Hiring the right Flask developer can be a game-changer for your web development projects. As Flask continues to gain popularity for its simplicity and flexibility, it's crucial to ask the right questions during interviews to assess candidates' Flask expertise effectively.
This blog post provides a comprehensive list of Flask interview questions tailored for different experience levels, from junior to senior developers. We've also included questions focused on specific areas like routing and testing to help you evaluate candidates' in-depth knowledge.
By using these questions, you'll be able to identify top Flask talent and make informed hiring decisions. Consider complementing your interview process with a Python skills assessment to get a well-rounded view of candidates' capabilities.
Table of contents
7 general Flask interview questions and answers to assess candidates
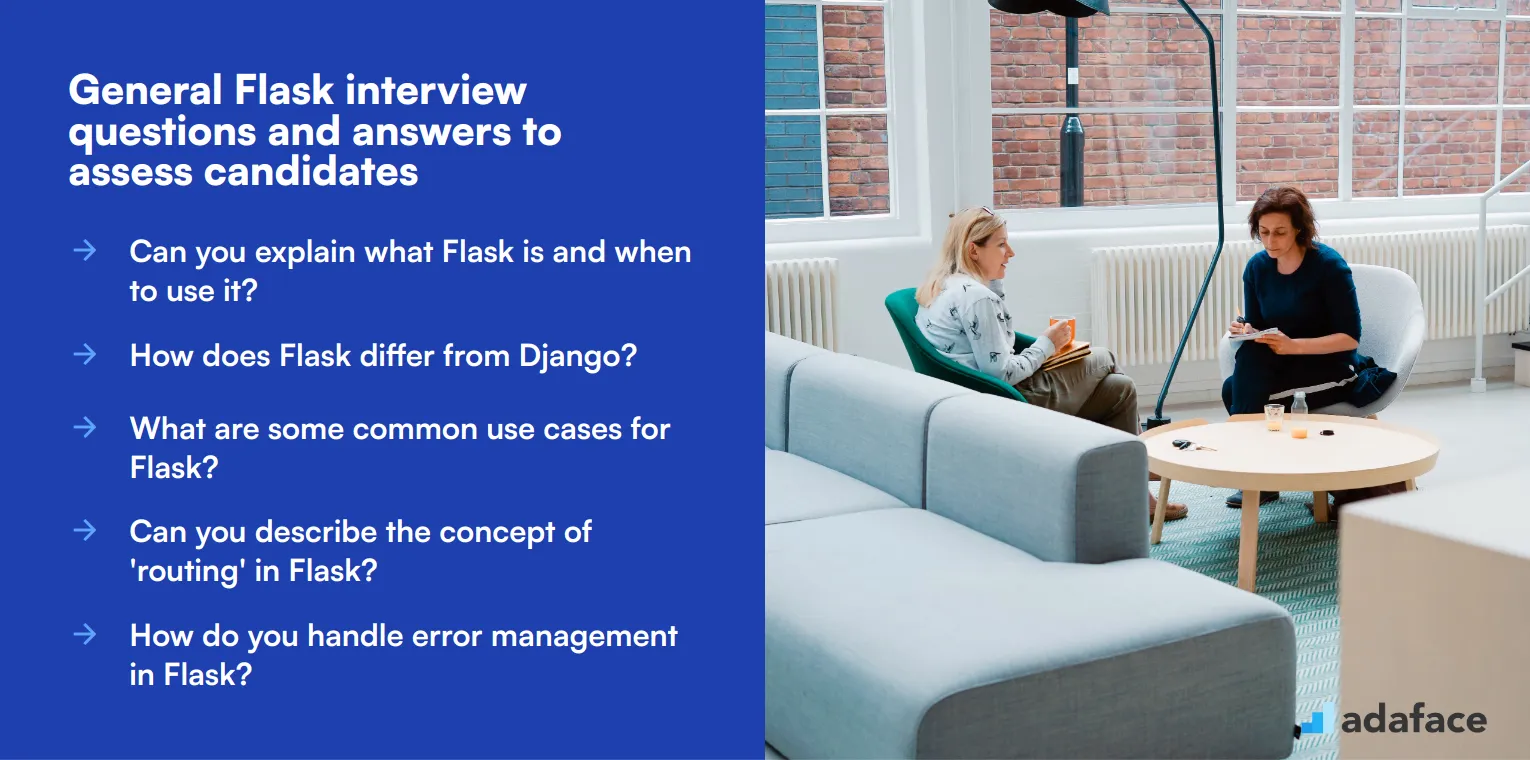
To assess whether your candidates have a solid grasp of Flask fundamentals, here are seven essential questions for your interview toolkit. These questions will help you discern their practical understanding of Flask and ensure you hire the right talent for your team.
1. Can you explain what Flask is and when to use it?
Flask is a micro web framework for Python. It’s designed to be lightweight and modular, making it perfect for developers who want to build web applications quickly and efficiently without the overhead of more complex frameworks.
An ideal candidate will highlight that Flask is best used for small to medium-sized applications where simplicity and flexibility are key. It’s also great for projects that require a custom stack or have specific requirements that larger frameworks might not accommodate.
Look for candidates who understand Flask’s strengths and can articulate why they would choose it over other frameworks. Follow up by asking for examples of past projects where they have successfully implemented Flask.
2. How does Flask differ from Django?
Flask and Django are both popular Python web frameworks, but they serve different purposes. Flask is minimalistic and gives developers more control and flexibility, while Django is a full-fledged framework that comes with a lot of built-in features and a more rigid structure.
Candidates should mention that Flask is best for projects needing a straightforward approach, whereas Django is suitable for larger applications requiring built-in features like an admin panel, ORM, and authentication system.
A strong response will show a candidate's ability to choose the right tool for the job and their understanding of the trade-offs between using Flask and Django.
3. What are some common use cases for Flask?
Flask is often used for building RESTful APIs, microservices, and prototyping. It’s also suitable for small to medium web applications and projects that need to be quickly deployed.
Candidates may also mention that Flask is ideal for projects where developers want more control over the components and configurations versus using a more opinionated framework.
An ideal answer will include specific examples and demonstrate the candidate’s experience with Flask in various contexts. Follow up by asking them to elaborate on any relevant projects they’ve worked on.
4. Can you describe the concept of 'routing' in Flask?
Routing in Flask refers to the mapping of URLs to functions. When a user accesses a specific URL, Flask calls the associated function to process the request and return a response.
Candidates should explain that routes are defined using decorators and that Flask allows for dynamic routing, enabling the creation of URLs that can accept parameters.
A strong candidate will be able to discuss the importance of routing in web applications and provide examples of how they’ve implemented it. You can link back to further understanding the skills required for web developers.
5. How do you handle error management in Flask?
Flask provides built-in support for handling errors through custom error handlers. Developers can define specific functions to handle different types of errors, such as 404 (Not Found) or 500 (Internal Server Error).
Candidates should mention the use of @app.errorhandler
decorators to create these custom error handling functions and how they can return custom error pages or JSON responses.
Look for responses that demonstrate a proactive approach to error management and a clear understanding of how to implement robust error handling in Flask applications.
