When hiring a Kotlin developer, pinpointing the right candidate can be challenging. Using strategic interview questions can help evaluate their knowledge and fit for your team, similar to understanding the skills required for Android developers.
This blog post provides a list of Kotlin interview questions tailored for different experience levels, from junior to mid-tier developers to specialists in coroutines and functional programming. Each section includes curated questions and sample answers designed to identify top talent for your roles.
By leveraging these questions, you can streamline your interview process and improve your hiring outcomes. Additionally, consider using our Kotlin online test before interviews to further assess candidates’ skills.
Table of contents
10 common Kotlin interview questions to ask your applicants
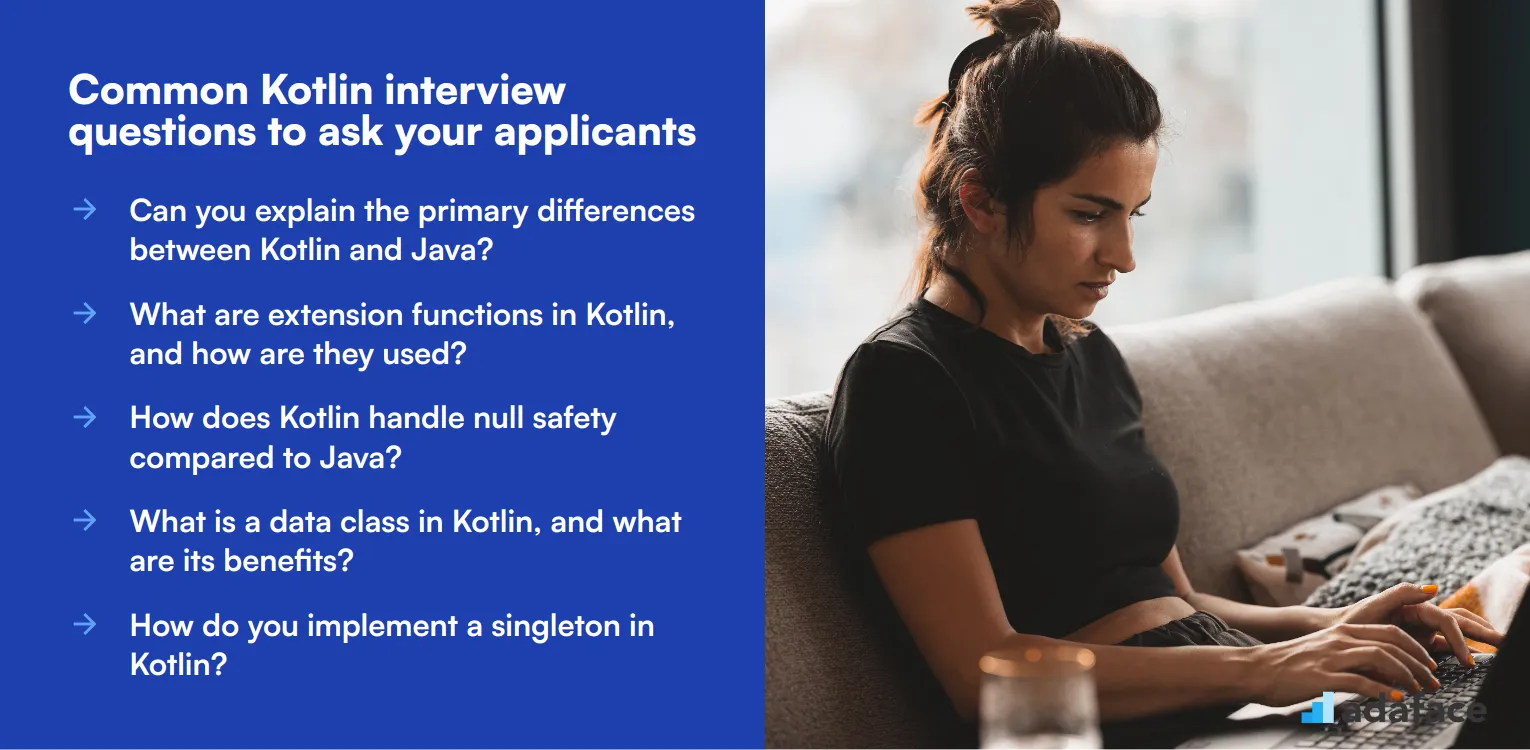
To assess whether your applicants possess the necessary skills for a Kotlin developer role, ask them some of these common Kotlin interview questions. This list is designed to help you evaluate both their technical knowledge and practical experience effectively. For a detailed overview of the required skills, you can refer to this Kotlin developer job description.
- Can you explain the primary differences between Kotlin and Java?
- What are extension functions in Kotlin, and how are they used?
- How does Kotlin handle null safety compared to Java?
- What is a data class in Kotlin, and what are its benefits?
- How do you implement a singleton in Kotlin?
- Explain the use of coroutines in Kotlin for asynchronous programming.
- Can you discuss the advantages of using Kotlin over other JVM languages?
- How would you manage state in a Kotlin-based Android application?
- What are sealed classes, and where would you use them?
- Can you describe the difference between 'val' and 'var' in Kotlin?
