Data Structures form the backbone of efficient programming and are a key topic in technical interviews. As a recruiter or hiring manager, having a solid list of Data Structures interview questions is crucial for assessing candidates' knowledge and problem-solving abilities.
This blog post provides a comprehensive set of Data Structures interview questions tailored for different experience levels and roles. We cover general questions, junior and senior developer-specific inquiries, process-related queries, and situational questions to help you evaluate candidates thoroughly.
By using these questions, you can gain deeper insights into a candidate's understanding of Data Structures and their application in real-world scenarios. Consider complementing your interview process with a Data Structures online test to streamline your hiring workflow and identify top talent efficiently.
Table of contents
7 general Data Structures interview questions and answers to assess applicants
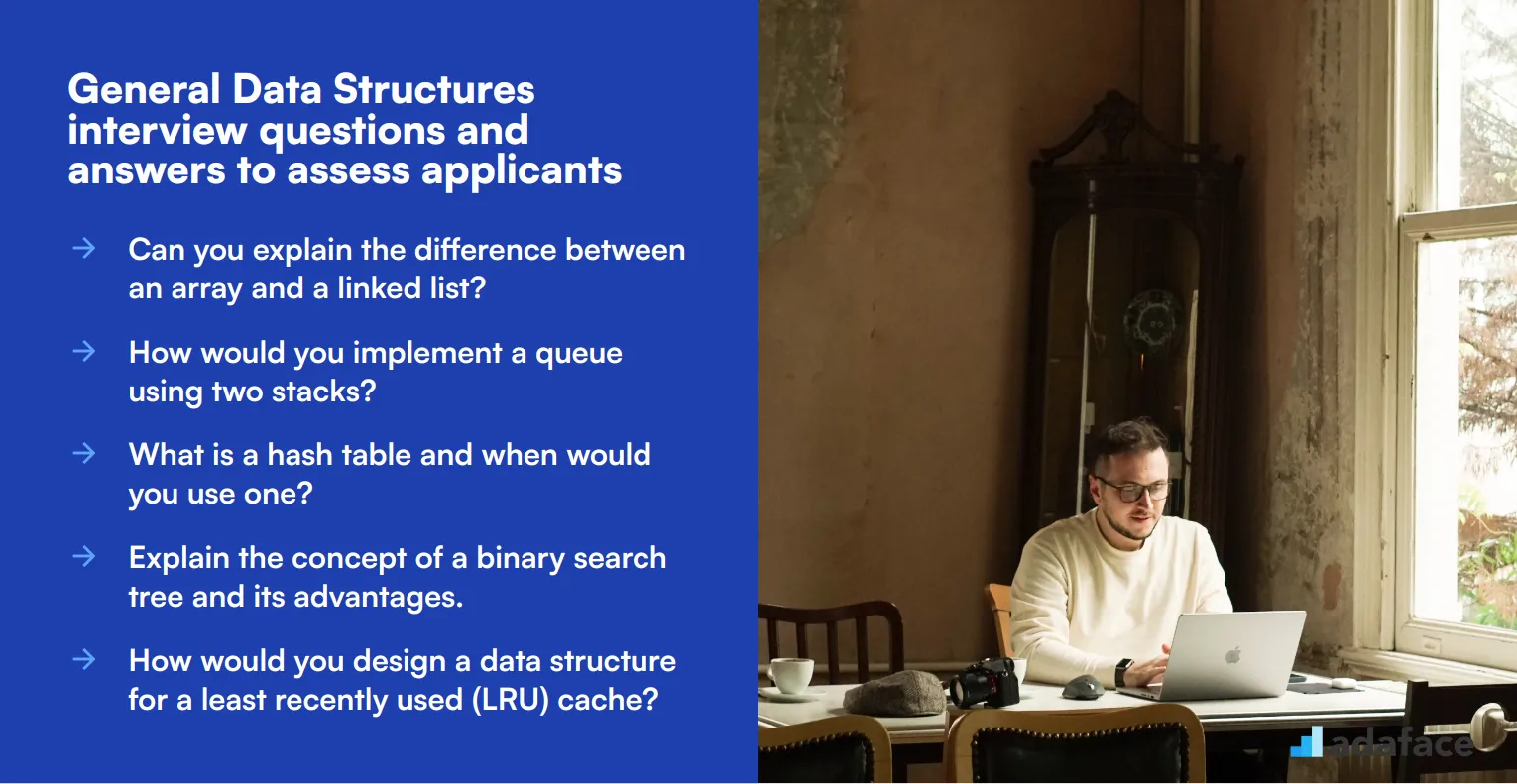
Ready to dive into the world of data structures? These seven questions will help you assess candidates' knowledge and problem-solving skills. Use them to gauge applicants' understanding of fundamental concepts and their ability to apply them in real-world scenarios. Remember, the goal is to spark discussions that reveal a candidate's thought process, not just their memorized facts.
1. Can you explain the difference between an array and a linked list?
Arrays and linked lists are both fundamental data structures, but they have key differences in how they store and access data.
Arrays store elements in contiguous memory locations, allowing for quick access to any element using an index. They have a fixed size, which is determined at the time of creation. Linked lists, on the other hand, consist of nodes where each node contains data and a reference (or link) to the next node. They can grow or shrink dynamically and don't require contiguous memory allocation.
Look for candidates who can explain the trade-offs between these structures. They should mention that arrays offer faster random access but are less flexible for insertion and deletion, while linked lists excel at insertion and deletion but have slower access times for arbitrary elements.
2. How would you implement a queue using two stacks?
Implementing a queue using two stacks is a classic problem that tests a candidate's ability to think creatively about data structures. The basic idea is to use one stack for enqueuing (adding) elements and another for dequeuing (removing) elements.
The process works like this:
- For enqueue operations, simply push the element onto the first stack.
