Hiring the right Java developers is crucial for any tech-driven company. Asking the right Core Java interview questions can help you identify top talent and ensure your team has the necessary skills to excel in Java development projects.
This blog post provides a comprehensive list of Core Java interview questions tailored for different experience levels and specific areas of expertise. From common questions for all candidates to advanced topics for senior developers, we cover a wide range of Java concepts and practical scenarios.
By using these questions, you can effectively evaluate candidates' Java knowledge and problem-solving abilities. Additionally, consider using a Java skills assessment before the interview to streamline your hiring process and focus on the most promising candidates.
Table of contents
10 common Core Java interview questions to ask your candidates
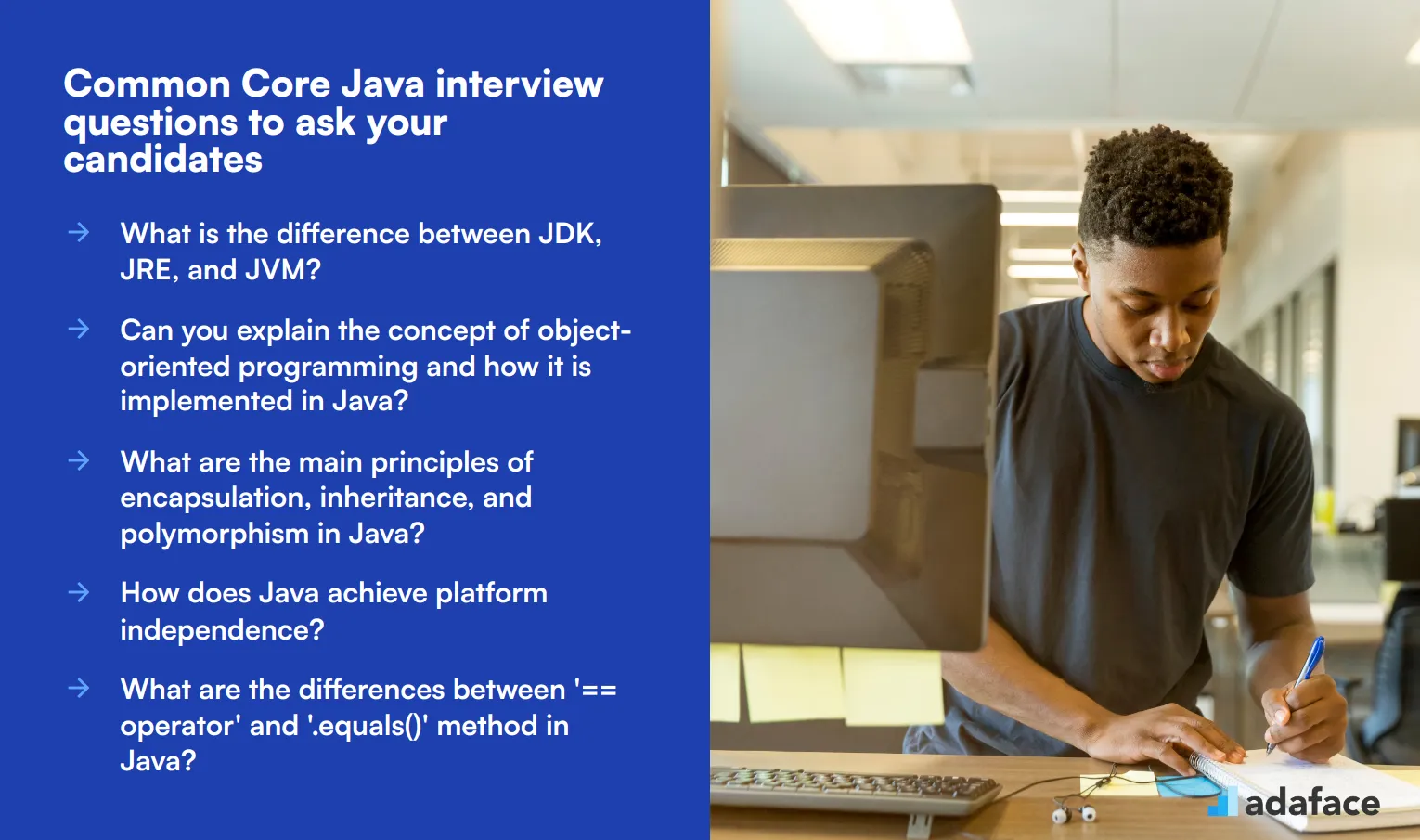
To ensure your candidates have a solid grasp of Core Java, consider using this list of common interview questions. These queries will help you assess their technical knowledge and practical experience, making your hiring process more effective. For more insights on what to look for in a candidate, check out our complete job descriptions.
