Hiring the right Laravel developer can be a challenge, especially when you're not sure what questions to ask during the interview process. A well-structured interview can help you identify candidates with the technical skills and problem-solving abilities needed for your team.
This blog post provides a comprehensive list of Laravel interview questions, categorized by experience level and topic. From general questions for beginners to advanced queries for senior developers, we've got you covered.
By using these questions, you can effectively assess candidates' Laravel expertise and make informed hiring decisions. Consider pairing these interview questions with a Laravel skills assessment to get a more complete picture of a candidate's abilities.
Table of contents
8 general Laravel interview questions and answers
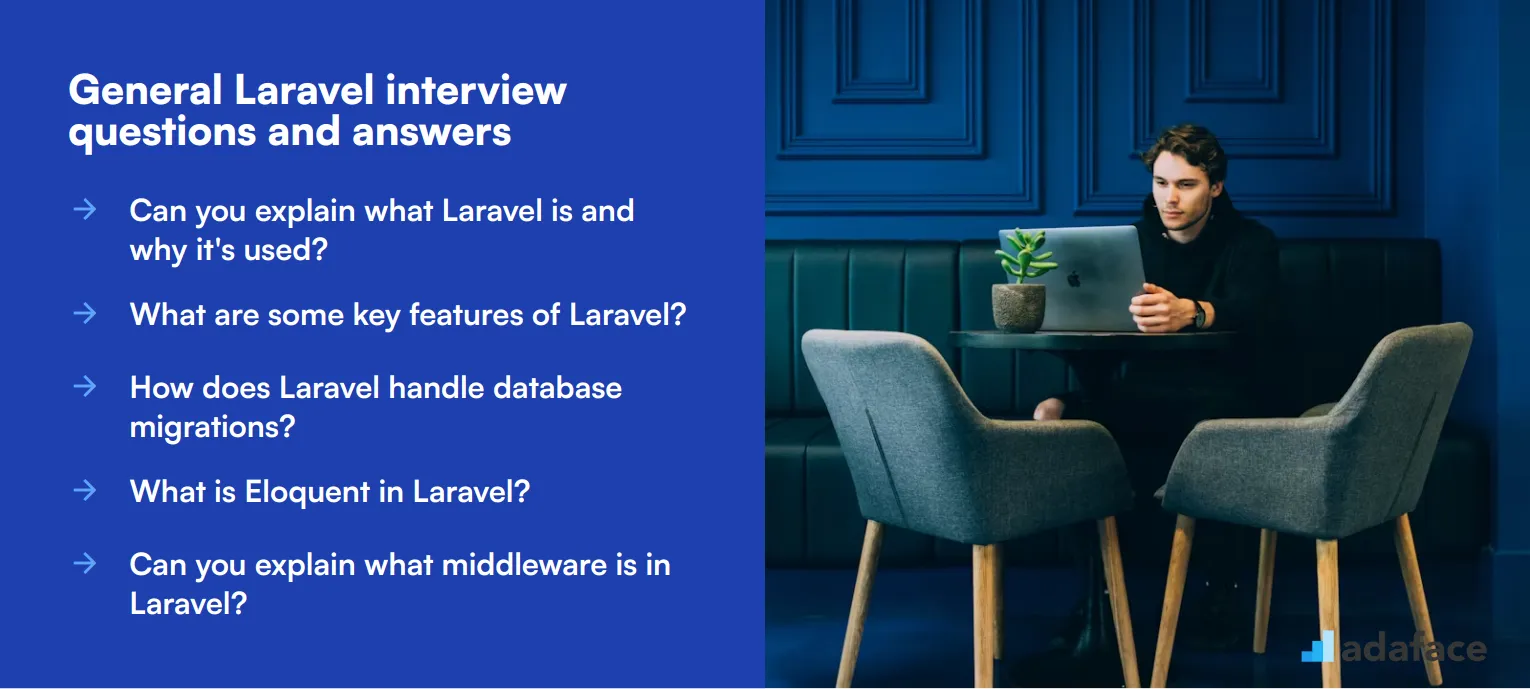
Welcome to the section where we simplify the complex world of Laravel for you! This list of interview questions and answers is designed to help recruiters and hiring managers gauge a candidate's understanding of Laravel basics. Use these questions to uncover whether your applicants have the foundational knowledge to succeed in your Laravel-based projects.
1. Can you explain what Laravel is and why it's used?
Laravel is a popular open-source PHP framework designed for web application development. It follows the Model-View-Controller (MVC) architectural pattern, which helps to separate the logic, presentation, and data layers of an application.
It's favored for its elegant syntax, robust features, and extensive libraries that simplify common tasks like routing, authentication, and caching. An ideal candidate should emphasize Laravel's ability to speed up development, enhance security, and facilitate code maintenance.
2. What are some key features of Laravel?
Laravel boasts several key features that make it a sought-after framework for web development:
Eloquent ORM: Provides a simple ActiveRecord implementation to work with databases.
Blade Templating Engine: Offers a clean, efficient way to create HTML templates.
Routing: Simplifies defining and managing routes.
Middleware: Adds extra layers of functionality to HTTP requests.
Look for candidates who not only list these features but also demonstrate an understanding of how each feature can be applied to solve real-world problems.
3. How does Laravel handle database migrations?
Laravel provides a migration system that helps in version control of databases. Migrations are like version control for your database, allowing your team to define and share the application's database schema definition.
Candidates should explain that migrations are typically used along with Laravel's Schema Builder to create and modify database tables and columns. They should also mention the ease of rollback and the ability to synchronize database changes across different environments.
Strong answers should include examples of how they have used migrations in past projects, highlighting their ability to manage database schema changes seamlessly.
4. What is Eloquent in Laravel?
Eloquent is Laravel's Object-Relational Mapper (ORM) that makes it easy to interact with your database. Each database table has a corresponding 'Model' which is used to interact with that table.
It provides an intuitive, ActiveRecord-like syntax for managing database records. Eloquent includes methods for common database operations such as Create, Read, Update, and Delete (CRUD).
Candidates should demonstrate a clear understanding of how Eloquent simplifies database operations and discuss any real-world scenarios where they have effectively used Eloquent to manage data.
5. Can you explain what middleware is in Laravel?
Middleware in Laravel is a type of filtering mechanism. It provides a convenient way to filter HTTP requests entering your application. For example, Laravel includes a middleware that verifies the user of your application is authenticated.
Middleware can be used for various tasks such as logging, authentication, and modifying request/response objects. They can be applied globally, to specific routes, or to route groups.
Look for candidates who can explain how middleware enhances the security and functionality of an application and provide examples of custom middleware they have created.
6. What is Blade templating engine in Laravel?
Blade is Laravel's powerful templating engine. Unlike other PHP templating engines, Blade does not restrict you from using plain PHP code in your views. All Blade views are compiled into plain PHP code and cached until they are modified.
Blade provides convenient shortcuts for common PHP functions and includes features like template inheritance and sections, which help in creating clean and maintainable templates.
Ideal responses should highlight Blade's efficiency and ease of use, and how it helps in creating dynamic, reusable web pages.
7. How does Laravel handle error and exception handling?
Laravel has robust error and exception handling mechanisms built-in. It uses the ExceptionHandler
class to handle all exceptions and provides a ready-to-use report
and render
method to log or display errors.
By default, Laravel logs errors in the storage directory, but this can be customized. Additionally, Laravel integrates seamlessly with third-party error tracking services.
Candidates should discuss the importance of error handling for maintaining application stability and security. They should also mention any custom error handling or logging implementations they have used in past projects.
8. What are service providers in Laravel?
Service providers are the central place of all Laravel application bootstrapping. They are responsible for binding services into the service container, registering event listeners, or even modifying the framework's default behavior.
Every service provider extends the Illuminate\Support\ServiceProvider
class and has two main methods: register
and boot
. The register
method is used to bind services into the container, while the boot
method is used to execute code after all services have been registered.
An ideal candidate should emphasize the role of service providers in making the Laravel framework highly extensible and customizable. They may also share examples of custom service providers they have created.
