Hiring the right Golang developers is crucial for building efficient and scalable applications. As an interviewer or hiring manager, having a comprehensive list of Golang interview questions can help you effectively assess candidates' skills and knowledge.
This blog post provides a curated selection of Golang interview questions and answers for various skill levels and aspects of the language. From basic concepts to advanced topics like concurrency, we cover a range of questions to help you evaluate applicants thoroughly.
By using these questions, you can gain valuable insights into candidates' Golang expertise and problem-solving abilities. Additionally, consider using a Golang online test to pre-screen candidates before the interview stage, ensuring you focus on the most promising applicants.
Table of contents
15 basic Golang interview questions and answers to assess applicants
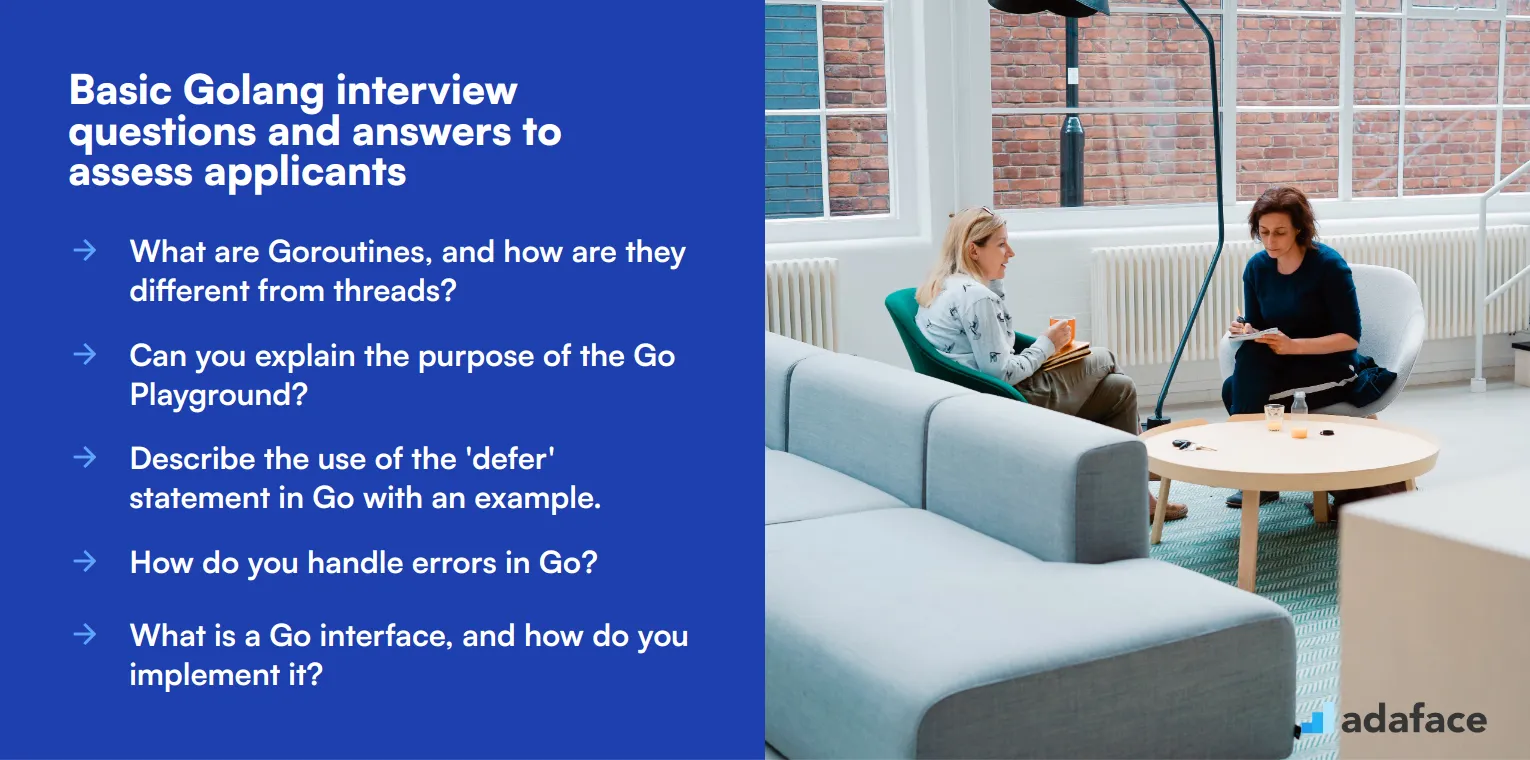
To determine if your applicants possess foundational knowledge and skills in Golang, use these 15 basic interview questions. By leveraging this list, you can efficiently assess whether candidates have the necessary expertise to excel in a Golang developer job.
- What are Goroutines, and how are they different from threads?
- Can you explain the purpose of the Go Playground?
- Describe the use of the 'defer' statement in Go with an example.
- How do you handle errors in Go?
- What is a Go interface, and how do you implement it?
- Explain the difference between a slice and an array in Golang.
- What is the significance of the 'init' function in Go?
- How does Go handle memory management and garbage collection?
- What are some common use cases for Go channels?
- How do you perform unit testing in Go?
- Explain the concept of 'embedding' in Go structs.
- What tools or libraries do you use for dependency management in Go?
- Describe the use of context package in Go.
- How would you handle concurrency in a Go program?
- What are the advantages of using Go over other programming languages?