- For dequeue operations, if the second stack is empty, pop all elements from the first stack and push them onto the second stack. Then pop from the second stack.
- If the second stack is not empty, simply pop from it.
Pay attention to how candidates explain the time complexity of these operations. An ideal answer should note that while enqueue operations are always O(1), dequeue operations are O(1) amortized but can be O(n) in the worst case when elements need to be transferred between stacks.
3. What is a hash table and when would you use one?
A hash table is a data structure that implements an associative array abstract data type, a structure that can map keys to values. It uses a hash function to compute an index into an array of buckets or slots, from which the desired value can be found.
Hash tables are particularly useful when you need fast insertion, deletion, and lookup operations. They're commonly used in:
- Implementing database indexes
- Caching mechanisms
- Symbol tables in compilers
- Associative arrays and sets in programming languages
Look for candidates who can explain the concept of collision resolution and discuss the trade-offs between different collision handling techniques like chaining and open addressing. They should also be able to discuss the importance of choosing a good hash function for performance optimization.
4. Explain the concept of a binary search tree and its advantages.
A binary search tree (BST) is a binary tree data structure with the key property that the left subtree of a node contains only nodes with keys less than the node's key, and the right subtree only nodes with keys greater than the node's key.
The main advantages of BSTs include:
- Efficient searching: Average case O(log n) for search, insert, and delete operations
- Ordered traversal: In-order traversal of a BST gives nodes in ascending order
- Relatively simple implementation compared to more complex self-balancing trees
When evaluating responses, look for candidates who can discuss the importance of tree balance for maintaining efficiency. They should also be aware that in worst-case scenarios (like inserting already sorted data), a BST can degenerate into a linked list, losing its performance benefits.
5. How would you design a data structure for a least recently used (LRU) cache?
An LRU cache is a type of cache that removes the least recently used items first when the cache reaches its capacity. A good design for an LRU cache typically combines a hash table for fast lookups and a doubly linked list for quick removal and addition of elements.
The basic structure would include:
- A hash table mapping keys to nodes in the doubly linked list
- A doubly linked list to maintain the order of usage
- Methods for get (accessing an item) and put (adding/updating an item)
- A capacity limit to determine when to evict items
Evaluate candidates based on their ability to explain how these components work together. They should describe how accessing or adding an item moves it to the front of the list, and how the least recently used item (at the tail of the list) is removed when the cache is full. Bonus points if they mention potential optimizations or discuss the time complexity of operations.
6. What is the difference between a stack and a queue, and can you give an example of when you'd use each?
Stacks and queues are both linear data structures, but they differ in how elements are added and removed. A stack follows the Last-In-First-Out (LIFO) principle, while a queue follows the First-In-First-Out (FIFO) principle.
Examples of stack usage:
- Function call stack in programming languages
