Hiring the right C++ developer can be a challenge, especially when you're not sure what questions to ask during the interview process. A well-structured set of interview questions can help you assess a candidate's technical skills, problem-solving abilities, and overall fit for your team.
This blog post provides a comprehensive list of C++ interview questions tailored for different experience levels, from basic to advanced. We've categorized the questions to cover fundamental concepts, data structures, memory management, and real-world scenarios that C++ developers might encounter.
By using these questions, you can gain valuable insights into a candidate's C++ proficiency and make informed hiring decisions. Consider combining these interview questions with a C++ skills assessment to get a more complete picture of a candidate's abilities before the interview stage.
Table of contents
10 basic C++ interview questions and answers to assess applicants
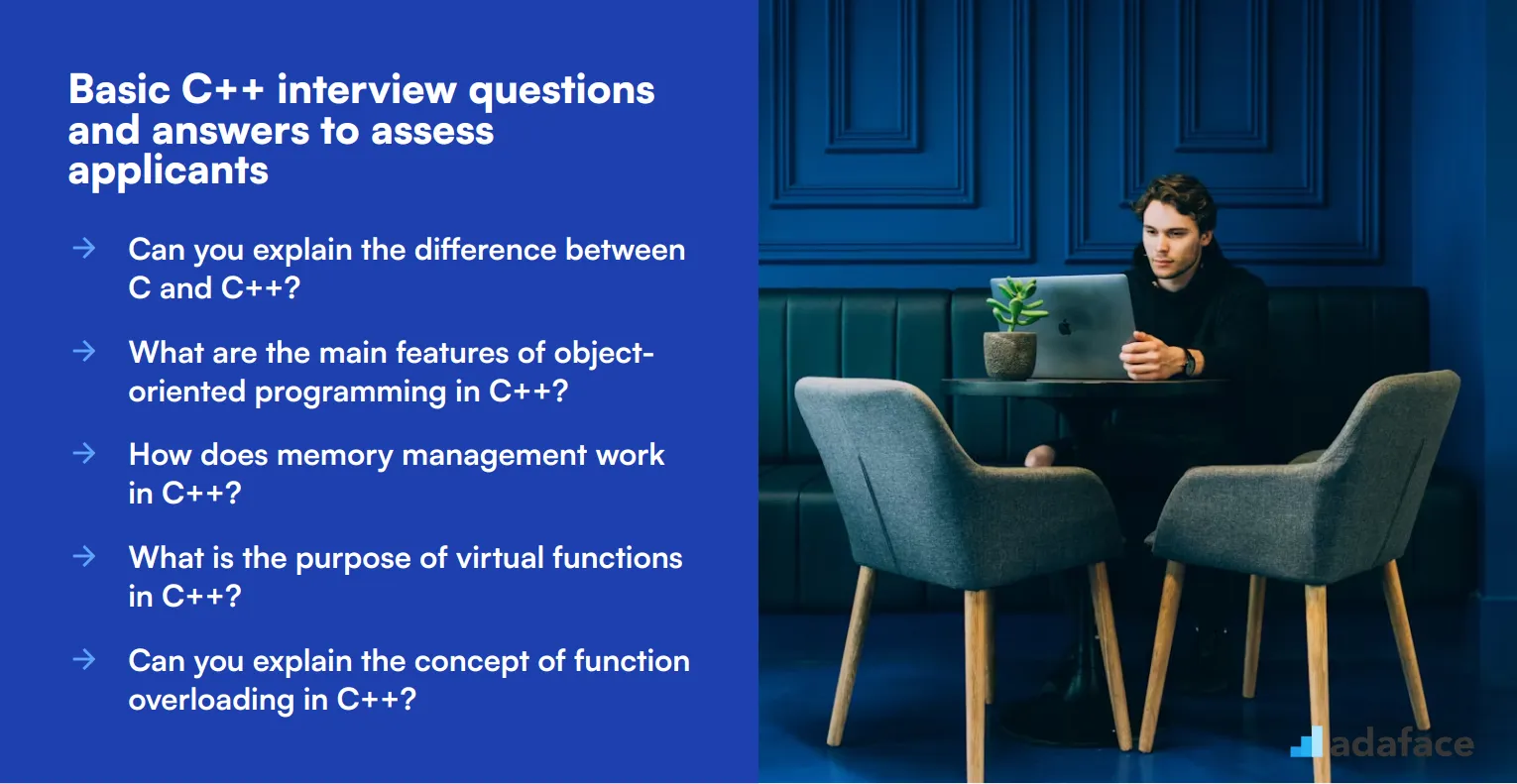
Ready to assess your C++ developer candidates? These 10 basic C++ interview questions are your secret weapon for spotting top talent. Use them to gauge applicants' fundamental understanding and problem-solving skills. Remember, these questions are designed to spark insightful discussions, not just elicit textbook answers. Let's dive in and find your next C++ superstar!
1. Can you explain the difference between C and C++?
C++ is an extension of C, adding object-oriented programming features. While C is a procedural language, C++ supports both procedural and object-oriented paradigms.
Key differences include:
- C++ supports classes and objects
- C++ allows function overloading
- C++ has built-in exception handling
- C++ includes references in addition to pointers
Look for candidates who can clearly articulate these differences and provide examples of when they'd use C++ over C. Strong answers will demonstrate an understanding of both languages' strengths and use cases.
2. What are the main features of object-oriented programming in C++?
The main features of object-oriented programming in C++ are:
- Encapsulation: Bundling data and methods that operate on that data
- Inheritance: Creating new classes based on existing ones
- Polymorphism: Using a single interface for different underlying forms
- Abstraction: Hiding complex implementation details
A strong candidate should be able to explain each concept and provide simple, real-world analogies. For example, they might compare inheritance to genetic traits passed from parents to children.
Pay attention to how well the candidate can relate these concepts to practical programming scenarios. Their ability to draw connections between theory and practice is a good indicator of their experience level.
3. How does memory management work in C++?
Memory management in C++ involves two main types: stack and heap. The stack is used for static memory allocation and is managed automatically. The heap is used for dynamic memory allocation and must be managed manually by the programmer.
Key points to listen for:
- Use of 'new' and 'delete' operators for heap allocation
- Understanding of memory leaks and how to prevent them
- Awareness of smart pointers as a modern alternative
An ideal response should demonstrate an understanding of the responsibilities that come with manual memory management. Look for candidates who mention best practices like RAII (Resource Acquisition Is Initialization) or discuss the trade-offs between different memory management strategies.
4. What is the purpose of virtual functions in C++?
Virtual functions in C++ are used to achieve runtime polymorphism. They allow a base class pointer to call the correct derived class function based on the actual object type at runtime.
Key points to listen for:
- Understanding of the 'virtual' keyword
- Explanation of the vtable mechanism
- Awareness of pure virtual functions and abstract classes
A strong candidate should be able to explain scenarios where virtual functions are beneficial, such as in designing extensible frameworks or implementing the Strategy pattern. Look for answers that demonstrate an understanding of both the technical implementation and the design implications of using virtual functions.
5. Can you explain the concept of function overloading in C++?
Function overloading in C++ allows multiple functions with the same name but different parameters. This enables creating functions that perform similar tasks but with different input types or number of arguments.
Key aspects to listen for:
- Understanding that return type alone is not sufficient for overloading
- Awareness of how the compiler resolves overloaded functions
- Knowledge of potential pitfalls, like ambiguous overloads
Look for candidates who can provide practical examples of when function overloading is useful, such as in mathematical operations or string manipulation. Strong answers might also touch on how overloading relates to object-oriented design principles like polymorphism.
6. What are templates in C++ and why are they useful?
Templates in C++ are a powerful feature that allows writing generic code. They enable creating functions or classes that can work with different data types without repeating code.
Key points to cover:
- Syntax for function and class templates
- Concept of compile-time polymorphism
- Use cases like creating container classes or generic algorithms
A strong candidate should be able to explain how templates improve code reusability and type safety. Look for answers that demonstrate an understanding of template specialization and the trade-offs of using templates, such as increased compile times and potentially cryptic error messages.
7. How does exception handling work in C++?
Exception handling in C++ provides a structured way to deal with runtime errors. It uses try, catch, and throw keywords to separate error-handling code from normal code flow.