- What is the difference between JDK, JRE, and JVM?
- Can you explain the concept of object-oriented programming and how it is implemented in Java?
- What are the main principles of encapsulation, inheritance, and polymorphism in Java?
- How does Java achieve platform independence?
- What are the differences between '== operator' and '.equals()' method in Java?
- Can you explain Exception Handling in Java and the difference between checked and unchecked exceptions?
- What is the purpose of the 'final' keyword in Java?
- How do you create a thread in Java? Can you describe the differences between using the Runnable interface and extending the Thread class?
- What are Java Collections, and can you name some commonly used collection classes?
- Can you explain the concept of garbage collection in Java and how it works?
5 Core Java interview questions and answers to evaluate junior developers
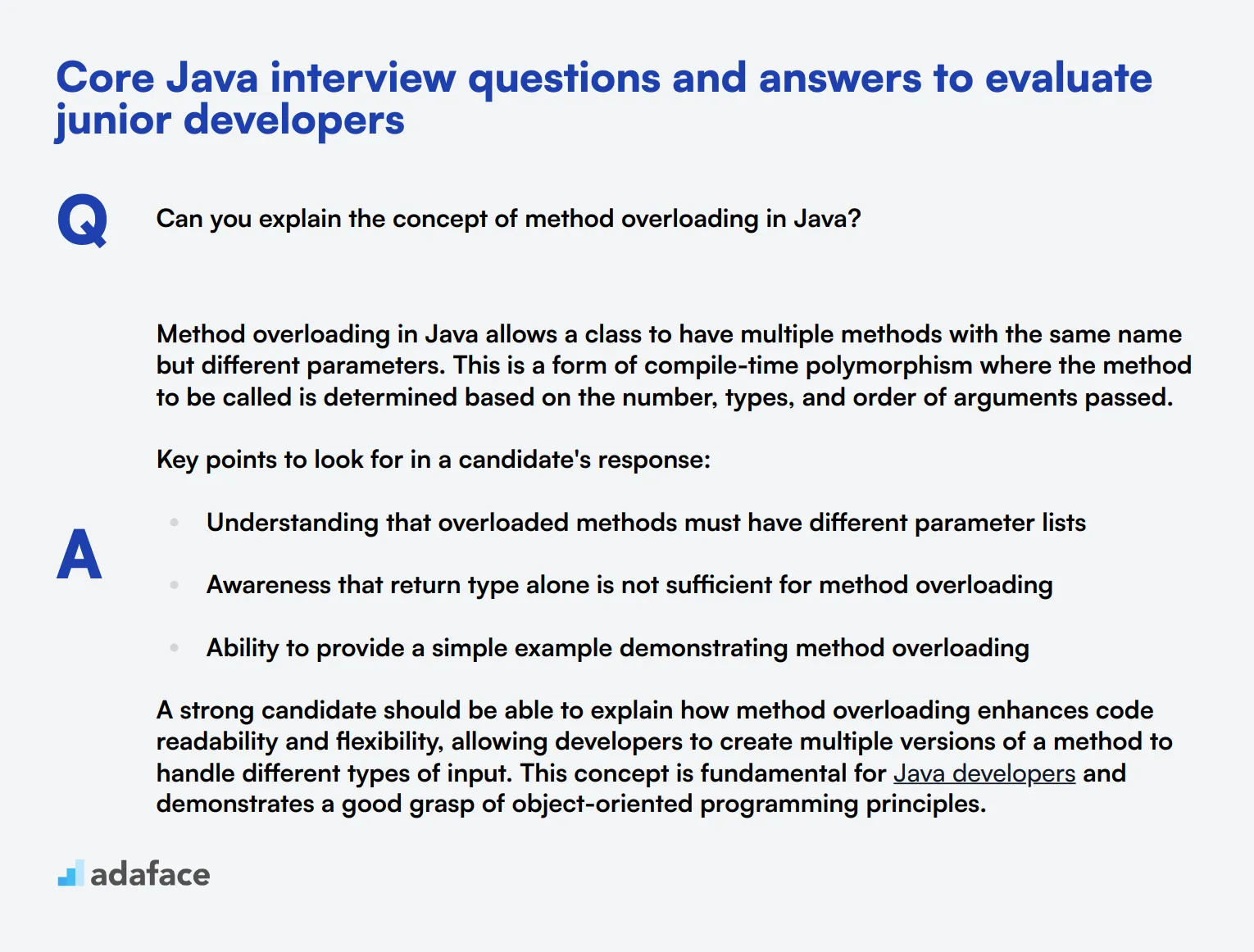
When evaluating junior developers for Core Java positions, it's crucial to ask questions that reveal their fundamental understanding and practical knowledge. This curated list of interview questions will help you assess candidates' grasp of key Java concepts and their ability to apply them in real-world scenarios. Use these questions to gain insights into a candidate's problem-solving skills and coding practices.
1. Can you explain the concept of method overloading in Java?
Method overloading in Java allows a class to have multiple methods with the same name but different parameters. This is a form of compile-time polymorphism where the method to be called is determined based on the number, types, and order of arguments passed.
Key points to look for in a candidate's response:
- Understanding that overloaded methods must have different parameter lists
- Awareness that return type alone is not sufficient for method overloading
- Ability to provide a simple example demonstrating method overloading
A strong candidate should be able to explain how method overloading enhances code readability and flexibility, allowing developers to create multiple versions of a method to handle different types of input. This concept is fundamental for Java developers and demonstrates a good grasp of object-oriented programming principles.
2. What is the difference between an abstract class and an interface in Java?
An abstract class and an interface are both used to achieve abstraction in Java, but they have several key differences:
- Abstract classes can have both abstract and non-abstract methods, while interfaces (prior to Java 8) can only have abstract methods.
- A class can extend only one abstract class, but it can implement multiple interfaces.
- Abstract classes can have instance variables, while interfaces can only have constants (public static final).
- Abstract classes can have constructors, while interfaces cannot.
Look for candidates who can clearly articulate these differences and understand when to use each. A strong response might also include knowledge of Java 8+ features like default and static methods in interfaces, showing awareness of language evolution.
3. How does Java handle memory management, and what is the role of the garbage collector?
Java handles memory management automatically through its built-in garbage collection mechanism. The garbage collector (GC) is responsible for identifying and deleting objects that are no longer being used by the program, freeing up memory for reuse.
Key points a good candidate should mention:
- Java creates objects in the heap memory
- The GC runs periodically to identify unreferenced objects
- Programmers don't need to explicitly deallocate memory
- The GC helps prevent memory leaks and dangling pointer errors
A strong candidate might also discuss different garbage collection algorithms, the concept of finalize() method, and the importance of proper resource management despite having a garbage collector. This understanding is crucial for writing efficient and memory-optimized Java applications.
4. Explain the concept of multithreading in Java and its benefits.
Multithreading in Java refers to the ability of a program to execute multiple threads concurrently. A thread is the smallest unit of execution within a process. Java provides built-in support for creating and managing threads through its java.lang.Thread class and java.lang.Runnable interface.
Benefits of multithreading include:
- Improved performance and responsiveness in applications
- Better utilization of CPU resources, especially on multi-core processors
- Ability to perform multiple tasks simultaneously
- Enhanced user experience in GUI applications
Look for candidates who can explain the basics of thread creation and lifecycle. They should also be aware of potential issues like race conditions and deadlocks. A strong candidate might discuss synchronization mechanisms and the importance of thread safety in concurrent programming.