- Undo mechanism in text editors
- Backtracking algorithms
Examples of queue usage:
- Task scheduling in operating systems
- Breadth-first search in graph algorithms
- Print job spooling
Look for candidates who can clearly articulate the operational differences and provide relevant real-world examples. They should understand that the choice between a stack and a queue depends on the specific requirements of the problem at hand, particularly regarding the order in which elements need to be processed.
7. Can you explain what a trie is and when it might be useful?
A trie, also called a prefix tree, is a tree-like data structure used to store and retrieve strings. Each node in the trie represents a character, and the path from the root to a node represents a prefix of one or more strings.
Tries are particularly useful for:
- Autocomplete and predictive text systems
- IP routing tables in network routers
- Spell checkers
- Implementing dictionaries with prefix-based searches
When evaluating responses, look for candidates who can explain the space-time tradeoffs of tries. They should mention that tries can be more space-efficient than storing whole strings when there are many common prefixes, and that they allow for fast prefix-based operations. Candidates might also discuss potential optimizations like path compression in Patricia tries.
20 Data Structures interview questions to ask junior developers
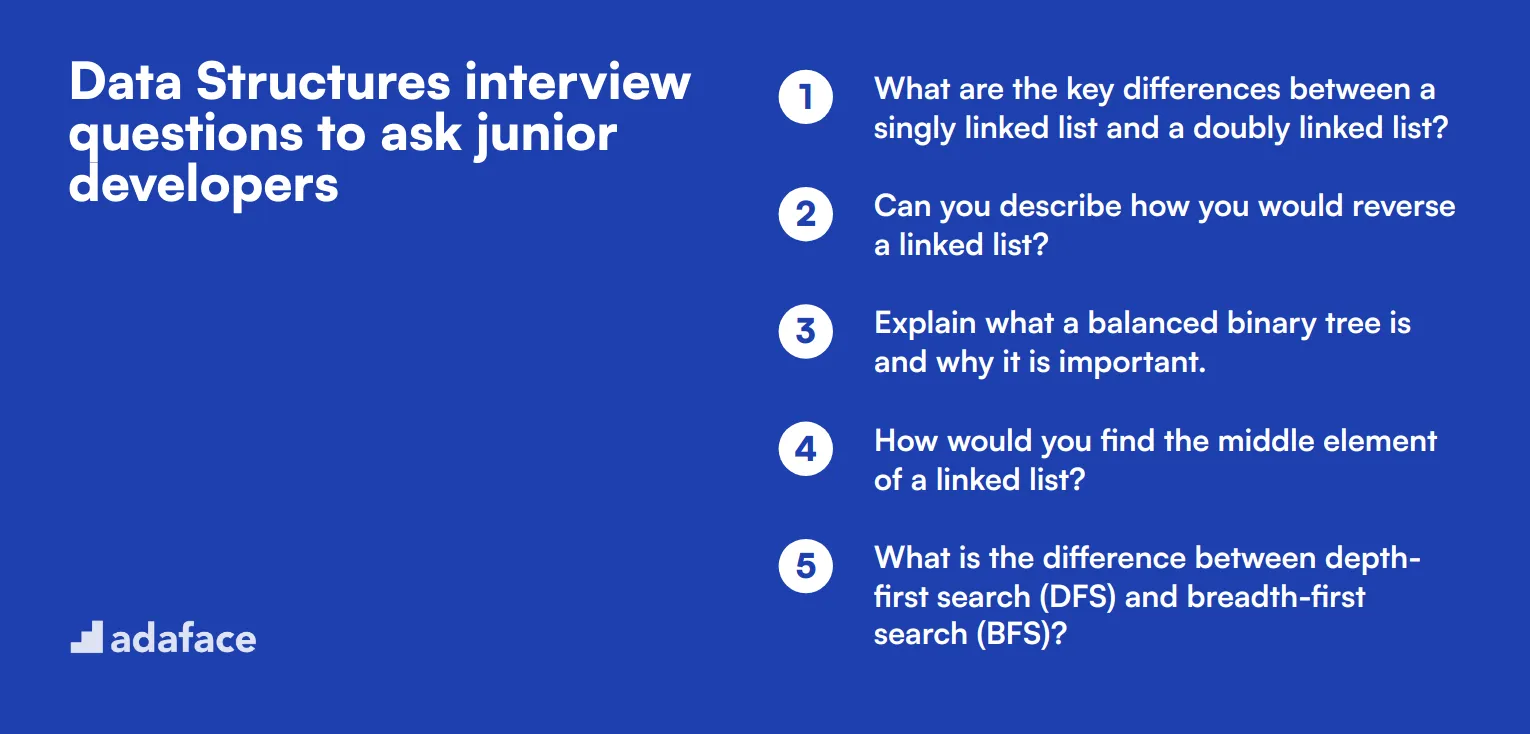
To effectively evaluate the foundational knowledge of junior developers in data structures, consider using these 20 targeted interview questions. Tailor these questions to fit your specific needs, whether you're hiring for a role like data engineer or a database developer. These questions will help you gauge their understanding and problem-solving skills.
- What are the key differences between a singly linked list and a doubly linked list?
- Can you describe how you would reverse a linked list?
- Explain what a balanced binary tree is and why it is important.
- How would you find the middle element of a linked list?
- What is the difference between depth-first search (DFS) and breadth-first search (BFS)?
- Can you explain what a priority queue is and how it works?
- How would you detect a cycle in a linked list?
- What are red-black trees and where are they used?
- Describe the advantages and disadvantages of using a hash table.
- How would you implement a stack using an array?
- What is a graph and how can it be represented in a computer program?
- Can you explain the difference between directed and undirected graphs?
- What is a heap and how is it different from a binary search tree?
- How would you merge two sorted linked lists?
- Explain what dynamic arrays are and how they differ from regular arrays.
- What is a segment tree and in what scenarios would you use one?
- How would you implement a queue using a circular array?
- What is the significance of AVL trees in data structure?
- Describe a scenario where you would use a skip list.
- How do you implement an adjacency matrix and an adjacency list for graph data structures?
8 advanced Data Structures interview questions and answers to evaluate senior developers
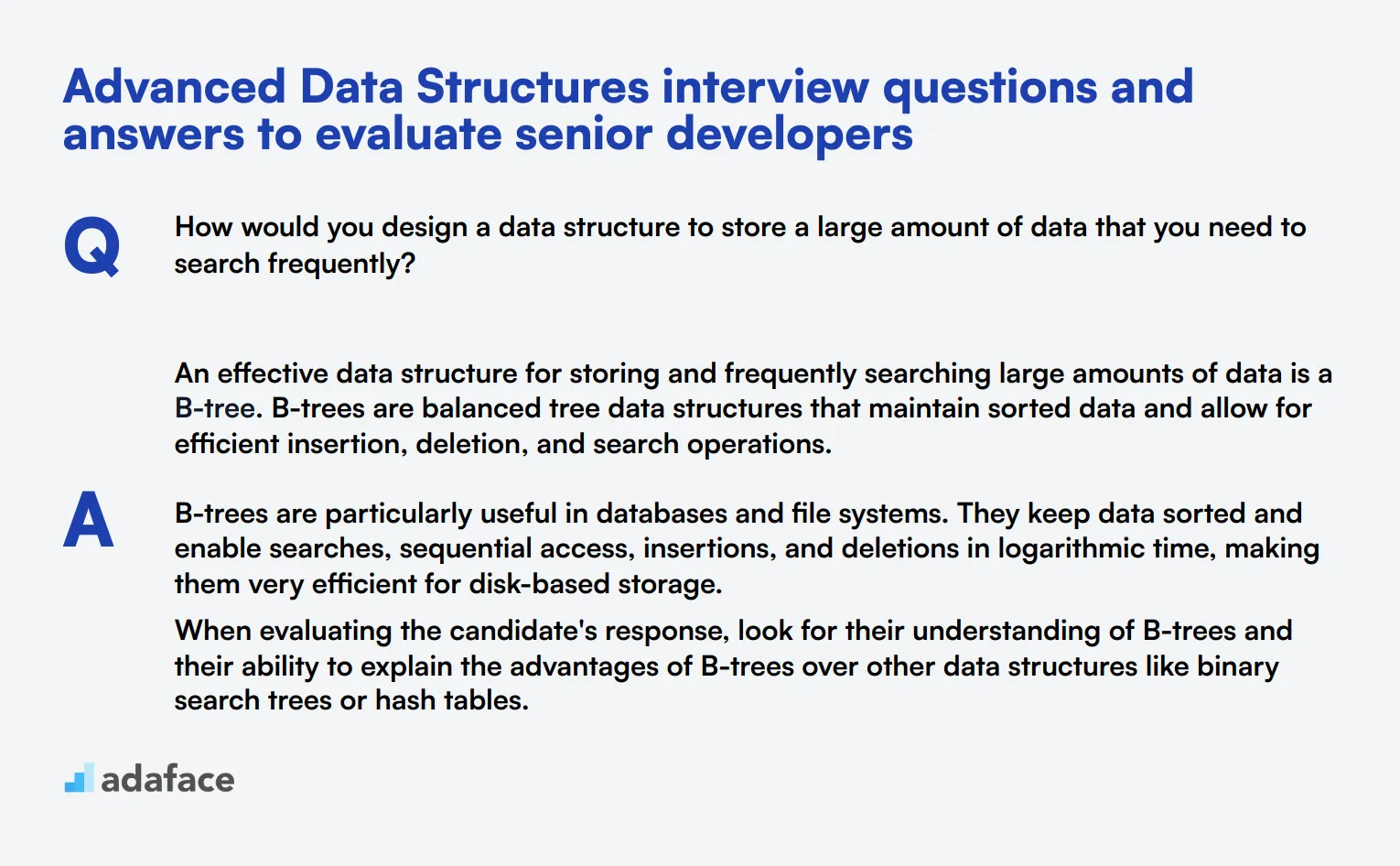
To evaluate whether your senior developer candidates have the advanced skills and deep understanding necessary for complex data structure tasks, ask them some of these advanced data structures interview questions. These questions are designed to help you identify candidates who possess a strong grasp of sophisticated concepts and can apply them effectively.