8 Golang interview questions and answers to evaluate junior developers
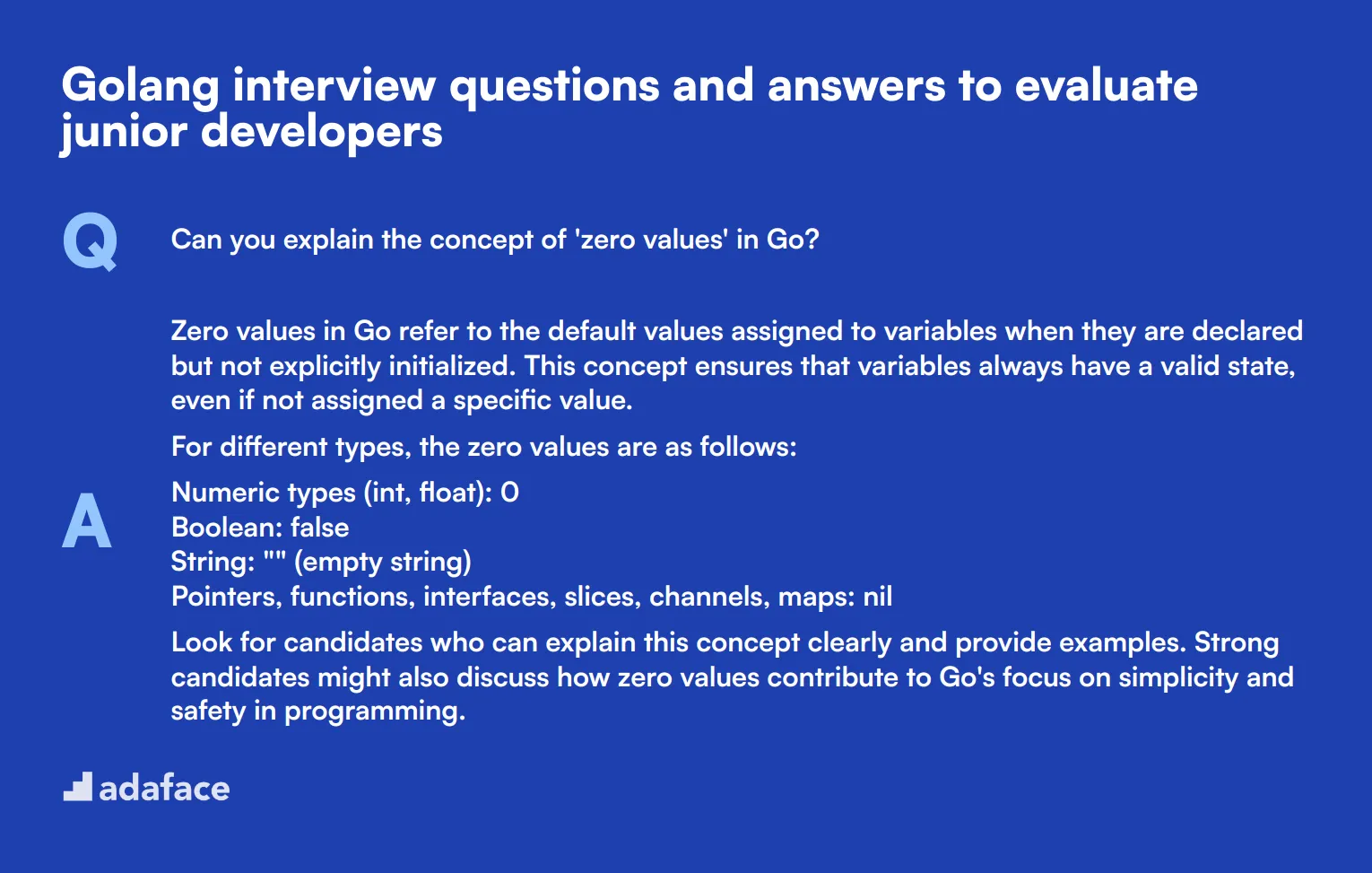
When evaluating junior Golang developers, it's crucial to assess their fundamental understanding and practical knowledge. These eight questions are designed to help you gauge a candidate's grasp of Go basics, problem-solving skills, and coding practices. Use them as a starting point to spark insightful discussions and get a clearer picture of your potential hire's capabilities.
1. Can you explain the concept of 'zero values' in Go?
Zero values in Go refer to the default values assigned to variables when they are declared but not explicitly initialized. This concept ensures that variables always have a valid state, even if not assigned a specific value.
For different types, the zero values are as follows:
- Numeric types (int, float): 0
- Boolean: false
- String: "" (empty string)
- Pointers, functions, interfaces, slices, channels, maps: nil
Look for candidates who can explain this concept clearly and provide examples. Strong candidates might also discuss how zero values contribute to Go's focus on simplicity and safety in programming.
2. How does Go handle method overloading?
Go does not support method overloading in the traditional sense. In Go, each method must have a unique name within its type. This design choice aligns with Go's philosophy of simplicity and readability.
Instead of overloading, Go encourages using descriptive method names or utilizing interfaces to achieve similar functionality. For example, instead of having multiple 'Print' methods with different parameters, you might have 'PrintToConsole' and 'PrintToFile'.
Look for candidates who understand this limitation and can explain alternative approaches. Strong candidates might discuss how this impacts code organization and naming conventions in Go projects.
3. What is the purpose of the 'blank identifier' in Go?
The blank identifier in Go, represented by an underscore (_), is used to ignore values that would otherwise be assigned to variables. It's particularly useful in situations where you need to satisfy Go's requirement that all variables be used, but you don't actually need the value.
Common use cases for the blank identifier include:
- Ignoring return values from functions
- In for-range loops when you only need the value or the index
- Importing packages for their side effects without using their exported identifiers
Evaluate how well the candidate understands this concept and if they can provide practical examples of its use. Strong candidates might also discuss how the blank identifier contributes to writing cleaner, more maintainable code.
4. Explain the difference between 'make' and 'new' functions in Go.
The 'make' and 'new' functions in Go are both used for memory allocation, but they serve different purposes:
- 'new(T)' allocates zeroed storage for a new item of type T and returns its address (a pointer to T)
- 'make(T, args)' creates slices, maps, and channels only, and returns an initialized (not zeroed) value of type T (not a pointer)
'new' is used for value types and user-defined types, like structs. 'make' is used for reference types (slices, maps, channels) that need initialization before use.
Look for candidates who can clearly articulate the differences and provide examples of when to use each. Strong candidates might also discuss memory management implications and common use cases for each function.
5. How does Go support concurrency at the language level?
Go supports concurrency at the language level through goroutines and channels. Goroutines are lightweight threads managed by the Go runtime, allowing for efficient concurrent execution. Channels provide a way for goroutines to communicate and synchronize their execution.
