Hiring the right Embedded C developers is crucial for projects involving hardware-software interactions. Effective interviewing techniques can help you identify candidates with the necessary skills and experience in this specialized field.
This blog post provides a comprehensive list of Embedded C interview questions, covering common topics, junior engineer evaluations, hardware interactions, and memory management. By using these questions, you can assess candidates' knowledge and problem-solving abilities in real-world Embedded C scenarios.
Incorporating these questions into your interview process will help you make informed hiring decisions for Embedded C positions. Consider using pre-employment assessments in combination with these interview questions to thoroughly evaluate candidates' Embedded C skills.
Table of contents
10 common Embedded C interview questions to ask your applicants
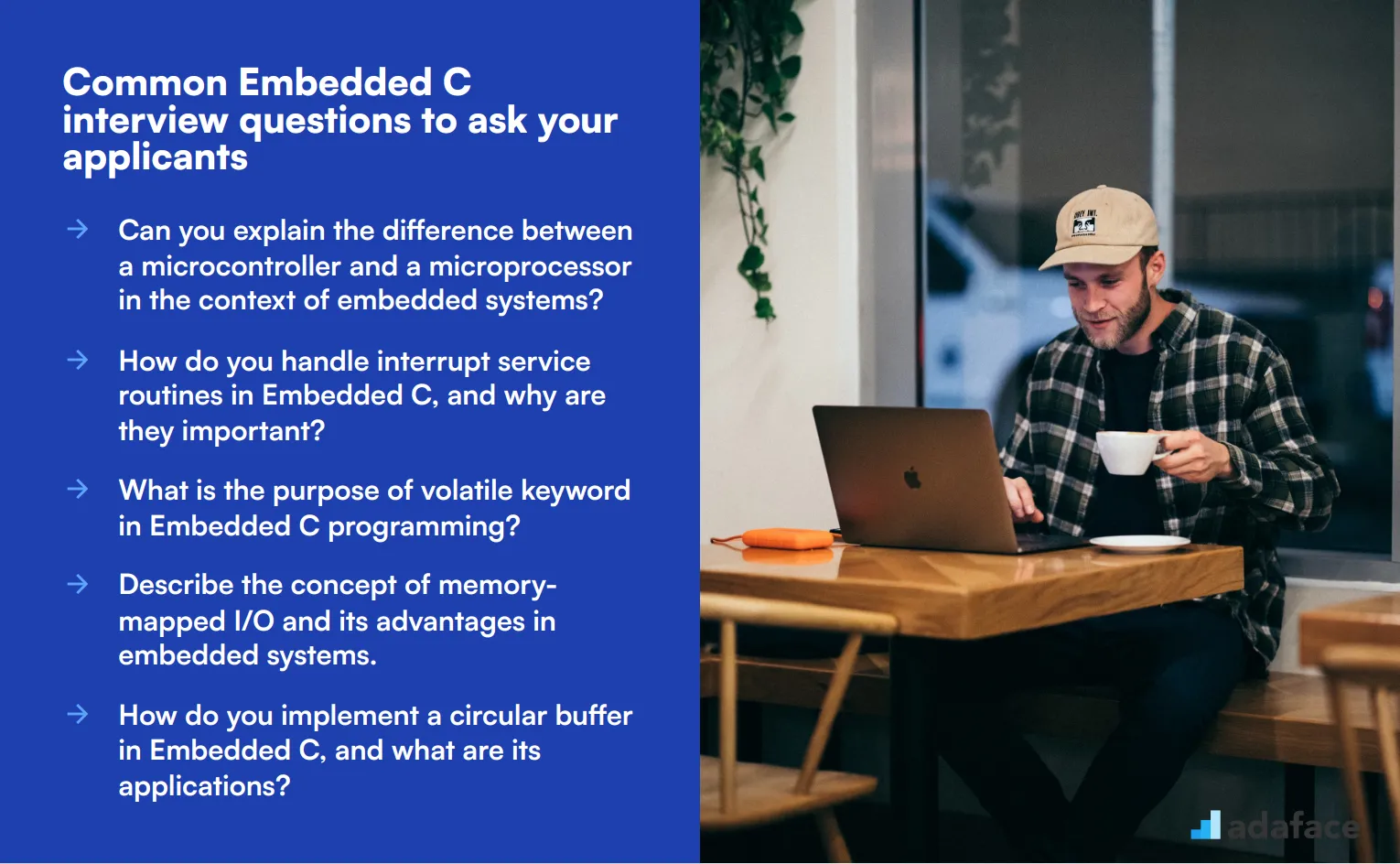
To assess the technical proficiency of embedded software engineer candidates, use these 10 common Embedded C interview questions. These questions are designed to evaluate a candidate's understanding of embedded systems, C programming, and real-time operating systems.
- Can you explain the difference between a microcontroller and a microprocessor in the context of embedded systems?
- How do you handle interrupt service routines in Embedded C, and why are they important?
- What is the purpose of volatile keyword in Embedded C programming?
- Describe the concept of memory-mapped I/O and its advantages in embedded systems.
- How do you implement a circular buffer in Embedded C, and what are its applications?
- Explain the difference between static and dynamic memory allocation in the context of embedded systems.
- What are watchdog timers, and how are they used in embedded systems?
- How do you optimize code for memory-constrained embedded systems?
- Describe the concept of task scheduling in a real-time operating system (RTOS).
- What are the key considerations when designing a device driver for an embedded system?
