Hiring the right Flutter developer can make or break your mobile app project. By asking targeted interview questions, you can effectively gauge a candidate's proficiency in Flutter development and ensure they have the skills required for mobile development.
This blog post provides a comprehensive list of Flutter interview questions tailored for different experience levels. From junior to senior developers, we cover essential topics including state management, widget lifecycle, and situational challenges.
Use these questions to conduct thorough technical interviews and identify top Flutter talent. For a more comprehensive evaluation, consider combining these interview questions with a Flutter skills assessment to streamline your hiring process.
Table of contents
Top 8 Flutter questions to ask in interviews
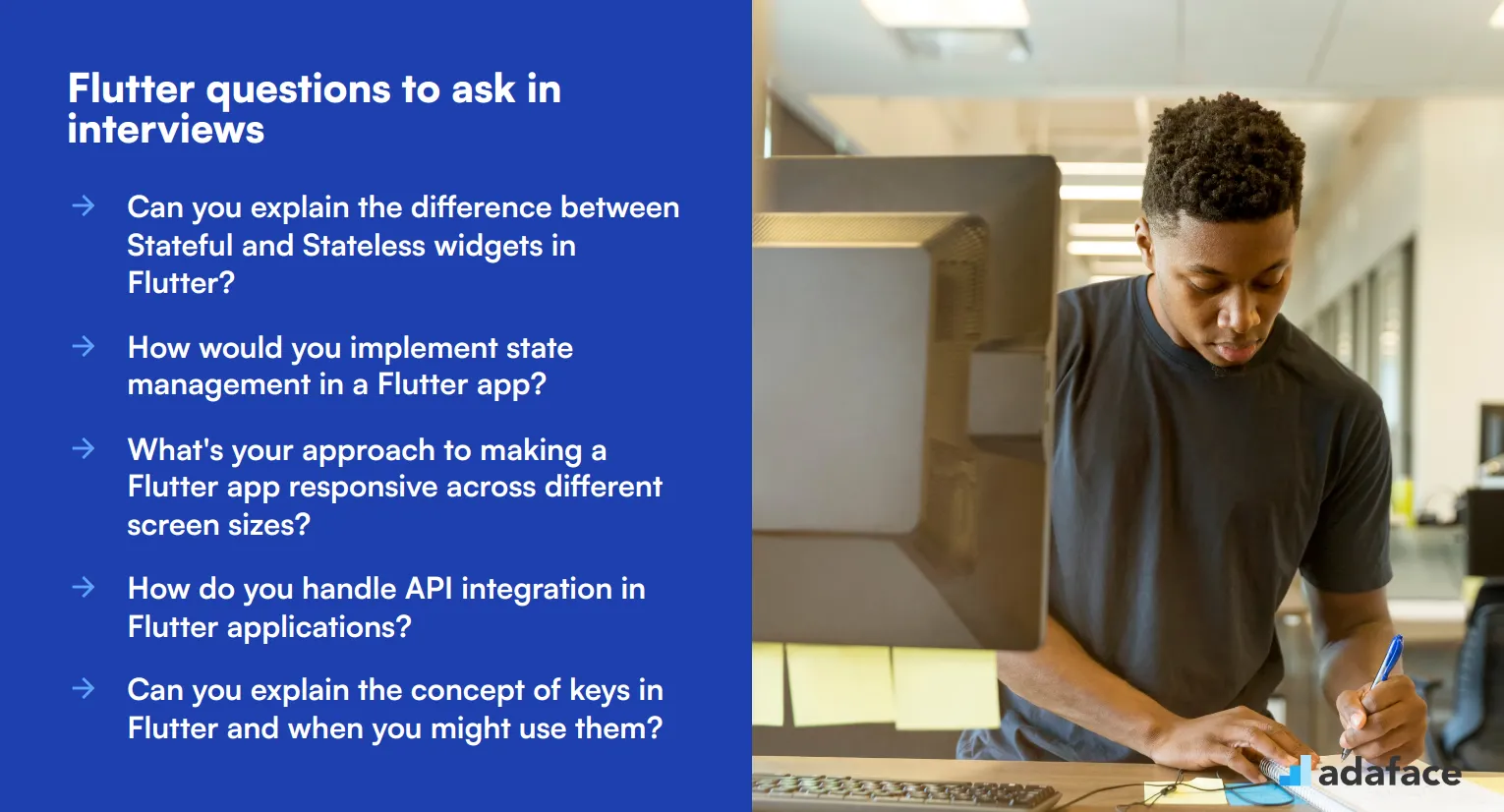
Ready to find your next Flutter superstar? These top 8 Flutter interview questions are designed to help you identify candidates with the right mix of technical know-how and problem-solving skills. Use them to spark insightful discussions and gauge a candidate's expertise in this versatile framework. Remember, the best interviews feel more like a chat between fellow developers than an interrogation!
1. Can you explain the difference between Stateful and Stateless widgets in Flutter?
A strong candidate should be able to clearly differentiate between Stateful and Stateless widgets. Stateless widgets are immutable, meaning once they are built, their properties can't change. They are used for parts of the user interface that don't need to change dynamically. Stateful widgets, on the other hand, can change their appearance in response to events triggered by user interactions or when they receive data.
Look for candidates who can provide examples of when to use each type of widget. They might mention using Stateless widgets for static UI elements like text or icons, and Stateful widgets for interactive elements like forms or animated components. A comprehensive answer might also touch on the performance implications of choosing one over the other.
2. How would you implement state management in a Flutter app?
An ideal response should demonstrate familiarity with multiple state management solutions in Flutter. Candidates might mention popular options like Provider, BLoC (Business Logic Component), Redux, or GetX. They should be able to explain the basics of at least one of these approaches and discuss its pros and cons.
Pay attention to candidates who can articulate when to use different state management solutions based on the app's complexity and requirements. They might discuss using simple solutions like setState() for small apps, and more robust options like BLoC for larger, more complex applications. Strong candidates will also touch on the importance of separating business logic from the UI for maintainability and testability.
3. What's your approach to making a Flutter app responsive across different screen sizes?
A comprehensive answer should cover several strategies for creating responsive Flutter apps. Candidates might mention using flexible widgets like Expanded, Flexible, and FractionallySizedBox to create layouts that adapt to different screen sizes. They should also be familiar with MediaQuery to access the device's screen dimensions and orientation.
Look for responses that discuss the importance of testing on various devices and using tools like Flutter's DevTools to simulate different screen sizes. Candidates might also mention using LayoutBuilder to create adaptive layouts or CustomMultiChildLayout for more complex, custom responsive designs. Bonus points if they bring up the concept of creating a responsive design system to ensure consistency across the app.
4. How do you handle API integration in Flutter applications?
Strong candidates should be able to outline a clear process for integrating APIs in Flutter apps. They might mention using packages like http or dio for making network requests, and discuss how to handle asynchronous operations using Future and async/await. They should also touch on the importance of error handling and displaying loading states to improve user experience.
Look for answers that demonstrate an understanding of RESTful API concepts and JSON parsing. Candidates might discuss using built-in dart:convert library or packages like json_serializable for more complex JSON structures. They should also be aware of best practices like separating API logic into a service layer and using models to represent API responses.
5. Can you explain the concept of keys in Flutter and when you might use them?
A good answer should demonstrate understanding that keys in Flutter are used to preserve state when widgets move around in the widget tree. Candidates should explain that keys are particularly useful when working with lists of widgets that can change dynamically, such as in a ListView or when animating widgets.
Look for responses that can provide specific examples of when keys are necessary. For instance, they might mention using keys when reordering items in a list to ensure Flutter can correctly identify which widget is which. Candidates should also be able to differentiate between different types of keys like ValueKey, ObjectKey, and GlobalKey, and explain scenarios where each might be appropriate.
6. How would you optimize the performance of a Flutter app?
Strong candidates should be able to discuss multiple strategies for optimizing Flutter app performance. They might mention techniques like using const constructors where possible, implementing list view optimizations (such as ListView.builder for long lists), and avoiding unnecessary rebuilds by using setState() judiciously.
Look for answers that demonstrate a holistic approach to performance optimization. Candidates might discuss the importance of profiling the app using Flutter DevTools to identify performance bottlenecks. They should also mention optimizing asset sizes, using appropriate image formats, and implementing efficient state management. Bonus points if they bring up advanced topics like using compute() for expensive operations or custom painters for complex animations.
