When interviewing candidates for a jQuery developer role, it's important to ask the right questions to assess their skills and experience. This ensures you select the best fit for your team and avoid the pitfalls of a bad hire.
In this blog post, we provide a comprehensive list of jQuery interview questions. These questions are categorized to help you evaluate applicants from basic to advanced levels, including specific aspects like DOM manipulation and event handling.
Using these questions, you can accurately gauge a candidate's expertise and suitability for your projects. Additionally, consider a pre-interview evaluation with our jQuery online test to streamline your hiring process.
Table of contents
15 basic jQuery interview questions and answers to assess applicants
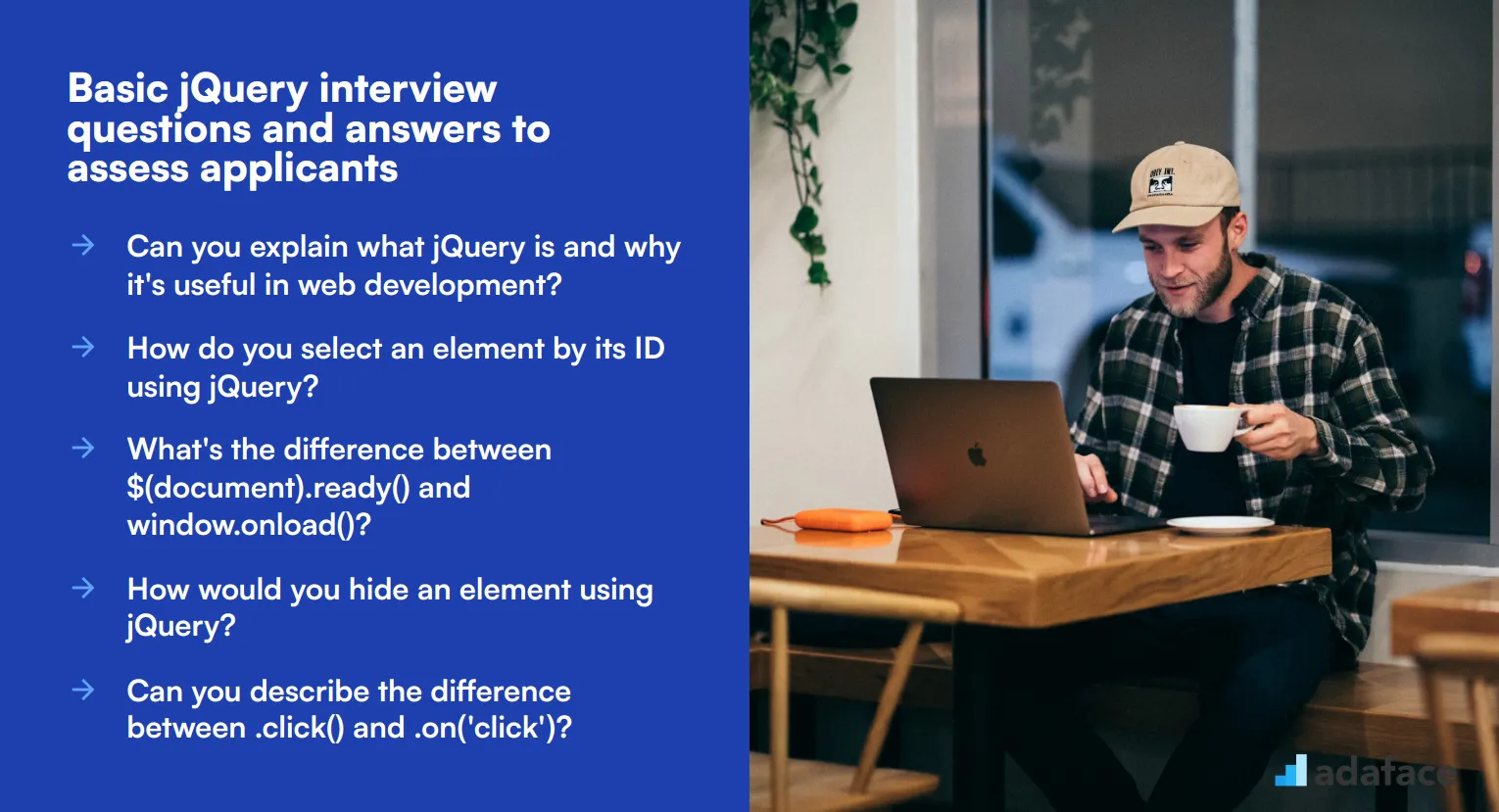
To assess a candidate's foundational knowledge of jQuery, use these 15 basic interview questions. They're designed to help you evaluate an applicant's understanding of core jQuery concepts and their ability to apply them in real-world scenarios. These questions are particularly useful when interviewing for entry-level to mid-level front-end development positions.
- Can you explain what jQuery is and why it's useful in web development?
- How do you select an element by its ID using jQuery?
- What's the difference between $(document).ready() and window.onload()?
- How would you hide an element using jQuery?
- Can you describe the difference between .click() and .on('click')?
- How do you perform an AJAX GET request using jQuery?
- What's the purpose of the .each() method in jQuery?
- How would you add a new element to the DOM using jQuery?
- Can you explain what method chaining is in jQuery?
- How do you handle form submission using jQuery?
- What's the difference between .attr() and .prop() methods?
- How would you animate an element's opacity using jQuery?
- Can you describe how event delegation works in jQuery?
- What's the purpose of the .data() method in jQuery?
- How would you select all elements with a specific class except for one particular element?