1. How would you design a data structure to store a large amount of data that you need to search frequently?
An effective data structure for storing and frequently searching large amounts of data is a B-tree. B-trees are balanced tree data structures that maintain sorted data and allow for efficient insertion, deletion, and search operations.
B-trees are particularly useful in databases and file systems. They keep data sorted and enable searches, sequential access, insertions, and deletions in logarithmic time, making them very efficient for disk-based storage.
When evaluating the candidate's response, look for their understanding of B-trees and their ability to explain the advantages of B-trees over other data structures like binary search trees or hash tables.
2. Can you explain what a Bloom filter is and when it might be useful?
A Bloom filter is a probabilistic data structure used to test whether an element is a member of a set. It is highly space-efficient but allows for false positives. This means it can tell you if an element is possibly in the set or definitely not in the set.
Bloom filters are particularly useful in applications where memory space is limited, and the cost of occasional false positives is acceptable, such as in cache mechanisms or in checking membership of large-scale data sets in distributed systems.
An ideal candidate should mention the trade-offs involved, such as the presence of false positives and the lack of false negatives, and give examples of scenarios where Bloom filters are advantageous.
3. Can you describe how a Fibonacci heap works and its applications?
A Fibonacci heap is a data structure consisting of a collection of trees which are min-heap ordered. It has a better amortized running time for some operations compared to other heap data structures.
Fibonacci heaps are particularly beneficial in algorithmic applications like Dijkstra's and Prim's algorithms for shortest paths and minimum spanning trees, respectively. Their key advantage is the decrease-key operation, which is more efficient than in binary or binomial heaps.
When candidates explain this, they should highlight the importance of amortized time complexity and why Fibonacci heaps can be more efficient for specific operations in certain algorithms.
4. What is a Deque and where would you use it?
A Deque (Double-Ended Queue) is an abstract data type that allows insertion and deletion of elements from both ends—front and back. This makes it flexible for various applications that require elements to be added or removed from both ends efficiently.
Deques are useful in scenarios like implementing palindromes checkers, maintaining the sliding window of maximum or minimum values in an array, and in some algorithms for breadth-first search (BFS).
In a candidate's response, look for their understanding of the versatility of Deques and examples of practical applications where such a data structure is beneficial.
5. Explain the concept of a Van Emde Boas tree and its advantages.
A Van Emde Boas tree is a tree data structure that supports efficient successor and predecessor queries in O(log log N) time, where N is the size of the universe of possible values. This makes it highly efficient for certain types of operations.
The Van Emde Boas tree is advantageous in scenarios where you need to perform a high number of predecessor and successor queries efficiently, such as in computational geometry and network routing.
Ideal responses should demonstrate a candidate’s understanding of the unique time complexities of the Van Emde Boas tree and how it can be applied to optimize specific algorithms.
6. What is a Splay tree and how does it differ from other self-balancing trees?
A Splay tree is a self-adjusting binary search tree where frequently accessed elements are moved closer to the root, thereby improving access times for those elements. This is achieved through a series of tree rotations called splaying.
The primary difference between splay trees and other self-balancing trees like AVL or Red-Black trees is that splay trees do not maintain a strict balance. Instead, they adjust dynamically based on access patterns, which can be highly efficient for certain workloads.
When candidates explain this, they should discuss the scenarios where splay trees are particularly useful, such as in data compression or implementing caches, and the trade-offs involved compared to other balanced trees.
7. Can you discuss the advantages and disadvantages of using a Skip list?
A Skip list is a data structure that allows fast search, insertion, and deletion operations in O(log N) average time. It consists of multiple linked lists at different levels, where each level skips over a fixed number of elements, allowing for rapid traversal.
Skip lists are advantageous because they are relatively simple to implement and provide good average-case performance without the need for complex balancing operations, unlike balanced trees. However, they can have higher memory overhead due to the multiple levels of linked lists.
In an ideal response, candidates should explain the trade-offs between skip lists and other structures like balanced trees or hash tables, and provide examples of where skip lists might be preferable.
8. Explain what a K-d tree is and its applications.
A K-d tree (K-dimensional tree) is a space-partitioning data structure for organizing points in a k-dimensional space. It is particularly useful in multidimensional search queries such as range searches and nearest neighbor searches.
K-d trees are commonly used in applications like computer graphics, machine learning for nearest neighbor classification, and in robotics for motion planning and collision detection.