20 Laravel interview questions to ask junior developers
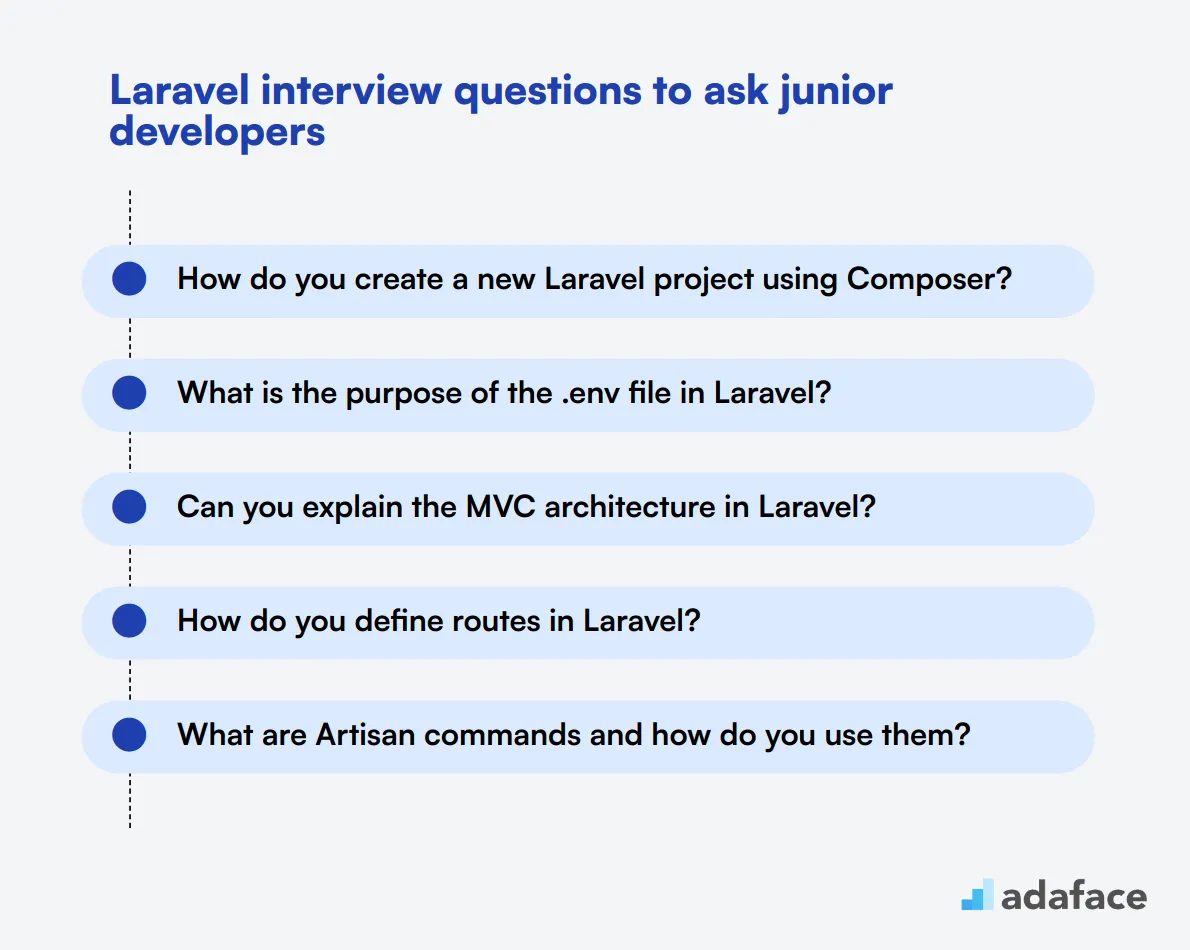
To assess the foundational knowledge of junior Laravel developers, use these 20 interview questions. They're designed to evaluate basic understanding and practical skills, helping you identify candidates who can hit the ground running in your projects.
- How do you create a new Laravel project using Composer?
- What is the purpose of the .env file in Laravel?
- Can you explain the MVC architecture in Laravel?
- How do you define routes in Laravel?
- What are Artisan commands and how do you use them?
- Explain the difference between GET and POST methods in Laravel.
- How do you create and use controllers in Laravel?
- What is the purpose of the 'php artisan tinker' command?
- How do you handle form validation in Laravel?
- What are seeders and factories in Laravel?
- How do you create and use custom helpers in Laravel?
- Explain the concept of dependency injection in Laravel.
- What is the purpose of the 'composer.json' file?
- How do you set up a database connection in Laravel?
- What are Laravel Collections and how are they used?
- How do you handle file uploads in Laravel?
- What is the purpose of the 'php artisan serve' command?
- How do you create and use view files in Laravel?
- What are Laravel's query scopes and how do you use them?
- How do you implement authentication in a Laravel application?
10 intermediate Laravel interview questions and answers to ask mid-tier developers
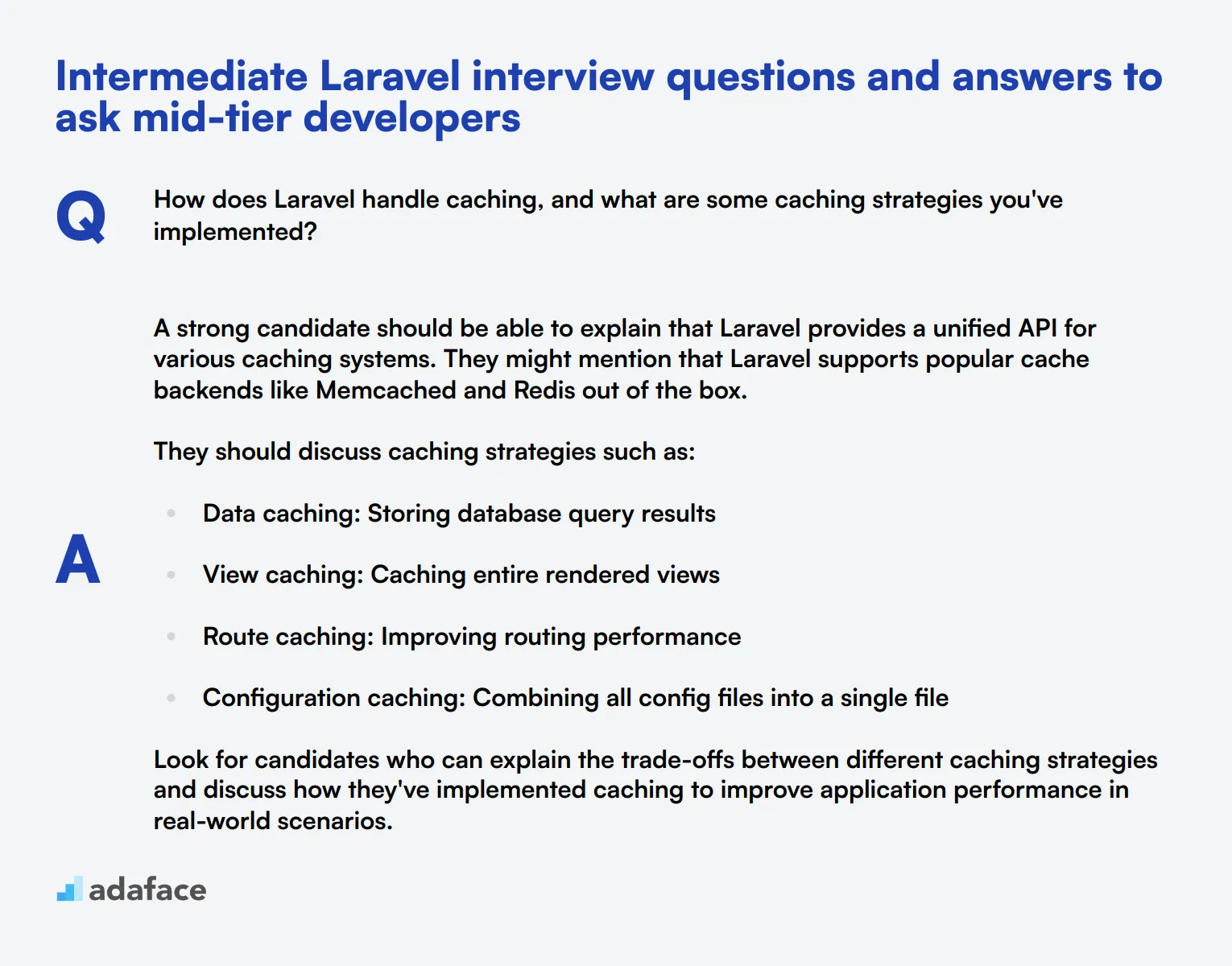
Ready to level up your Laravel interview game? These 10 intermediate questions are perfect for assessing mid-tier developers. They'll help you gauge a candidate's deeper understanding of Laravel's architecture and best practices. Remember, the goal isn't to stump them, but to spark insightful discussions about Laravel development.
1. How does Laravel handle caching, and what are some caching strategies you've implemented?
A strong candidate should be able to explain that Laravel provides a unified API for various caching systems. They might mention that Laravel supports popular cache backends like Memcached and Redis out of the box.
They should discuss caching strategies such as:
- Data caching: Storing database query results
- View caching: Caching entire rendered views
- Route caching: Improving routing performance
- Configuration caching: Combining all config files into a single file
Look for candidates who can explain the trade-offs between different caching strategies and discuss how they've implemented caching to improve application performance in real-world scenarios.