8 Embedded C interview questions and answers to evaluate junior engineers
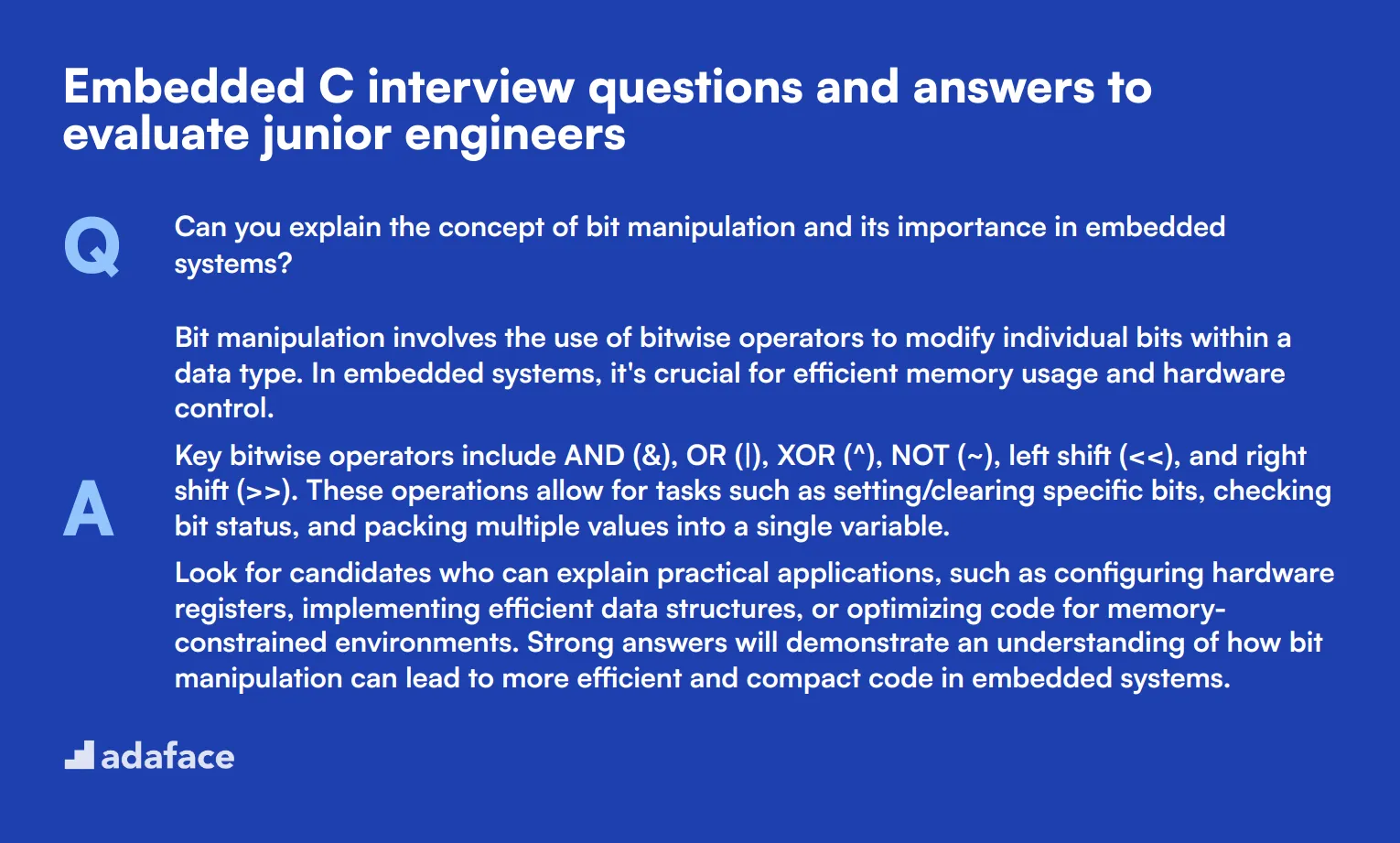
Ready to put your junior embedded software engineer candidates through their paces? These 8 Embedded C interview questions are designed to help you assess their foundational knowledge and problem-solving skills. Use them to spark insightful discussions and gauge a candidate's potential fit for your team.
1. Can you explain the concept of bit manipulation and its importance in embedded systems?
Bit manipulation involves the use of bitwise operators to modify individual bits within a data type. In embedded systems, it's crucial for efficient memory usage and hardware control.
Key bitwise operators include AND (&), OR (|), XOR (^), NOT (~), left shift (<<), and right shift (>>). These operations allow for tasks such as setting/clearing specific bits, checking bit status, and packing multiple values into a single variable.
Look for candidates who can explain practical applications, such as configuring hardware registers, implementing efficient data structures, or optimizing code for memory-constrained environments. Strong answers will demonstrate an understanding of how bit manipulation can lead to more efficient and compact code in embedded systems.
2. How do you handle endianness issues in embedded systems?
Endianness refers to the order in which bytes are arranged in multi-byte data types. In embedded systems, it's crucial to handle endianness correctly when interfacing with different hardware or transmitting data between systems.
To handle endianness issues, developers can use techniques such as:
- Using byte-swapping functions (e.g., htons, ntohs)
- Defining data structures with explicit byte ordering
- Using union types to access individual bytes of multi-byte data
- Employing compiler-specific pragmas or attributes to control data alignment
Evaluate candidates based on their awareness of endianness challenges and their ability to propose practical solutions. Strong answers will include mentions of cross-platform considerations and the importance of clear documentation for endianness-related code.
3. Describe the concept of debouncing in embedded systems and how you would implement it.
Debouncing is a technique used to eliminate the effects of mechanical switch bouncing in embedded systems. When a physical switch is pressed or released, it can rapidly oscillate between open and closed states before settling, potentially causing multiple unintended triggers.
A basic software debouncing implementation might involve:
- Detecting the initial state change of the switch
- Starting a timer
- Ignoring any further state changes for a set period (e.g., 50ms)
- Reading the final state after the debounce period
Look for candidates who understand the importance of debouncing in real-world applications. Strong answers might include mentions of hardware debouncing alternatives, trade-offs between different debouncing methods, and considerations for time-critical systems.