8 Kotlin interview questions and answers to evaluate junior developers
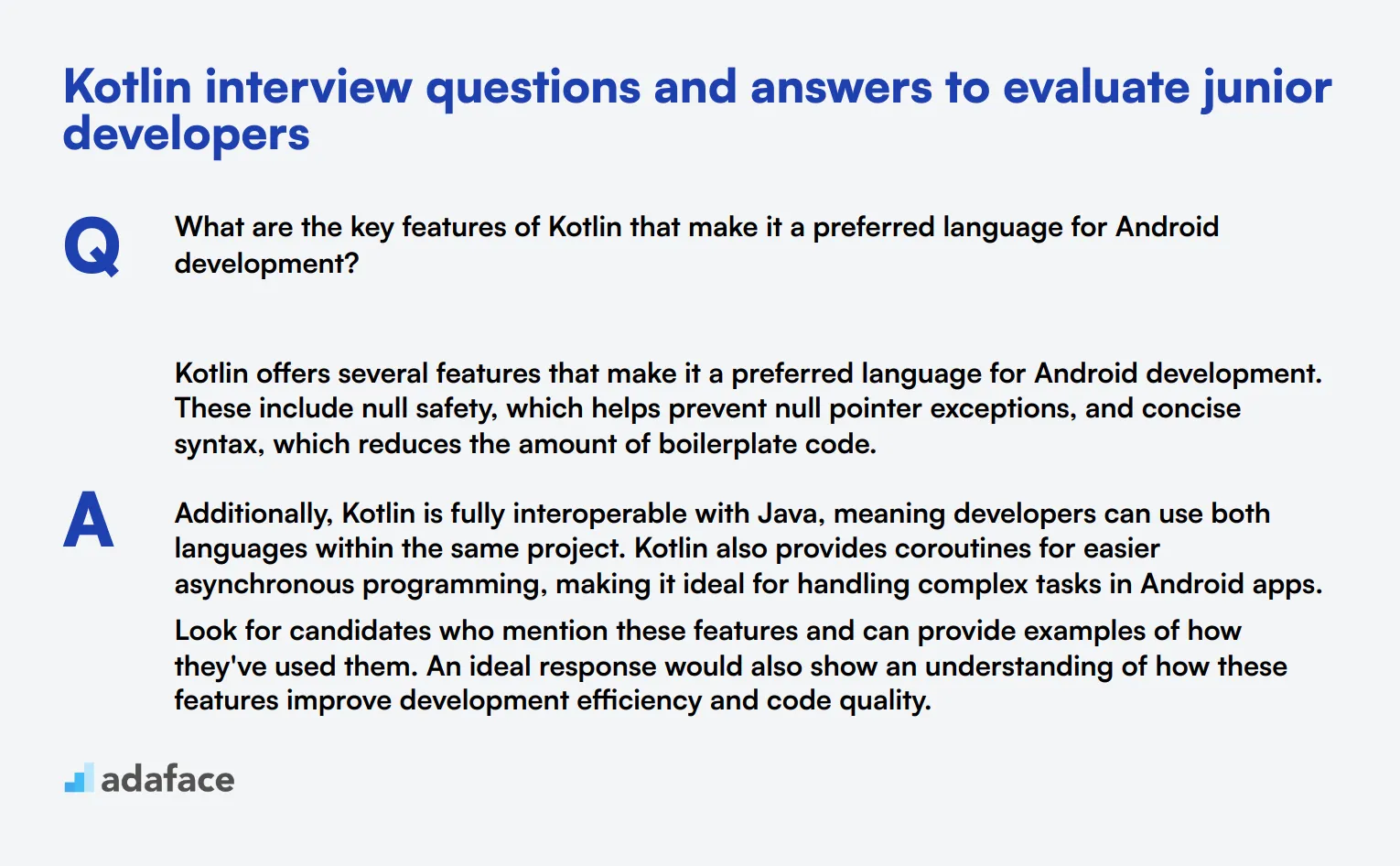
To assess the Kotlin knowledge of your junior developer candidates, these interview questions will help you evaluate their understanding of basic concepts and principles. Use this list during your interviews to identify candidates who have a solid grasp of Kotlin and its applications in real-world scenarios.
1. What are the key features of Kotlin that make it a preferred language for Android development?
Kotlin offers several features that make it a preferred language for Android development. These include null safety, which helps prevent null pointer exceptions, and concise syntax, which reduces the amount of boilerplate code.
Additionally, Kotlin is fully interoperable with Java, meaning developers can use both languages within the same project. Kotlin also provides coroutines for easier asynchronous programming, making it ideal for handling complex tasks in Android apps.
Look for candidates who mention these features and can provide examples of how they've used them. An ideal response would also show an understanding of how these features improve development efficiency and code quality.
2. How does Kotlin's type inference improve code readability?
Kotlin's type inference allows the compiler to automatically deduce the type of a variable without explicitly stating it, which leads to cleaner and more readable code. This reduces redundancy and makes the code easier to maintain.
For example, instead of writing val age: Int = 25
, you can simply write val age = 25
, and Kotlin will infer that the type is Int
. This feature minimizes the verbosity often seen in other languages like Java.
Strong candidates will highlight how type inference contributes to both code simplicity and developer productivity. They should also be able to articulate situations where explicit type declarations might still be necessary.
3. Can you explain what smart casts are and how they are used in Kotlin?
Smart casts in Kotlin automatically handle type casting when the compiler can guarantee the type. This eliminates the need for explicit type casts. For example, if you check that a variable is of a certain type, Kotlin will automatically cast it for you within that scope.
This functionality simplifies the code and reduces the risk of casting errors. It enhances both readability and safety by ensuring that types are correctly handled without additional casting syntax.
An ideal candidate will explain smart casts with examples and discuss how they improve code safety and readability. Look for candidates who can also differentiate between smart casts and traditional type casting.
4. What is the difference between `open` and `final` keywords in Kotlin?
In Kotlin, all classes and methods are final
by default, meaning they cannot be inherited unless explicitly declared otherwise. The open
keyword allows classes and methods to be inherited.
This approach encourages more deliberate and controlled use of inheritance, which can improve code stability and reduce potential errors from unintended overrides.
Candidates should demonstrate an understanding of how the open
and final
keywords impact class design and inheritance. Look for responses that emphasize the importance of controlled inheritance in maintaining code quality.
5. How do you handle exceptions in Kotlin?
In Kotlin, exceptions are handled similar to other languages using try
, catch
, and finally
blocks. However, Kotlin does not have checked exceptions, which means it doesn't force you to catch or declare exceptions.