7. What's your experience with Flutter's widget testing and integration testing?
A comprehensive answer should cover both widget testing and integration testing in Flutter. For widget testing, candidates should be familiar with the flutter_test package and be able to explain how to write tests that interact with widgets, find specific widgets in the tree, and verify their properties or behavior.
For integration testing, look for familiarity with the integration_test package. Candidates should be able to explain how to write tests that interact with the app as a user would, including tapping buttons, entering text, and verifying that the correct screens are displayed. They might also mention the importance of running tests on actual devices or emulators to catch platform-specific issues.
8. How do you approach internationalization (i18n) in Flutter apps?
A strong response should demonstrate familiarity with Flutter's built-in internationalization support. Candidates should mention using the intl package and explain the process of setting up localization delegates and localized string lookups. They might discuss creating arb files for different languages and using the Intl.message() function to define translatable strings.
Look for answers that go beyond just translating text. Candidates should consider other aspects of internationalization, such as handling different date formats, currencies, and text directionality (RTL vs LTR). They might also mention using the device's locale to automatically select the appropriate language and the importance of testing the app in multiple languages to ensure layouts work correctly with varying text lengths.
20 Flutter interview questions to ask junior developers
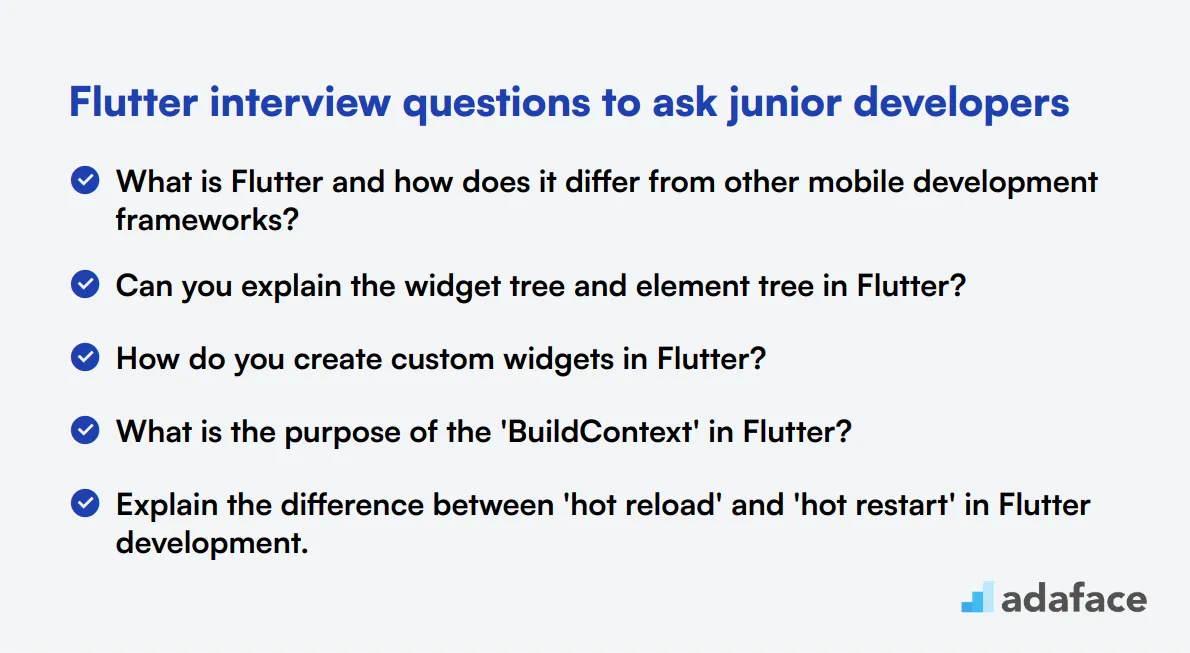
To assess the foundational knowledge of junior Flutter developers, use these 20 interview questions. They cover essential concepts and practical skills, helping you identify candidates with a solid grasp of Flutter basics and potential for growth.
- What is Flutter and how does it differ from other mobile development frameworks?
- Can you explain the widget tree and element tree in Flutter?
- How do you create custom widgets in Flutter?
- What is the purpose of the 'BuildContext' in Flutter?
- Explain the difference between 'hot reload' and 'hot restart' in Flutter development.
- How do you handle user input in Flutter?
- What are Flutter packages and how do you use them in your projects?
- Can you describe the Flutter app lifecycle?
- How do you implement navigation in a Flutter app?
- What is the purpose of the 'pubspec.yaml' file in a Flutter project?
- How do you handle exceptions in Flutter?
- What are mixins in Dart and how are they used in Flutter?
- Can you explain what Flutter streams are and provide an example of their use?
- How do you implement animations in Flutter?
- What is the difference between 'SizedBox' and 'Container' widgets?
- How do you access platform-specific features in Flutter?
- Can you explain what Flutter's 'InheritedWidget' is used for?
- How do you implement form validation in Flutter?
- What is the purpose of the 'async' and 'await' keywords in Dart?
- How do you handle device orientation changes in a Flutter app?
10 intermediate Flutter interview questions and answers to ask mid-tier developers
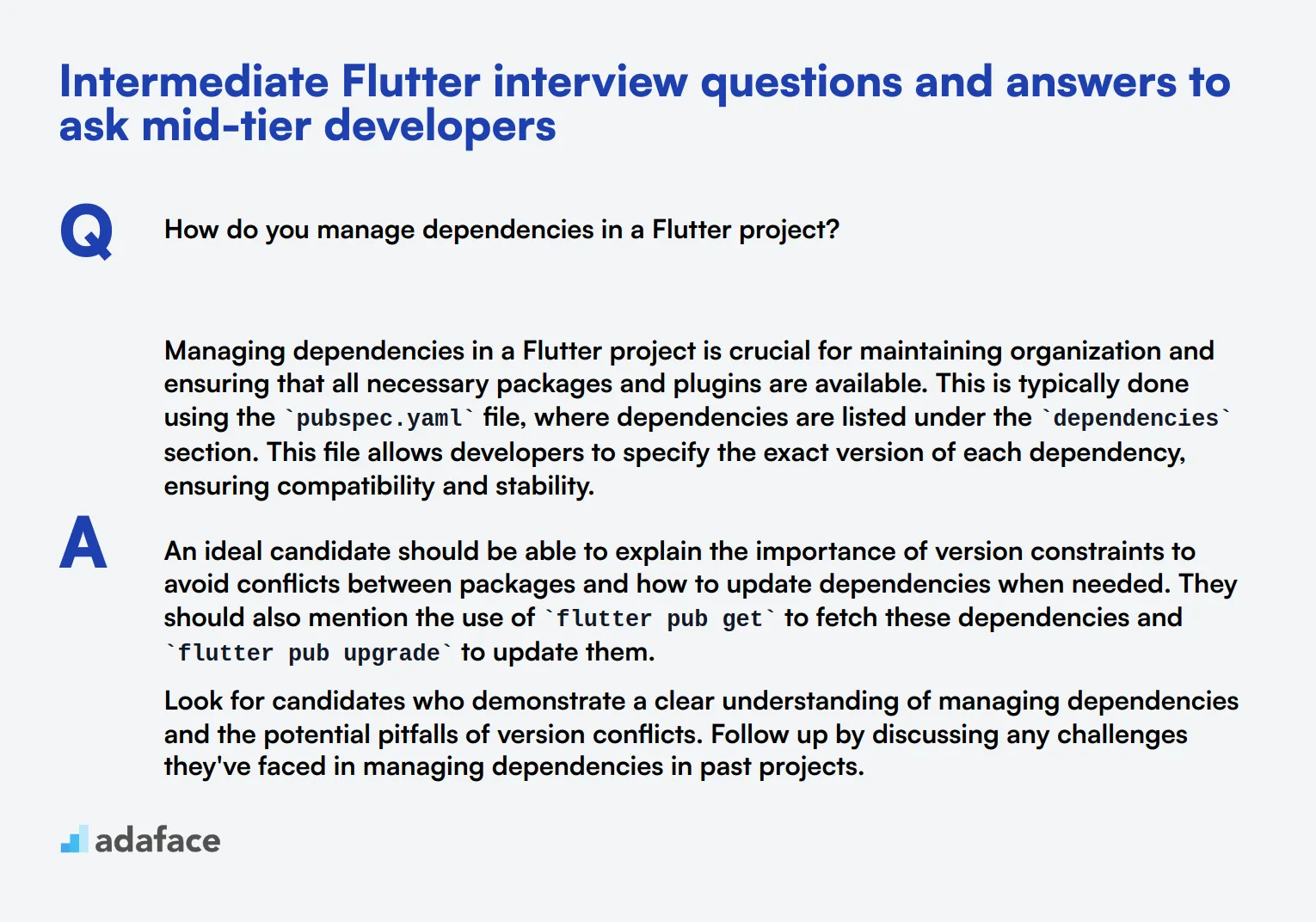
To determine whether your mid-tier Flutter developers possess the necessary skills and knowledge, ask them some of these 10 intermediate Flutter interview questions. These questions are designed to assess their problem-solving abilities and understanding of Flutter's more nuanced features, ensuring they can handle complex tasks in your projects.
