding sensitive information and using environment variables to manage configurations. Look for an understanding of how to securely manage and switch between different environments during development and deployment.
7. What is your approach to logging in a NodeJS application?
Logging in a NodeJS application typically involves capturing and storing various types of information, such as errors, warnings, and general activity logs. This can be done using built-in modules like console or external libraries like Winston or Morgan.
A strong candidate should explain the importance of logging for debugging and monitoring application performance. They should also discuss best practices, such as log levels, structured logging, and log rotation. Look for an understanding of how to implement a scalable and maintainable logging strategy.
8. Can you explain what an event emitter is in NodeJS?
An event emitter in NodeJS is an object that facilitates the communication of various events between different parts of an application. It allows you to emit named events that can be listened for and responded to by event listeners. This pattern is central to NodeJS’s event-driven architecture.
Candidates should mention how event emitters are used to handle asynchronous events and provide examples of common use cases, such as handling user actions or system events. Look for an understanding of how event emitters contribute to the modularity and scalability of a NodeJS application.
12 intermediate NodeJS interview questions and answers to ask mid-tier developers
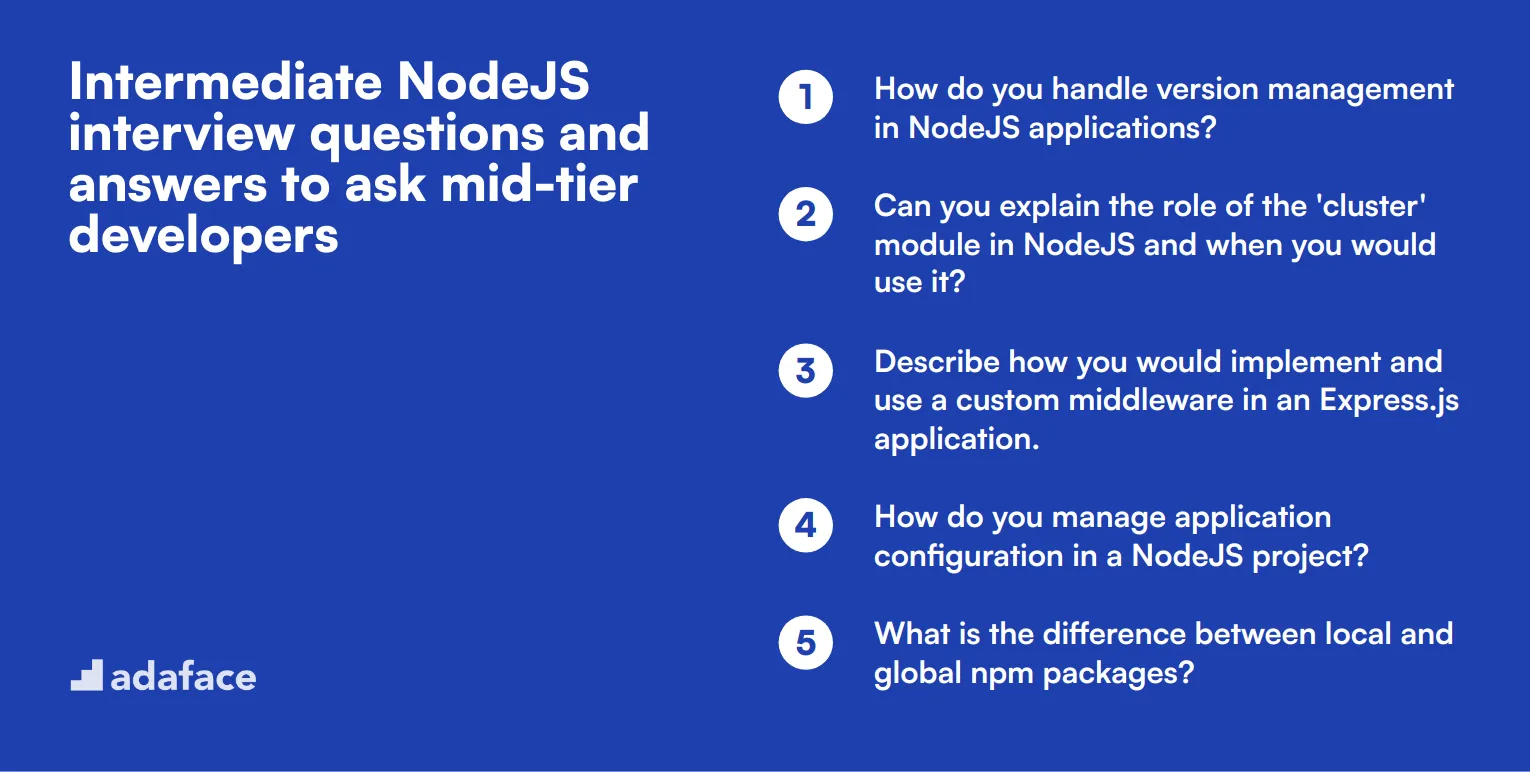
To assess whether your candidates possess the right skills for mid-level NodeJS development, use this list of intermediate questions. These questions are designed to dig deeper into the practical knowledge and problem-solving abilities that are crucial for effective NodeJS development. Explore more on how to effectively utilize this guide for your interview process.