6. What is the purpose of `Flask-SQLAlchemy`?
Flask-SQLAlchemy is an extension that simplifies the use of SQLAlchemy with Flask. It provides a set of tools and helpers that make database integration more seamless and less error-prone.
Candidates should explain that Flask-SQLAlchemy offers an ORM layer, allowing developers to work with databases using Python objects instead of raw SQL queries.
An ideal candidate will discuss their experience using Flask-SQLAlchemy and provide examples of how it improved their workflow. Follow up by asking about specific projects where they’ve utilized this extension.
7. How do you manage configurations in a Flask application?
Flask allows configurations to be managed through a variety of methods, such as environment variables, configuration files, or directly within the application code. This flexibility helps tailor the setup to the specific needs of the project.
Candidates should mention common practices like creating a config.py
file, using app.config.from_object()
, or employing environment-specific configurations for development, testing, and production.
Look for candidates who understand the importance of managing configurations effectively and can discuss specific techniques they’ve used in past projects.
20 Flask interview questions to ask junior developers
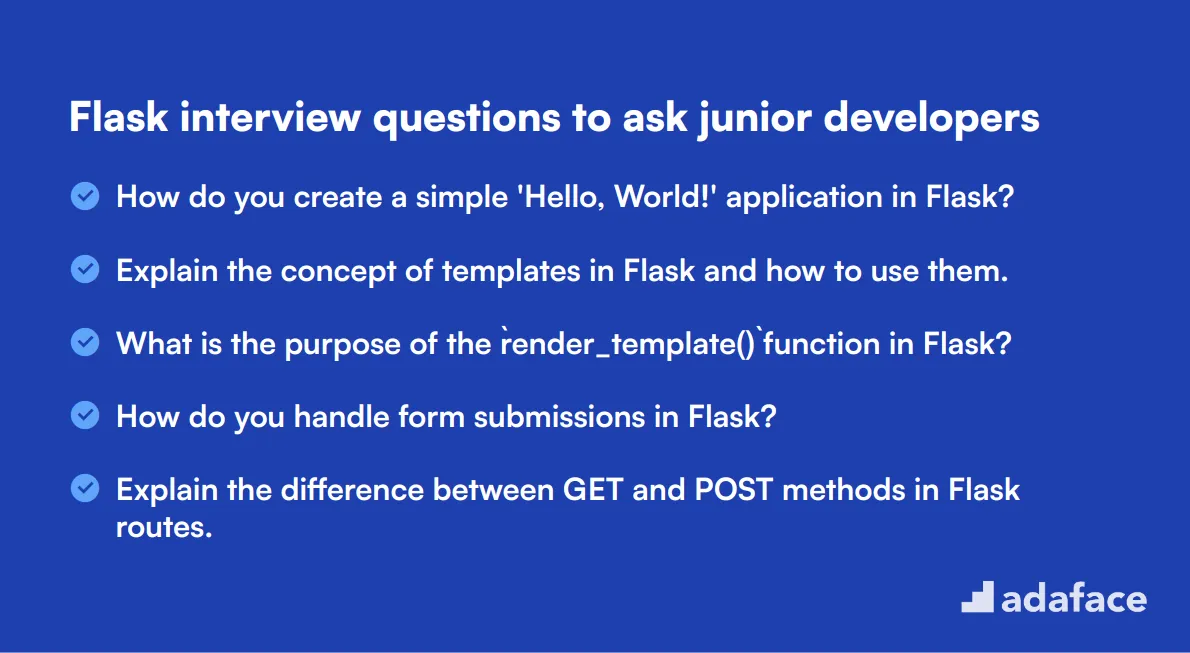
To assess the Flask proficiency of junior web developers, use these 20 interview questions. They cover essential concepts and practical applications, helping you gauge candidates' understanding of Flask basics and their ability to apply them in real-world scenarios.
- How do you create a simple 'Hello, World!' application in Flask?
- Explain the concept of templates in Flask and how to use them.
- What is the purpose of the `render_template()` function in Flask?
- How do you handle form submissions in Flask?
- Explain the difference between GET and POST methods in Flask routes.
- What are Flask extensions and can you name a few commonly used ones?
- How do you set up a database connection in a Flask application?
- Explain the concept of blueprints in Flask and when you might use them.
- How do you implement user authentication in a Flask application?
- What is the purpose of the `request` object in Flask?
- How do you handle file uploads in Flask?
- Explain the concept of sessions in Flask and how to use them.
- What is Flask-WTF and how does it simplify form handling?
- How do you implement RESTful APIs using Flask?
- Explain the purpose of the `app.config` object in Flask.
- How do you handle CORS (Cross-Origin Resource Sharing) in Flask?
- What is Jinja2 and how is it used in Flask?
- How do you deploy a Flask application to a production environment?
- Explain the concept of middleware in Flask and provide an example.
- How do you implement custom error pages in Flask?
10 intermediate Flask interview questions and answers to ask mid-tier developers
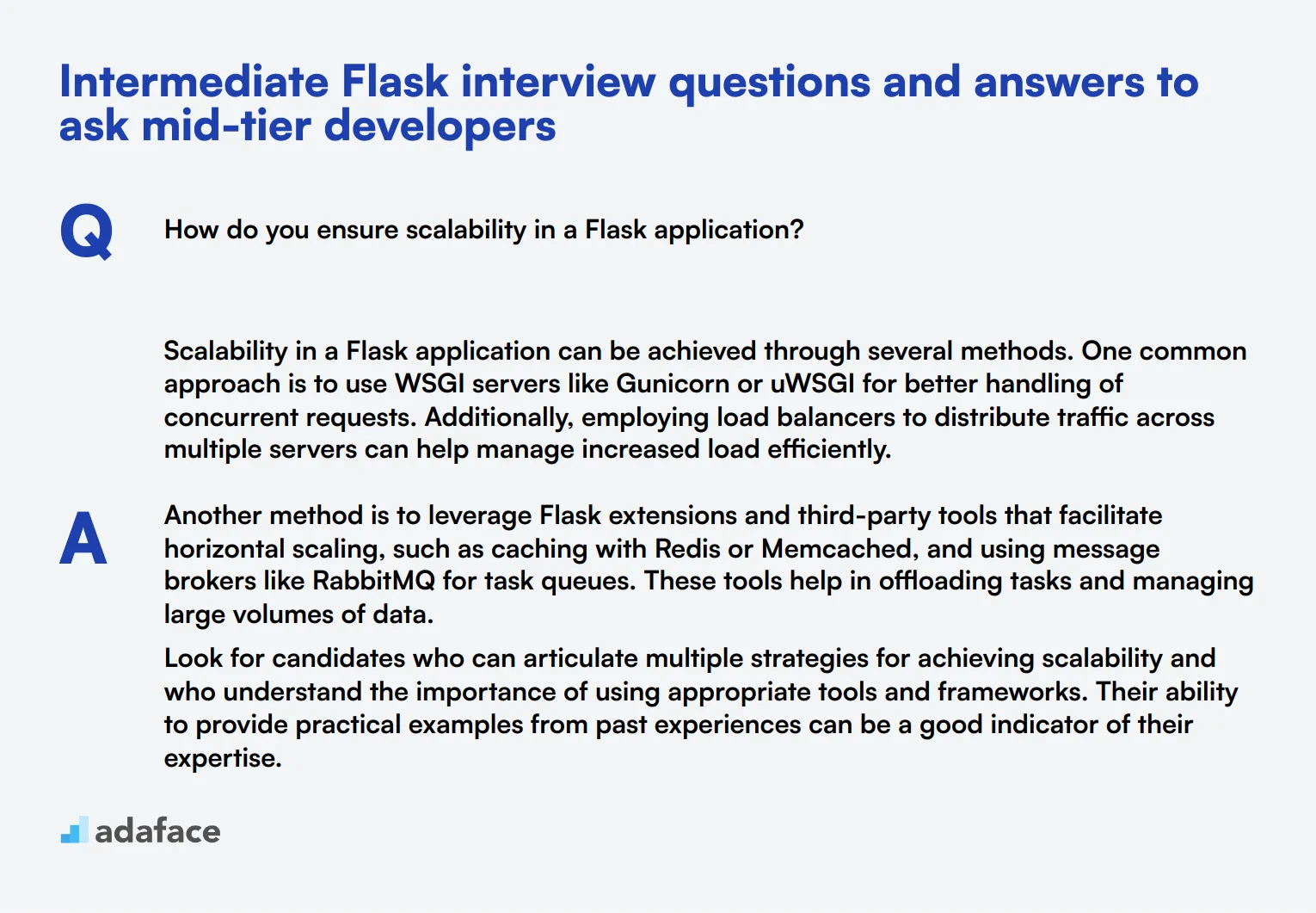
To assess whether your mid-tier developer candidates possess the necessary skills and knowledge to work effectively with Flask, use these intermediate interview questions. They will help you gauge their understanding of more complex Flask concepts and their ability to handle practical scenarios.