1. How do you manage dependencies in a Flutter project?
Managing dependencies in a Flutter project is crucial for maintaining organization and ensuring that all necessary packages and plugins are available. This is typically done using the pubspec.yaml
file, where dependencies are listed under the dependencies
section. This file allows developers to specify the exact version of each dependency, ensuring compatibility and stability.
An ideal candidate should be able to explain the importance of version constraints to avoid conflicts between packages and how to update dependencies when needed. They should also mention the use of flutter pub get
to fetch these dependencies and flutter pub upgrade
to update them.
Look for candidates who demonstrate a clear understanding of managing dependencies and the potential pitfalls of version conflicts. Follow up by discussing any challenges they've faced in managing dependencies in past projects.
2. Can you explain how you would handle persistent data storage in a Flutter app?
Persistent data storage in Flutter can be handled using various methods such as shared preferences, SQLite, and third-party packages like Hive or Moor. Shared preferences are often used for simple key-value pairs, while SQLite is suitable for more complex relational data.
An ideal candidate should discuss the use of shared preferences for storing small amounts of data like user settings, and SQLite or NoSQL solutions for handling more extensive data requirements. They should also touch on the importance of choosing the right storage solution based on the app's needs.
Look for candidates who can clearly differentiate between different storage solutions and provide reasons for their choices. Follow up by asking about any specific projects where they've implemented persistent storage.
3. How do you manage asynchronous operations in Flutter?
Managing asynchronous operations in Flutter typically involves the use of async
and await
keywords, along with Future
and Stream
classes. These tools help handle operations like HTTP requests, file I/O, and database access without blocking the main thread.
An ideal candidate should explain how async
and await
facilitate writing asynchronous code that looks and behaves like synchronous code. They should also discuss the use of FutureBuilder
and StreamBuilder
widgets for building UI components that depend on asynchronous data.
Look for candidates who can clearly articulate the importance of asynchronous programming and provide examples of how they've managed async operations in their projects. Follow up by discussing any challenges they've faced with async code.
4. What is your approach to debugging in Flutter?
Debugging in Flutter can be done using various tools such as the Flutter DevTools, the Dart analyzer, and the built-in debugging capabilities of IDEs like Android Studio and Visual Studio Code. These tools help identify and fix issues in the code.
An ideal candidate should mention techniques like using breakpoints, logging, and inspecting the widget tree. They should also discuss how they use the Flutter DevTools to monitor performance and track down memory leaks.
Look for candidates who demonstrate a methodical approach to debugging and can provide examples of how they've resolved complex issues in their projects. Follow up by asking about any specific debugging tools they prefer.
5. How do you handle network connectivity issues in a Flutter app?
Handling network connectivity issues in a Flutter app involves checking the network status and managing offline states. This can be done using packages like connectivity
and connectivity_plus
, which help monitor the network connection.
An ideal candidate should explain how they use these packages to check connectivity status and handle scenarios where the network is unavailable. They should also discuss strategies like caching data locally and providing user feedback when connectivity issues occur.
Look for candidates who can articulate their approach to managing network connectivity and provide examples of how they've handled such issues in their projects. Follow up by asking about any challenges they've faced with network reliability.
6. What is your experience with using Flutter for web development?
Using Flutter for web development involves leveraging the same codebase to create applications that run on the web. This cross-platform capability allows developers to target web, mobile, and desktop platforms with a single codebase.
An ideal candidate should discuss their experience with adapting Flutter apps for the web, including handling responsive design and ensuring compatibility across different browsers. They should also mention any challenges they've faced, such as optimizing performance and managing different screen sizes.
Look for candidates who can provide specific examples of their work with Flutter for web and demonstrate an understanding of the unique challenges involved. Follow up by asking about any tools or libraries they use to facilitate web development.
7. How do you manage app permissions in a Flutter project?
Managing app permissions in a Flutter project involves requesting and handling permissions for accessing device features like camera, location, and storage. This can be done using packages like permission_handler
.
An ideal candidate should explain how they use the permission_handler
package to request permissions at runtime and handle user responses. They should also discuss strategies for providing a seamless user experience when permissions are denied.
Look for candidates who demonstrate a clear understanding of managing app permissions and can provide examples of how they've handled permissions in their projects. Follow up by asking about any challenges they've faced with app permissions.
8. Can you describe your approach to error handling in a Flutter app?
Error handling in a Flutter app involves catching and managing exceptions to prevent crashes and provide a better user experience. This can be done using try-catch
blocks, custom error messages, and error reporting tools.
An ideal candidate should discuss their approach to catching exceptions, displaying user-friendly error messages, and logging errors for further analysis. They should also mention the importance of handling both synchronous and asynchronous errors.
Look for candidates who can clearly explain their error handling strategies and provide examples of how they've managed errors in their projects. Follow up by asking about any specific tools or libraries they use for error handling.
9. How do you handle background tasks in a Flutter app?
Handling background tasks in a Flutter app involves using packages like background_fetch
and workmanager
to perform tasks even when the app is not in the foreground. These tasks can include fetching data, sending notifications, and performing scheduled operations.
An ideal candidate should explain how they use these packages to schedule and manage background tasks, including handling platform-specific limitations and optimizing battery usage. They should also discuss strategies for ensuring that background tasks do not negatively impact the user experience.
Look for candidates who demonstrate a clear understanding of managing background tasks and can provide examples of how they've implemented such tasks in their projects. Follow up by asking about any challenges they've faced with background task management.
10. What strategies do you use to ensure code quality in your Flutter projects?
Ensuring code quality in Flutter projects involves following best practices, using code linters, writing tests, and conducting code reviews. These strategies help maintain a high standard of code and prevent issues from arising in production.
An ideal candidate should discuss their use of tools like flutter analyze
for static code analysis, writing unit and integration tests, and following coding standards. They should also emphasize the importance of regular code reviews and continuous integration to catch issues early.
Look for candidates who can clearly explain their approach to maintaining code quality and provide examples of how they've implemented these strategies in their projects. Follow up by asking about any specific tools or practices they use to ensure code quality.