- How do you handle version management in NodeJS applications?
- Can you explain the role of the 'cluster' module in NodeJS and when you would use it?
- Describe how you would implement and use a custom middleware in an Express.js application.
- How do you manage application configuration in a NodeJS project?
- What is the difference between local and global npm packages?
- Can you explain the concept of 'callback hell' and how to avoid it?
- Describe how you would use the 'fs' module to read and write files asynchronously.
- What are some best practices for structuring a large-scale NodeJS application?
- Can you explain how you would use WebSockets in a NodeJS application for real-time communication?
- How do you handle session management and authentication in a NodeJS application?
- What is your approach to testing NodeJS applications?
- Describe a scenario where you had to debug a NodeJS application and how you resolved the issue.
6 NodeJS interview questions and answers related to asynchronous programming
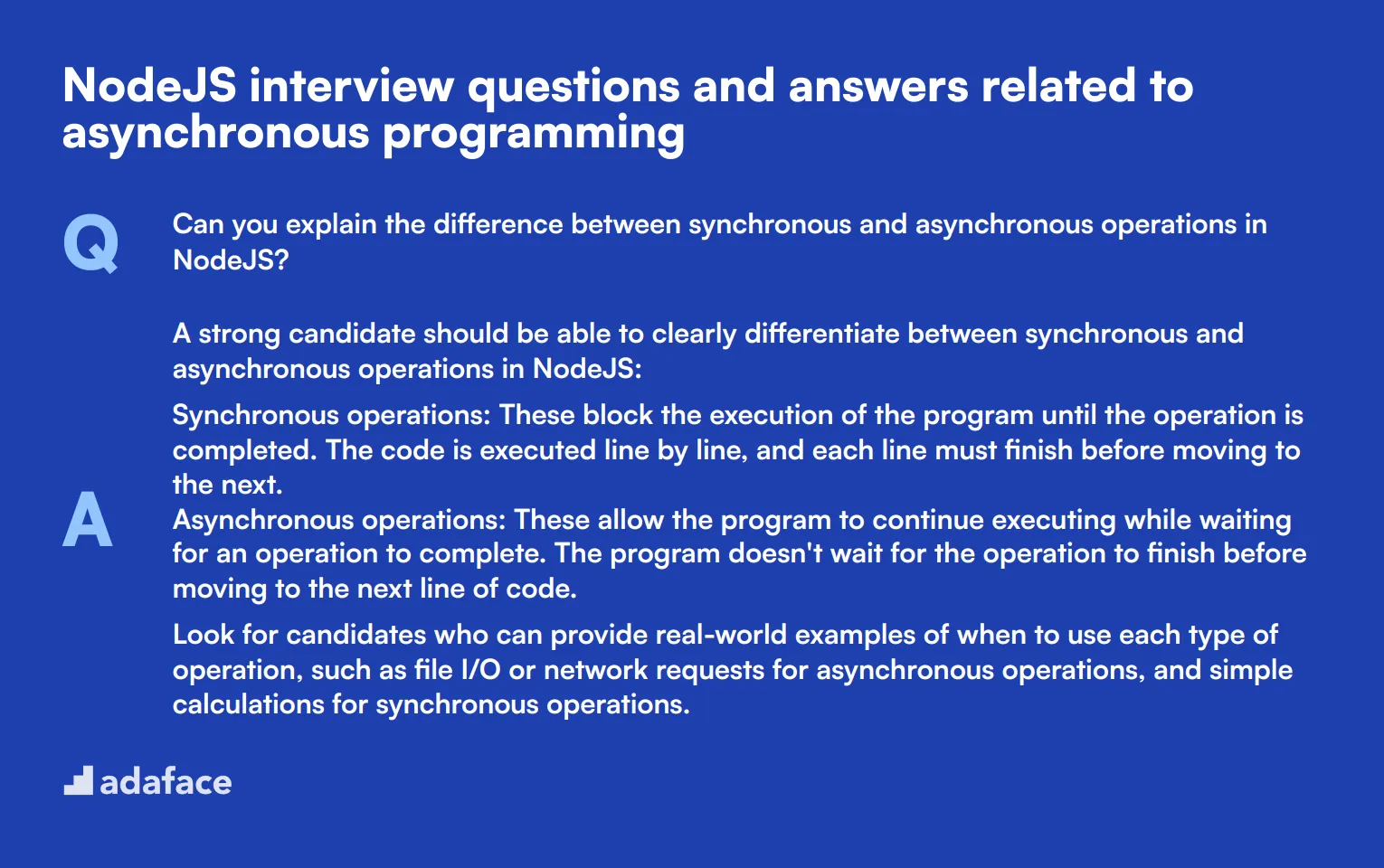
Ready to dive into the world of asynchronous programming in NodeJS? These six questions will help you gauge a candidate's understanding of this crucial concept. Use them to assess skills and uncover how well your potential hires can handle the non-blocking nature of NodeJS.