This can lead to cleaner code as developers are not required to handle exceptions they don't intend to. Instead, they can focus on catching only those exceptions that are relevant to their application's logic.
An ideal candidate will mention how this approach simplifies the code and discuss best practices for exception handling, such as catching only specific exceptions and providing meaningful error messages.
6. What are higher-order functions in Kotlin and why are they useful?
Higher-order functions are functions that take other functions as parameters or return a function. This feature allows for more flexible and reusable code, enabling developers to pass behavior rather than data.
For example, higher-order functions can be used to implement callbacks, event listeners, or custom sorting logic. They promote functional programming practices and can lead to cleaner, more maintainable code.
Look for candidates who can describe real-world scenarios where they've used higher-order functions and can explain the benefits of this approach in terms of code reusability and modularity.
7. What is destructuring in Kotlin and how is it useful?
Destructuring in Kotlin allows you to unpack an object into multiple variables. This is particularly useful for handling data classes or pairs, where you can assign each component to a separate variable in a single statement.
For example, you can destructure a data class representing a point into its x and y coordinates directly. This makes the code more readable and concise, especially when dealing with complex data structures.
Candidates should provide examples of how destructuring simplifies their code and improves readability. Look for explanations that demonstrate a clear understanding of how and when to use this feature effectively.
8. Can you explain the concept of lazy initialization in Kotlin?
Lazy initialization in Kotlin is a technique where the initialization of a variable is deferred until it is first accessed. This is done using the lazy
function, which takes a lambda and initializes the variable only when needed.
This approach can improve performance by avoiding unnecessary computations and memory usage. For example, you might use lazy initialization for a resource-intensive object that is not always needed.
An ideal candidate will explain both the benefits and potential pitfalls of lazy initialization, such as thread-safety concerns. They should also provide examples of when this technique is particularly useful.
15 intermediate Kotlin interview questions and answers to ask mid-tier developers
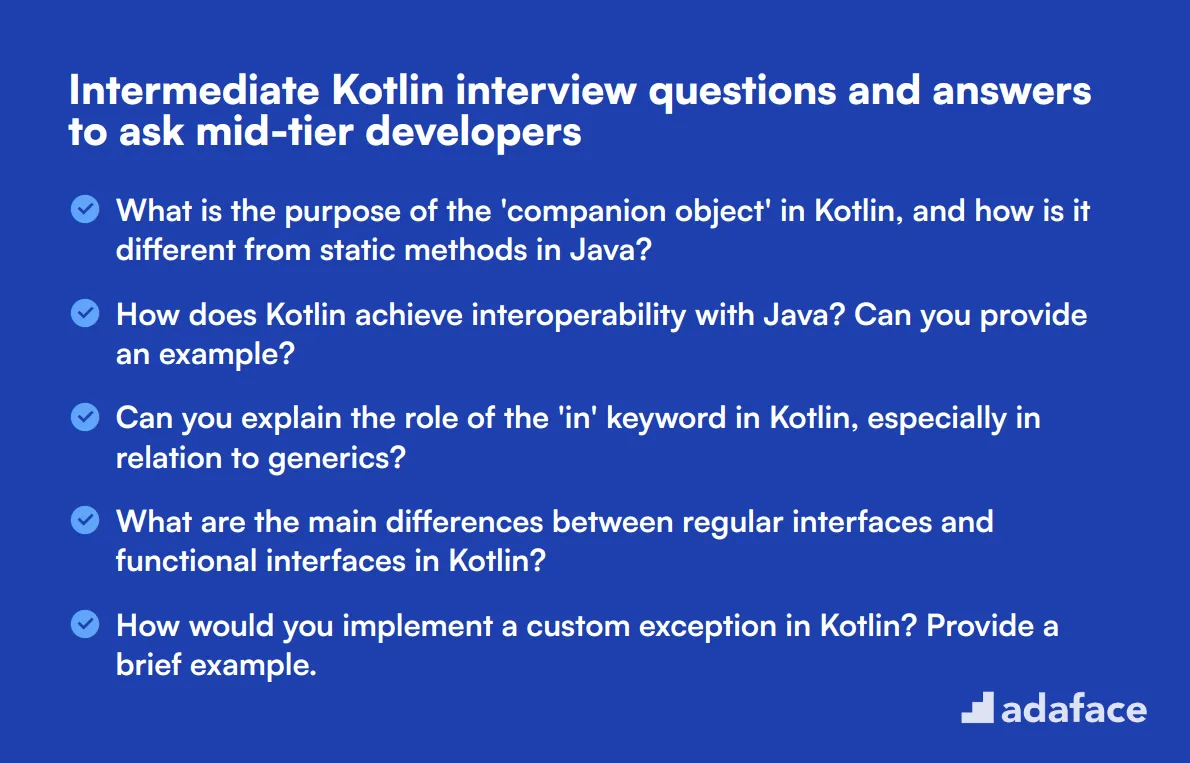
To assess whether mid-tier developers possess a solid grasp of Kotlin concepts, use these intermediate interview questions. They can help you gauge the technical skills and practical knowledge necessary for a successful Kotlin developer role, as outlined in our Kotlin developer job description.
- What is the purpose of the 'companion object' in Kotlin, and how is it different from static methods in Java?
- How does Kotlin achieve interoperability with Java? Can you provide an example?
- Can you explain the role of the 'in' keyword in Kotlin, especially in relation to generics?
- What are the main differences between regular interfaces and functional interfaces in Kotlin?
- How would you implement a custom exception in Kotlin? Provide a brief example.
- What is the significance of the 'lateinit' modifier in Kotlin, and when should it be used?
- Can you describe the process of creating a DSL (Domain Specific Language) in Kotlin?
- What are inline functions in Kotlin, and how do they enhance performance?