Candidates should mention how K-d trees partition space and provide examples of practical applications where such a structure is advantageous. Look for an understanding of the trade-offs in terms of performance and complexity.
10 Data Structures interview questions about processes and tasks
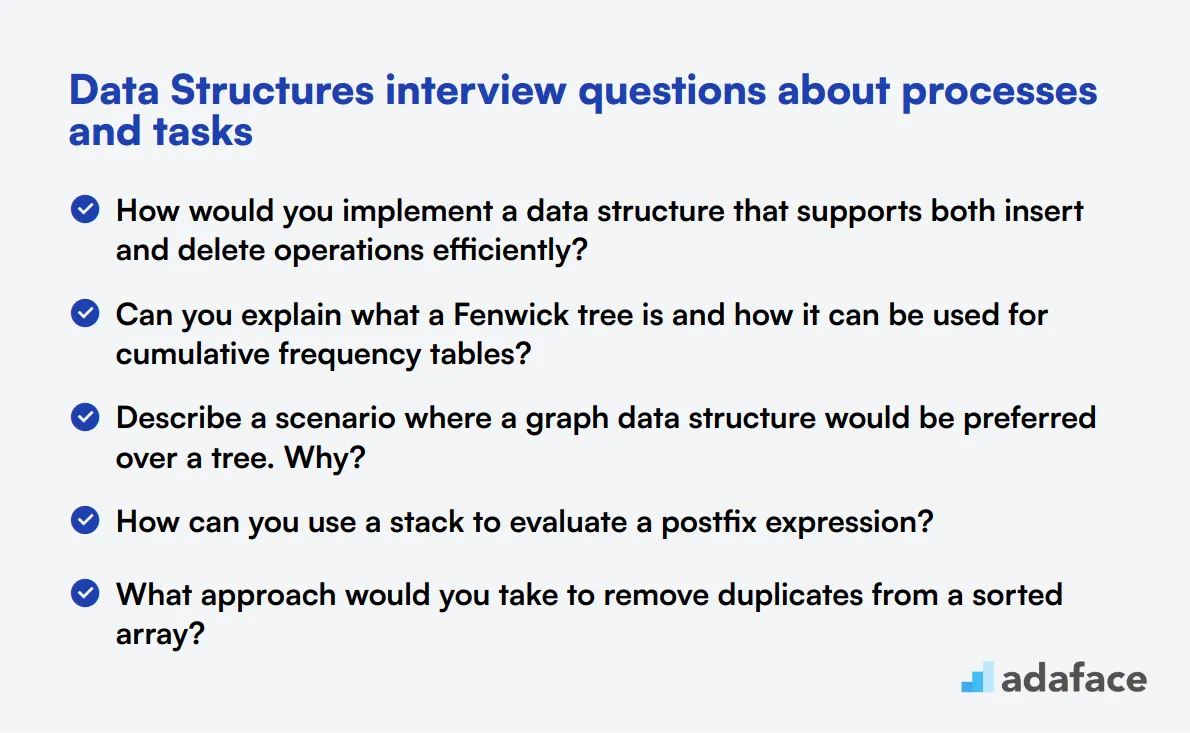
To accurately assess candidates' ability to handle various data processes, use this list of interview questions. These questions will help you gauge their understanding of data structure applications in real-world scenarios, ensuring you find the right fit for your team of data professionals like a data engineer.
- How would you implement a data structure that supports both insert and delete operations efficiently?
- Can you explain what a Fenwick tree is and how it can be used for cumulative frequency tables?
- Describe a scenario where a graph data structure would be preferred over a tree. Why?
- How can you use a stack to evaluate a postfix expression?
- What approach would you take to remove duplicates from a sorted array?
- How would you implement a data structure that supports finding the median in constant time?
- Can you explain the concept of a disjoint-set data structure and its applications?
- What is a bloom filter, and how can it be used to optimize searching in large datasets?
- How would you design a data structure for an autocomplete feature?
- Explain how you can use a heap to find the k largest elements in an array.
10 Data Structures situational interview questions with answers for hiring top developers
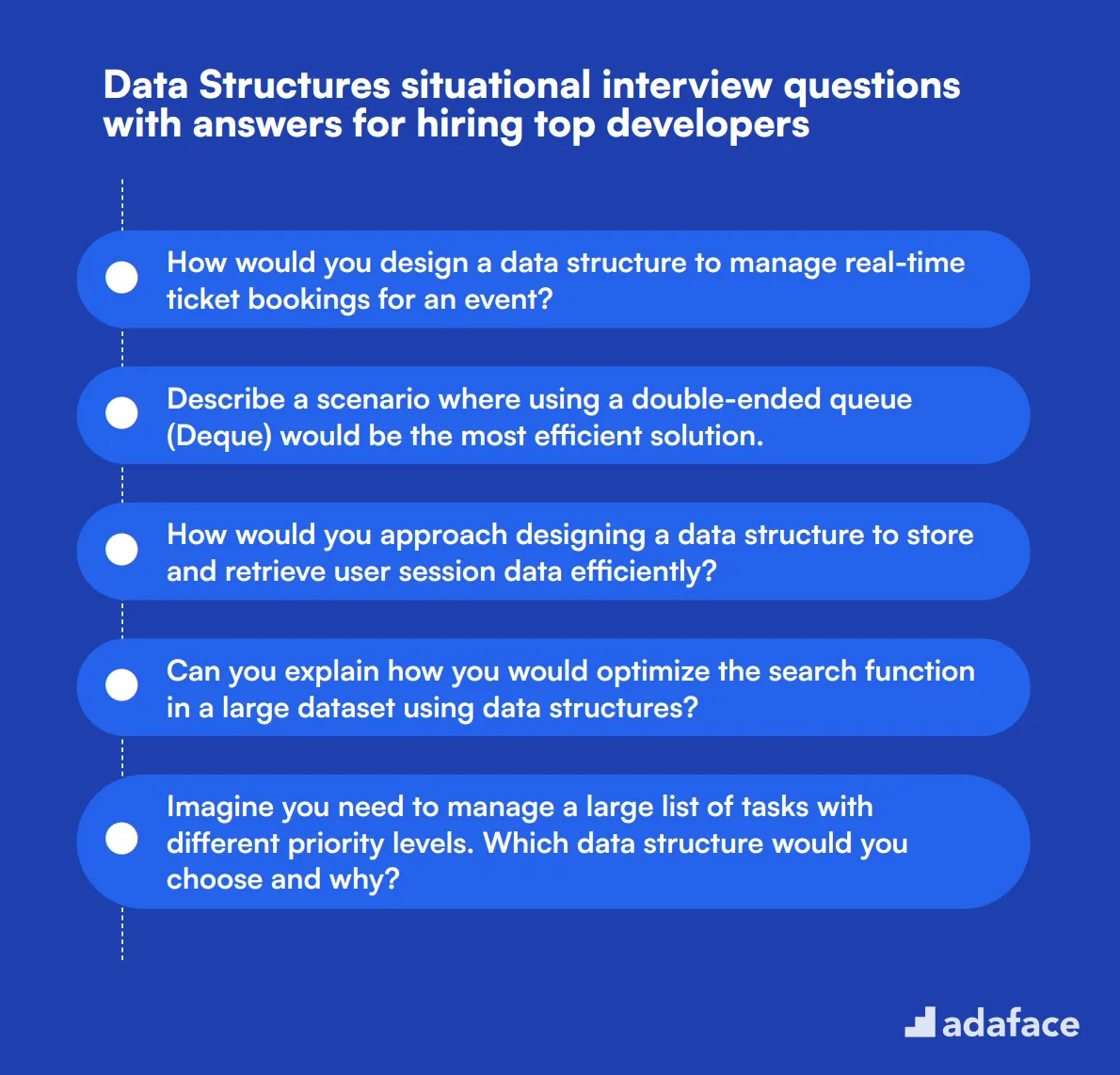
To identify top developers with practical knowledge in data structures, consider using some of these situational interview questions designed to assess problem-solving skills and technical expertise. These questions are particularly useful for roles such as data engineers and database developers, allowing you to gauge how candidates handle real-world scenarios.