Key concepts to listen for:
- Understanding of try-catch blocks
- Proper use of the throw keyword
- Knowledge of exception hierarchies and catching multiple exceptions
Look for candidates who can explain best practices, such as using specific exception types, handling exceptions at the appropriate level, and cleaning up resources properly. Strong answers might also touch on the performance implications of exceptions and when to use them versus other error-handling techniques.
8. What is the difference between references and pointers in C++?
References and pointers in C++ are both used to indirectly access variables, but they have some key differences:
- References must be initialized when declared, pointers can be initialized later
- References cannot be null, pointers can
- References cannot be reassigned to refer to different objects, pointers can
A good answer should also mention:
- References are often safer and easier to use
- Pointers offer more flexibility, especially for dynamic memory allocation
- The syntax differences in declaration and usage
Look for candidates who can explain when to use each and why. Strong answers might include discussion of const references for function parameters or the use of smart pointers in modern C++.
9. What are the access specifiers in C++ and how do they affect class members?
C++ has three main access specifiers: public, private, and protected. These control the visibility and accessibility of class members:
- Public: Accessible from anywhere
- Private: Accessible only within the class
- Protected: Accessible within the class and its derived classes
Look for candidates who understand how these specifiers relate to encapsulation and data hiding. Strong answers might discuss the principle of keeping data members private and providing public methods for interaction, or explain how protected members facilitate certain inheritance patterns.
10. Can you explain the concept of operator overloading in C++?
Operator overloading in C++ allows redefining the behavior of operators for user-defined types. This enables custom types to use familiar operator syntax, making code more intuitive and readable.
Key points to cover:
- Syntax for overloading operators as member functions or global functions
- Common operators to overload (e.g., +, -, ==, <<)
- Limitations and best practices in operator overloading
A strong candidate should be able to provide examples of when operator overloading is useful, such as with complex number or matrix classes. Look for answers that demonstrate an understanding of the potential pitfalls, like maintaining expected operator behavior and avoiding unnecessary overloading.
20 C++ interview questions to ask junior developers
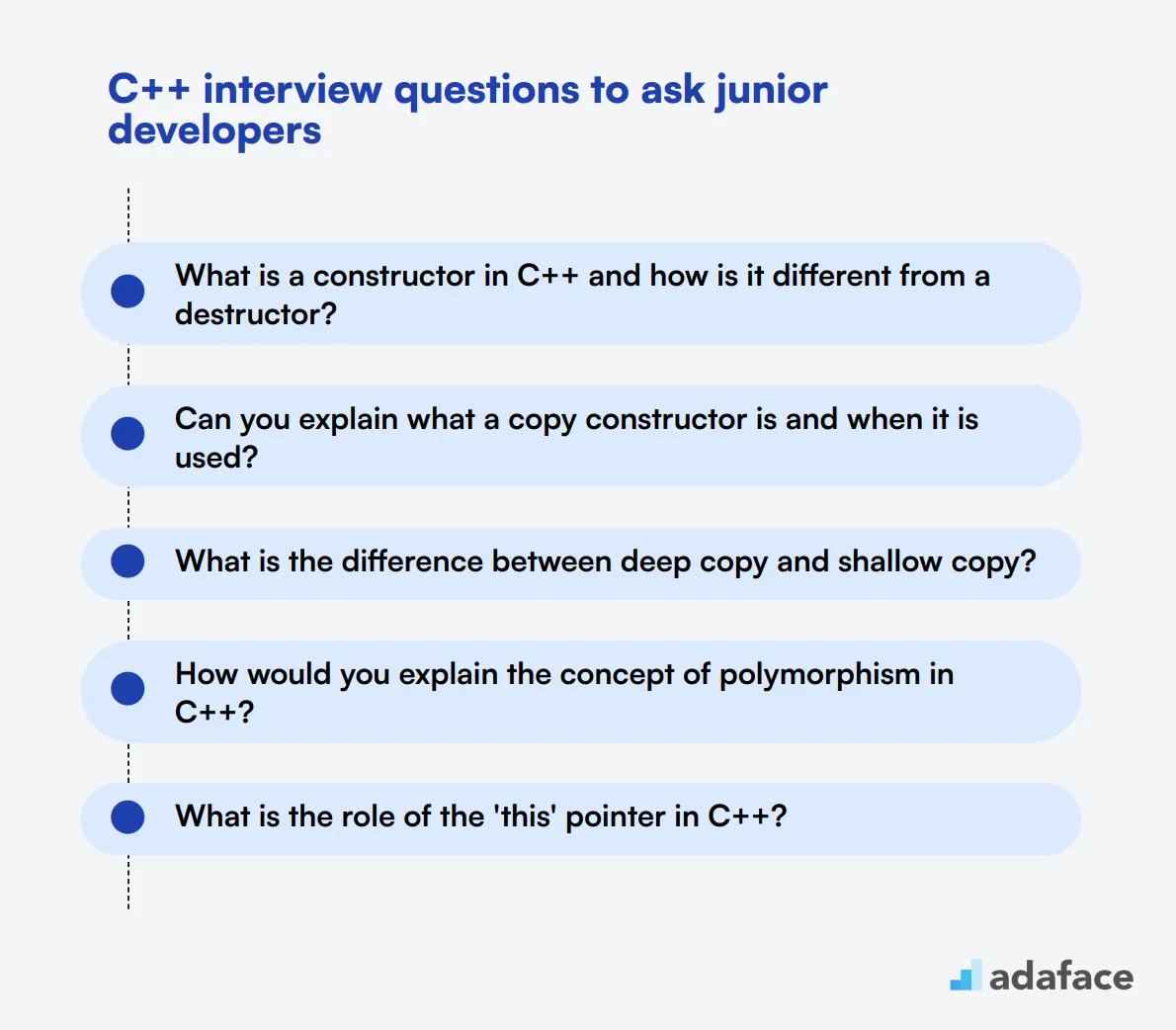
To assess whether your junior developer candidates possess the necessary skills and understanding of C++, consider incorporating these 20 targeted interview questions into your hiring process. These questions will help you gauge the applicants' technical knowledge and problem-solving abilities, ensuring they are well-prepared for the role. For more insights, refer to this C++ developer job description.
- What is a constructor in C++ and how is it different from a destructor?
- Can you explain what a copy constructor is and when it is used?
- What is the difference between deep copy and shallow copy?
- How would you explain the concept of polymorphism in C++?
- What is the role of the 'this' pointer in C++?
- Can you describe what namespaces are and why they are important?
- What is a friend function and what is its purpose in C++?
- How do you manage dynamic memory allocation using new and delete in C++?
- What is the purpose of the 'const' keyword in C++?
- How does C++ handle multiple inheritance and what are the potential issues?
- What is the difference between a class and a struct in C++?
- Can you explain what a pure virtual function is and its role in C++?
- What are smart pointers and why are they used in C++?
- How does the Standard Template Library (STL) benefit C++ development?
- What is RAII (Resource Acquisition Is Initialization) and how does it work?
- Can you discuss the different types of inheritance in C++?
- What is the difference between 'overloading' and 'overriding' methods in C++?
- How do you ensure thread safety in a C++ application?
- What are some common pitfalls when using pointers in C++?
- Can you explain the concept of move semantics in C++ and its benefits?