4. How would you implement a simple state machine in Embedded C?
A simple state machine in Embedded C can be implemented using an enumeration to define states and a switch statement to handle state transitions. The basic structure might look like this:
- Define an enum for possible states
- Create a variable to hold the current state
- Implement a switch statement that handles each state
- Within each case, perform state-specific actions and set the next state
More advanced implementations might use function pointers or lookup tables to improve modularity and reduce switch statement complexity.
Evaluate candidates based on their ability to explain the concept clearly and discuss potential optimizations. Strong answers might include considerations for handling events, implementing guards or conditions for state transitions, and ensuring the state machine is deterministic and easy to debug.
5. Explain the concept of memory alignment and its importance in embedded systems.
Memory alignment refers to the way data is arranged and accessed in memory. In embedded systems, proper alignment is crucial for optimal performance and, in some cases, for correct operation of the system.
Key points about memory alignment include:
- Different data types often have different alignment requirements
- Misaligned access can lead to performance penalties or hardware exceptions on some architectures
- Compilers typically handle alignment automatically, but manual control may be necessary in some cases
- Packed structures can be used to save memory but may impact performance
Look for candidates who understand the trade-offs between memory efficiency and performance. Strong answers might include discussions on how to manually control alignment, the use of compiler-specific pragmas or attributes, and considerations for cross-platform development where alignment requirements may differ.
6. How would you implement a simple scheduler for a bare-metal embedded system?
Implementing a simple scheduler for a bare-metal system involves creating a basic task management system without the support of an operating system. A basic approach might include:
- Defining a structure to represent tasks, including function pointers and scheduling information
- Creating an array or linked list to store tasks
- Implementing a timer interrupt to trigger task switching
- Developing functions to add, remove, and switch between tasks
The scheduler would typically use a round-robin or priority-based algorithm to determine which task to run next. It's important to consider factors like context switching, stack management, and handling of shared resources.
Evaluate candidates based on their understanding of task management concepts and their ability to discuss trade-offs in scheduler design. Strong answers might include considerations for determinism, handling of periodic vs. aperiodic tasks, and techniques for minimizing scheduler overhead in resource-constrained systems.
7. Describe the concept of watchdog timers and how you would use them in an embedded system.
Watchdog timers are hardware or software mechanisms used to detect and recover from software malfunctions. They work by requiring the software to periodically 'kick' or reset the timer; if the software fails to do so, the watchdog assumes a malfunction and triggers a system reset.
Key points about using watchdog timers include:
- They help improve system reliability by recovering from unexpected hangs or infinite loops
- Proper placement of watchdog kicks is crucial to ensure all critical parts of the code are executed
- Care must be taken to avoid false triggers during expected long operations
- Some systems may log watchdog events for later analysis
Look for candidates who understand both the benefits and potential pitfalls of watchdog timers. Strong answers might include discussions on hardware vs. software watchdogs, strategies for determining appropriate timeout periods, and techniques for graceful system recovery after a watchdog reset.
8. How would you approach debugging a hard fault in an ARM Cortex-M based system?
Debugging a hard fault in an ARM Cortex-M system requires a systematic approach to identify the root cause of the fault. Key steps might include:
- Examining fault status registers (CFSR, HFSR, DFSR) to determine the type of fault
- Analyzing the stack frame to identify the instruction that caused the fault
- Using debugger features like breakpoints and memory watches to isolate the issue
- Reviewing the code around the fault location for potential causes (e.g., null pointer dereferences, stack overflows)
Advanced techniques might involve using fault handlers to capture additional diagnostic information or employing hardware trace capabilities for more detailed analysis.
Evaluate candidates based on their familiarity with ARM architecture and debugging tools. Strong answers should demonstrate a methodical approach to problem-solving and knowledge of common causes of hard faults in embedded systems. Look for mentions of preventive measures and best practices to avoid hard faults in the first place.
14 Embedded C interview questions about hardware interactions
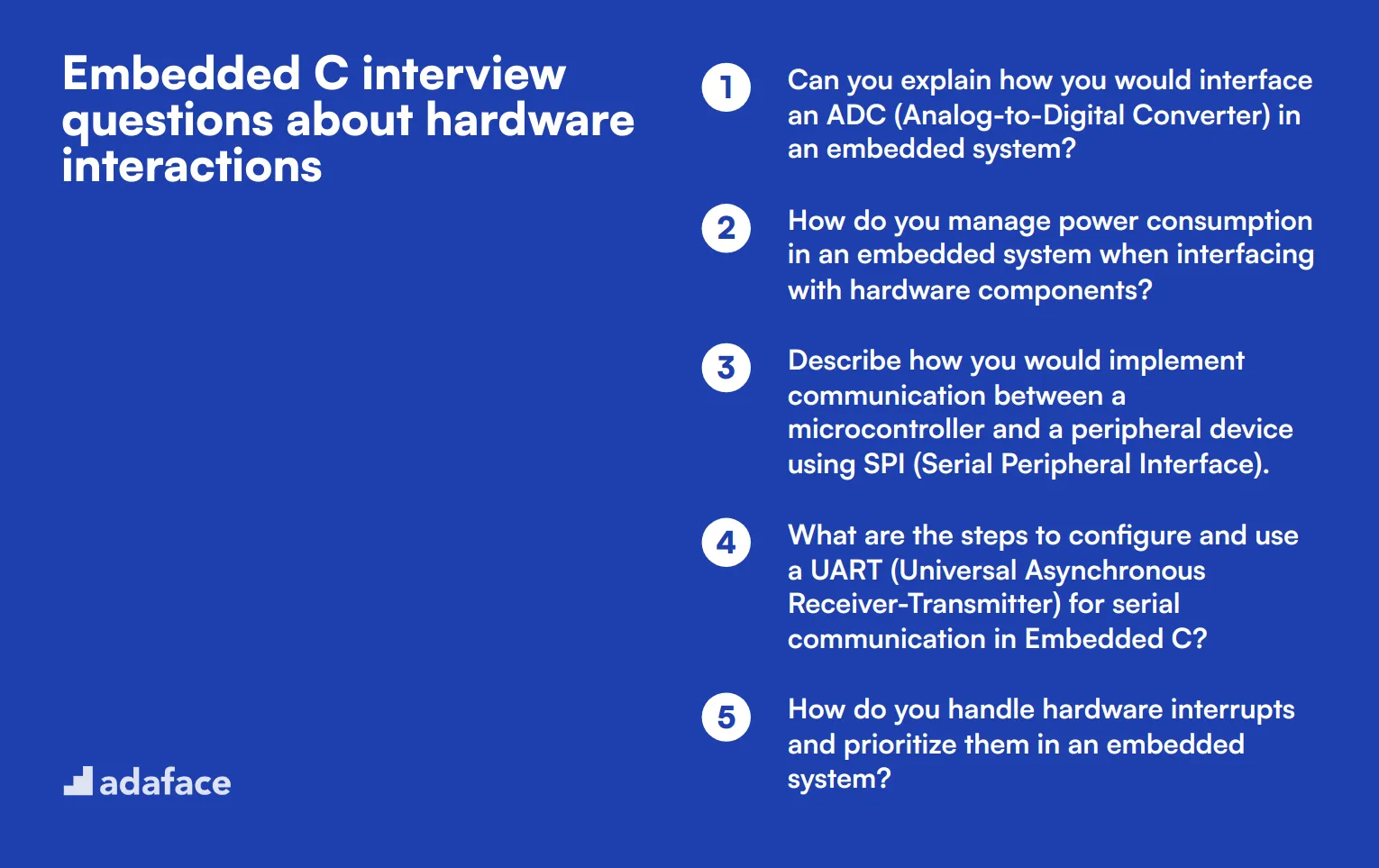
To determine whether your applicants have the right skills to handle hardware interactions in Embedded C, refer to some of these 14 essential interview questions. These questions will help you gauge their practical understanding and expertise, ensuring they can effectively manage hardware-related tasks in embedded systems. For more insights on evaluating technical candidates, explore skills required for embedded software engineer.
- Can you explain how you would interface an ADC (Analog-to-Digital Converter) in an embedded system?
- How do you manage power consumption in an embedded system when interfacing with hardware components?
- Describe how you would implement communication between a microcontroller and a peripheral device using SPI (Serial Peripheral Interface).
- What are the steps to configure and use a UART (Universal Asynchronous Receiver-Transmitter) for serial communication in Embedded C?
- How do you handle hardware interrupts and prioritize them in an embedded system?
- Explain how you would use DMA (Direct Memory Access) to optimize data transfer in an embedded system.
- What considerations are important when designing an interface between a microcontroller and an external memory device?
- How would you implement I2C (Inter-Integrated Circuit) communication in Embedded C?
- Describe the process of setting up PWM (Pulse Width Modulation) for controlling motor speed in an embedded application.
- How do you handle debouncing for a mechanical switch input in Embedded C?
- What strategies do you use to ensure reliable data acquisition from sensors in an embedded system?
- Can you explain how you would implement software to handle multiple simultaneous hardware events?
- Describe how you would test and validate the hardware interfaces of an embedded system.
- How do you manage and troubleshoot communication errors in embedded systems?
9 Embedded C interview questions and answers related to memory management
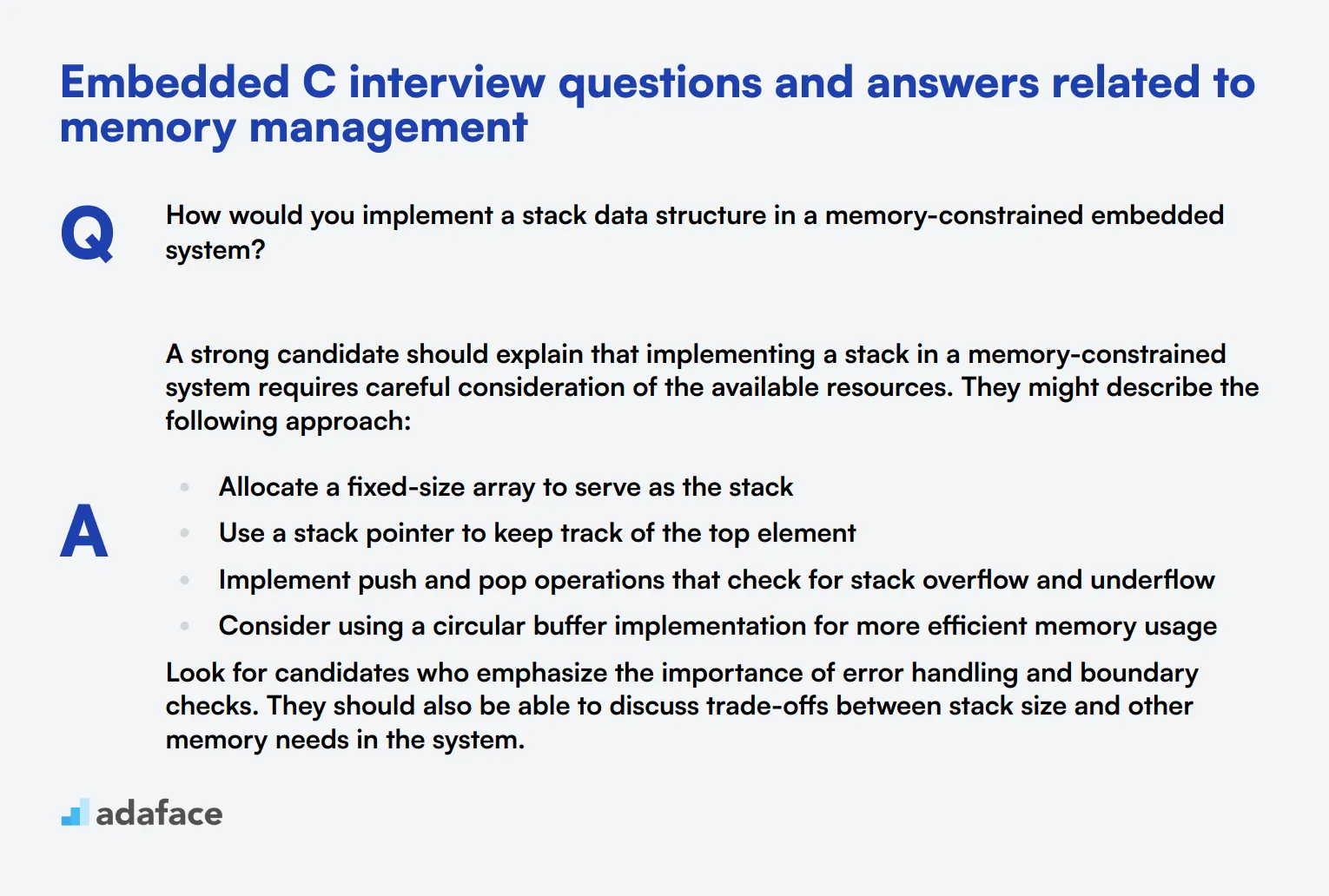
Memory management is a crucial aspect of embedded C programming. These nine questions will help you assess a candidate's understanding of memory-related concepts and their ability to optimize code for memory-constrained systems. Use them to gauge how well applicants can handle the unique challenges of embedded systems development.
1. How would you implement a stack data structure in a memory-constrained embedded system?
A strong candidate should explain that implementing a stack in a memory-constrained system requires careful consideration of the available resources. They might describe the following approach:
- Allocate a fixed-size array to serve as the stack
- Use a stack pointer to keep track of the top element
- Implement push and pop operations that check for stack overflow and underflow
- Consider using a circular buffer implementation for more efficient memory usage
Look for candidates who emphasize the importance of error handling and boundary checks. They should also be able to discuss trade-offs between stack size and other memory needs in the system.
2. Can you explain the concept of memory fragmentation and how it affects embedded systems?