1. How do you ensure scalability in a Flask application?
Scalability in a Flask application can be achieved through several methods. One common approach is to use WSGI servers like Gunicorn or uWSGI for better handling of concurrent requests. Additionally, employing load balancers to distribute traffic across multiple servers can help manage increased load efficiently.
Another method is to leverage Flask extensions and third-party tools that facilitate horizontal scaling, such as caching with Redis or Memcached, and using message brokers like RabbitMQ for task queues. These tools help in offloading tasks and managing large volumes of data.
Look for candidates who can articulate multiple strategies for achieving scalability and who understand the importance of using appropriate tools and frameworks. Their ability to provide practical examples from past experiences can be a good indicator of their expertise.
2. What are some ways to improve the performance of a Flask application?
Improving the performance of a Flask application involves optimizing both the server and the application code. Caching static content and API responses is a highly effective method. This can be achieved using extensions like Flask-Caching.
Database query optimization is another crucial factor. Ensuring that queries are efficient and using indexing can significantly speed up data retrieval. Additionally, lazy loading can be used to load data only when necessary, reducing the initial load time.
Seek candidates who can discuss various techniques and tools for performance optimization. Their ability to provide specific examples and explain the impact of these optimizations will demonstrate their practical knowledge and experience.
3. How do you handle session management in Flask?
Session management in Flask can be handled using the built-in session object, which allows you to store session data on the server side. By default, Flask stores session data in signed cookies, but this can be configured to use server-side storage like Redis for better security and scalability.
To implement server-side sessions, you can use extensions such as Flask-Session. This helps in managing user sessions more securely and efficiently, especially for applications requiring high security and performance.
Look for candidates who understand the importance of secure session management and can explain the use of different methods and tools. Their ability to discuss the pros and cons of each approach will indicate a deeper understanding of session management in Flask.
4. What is the role of middleware in a Flask application?
Middleware in a Flask application acts as a bridge between the request and response cycles. It allows you to process requests globally before they reach the route handlers and to modify responses before they are sent back to the client.
You can use middleware for tasks such as logging, authentication, input validation, and request modification. Middleware functions can be added using Flask's before_request
, after_request
, and teardown_request
decorators.
Ideal candidates should be able to explain how middleware functions work and provide examples of practical use cases. Their ability to discuss various middleware applications and their impact on the overall application flow is crucial.
5. How do you handle asynchronous tasks in Flask?
Asynchronous tasks in Flask can be managed using task queues like Celery. Celery allows you to offload long-running tasks to background workers, ensuring that your main application remains responsive.
To integrate Celery with Flask, you need to configure a message broker like RabbitMQ or Redis. You then define tasks that can be executed asynchronously and trigger these tasks from your Flask routes or other parts of the application.
Candidates should demonstrate an understanding of the importance of asynchronous task management and be able to explain the integration and configuration process. Look for practical experience with task queues and message brokers.
6. How do you secure a Flask application?
Securing a Flask application involves multiple layers of security measures. Some key practices include using HTTPS to encrypt data in transit and validating user inputs to prevent injection attacks like SQL injection and XSS.
Flask provides several extensions to enhance security, such as Flask-Security for authentication, Flask-Talisman for HTTP security headers, and Flask-Limiter for rate limiting. These tools help in implementing robust security measures.
Candidates should be able to discuss various security best practices and tools. Their ability to provide examples of security implementations and explain the reasoning behind them will demonstrate their understanding of application security.
7. What are the benefits of using Flask for microservices architecture?
Flask is lightweight and modular, making it an excellent choice for building microservices. Its simplicity allows developers to create small, independent services that can communicate with each other via APIs.
Flask's flexibility means that you can easily integrate it with other tools and services, such as message brokers, databases, and authentication systems. This makes it easier to build and maintain a microservices architecture.
Seek candidates who can explain the specific advantages of using Flask in a microservices environment. Their ability to discuss real-world examples and the benefits of a modular approach will be a good indicator of their practical experience.
8. How do you handle logging in a Flask application?
Logging in a Flask application can be managed using Python's built-in logging module. You can configure different logging handlers to direct logs to various destinations, such as files, databases, or external logging services.
Flask also supports logging through its app.logger
object, which can be configured to capture different levels of log messages, such as DEBUG, INFO, WARNING, ERROR, and CRITICAL. Proper logging helps in monitoring and debugging the application.
Candidates should be able to explain how to set up and configure logging in Flask. Their ability to discuss the importance of logging and provide examples of how they have used logging to troubleshoot issues will demonstrate their practical knowledge.
9. How do you manage dependencies in a Flask project?
Managing dependencies in a Flask project is typically done using a requirements.txt
file, where you list all the required packages and their versions. This file can be used with tools like pip to install and manage dependencies.
Another popular tool for managing dependencies is Poetry, which provides more advanced dependency management features, including virtual environment management and dependency resolution.
Look for candidates who can discuss the importance of managing dependencies and the tools they have used. Their ability to explain how they ensure consistency and compatibility in their projects will be a good indicator of their project management skills.
10. What are some best practices for structuring a Flask project?
A well-structured Flask project should follow the MVC (Model-View-Controller) pattern, separating concerns and making the codebase more maintainable. This involves organizing your application into modules for models, views, controllers, static files, and templates.
Using blueprints is another best practice, as it allows you to modularize your application and make it easier to manage. Each blueprint can represent a different module or feature, promoting code reusability and separation of concerns.