10 intermediate C++ interview questions and answers to ask mid-tier developers
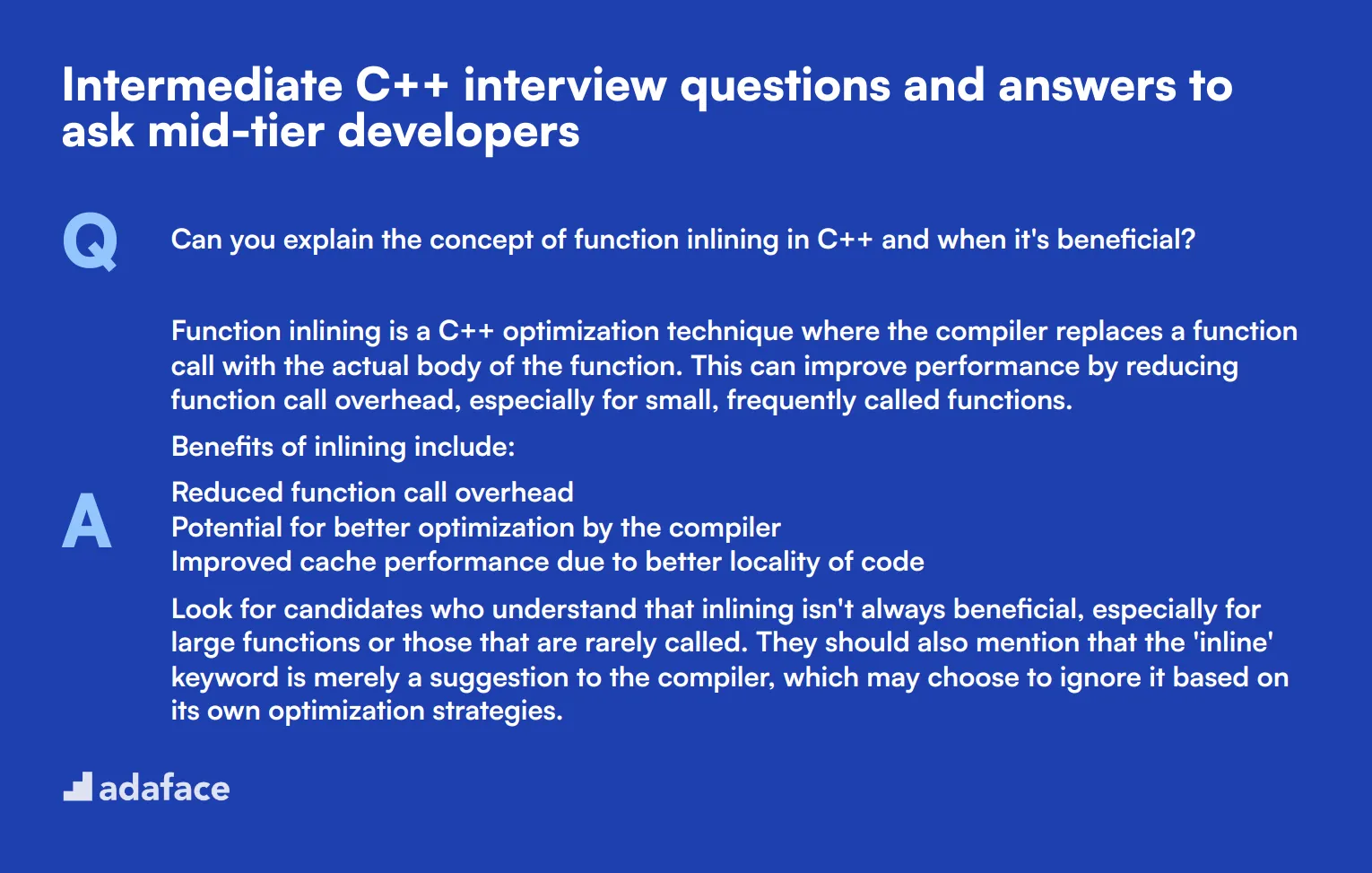
Ready to level up your C++ interviews? These 10 intermediate questions are perfect for assessing mid-tier developers. They'll help you gauge a candidate's depth of knowledge without diving into overly complex territory. Use these questions to spark insightful discussions and uncover the true potential of your C++ candidates.
1. Can you explain the concept of function inlining in C++ and when it's beneficial?
Function inlining is a C++ optimization technique where the compiler replaces a function call with the actual body of the function. This can improve performance by reducing function call overhead, especially for small, frequently called functions.
Benefits of inlining include:
- Reduced function call overhead
- Potential for better optimization by the compiler
- Improved cache performance due to better locality of code
Look for candidates who understand that inlining isn't always beneficial, especially for large functions or those that are rarely called. They should also mention that the 'inline' keyword is merely a suggestion to the compiler, which may choose to ignore it based on its own optimization strategies.
2. How does the 'auto' keyword work in C++11 and later versions?
The 'auto' keyword in C++11 and later versions allows for type inference, where the compiler automatically deduces the type of a variable based on its initializer. This can make code more concise and easier to maintain, especially when dealing with complex types.
For example:
auto x = 5;
// x is deduced to be an intauto str = "Hello";
// str is deduced to be a const char*auto vec = std::vector<int>{1, 2, 3};
// vec is deduced to be a std::vector
Look for candidates who understand that 'auto' doesn't make C++ a dynamically typed language. They should also be aware of potential pitfalls, such as unexpected type deductions with initializer lists or the potential for code to become less readable if overused.
3. What are lambda expressions in C++ and how are they useful?
Lambda expressions in C++ are a way to create anonymous function objects. They allow you to write inline functions that can capture variables from the enclosing scope. Lambdas are particularly useful for writing short, one-off functions that are passed as arguments to algorithms or used in callback scenarios.
A basic lambda syntax looks like this: [capture clause](parameters) -> return_type { function body }
Strong candidates should be able to explain the capture clause (by value, by reference, or both) and discuss use cases such as sorting with custom comparators or implementing callbacks. They might also mention that lambdas can improve code readability by keeping related functionality close together.
4. Can you explain the concept of RAII (Resource Acquisition Is Initialization) in C++?
RAII is a C++ programming technique that ties the life cycle of a resource (like memory, file handles, or network sockets) to the lifetime of an object. The main idea is that when an object is created, it acquires the resources it needs, and when the object is destroyed, it releases those resources.
Key aspects of RAII include:
- Resource acquisition during object construction
- Resource release during object destruction
- Automatic resource management, even in the face of exceptions
Look for candidates who can explain how RAII helps prevent resource leaks and makes code exception-safe. They should be able to give examples, such as using smart pointers for memory management or custom classes for managing file handles. A good candidate might also mention how RAII relates to the broader concept of exception safety in C++.
5. What is the difference between std::vector and std::array in C++?
std::vector and std::array are both container classes in C++, but they have some key differences:
- std::vector has dynamic size, while std::array has a fixed size
- std::vector allocates memory on the heap, while std::array is typically allocated on the stack
- std::vector can grow and shrink at runtime, while std::array's size is determined at compile-time
std::vector is more flexible and is used when the number of elements is not known at compile-time or when you need to add or remove elements. std::array is more efficient for small, fixed-size arrays and provides bounds checking in debug mode.
A strong candidate should be able to discuss the performance implications of each container and scenarios where one might be preferred over the other. They might also mention that std::array can be used as a safer alternative to C-style arrays.
6. How does the 'explicit' keyword affect class constructors in C++?
The 'explicit' keyword, when applied to a constructor, prevents that constructor from being used for implicit type conversions. This helps avoid unexpected and potentially error-prone conversions.
For example, if you have a class 'MyString' with a constructor that takes an integer, marking it as explicit prevents code like MyString str = 42;
from compiling. Instead, you would need to write MyString str(42);
or MyString str = MyString(42);
.
Look for candidates who understand that 'explicit' is a way to make code more predictable and less prone to subtle bugs. They should be able to explain scenarios where implicit conversions might lead to unexpected behavior and how 'explicit' can prevent these issues.
7. Can you explain what perfect forwarding is in C++ and why it's useful?
Perfect forwarding in C++ is a technique that allows a function template to pass its arguments to another function while preserving the value category (lvalue or rvalue) and cv-qualifiers (const or volatile) of the arguments. This is typically achieved using universal references (T&&) and std::forward.
Perfect forwarding is useful in scenarios where you want to write generic code that can efficiently pass arguments to other functions without unnecessary copying or conversion. It's particularly important in the implementation of factories, constructors, and other wrapper functions.
A strong candidate should be able to explain the syntax of perfect forwarding (using T&& and std::forward
8. What is the rule of five in C++ and why is it important?
The rule of five in C++ states that if a class requires a user-defined destructor, copy constructor, or copy assignment operator, it almost certainly requires all five of the following special member functions:
- Destructor
- Copy constructor
- Copy assignment operator
- Move constructor
- Move assignment operator
This rule is important because it ensures that a class correctly manages its resources (like dynamically allocated memory) in all scenarios, including when objects are copied, moved, or destroyed. Implementing these functions correctly prevents resource leaks and ensures proper behavior when objects are transferred or deallocated.