Memory fragmentation occurs when free memory becomes divided into small, non-contiguous blocks. This can be particularly problematic in embedded systems with limited memory resources. A knowledgeable candidate should explain:
- Internal fragmentation: Wasted space within allocated memory blocks
- External fragmentation: Free memory scattered in small chunks between allocated blocks
- Impact on performance: Difficulty in allocating large contiguous blocks of memory
- Mitigation strategies: Using memory pools, implementing defragmentation algorithms, or using a memory allocator designed for embedded systems
An ideal response should demonstrate an understanding of how fragmentation can lead to memory allocation failures and system instability over time, even when there appears to be sufficient free memory available.
3. How do you handle memory leaks in an embedded system with limited resources?
Handling memory leaks in embedded systems is critical due to their limited resources. A strong candidate should outline a comprehensive approach:
- Use static allocation where possible to avoid dynamic memory management issues
- Implement proper memory management practices, such as matching every malloc() with a free()
- Utilize memory analysis tools during development to detect leaks
- Implement memory monitoring systems to track allocations and deallocations
- Consider implementing a custom memory allocator with built-in leak detection
Look for candidates who emphasize the importance of proactive measures and regular code reviews. They should also mention the potential need for system resets in long-running embedded systems to mitigate the impact of small, undetected leaks.
4. Explain the concept of memory overlays and when you might use them in embedded systems.
Memory overlays are a technique used to manage limited memory resources by allowing different parts of a program to share the same memory space at different times. A knowledgeable candidate should explain:
- Overlays involve dividing the program into segments that can be loaded and unloaded as needed
- They are useful when the total program size exceeds available memory
- Overlays require careful planning of program structure and execution flow
- They can significantly reduce memory requirements but may impact performance due to loading/unloading overhead
An ideal response should include examples of when overlays might be appropriate, such as in systems with complex functionality but limited RAM. Candidates should also discuss the trade-offs between memory savings and potential performance impacts.
5. How would you optimize string handling in a memory-constrained embedded system?
Optimizing string handling in embedded systems is crucial for efficient memory usage. A strong candidate should suggest several strategies:
- Use fixed-size character arrays instead of dynamic allocation when possible
- Implement string pooling to reuse common strings
- Consider using string interning for frequently compared strings
- Utilize string compression techniques for long, repetitive strings
- Implement custom, lightweight string handling functions instead of using standard library functions
Look for candidates who emphasize the importance of avoiding string duplication and minimizing dynamic memory allocation. They should also be able to discuss the trade-offs between memory usage and processing overhead for various optimization techniques.
6. What is the difference between const and #define in terms of memory usage in embedded systems?
Understanding the difference between const and #define is important for efficient memory usage. A knowledgeable candidate should explain:
- const creates a read-only variable that occupies memory
- #define is a preprocessor directive that performs text substitution and doesn't use memory
- const variables can be type-checked and debugged more easily
- #define macros are expanded inline, potentially leading to code bloat in some cases
An ideal response should include discussion of when to use each approach. For example, const might be preferred for values that need to be accessed frequently or require type safety, while #define might be better for simple constant values or expressions that don't need to occupy memory.