- How do you perform dependency injection in a Kotlin application?
- Can you explain the concept of type aliases in Kotlin and when you might use them?
- What is the difference between a suspend function and a regular function in Kotlin?
- How do you use the 'apply' and 'with' functions in Kotlin? Can you provide examples?
- What is the purpose of the 'by' keyword in Kotlin, especially in delegation?
- How do you manage collections in Kotlin? What are the key collection types?
- Can you explain the use of 'object expressions' and 'object declarations' in Kotlin?
7 Kotlin interview questions and answers related to coroutines
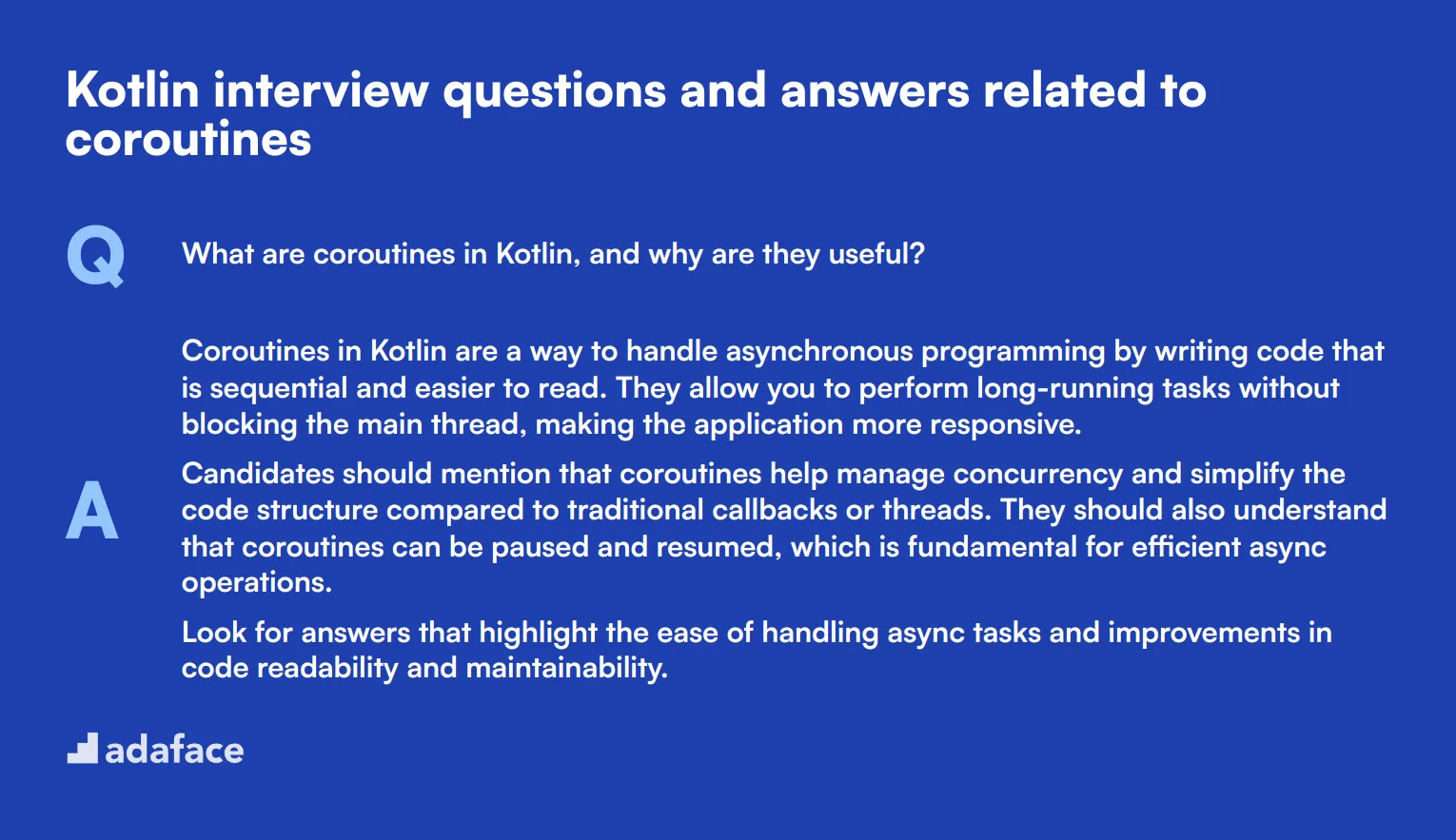
To assess whether your candidates have the right knowledge and understanding of Kotlin coroutines, use these 7 interview questions. They will help you gauge their grasp on handling asynchronous programming and concurrency in Kotlin.
1. What are coroutines in Kotlin, and why are they useful?
Coroutines in Kotlin are a way to handle asynchronous programming by writing code that is sequential and easier to read. They allow you to perform long-running tasks without blocking the main thread, making the application more responsive.
Candidates should mention that coroutines help manage concurrency and simplify the code structure compared to traditional callbacks or threads. They should also understand that coroutines can be paused and resumed, which is fundamental for efficient async operations.
Look for answers that highlight the ease of handling async tasks and improvements in code readability and maintainability.
2. How does a coroutine differ from a traditional thread?
Coroutines are lightweight and more efficient compared to traditional threads. While threads are managed by the operating system, coroutines are managed by the Kotlin runtime, allowing for thousands of coroutines to run concurrently without a significant overhead.
Candidates should explain that coroutines don't block the thread they run on but rather suspend execution, freeing up the thread for other tasks. This makes coroutines more suitable for applications that require a large number of concurrent tasks.
Look for an understanding of the efficiency and scalability benefits of coroutines over traditional threads.
3. What is the role of 'suspend' keyword in Kotlin coroutines?
The 'suspend' keyword in Kotlin marks a function or lambda as suspendable, meaning it can be paused and resumed at a later time. This allows the function to execute long-running operations without blocking the main thread.