Look for candidates who can explain why each of these functions is necessary and how they relate to each other. They should also be aware that in many cases, the default implementations provided by the compiler may be sufficient, and explicitly defining these functions is only necessary when the class manages resources that require special handling.
9. How does std::unique_ptr differ from std::shared_ptr, and when would you use each?
std::unique_ptr and std::shared_ptr are both smart pointers in C++, but they have different ownership semantics:
- std::unique_ptr represents exclusive ownership of a dynamically allocated object. Only one unique_ptr can own the object at a time.
- std::shared_ptr represents shared ownership. Multiple shared_ptrs can own the same object, and the object is deleted when the last shared_ptr is destroyed.
You would use std::unique_ptr when:
- You need a single, clear owner for a resource
- You want to transfer ownership (e.g., returning from a factory function)
- You're implementing a PIMPL (Pointer to Implementation) idiom
You would use std::shared_ptr when:
- Multiple objects need to share ownership of a resource
- You're dealing with complex object graphs where ownership is not clear-cut
- You need reference-counted resource management
A strong candidate should be able to discuss the performance implications of each (unique_ptr has less overhead) and mention that shared_ptr comes with the cost of atomic reference counting. They might also touch on the concept of weak_ptr as a companion to shared_ptr for breaking circular references.
10. Can you explain what a variadic template is in C++ and provide an example of its use?
A variadic template in C++ is a template that can accept any number of template arguments of any type. It's defined using an ellipsis (...) and allows you to create functions or classes that can work with an arbitrary number of arguments.
A common use case for variadic templates is implementing functions like printf or std::make_shared. For example, a simple variadic function to sum any number of arguments might look like this:
template<typename T>
T sum(T t) {
return t;
}
template<typename T, typename... Args>
T sum(T first, Args... args) {
return first + sum(args...);
}
Look for candidates who can explain the recursion in variadic templates and how parameter packs are expanded. They should also be able to discuss the benefits of variadic templates, such as type-safety and the ability to create more flexible and reusable code. A strong candidate might mention fold expressions (introduced in C++17) as a way to simplify variadic template code.
14 C++ interview questions about data structures
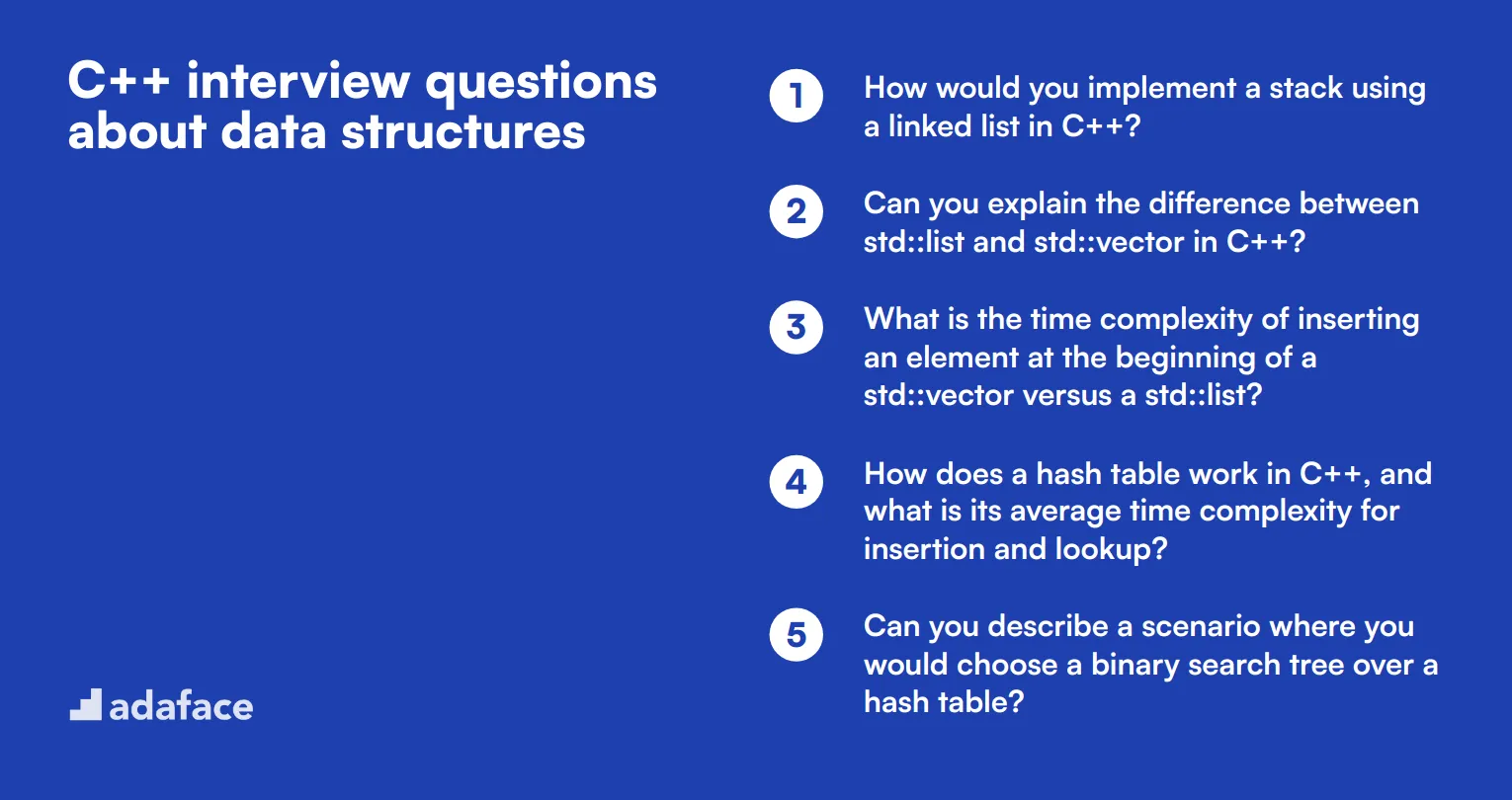
To assess a candidate's proficiency in C++ data structures, use these interview questions. They'll help you gauge the software developer's understanding of fundamental data structures and their implementation in C++. These questions are designed to be straightforward yet revealing, perfect for evaluating both theoretical knowledge and practical skills.
- How would you implement a stack using a linked list in C++?
- Can you explain the difference between std::list and std::vector in C++?
- What is the time complexity of inserting an element at the beginning of a std::vector versus a std::list?
- How does a hash table work in C++, and what is its average time complexity for insertion and lookup?
- Can you describe a scenario where you would choose a binary search tree over a hash table?
- What is a priority queue in C++, and how is it typically implemented?
- How would you implement a circular buffer in C++?
- Can you explain the difference between a min-heap and a max-heap?
- What is the purpose of the std::set container in C++, and how does it maintain its elements?
- How would you implement a trie (prefix tree) data structure in C++?
- Can you describe the internal workings of std::unordered_map in C++?
- What is the difference between std::map and std::unordered_map in terms of performance and use cases?
- How would you implement a graph data structure in C++?
- Can you explain how a B-tree differs from a binary search tree and when you might use it?