1. Can you explain the difference between synchronous and asynchronous operations in NodeJS?
A strong candidate should be able to clearly differentiate between synchronous and asynchronous operations in NodeJS:
- Synchronous operations: These block the execution of the program until the operation is completed. The code is executed line by line, and each line must finish before moving to the next.
- Asynchronous operations: These allow the program to continue executing while waiting for an operation to complete. The program doesn't wait for the operation to finish before moving to the next line of code.
Look for candidates who can provide real-world examples of when to use each type of operation, such as file I/O or network requests for asynchronous operations, and simple calculations for synchronous operations.
2. How does NodeJS handle concurrent requests without using threads?
A knowledgeable candidate should explain that NodeJS uses an event-driven, non-blocking I/O model to handle concurrent requests without relying on traditional threading. They should mention the following key points:
- NodeJS runs on a single thread, utilizing an event loop.
- Asynchronous operations are offloaded to the system kernel whenever possible.
- Callbacks and events are used to handle the results of these operations.
- This approach allows NodeJS to handle many concurrent connections efficiently without the overhead of thread management.
Look for candidates who can explain how this model contributes to NodeJS's scalability and performance, especially in I/O-heavy applications.
3. What are the advantages and potential pitfalls of using callbacks in NodeJS?
A well-informed candidate should be able to discuss both the benefits and drawbacks of using callbacks in NodeJS:
Advantages:
- Allows for non-blocking code execution
- Enables handling of asynchronous operations
- Provides a way to continue code execution after an operation completes
Potential pitfalls:
- Can lead to callback hell (nested callbacks)
- Makes error handling more complicated
- Can make code harder to read and maintain
Look for candidates who can suggest alternatives to callbacks, such as Promises or async/await, and explain when they might be more appropriate. A strong answer will demonstrate an understanding of asynchronous programming patterns and their impact on code quality.
4. How do Promises improve upon callbacks in handling asynchronous operations?
A competent candidate should explain that Promises provide a more structured way to handle asynchronous operations compared to callbacks. They should highlight the following improvements:
- Cleaner and more readable code structure
- Better error handling through .catch() method
- Ability to chain multiple asynchronous operations
- Avoidance of callback hell
- Built-in methods like Promise.all() for handling multiple asynchronous operations
Look for candidates who can provide examples of how Promises can be used in real-world scenarios, such as making API calls or reading files. They should also be able to explain the states of a Promise (pending, fulfilled, rejected) and how to create a new Promise.
5. Can you explain the concept of 'async/await' and how it relates to Promises?
A knowledgeable candidate should explain that async/await is a syntactic sugar built on top of Promises, making asynchronous code look and behave more like synchronous code. They should mention:
- 'async' keyword is used to define a function that returns a Promise
- 'await' keyword is used inside an async function to wait for a Promise to resolve
- It allows for writing asynchronous code in a more linear, readable manner
- Error handling can be done using traditional try/catch blocks
Look for candidates who can compare async/await with Promises and callbacks, explaining the benefits in terms of code readability and error handling. They should also be able to provide examples of when to use async/await, such as in complex asynchronous workflows or when working with multiple API calls.
6. How would you handle multiple asynchronous operations that depend on each other in NodeJS?
A strong candidate should be able to describe multiple approaches to handling dependent asynchronous operations:
- Callbacks: Nesting callbacks, but mentioning the risk of callback hell
- Promises: Using .then() chains or Promise.all()
- Async/await: Using async functions with multiple await statements
They should explain the pros and cons of each approach, such as:
- Callbacks: Simple but can lead to deeply nested code
- Promises: More structured but can still become complex with many operations
- Async/await: Most readable and maintainable, but requires newer JavaScript features
Look for candidates who can provide real-world examples and discuss error handling strategies for each approach. They should also be able to explain how to choose the best method based on the specific requirements of a project.