Candidates should be able to discuss these best practices and provide examples of how they have structured their Flask projects. Their ability to articulate the benefits of a well-organized codebase will demonstrate their understanding of software architecture.
15 advanced Flask interview questions to ask senior developers
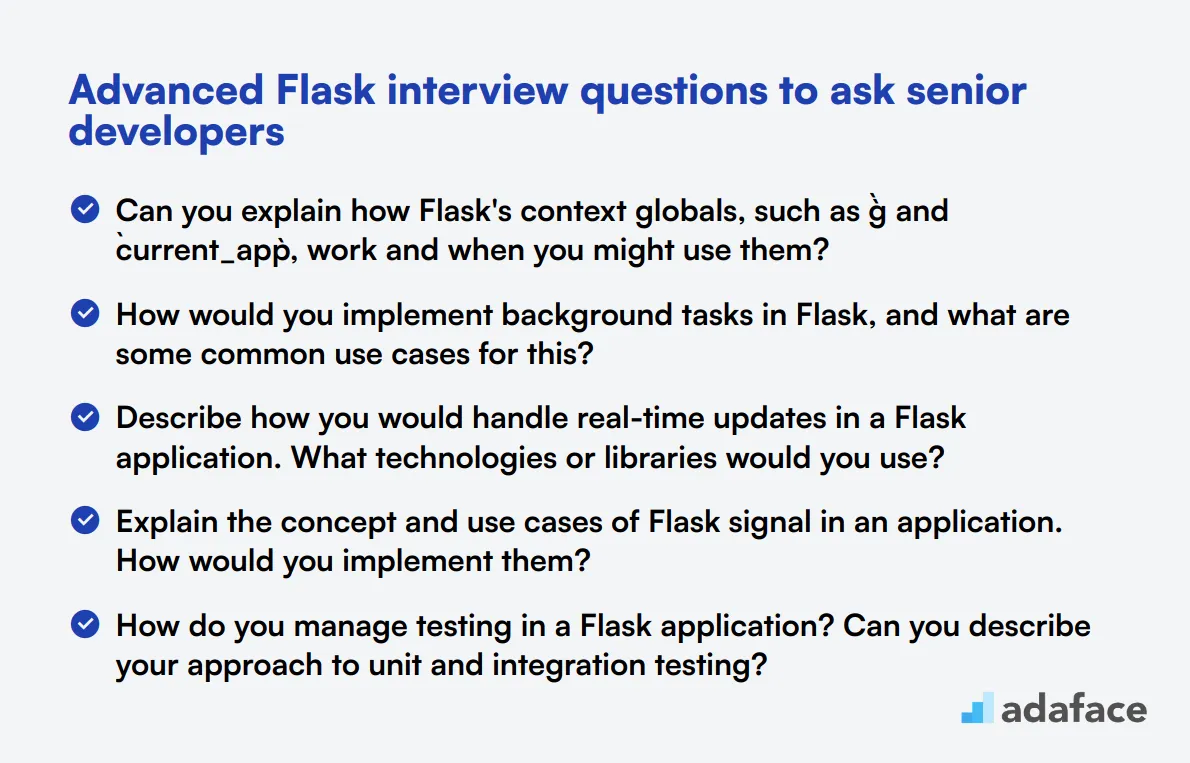
To ensure your candidates have the advanced skills needed for senior-level positions, consider using this list of advanced Flask interview questions. These questions are designed to gauge a developer's deep understanding of Flask and their ability to handle complex tasks with the framework. For a comprehensive overview of what to look for in a senior developer, you can also check out full-stack developer job description.
- Can you explain how Flask's context globals, such as `g` and `current_app`, work and when you might use them?
- How would you implement background tasks in Flask, and what are some common use cases for this?
- Describe how you would handle real-time updates in a Flask application. What technologies or libraries would you use?
- Explain the concept and use cases of Flask signal in an application. How would you implement them?
- How do you manage testing in a Flask application? Can you describe your approach to unit and integration testing?
- What strategies would you use to optimize the performance of a Flask application?
- Can you discuss how you would handle database migrations in Flask? What libraries or tools would you utilize?
- How do you implement role-based access control (RBAC) in a Flask application?
- Explain the purpose and use of Flask's `before_request` and `after_request` decorators.
- Describe how you would secure a Flask REST API. What are some potential threats and how would you mitigate them?
- How would you design a scalable architecture for a large Flask application?
- Can you explain the advantages and limitations of using Flask in microservices architecture?
- How do you handle internationalization (i18n) and localization (l10n) in a Flask application?
- What are some best practices for error handling and logging in a Flask application?
- Can you discuss how to use Flask with a front-end framework like React or Angular?
8 Flask interview questions and answers related to routing
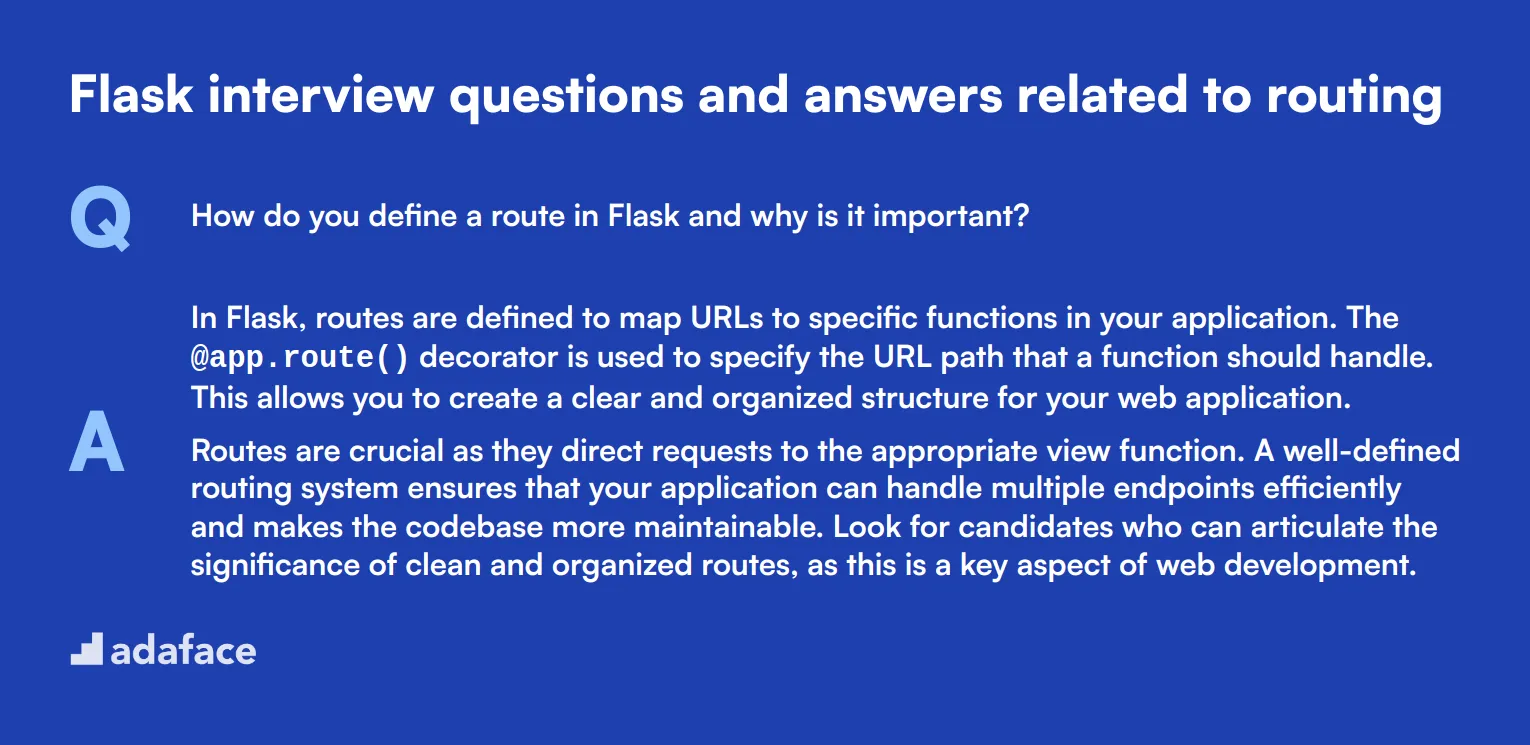
To assess if candidates can navigate and leverage the routing capabilities of Flask, use this list of interview questions. These questions will help you determine whether the applicant has a firm grasp on how to structure and manage routes efficiently in a Flask application.