9 C++ interview questions and answers related to memory management
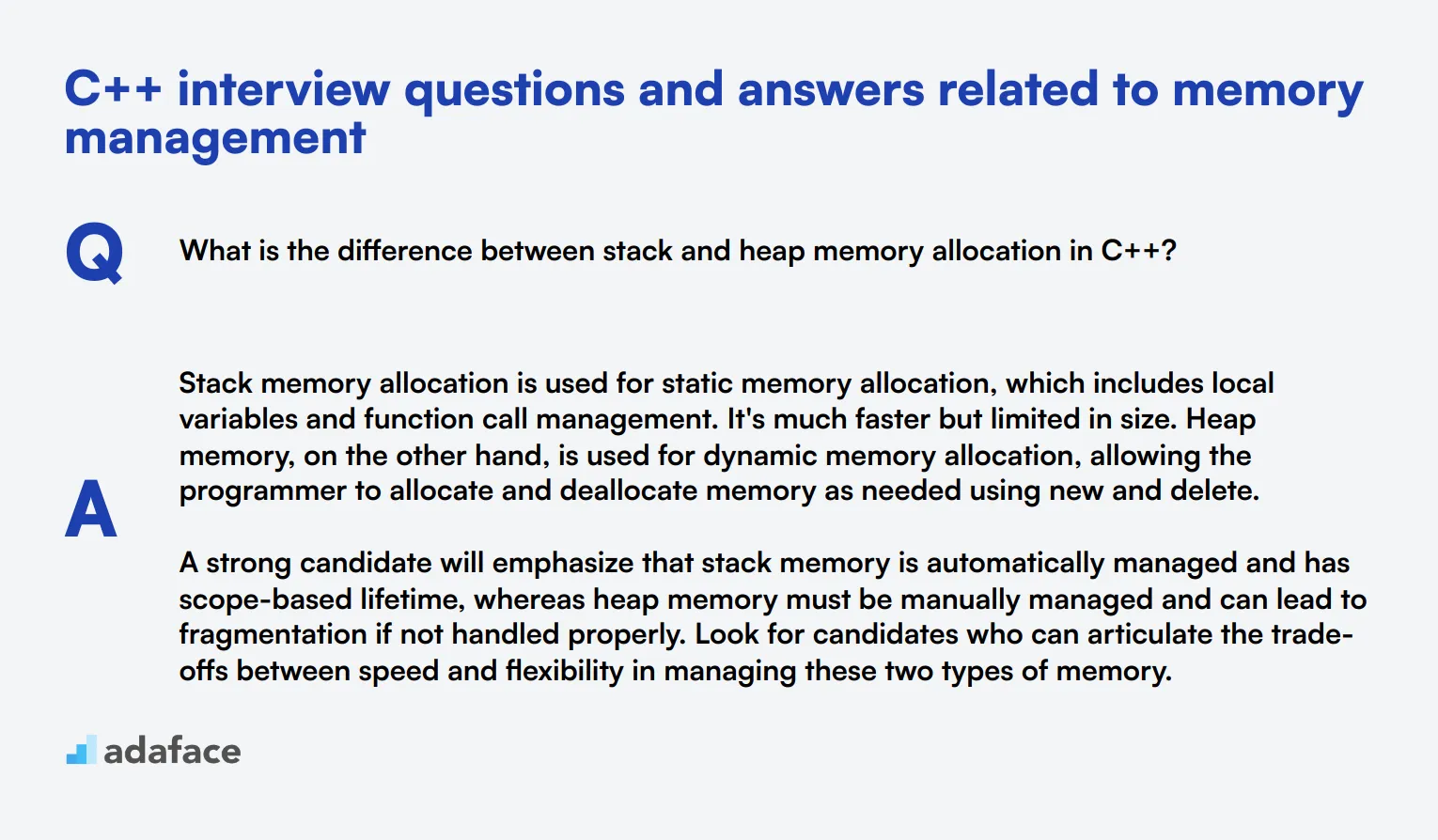
In C++ interviews, understanding how candidates manage memory is crucial because it directly impacts the performance and reliability of software. Use this list of memory management questions to gauge your applicants' proficiency in handling one of the most critical aspects of C++ programming.
1. What is the difference between stack and heap memory allocation in C++?
Stack memory allocation is used for static memory allocation, which includes local variables and function call management. It's much faster but limited in size. Heap memory, on the other hand, is used for dynamic memory allocation, allowing the programmer to allocate and deallocate memory as needed using new and delete.
A strong candidate will emphasize that stack memory is automatically managed and has scope-based lifetime, whereas heap memory must be manually managed and can lead to fragmentation if not handled properly. Look for candidates who can articulate the trade-offs between speed and flexibility in managing these two types of memory.
2. How do you prevent memory leaks in C++?
To prevent memory leaks in C++, it's essential to ensure that every allocation with new has a corresponding deallocation with delete. Using smart pointers like std::unique_ptr and std::shared_ptr can also help manage memory automatically and prevent leaks.
Candidates should also be familiar with tools like Valgrind for detecting memory leaks and other memory-related issues. The ideal response should include examples of good practices, such as avoiding raw pointers and using RAII (Resource Acquisition Is Initialization) principles.
Smart pointers are a key topic to listen for, as their correct use is a sign of modern, effective C++ programming.
3. What is a memory leak, and how can it be detected?
A memory leak occurs when a program allocates memory but fails to deallocate it, leading to wasted memory resources that can eventually exhaust the system's available memory. It can be detected using tools like Valgrind or by employing built-in debugging features in Integrated Development Environments (IDEs).
An ideal candidate will provide a clear definition of a memory leak and mention specific tools or techniques for detecting them. They should also discuss the importance of regular code reviews and automated testing in identifying and fixing memory leaks.
4. Can you explain the concept of memory fragmentation and its impact on application performance?
Memory fragmentation refers to the condition where free memory is divided into small, non-contiguous blocks, making it difficult to allocate large contiguous blocks of memory. This can lead to inefficient memory usage and degrade application performance over time.
Candidates should talk about how memory fragmentation can be minimized through careful allocation and deallocation strategies, as well as the use of memory pools. Look for responses that demonstrate an understanding of the impact of fragmentation on both performance and application stability.
5. What are smart pointers and how do they help in memory management?
Smart pointers are wrappers around raw pointers that manage the lifetime of the object they point to, ensuring that the object is properly deleted when it is no longer needed. They help prevent memory leaks and dangling pointers by automating resource management.
There are different types of smart pointers in C++, such as std::unique_ptr, std::shared_ptr, and std::weak_ptr, each serving different purposes. For example, std::unique_ptr provides exclusive ownership of a resource, whereas std::shared_ptr allows multiple pointers to manage a single resource.
Candidates should articulate how and when to use each type of smart pointer. An ideal response will include practical examples and demonstrate a thorough understanding of how smart pointers contribute to safer and more efficient memory management.
6. What is the purpose of the delete operator in C++?
The delete operator in C++ is used to deallocate memory that was previously allocated by the new operator. This helps in freeing up resources and preventing memory leaks.
There are two forms of delete: delete and delete[]. The former is used to deallocate memory for a single object, while the latter is used for arrays of objects. Proper use of delete is crucial for managing dynamic memory in C++ applications.
Look for candidates who not only understand the purpose of delete but also emphasize the importance of pairing each new with a corresponding delete to avoid memory leaks.
7. Can you explain what a dangling pointer is and how to avoid it?
A dangling pointer is a pointer that points to memory that has already been freed or deallocated. Accessing a dangling pointer can lead to undefined behavior, including crashes and corrupted data.
To avoid dangling pointers, always set pointers to nullptr (or NULL in older C++) after deleting them. Using smart pointers, which automatically manage the deallocation of memory, can also help prevent this issue.
Candidates should highlight the importance of consistent memory management practices and be able to discuss the risks associated with dangling pointers. An ideal answer will include practical tips for avoiding this common pitfall in C++.
8. What is the role of the new operator in C++?
The new operator in C++ is used to allocate memory on the heap for an object or array of objects. It returns a pointer to the allocated memory. Unlike stack memory, heap memory must be manually managed, which means that memory allocated with new must be deallocated with delete.
Using new allows for dynamic memory allocation, giving programs the flexibility to allocate memory as needed during runtime. However, it also requires careful management to prevent memory leaks and fragmentation.
Candidates should understand both the benefits and responsibilities that come with using the new operator. Look for a discussion on best practices and potential pitfalls associated with dynamic memory allocation.
9. How can you minimize memory usage in a C++ program?
Minimizing memory usage in a C++ program involves several strategies, such as using appropriate data types, avoiding unnecessary memory allocations, and reusing memory wherever possible. It's also beneficial to use memory-efficient algorithms and data structures.
Techniques like pool allocation, where memory is allocated in large blocks and then subdivided, can help reduce fragmentation and overhead. Another approach is to use smart pointers to manage memory automatically and reduce the risk of leaks.
An ideal candidate will provide a well-rounded answer that includes both high-level strategies and specific techniques. They should also demonstrate an understanding of the trade-offs involved in different memory management approaches.