5. What are Java Annotations and how are they used?
Java Annotations are metadata tags that can be added to Java source code. They provide additional information about the program to the compiler and JVM. Annotations can be applied to classes, methods, variables, parameters, and packages.
Common uses of annotations include:
- Providing instructions to the compiler (@Override, @Deprecated)
- Runtime processing (@Test for JUnit)
- Compile-time processing for code generation
- Framework configuration (e.g., in Spring or Hibernate)
A good candidate should be able to explain the basic syntax of annotations and provide examples of common annotations. Look for understanding of how annotations can simplify code, reduce boilerplate, and enhance maintainability. Advanced candidates might mention creating custom annotations or discuss annotation processors.
15 advanced Core Java interview questions to ask senior developers
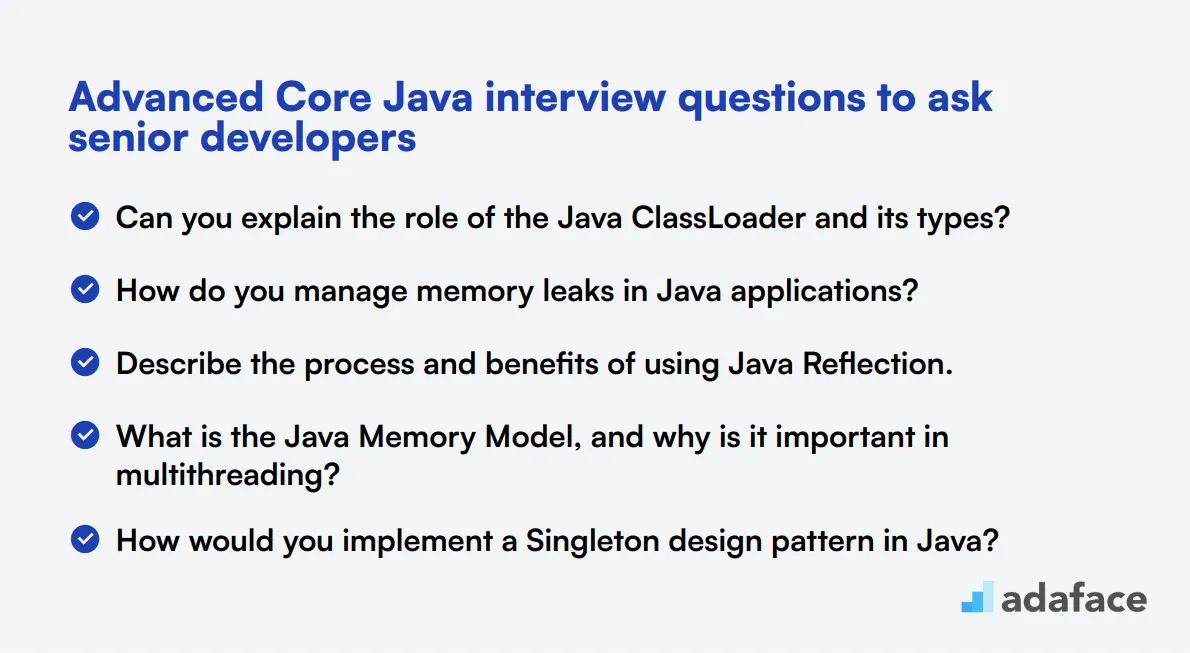
To find out if a candidate has the advanced expertise needed for a senior Java developer role, consider asking them these 15 in-depth Core Java interview questions. These questions are designed to assess their ability to handle complex tasks and their understanding of sophisticated Java concepts. You can reference more about the skills required for a Java developer in our detailed guide.
- Can you explain the role of the Java ClassLoader and its types?
- How do you manage memory leaks in Java applications?
- Describe the process and benefits of using Java Reflection.
- What is the Java Memory Model, and why is it important in multithreading?
- How would you implement a Singleton design pattern in Java?