- How would you design a data structure to manage real-time ticket bookings for an event?
- Describe a scenario where using a double-ended queue (Deque) would be the most efficient solution.
- How would you approach designing a data structure to store and retrieve user session data efficiently?
- Can you explain how you would optimize the search function in a large dataset using data structures?
- Imagine you need to manage a large list of tasks with different priority levels. Which data structure would you choose and why?
- How would you design a data structure to handle autocomplete suggestions for a search engine?
- Describe how you would implement a newsroom feed that always shows the most recent articles at the top.
- How would you design a data structure to support real-time analytics on streaming data?
- Explain how you would manage a system that tracks the highest scores in a game leaderboard.
- How would you implement a system to efficiently find and remove the oldest log entries in a rotating log file?
Which Data Structures skills should you evaluate during the interview phase?
In a single interview, it's challenging to evaluate all the candidate's skills comprehensively. However, when assessing Data Structures expertise, a few core skills can provide critical insights into their potential. Focusing on these skills will help you identify the most qualified candidates for your technical team.
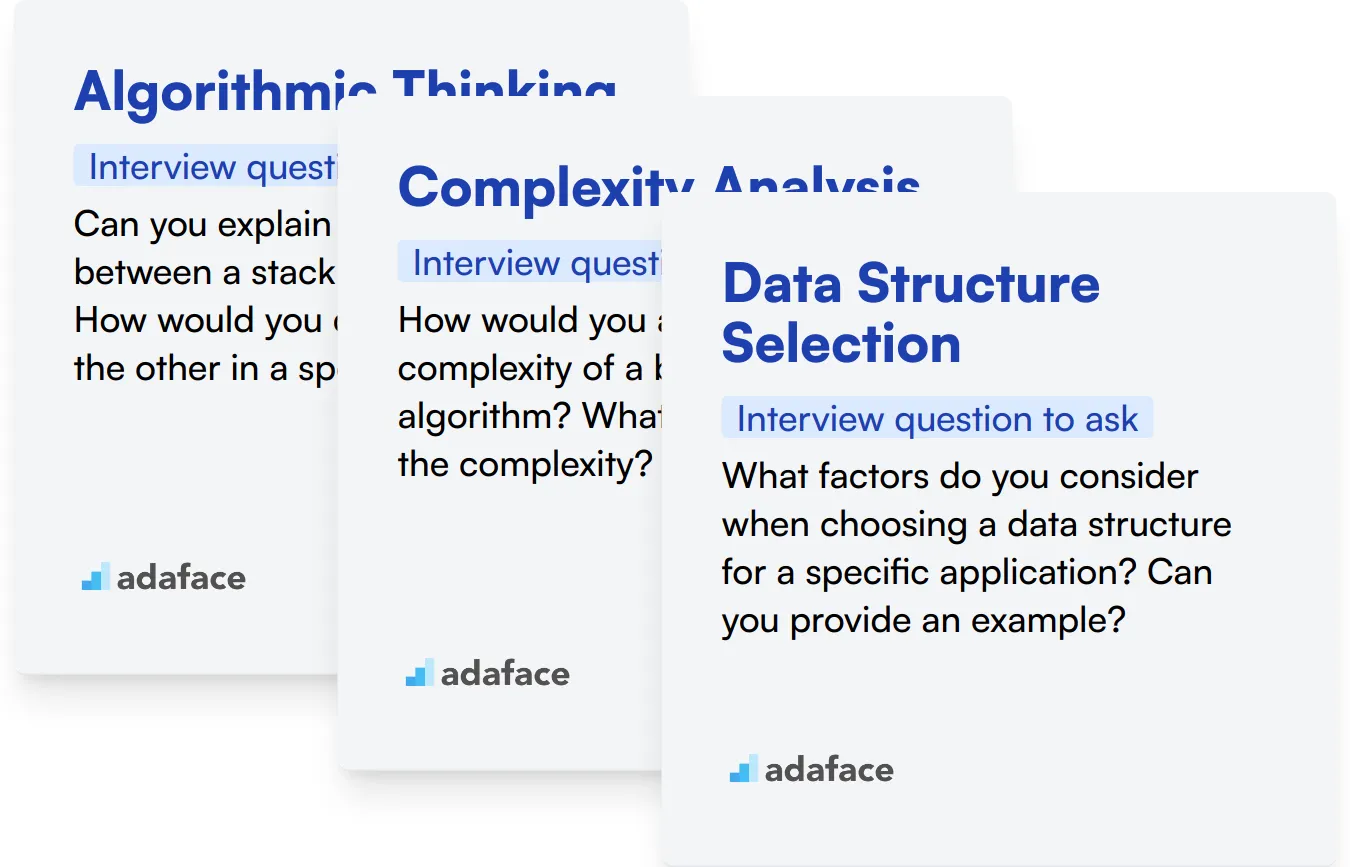
Algorithmic Thinking
You can utilize an assessment test that includes relevant multiple-choice questions to measure this skill effectively. For a structured evaluation, consider checking out the Data Structures test in our library.