15 advanced Flutter interview questions to ask senior developers
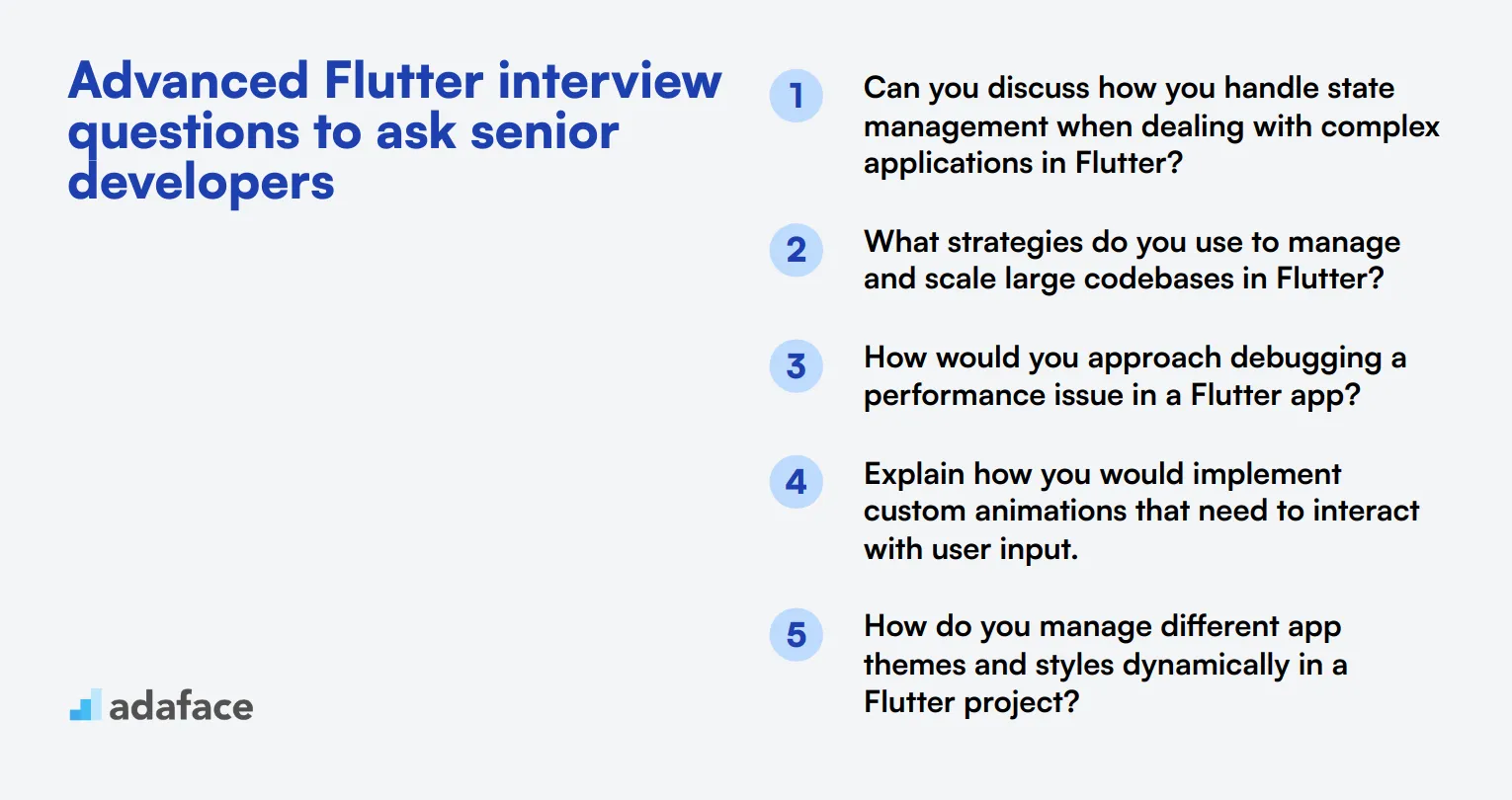
To ensure you hire a senior Flutter developer with the right expertise, use these advanced Flutter interview questions. These questions are designed to assess a candidate's depth of knowledge and problem-solving skills. For more insights on what to look for in a developer, check out our Flutter developer job description.
- Can you discuss how you handle state management when dealing with complex applications in Flutter?
- What strategies do you use to manage and scale large codebases in Flutter?
- How would you approach debugging a performance issue in a Flutter app?
- Explain how you would implement custom animations that need to interact with user input.
- How do you manage different app themes and styles dynamically in a Flutter project?
- What are your best practices for ensuring high code quality and maintainability in Flutter?
- Describe how you would handle offline functionality and data synchronization in a Flutter app.
- Can you explain how to use and manage different build flavors in Flutter?
- What is your approach to integrating third-party libraries and plugins into a Flutter project?
- How do you test and ensure the reliability of asynchronous code in Flutter?
- Can you describe a challenging problem you faced in Flutter development and how you solved it?
- How do you implement and manage security best practices in a Flutter app?
- What techniques do you use for optimizing the rendering performance of custom widgets?
- Describe your experience with Continuous Integration/Continuous Deployment (CI/CD) in Flutter projects.
- How do you approach refactoring legacy code in an existing Flutter application?
7 Flutter interview questions and answers related to state management
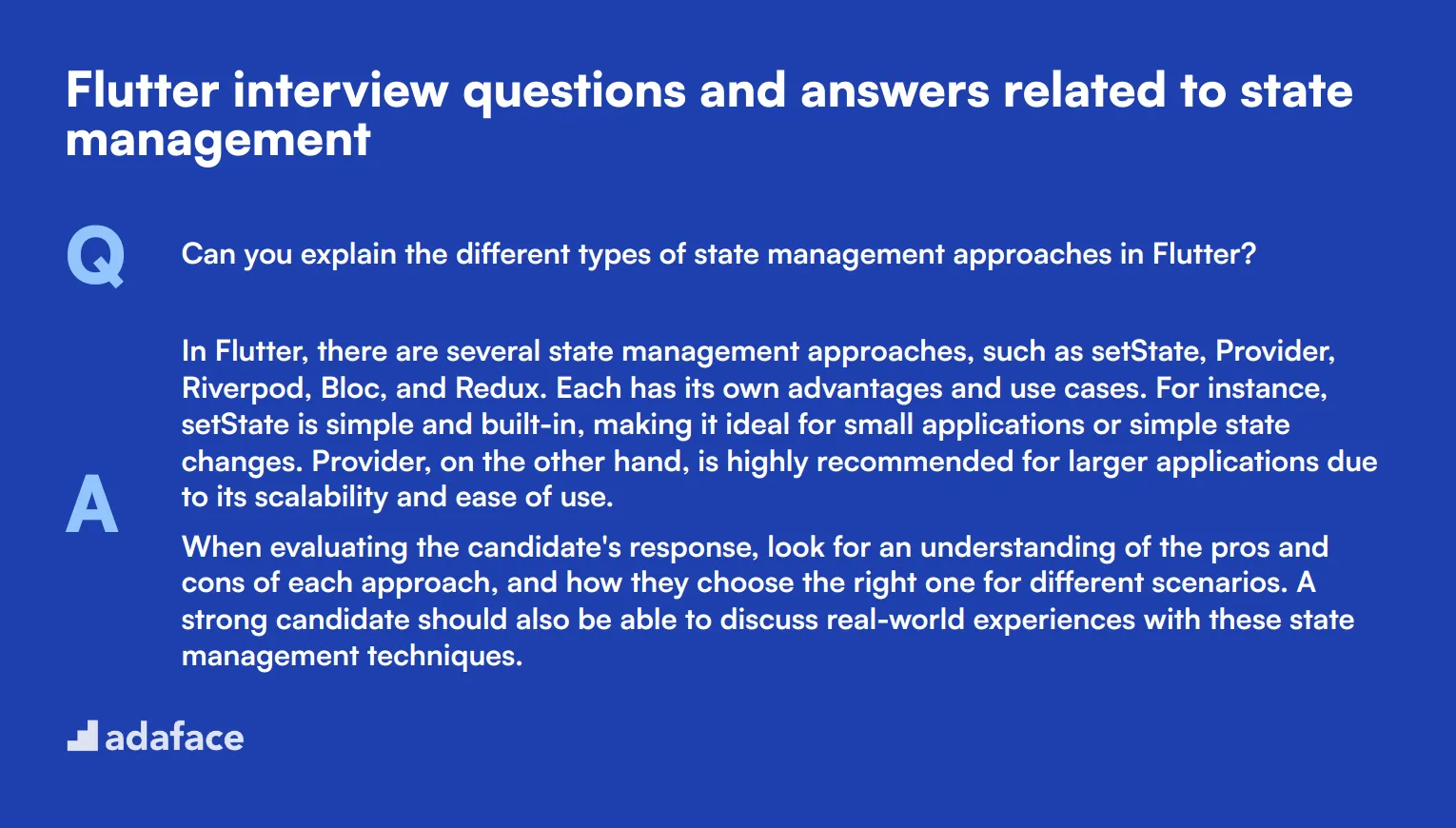
To determine whether your applicants have a firm grasp on state management in Flutter, use these 7 interview questions. These questions will help you identify candidates who can efficiently manage state in a Flutter application, ensuring smooth and responsive user experiences.
1. Can you explain the different types of state management approaches in Flutter?
In Flutter, there are several state management approaches, such as setState, Provider, Riverpod, Bloc, and Redux. Each has its own advantages and use cases. For instance, setState is simple and built-in, making it ideal for small applications or simple state changes. Provider, on the other hand, is highly recommended for larger applications due to its scalability and ease of use.
When evaluating the candidate's response, look for an understanding of the pros and cons of each approach, and how they choose the right one for different scenarios. A strong candidate should also be able to discuss real-world experiences with these state management techniques.