2. Can you explain Laravel's event broadcasting system and when you might use it?
A knowledgeable candidate should explain that Laravel's event broadcasting allows you to broadcast your server-side Laravel events to your client-side JavaScript application.
They might mention use cases such as:
- Real-time notifications
- Live chat applications
- Collaborative tools (e.g., shared document editing)
- Live updates for dashboards or analytics
Look for candidates who can discuss the concept of channels, how to configure broadcasting drivers (like Pusher or Redis), and how broadcasting integrates with Laravel Echo on the client side. They should also be able to explain the benefits of using event broadcasting for creating real-time, reactive applications.
3. How do you handle API versioning in Laravel, and why is it important?
A proficient candidate should explain that API versioning is crucial for maintaining backward compatibility while allowing for future improvements. They might discuss several approaches to API versioning in Laravel:
- URL versioning (e.g., /api/v1/users)
- Header versioning (using Accept or Custom-Version headers)
- Query parameter versioning (e.g., /api/users?version=1)
They should be able to explain how to implement these methods using Laravel's routing system and middleware.
Look for candidates who can discuss the pros and cons of different versioning strategies and explain how they've handled API evolution in past projects. They should also touch on the importance of documentation and communication when introducing new API versions.
4. Explain Laravel's job queues and when you would use them.
A competent candidate should explain that Laravel's job queues allow you to defer time-consuming tasks, such as sending emails or processing large datasets, to be processed in the background. This improves the application's response time and overall user experience.
They might mention common use cases for job queues:
- Sending bulk emails
- Generating reports
- Processing uploads
- Interacting with external APIs
Look for candidates who can discuss different queue drivers (like database, Redis, or Amazon SQS), explain how to create and dispatch jobs, and mention features like delayed job execution and job batching. They should also be able to explain how job queues contribute to building scalable applications.
5. How do you implement and use Laravel's policy classes for authorization?
An experienced candidate should explain that policy classes in Laravel are used to organize authorization logic around a particular model or resource. They encapsulate the logic for determining if a user can perform various actions on a resource.
They should be able to describe the process of:
- Creating a policy class using Artisan commands
- Defining methods for different actions (view, create, update, delete)
- Registering the policy in the AuthServiceProvider
- Using the policy in controllers or blade templates
Look for candidates who can discuss real-world scenarios where they've used policies to implement complex authorization rules. They should also be able to explain how policies relate to Laravel's Gate facade and middleware for authorization.
6. What are Laravel's service containers and how do they facilitate dependency injection?
A knowledgeable candidate should explain that Laravel's service container is a powerful tool for managing class dependencies and performing dependency injection. It allows you to define complex dependencies and their resolutions in a centralized location.
They might discuss:
- Binding interfaces to implementations
- Automatic resolution of dependencies
- Contextual binding
- Tagging
Look for candidates who can provide examples of how they've used the service container to improve code modularity and testability. They should be able to explain the benefits of dependency injection and how it relates to the SOLID principles, particularly the Dependency Inversion Principle.
7. How do you implement and use Laravel's custom validation rules?
A proficient candidate should explain that Laravel allows you to create custom validation rules when the built-in rules aren't sufficient. They should be able to describe the process of creating a custom rule:
- Generate a new rule class using Artisan
- Implement the passes method to define the validation logic
- Implement the message method to define the error message
- Register the rule in the AppServiceProvider or use it directly in validation
Look for candidates who can provide examples of custom rules they've created in past projects. They should also be able to discuss when it's appropriate to create a custom rule versus using Laravel's built-in rule combinations or closure-based custom rules.
8. Explain Laravel's model observers and when you might use them.
An experienced candidate should explain that model observers in Laravel allow you to hook into various model events such as creating, created, updating, updated, deleting, and deleted. This provides a way to centralize handling of model events without cluttering the model itself.
They might mention use cases such as:
- Sending notifications when a model is created or updated
- Updating related models
- Logging changes for audit purposes
- Clearing caches when data changes
Look for candidates who can discuss how observers compare to model events and when they prefer to use one over the other. They should also be able to explain how to register observers and how they contribute to keeping models lean and focused on their primary responsibility.
9. How do you implement and use Laravel's query scopes?
A competent candidate should explain that query scopes in Laravel allow you to encapsulate common query constraints that you can easily re-use throughout your application. They should be able to describe both local and global scopes.
For local scopes, they might explain:
- How to define a scope method in the model
- How to use the scope in queries
For global scopes, they should discuss:
- Creating a separate class for the global scope
- Implementing the apply method
- Registering the global scope with the model
Look for candidates who can provide examples of how they've used scopes to make their code more readable and maintainable. They should also be able to discuss scenarios where scopes are particularly useful, such as implementing multi-tenancy or soft deletes.
10. Explain Laravel's rate limiting feature and when you would use it.
A knowledgeable candidate should explain that Laravel's rate limiting feature allows you to limit the number of requests a user or IP can make to your application within a specified time frame. This is crucial for preventing abuse and ensuring fair usage of your API or application.
They might discuss:
- Configuring rate limiters in the app/Providers/RouteServiceProvider.php file
- Applying rate limiting to routes or route groups
- Using the throttle middleware
- Customizing the response when the limit is exceeded
Look for candidates who can provide examples of when they've implemented rate limiting, such as in API development or to protect against brute force attacks. They should also be able to discuss how rate limiting contributes to application security and performance optimization.
19 advanced Laravel interview questions to ask senior developers
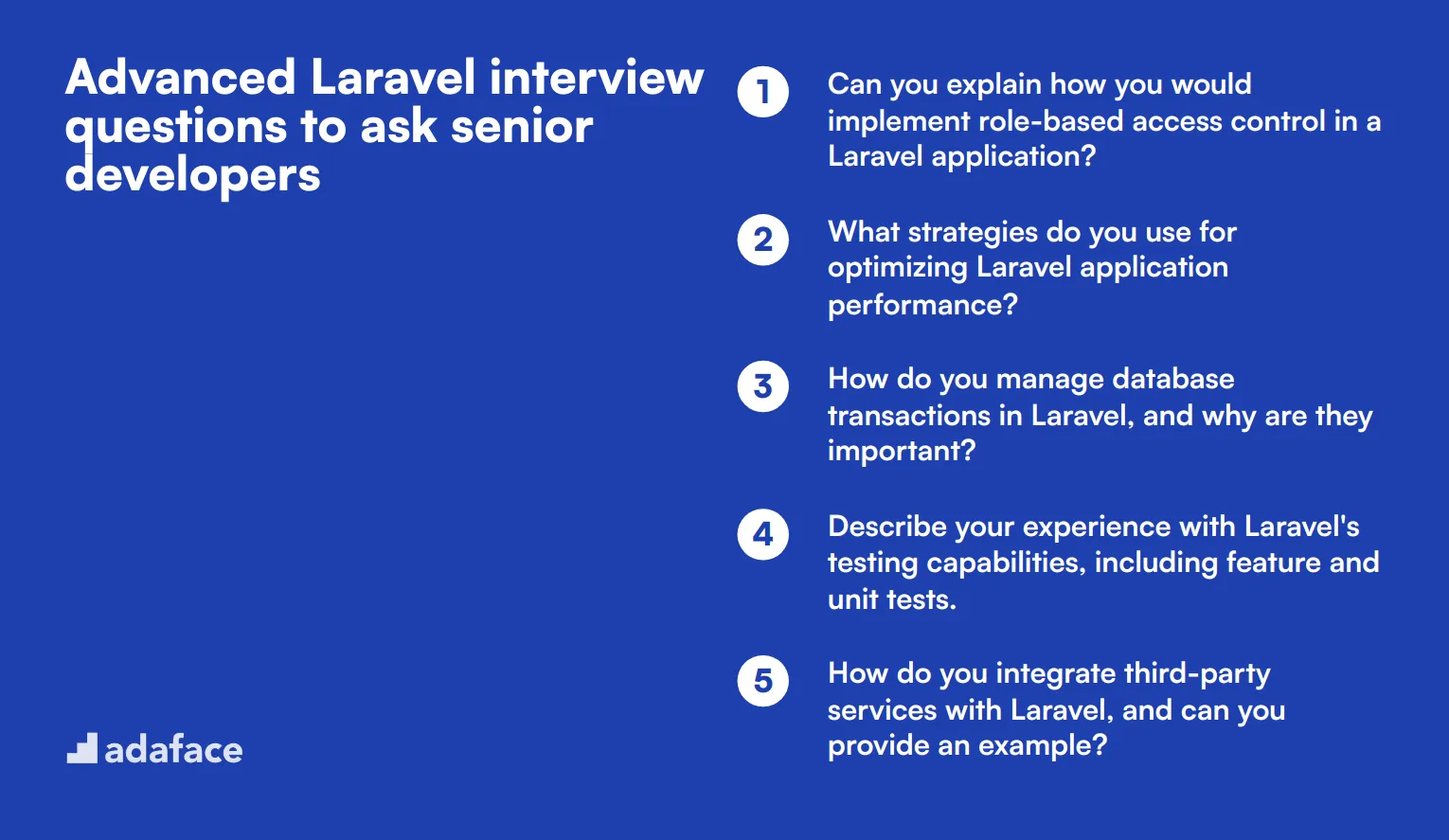
To assess whether candidates possess the necessary expertise in advanced Laravel concepts, use this list of interview questions. These inquiries are designed to evaluate a senior developer's ability to tackle complex tasks and make informed decisions in real-world scenarios. For a comprehensive understanding of the role, consider reviewing the skills required for a Laravel developer.