8 jQuery interview questions and answers to evaluate junior developers
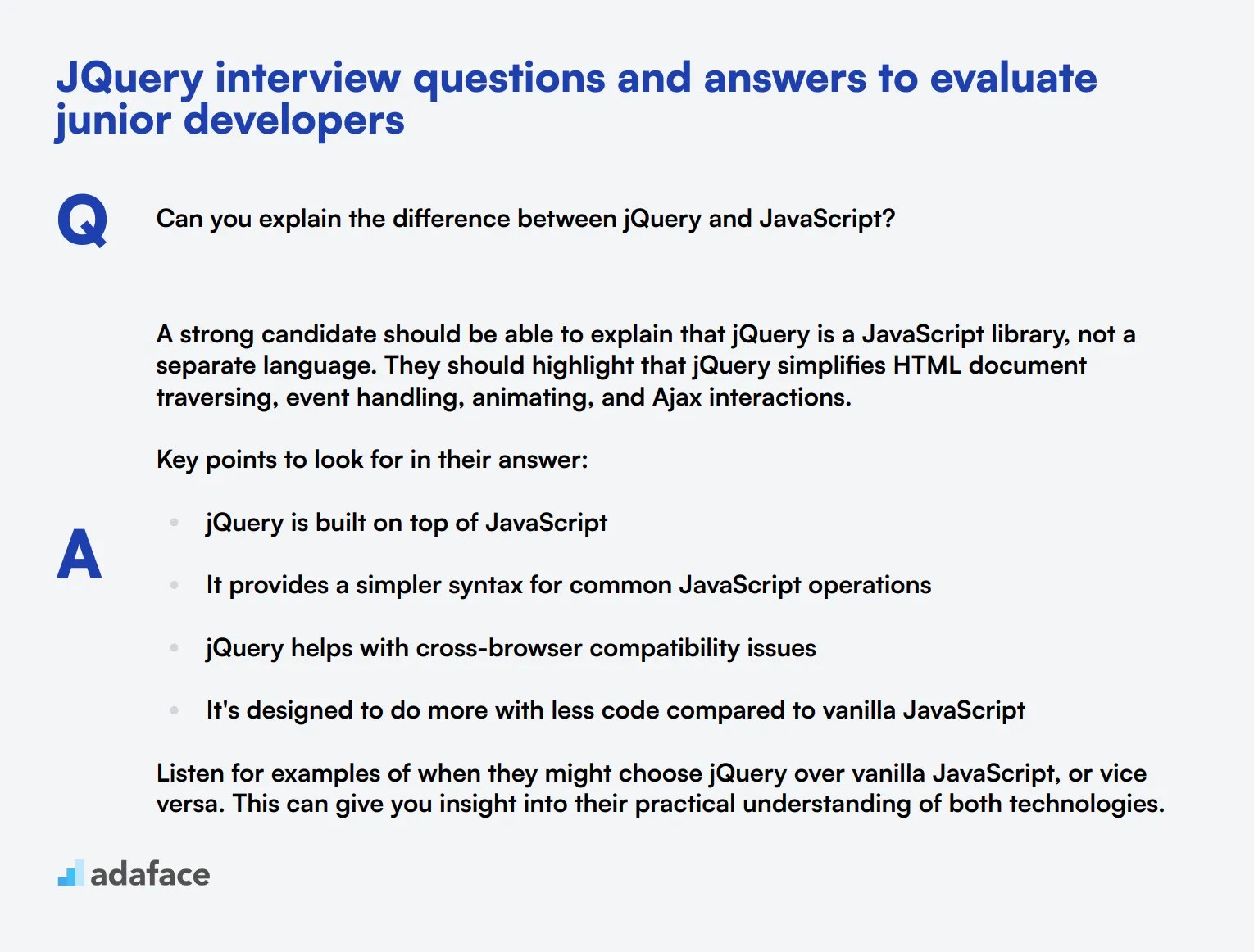
When interviewing junior developers for jQuery positions, it's crucial to assess their foundational knowledge and problem-solving skills. These 8 questions will help you evaluate candidates' understanding of jQuery basics and their ability to apply them in real-world scenarios. Use them to gauge both technical proficiency and practical thinking in your front-end developer interviews.
1. Can you explain the difference between jQuery and JavaScript?
A strong candidate should be able to explain that jQuery is a JavaScript library, not a separate language. They should highlight that jQuery simplifies HTML document traversing, event handling, animating, and Ajax interactions.
Key points to look for in their answer:
- jQuery is built on top of JavaScript
- It provides a simpler syntax for common JavaScript operations
- jQuery helps with cross-browser compatibility issues
- It's designed to do more with less code compared to vanilla JavaScript
Listen for examples of when they might choose jQuery over vanilla JavaScript, or vice versa. This can give you insight into their practical understanding of both technologies.
2. How would you optimize a jQuery-heavy website for better performance?
A knowledgeable candidate should discuss several optimization strategies:
- Minimizing DOM manipulation by caching jQuery selections
- Using event delegation for better performance with dynamic elements
- Employing jQuery's
.on()
method for event handling
- Considering alternatives like vanilla JavaScript for simple operations
- Minifying and concatenating jQuery and custom scripts
Look for candidates who can explain the reasoning behind these optimizations. They should understand that excessive DOM manipulation and redundant jQuery selections can slow down a website significantly.
An ideal response might also include mention of tools or techniques for performance testing and monitoring, showing a holistic approach to optimization.
3. Describe a situation where you had to debug a jQuery issue. What was your approach?
This question assesses problem-solving skills and real-world experience. A good answer might include:
- Using browser developer tools to inspect elements and check for errors
- Employing console.log() statements to track variable values and function execution
- Isolating the problem by creating a minimal test case
- Checking jQuery documentation for correct usage of methods
- Seeking help from online communities if needed, after thorough personal investigation
Pay attention to the candidate's systematic approach to problem-solving. They should demonstrate logical thinking and persistence in tackling issues.
Look for candidates who also mention preventive measures, such as writing clean, modular code and using version control. This indicates a mature approach to development that goes beyond just fixing immediate problems.
4. How would you handle browser compatibility issues when using jQuery?
A competent candidate should discuss strategies such as:
- Leveraging jQuery's built-in cross-browser compatibility features
- Testing on multiple browsers and versions
- Using feature detection instead of browser detection
- Employing polyfills or fallbacks for unsupported features
- Staying updated with jQuery versions and their browser support
Look for candidates who understand that while jQuery helps with many compatibility issues, it's not a silver bullet. They should be aware of the limitations and know when additional measures are necessary.
An excellent answer might also touch on the importance of progressive enhancement and graceful degradation in web development. This shows a broader understanding of creating robust, accessible web applications.