2. How do you decide when to use a global state versus a local state in a Flutter app?
Global state management is used when you need to share state across multiple parts of the application, such as user authentication status or theme settings. Local state, on the other hand, is suitable for managing temporary or UI-specific states like form inputs or an individual widget’s state.
An ideal candidate will be able to provide examples of when they have used both global and local state management effectively. They should demonstrate a clear understanding of the implications of each approach on the app's performance and maintainability.
3. What are the potential pitfalls of improper state management in Flutter, and how can they be avoided?
Improper state management can lead to issues like redundant rebuilds, memory leaks, and poor performance. These issues can degrade the user experience significantly. To avoid these pitfalls, it’s crucial to choose the right state management approach and follow best practices such as avoiding unnecessary rebuilds, properly disposing of controllers, and using efficient state management solutions.
Look for answers that show the candidate has practical experience with these challenges and has implemented strategies to mitigate them. A strong candidate will also mention the importance of profiling and debugging tools to monitor and optimize state management.
4. Can you describe how you would use Provider for state management in a Flutter project?
Provider is a popular state management solution in Flutter that allows for easy and efficient state sharing across the widget tree. It works by using ChangeNotifier to notify listeners when state changes. The Provider package includes tools for dependency injection and state management, making it a comprehensive solution for many applications.
Candidates should explain the basic workflow: creating a ChangeNotifier class to hold the state, providing it to the widget tree using the Provider widget, and then accessing it in child widgets using context.watch or context.read. Look for candidates who can articulate the benefits of using Provider, such as improved code organization and reduced boilerplate.
5. How do you manage and test complex state management logic in Flutter?
Managing complex state management logic in Flutter often involves breaking down the state into smaller, manageable parts and using robust state management solutions like Bloc or Redux. Testing involves writing unit tests to verify individual pieces of logic and integration tests to ensure different parts work together as expected.
A candidate’s response should highlight their experience with writing tests for state management logic, including how they use mock data and dependency injection to isolate the logic under test. Strong candidates will also discuss their approach to continuous integration and how it helps maintain code quality.
6. How would you handle state management in a Flutter app that requires real-time updates?
For real-time updates, it’s essential to use state management solutions that can handle frequent changes efficiently. Solutions like StreamBuilder with a combination of Provider or Bloc can be used to listen to real-time data streams and update the UI accordingly.
Evaluate whether the candidate understands how to implement and manage streams, including ensuring that listeners are properly disposed of to avoid memory leaks. A strong candidate will also mention their experience with handling real-time data in past projects and the challenges they faced.
7. What is the role of ChangeNotifier in Flutter state management, and how do you use it?
ChangeNotifier is a class provided by Flutter that allows objects to notify listeners when a change occurs. It's a core component in the Provider package and is used to manage state by extending ChangeNotifier and calling notifyListeners() whenever the state changes.
When assessing the candidate’s answer, look for a clear explanation of how they have implemented ChangeNotifier in their projects. They should discuss how they structure their state management logic and ensure that listeners are efficiently managed. A strong candidate will also mention any challenges they’ve faced and how they overcame them.
9 Flutter interview questions and answers related to widget lifecycle
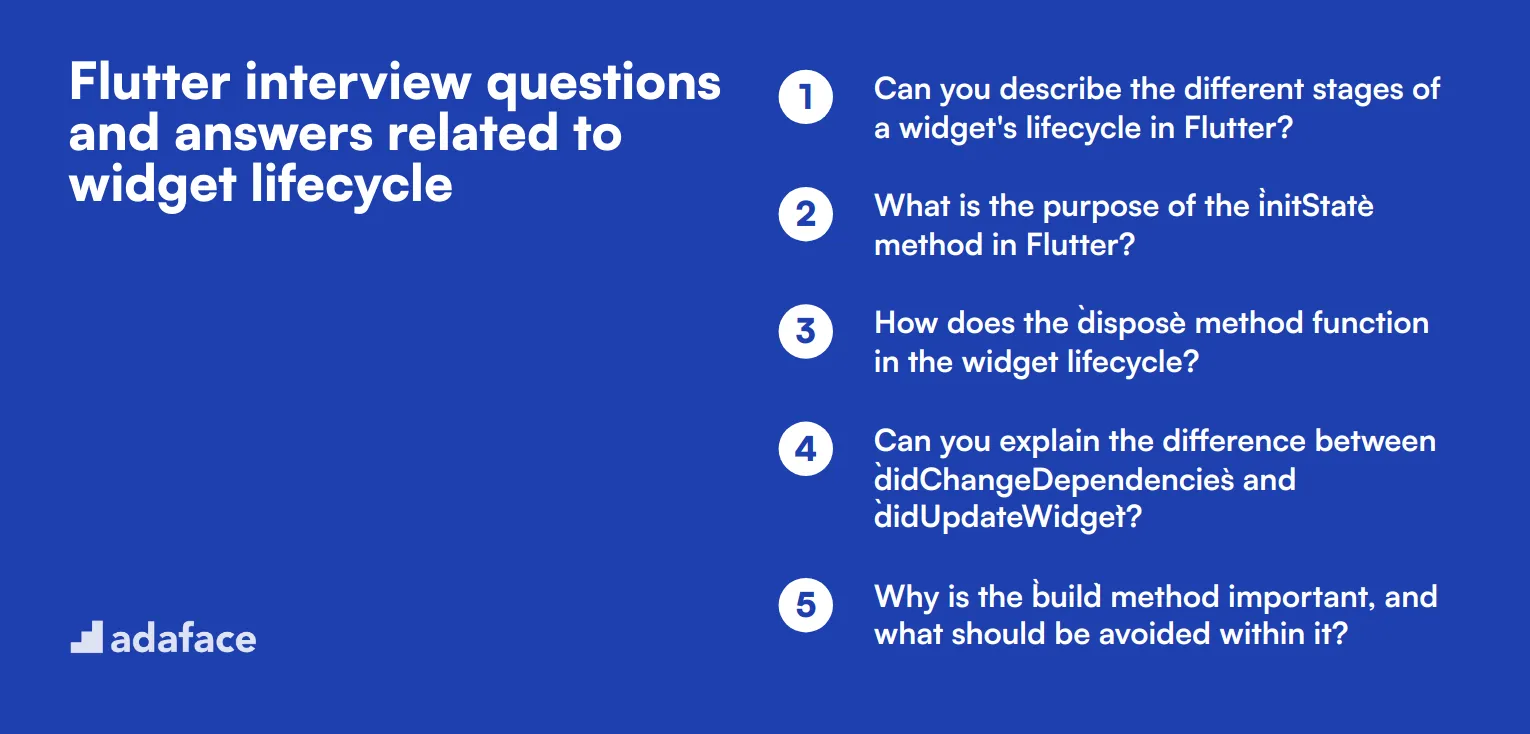
To assess a candidate's understanding of the widget lifecycle in Flutter, these questions are your go-to resource. Understanding the widget lifecycle is crucial for developers to build efficient and responsive Flutter applications. Use these questions to delve into the candidate's practical knowledge and experience.
1. Can you describe the different stages of a widget's lifecycle in Flutter?
In Flutter, a widget goes through several stages in its lifecycle. Initially, the widget is created with the createState
method. This is followed by the initState
method, where you can perform one-time initializations. The didChangeDependencies
method is called after initState
and whenever the widget's dependencies change.
During the build phase, the build
method is called, producing the widget's visual representation. The widget may then go through updates via the setState
method. Finally, the dispose
method is invoked when the widget is removed from the tree, allowing for cleanup tasks.
Look for candidates who can clearly explain each stage and provide examples of when and why each method is used. Their response should demonstrate a solid grasp of the widget lifecycle, showing they can manage state and resources efficiently.
2. What is the purpose of the `initState` method in Flutter?
The initState
method is a crucial part of a widget's lifecycle in Flutter. It is called once when the state object is created, before the build
method. This is the ideal place to perform one-time initializations, such as setting up animations, fetching data, or initializing state variables.
Unlike the build
method, which can be called multiple times, initState
is called only once, ensuring that the initial setup is done efficiently and without redundancy.
Candidates should demonstrate an understanding of when to use initState
and provide examples of typical tasks performed in this method. Look for clarity in their explanation and practical knowledge of its use.
3. How does the `dispose` method function in the widget lifecycle?
The dispose
method is called when a widget is removed from the widget tree permanently. This method is used to clean up any resources that the widget was using, such as closing streams, canceling timers, or disposing of controllers.