- Can you explain how you would implement role-based access control in a Laravel application?
- What strategies do you use for optimizing Laravel application performance?
- How do you manage database transactions in Laravel, and why are they important?
- Describe your experience with Laravel's testing capabilities, including feature and unit tests.
- How do you integrate third-party services with Laravel, and can you provide an example?
- What is your approach to handling and logging errors in a Laravel application?
- Can you explain the process of creating and using Laravel packages?
- How do you ensure security best practices in Laravel applications?
- What are the benefits of using Laravel Mix in your projects?
- Can you discuss how to implement multi-tenancy in Laravel?
- How do you handle background tasks and scheduling in Laravel?
- What strategies do you use for implementing caching in Laravel applications?
- Can you explain the differences between Laravel's route model binding and traditional binding?
- How do you manage configuration across different environments in a Laravel application?
- What is your experience with Laravel's API resources and how do you use them?
- How do you implement custom logging channels in Laravel?
- Can you explain the concept of service containers and how you utilize them in your projects?
- What techniques do you use for optimizing database queries in Laravel?
- How do you approach the deployment of a Laravel application in a production environment?
9 Laravel interview questions and answers related to technical definitions
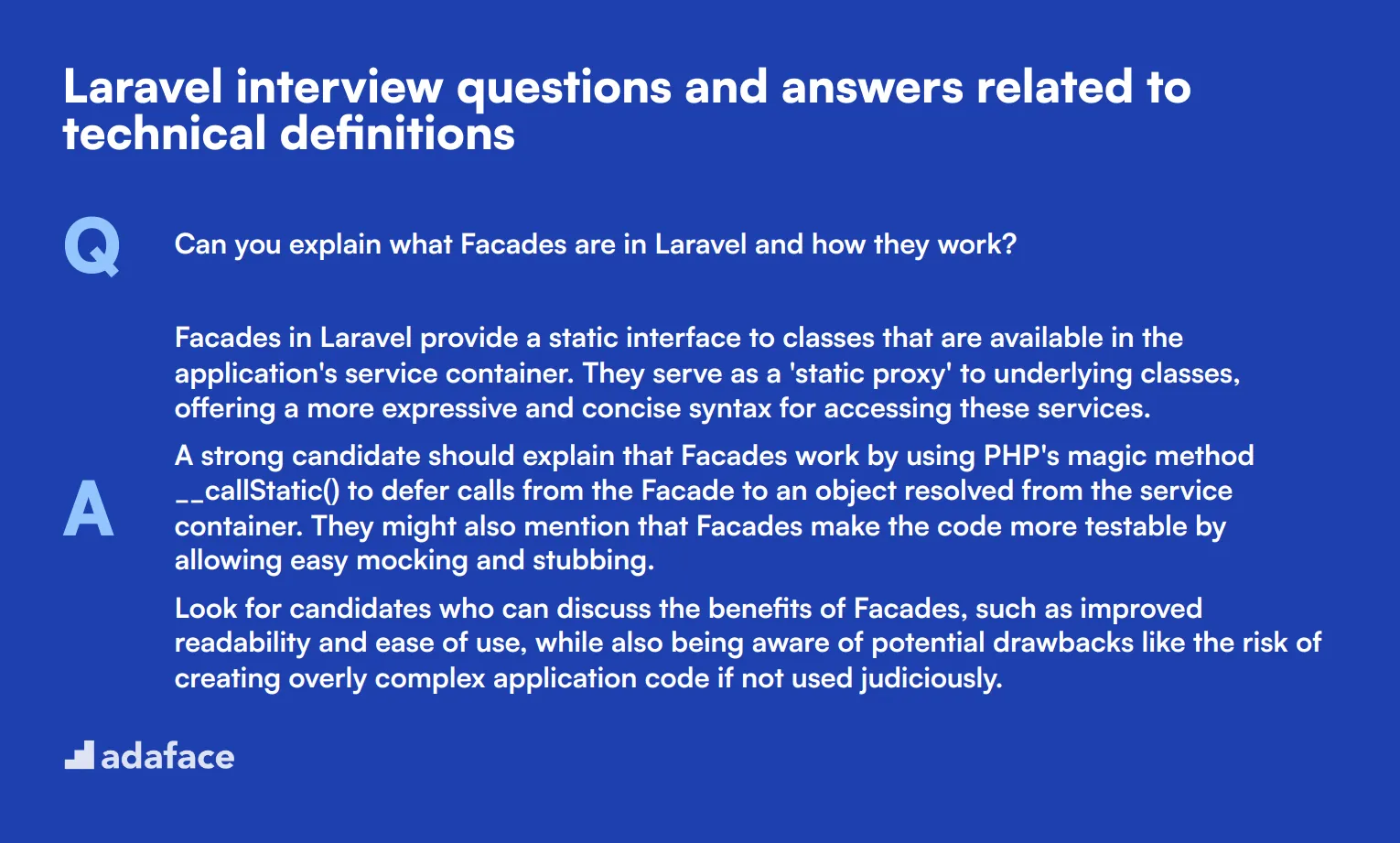
To assess whether your Laravel developer candidates have a solid grasp of technical concepts, use these 9 interview questions about technical definitions. These questions will help you gauge the depth of their Laravel knowledge and their ability to articulate complex ideas, which is crucial for effective team communication and problem-solving in web development projects.
1. Can you explain what Facades are in Laravel and how they work?
Facades in Laravel provide a static interface to classes that are available in the application's service container. They serve as a 'static proxy' to underlying classes, offering a more expressive and concise syntax for accessing these services.
A strong candidate should explain that Facades work by using PHP's magic method __callStatic() to defer calls from the Facade to an object resolved from the service container. They might also mention that Facades make the code more testable by allowing easy mocking and stubbing.
Look for candidates who can discuss the benefits of Facades, such as improved readability and ease of use, while also being aware of potential drawbacks like the risk of creating overly complex application code if not used judiciously.
2. What is the purpose of the Laravel service container?
The Laravel service container is a powerful tool for managing class dependencies and performing dependency injection. It's essentially a container for all the various services that the application needs to function.
A knowledgeable candidate should explain that the service container can automatically resolve dependencies for classes without requiring manual configuration. They might also mention that it allows for the binding of interfaces to concrete implementations, facilitating more flexible and maintainable code.
Pay attention to candidates who can discuss practical uses of the service container, such as registering services, resolving dependencies, and how it contributes to writing more modular and testable code in Laravel applications.
3. How does Laravel handle database transactions?
Laravel provides a simple and expressive way to handle database transactions. Transactions ensure that a series of database operations are treated as a single unit of work, either all succeeding or all failing together.
A competent candidate should explain that Laravel offers both a fluent interface and a closure-based approach for working with transactions. They might demonstrate knowledge of the DB::transaction() method, which automatically handles commit and rollback operations based on whether an exception is thrown within the transaction closure.
Look for candidates who can discuss best practices for using transactions, such as keeping transaction blocks as short as possible and understanding when to use them (e.g., when multiple related database operations need to succeed or fail as a unit).
4. What are Laravel's query scopes and how are they used?
Query scopes in Laravel allow you to define common sets of constraints that you can easily re-use throughout your application. They help in keeping your code DRY (Don't Repeat Yourself) and make your queries more readable and maintainable.
A proficient candidate should explain that there are two types of scopes: local scopes and global scopes. Local scopes are defined as methods in the model and can be chained onto an existing query. Global scopes are applied to all queries for a given model.
Evaluate candidates based on their ability to provide examples of when and how to use query scopes effectively. They should be able to discuss scenarios where scopes can simplify complex queries or standardize certain conditions across an application.