Key features of Go's concurrency model include:
- Easy creation of goroutines using the 'go' keyword
- Channels for safe communication between goroutines
- The select statement for managing multiple channel operations
- Sync package for more traditional synchronization primitives
Assess the candidate's understanding of these concepts and their ability to explain how they contribute to writing concurrent programs. Look for insights into the advantages of Go's approach compared to traditional threading models.
6. What are empty structs in Go and when might you use them?
Empty structs in Go are structs with no fields, declared as 'struct{}'. They occupy zero bytes of storage, making them memory-efficient. While they might seem counterintuitive, empty structs have several practical uses in Go programming.
Common use cases for empty structs include:
- Implementing sets (using map[key]struct{})
- Signaling in channels without sending data
- As placeholders in APIs for future extensibility
Look for candidates who can explain the concept clearly and provide practical examples. Strong candidates might discuss the memory efficiency aspect and how empty structs can lead to more idiomatic Go code in certain scenarios.
7. How does type assertion work in Go?
Type assertion in Go is a way to extract the underlying concrete type from an interface type. It's used when you have a value of an interface type but need to access methods or fields that are specific to the concrete type.
The syntax for type assertion is x.(T), where x is a value of interface type and T is the type you're asserting x to be. Type assertion can be used in two ways:
- Single-value form: v := x.(T)
- Two-value form: v, ok := x.(T)
Evaluate the candidate's understanding of when and how to use type assertions. Look for awareness of the panic risk in the single-value form and the safer two-value form. Strong candidates might discuss type switches as an alternative for multiple type checks.
8. Explain the concept of method sets in Go.
Method sets in Go define the set of methods that are associated with a type. They are important in determining which types satisfy an interface. The method set of a type T consists of all methods with receiver type T, while the method set of a pointer type *T includes methods with receiver *T or T.
This concept is crucial for understanding how interfaces work in Go:
- Values of type T satisfy an interface if T's method set is a superset of the interface
- Pointers to T satisfy an interface if *T's method set is a superset of the interface
Look for candidates who can explain this concept clearly and understand its implications for interface satisfaction. Strong candidates might discuss how this affects the choice between value and pointer receivers in method declarations.
12 Golang questions related to syntax and semantics
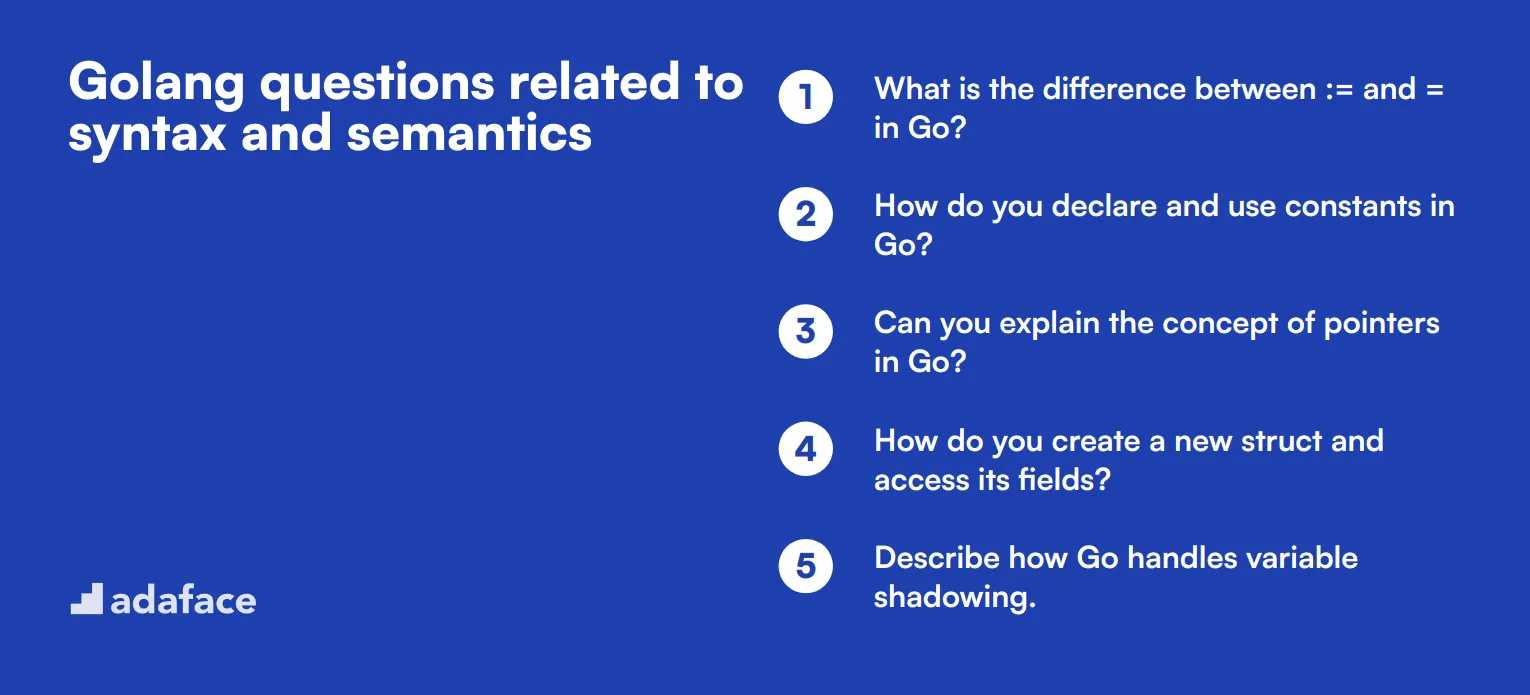
To determine whether your candidates have a solid grasp of Golang's syntax and semantics, consider using some of these essential interview questions. These questions are designed to evaluate an applicant's practical understanding of the language, which is crucial for roles such as Golang developer.
- What is the difference between := and = in Go?
- How do you declare and use constants in Go?
- Can you explain the concept of pointers in Go?
- How do you create a new struct and access its fields?
- Describe how Go handles variable shadowing.
- What are the different types of loops in Go and how do they work?
- How do you declare and use a function with multiple return values?
- What is a type switch in Go?
- How do you handle panics in Go?
- Explain how to use Go's built-in 'recover' function.
- How does Go support anonymous functions and closures?