10 NodeJS interview questions about event-driven architecture
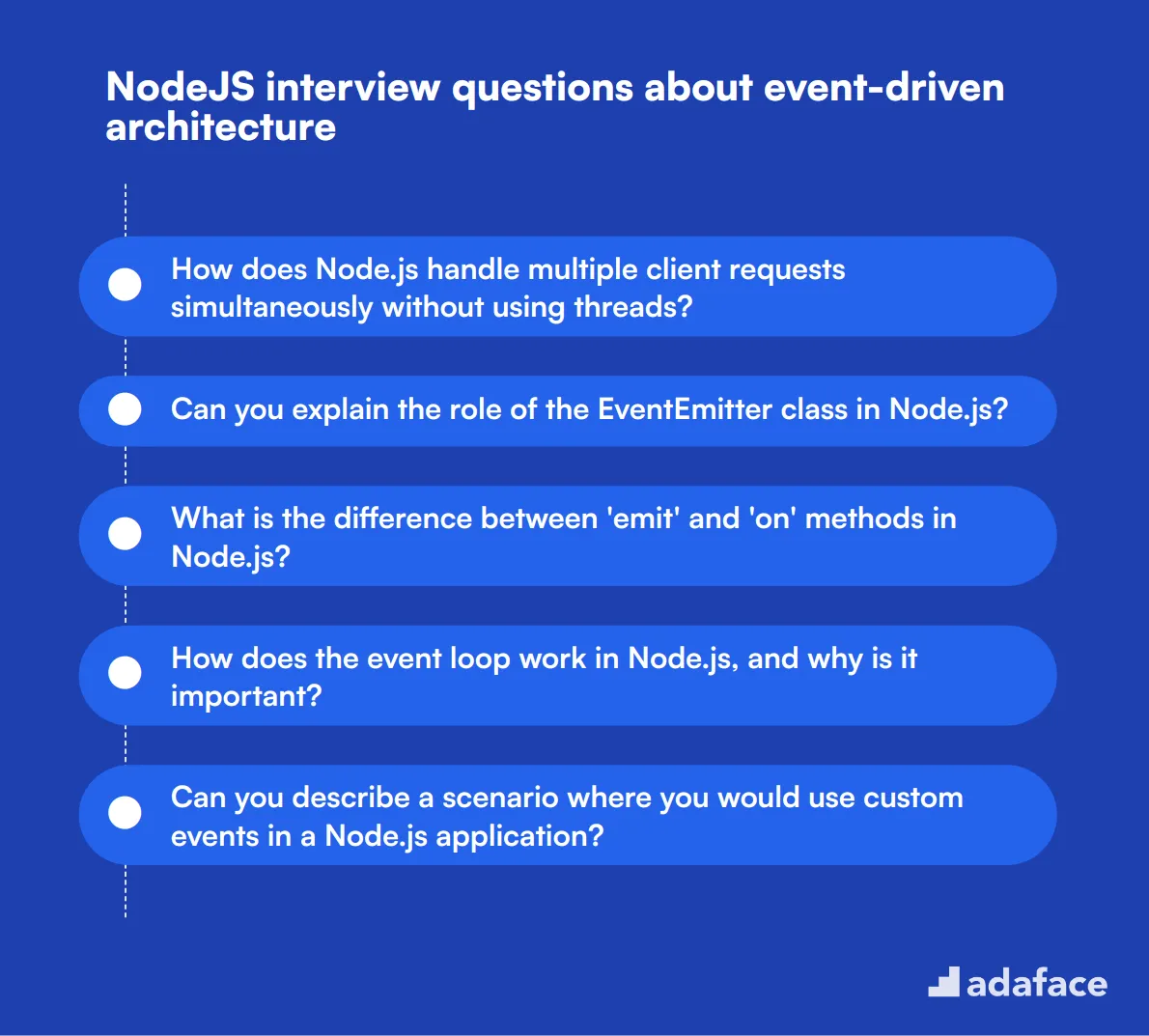
To assess a candidate's understanding of Node.js's event-driven architecture, use these 10 interview questions. These queries will help you gauge the applicant's grasp of core concepts and their ability to apply them in real-world scenarios.
- How does Node.js handle multiple client requests simultaneously without using threads?
- Can you explain the role of the EventEmitter class in Node.js?
- What is the difference between 'emit' and 'on' methods in Node.js?
- How does the event loop work in Node.js, and why is it important?
- Can you describe a scenario where you would use custom events in a Node.js application?
- What are the advantages of using an event-driven architecture in Node.js?
- How do you handle errors in an event-driven Node.js application?
- Can you explain the concept of 'non-blocking I/O' in the context of Node.js?
- How does Node.js achieve high performance with its single-threaded model?
- What is the purpose of the 'process' object in Node.js and how is it related to events?