Proper use of the dispose
method ensures that your app does not leak memory and keeps performance optimal by releasing resources that are no longer needed.
An ideal candidate should explain the importance of resource management and provide scenarios where failing to implement dispose
could lead to performance issues or memory leaks.
4. Can you explain the difference between `didChangeDependencies` and `didUpdateWidget`?
didChangeDependencies
is called after initState
and whenever the dependencies of the widget change. This is typically used when you rely on data from an InheritedWidget
and want to react to changes in that data.
didUpdateWidget
is called whenever the parent widget rebuilds and passes new configurations to the widget. This method allows you to update the state based on the new widget properties.
Candidates should articulate the specific use cases for each method and provide examples to illustrate how they have handled dependency changes or updates in their projects. This shows they understand the dynamic nature of Flutter's widget tree.
5. Why is the `build` method important, and what should be avoided within it?
The build
method is essential as it describes how to display the widget in the UI. It is called whenever the widget needs to be rendered or re-rendered, making it a central part of the widget lifecycle.
However, it is crucial to avoid performing heavy computations or long-running tasks inside the build
method, as this can lead to performance issues. Instead, these tasks should be done outside the build
method, such as in initState
, asynchronous calls, or separate methods.
Look for candidates who emphasize the importance of keeping the build
method efficient and can suggest best practices for managing performance. Their insights into avoiding pitfalls here are vital for building responsive and efficient applications.
6. What happens when `setState` is called, and why is it important?
Calling setState
in Flutter triggers a rebuild of the widget, allowing the UI to reflect changes in the state. This is crucial for maintaining a dynamic and interactive user interface.
When setState
is called, it schedules a build and updates the UI based on the new state. It is important to use setState
correctly to ensure that only the affected parts of the UI are rebuilt, keeping the application performant.
Candidates should articulate why and when to use setState
, providing examples of how they manage state changes in their projects. Look for a clear understanding of its importance in maintaining UI consistency and performance.
7. How do you handle stateful updates efficiently in the widget lifecycle?
Efficient stateful updates in Flutter involve using setState
judiciously to minimize unnecessary rebuilds. Breaking down the UI into smaller, reusable widgets can help isolate state changes to specific parts of the widget tree.
Using state management solutions like Provider or Riverpod can also enhance efficiency by decoupling state from the UI and providing more granular control over state updates.
Candidates should discuss strategies they use to manage state efficiently, such as leveraging state management libraries and structuring their widget tree to optimize performance. Look for practical examples and a thoughtful approach to state management.
8. What are some common mistakes to avoid in the widget lifecycle, and how can they impact the app?
Common mistakes include performing heavy computations in the build
method, not cleaning up resources in the dispose
method, and misusing setState
leading to unnecessary rebuilds.
These mistakes can lead to performance issues, memory leaks, and a sluggish user experience. Properly managing the widget lifecycle is crucial for building responsive and efficient Flutter applications.
Candidates should identify these pitfalls and suggest best practices to avoid them. Look for an understanding of the impact of these mistakes and a proactive approach to ensuring optimal app performance.
9. How would you debug issues related to the widget lifecycle in Flutter?
Debugging widget lifecycle issues involves using Flutter's built-in tools like the Flutter DevTools, which provide insights into the widget tree and lifecycle events. Adding logging statements in lifecycle methods can also help trace the flow of execution.
Understanding the common lifecycle methods and their sequence is essential for identifying where things might be going wrong. Using breakpoints and stepping through code in an IDE like Android Studio or Visual Studio Code can also be effective.
Candidates should discuss their debugging strategies and tools they use to diagnose and fix lifecycle-related issues. Look for a systematic approach and familiarity with Flutter's debugging capabilities.
8 situational Flutter interview questions with answers for hiring top developers
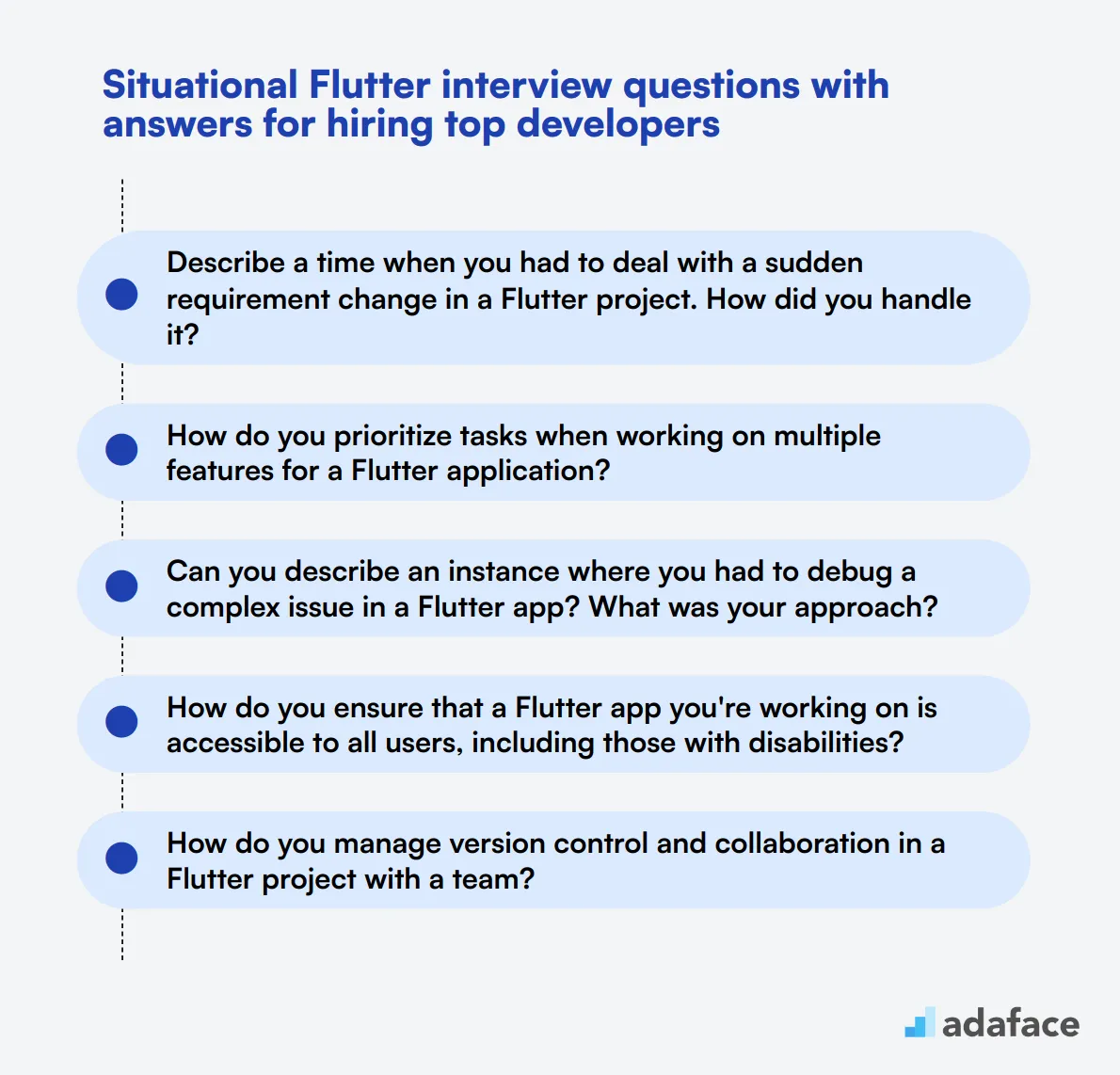
To identify top Flutter developers, use these situational questions to gauge how candidates tackle real-world challenges. These questions are perfect for diving deeper into a developer's problem-solving skills and practical experience.
1. Describe a time when you had to deal with a sudden requirement change in a Flutter project. How did you handle it?
A sudden requirement change can be a common scenario in software development. An ideal candidate would explain how they first assessed the new requirement's impact on the project timeline and existing code. They should also mention their communication strategy with stakeholders to realign expectations.
Look for candidates who can show adaptability and problem-solving skills. They should be able to present a clear strategy on how they managed the change without compromising the quality of the project.
2. How do you prioritize tasks when working on multiple features for a Flutter application?
Prioritization is crucial when juggling multiple features. A thoughtful response might include using methodologies like Agile or Kanban to manage tasks effectively. They might prioritize based on feature dependencies, deadlines, or user impact.