5. Explain the concept of method injection in Laravel.
Method injection in Laravel is a form of dependency injection where dependencies are injected directly into a method rather than through a class constructor. This is particularly useful when a class doesn't always need a dependency, but only requires it for specific methods.
A knowledgeable candidate should explain that Laravel's service container automatically resolves these dependencies when the method is called. They might also mention that this feature is commonly used in controller methods, where different actions might require different dependencies.
Look for candidates who can discuss the benefits of method injection, such as improved performance (by not loading unnecessary dependencies) and more flexible code structure. They should also be able to compare it with constructor injection and explain when each approach is more appropriate.
6. What are Laravel's database seeders and how are they used?
Database seeders in Laravel are a way to populate your database with test or initial data. They are particularly useful for setting up consistent test data or for initializing a database with default values needed for the application to run.
A strong candidate should explain that seeders are PHP classes, typically stored in the database/seeders directory. They might mention the run method, which is called when the seeder is executed, and how seeders can be used in conjunction with model factories to generate large amounts of sample data efficiently.
Assess candidates based on their understanding of when and how to use seeders effectively. They should be able to discuss best practices, such as using seeders for development and testing environments, and how to structure seeders for complex data relationships.
7. How does Laravel implement the repository pattern?
The repository pattern in Laravel is an architectural pattern that abstracts the data layer, providing a more loosely coupled approach to data access. While Laravel doesn't implement this pattern out of the box, it provides the tools and flexibility to easily implement it.
A proficient candidate should explain that implementing the repository pattern typically involves creating interfaces that define method contracts and concrete repository classes that implement these interfaces. They might discuss how this pattern can be used with Laravel's service container for dependency injection.
Look for candidates who can articulate the benefits of using the repository pattern, such as improved testability, code organization, and the ability to swap out data sources without affecting the rest of the application. They should also be aware of potential drawbacks, like increased complexity for smaller applications.
8. What is the purpose of Laravel's service providers?
Service providers in Laravel are the central place of all application bootstrapping. They are responsible for registering services, event listeners, middleware, and even routes. They allow you to organize related bootstrapping logic into a single location.
A knowledgeable candidate should explain that service providers have two primary methods: register() for binding things into the service container, and boot() for performing tasks after all providers are registered. They might also mention the difference between deferred and non-deferred providers.
Evaluate candidates based on their understanding of when and how to create custom service providers. They should be able to discuss scenarios where custom providers are beneficial, such as when integrating third-party packages or organizing complex application bootstrapping logic.
9. How does Laravel handle broadcasting and what is its primary use case?
Laravel's event broadcasting allows you to broadcast your server-side Laravel events to your client-side JavaScript application. This feature is primarily used for building real-time, live-updating user interfaces.
A strong candidate should explain that broadcasting works by pushing server-side events over WebSockets to the client-side. They might mention Laravel Echo, a JavaScript library that makes it easy to subscribe to channels and listen for events on the client-side.
Look for candidates who can discuss practical use cases for broadcasting, such as real-time notifications, live chat applications, or collaborative tools. They should also be aware of the different broadcasting drivers Laravel supports (Pusher, Redis, etc.) and their implications for application architecture.
9 Laravel interview questions and answers related to framework
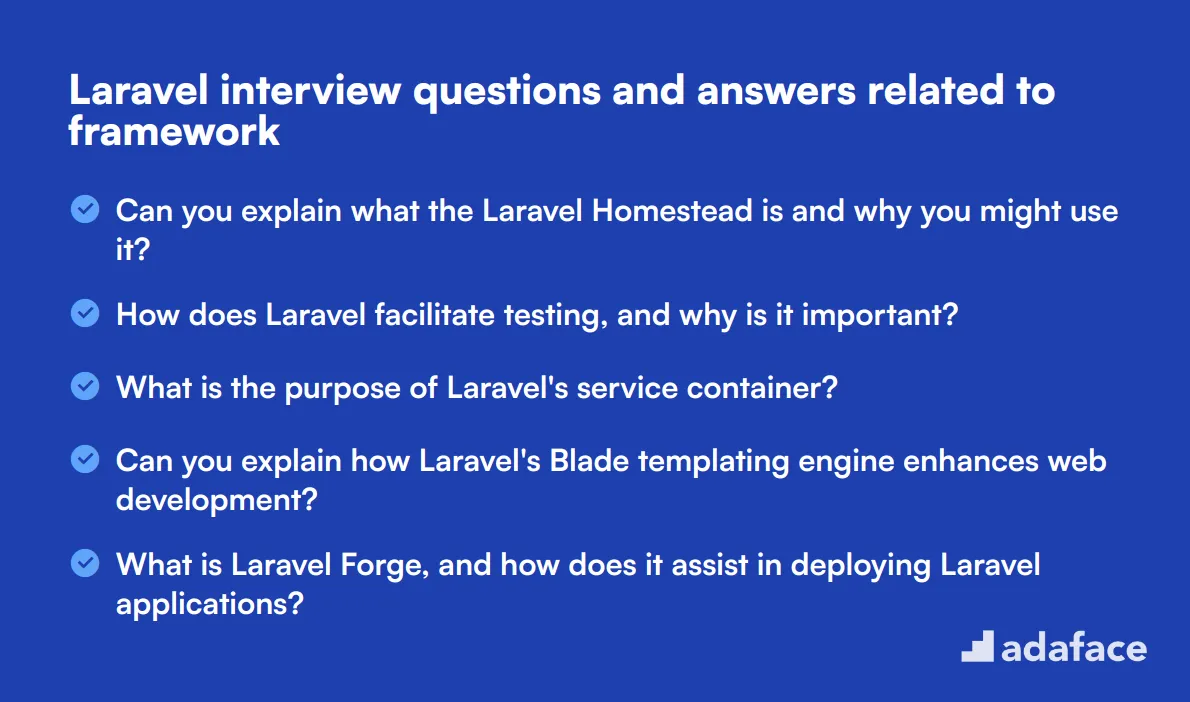
If you're looking to identify whether candidates have a solid grasp of Laravel, this question list will be your go-to resource. Use these questions during interviews to gauge their understanding of the framework's core principles and practical applications.
1. Can you explain what the Laravel Homestead is and why you might use it?
Laravel Homestead is a pre-packaged Vagrant box that provides a robust development environment without requiring you to install PHP, a web server, and other server software on your local machine. This is especially useful for maintaining a consistent environment across different development and production setups.
Candidates should articulate that Homestead simplifies the setup process and ensures that the development environment mimics the production environment as closely as possible. Look for answers that highlight ease of use, consistency, and the ability to quickly set up a fresh environment.
Ideal responses should also touch on the benefits of using Homestead for team collaboration, as all team members can work in identical environments, reducing the 'it works on my machine' problem.
2. How does Laravel facilitate testing, and why is it important?
Laravel makes testing a breeze by providing a built-in testing suite based on PHPUnit. This enables developers to write unit tests and feature tests to ensure that their application works as expected.
Candidates should explain that Laravel's testing tools help catch bugs early, improve code quality, and make refactoring safer. Strong responses should emphasize the role of automated tests in maintaining software stability and reliability.
Look for candidates who mention specific testing features like database migrations for testing, mock objects, and the ability to simulate HTTP requests. This shows a deep understanding of how to implement comprehensive testing strategies.
3. What is the purpose of Laravel's service container?
The service container in Laravel is a powerful tool used for managing dependencies and performing dependency injection. It essentially acts as a central registry where objects and their dependencies are stored and retrieved when needed.
A strong candidate should describe how the service container allows for more flexible and scalable code by decoupling class dependencies. This makes the application easier to manage and test, as you can inject mock dependencies during testing.
Look for explanations that highlight practical use cases, such as injecting services into controllers or managing complex dependency trees. Candidates should show an understanding of how this promotes better software architecture and maintainability.
4. Can you explain how Laravel's Blade templating engine enhances web development?
Blade is Laravel's powerful, simple, and lightweight templating engine that allows developers to use plain PHP code in views. It provides a convenient syntax for common tasks like loops and conditionals, making it easier to write and maintain HTML templates.
Candidates should mention that Blade templates are compiled into plain PHP code and cached, which improves performance. They should also highlight Blade's ability to include layouts and components, facilitating code reuse and reducing duplication.
Ideal responses should touch on the advantages of using Blade for web development, such as better readability and maintainability of view files, and the ease of integrating dynamic data into templates.
5. What is Laravel Forge, and how does it assist in deploying Laravel applications?
Laravel Forge is a server management tool that simplifies the deployment of Laravel applications. It automates the process of setting up, managing, and deploying web applications on various cloud service providers.
Candidates should explain that Forge helps in server provisioning, setting up databases, installing software, and configuring environments. It takes care of routine tasks, allowing developers to focus more on coding and less on server management.