Candidates should explain that suspend functions can only be called from other suspend functions or coroutine scopes, and they are the building blocks of coroutine-based async programming in Kotlin.
Look for an understanding of how suspend functions contribute to non-blocking, efficient asynchronous operations.
4. Can you explain the difference between 'launch' and 'async' in Kotlin coroutines?
The 'launch' function in Kotlin coroutines is used to start a new coroutine that performs work and doesn't return a result. It's mainly used for tasks that run independently and whose results are not needed by the caller.
On the other hand, 'async' is used to start a coroutine that performs work and returns a result encapsulated in a Deferred object. You can then use the 'await' method to get the result of the async coroutine.
Look for explanations that highlight the use-cases for each function and an understanding of how they differ in terms of result handling.
5. What is a coroutine scope and why is it important?
A coroutine scope defines the lifecycle of coroutines. It ensures that coroutines are launched within a boundary and allows for structured concurrency, meaning that all coroutines are completed before the scope itself completes.
Candidates should mention that coroutine scopes help in managing coroutines efficiently and prevent memory leaks by automatically cancelling coroutines when the scope is cancelled.
Look for an understanding of the significance of coroutine scope in managing the lifecycle and structure of coroutines effectively.
6. How can you handle exceptions in Kotlin coroutines?
Exceptions in Kotlin coroutines can be handled using standard try-catch blocks, similar to synchronous code. Additionally, you can use coroutine-specific mechanisms like CoroutineExceptionHandler to handle uncaught exceptions.
Candidates should understand that structured concurrency in coroutines allows for better error handling and recovery strategies. They should also mention that the parent coroutine scope can manage and handle exceptions from child coroutines.
Look for detailed explanations of different methods for handling exceptions and an understanding of their implications in coroutine-based applications.
7. What is 'structured concurrency' in the context of Kotlin coroutines?
Structured concurrency in Kotlin coroutines refers to the practice of organizing coroutines in a structured, hierarchical manner. It ensures that child coroutines are properly managed by their parent scopes, making the code more predictable and easier to maintain.
Candidates should mention that structured concurrency helps in controlling the lifecycle of coroutines, ensuring that all child coroutines are completed or cancelled when the parent scope completes or is cancelled.
Look for an understanding of how structured concurrency improves code reliability and helps in preventing resource leaks in coroutine-based programs.
12 Kotlin interview questions about functional programming
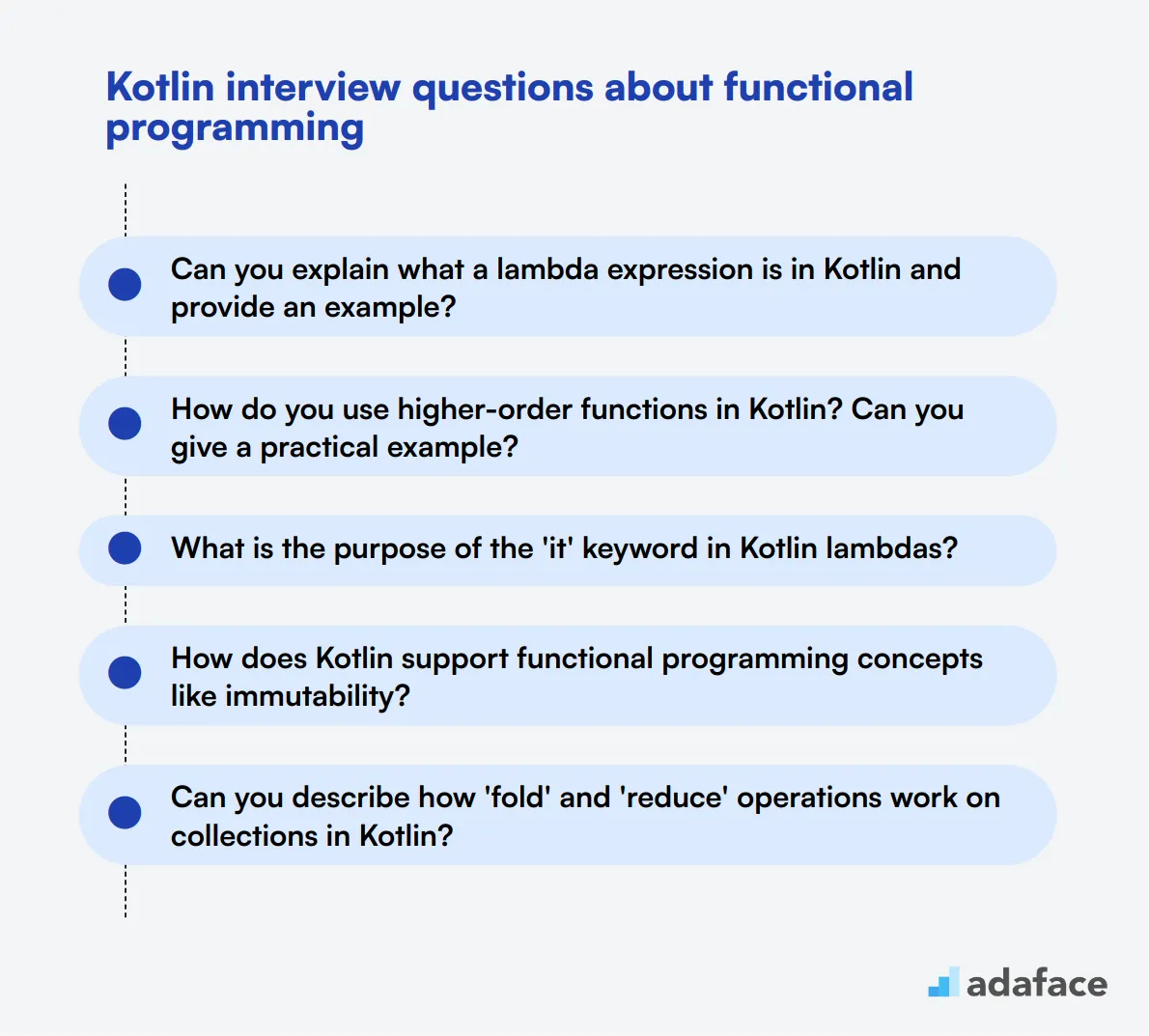
To evaluate whether your candidates are proficient in functional programming using Kotlin, utilize some of these 12 targeted interview questions. These questions will help you identify if they have the right skills to handle complex tasks, similar to those described in Kotlin developer job descriptions.