5. What are some alternatives to jQuery, and in what situations might you choose them?
A well-informed candidate should be able to discuss modern alternatives like:
- Vanilla JavaScript (with explanations of how modern browsers have improved native DOM manipulation)
- React, Vue, or Angular for building complex, interactive UIs
- Smaller, more focused libraries like Cash or Zepto for specific jQuery-like functionality
- CSS for animations and transitions that were previously handled by jQuery
They should explain scenarios where these alternatives might be preferable, such as:
- Projects requiring a full front-end framework (React, Vue, Angular)
- Lightweight websites where vanilla JS is sufficient
- Modern web applications where browser compatibility is less of a concern
Look for candidates who demonstrate an understanding of the evolving front-end landscape and can make informed decisions based on project requirements. This shows adaptability and a commitment to using the best tool for the job.
6. How do you ensure your jQuery code is maintainable and scalable?
A strong candidate should discuss practices like:
- Writing modular, reusable code
- Using meaningful naming conventions for variables and functions
- Implementing proper error handling and logging
- Avoiding global variables and namespace pollution
- Employing design patterns appropriate for jQuery (e.g., module pattern)
- Consistently formatting code and following style guides
They might also mention the importance of documentation, both inline comments and external documentation for larger projects. Version control and code reviews should be part of their workflow.
Look for candidates who understand that maintainability isn't just about writing code, but also about creating an environment where code can be easily understood, modified, and expanded by other developers. This demonstrates a team-oriented mindset crucial for larger projects.
7. Can you explain the concept of event bubbling in jQuery and how you might use it?
A knowledgeable candidate should explain that event bubbling is the process by which an event triggered on a nested element 'bubbles up' through its parent elements in the DOM tree. They should be able to describe how this can be useful in event delegation.
Key points to look for:
- Understanding of the DOM tree structure
- Explanation of how event bubbling can improve performance by reducing the number of event listeners
- Knowledge of how to use jQuery's
.on()
method for event delegation
- Awareness of when to stop event propagation using
.stopPropagation()
An excellent answer might include a practical example, such as handling click events on dynamically added table rows by attaching a single event listener to the table. This demonstrates the ability to apply theoretical knowledge to real-world scenarios.
8. How would you integrate jQuery with a backend API?
A competent candidate should be able to explain the use of jQuery's AJAX methods to interact with a backend API. They should mention:
- Using
$.ajax()
,$.get()
, or$.post()
methods for making HTTP requests
- Handling JSON data returned from the API
- Implementing error handling for failed requests
- Considering security implications, such as CORS issues
Look for candidates who also discuss:
- Structuring API calls in a modular, reusable way
- Using promises or async/await for better handling of asynchronous operations
- Implementing loading indicators for better user experience
- Caching API responses when appropriate to reduce server load
An ideal response might touch on the importance of proper back-end integration and how it affects the overall application architecture. This shows a holistic understanding of web development beyond just front-end concerns.
10 advanced jQuery interview questions to ask senior developers
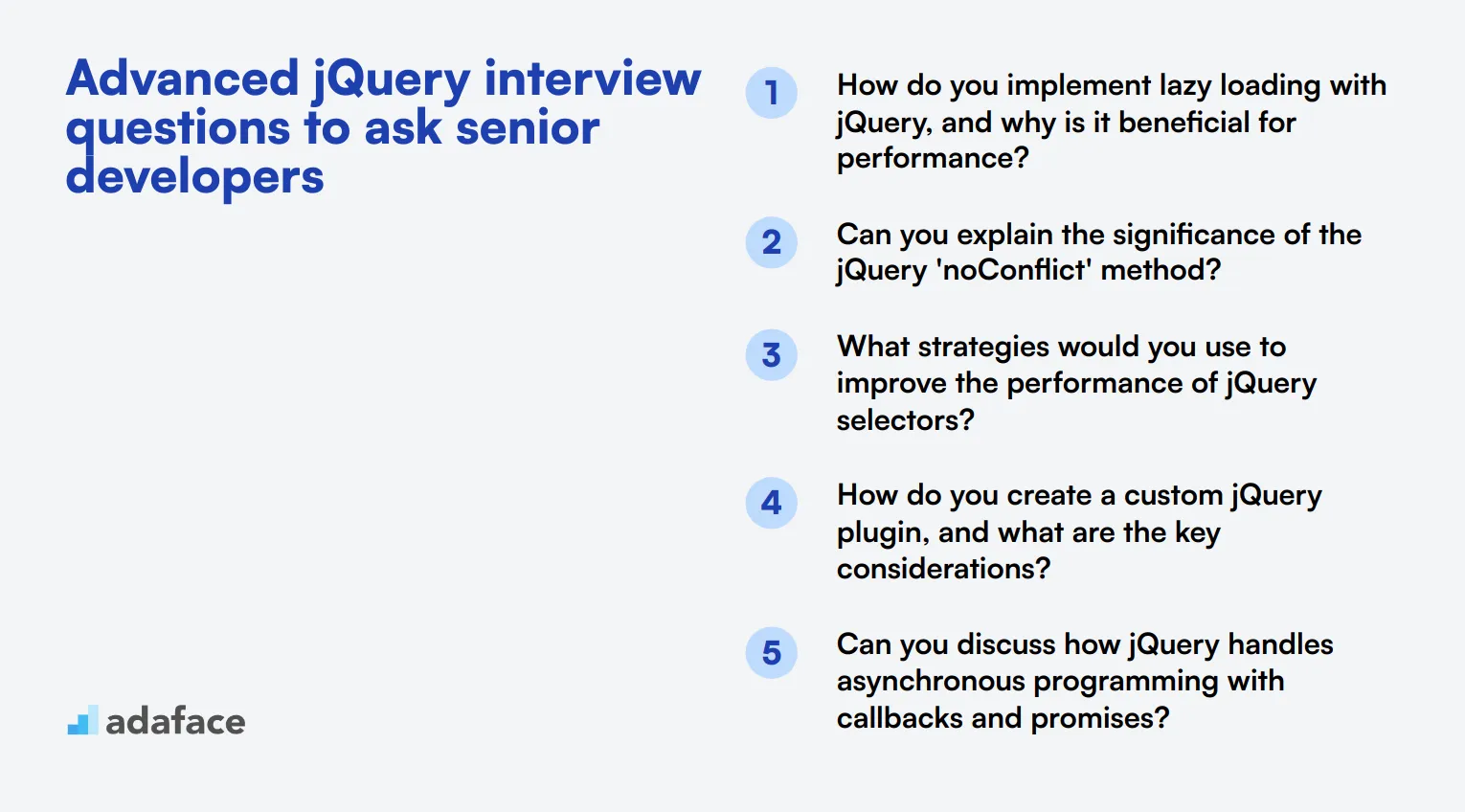
To assess a candidate's advanced jQuery skills, use these thoughtful questions during your interview process. This focused list will help you gauge their technical understanding and practical experience with jQuery, ensuring they are well-prepared for the role of a jQuery developer.