Additionally, asking targeted interview questions can help gauge their level of understanding in algorithmic thinking. Here's a question that can provide insight into their skills:
Can you explain the difference between a stack and a queue? How would you choose one over the other in a specific scenario?
When this question is posed, look for clarity in their explanation and the ability to discuss use cases for each structure. A strong candidate will demonstrate not just knowledge but also practical application in their response.
Complexity Analysis
To evaluate this skill, consider using an assessment that includes relevant MCQs. You might find useful questions in the Data Structures test available in our library.
You can also ask specific questions to assess their grasp of complexity analysis. Here’s a question to consider:
How would you analyze the time complexity of a binary search algorithm? What factors influence the complexity?
When discussing this question, pay attention to their ability to articulate the steps of the analysis and recognize factors that affect efficiency, such as input size and structure.
Data Structure Selection
You can assess this capability through an assessment that includes targeted questions. For example, the Data Structures test in our library can be a resourceful tool for this purpose.
As part of the interview, consider asking questions that assess their decision-making process. Here’s one you can use:
What factors do you consider when choosing a data structure for a specific application? Can you provide an example?
As they answer, listen for their understanding of trade-offs between different structures and how they relate to the application's requirements. Strong candidates will cite examples from previous experience.
Hire top talent with Data Structures skills tests and the right interview questions
If you're looking to hire someone with strong Data Structures skills, it's important to ensure that they possess those skills accurately. This helps you avoid costly hiring mistakes and find the right fit for your team.
The most effective way to assess these skills is by using skill tests. Consider utilizing the Data Structures online test to accurately gauge candidates' abilities.
Once you complete the test, you can shortlist the best applicants and invite them for interviews. This streamlined approach saves time and increases your chances of finding a qualified candidate.
To get started, you can sign up for our platform here and access a range of relevant assessments tailored to your hiring needs.
Data Structures Test
Download Data Structures interview questions template in multiple formats
Data Structures Interview Questions FAQs
Focus on understanding of fundamental concepts, problem-solving skills, and the ability to apply knowledge in practical scenarios.
Ask simpler, concept-based questions for junior candidates and complex, scenario-based questions for senior candidates.
They provide insights into how candidates handle real-world challenges, their thought process, and their problem-solving tactics.
A good question tests both theoretical knowledge and practical application, and is open-ended to gauge depth of understanding.
Data Structures are fundamental to many technical roles, so it's crucial to assess candidates' proficiency in this area thoroughly.
Present them with a problem and ask them to explain their approach, discussing the choice of Data Structures and the algorithms involved.
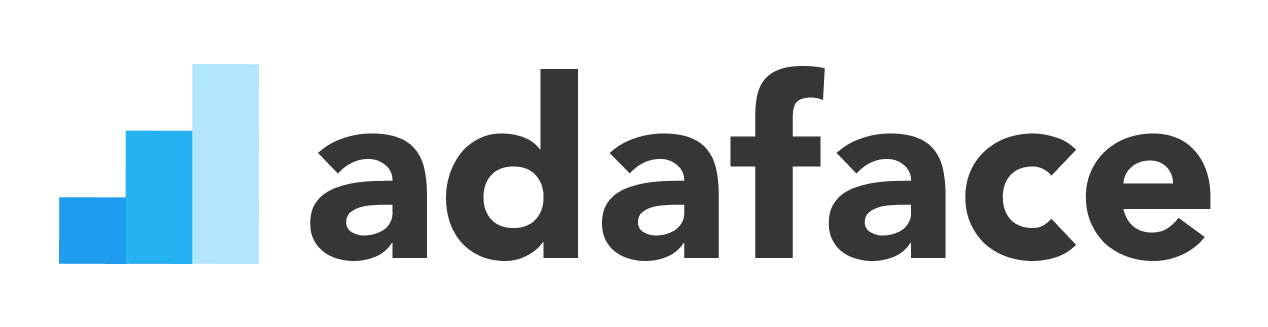
40 min skill tests.
No trick questions.
Accurate shortlisting.
We make it easy for you to find the best candidates in your pipeline with a 40 min skills test.