- Can you explain what a lambda expression is in Kotlin and provide an example?
- How do you use higher-order functions in Kotlin? Can you give a practical example?
- What is the purpose of the 'it' keyword in Kotlin lambdas?
- How does Kotlin support functional programming concepts like immutability?
- Can you describe how 'fold' and 'reduce' operations work on collections in Kotlin?
- What are inline functions in Kotlin and why are they beneficial in functional programming?
- Explain the concept of tail recursion in Kotlin and how it optimizes recursive function calls.
- How does Kotlin's 'let' function work and when would you use it?
- What is the difference between 'map' and 'flatMap' in Kotlin?
- Can you explain the use of 'filter' and 'filterNot' functions in Kotlin?
- How would you use 'associateBy' and 'groupBy' functions in Kotlin for transforming collections?
- What are anonymous functions in Kotlin and how do they differ from lambda expressions?
Which Kotlin skills should you evaluate during the interview phase?
While it's impossible to assess every aspect of a candidate's Kotlin proficiency in a single interview, focusing on key skills can provide valuable insights. The following core competencies are particularly important when evaluating Kotlin developers.
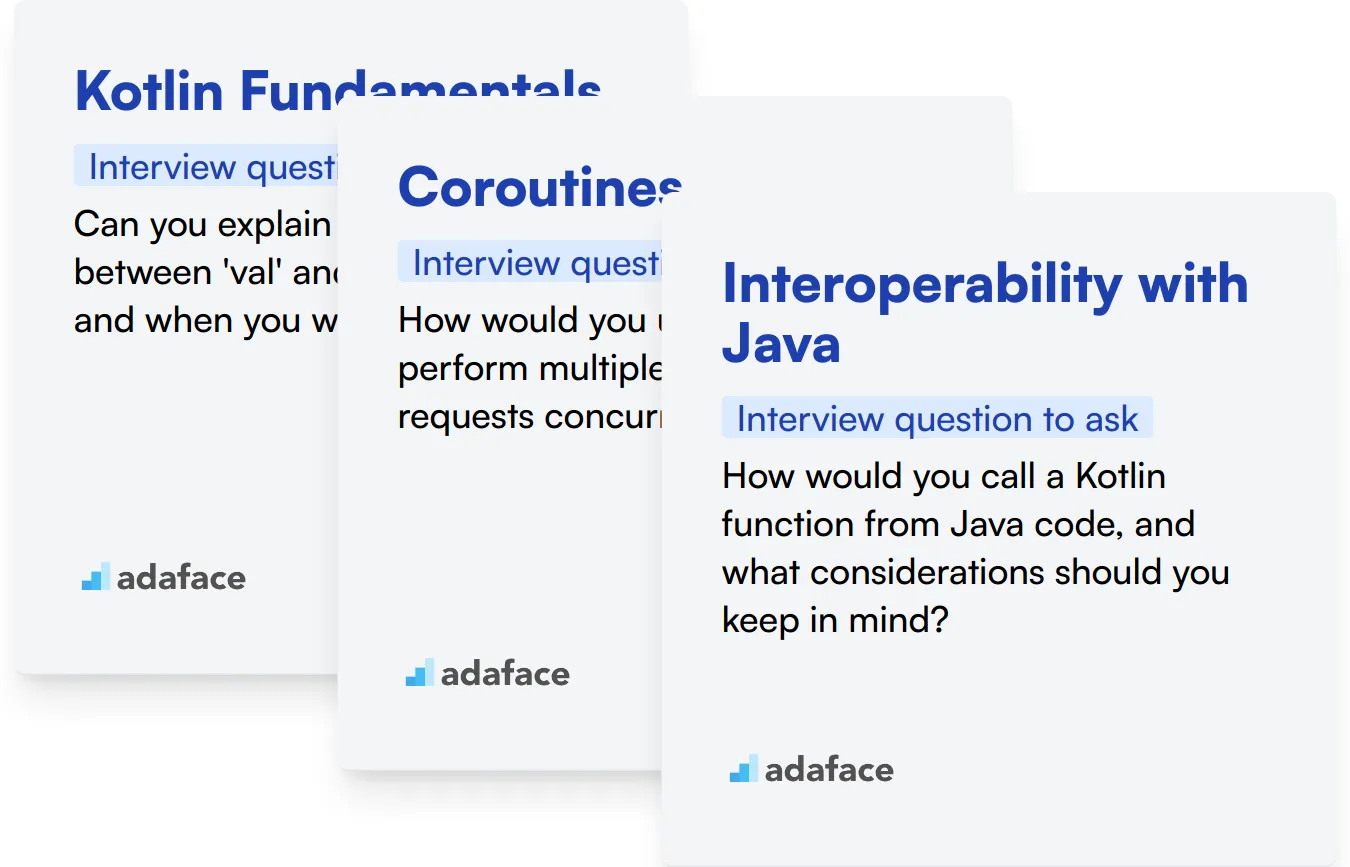
Kotlin Fundamentals
A strong grasp of Kotlin fundamentals is essential for any developer working with the language. This includes understanding Kotlin's syntax, null safety features, and functional programming concepts.