- What are the rules for naming variables and functions in Go?
7 Golang interview questions and answers related to concurrency
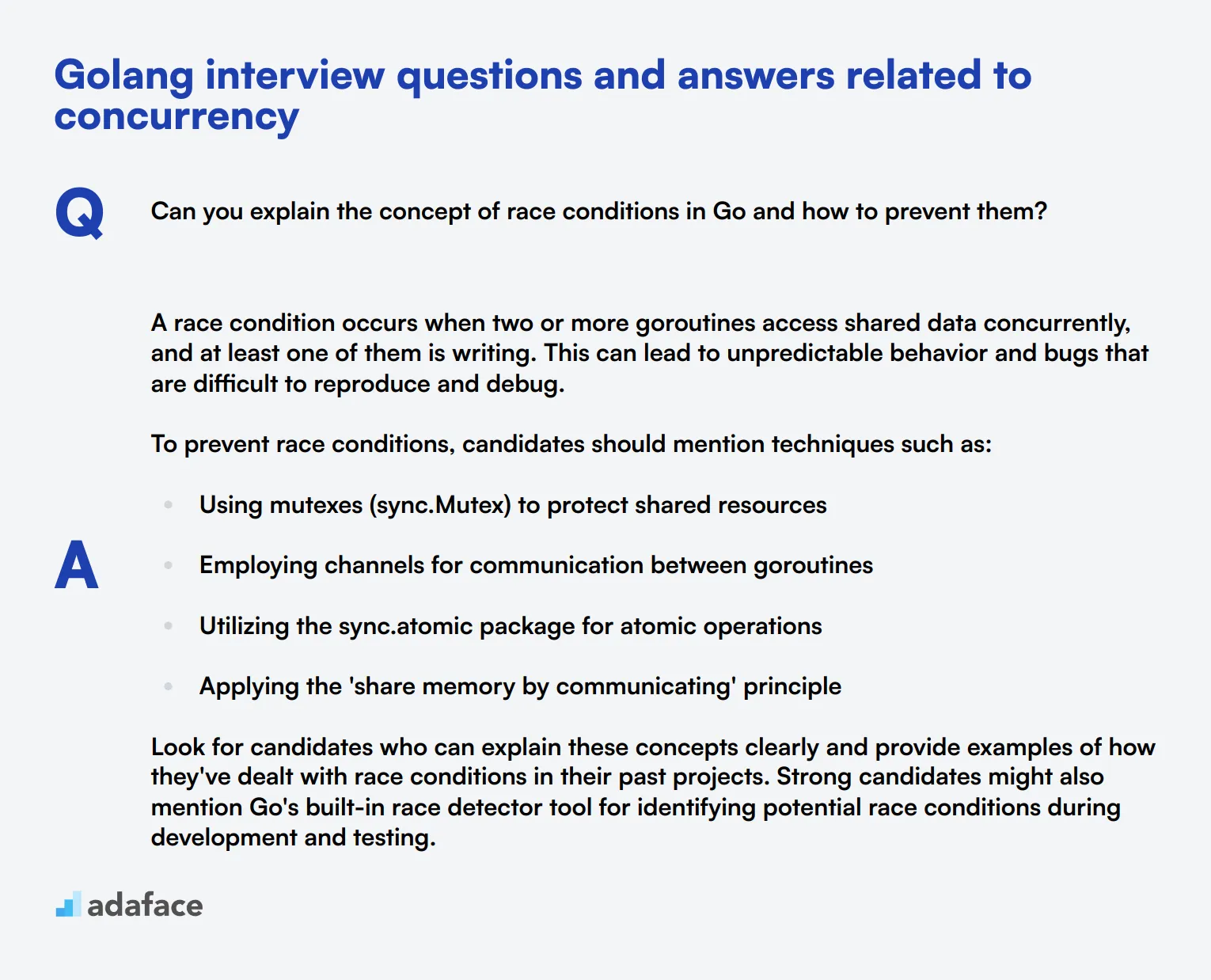
When interviewing for a Golang developer position, it's crucial to assess a candidate's understanding of concurrency, a key feature of the language. These seven questions will help you gauge a candidate's grasp of Go's concurrent programming concepts and their ability to apply them in real-world scenarios. Use these questions to evaluate skills and identify top talent for your team.
1. Can you explain the concept of race conditions in Go and how to prevent them?
A race condition occurs when two or more goroutines access shared data concurrently, and at least one of them is writing. This can lead to unpredictable behavior and bugs that are difficult to reproduce and debug.
To prevent race conditions, candidates should mention techniques such as:
- Using mutexes (sync.Mutex) to protect shared resources
- Employing channels for communication between goroutines
- Utilizing the sync.atomic package for atomic operations
- Applying the 'share memory by communicating' principle
Look for candidates who can explain these concepts clearly and provide examples of how they've dealt with race conditions in their past projects. Strong candidates might also mention Go's built-in race detector tool for identifying potential race conditions during development and testing.
2. How does the select statement work in Go, and what are its use cases?
The select statement in Go is used to choose from multiple send/receive channel operations. It blocks until one of its cases can run, then executes that case. If multiple cases are ready, it chooses one at random.
Common use cases for the select statement include:
- Implementing timeouts
- Non-blocking channel operations
- Combining inputs from multiple channels
- Implementing cancellation and graceful shutdown
A strong candidate should be able to provide examples of how they've used select in their projects. Look for answers that demonstrate an understanding of how select can improve the efficiency and responsiveness of concurrent programs. Follow up by asking about specific scenarios where they found select particularly useful.
3. What is the purpose of WaitGroups in Go, and how do you use them?
WaitGroups in Go are used to wait for a collection of goroutines to finish executing before proceeding. They're part of the sync package and are particularly useful for managing concurrent operations where you need to ensure all tasks are completed before moving on.
The basic usage of WaitGroups involves three main steps:
- Creating a WaitGroup
- Calling Add() to set the number of goroutines to wait for
- Calling Done() in each goroutine when it finishes
- Calling Wait() to block until all goroutines have called Done()
Look for candidates who can explain these steps clearly and provide examples of how they've used WaitGroups in their work. Strong candidates might also discuss potential pitfalls, such as forgetting to call Done() or mismatching Add() and Done() calls, and how to avoid them. Consider asking about alternative synchronization techniques they might use in different scenarios.