8 situational C++ interview questions with answers for hiring top developers
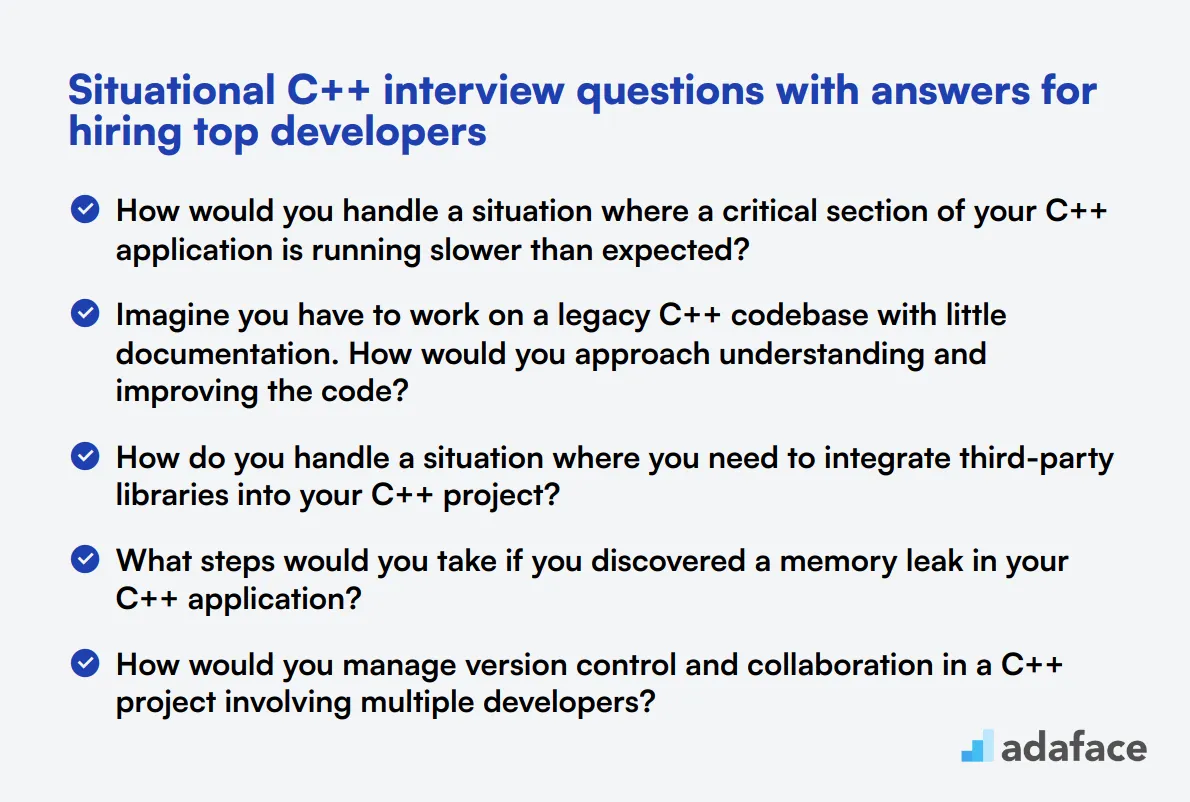
To hire top C++ developers who can handle real-world scenarios, these situational interview questions will help you assess their problem-solving skills and adaptability. Use this list to dig deeper into their thought processes and practical knowledge during the interview.
1. How would you handle a situation where a critical section of your C++ application is running slower than expected?
To address a performance issue in a critical section, I would first profile the application to identify the bottleneck. Tools like gprof or Valgrind can be useful for this. Once I have pinpointed the slow section, I'd analyze the code to understand why it's performing poorly.
Next, I would look into optimizing algorithms or data structures used in that section. For instance, if a loop is consuming too much time, I might consider optimizing it or using a more efficient algorithm. Additionally, I'd check for any unnecessary operations or memory allocations that can be reduced or eliminated.
The ideal candidate should demonstrate a structured approach to problem-solving, including profiling, analyzing, and optimizing code. Look for answers that show practical knowledge and the ability to apply theoretical concepts to real-world problems.
2. Imagine you have to work on a legacy C++ codebase with little documentation. How would you approach understanding and improving the code?
First, I'd start by setting up the development environment and running the existing tests to ensure everything is functioning as expected. If tests are not available, my initial step would be to write basic tests to cover the critical parts of the application.
Next, I would spend time reading through the code to understand its structure and functionality. Tools like Doxygen can help generate documentation from the code, making it easier to navigate. I'd also make small, incremental changes and document my findings to build a better understanding of the codebase.
Candidates who can articulate a systematic approach to understanding and improving legacy code are valuable. Look for those who emphasize testing, incremental changes, and documentation as key steps in their process.
3. How do you handle a situation where you need to integrate third-party libraries into your C++ project?
To integrate third-party libraries, I would first evaluate the library's compatibility with my project, including the required dependencies and license agreements. I would then follow the documentation provided by the library to include it in my build system, ensuring that my project can locate and link to the library files.
After successful integration, I'd write unit tests to verify that the library functions as expected within my application. This helps catch any issues early and ensures that the integration does not introduce new bugs.
An ideal candidate should exhibit a clear understanding of the steps involved in integrating third-party libraries, including compatibility checks, documentation usage, and thorough testing.
4. What steps would you take if you discovered a memory leak in your C++ application?
Upon discovering a memory leak, the first step is to reproduce the issue in a controlled environment. Tools like Valgrind or AddressSanitizer can help detect and diagnose memory leaks by providing detailed reports on memory usage.
Once identified, I would analyze the code to find the root cause of the leak. This often involves checking for mismatched allocations and deallocations, such as forgetting to use delete
after new
. After fixing the leak, I would run the tests again to ensure that the issue is resolved and that no new leaks have been introduced.
Candidates should demonstrate a thorough understanding of memory management in C++ and the tools available for diagnosing memory leaks. Look for a methodical approach to identifying and resolving such issues.
5. How would you manage version control and collaboration in a C++ project involving multiple developers?
Version control is crucial for collaboration. I would use a system like Git to manage the project's codebase. This involves setting up a repository and defining a branching strategy, such as GitFlow, to organize the development workflow.
For collaboration, regular code reviews and continuous integration (CI) pipelines are essential. Code reviews help maintain code quality and facilitate knowledge sharing, while CI ensures that changes are tested and integrated smoothly. Tools like GitHub or GitLab can streamline these processes.
An ideal candidate will highlight the importance of version control and collaboration tools, along with practices that ensure smooth teamwork and high code quality. Look for experience with specific tools and strategies that facilitate effective project management.
6. Can you describe a scenario where you had to debug a particularly tricky issue in a C++ application? How did you resolve it?
In a previous project, I encountered a segmentation fault that would occur intermittently. To resolve this, I first used a debugger like GDB to trace the execution and identify where the fault occurred. By analyzing the stack trace, I was able to pinpoint the location in the code.
I discovered that the issue was due to a dangling pointer—an object was being accessed after it had been deleted. To fix this, I ensured proper ownership and lifecycle management of the object, using smart pointers where appropriate to manage memory automatically.
Candidates should demonstrate strong debugging skills and an ability to systematically diagnose and resolve issues. Look for those who can explain their thought process and the tools they used to identify and fix the problem.
7. How would you ensure the security of a C++ application you're developing?
Ensuring the security of a C++ application involves several best practices. First, I would follow secure coding guidelines to avoid common vulnerabilities like buffer overflows, injection attacks, and race conditions. Regular code reviews and static analysis tools can help identify potential security issues early in the development process.
Additionally, I would implement proper input validation and error handling to prevent unauthorized access and data corruption. Using libraries and frameworks that are regularly updated and maintained can also help mitigate security risks.
An ideal candidate should be aware of common security vulnerabilities and demonstrate a proactive approach to mitigating them. Look for answers that emphasize secure coding practices, regular reviews, and the use of reliable libraries.
8. What strategies would you employ to improve the maintainability of a large C++ codebase?
To improve maintainability, I would focus on writing clear and modular code. This includes using meaningful names for variables and functions, adhering to consistent coding standards, and breaking down complex functions into smaller, reusable components.