1. How do you define a route in Flask and why is it important?
In Flask, routes are defined to map URLs to specific functions in your application. The @app.route()
decorator is used to specify the URL path that a function should handle. This allows you to create a clear and organized structure for your web application.
Routes are crucial as they direct requests to the appropriate view function. A well-defined routing system ensures that your application can handle multiple endpoints efficiently and makes the codebase more maintainable. Look for candidates who can articulate the significance of clean and organized routes, as this is a key aspect of web development.
2. What is the role of URL parameters in Flask routing?
URL parameters in Flask allow you to pass data directly within the URL itself. This is done by defining variables in the route, which are then passed to the view function. For example, in a route like /user/<username>
, the username is a URL parameter that gets passed to the corresponding function.
URL parameters are useful for creating dynamic URLs. They help in building RESTful APIs and are essential for applications that need to handle user-specific data. Ideal candidates should understand not only how to use URL parameters but also when to apply them for optimal efficiency.
3. How would you handle a situation where you have multiple routes pointing to the same function?
In Flask, you can have multiple routes pointing to the same function by using multiple decorators for the same function. This is useful when you want to provide different URLs for the same resource or action.
Handling this efficiently requires an understanding of how to manage route decorators and ensure that the logic within the function can handle requests from multiple paths. Look for candidates who can explain this concept clearly and discuss scenarios where it might be beneficial to use multiple routes for a single function.
4. Can you explain the concept of 'route protection' in Flask?
Route protection in Flask involves restricting access to certain routes or endpoints based on specific conditions, such as user authentication or permissions. This is often implemented using decorators like @login_required
from Flask extensions such as Flask-Login.
Route protection ensures that sensitive parts of the application are accessible only to authorized users. Candidates should be able to discuss different methods of implementing route protection and the importance of securing routes in a web application. For further insights, you can check how various software developer job descriptions emphasize security measures.
5. What are some best practices for organizing routes in a large Flask application?
In a large Flask application, it is best practice to organize routes using blueprints. Blueprints allow you to group related routes and handlers into separate modules, making the codebase more modular and easier to manage.
Other best practices include using clear and consistent naming conventions for routes, avoiding deeply nested routes, and documenting each route's purpose and function. Candidates should be able to articulate these practices and explain how they contribute to maintainability and scalability of the application.
6. How do you handle route conflicts in Flask?
Route conflicts in Flask occur when two or more routes are assigned the same URL path. This can be resolved by ensuring that each route has a unique path. If routes need to share similar paths, parameters or different HTTP methods can be used to distinguish them.
Candidates should demonstrate an understanding of how to avoid and resolve route conflicts, ensuring that each endpoint is uniquely identifiable. An ideal response would include examples of how they have managed such conflicts in past projects.
7. What is the significance of the `methods` parameter in Flask routing?
The methods
parameter in Flask routing specifies the HTTP methods that a route should respond to, such as GET, POST, PUT, DELETE, etc. This allows a single URL to handle different types of requests based on the specified methods.
Understanding the significance of the methods
parameter is crucial for building RESTful services and ensuring that your application behaves correctly depending on the type of request. Look for candidates who can explain how they have utilized different HTTP methods in their routing logic.
8. How do you ensure that your Flask routes are both SEO-friendly and user-friendly?
Creating SEO-friendly and user-friendly routes involves using clear, descriptive, and concise URL paths. Avoid using special characters, numbers, or random strings in URLs. Instead, use meaningful keywords that describe the content or functionality of the page.
Candidates should also discuss the importance of URL readability and how it impacts both user experience and search engine ranking. Look for responses that highlight a thoughtful approach to designing routes that cater to both users and search engines.
9 Flask interview questions and answers related to testing
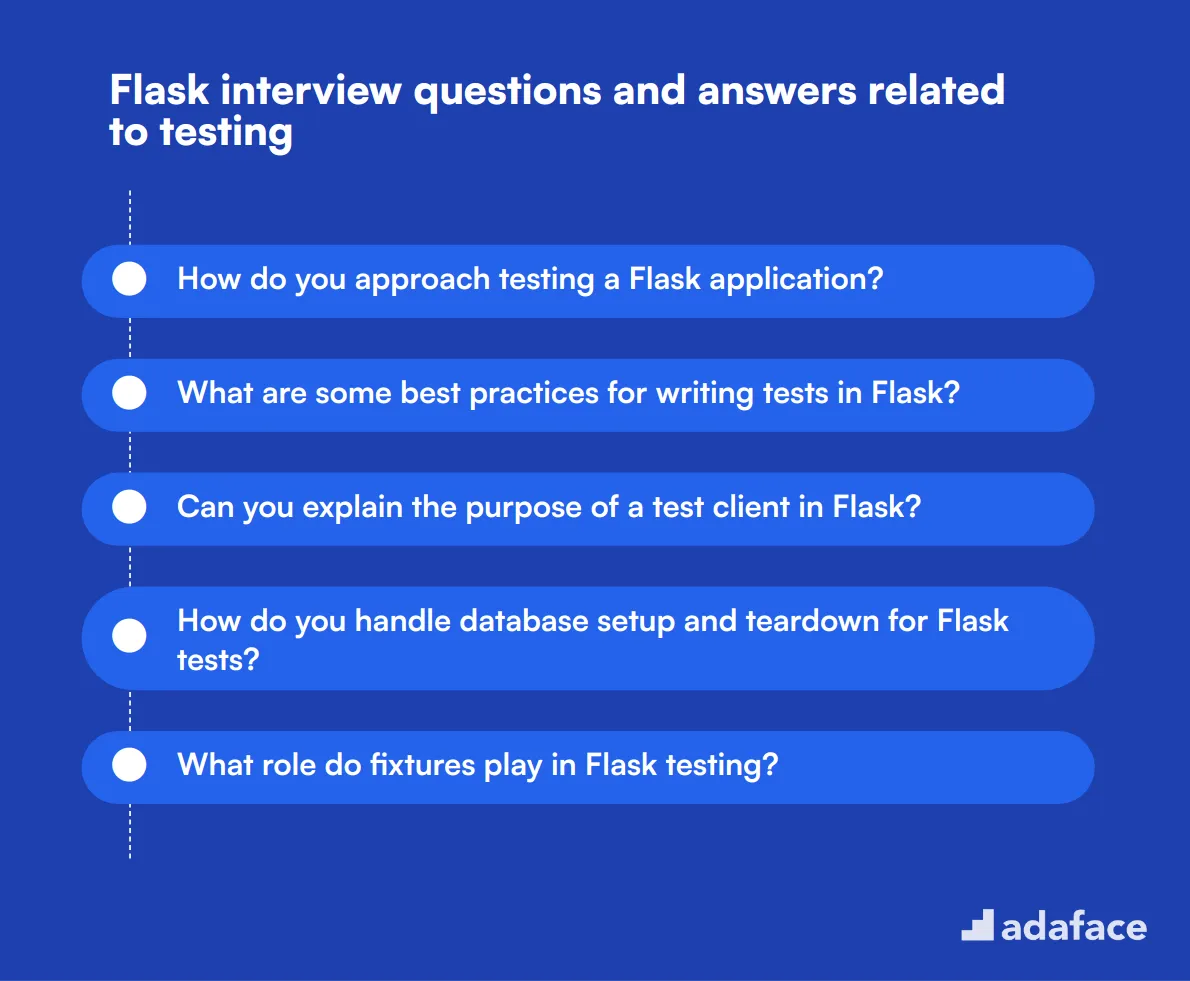
To ensure your candidates can write reliable and maintainable Flask applications, consider asking them some of these key questions related to testing. These questions will help you gauge their understanding of testing principles and their ability to implement them effectively in a Flask environment.