4. Explain the difference between buffered and unbuffered channels in Go.
Buffered and unbuffered channels in Go differ in how they handle send and receive operations:
Unbuffered channels (created with make(chan T)):
- Have no capacity to store values
- Synchronize send and receive operations
- Sending blocks until another goroutine receives
- Receiving blocks until another goroutine sends
Buffered channels (created with make(chan T, size)):
- Have a capacity to store a fixed number of values
- Allow asynchronous send operations as long as the buffer isn't full
- Sending only blocks when the buffer is full
- Receiving only blocks when the buffer is empty
Look for candidates who can clearly articulate these differences and provide examples of when to use each type. Strong candidates might discuss the trade-offs between the two, such as how buffered channels can improve performance in certain scenarios but may hide synchronization issues. Ask about specific use cases where they've employed each type of channel in their Go projects.
5. How does Go's garbage collector handle concurrent programs?
Go's garbage collector is designed to work efficiently with concurrent programs. It uses a concurrent, tri-color, mark-and-sweep algorithm that allows it to run concurrently with the application code, minimizing pause times.
Key features of Go's garbage collector in concurrent contexts include:
- Concurrent marking and sweeping phases
- Write barriers to ensure correctness during concurrent execution
- Incremental collection to distribute work over time
- Parallel execution to utilize multiple CPU cores
Look for candidates who can explain these concepts and their implications for performance. Strong candidates might discuss how the garbage collector's behavior affects their design decisions in concurrent programs, such as considering object lifetimes and allocation patterns. They might also mention tools like runtime.ReadMemStats() for monitoring garbage collection behavior in production environments.
6. What is a mutex in Go, and how does it differ from channels for synchronization?
A mutex (mutual exclusion) in Go is a synchronization primitive used to protect shared resources from concurrent access. It's part of the sync package and provides Lock() and Unlock() methods to control access to critical sections of code.
Differences between mutexes and channels for synchronization:
- Mutexes are used for exclusive access to shared memory
- Channels are used for communication and synchronization by passing data
- Mutexes are typically faster for simple shared memory access
- Channels provide a higher-level abstraction and can be easier to reason about
Look for candidates who can explain when to use mutexes versus channels. Strong candidates might discuss the 'share memory by communicating' principle in Go and how it influences their choice between mutexes and channels. They should also be aware of potential issues like deadlocks and how to avoid them. Consider asking about real-world scenarios where they've had to choose between these synchronization methods.
7. How do you implement graceful shutdown of a concurrent Go program?
Implementing graceful shutdown in a concurrent Go program involves coordinating the termination of multiple goroutines and ensuring that all resources are properly released. A common approach uses a combination of channels, context cancellation, and WaitGroups.
Key steps in implementing graceful shutdown:
- Use a context with cancellation for signaling shutdown
- Listen for termination signals (e.g., SIGINT, SIGTERM)
- Cancel the context when a termination signal is received
- Use the cancelled context to signal goroutines to stop
- Use WaitGroups to ensure all goroutines have finished
- Close any open resources (e.g., database connections, file handles)
Look for candidates who can outline a clear strategy for graceful shutdown. Strong candidates might discuss the importance of timeout mechanisms to prevent indefinite waiting, and how they handle cleanup of resources in long-running goroutines. Ask about specific challenges they've faced in implementing graceful shutdown in production systems and how they overcame them.
6 situational Golang interview questions with answers for hiring top developers
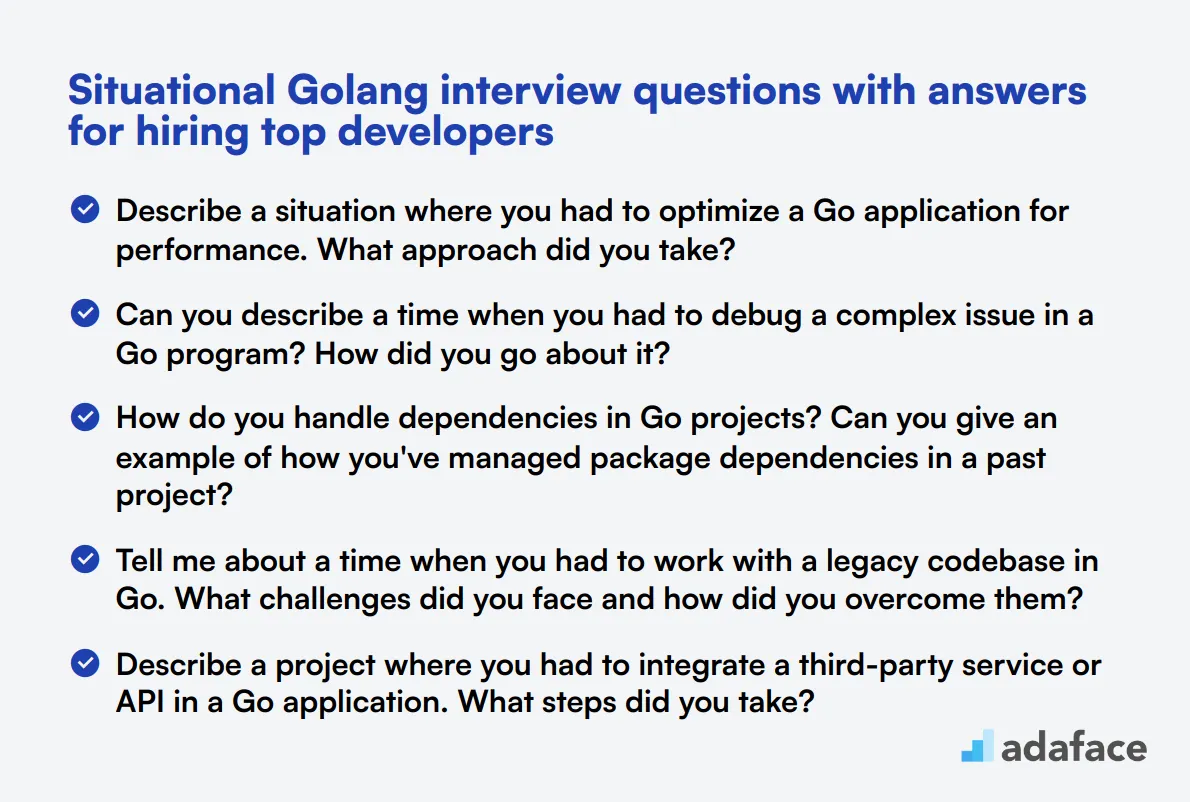
To identify top-notch Golang developers who can handle real-world challenges, these situational interview questions will help you gauge their problem-solving skills, adaptability, and practical knowledge. Use these questions when you want to go beyond textbook definitions and see how candidates think on their feet.