7. How would you implement a memory pool in an embedded system, and what are its advantages?
Implementing a memory pool in an embedded system involves pre-allocating a large block of memory and dividing it into fixed-size chunks. A strong candidate should outline the implementation steps:
- Allocate a large array or memory block
- Divide the block into fixed-size chunks
- Maintain a free list of available chunks
- Implement allocation and deallocation functions to manage the pool
Advantages of memory pools include:
- Reduced fragmentation
- Predictable allocation times
- Elimination of heap exhaustion issues
- Improved performance due to reduced allocation overhead
Look for candidates who can discuss the trade-offs, such as potential memory waste due to fixed chunk sizes, and scenarios where memory pools are particularly beneficial in embedded software development.
8. Explain the concept of memory barriers and when they might be necessary in embedded systems.
Memory barriers are instructions that ensure the order of memory operations in multi-core or multi-threaded embedded systems. A knowledgeable candidate should explain:
- Memory barriers prevent out-of-order execution or memory access reordering
- They are necessary when dealing with shared memory or memory-mapped I/O
- Different types of barriers (e.g., read, write, full) provide different levels of ordering guarantees
- Improper use of memory barriers can lead to race conditions or data inconsistencies
An ideal response should include examples of when memory barriers are crucial, such as in interrupt handlers or when implementing lock-free data structures. Candidates should also demonstrate awareness of the performance implications of using memory barriers.
9. How would you handle stack overflow detection in an embedded system?
Detecting stack overflow is critical for preventing system crashes and ensuring reliable operation. A strong candidate should outline several approaches:
- Use hardware-supported stack overflow detection if available
- Implement software-based detection by placing a known pattern at the stack bottom and periodically checking its integrity
- Utilize compiler options that insert stack checking code
- Monitor stack usage during development and testing phases
- Implement a separate stack for interrupt handlers to prevent interference with the main stack
Look for candidates who emphasize the importance of proactive measures, such as careful stack size estimation and allocation. They should also be able to discuss recovery strategies in case a stack overflow is detected, such as system resets or fallback to a safe mode of operation.
Which Embedded C skills should you evaluate during the interview phase?
While it's impossible to assess every relevant skill of your candidates in a single interview, there are key Embedded C skills that are essential to evaluate. Focusing on these core competencies will help you gauge the candidate's suitability for the role effectively.
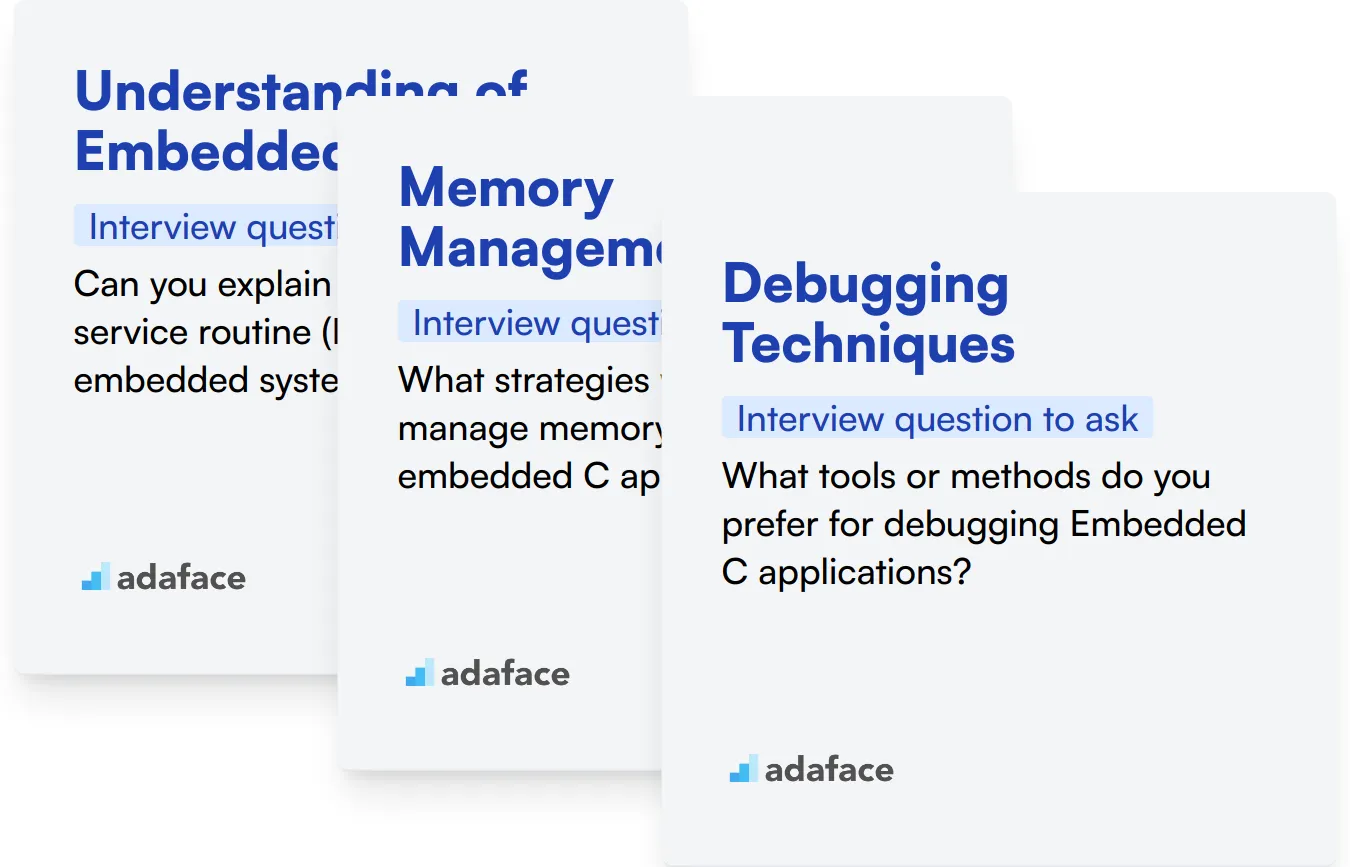
Understanding of Embedded Systems
Consider using an assessment test with relevant MCQs to gauge their understanding of Embedded Systems. Our Embedded Systems test can be a good starting point.