7 situational NodeJS interview questions for hiring top developers
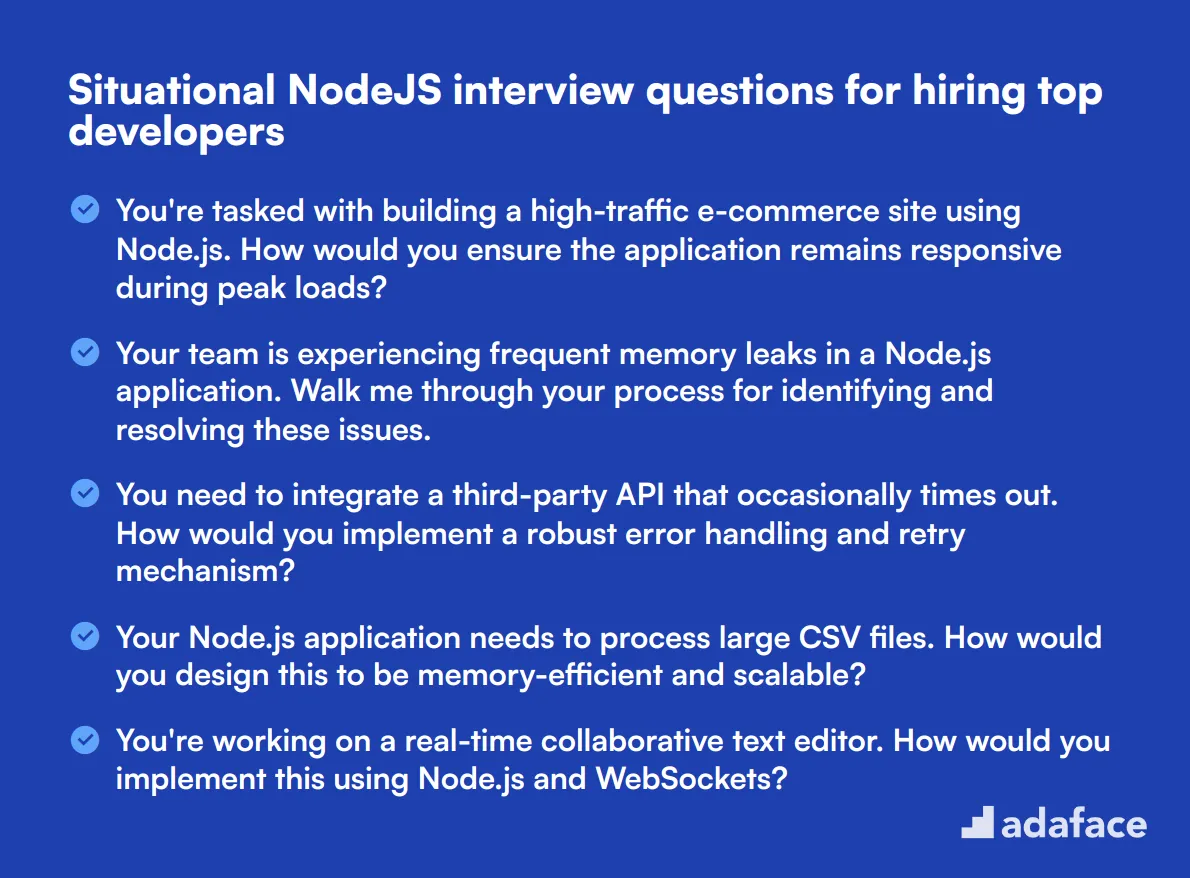
To assess a candidate's practical problem-solving skills and real-world experience with Node.js, consider using these situational interview questions. These scenarios will help you evaluate how potential hires approach complex issues and leverage Node.js features in actual development situations.
- You're tasked with building a high-traffic e-commerce site using Node.js. How would you ensure the application remains responsive during peak loads?
- Your team is experiencing frequent memory leaks in a Node.js application. Walk me through your process for identifying and resolving these issues.
- You need to integrate a third-party API that occasionally times out. How would you implement a robust error handling and retry mechanism?
- Your Node.js application needs to process large CSV files. How would you design this to be memory-efficient and scalable?
- You're working on a real-time collaborative text editor. How would you implement this using Node.js and WebSockets?
- Your team is transitioning from callbacks to Promises in a large Node.js codebase. What strategy would you use to manage this transition smoothly?
- You're tasked with optimizing database queries in a Node.js application. What steps would you take to improve performance?
Which NodeJS skills should you evaluate during the interview phase?
While it's impossible to assess every aspect of a candidate's NodeJS expertise in a single interview, focusing on core skills is crucial. The following key areas provide a solid foundation for evaluating NodeJS proficiency.