1. Describe a situation where you had to optimize a Go application for performance. What approach did you take?
When optimizing a Go application for performance, it’s crucial to first identify the bottlenecks. This often involves profiling the application to understand where most of the time is being spent. Common tools for this include pprof, which can give detailed insights into CPU and memory usage.
Once the bottlenecks are identified, candidates might discuss various strategies such as optimizing algorithms, reducing memory allocations, or using more efficient data structures. They may also mention the importance of concurrency in Go and how they utilized goroutines and channels to improve performance.
Look for candidates who can clearly explain their process of identifying and resolving performance issues. They should demonstrate a methodical approach and provide specific examples of the techniques they used.
2. Can you describe a time when you had to debug a complex issue in a Go program? How did you go about it?
Debugging a complex issue in a Go program often starts with reproducing the problem consistently. This can involve writing unit tests that isolate the issue or using log statements to trace the program’s execution.
Candidates might discuss using Go’s built-in debugging tools such as Delve to step through the code and inspect variables. They might also mention checking for common issues like race conditions or memory leaks.
Strong candidates will provide a structured approach to debugging, including how they isolated the problem, the tools they used, and the steps they took to resolve it. Look for a logical and systematic problem-solving process.
3. How do you handle dependencies in Go projects? Can you give an example of how you've managed package dependencies in a past project?
Handling dependencies in Go typically involves using Go modules, which is the dependency management system introduced in Go 1.11. Candidates should explain how they use go.mod
and go.sum
files to manage versions of dependencies.
They might also discuss their process for updating dependencies, ensuring compatibility, and resolving conflicts. For example, they could mention running go get -u
to update dependencies and using semantic versioning to avoid breaking changes.
Ideal candidates will demonstrate a clear understanding of Go modules and provide a specific example of how they have managed dependencies in a project. They should also emphasize the importance of maintaining a clean and organized go.mod
file.
4. Tell me about a time when you had to work with a legacy codebase in Go. What challenges did you face and how did you overcome them?
Working with a legacy codebase often involves understanding the existing architecture and code conventions. Candidates might describe starting with thorough documentation and code reviews to get up to speed.
Challenges could include outdated dependencies, lack of tests, or convoluted code. They might discuss strategies like refactoring for readability, adding unit tests to ensure stability, and gradually updating dependencies.
Look for candidates who can clearly articulate the challenges they faced and the steps they took to address them. They should show an ability to balance maintaining existing functionality with making improvements.
5. Describe a project where you had to integrate a third-party service or API in a Go application. What steps did you take?
Integrating a third-party service typically involves understanding the API documentation and setting up the necessary authentication. Candidates might describe using Go’s net/http
package or a specific client library provided by the service.
They could discuss handling errors and edge cases, such as rate limiting or service outages. They might also mention testing the integration thoroughly to ensure it works as expected under different conditions.
Ideal candidates will provide a detailed description of their approach, including how they handled authentication, error handling, and testing. They should demonstrate an understanding of best practices for integrating third-party services.
6. Have you ever had to make a significant change to a Go application’s architecture? What was the reason and how did you approach it?
Making a significant change to an application’s architecture often starts with identifying the need for the change, such as improving scalability or maintainability. Candidates might describe conducting a thorough analysis and planning phase.
They may discuss breaking down the changes into manageable tasks, ensuring backward compatibility, and implementing changes incrementally. They could also mention using design patterns or architectural principles like microservices or event-driven architecture.
Look for candidates who can provide a well-structured approach to making architectural changes. They should demonstrate careful planning, a clear understanding of the benefits and drawbacks, and effective communication with the team.
Which Golang skills should you evaluate during the interview phase?
While it's impossible to gauge every facet of a candidate's capabilities in a single interview, focusing on core Golang skills can provide crucial insights into their potential to excel. Identifying these competencies ensures you assess what's most relevant for success in your Golang development roles.