1. How do you approach testing a Flask application?
Testing a Flask application involves several steps: setting up a test environment, writing test cases, and using testing tools. Typically, developers use frameworks like pytest to write and execute their tests.
In addition to writing unit tests for individual functions, it's essential to write integration tests to ensure that different parts of the application work together as expected. Developers should also be familiar with mocking to isolate the code being tested.
An ideal candidate will describe a thorough approach that includes unit tests, integration tests, and the use of mock objects. Follow up by asking about their experience with specific testing tools.
2. What are some best practices for writing tests in Flask?
Best practices for writing tests in Flask include ensuring tests are independent, reproducible, and cover both positive and negative scenarios. Tests should be written in such a way that they can run in any order and still produce the same results.
It's also important to use fixtures to set up the test environment and tear it down after tests have run, ensuring no leftover state affects subsequent tests. Using tools like pytest makes it easier to manage these fixtures.
A strong candidate will mention practices like test isolation, use of fixtures, and comprehensive coverage. This ensures they understand how to maintain a clean and effective testing suite.
3. Can you explain the purpose of a test client in Flask?
A test client in Flask is used to simulate requests to the application without running a live server. This allows developers to test endpoints and their responses in a controlled environment.
By using a test client, you can send GET, POST, and other HTTP requests to your application and verify the responses, status codes, and any side effects on the database or other components.
Look for candidates who understand the importance of isolated testing and how a test client helps in verifying the behavior of different routes and views in a Flask application.
4. How do you handle database setup and teardown for Flask tests?
Handling database setup and teardown for Flask tests typically involves creating a separate test database and using fixtures to populate it with necessary test data before each test runs. After each test, the database is reset to ensure a clean environment.
Developers often use SQLAlchemy’s create_all()
and drop_all()
methods to manage the test database schema. They may also use factories to generate test data dynamically.
An ideal response should mention test data isolation, use of fixtures, and ensuring that the test database is separate from the production database to avoid any accidental data loss.
5. What role do fixtures play in Flask testing?
Fixtures in Flask testing are used to set up the environment needed for tests to run. This can include creating and configuring the Flask app, setting up the database, and populating it with initial data.
Fixtures ensure that each test starts with a known state, making tests reliable and repeatable. They also help in cleaning up resources after tests have run to prevent any side effects.
Candidates should demonstrate an understanding of how to properly set up and tear down their test environment using fixtures. Follow up by asking about their experience with tools like pytest fixtures.
6. How can you mock external services in Flask tests?
Mocking external services in Flask tests involves replacing parts of the application that make external calls with mock objects that simulate the behavior of those services. This allows tests to run without making actual external calls, which can be slow and unreliable.
Tools like the unittest.mock
library in Python are commonly used to create mock objects. These mocks can be configured to return specific values or raise exceptions to test different scenarios.
Look for candidates who can explain the importance of isolating tests from external dependencies and their experience with mocking libraries. This ensures they can write reliable tests that don't depend on external services.
7. What is the importance of code coverage in Flask testing?
Code coverage measures the extent to which the source code is tested by the test suite. High code coverage indicates that more parts of the code are executed during testing, which can help identify untested areas and potential bugs.
While 100% coverage is not always necessary, aiming for high coverage ensures that critical paths and edge cases are tested. Tools like coverage.py
can be used to measure and report code coverage metrics.
An ideal candidate will understand that code coverage helps ensure the reliability and robustness of the application. They should also discuss balancing coverage with the quality and relevance of tests.
8. How do you ensure your Flask tests are maintainable?
Ensuring maintainable tests involves writing clear and concise test cases that are easy to understand and modify. Tests should be organized in a logical structure, and any setup or teardown code should be reused through fixtures or helper functions.
It's also important to keep tests up to date with changes in the application. Regularly reviewing and refactoring tests helps maintain their relevance and effectiveness.
Candidates should discuss strategies for keeping tests clean and organized and ensuring they remain relevant as the application evolves. Follow up by asking about their experience with maintaining large test suites.
9. What strategies would you use to debug failing Flask tests?
Debugging failing Flask tests often involves examining the test output and logs to identify where the failure occurred. Adding additional logging or using a debugger can help pinpoint the exact cause.
It's also useful to isolate the failing test and run it independently to understand its behavior. Comparing the failing test case to similar passing cases can also provide insights.
Look for candidates who can articulate a systematic approach to identifying and resolving test failures. This ensures they can efficiently debug issues and maintain a reliable test suite.
Which Flask skills should you evaluate during the interview phase?
While it's impossible to assess every aspect of a candidate's Flask proficiency in a single interview, focusing on key skills can provide valuable insights. The following core competencies are particularly important when evaluating Flask developers.