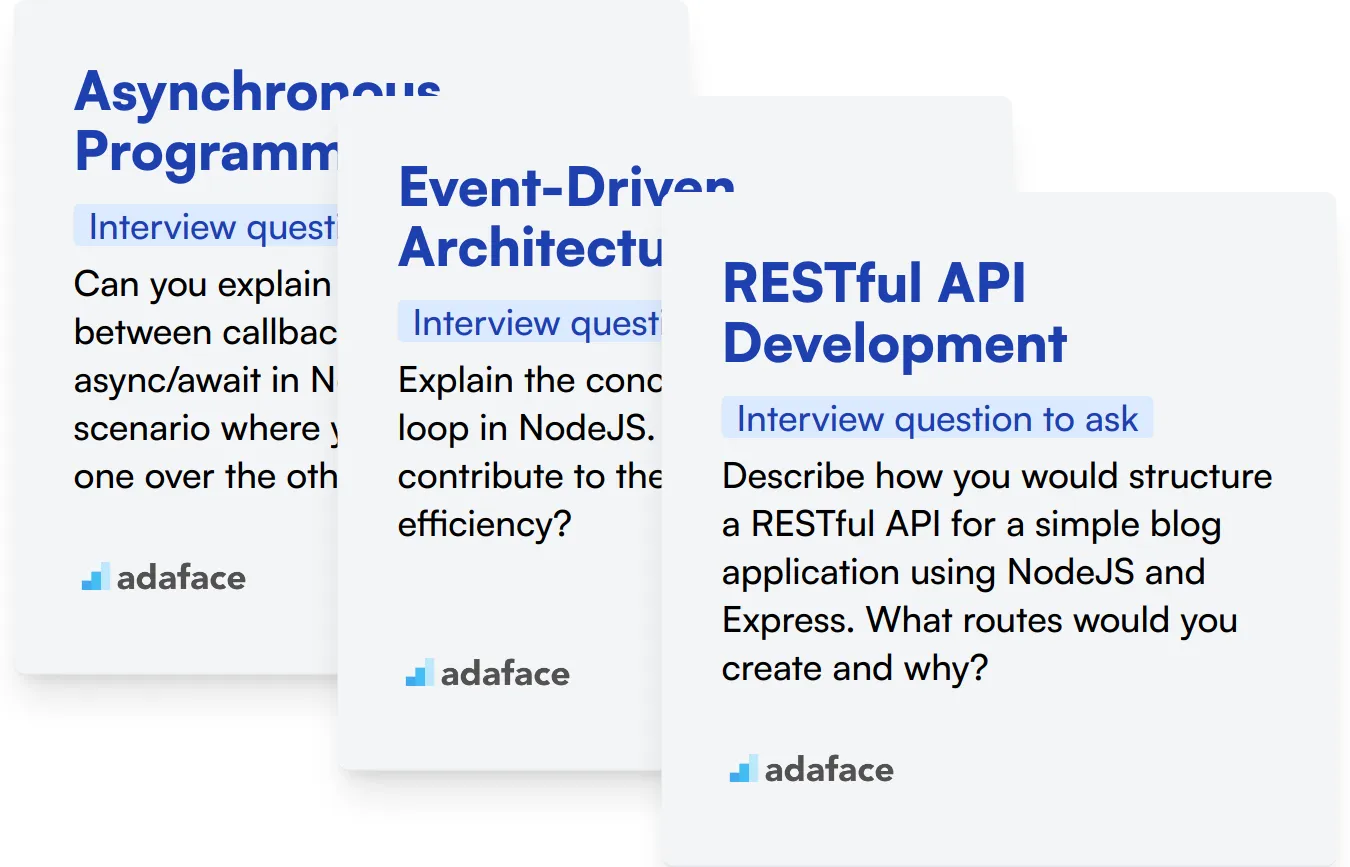
Asynchronous Programming
Asynchronous programming is at the heart of NodeJS. It allows for non-blocking I/O operations, enabling efficient handling of multiple concurrent connections.
To evaluate this skill, consider using an assessment test with relevant MCQs. This can help filter candidates based on their understanding of asynchronous concepts.
During the interview, you can ask targeted questions to gauge the candidate's grasp of asynchronous programming. Here's an example:
Can you explain the difference between callbacks, promises, and async/await in NodeJS? Provide a scenario where you would prefer one over the others.
Look for answers that demonstrate a clear understanding of these asynchronous patterns. The candidate should be able to explain the pros and cons of each approach and provide a relevant use case.
Event-Driven Architecture
NodeJS is built on an event-driven architecture. Understanding this concept is key to developing scalable and responsive applications.
Consider using an assessment test that includes questions on event emitters, listeners, and the event loop. This can help identify candidates with a solid grasp of event-driven programming.
To further evaluate this skill during the interview, you can ask a question like:
Explain the concept of the event loop in NodeJS. How does it contribute to the platform's efficiency?
Look for responses that demonstrate an understanding of how the event loop manages asynchronous operations and enables non-blocking I/O. The candidate should be able to explain how this contributes to NodeJS's performance in handling concurrent requests.
RESTful API Development
Creating RESTful APIs is a common task in NodeJS development. This skill is essential for building scalable and maintainable web applications.
An assessment test focusing on RESTful principles, HTTP methods, and common NodeJS frameworks like Express can be valuable in evaluating this skill.
During the interview, you can ask a question to assess the candidate's understanding of RESTful API development:
Describe how you would structure a RESTful API for a simple blog application using NodeJS and Express. What routes would you create and why?
Look for answers that demonstrate knowledge of RESTful principles, proper route naming conventions, and appropriate use of HTTP methods. The candidate should be able to explain their design choices and how they align with RESTful best practices.
3 Strategic Tips for Effective NodeJS Interviews
Before you start implementing what you've learned, consider these essential tips to enhance your NodeJS interview process.
1. Incorporate Skills Tests Prior to Interviews
Utilizing skills tests before interviews helps you filter candidates effectively, ensuring that you only meet with those who possess the required NodeJS expertise. This pre-screening method can significantly streamline the hiring process.