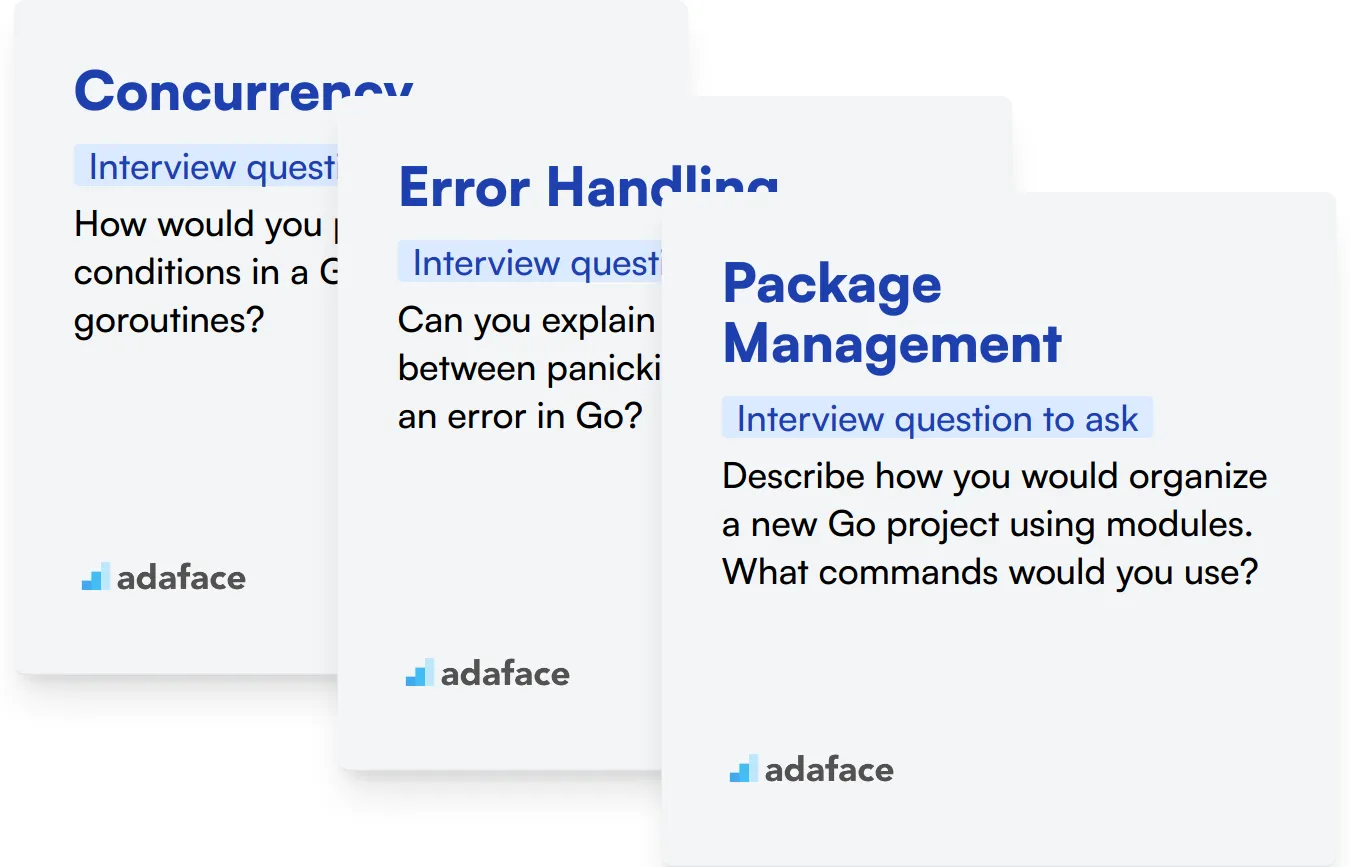
Concurrency
Concurrency is a fundamental aspect of Golang, designed to handle multiple processes running simultaneously. Mastering concurrency allows developers to write more efficient, faster-executing code, especially in networked applications and multi-threaded tasks.
To initially screen for proficiency in concurrency, consider integrating an assessment with relevant MCQs. Adaface offers a tailored Golang assessment that includes concurrency topics.
Further evaluate the candidate's understanding by posing specific concurrency questions during the interview.
How would you prevent race conditions in a Go program using goroutines?
Look for candidates to discuss the use of mutexes or channels to synchronize shared resources, demonstrating a clear understanding of safe concurrency practices.
Error Handling
Effective error handling in Golang is critical as it ensures robust applications by managing runtime errors gracefully. It's integral for maintaining code reliability and service continuity.
Ask targeted questions to determine how well a candidate can implement error handling strategies.
Can you explain the difference between panicking and returning an error in Go?
Candidates should clearly explain scenarios where it's appropriate to use panics versus returning errors, showing their ability to choose the right approach based on the situation.
Package Management
Understanding Go's package management is vital for maintaining scalable and manageable code bases. Candidates should be familiar with creating and managing modules and dependencies effectively.
You might use a comprehensive Golang test to initially assess candidates' knowledge on package management through a series of focused MCQs.
During the interview, delve deeper into their practical knowledge with application-specific questions.
Describe how you would organize a new Go project using modules. What commands would you use?
Expect detailed responses on initializing new modules, managing dependencies, and structuring the project for readability and maintenance.
Hire top talent with Golang skills tests and the right interview questions
When hiring for a role that requires Golang skills, it is crucial to ensure candidates possess the right expertise. Accurately assessing these skills can help you find the best fit for your team.
One efficient way to evaluate these skills is by utilizing skill tests. Consider using our Golang online test to gain insights into candidates' proficiency.
After administering the test, you can effectively shortlist the best applicants based on their performance. This allows you to focus your interview efforts on the most qualified candidates.
To get started, visit our online assessment platform and sign up. This will enable you to streamline your hiring process and find the ideal Golang developers for your team.
Go/Golang Online Test
Download Golang interview questions template in multiple formats
Golang Interview Questions FAQs
Basic questions cover topics like the Go runtime, data types, and basic syntax. These help gauge foundational understanding.
You can focus on questions that test their understanding of basic concepts and their ability to write simple programs.
Questions could include topics like pointers, slices, maps, and struct types. These reveal candidates' depth of language understanding.
Golang heavily uses goroutines and channels. Questions about concurrency help assess candidates' ability to handle parallel tasks.
Situational questions present real-world problems to see how candidates apply their knowledge and problem-solving skills.
Skill tests provide a practical assessment, measuring candidates' abilities to implement solutions within a time frame.
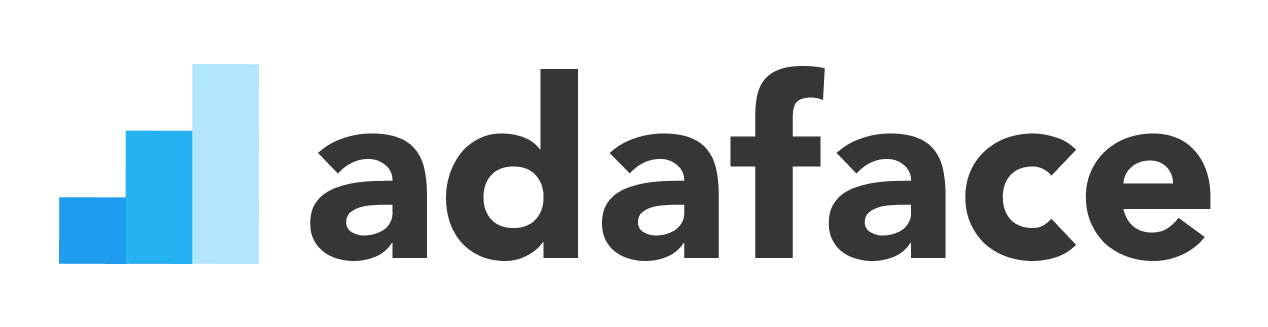
40 min skill tests.