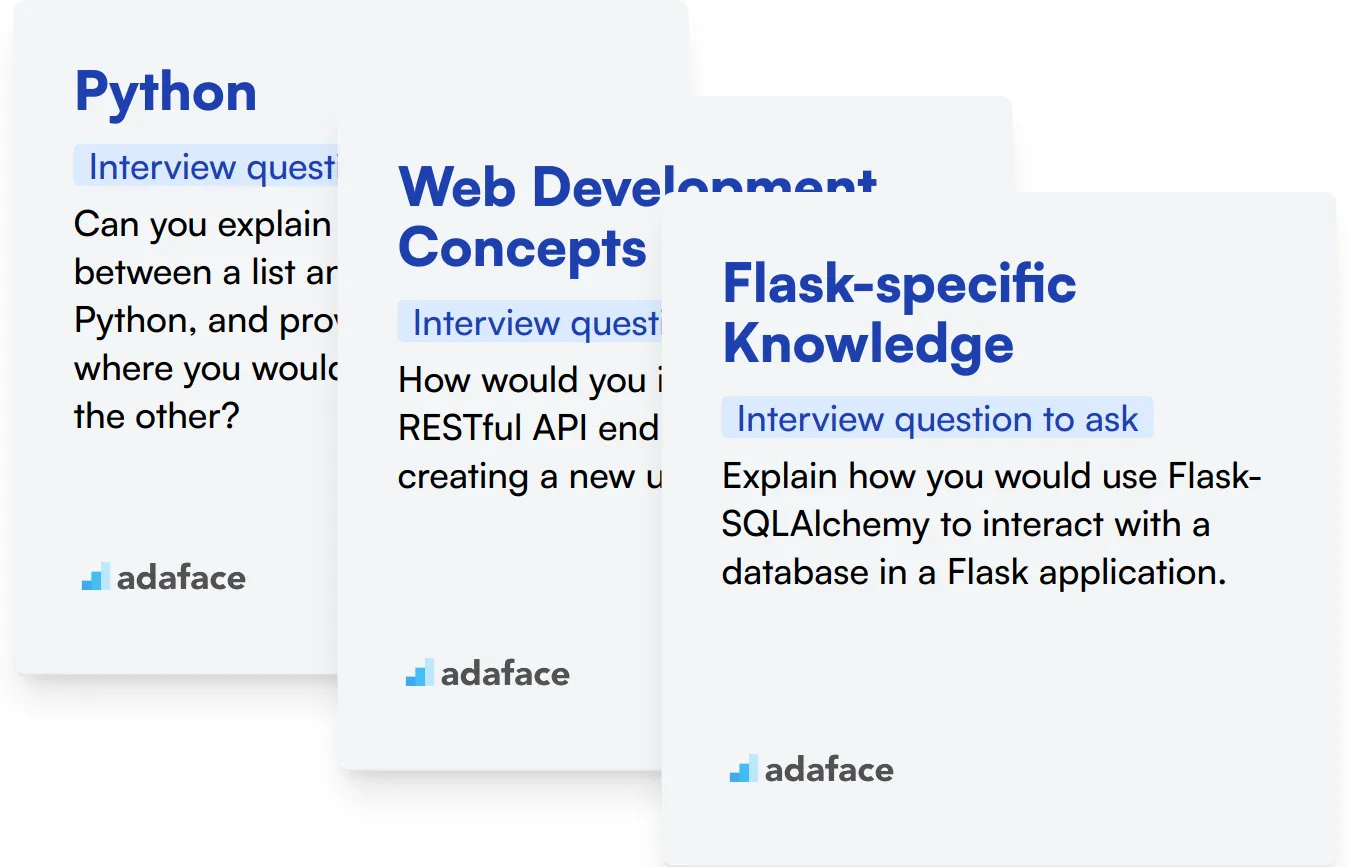
Python
Python is the foundation of Flask development. A strong grasp of Python syntax, data structures, and object-oriented programming is essential for working with Flask effectively.
To evaluate Python skills, consider using an assessment test with relevant MCQs. This can help filter candidates based on their Python proficiency.
During the interview, ask targeted questions to gauge the candidate's Python expertise. Here's an example:
Can you explain the difference between a list and a tuple in Python, and provide a scenario where you would choose one over the other?
Look for answers that demonstrate understanding of mutability, performance considerations, and appropriate use cases for each data structure. A strong candidate will provide clear examples and explain the trade-offs involved.
Web Development Concepts
Knowledge of web development fundamentals is crucial for Flask developers. This includes understanding HTTP protocols, RESTful APIs, and client-server architecture.
Consider using a web development assessment to evaluate candidates' understanding of these concepts.
To assess this skill during the interview, you can ask a question like:
How would you implement a RESTful API endpoint in Flask for creating a new user?
Look for responses that include proper route definition, HTTP method handling, request parsing, and appropriate status code returns. A good answer will also mention input validation and error handling.
Flask-specific Knowledge
Familiarity with Flask's core features, such as routing, templating, and extensions, is essential. Candidates should understand how to structure a Flask application and leverage its ecosystem.
To evaluate Flask-specific knowledge, consider asking:
Explain how you would use Flask-SQLAlchemy to interact with a database in a Flask application.
Look for answers that demonstrate understanding of model definition, database configuration, and basic CRUD operations using Flask-SQLAlchemy. Strong candidates will also mention best practices for query optimization and connection management.
Maximizing the Effectiveness of Flask Interview Questions
Before putting your Flask interview questions to use, consider these tips to enhance your candidate evaluation process. These strategies will help you make the most of your interviews and select the best Flask developers for your team.
1. Implement Skills Tests Before Interviews
Start by using skills tests to evaluate candidates' Flask proficiency before the interview stage. This approach helps you focus on candidates with proven technical abilities, saving time and resources.
For Flask developers, consider using a Python online test to assess their core language skills. Additionally, include tests for JavaScript and SQL to evaluate their full-stack capabilities.
By implementing these tests, you can create a shortlist of qualified candidates based on objective data. This allows you to dedicate interview time to exploring candidates' problem-solving skills and cultural fit.
2. Curate a Balanced Set of Interview Questions
Prepare a mix of Flask-specific questions and related web development topics. This combination helps you assess the candidate's overall expertise in building web applications.
Include questions about REST APIs, database management, and front-end technologies like JavaScript. You might also want to explore their knowledge of DevOps practices and cloud platforms.
Don't forget to assess soft skills such as communication and teamwork. These qualities are crucial for a developer to succeed in a collaborative environment.
3. Master the Art of Follow-Up Questions
Asking follow-up questions is key to understanding a candidate's true depth of knowledge. It helps you distinguish between those who have memorized answers and those with practical experience.
For example, after asking about Flask's request object, follow up with a question about how they've used it in a real project. This approach reveals their ability to apply knowledge in practical scenarios.
Evaluate Flask skills accurately with interview questions and skill tests
If you're looking to hire someone with Flask skills, it's important to assess their abilities accurately. The most effective way to do this is by using skill tests. Consider using our Python online test to evaluate candidates' Flask proficiency.
After using the test to shortlist top applicants, you can invite them for interviews. To streamline your hiring process and find the best Flask developers, check out our online assessment platform for a complete solution.
Python Online Test
Download Flask interview questions template in multiple formats
Flask Interview Questions FAQs
The questions cover junior, intermediate, and senior developer skill levels, as well as general Flask knowledge, routing, and testing.
Use a mix of questions appropriate for the role's level, and combine them with skill tests for a thorough assessment.
Answers are provided for many questions, particularly in the general, intermediate, routing, and testing sections.
Regularly review and update your questions to keep pace with Flask framework updates and industry trends.