You can further evaluate this skill by asking targeted interview questions. One effective question you might ask is:
Can you explain how the interrupt service routine (ISR) works in an embedded system?
Look for a clear explanation of how ISRs are triggered and how they interact with the main program flow. Candidates should demonstrate an understanding of priorities, context switching, and the importance of ISRs in real-time systems.
Memory Management
Using a targeted assessment will help filter candidates based on their memory management skills. For instance, our memory management test can effectively gauge this knowledge.
Consider asking the candidate specific questions to dive deeper into their understanding. For instance:
What strategies would you use to manage memory efficiently in an embedded C application?
Be attentive to their response regarding static vs. dynamic memory allocation, the importance of handling memory leaks, and techniques such as memory pools or buffers that help optimize memory usage.
Debugging Techniques
To evaluate this skill, consider incorporating a quick assessment test with relevant MCQs. You can find useful resources in our Embedded C test.
A targeted interview question to assess debugging skills could be:
What tools or methods do you prefer for debugging Embedded C applications?
Look for familiarity with various debugging tools (like JTAG or GDB) and methodologies (such as print debugging or using breakpoints) that can indicate their hands-on experience and problem-solving approach.
3 Tips for Effectively Using Embedded C Interview Questions
Before you start implementing what you've learned, consider these practical tips to enhance your interview process.
1. Incorporate Skills Tests Before Interviews
Using skills tests prior to interviews helps identify candidates who possess the necessary technical abilities. For Embedded C positions, consider employing our Embedded C online test to evaluate candidates' proficiency.
This initial testing not only saves time but also ensures you have a shortlist of candidates who meet the technical requirements. Incorporating this into your process can lead to more focused interviews, allowing you to assess candidates on deeper aspects of their skills.
By filtering candidates through these tests, you set the stage for a more effective interview process, leading to informed hiring decisions as you move to the next step.
2. Curate Your Interview Questions Strategically
With limited time during interviews, it's important to choose questions that effectively evaluate the candidate's skills. Focus on a small set of questions that cover key competencies relevant to Embedded C and hardware interactions, while also considering related topics like memory management or software engineering.
Refer to other relevant interview questions, such as those related to data structures or software engineering, to ensure comprehensive skill evaluation.
This targeted approach maximizes your ability to assess candidates on the most pertinent aspects, leading to better hiring outcomes.
3. Ask Follow-Up Questions to Gauge Depth
Relying solely on initial interview questions may not reveal the depth of a candidate's knowledge. Follow-up questions help uncover true expertise and can address any gaps where candidates may lack depth or experience.
For instance, if a candidate states they have experience with pointers in Embedded C, a good follow-up question could be, 'Can you explain the difference between a pointer and a reference?' This not only tests their understanding but also reveals their ability to articulate complex concepts.
Leveraging Embedded C Interview Questions and Skills Tests for Effective Hiring
When hiring for roles requiring Embedded C skills, confirming candidates have the necessary competencies is key. Using targeted Embedded C Online Tests is a reliable method to assess these skills accurately before proceeding further in your recruitment process.
After administering these tests, the top candidates can be easily shortlisted for interviews. To streamline your hiring process further, consider visiting our Online Assessment Platform and explore options for setting up your next recruitment drive.
Embedded C Online Test
Download Embedded C interview questions template in multiple formats
Embedded C Interview Questions FAQs
Look for strong programming skills, experience with hardware interactions, and good problem-solving abilities.
You can ask them questions related to pointers, dynamic memory allocation, and memory leaks in Embedded C.
Embedded systems often interface with hardware directly, so understanding how candidates handle hardware interactions is key.
Ask about basic programming concepts, simple debugging scenarios, and fundamental memory management questions.
Combine these questions with practical tests and real-world problem scenarios to get a holistic view of the candidate's skills.
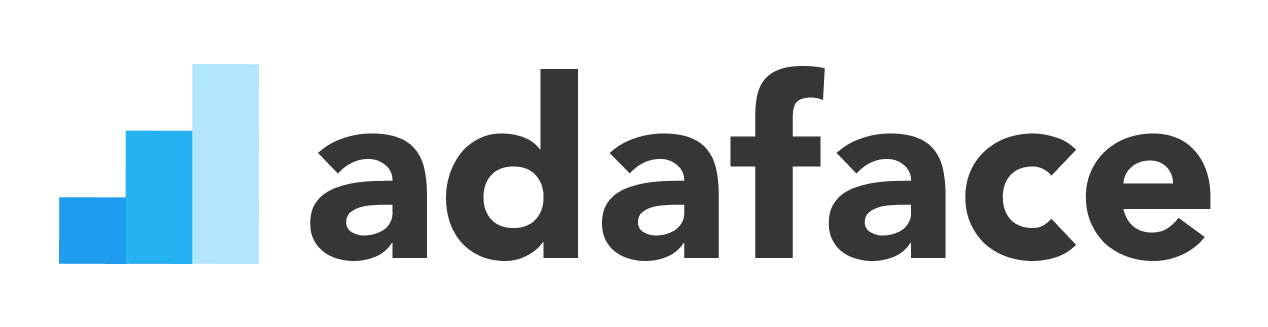
40 min skill tests.
No trick questions.
Accurate shortlisting.
We make it easy for you to find the best candidates in your pipeline with a 40 min skills test.