Look for answers that highlight how Forge enhances deployment efficiency, reduces manual errors, and allows for seamless scaling. Strong candidates might also mention the integration with Git repositories for automated deployments.
6. How does Laravel's localization feature work, and why is it useful?
Laravel's localization feature allows applications to support multiple languages, making it easier to reach a global audience. It provides a simple way to manage translations by storing language strings in various files within the resources/lang
directory.
Candidates should describe how developers can use localization to switch between languages dynamically based on user preferences or browser settings. This enhances the user experience by providing content in the user's native language.
Ideal responses should mention the importance of localization in applications targeting diverse markets and how it contributes to better user engagement. Look for candidates who can discuss practical scenarios where they implemented localization in their projects.
7. Can you explain the concept of 'queues' in Laravel and when you would use them?
Queues in Laravel allow you to defer the processing of time-consuming tasks, such as sending emails or processing uploads, to a later time. This helps in improving the performance and responsiveness of your application by handling these tasks asynchronously.
Candidates should mention that Laravel provides a unified API for different queue backends, such as Beanstalkd, Amazon SQS, and Redis. This flexibility makes it easier to integrate queues into various environments and use cases.
Look for answers that highlight the importance of queues in managing background tasks and improving application performance. Strong candidates might share specific examples of how they used queues to handle resource-intensive operations efficiently.
8. How does Laravel's built-in authentication system work?
Laravel's built-in authentication system provides a straightforward way to handle user authentication. It includes features like registration, login, password reset, and email verification out of the box, reducing the need for custom implementations.
Candidates should explain that Laravel uses middleware to protect routes, ensuring that only authenticated users can access certain parts of the application. The system also provides various authentication guards, such as session and token-based guards, to suit different needs.
Look for candidates who can discuss the ease of setting up authentication using Laravel's scaffolding commands and how it enhances security in applications. Strong responses might include practical examples of implementing custom authentication logic.
9. What is Laravel's task scheduling, and how does it simplify cron job management?
Laravel's task scheduling offers a convenient way to manage scheduled tasks using a simple, fluent API. It allows developers to define scheduled tasks in a single location, the app/Console/Kernel.php
file, without needing to SSH into the server to edit cron entries.
Candidates should mention that the task scheduler can handle various task frequencies, such as hourly, daily, or even custom intervals. It also provides methods for task chaining, conditional execution, and task output management.
Look for answers that highlight how Laravel's task scheduling simplifies the management of cron jobs, improves readability, and reduces server configuration errors. Candidates should show an understanding of how this feature enhances application maintenance and operational efficiency.
7 situational Laravel interview questions with answers for hiring top developers
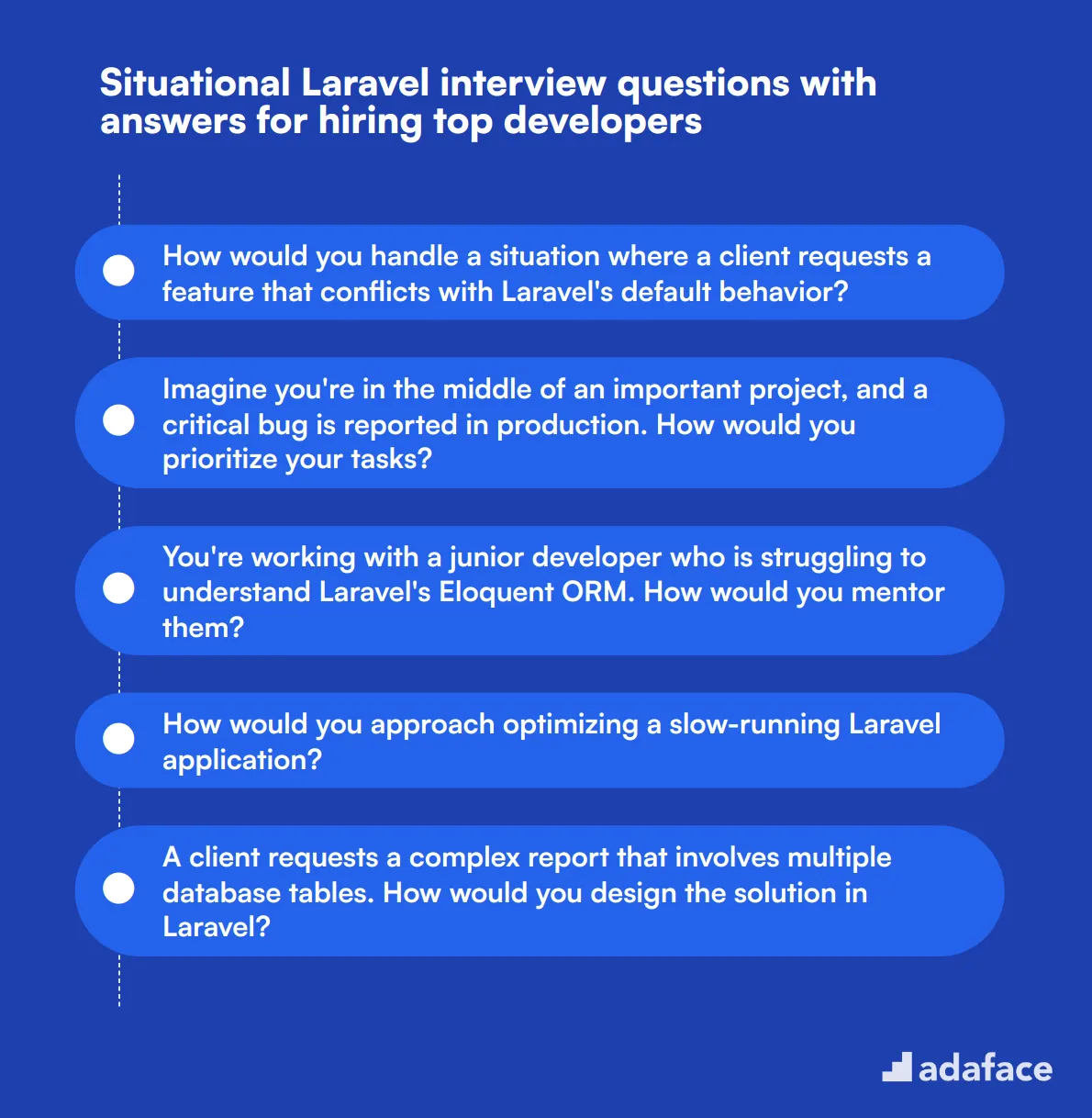
To gauge whether your applicants can handle real-world challenges with Laravel, ask them some of these situational interview questions. These questions are designed to go beyond textbook knowledge and assess how candidates would respond to practical scenarios.
1. How would you handle a situation where a client requests a feature that conflicts with Laravel's default behavior?
In such a scenario, it's essential to communicate clearly with the client. I would first explain why the requested feature conflicts with Laravel's default behavior and discuss the potential consequences of implementing it.
If the client still insists, I would explore alternative solutions that align with Laravel's best practices while meeting the client's needs. This might involve custom coding or using third-party packages that extend Laravel's capabilities.
What to look for: Look for candidates who emphasize clear communication, client education, and finding a balanced solution that maintains code integrity.
2. Imagine you're in the middle of an important project, and a critical bug is reported in production. How would you prioritize your tasks?
First, I would assess the severity of the bug and its impact on the application. If it's a critical bug affecting many users or core functionality, it would take immediate priority.
I would communicate the situation to the team and stakeholders, pausing less critical tasks to focus on resolving the bug. Once resolved, I would implement measures to prevent similar issues in the future.
What to look for: Ideal candidates should demonstrate strong problem-solving skills, the ability to prioritize effectively, and clear communication with the team and stakeholders.
3. You're working with a junior developer who is struggling to understand Laravel's Eloquent ORM. How would you mentor them?
I would start by breaking down complex concepts into simpler terms and using analogies to make Eloquent ORM easier to understand. Providing hands-on examples and pairing programming sessions can also be highly effective.
Additionally, I would recommend useful resources like Laravel's official documentation and community tutorials to support their learning.
What to look for: Strong candidates should emphasize patience, the ability to simplify complex topics, and providing ongoing support and resources.
4. How would you approach optimizing a slow-running Laravel application?
I would start by profiling the application to identify bottlenecks using tools like Laravel Telescope or external profilers. Common areas to check include database queries, caching strategies, and middleware performance.
After identifying the bottlenecks, I would implement optimizations such as query optimization, effective use of caching, and minimizing middleware where possible.
What to look for: Look for candidates who can systematically identify and address performance issues, demonstrating a methodical approach to optimization.
5. A client requests a complex report that involves multiple database tables. How would you design the solution in Laravel?