An ideal candidate should demonstrate a structured approach to task management and the ability to balance short-term and long-term goals. Look for examples of tools or techniques they use to stay organized.
3. Can you describe an instance where you had to debug a complex issue in a Flutter app? What was your approach?
Debugging complex issues requires a systematic approach. Candidates might explain how they reproduced the issue, isolated the problem area, and used Flutter's debugging tools to identify the root cause. They might also mention collaborating with team members to gather different perspectives.
Look for candidates who can articulate a clear, logical debugging process. Their response should reflect critical thinking and effective use of debugging tools.
4. How do you ensure that a Flutter app you're working on is accessible to all users, including those with disabilities?
Accessibility is a key aspect of app development. Candidates might discuss checking for color contrast, using semantic widgets, and enabling screen reader support. They may also mention conducting usability tests with diverse user groups.
The ideal response should display an understanding of accessibility best practices and a commitment to creating inclusive applications. Look for specific examples of how they've implemented accessibility features in past projects.
5. How do you manage version control and collaboration in a Flutter project with a team?
Effective version control is essential for team collaboration. Candidates might talk about using Git for version control, setting up a branching strategy, and conducting code reviews through pull requests. They may also mention using platforms like GitHub or Bitbucket.
An ideal candidate should show proficiency in version control systems and the ability to collaborate seamlessly with a team. Their answer should reflect good practices in maintaining code integrity and facilitating team communication.
6. Tell me about a time when you had to optimize an existing Flutter app for better performance. What steps did you take?
Optimizing performance often starts with identifying performance bottlenecks. Candidates might mention profiling the app to find issues, optimizing build times, reducing widget rebuilds, and using lightweight widgets. They may also talk about lazy loading images or minimizing the use of expensive operations.
The ideal response should showcase a substantial understanding of performance optimization techniques in Flutter. Look for specific examples of how they've achieved noticeable performance improvements in their projects.
7. Describe a situation where you had to integrate a third-party library or plugin into a Flutter app. What challenges did you face and how did you overcome them?
Integrating third-party libraries can sometimes be challenging. Candidates might discuss examining the library's documentation, ensuring compatibility with existing code, and handling any conflicts or issues during integration. They may also mention testing the integration thoroughly to ensure stability.
Look for candidates who can demonstrate problem-solving skills and the ability to quickly adapt to and troubleshoot third-party integrations. Their response should reflect practical experience and an understanding of best practices in integration.
8. How do you handle user feedback and bug reports for a Flutter application you have developed?
Handling user feedback and bug reports effectively is crucial for maintaining an app. Candidates might explain how they use tools like issue trackers to collect and prioritize feedback. They may also mention conducting root cause analysis for reported bugs and releasing timely updates to address them.
The ideal response should reflect a systematic approach to managing user feedback and maintaining app quality. Look for candidates who emphasize the importance of continuous improvement and user satisfaction.
Which Flutter skills should you evaluate during the interview phase?
While it's impossible to fully assess a candidate's capabilities in a single interview, focusing on key skills can provide significant insights. For Flutter developers, certain core skills are indispensable for gauging their proficiency and fit for the role.
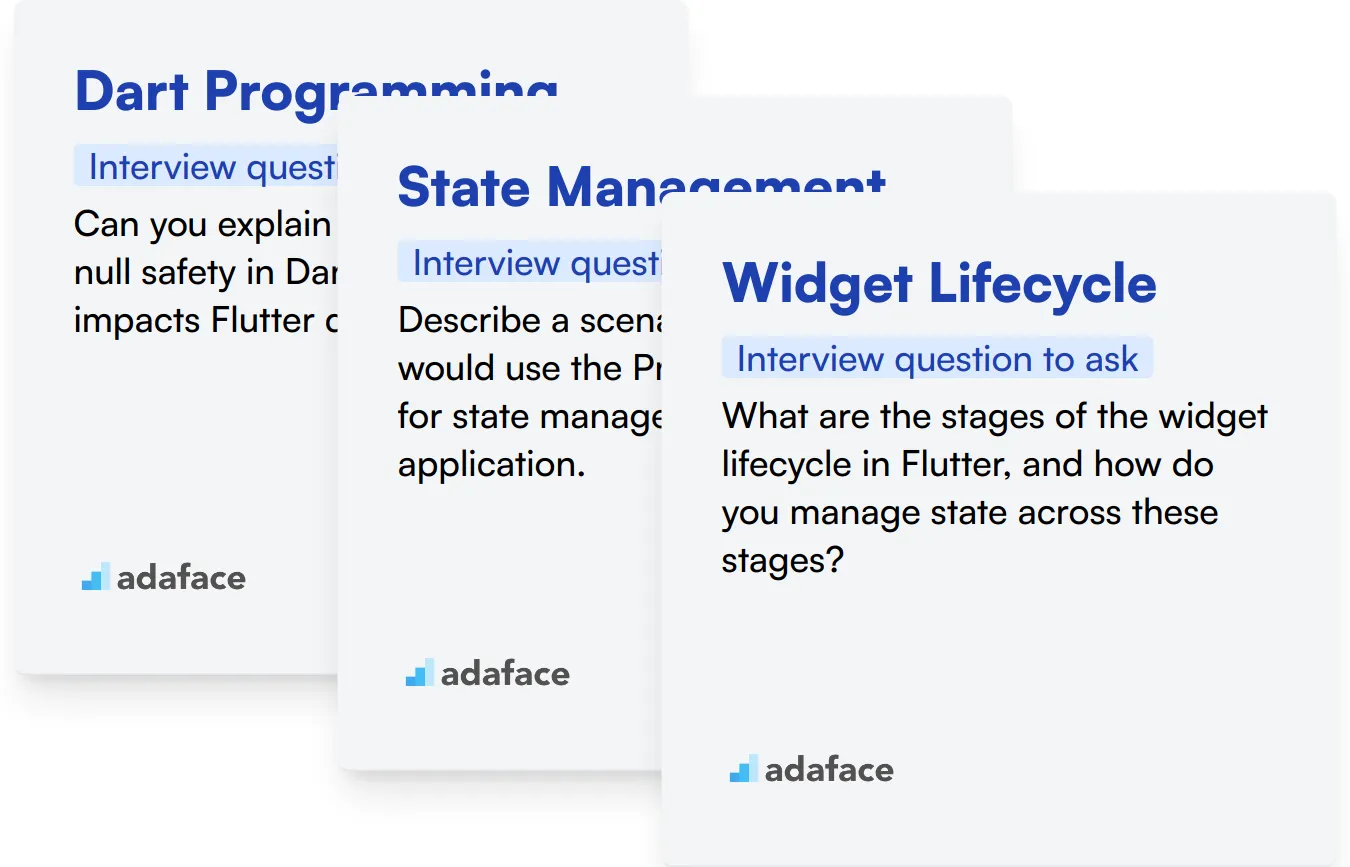
Dart Programming
Dart is the programming language used exclusively for Flutter development. A strong grasp of Dart is necessary as it forms the backbone of creating seamless and responsive applications on the Flutter framework.
To effectively assess this skill, consider using a Dart-specific test to filter candidates proficient in Dart. Our Dart test can streamline this process seamlessly.
You can also evaluate this competency through targeted interview questions that delve into foundational Dart concepts.
Can you explain the concept of null safety in Dart and how it impacts Flutter development?
Look for a candidate's understanding of type safety features in Dart and their ability to apply these concepts to enhance application robustness and maintenance in Flutter.
State Management
Effective state management is crucial in Flutter to ensure applications behave predictably as they scale. It involves managing the state of widgets when the data changes over time.
Consider integrating questions on state management into your assessment to evaluate this expertise. While we do not offer a specific test for this, tailored MCQs in the Flutter test can cover this aspect.
In addition, questioning candidates directly on this topic can reveal their practical understanding and approach.
Describe a scenario where you would use the Provider package for state management in a Flutter application.
The answer should demonstrate the candidate's decision-making process regarding state management solutions and their ability to implement these effectively in Flutter.
Widget Lifecycle
Understanding the widget lifecycle is key for optimizing the performance of Flutter applications. It helps developers manage resources efficiently and render UI effectively.
To assess candidates on this, ask specific questions that reveal their knowledge and experience with the widget lifecycle.