- Can you explain the difference between ConcurrentHashMap and HashMap?
- What are the principles and benefits of the Stream API in Java 8?
- Can you discuss the internal workings of the Java HashMap?
- Explain the concept of lambda expressions and their uses in Java.
- How does the Fork/Join framework in Java work?
- What are the key differences between Java 8 and previous versions?
- How would you optimize the performance of a Java application?
- What is the role of the ExecutorService framework in Java?
- Can you explain the difference between Future and CompletableFuture in Java?
- Describe how you would handle security concerns in a Java application.
7 Core Java interview questions and answers related to OOP concepts
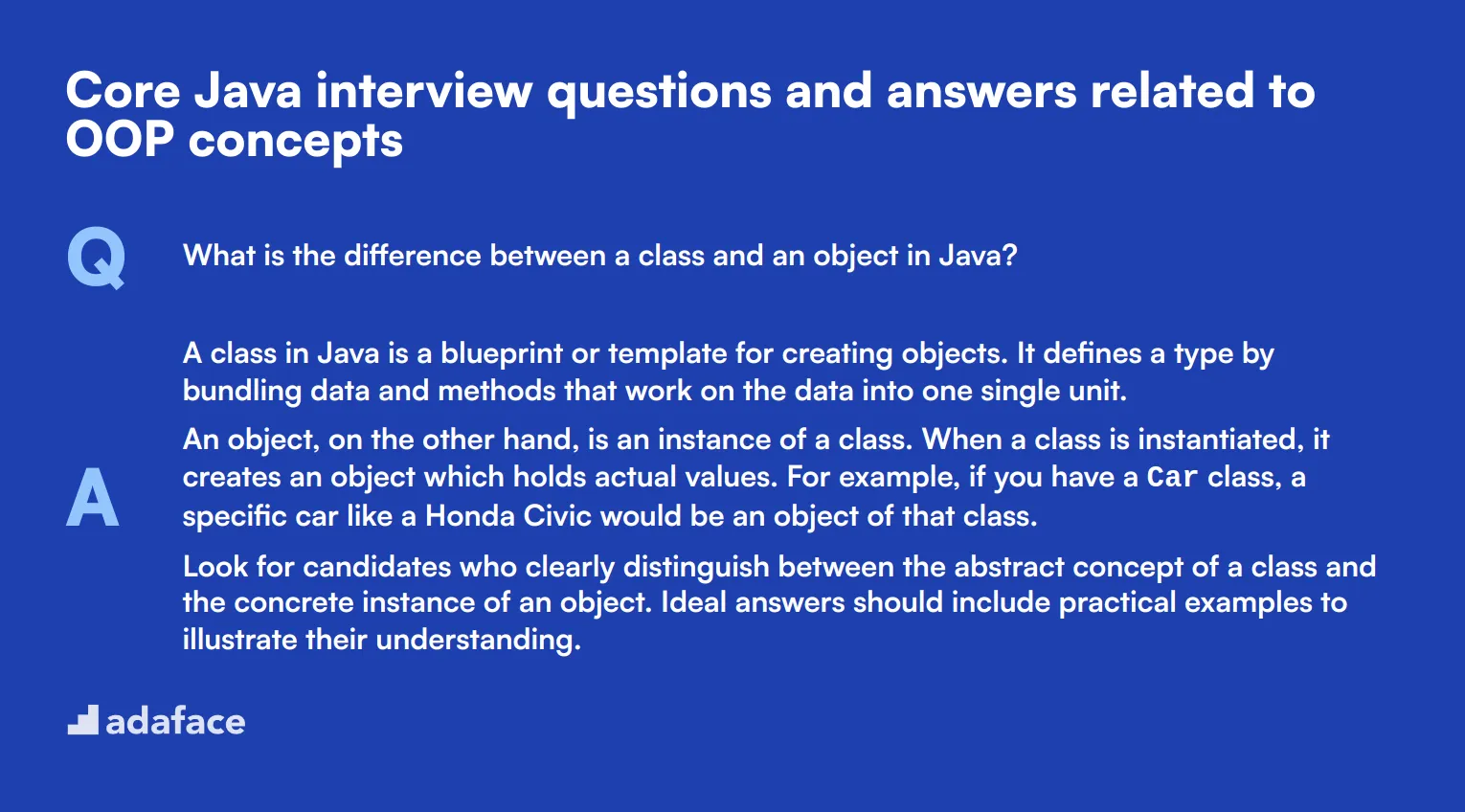
To ensure your candidates grasp the essentials of object-oriented programming in Java, consider these core interview questions. This list will help you evaluate their understanding of key OOP concepts and identify those who can effectively apply these principles in real-world scenarios.