To evaluate this skill efficiently, consider using an assessment test with relevant multiple-choice questions. This can help filter candidates based on their basic Kotlin knowledge.
During the interview, you can ask targeted questions to gauge the candidate's understanding of Kotlin basics. Here's an example question:
Can you explain the difference between 'val' and 'var' in Kotlin, and when you would use each?
Look for answers that demonstrate understanding of immutability ('val' for read-only variables) and mutability ('var' for mutable variables). A good response should also include scenarios where each would be appropriate.
Coroutines
Coroutines are a key feature in Kotlin for managing asynchronous programming. Understanding how to use coroutines effectively is crucial for developing efficient Kotlin applications.
To assess a candidate's knowledge of coroutines, consider asking a question like this:
How would you use coroutines to perform multiple network requests concurrently?
Look for answers that mention using launch or async functions within a coroutine scope. The candidate should also discuss how to handle exceptions and cancellations in coroutines.
Interoperability with Java
Kotlin's interoperability with Java is one of its strongest features. Developers should be able to work with both Kotlin and Java code in the same project seamlessly.
To evaluate this skill, you might ask:
How would you call a Kotlin function from Java code, and what considerations should you keep in mind?
Look for answers that mention using the @JvmStatic annotation for static methods, handling nullable types correctly, and understanding how Kotlin's properties are translated to Java getters and setters.
3 tips for using Kotlin interview questions
Before you start putting what you've learned to use, here are our tips to maximize the effectiveness of your Kotlin interview questions.
1. Incorporate skills tests before interviews
One effective way to streamline your interview process is to incorporate skills tests before conducting interviews. Skill tests can help you filter out candidates who may not have the required proficiency, saving you time and effort.
Consider using our Kotlin online test to evaluate the candidates' coding proficiency. This test assesses their understanding of Kotlin syntax, data structures, and problem-solving skills.
Using skill tests before interviews ensures that only capable candidates move forward in the hiring process, allowing for a more focused and productive interview. This approach helps in obtaining a clear picture of candidates' abilities before investing time in face-to-face interactions.
2. Compile a concise list of interview questions
Given the limited time during interviews, it's crucial to compile a concise list of relevant questions. Focus on questions that assess both technical skills and problem-solving capabilities.
For a well-rounded evaluation, consider including questions related to other relevant skills such as Java and Android development, depending on the role requirements.
Such an approach allows you to evaluate candidates on multiple fronts, leading to a more comprehensive assessment of their suitability for the role.
3. Ask follow-up questions during the interview
Using pre-determined questions alone won't be enough to gauge a candidate’s depth of knowledge. It's important to ask follow-up questions to explore their understanding and problem-solving process further.
For instance, if you ask a candidate about Kotlin coroutines, follow up with a question like, 'Can you describe a situation where coroutines would be particularly advantageous?' This helps you understand the candidate's practical experience and how they apply their knowledge in real-world scenarios.
Using Kotlin Interview Questions and Skills Tests to Secure Top Talent
When aiming to hire professionals adept in Kotlin, it's important to verify their proficiency accurately. The most straightforward way to assess these skills is through tailored skill tests. Explore our Kotlin Online Test to ensure that candidates meet your requirements.
After implementing these tests, you can effectively shortlist the top candidates for interviews. To move forward with finding the right fit for your team, consider signing up on our platform or exploring our various assessment options. Get started by visiting our signup page or our test library.
Kotlin Online Test
Download Kotlin interview questions template in multiple formats
Kotlin Interview Questions FAQs
Kotlin is often preferred due to its concise syntax, improved type safety, and seamless interoperability with Java.
Kotlin coroutines are a way to simplify asynchronous programming by allowing users to write code in a sequential style.
Functional programming in Kotlin allows for cleaner and more maintainable code by using features like lambdas, higher-order functions, and immutability.
Evaluate a junior Kotlin developer by assessing their understanding of basic concepts, their problem-solving skills, and their ability to write clean and readable code.
Common mistakes include improper handling of nullability, overlooking coroutine best practices, and failing to leverage Kotlin's concise syntax and features.
Prepare by understanding the key concepts of Kotlin, creating a structured set of questions, and knowing the ideal answers to assess the candidate's proficiency.
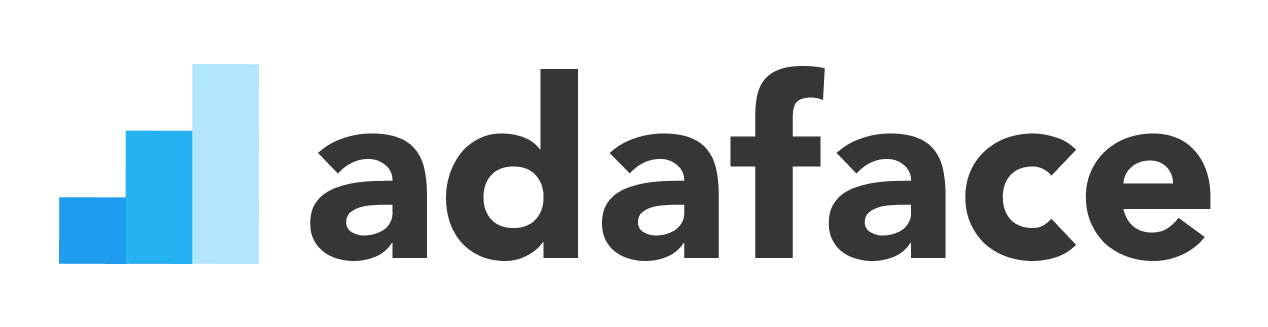
40 min skill tests.
No trick questions.
Accurate shortlisting.
We make it easy for you to find the best candidates in your pipeline with a 40 min skills test.