- How do you implement lazy loading with jQuery, and why is it beneficial for performance?
- Can you explain the significance of the jQuery 'noConflict' method?
- What strategies would you use to improve the performance of jQuery selectors?
- How do you create a custom jQuery plugin, and what are the key considerations?
- Can you discuss how jQuery handles asynchronous programming with callbacks and promises?
- What are the potential pitfalls of using jQuery's .animate() method, and how can you avoid them?
- How would you use jQuery to manipulate CSS classes based on scroll events?
- Can you explain how to manage multiple jQuery versions on the same page?
- What techniques can you use to ensure cross-browser compatibility with jQuery?
- How do you test jQuery code effectively? What tools or frameworks do you prefer?
9 jQuery interview questions and answers related to DOM manipulation
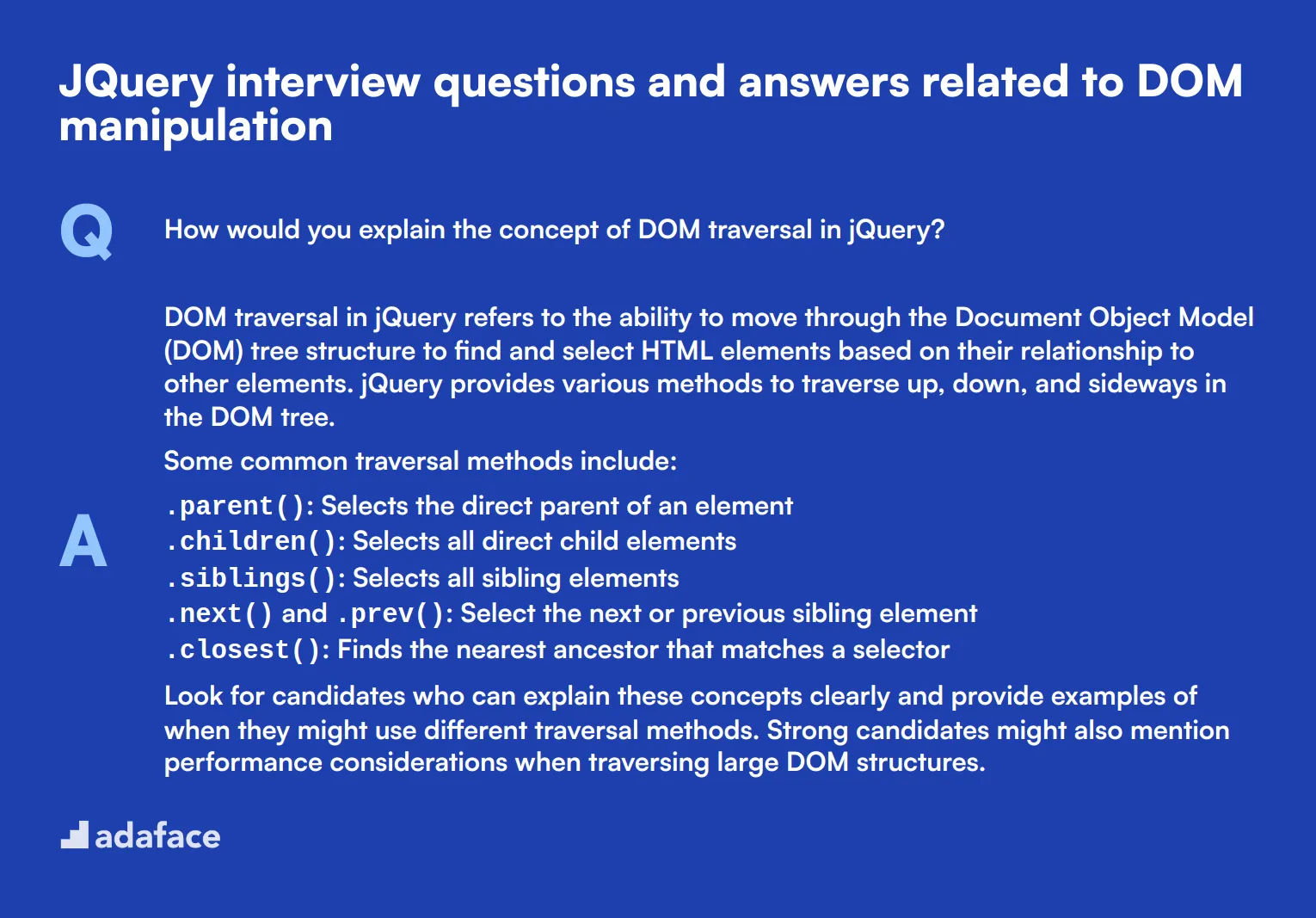
Ready to dive into the world of DOM manipulation with jQuery? These nine questions will help you assess a candidate's ability to dynamically interact with web page elements. Whether you're hiring a front-end developer or a full-stack wizard, these questions will give you insight into their jQuery prowess. Use them to spark discussions and uncover the depth of their knowledge.
1. How would you explain the concept of DOM traversal in jQuery?
DOM traversal in jQuery refers to the ability to move through the Document Object Model (DOM) tree structure to find and select HTML elements based on their relationship to other elements. jQuery provides various methods to traverse up, down, and sideways in the DOM tree.
Some common traversal methods include:
.parent()
: Selects the direct parent of an element
.children()
: Selects all direct child elements
.siblings()
: Selects all sibling elements
.next()
and.prev()
: Select the next or previous sibling element
.closest()
: Finds the nearest ancestor that matches a selector
Look for candidates who can explain these concepts clearly and provide examples of when they might use different traversal methods. Strong candidates might also mention performance considerations when traversing large DOM structures.
2. Can you describe the difference between .append() and .appendTo() methods in jQuery?
The .append()
and .appendTo()
methods in jQuery are both used to add content to the DOM, but they differ in their syntax and how they're applied:
.append()
: This method adds the specified content as the last child of each element in the jQuery set of matched elements. The syntax is$('selector').append(content)
.