Documentation is also crucial. I would ensure that the code is well-commented and accompanied by comprehensive documentation, explaining the design decisions and functionality. Additionally, automated tests can help maintain code quality and facilitate easier refactoring.
Candidates should highlight the importance of clean code, consistent standards, and thorough documentation. Look for those who understand the value of automated tests and modular design in maintaining a large codebase.
Which C++ skills should you evaluate during the interview phase?
Conducting a thorough C++ interview can be challenging as it's not feasible to assess every possible skill in a limited time. However, there are core skills that you should focus on to effectively evaluate a candidate's proficiency with C++.
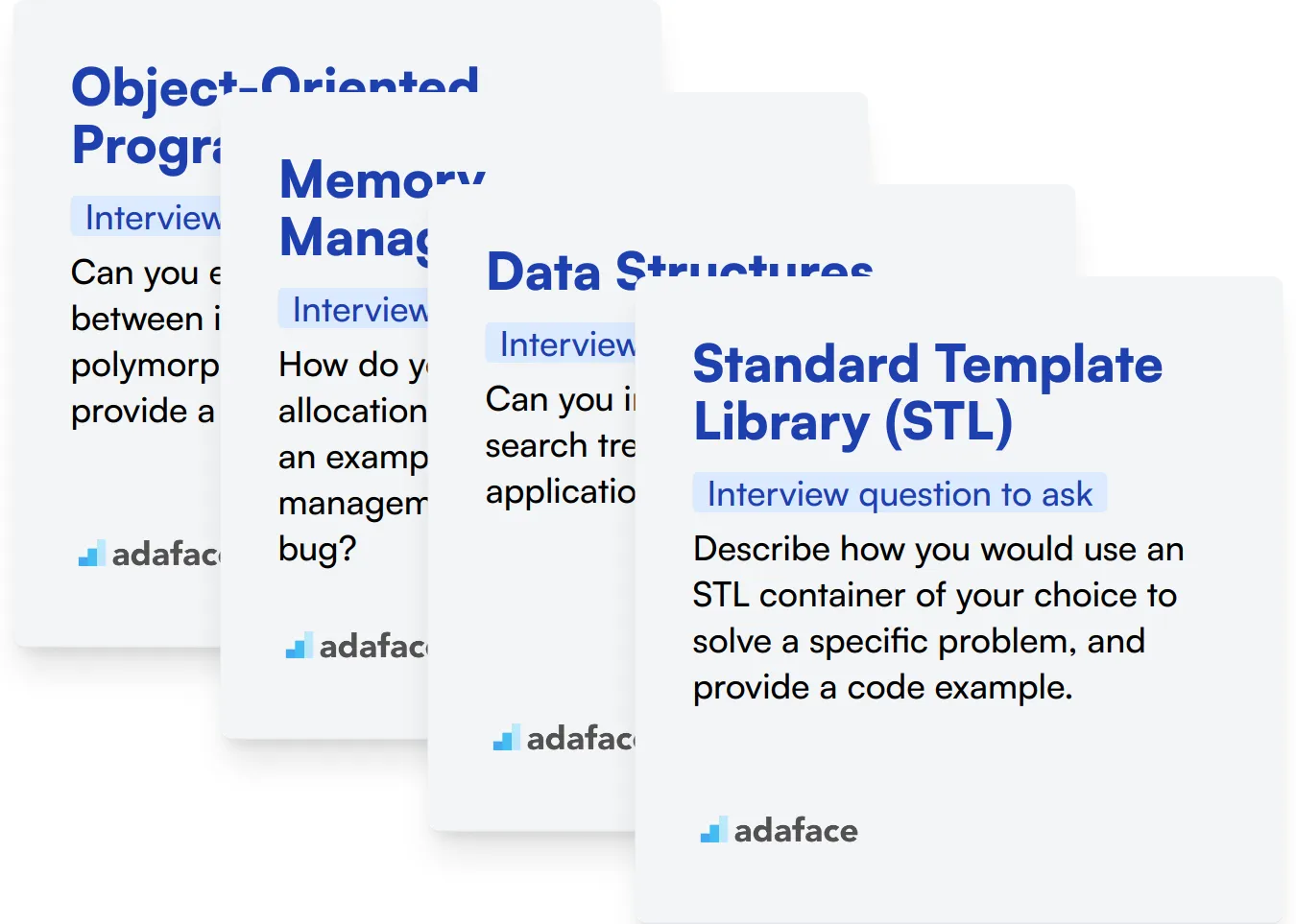
Object-Oriented Programming (OOP)
Understanding OOP is critical for any C++ developer as it forms the backbone of C++ programming. It helps in organizing complex programs into simpler, modular pieces, which are easier to manage and maintain.
You can use an assessment test that includes relevant MCQs to filter out candidates proficient in OOP. Check out the C++ online test for this purpose.
In addition to MCQs, you can ask targeted interview questions to judge the candidate's understanding of OOP concepts.
Can you explain the difference between inheritance and polymorphism in C++ and provide a code example?
When the candidate answers, look for a clear understanding of both concepts and practical examples demonstrating their application in real-world scenarios.
Memory Management
Memory management is a crucial skill for C++ developers as it directly affects the performance and reliability of applications. It includes understanding pointers, dynamic allocation, and deallocation of memory.
You can utilize a skill assessment test with relevant MCQs to gauge the candidate's expertise in memory management. The C++ online test covers such questions.
To further assess this skill, ask specific interview questions related to memory management.
How do you manage memory allocation in C++? Can you give an example where poor memory management could lead to a bug?
Look for answers that demonstrate an understanding of best practices in memory management and awareness of potential pitfalls.
Data Structures
Knowledge of data structures is essential for efficient problem-solving in C++. Common data structures like arrays, linked lists, and trees are frequently used in development.
Consider using an assessment test that includes relevant MCQs to evaluate a candidate's understanding of data structures. The C++ online test is suitable for this.
You can also ask targeted questions during the interview to assess the candidate's familiarity with data structures.
Can you implement a binary search tree in C++ and explain its applications?
Evaluate the candidate's ability to write correct and efficient code, as well as their understanding of the use cases of the data structure.
Standard Template Library (STL)
The Standard Template Library (STL) is a powerful library in C++ that provides reusable components. Familiarity with STL allows developers to write more efficient and maintainable code.
You can filter out candidates skilled in STL by using an assessment test with relevant MCQs. The C++ online test can be beneficial here.
Ask specific interview questions to gauge the candidate's knowledge and practical experience with STL.
Describe how you would use an STL container of your choice to solve a specific problem, and provide a code example.
Seek answers that show a deep understanding of STL containers and their appropriate usage in solving real-world problems.
3 Strategic Tips for Leveraging C++ Interview Questions
Before you start utilizing the C++ interview questions we've discussed, here are three strategic tips to enhance your interviewing process.
1. Incorporate Skills Tests in the Preliminary Stages
Using skills tests prior to interviews can significantly streamline your recruitment process by ensuring only qualified candidates reach the interview stage. This step filters out applicants who may not meet the technical requirements of the position.
For C++ roles, consider integrating tests such as the C++ Online Test to assess basic proficiency, or the Data Structures Online Test for candidates expected to manage complex data within your projects.
These tests not only validate the technical skills of candidates but also save valuable time during interviews by allowing you to focus on deeper aspects of candidate evaluation. Transitioning from a skills test to in-depth interviews enhances the quality of your hiring decisions.
2. Curate Your Interview Questions Carefully
Interview time is limited, making it imperative to ask the right questions that reveal the most about a candidate's capabilities and fit for the role. Choose questions that effectively evaluate the skills crucial to the position.
Explore integrating questions from additional areas such as C# or data structure knowledge, depending on the role's requirements. This approach ensures a holistic assessment of each candidate.
Prioritize questions that delve into real-world problems and scenarios relevant to your projects. This not only assesses technical knowledge but also problem-solving skills and adaptability.
3. Emphasize Follow-Up Questions
Relying solely on pre-set interview questions might not give you the full picture of a candidate's expertise or potential. It's essential to ask follow-up questions that probe deeper into their responses.
For example, if a candidate discusses a project where they used C++, ask them to explain the decision-making process behind choosing certain data structures. This follow-up can reveal their depth of understanding and ability to apply concepts in practical scenarios.