Try for freeRelated posts
Free resources
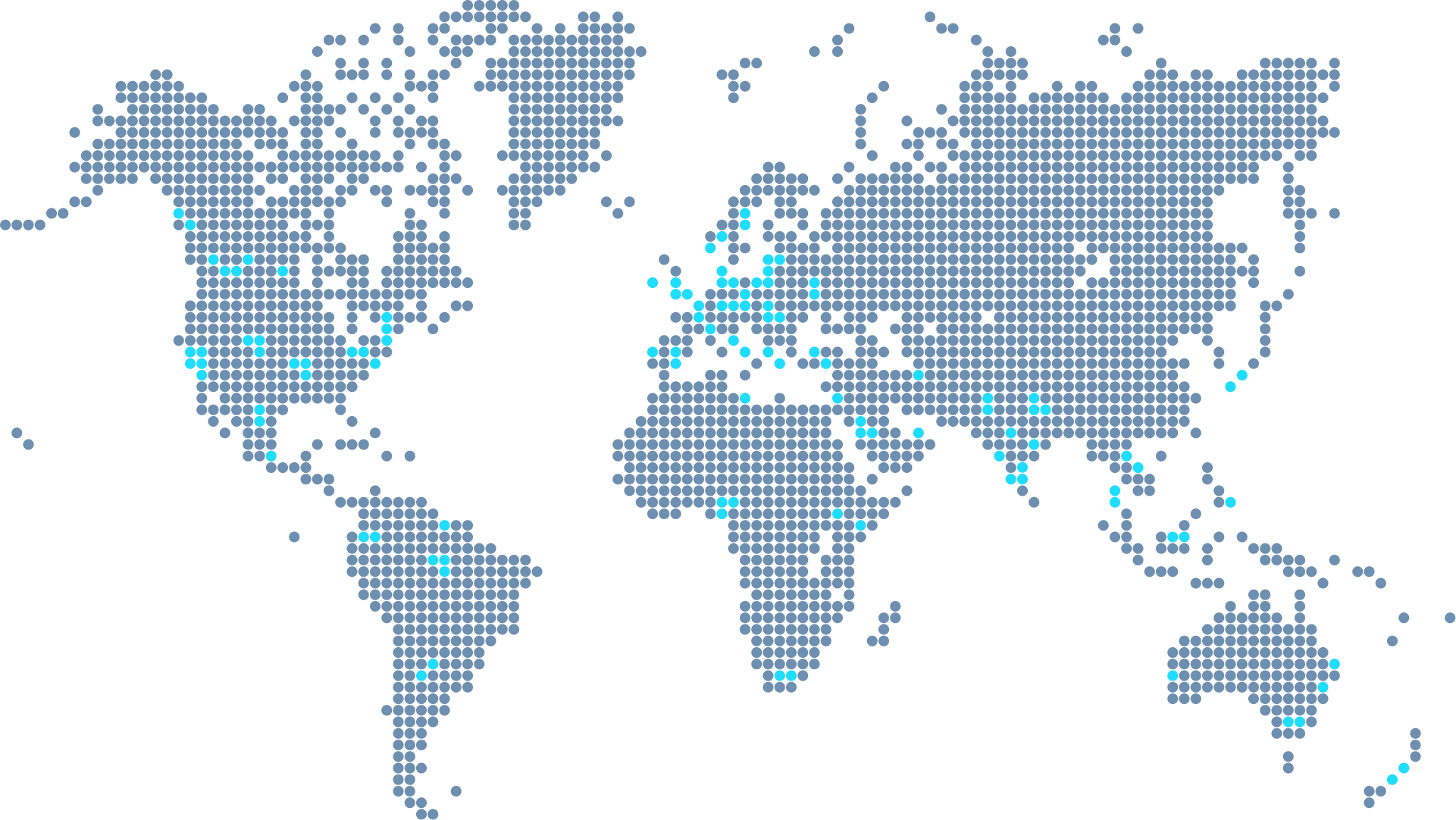
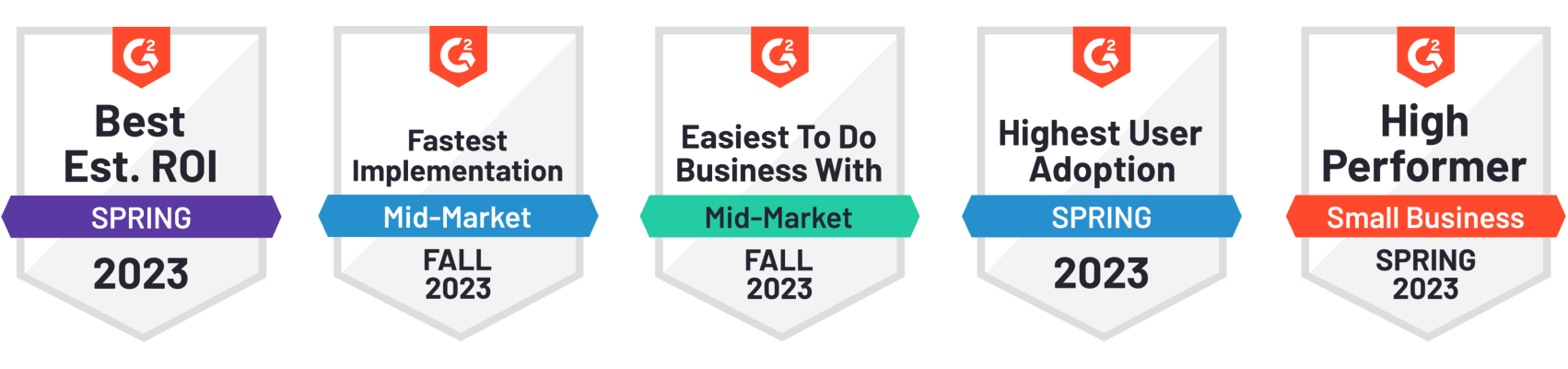