What are the stages of the widget lifecycle in Flutter, and how do you manage state across these stages?
Expect detailed explanations on each stage—creation, updating, and disposal—and how the candidate handles state transitions to maintain performance and user experience.
Hire the Best Flutter Developers with Adaface
If you're looking to hire someone with Flutter skills, you need to ensure they meet your technical requirements.
The best way to do this is by using skill tests. You can use our Flutter Test to assess candidates' Flutter expertise.
After administering this test, you can shortlist the top applicants and call them for interviews.
To get started, you can sign up here or explore our test library for more information.
Flutter & Dart Test
Download Flutter interview questions template in multiple formats
Flutter Interview Questions FAQs
The questions cover junior, mid-tier, and senior Flutter developer skill levels, as well as specific topics like state management and widget lifecycle.
The post includes 8 situational Flutter interview questions with answers to help assess candidates in real-world scenarios.
Yes, the post includes 20 Flutter interview questions tailored for junior developers.
Yes, there are 7 Flutter interview questions and answers related to state management.
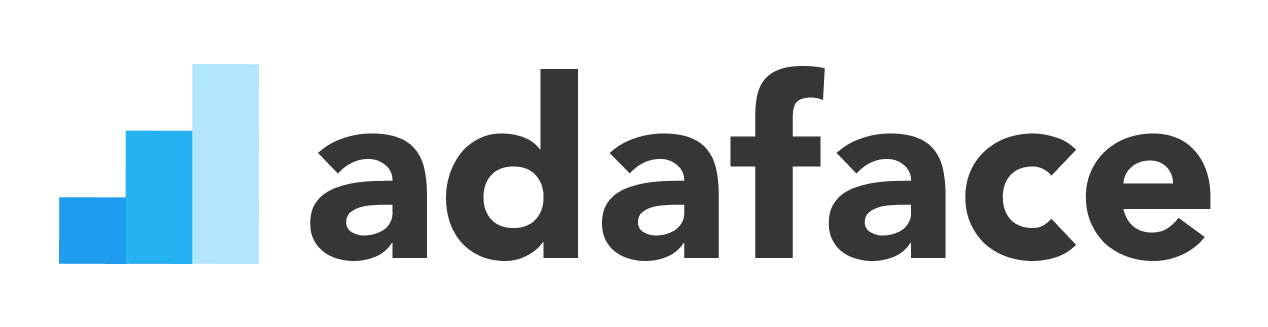
40 min skill tests.
No trick questions.
Accurate shortlisting.
We make it easy for you to find the best candidates in your pipeline with a 40 min skills test.
Try for freeRelated posts
Free resources
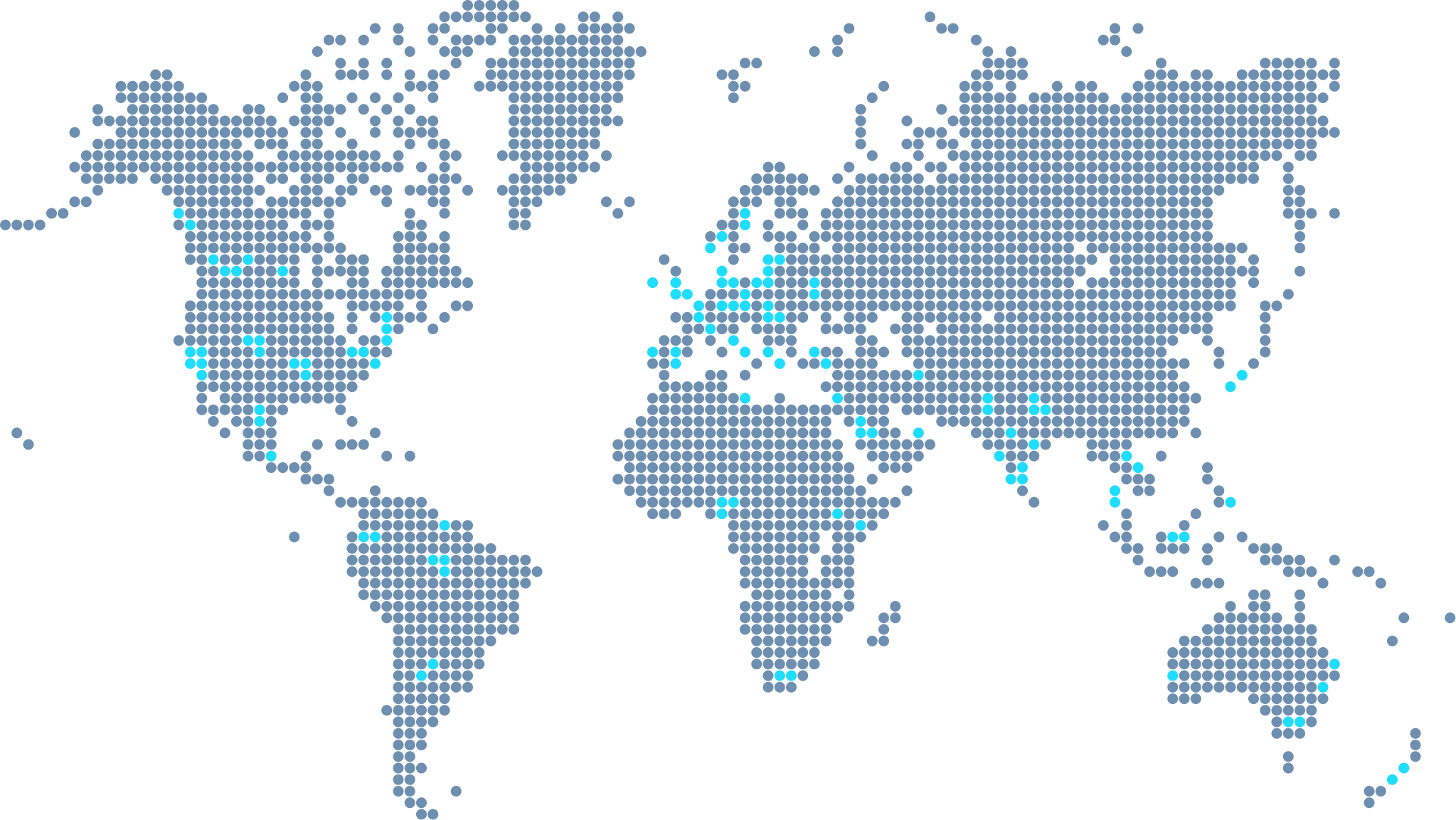
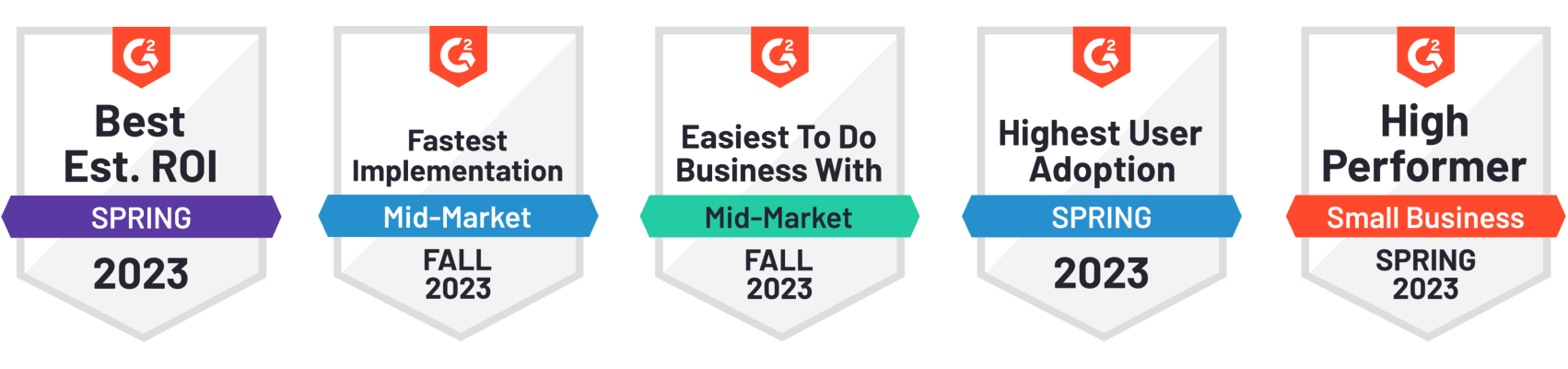