I would start by understanding the specific requirements for the report. Then, I would design the database queries, potentially using Eloquent relationships to fetch the necessary data efficiently.
For complex data manipulation, I might use Eloquent's query builder or raw SQL queries if needed. The final step would be to format the data in a way that meets the client's reporting needs.
What to look for: Ideal candidates should show a clear understanding of Eloquent relationships and efficient data fetching techniques, along with a knack for translating requirements into technical solutions.
6. How do you handle a situation where your Laravel application needs to integrate with a third-party API that has limited documentation?
I would begin by reviewing any available documentation and resources from the third-party provider. If documentation is limited, I would explore the API endpoints using tools like Postman to understand their behavior.
I would also reach out to the provider for additional support if needed. Once familiar with the API, I would implement the integration in Laravel, ensuring proper error handling and logging.
What to look for: Look for candidates who are resourceful, able to work with limited information, and emphasize thorough testing and error handling in their integrations.
7. You inherit a Laravel project with no documentation. How would you go about understanding and maintaining the project?
I would start by exploring the project's structure, focusing on key areas like routes, controllers, and models. Using Laravel's in-built tools like Artisan commands can help generate useful insights.
I would also run the application locally to understand its functionality and identify any immediate issues. Gradually, I would document my findings and establish a plan for maintaining and improving the codebase.
What to look for: Strong candidates should be able to navigate and understand existing code with minimal guidance, and emphasize the importance of creating documentation for future reference.
Which Laravel skills should you evaluate during the interview phase?
While it's unrealistic to gauge all facets of a candidate's capabilities through a single interview, it's imperative to focus on certain key Laravel skills that provide a solid indication of their proficiency and potential fit for the role.
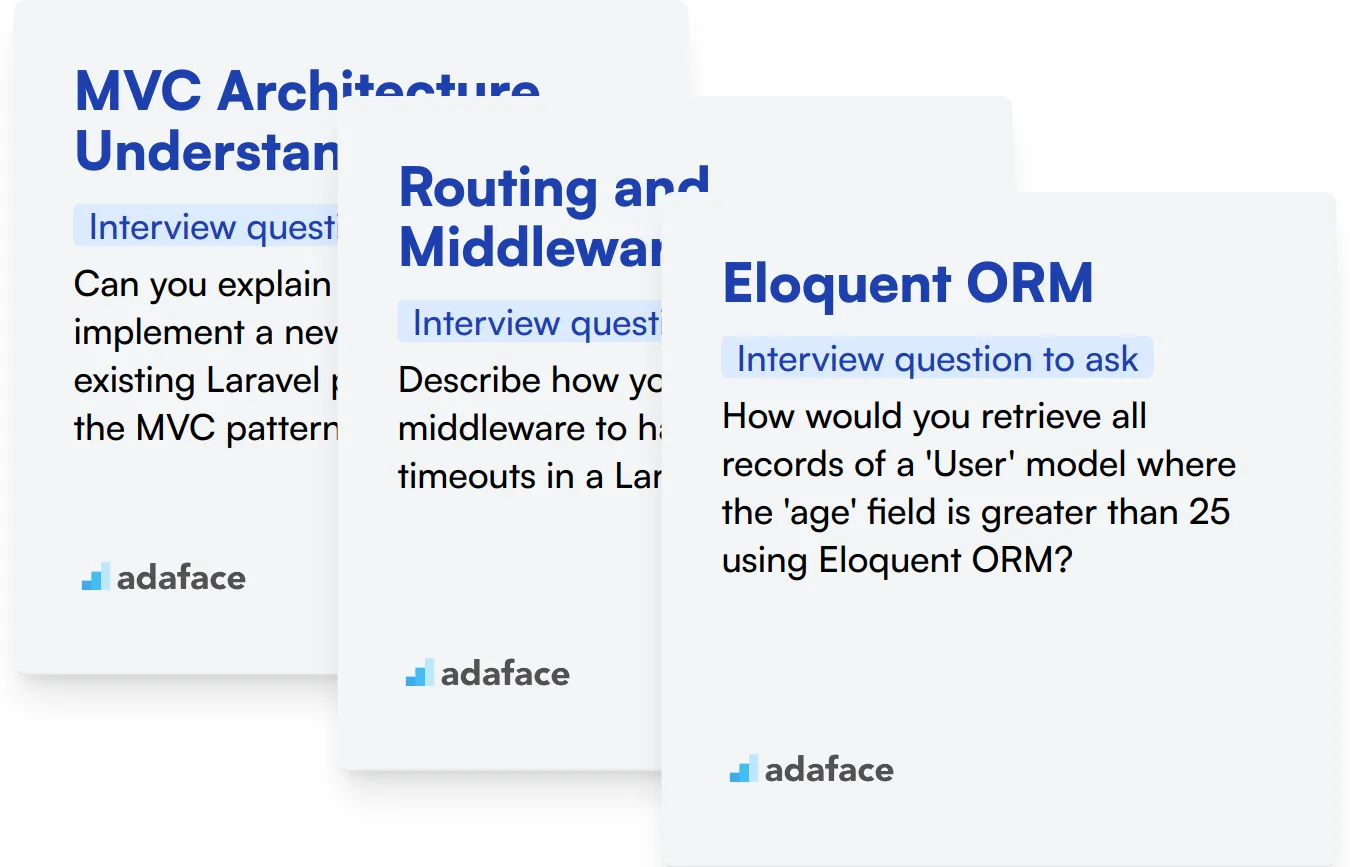
MVC Architecture Understanding
A profound understanding of the MVC (Model-View-Controller) architecture is fundamental for any Laravel developer. This pattern is at the core of Laravel, facilitating clarity between logic and presentation, which helps in building organized, efficient web applications.
To screen candidates efficiently, consider utilizing an assessment that covers MVC principles. You can try our Laravel Developer Online Test which includes relevant MCQs to assess this skill effectively.
Additionally, probing their practical knowledge with targeted interview questions can provide deeper insights into their expertise.
Can you explain how you would implement a new feature in an existing Laravel project following the MVC pattern?
Look for candidates who articulate clear separation of concerns among the model, view, and controller. Their response should demonstrate a structured approach to integrating and scaling features appropriately.
Routing and Middleware
Effective routing and use of middleware are essential for building and securing web applications in Laravel. These skills help in directing traffic within applications and applying necessary filters to HTTP requests.
Using a pre-built test can streamline the initial evaluation process. The Laravel Developer Online Test at Adaface, includes sections evaluating knowledge of routing and middleware.
In interviews, discussing real-world scenarios helps assess how well a developer understands and applies these concepts.
Describe how you would use middleware to handle session timeouts in a Laravel application.
Look for detailed explanations on the use of middleware for session management. Effective answers should include specific Laravel middleware methods along with a rationale for their use.
Eloquent ORM
Laravel's Eloquent ORM provides a beautifully simple ActiveRecord implementation for working with the database. Each database table has a corresponding "Model" that allows interacting with that table. Understanding ORM is indicative of a developer's ability to manipulate database records efficiently.
You might find it useful to incorporate questions from our Laravel Developer Online Test that focus on Eloquent ORM, to efficiently evaluate this skill.
Delving into specifics with a practical question can help illuminate a candidate’s depth of knowledge in Eloquent ORM.
How would you retrieve all records of a 'User' model where the 'age' field is greater than 25 using Eloquent ORM?
The candidate should demonstrate proficiency in using Eloquent methods. Effective answers should show fluent use of the ORM's query-building methods to achieve concise and readable database queries.
Tips for Effective Laravel Interview Questions
Before putting your newfound knowledge into action, here are some tips to make your Laravel interviews more effective.
1. Start with a Skills Assessment
Begin your hiring process with a Laravel skills assessment. This approach helps you quickly identify candidates with the right technical abilities.
Consider using a Laravel developer test or a PHP Laravel test to evaluate core competencies. These assessments can gauge a candidate's proficiency in Laravel fundamentals, PHP, and related technologies.
By using skills tests, you can create a shortlist of qualified candidates more efficiently. This allows you to focus your interviews on the most promising applicants, saving time and resources in the long run.
2. Curate a Balanced Set of Interview Questions
When preparing for the interview, select a mix of questions that cover various aspects of Laravel development. Aim for a balance between technical knowledge, problem-solving skills, and practical experience.
Consider including questions about related technologies such as JavaScript or SQL. This will help you assess the candidate's overall web development skills.
Remember to include questions that evaluate soft skills like communication and teamwork. These are equally important for a developer's success in a team environment.
3. Ask Thoughtful Follow-up Questions
Don't just stick to a script; be prepared to ask follow-up questions based on the candidate's responses. This approach helps you gauge the depth of their knowledge and problem-solving abilities.