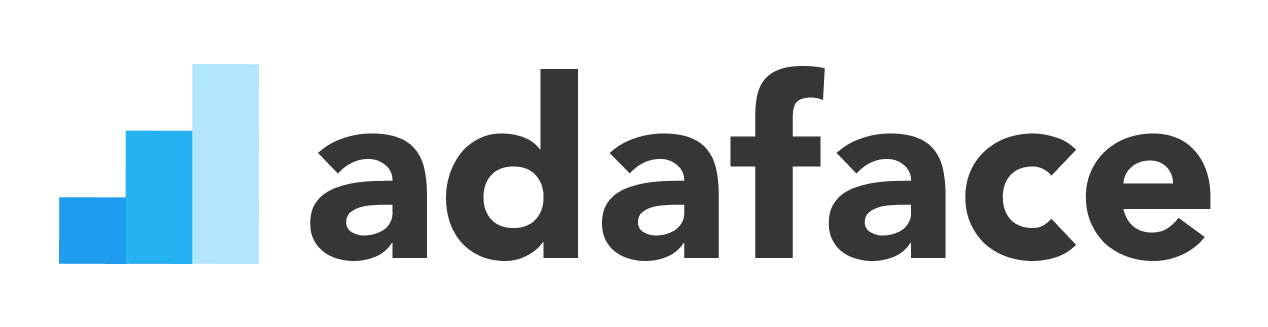
40 min skill tests.
No trick questions.
Accurate shortlisting.
We make it easy for you to find the best candidates in your pipeline with a 40 min skills test.
Try for freeRelated posts
Free resources
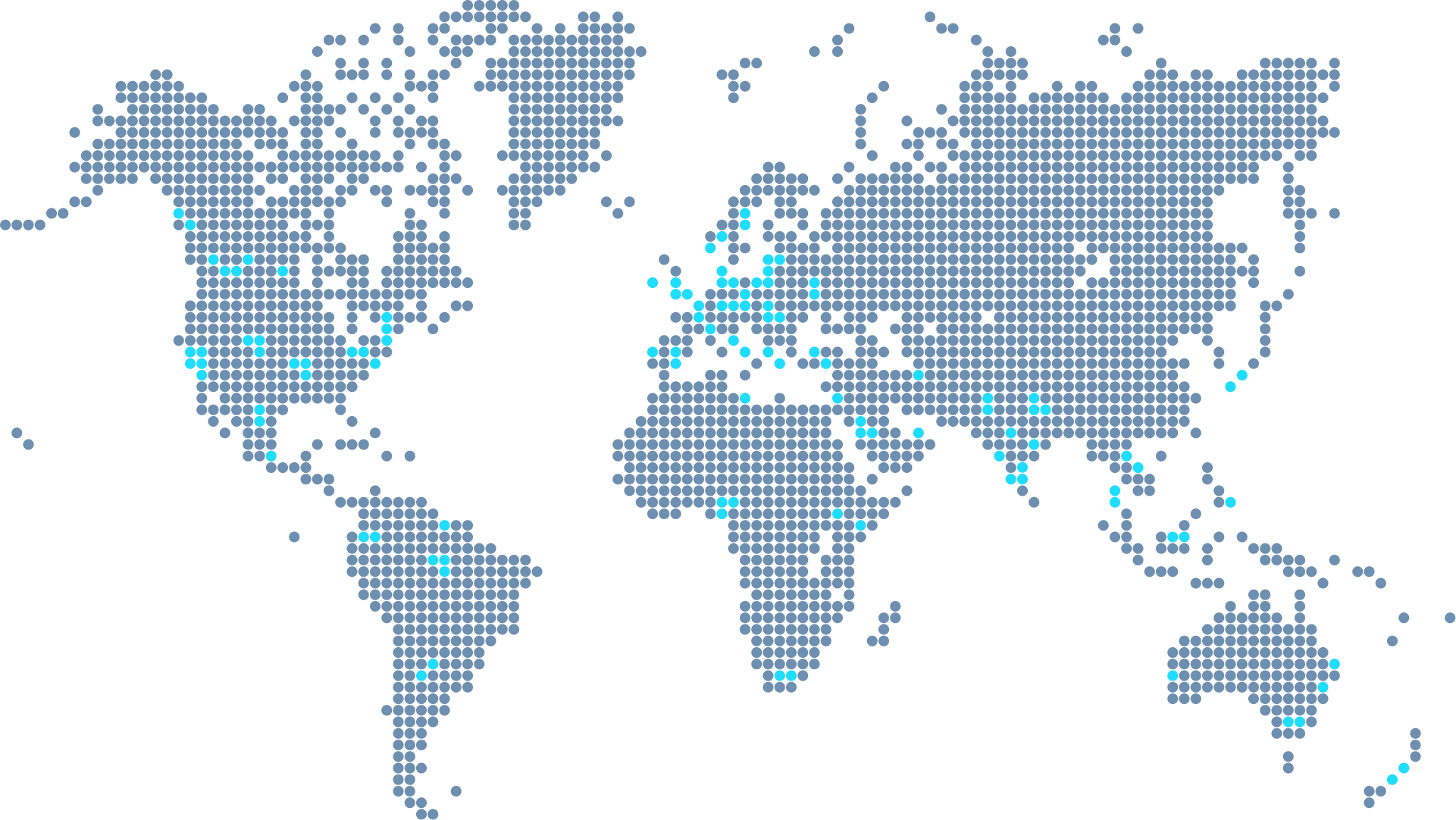
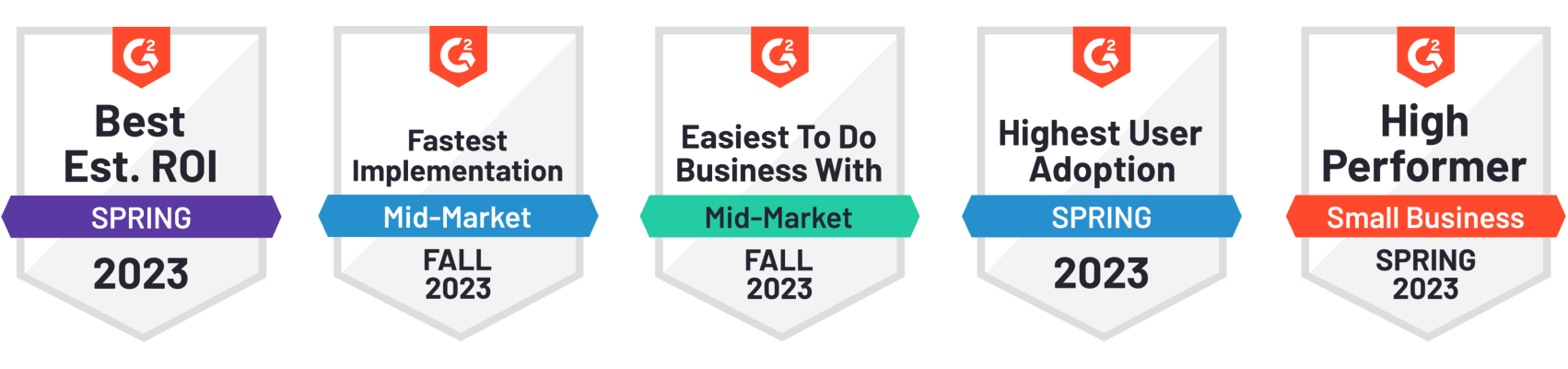