.appendTo()
: This method adds the selected elements to the end of the target specified by the parameter. The syntax is$('content').appendTo('selector')
.
When evaluating responses, look for candidates who can clearly articulate the difference in syntax and provide practical examples of when they might use each method. Strong candidates might also discuss the flexibility these methods offer in manipulating the DOM and mention their counterparts .prepend()
and .prependTo()
.
3. How would you use jQuery to clone an element and all of its contents?
To clone an element and all of its contents using jQuery, you would use the .clone()
method. This method creates a deep copy of the selected element(s), including all child nodes, text, and attributes.
The basic syntax is:
var clone = $('#elementToClone').clone();
$('#destination').append(clone);
Look for candidates who can explain that the .clone()
method by default copies all children and text nodes. They should also mention that you can pass true
as an argument to .clone(true)
to copy all event handlers and data associated with the element as well. Strong candidates might discuss potential use cases for cloning, such as dynamically duplicating form fields or creating copies of complex DOM structures for manipulation.
4. Explain the concept of event delegation in jQuery and why it's useful.
Event delegation in jQuery is a technique where you attach a single event listener to a parent element instead of attaching multiple listeners to individual child elements. This approach leverages the fact that events bubble up through the DOM tree.
The main benefits of event delegation are:
- Improved performance, especially for large DOM structures
- Ability to handle dynamically added elements without reattaching event listeners
- Reduced memory usage by having fewer event listeners
When assessing responses, look for candidates who can explain how to implement event delegation using the .on()
method with a selector parameter. For example: $('#parentElement').on('click', '.childSelector', function() { ... })
. Strong candidates might also discuss scenarios where event delegation is particularly useful, such as in dynamic web applications with frequently changing DOM elements.
5. How would you use jQuery to toggle between two CSS classes on an element?
To toggle between two CSS classes on an element using jQuery, you can use the .toggleClass()
method. This method adds the specified class if it's not present and removes it if it is present.
The basic syntax for toggling a single class is:
$('#element').toggleClass('className');
To toggle between two classes, you can chain two .toggleClass()
calls:
$('#element').toggleClass('class1').toggleClass('class2');
When evaluating responses, look for candidates who understand that .toggleClass()
can take multiple class names as arguments. They should also be able to explain scenarios where toggling classes is useful, such as showing/hiding elements or changing styles based on user interactions. Strong candidates might mention the ability to pass a boolean value to force a class to be added or removed, or discuss using callbacks with .toggleClass()
for more complex logic.
6. Describe how you would use jQuery to smoothly scroll to a specific element on a page.
To smoothly scroll to a specific element on a page using jQuery, you can use the .animate()
method in combination with the .scrollTop()
method. Here's a basic approach:
- First, get the position of the target element using
.offset().top
- Then, animate the
scrollTop
property of the html and body elements
The code might look like this:
```
$('html, body').animate({
scrollTop: $('#targetElement').offset().top
}, 1000);
```
When assessing responses, look for candidates who understand the concept of animating the scroll position. They should be able to explain the parameters of the .animate()
method, including the duration and easing options. Strong candidates might discuss ways to enhance this basic implementation, such as accounting for fixed headers, adding callbacks for actions after scrolling, or mentioning alternative methods like using CSS scroll-behavior
for modern browsers.
7. How would you use jQuery to create a simple fade-in effect for elements as they enter the viewport?
Creating a fade-in effect for elements as they enter the viewport involves a combination of jQuery's animation capabilities and scroll event handling. Here's a general approach:
- Hide all elements that should fade in initially
- Attach a scroll event listener to the window
- On scroll, check if each hidden element is in the viewport
- If an element is in the viewport, fade it in
When evaluating responses, look for candidates who can outline this process and explain how to implement each step. They should mention using .scroll()
for the event listener, .offset()
and .height()
to calculate element positions, and .fadeIn()
for the animation. Strong candidates might discuss optimizing performance by throttling the scroll event, using CSS transitions instead of jQuery animations for better performance, or implementing a more robust solution using the Intersection Observer API.
8. Explain how you would use jQuery to implement a simple accordion-style expandable/collapsible section.
Implementing an accordion-style expandable/collapsible section with jQuery typically involves the following steps:
- Set up HTML structure with headers and content sections
- Hide all content sections initially
- Attach click event handlers to headers
- When a header is clicked, toggle its associated content section
Look for candidates who can describe this process and provide a basic code structure. They should mention using methods like .hide()
, .show()
, or .slideToggle()
for animations. Strong candidates might discuss adding classes for visual feedback, handling multiple open sections vs. single open section behavior, or mention accessibility considerations like proper ARIA attributes for screen readers.
9. How would you use jQuery to create a simple infinite scroll effect?
Creating an infinite scroll effect with jQuery involves detecting when the user has scrolled near the bottom of the page and then loading more content. Here's a basic approach:
- Attach a scroll event listener to the window
- Calculate the scroll position relative to the document height
- When the user is near the bottom, trigger a function to load more content
- Append the new content to the existing content container
When assessing responses, look for candidates who can outline these steps and explain how to implement them using jQuery methods. They should mention using .scroll()
, .height()
, .scrollTop()
, and AJAX methods for loading content. Strong candidates might discuss optimizing performance by debouncing or throttling the scroll event, implementing a loading indicator, or handling cases where all content has been loaded.