Use C++ interview questions and skills tests to hire talented developers
If you are looking to hire someone with C++ skills, it's important to ensure they possess those skills accurately. The best way to do this is through skill tests like our C++ online test.
Once you use this test, you can shortlist the best applicants and call them for interviews. To get started, consider signing up on our online assessment platform.
C++ Online Test
Download C++ interview questions template in multiple formats
C++ Interview Questions FAQs
This post includes 71 C++ interview questions covering various difficulty levels and topics.
The questions range from basic to advanced, including topics on data structures, memory management, and situational scenarios.
Yes, the post includes questions tailored for junior developers, mid-tier developers, and top developers.
Use them to assess candidates' knowledge, problem-solving skills, and practical experience in C++ programming.
Yes, answers are provided for many of the questions to help interviewers evaluate responses.
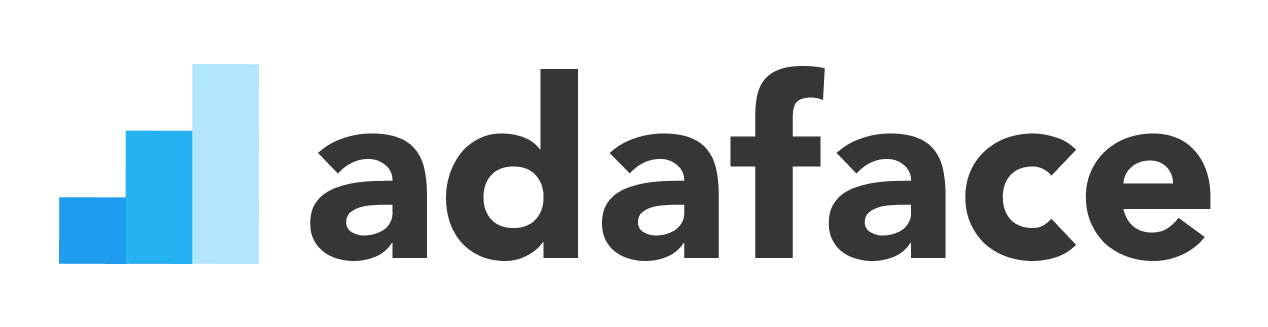
40 min skill tests.
No trick questions.
Accurate shortlisting.
We make it easy for you to find the best candidates in your pipeline with a 40 min skills test.
Try for freeRelated posts
Free resources
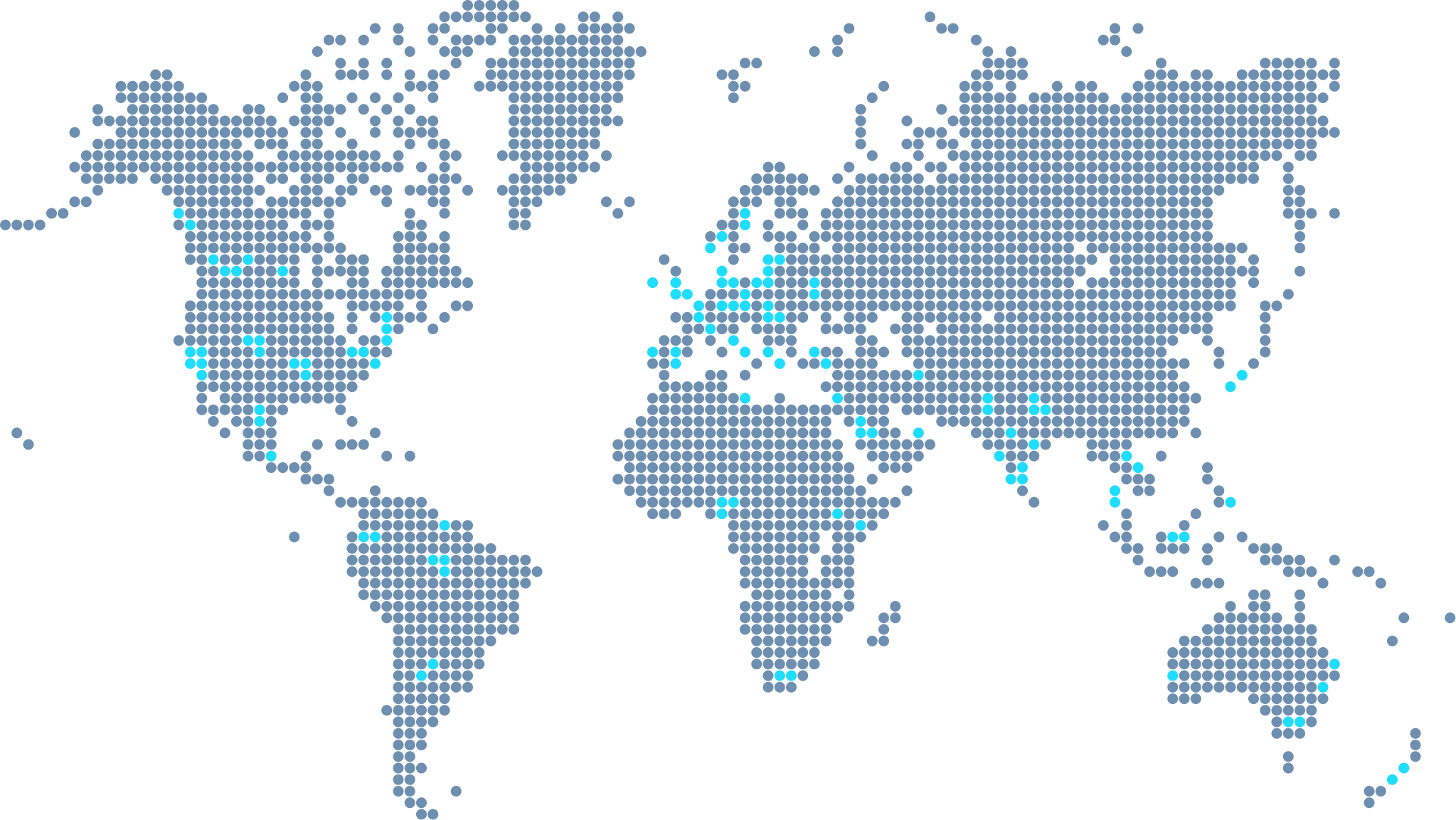
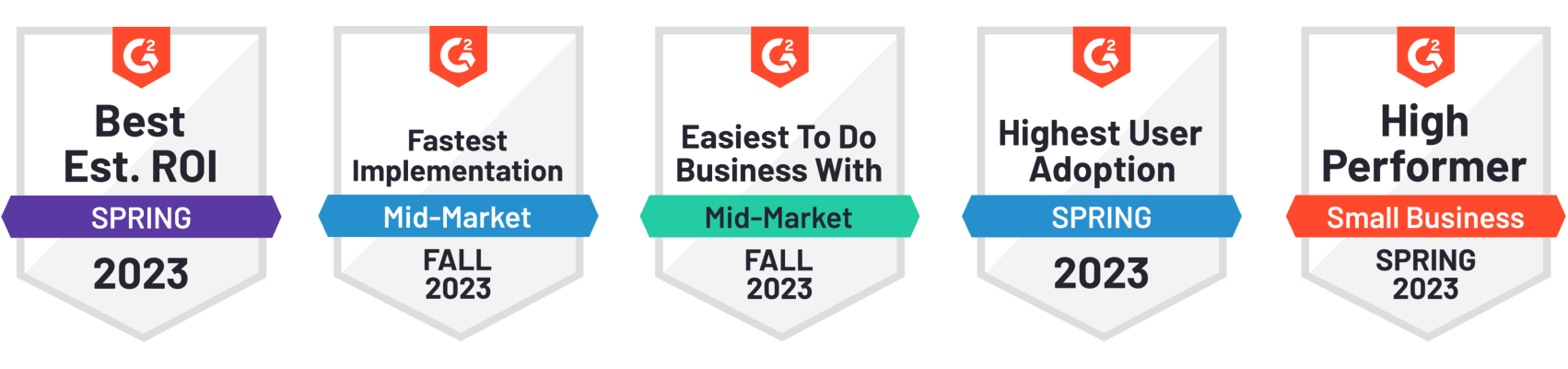