For example, if you ask about Laravel's Eloquent ORM, a good follow-up might be to ask how they would optimize database queries for a large-scale application. This helps you understand their practical experience and ability to apply Laravel concepts in real-world scenarios.
Leverage Skill Tests to Identify and Hire Top Laravel Developers
When seeking to hire developers with specific skills such as Laravel, it's important to verify their expertise accurately. The most straightforward way to assess these skills is through targeted skill tests. Consider using Adaface's Laravel Developer Online Test or our PHP Laravel Test to ensure candidates meet your requirements.
After administering the appropriate skill tests, you can efficiently shortlist the top candidates for interviews. To further streamline your hiring process, sign up on the Adaface Dashboard and explore our diverse range of Laravel and other technical assessments to secure the best talent for your team.
Laravel Online Test
Download Laravel interview questions template in multiple formats
Laravel Interview Questions FAQs
Focus on fundamental concepts like routing, controllers, blade templates, and Eloquent ORM.
Use situational and coding questions to understand their approach to solving real-world problems.
Ask advanced questions on database optimization, custom package development, and performance tuning.
Technical definitions show a candidate's understanding of core concepts, which is crucial, especially for junior roles.
Yes, skill tests are effective in evaluating practical coding skills before the actual interview.
Ask questions about handling tight deadlines, large-scale projects, and debugging complex issues successfully.
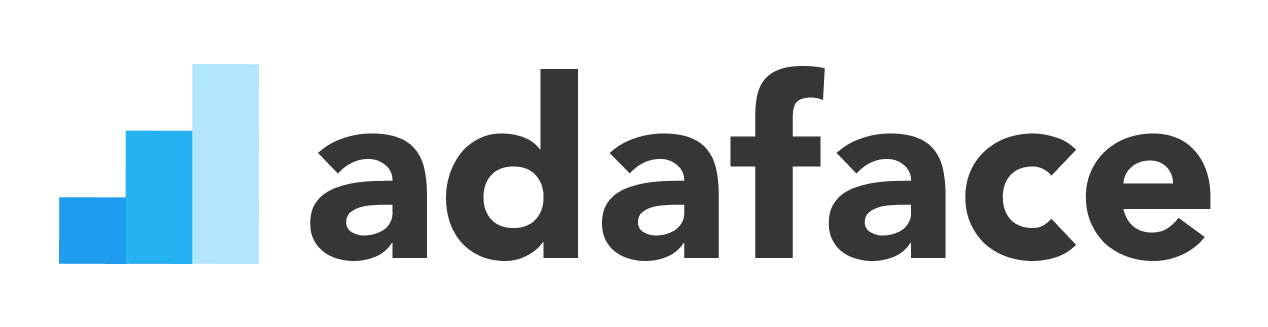
40 min skill tests.
No trick questions.
Accurate shortlisting.
We make it easy for you to find the best candidates in your pipeline with a 40 min skills test.
Try for freeRelated posts
Free resources
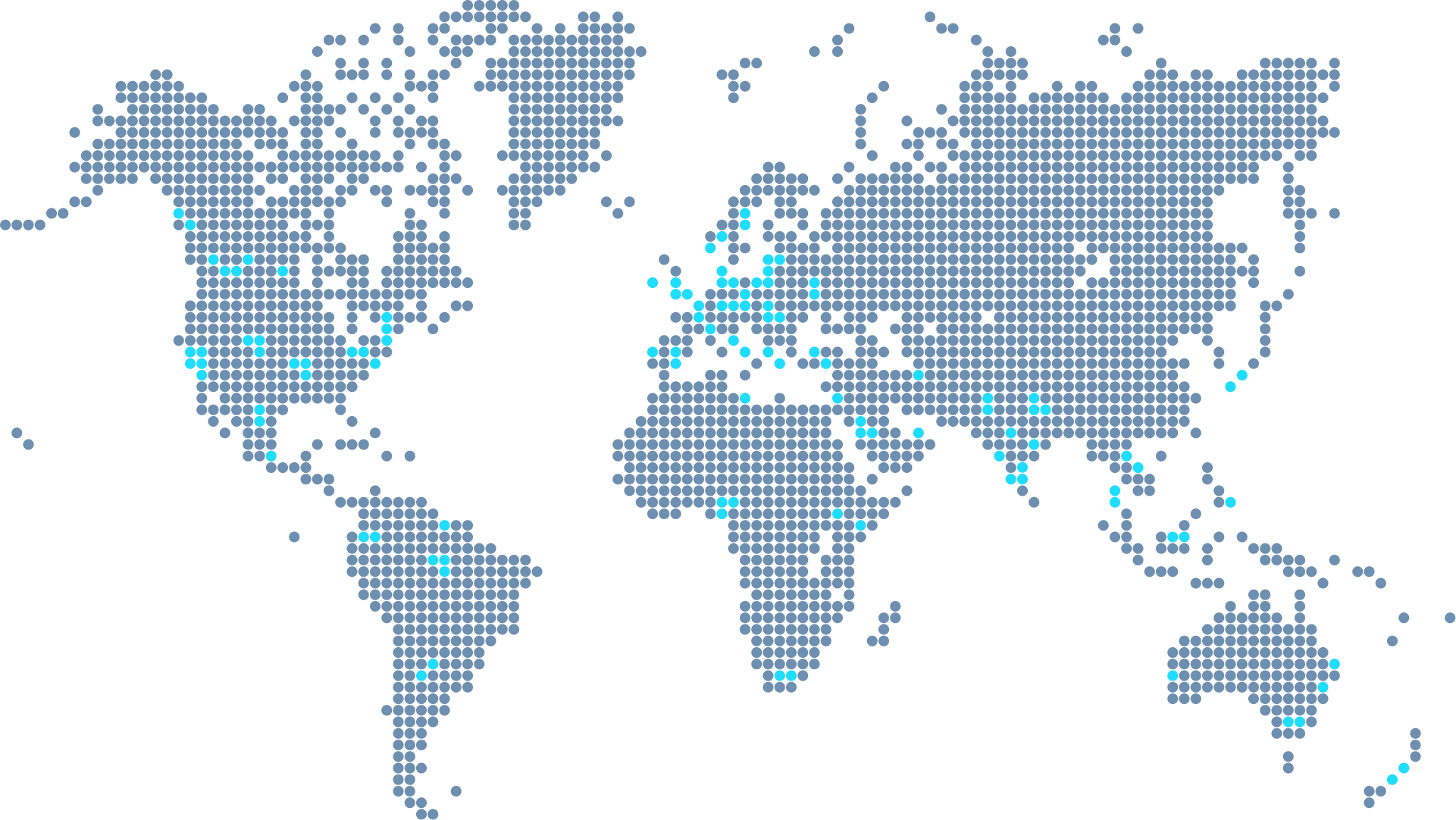
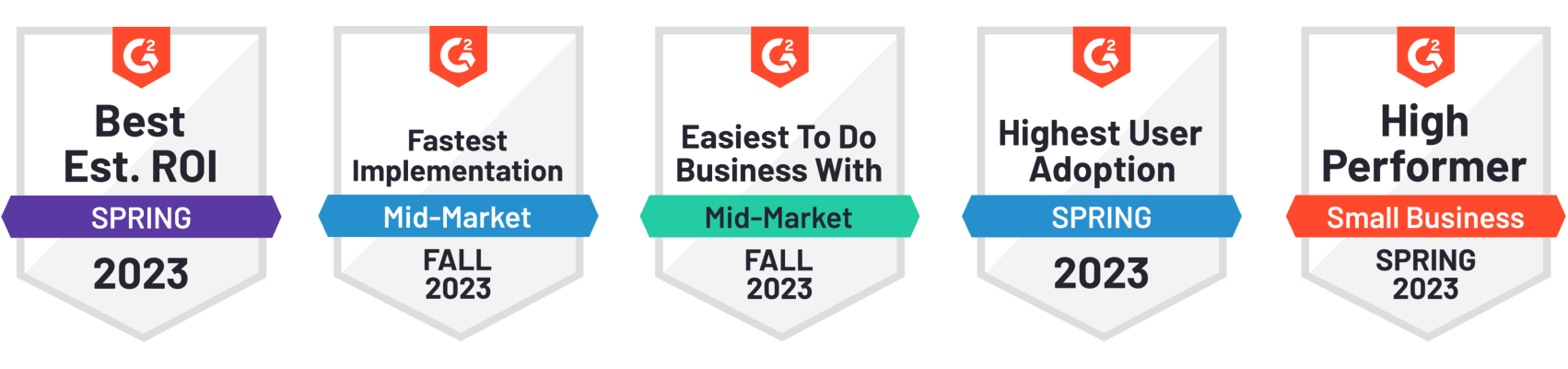