12 jQuery interview questions about event handling
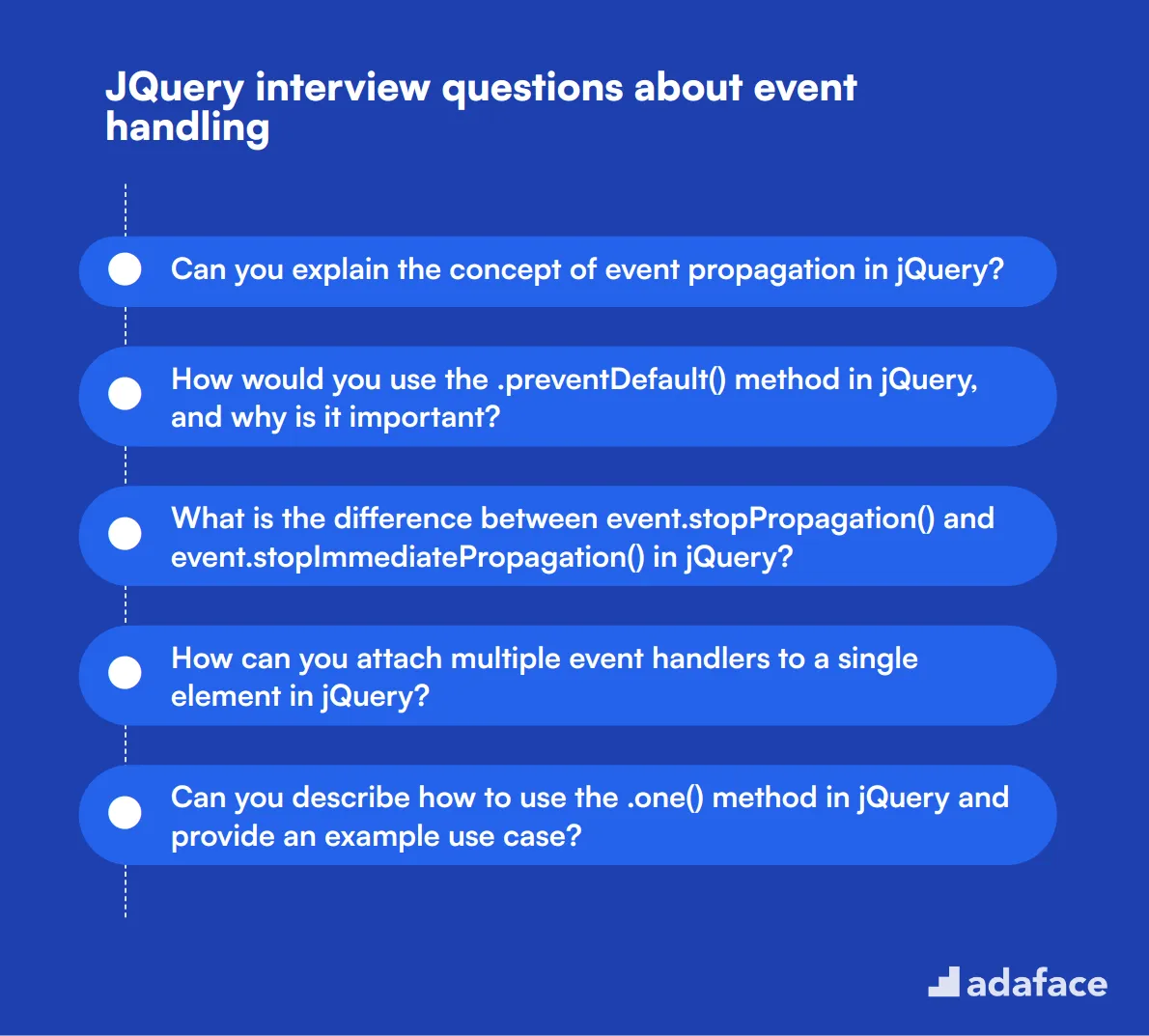
To determine whether your applicants have the necessary event handling skills in jQuery, use these 12 targeted interview questions. They will help you assess the candidate’s ability to manage events effectively, an essential skill for any front-end developer.
- Can you explain the concept of event propagation in jQuery?
- How would you use the .preventDefault() method in jQuery, and why is it important?
- What is the difference between event.stopPropagation() and event.stopImmediatePropagation() in jQuery?
- How can you attach multiple event handlers to a single element in jQuery?
- Can you describe how to use the .one() method in jQuery and provide an example use case?
- How would you handle custom events in jQuery?
- Explain the purpose and usage of the .off() method in jQuery.
- How can you use jQuery to handle events on dynamically added elements?
- What is the significance of the 'event.target' property in jQuery event handling?
- How would you implement a debounce function in jQuery to limit the rate of event handling?
- Can you explain the difference between .hover() and .mouseenter()/mouseleave() in jQuery?
- How do you pass additional data to event handlers in jQuery?
Which jQuery skills should you evaluate during the interview phase?
While it's challenging to assess every aspect of a candidate's jQuery expertise in a single interview, focusing on core skills can provide valuable insights. The following key areas are particularly important when evaluating jQuery proficiency.
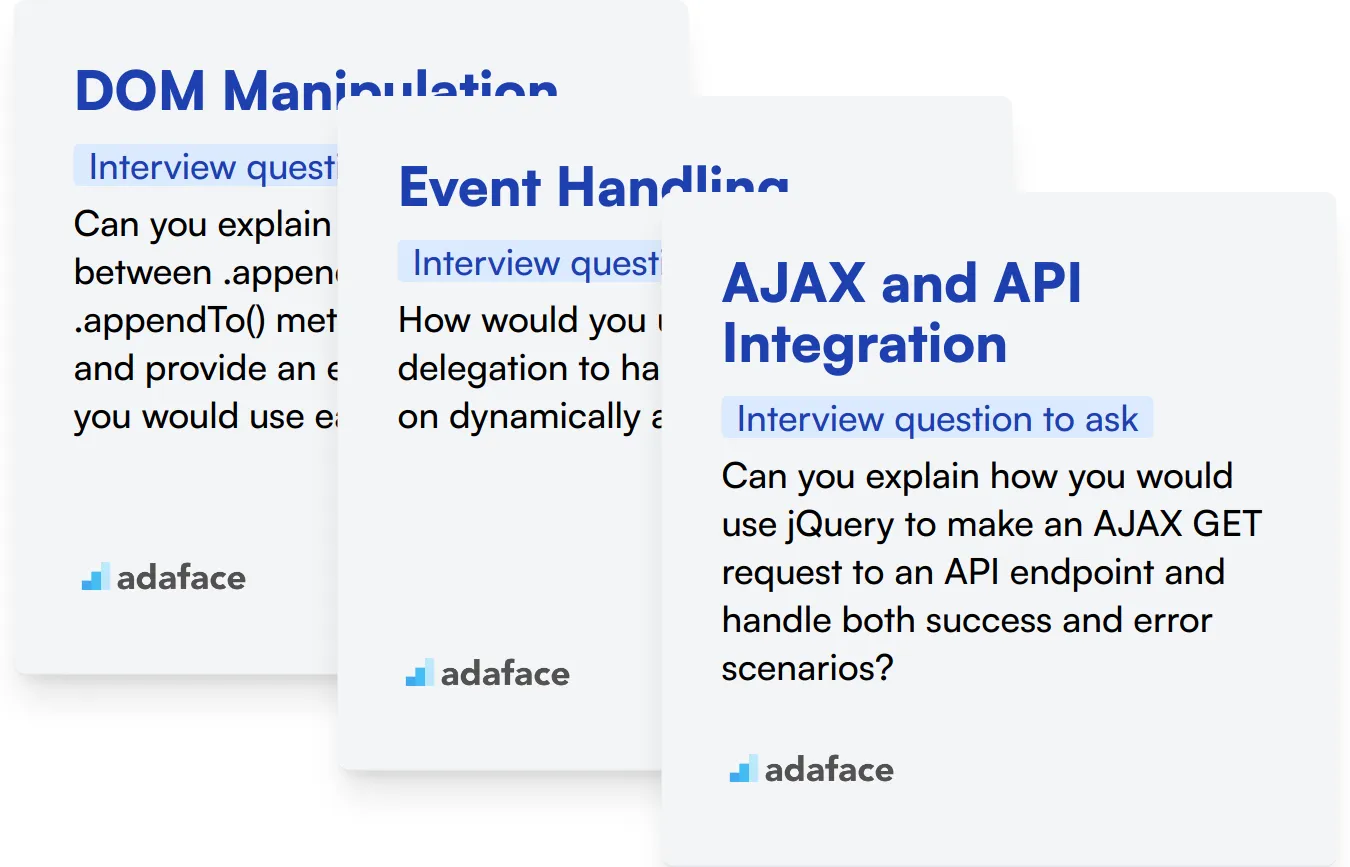
DOM Manipulation
DOM manipulation is a cornerstone of jQuery. It allows developers to efficiently interact with and modify web page elements, making it essential for creating dynamic and responsive user interfaces.
To evaluate this skill, consider using an assessment test with relevant multiple-choice questions. This can help filter candidates based on their understanding of DOM manipulation techniques.
During the interview, you can ask targeted questions to gauge the candidate's proficiency in DOM manipulation. Here's an example question to consider:
Can you explain the difference between .append() and .appendTo() methods in jQuery, and provide an example of when you would use each?
Look for answers that demonstrate a clear understanding of both methods. The candidate should explain that .append() adds content to the end of selected elements, while .appendTo() adds selected elements to the end of another element. They should also provide relevant use cases for each method.
Event Handling
Effective event handling is crucial for creating interactive web applications. jQuery simplifies this process, making it easier to respond to user actions and browser events.
An assessment test with questions focusing on event handling can help identify candidates with strong skills in this area. Consider including scenarios that test understanding of event delegation and custom events.
To further evaluate a candidate's event handling skills, you can ask specific questions during the interview. Here's an example:
How would you use event delegation to handle click events on dynamically added elements?
Look for answers that demonstrate understanding of event delegation. The candidate should explain how to attach an event listener to a parent element and use the .on() method with a selector to handle events on dynamically added child elements. They should also mention the performance benefits of this approach.
AJAX and API Integration
jQuery's AJAX capabilities are vital for modern web development. Proficiency in this area allows developers to create seamless, dynamic user experiences by interacting with server-side APIs without page reloads.
To assess AJAX skills, include questions about jQuery's $.ajax() method, promises, and error handling in your assessment test. This will help identify candidates who understand asynchronous programming concepts.
During the interview, you can ask more in-depth questions about AJAX and API integration. Here's an example:
Can you explain how you would use jQuery to make an AJAX GET request to an API endpoint and handle both success and error scenarios?
Look for answers that demonstrate a clear understanding of jQuery's AJAX methods. The candidate should be able to explain how to structure an AJAX request, including setting up success and error callbacks or using promises. They should also mention error handling best practices and how to update the DOM with the received data.
Enhance Your Hiring Strategy with jQuery Skills Tests and Targeted Interview Questions
When aiming to hire individuals with jQuery expertise, it's crucial to accurately ascertain their technical skills. Verifying these skills sets the foundation for identifying truly qualified candidates.
The most reliable way to evaluate these skills is through standardized testing. Consider using the jQuery Online Test from Adaface to ensure candidates meet your job requirements.
After candidates complete the skills test, you can efficiently shortlist the top performers. These individuals are your prime candidates for further interviews, ensuring you focus your time and resources effectively.
To move forward, invite your shortlisted candidates to explore our detailed hiring process. Get started by signing them up at Adaface Signup or learn more about our testing options at our Online Assessment Platform.
JQuery Online Test
Download jQuery interview questions template in multiple formats
jQuery Interview Questions FAQs
The questions cover basic, junior, and senior-level jQuery skills, including DOM manipulation and event handling.
There are 15 basic questions, 8 for junior developers, 12 advanced questions, 9 on DOM manipulation, and 12 on event handling.
Yes, these questions are suitable for both in-person and remote jQuery interviews.
Use them to assess candidates' jQuery knowledge, problem-solving skills, and coding practices during technical interviews.
Yes, answers are included to help interviewers evaluate candidates' responses accurately.
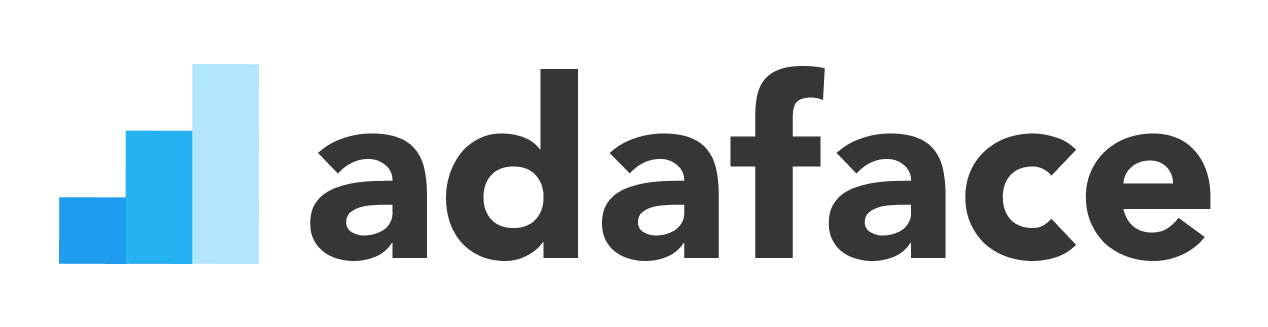
40 min skill tests.