Try for freeRelated posts
Free resources
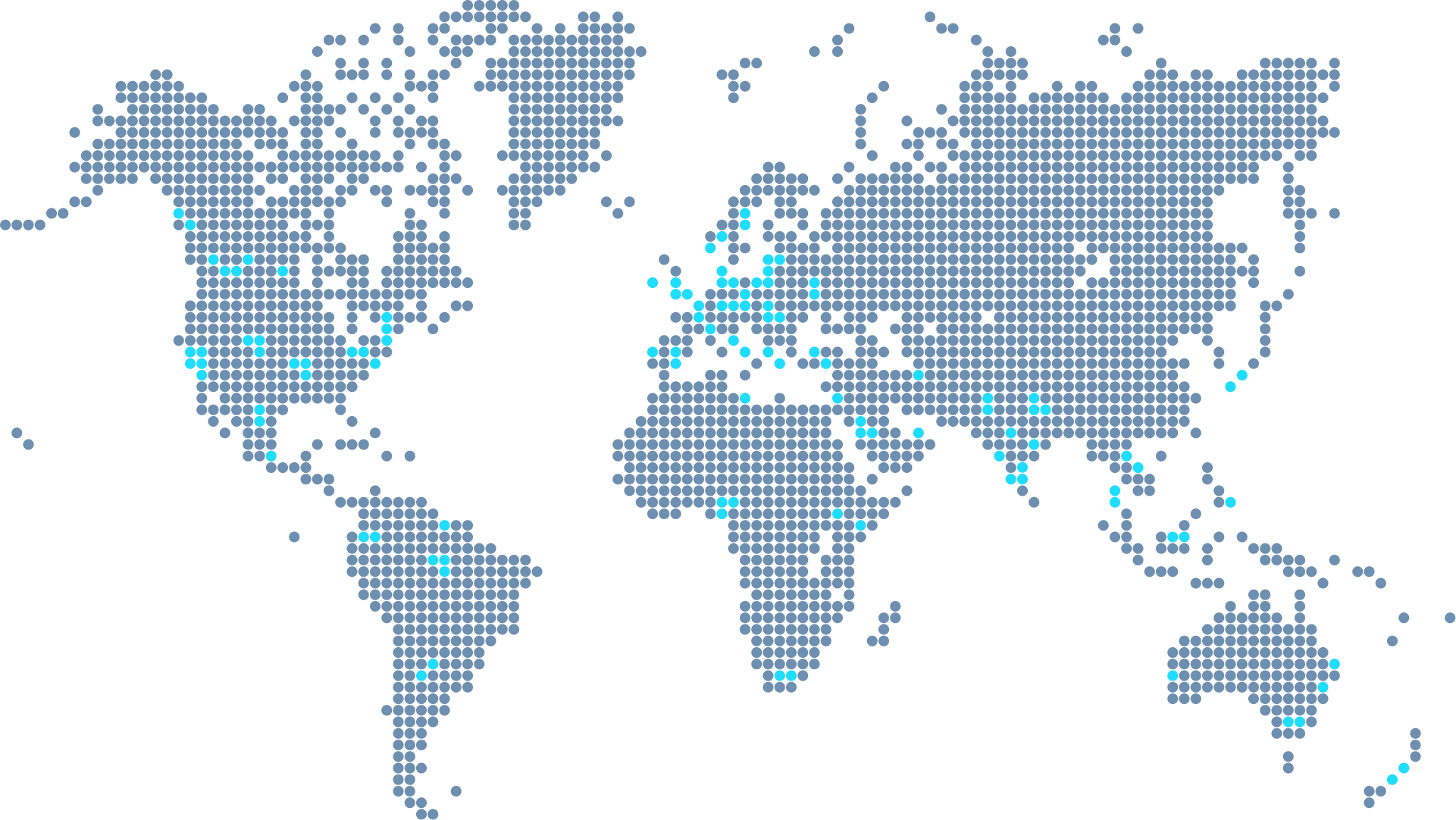
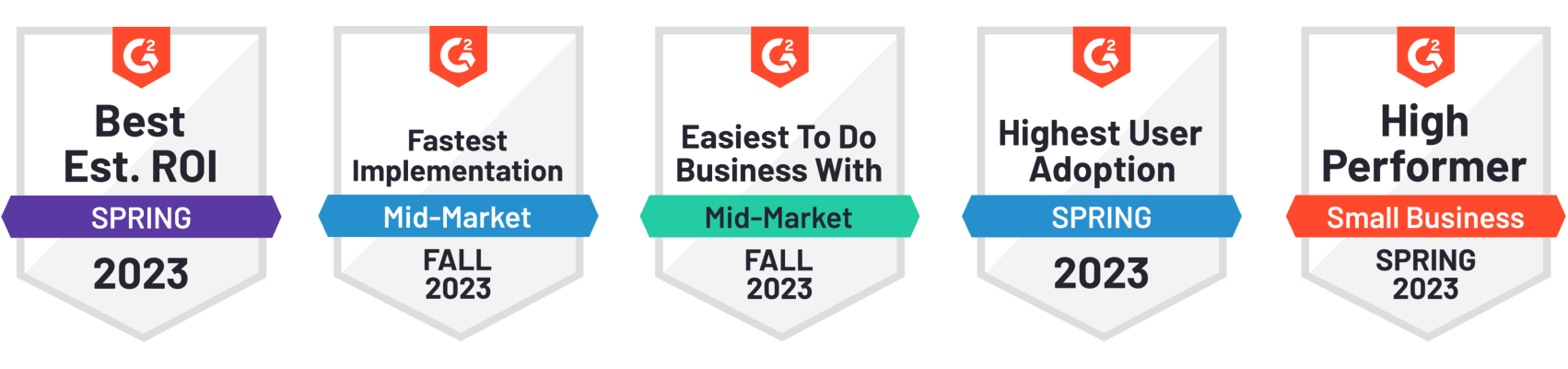