No trick questions.
Accurate shortlisting.
We make it easy for you to find the best candidates in your pipeline with a 40 min skills test.
Try for freeRelated posts
Free resources
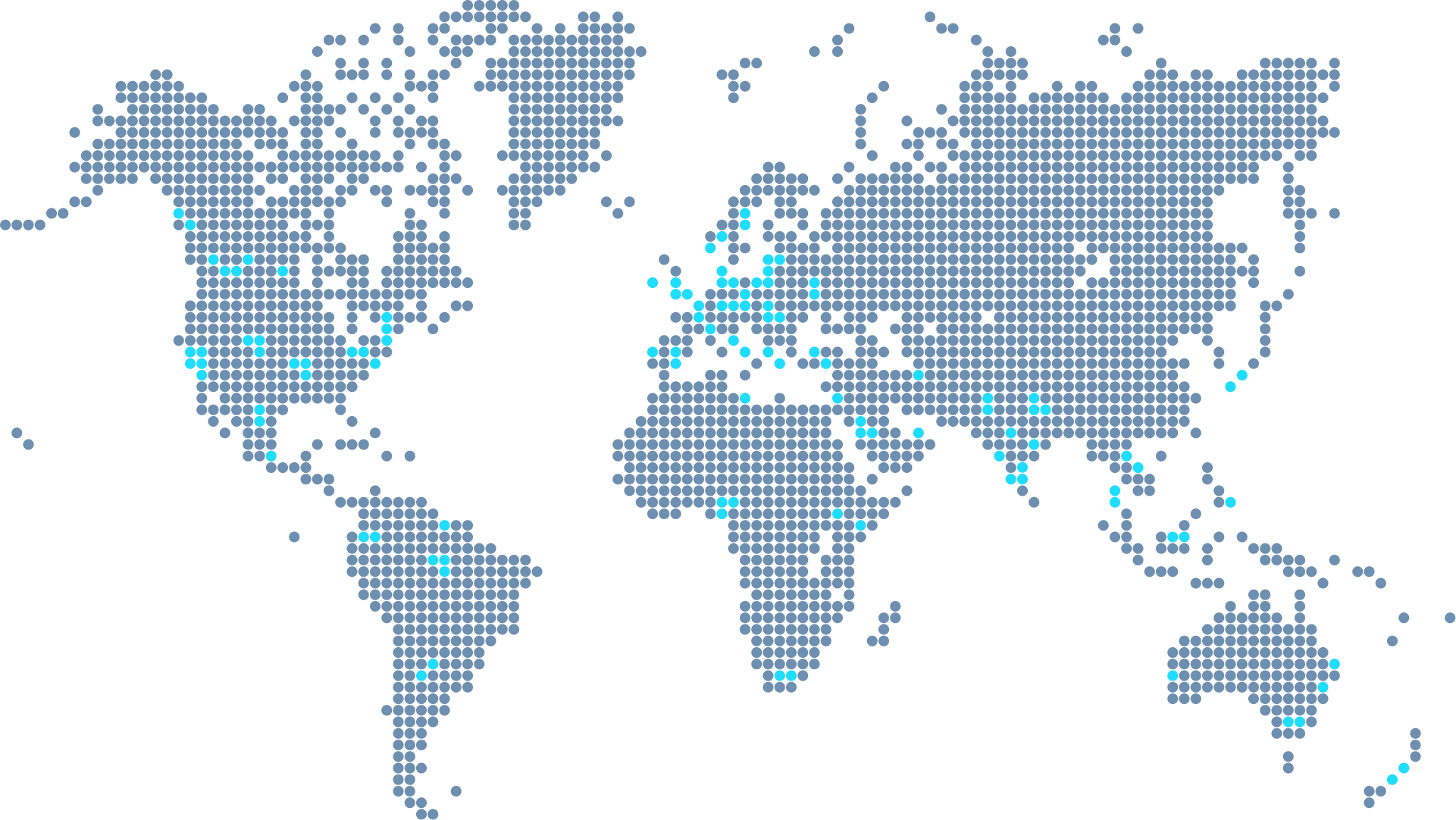
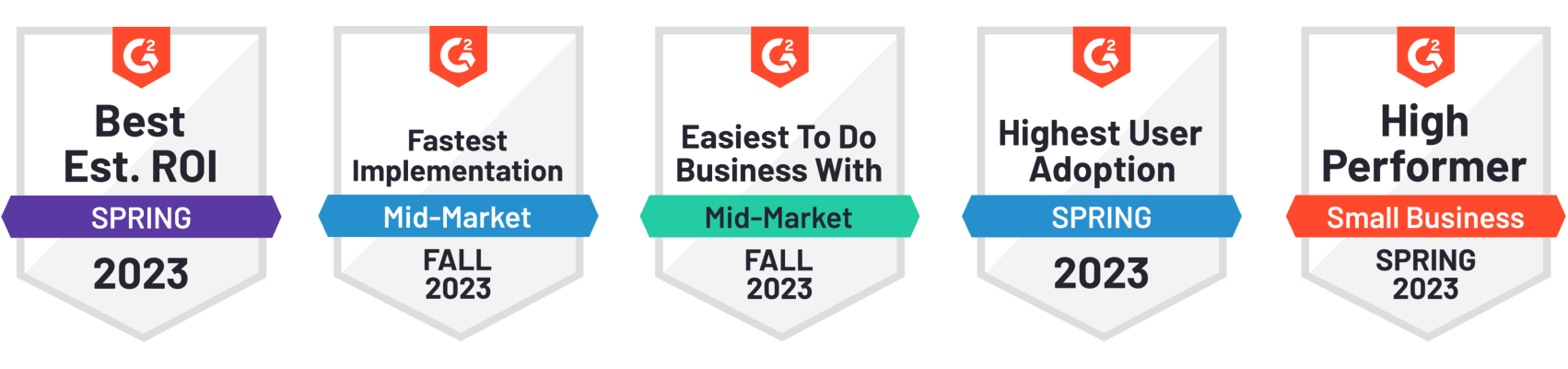