No trick questions.
Accurate shortlisting.
We make it easy for you to find the best candidates in your pipeline with a 40 min skills test.
Try for freeRelated posts
Free resources
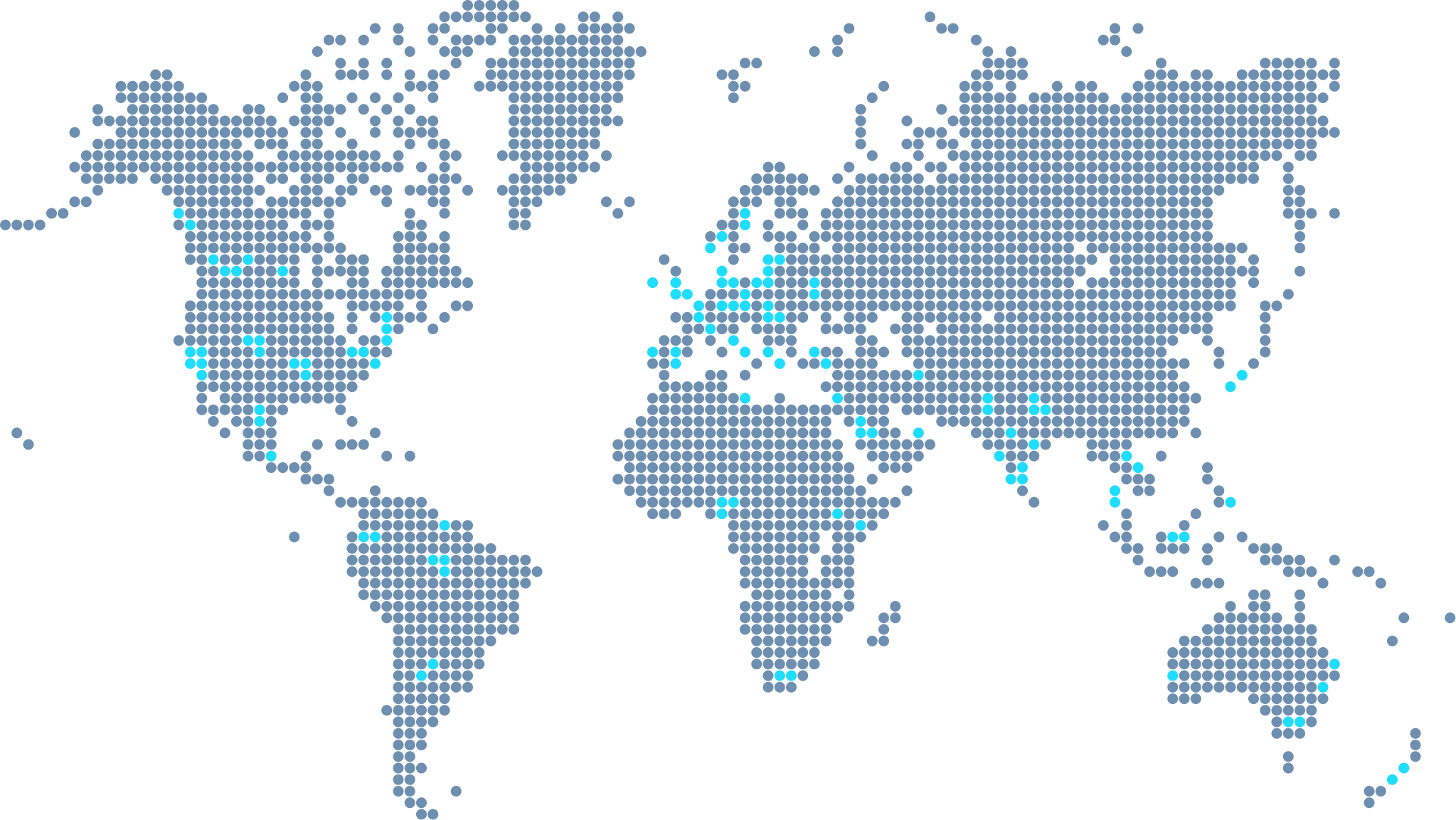
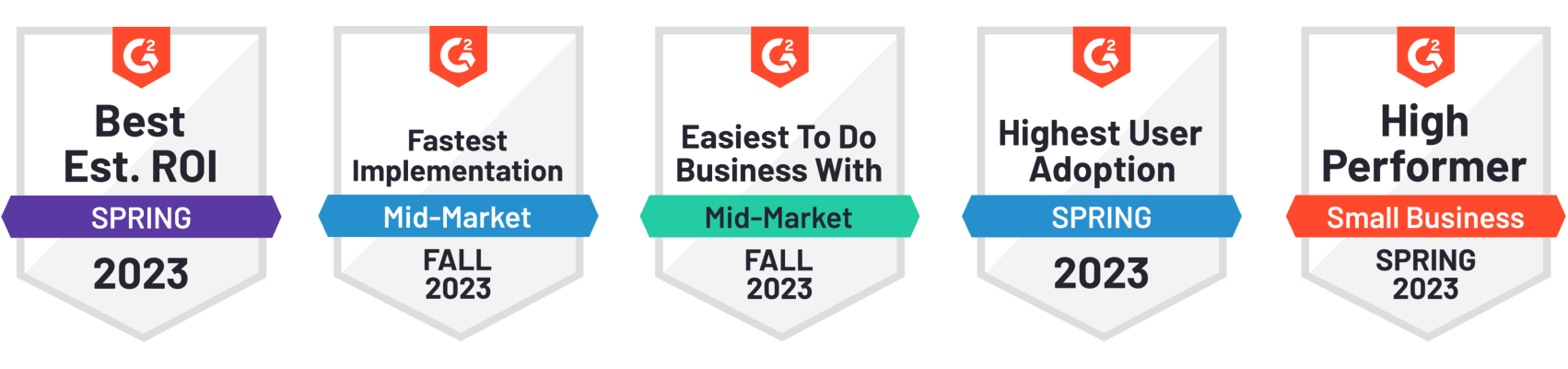