Try for freeRelated posts
Free resources
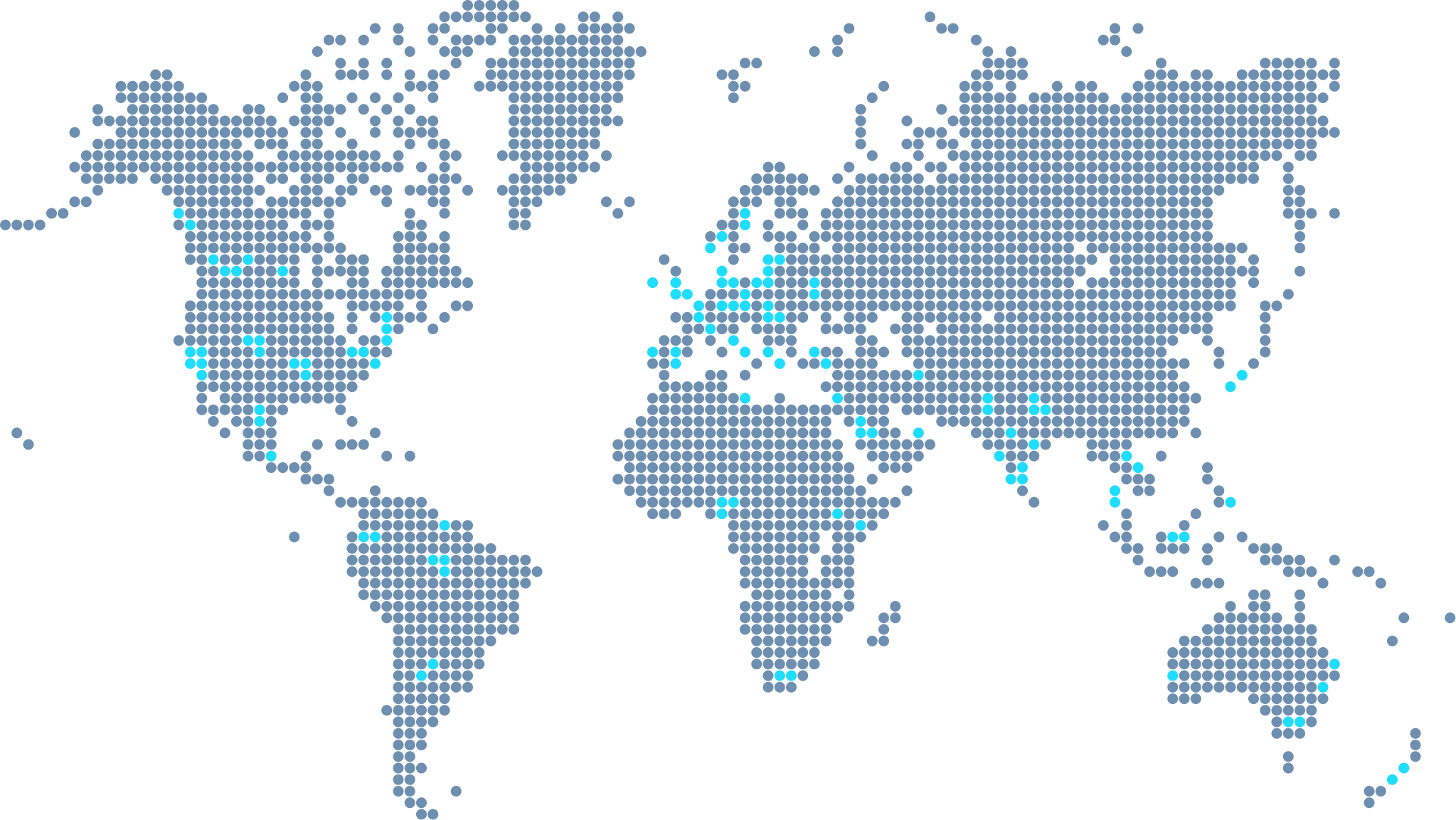
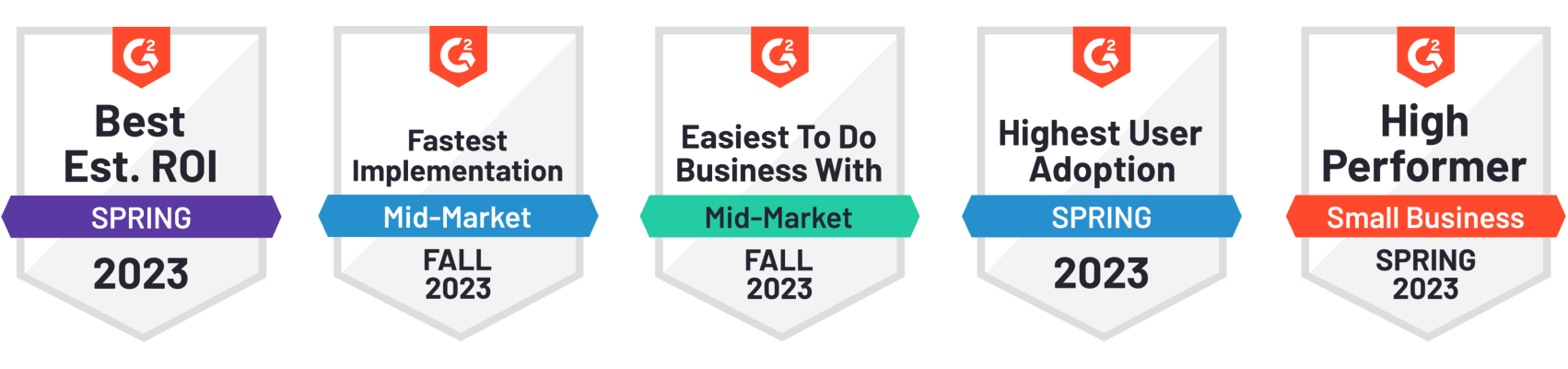