For NodeJS positions, consider tests like the NodeJS Test or the JavaScript, Node, SQL Test. These assessments evaluate candidates on key competencies, providing actionable insights into their skill levels.
By administering these tests early, you can focus your interview time on the most promising candidates, leading to more productive discussions and better hiring outcomes.
2. Curate a Focused Set of Interview Questions
Time is of the essence during interviews, and selecting the right questions can significantly impact your ability to assess candidates effectively. Aim for a balanced mix of technical and soft skills questions to evaluate both knowledge and cultural fit.
Consider relevant topics such as asynchronous programming or event-driven architecture. You may also find it useful to explore questions from our JavaScript Interview Questions list, which cover essential skills for developers.
By prioritizing thoughtfully curated questions, you can ensure that your interview time is maximized, facilitating a deeper evaluation of each candidate.
3. Ask Insightful Follow-Up Questions
Using initial interview questions is a good start, but it’s the follow-up questions that often reveal the true depth of a candidate's knowledge. Candidates sometimes provide surface-level answers that may not accurately reflect their capabilities.
For example, if a candidate states they are familiar with Promises in NodeJS, a good follow-up could be, 'Can you explain how you would handle a scenario where a Promise could potentially reject?' This question encourages deeper thinking and reveals their understanding of error handling in asynchronous programming.
Use NodeJS interview questions and skills tests to hire talented developers
If you are looking to hire someone with NodeJS skills, you need to ensure they have those skills accurately. The best way to do this is to use skill tests. Check out our NodeJS test and JavaScript NodeSQL test.
Once you use these tests, you can shortlist the best applicants and call them for interviews. Next, you can sign up on our platform or explore our online assessment platform for more information.
Node.js Online Test
Download NodeJS interview questions template in multiple formats
NodeJS Interview Questions FAQs
The questions cover basic, junior, intermediate, and advanced levels, including topics like asynchronous programming and event-driven architecture.
The post includes 15 basic, 8 junior, 12 intermediate, and 23 advanced NodeJS interview questions across various topics.
Yes, the post includes 7 situational NodeJS interview questions designed for hiring top developers.
Yes, the post offers 3 strategic tips for conducting effective NodeJS interviews and suggests using skills tests alongside interview questions.
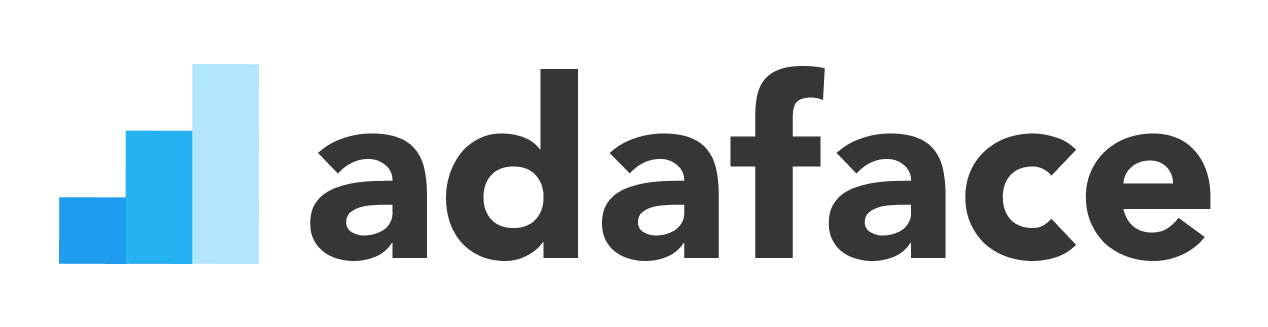
40 min skill tests.
No trick questions.
Accurate shortlisting.
We make it easy for you to find the best candidates in your pipeline with a 40 min skills test.
Try for freeRelated posts
Free resources
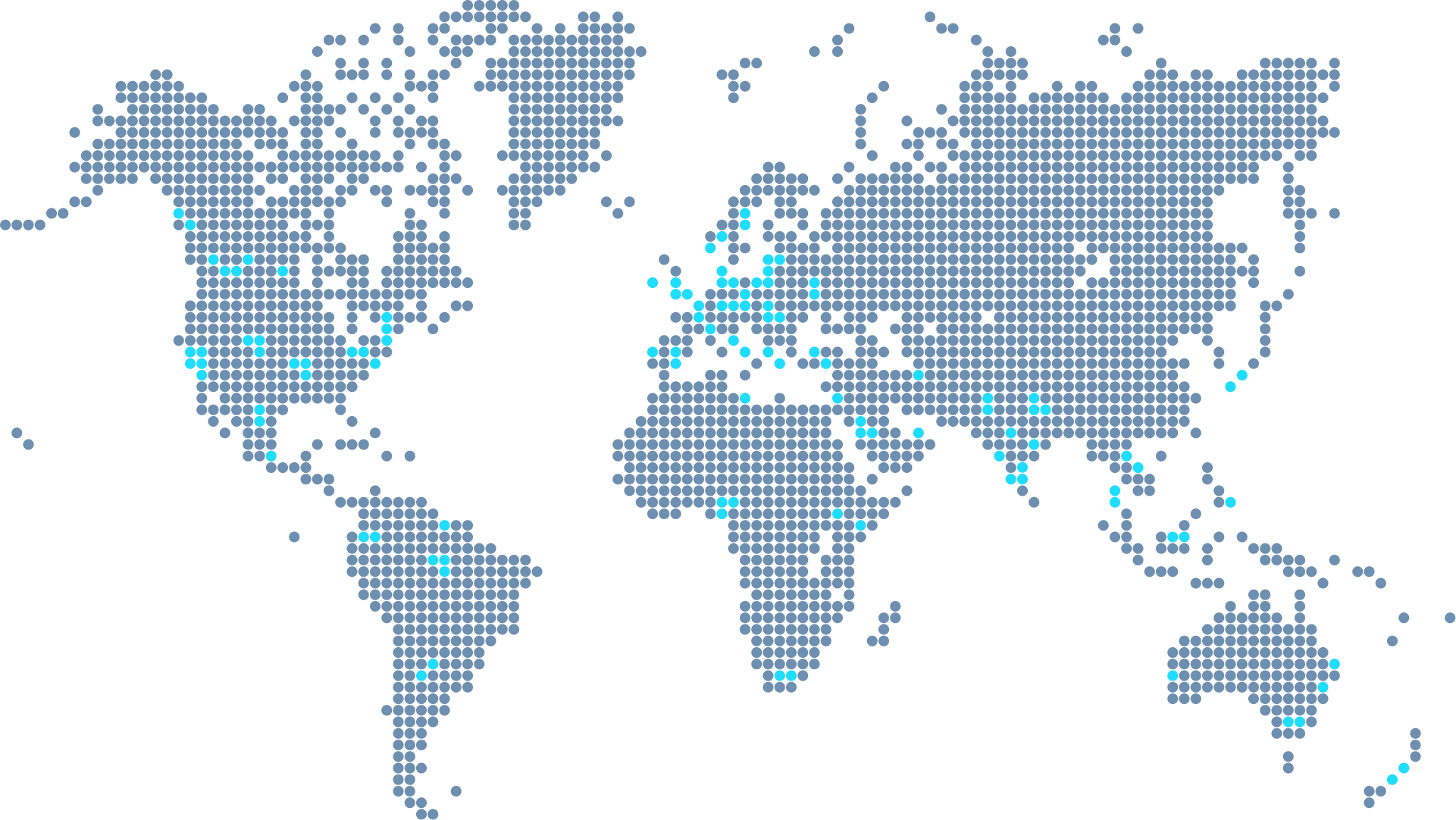
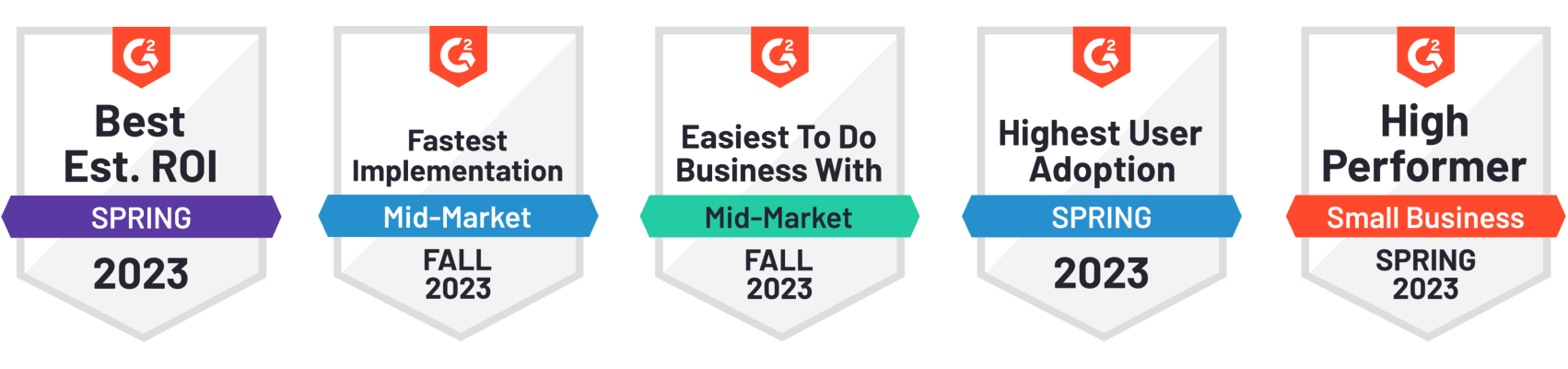