1. What is the difference between a class and an object in Java?
A class in Java is a blueprint or template for creating objects. It defines a type by bundling data and methods that work on the data into one single unit.
An object, on the other hand, is an instance of a class. When a class is instantiated, it creates an object which holds actual values. For example, if you have a Car
class, a specific car like a Honda Civic would be an object of that class.
Look for candidates who clearly distinguish between the abstract concept of a class and the concrete instance of an object. Ideal answers should include practical examples to illustrate their understanding.
2. Can you explain the concept of inheritance in Java?
Inheritance in Java is a mechanism where one class acquires the properties and behaviors (methods) of another class. The class which inherits the properties is called the subclass (or derived class), and the class from which properties are inherited is called the superclass (or base class).
This enables reusability of code and can help in overriding methods to give specific implementation to the subclass. For example, a Vehicle
class might have a method move()
, which is inherited by the Car
and Bike
subclasses but can be overridden to provide specific implementations.
An ideal candidate should explain the benefits of inheritance such as code reusability and method overriding. They should also mention the 'extends' keyword used in Java for inheritance.
3. What is polymorphism in Java and how is it useful?
Polymorphism in Java allows methods to do different things based on the object it is acting upon, even though they share the same name. There are two types of polymorphism: compile-time (method overloading) and runtime (method overriding).
Polymorphism helps in writing more generic and reusable code. For example, you can have a superclass reference variable pointing to a subclass object. Methods in the subclass would be called at runtime, providing specific behavior while maintaining a common interface.
Look for responses that emphasize the advantages of polymorphism such as flexibility and the ability to handle different data types and objects with a uniform interface. Practical examples will help demonstrate the candidate's understanding.
4. What do you understand by encapsulation in Java?
Encapsulation is one of the fundamental principles of OOP. It is the technique of wrapping data (variables) and code (methods) together as a single unit. In Java, this is achieved using classes.
By making the fields in a class private and providing public getter and setter methods to modify and view the values of the fields, you can protect the data from unintended interference and misuse. This also makes the class more maintainable and flexible.
Ideal answers should highlight the benefits of encapsulation such as data hiding, increased security, and improved modularity. Candidates should give examples like using private variables and public methods to control access.
5. What is abstraction in Java and why is it important?
Abstraction in Java is the concept of hiding the complex implementation details and showing only the essential features of the object. It can be achieved using abstract classes and interfaces.
Abstraction is important because it reduces complexity and allows the developer to focus on interactions at a higher level. For instance, a Car
class might expose functionalities like drive()
without revealing the intricate details of how the drive()
method is implemented.
Candidates should explain how abstraction helps in simplifying design and improving code maintainability. Look for examples where abstraction is applied to hide complex logic from the user.
6. How do constructors work in Java?
A constructor in Java is a special method used to initialize objects. It is called when an instance of a class is created. Constructors have the same name as the class and do not have a return type.
There can be two types of constructors: default constructors (with no parameters) and parameterized constructors (with parameters). They are used to set initial values for object attributes or to run any startup procedures.
Evaluate candidates on their understanding of constructors by looking for explanations on constructor overloading and the role of the 'this' keyword. Practical examples of when to use default versus parameterized constructors will also be useful.
7. What is the role of 'this' keyword in Java?
The 'this' keyword in Java is a reference variable that refers to the current object. It is used to avoid confusion between class attributes and parameters with the same name, to invoke current class methods, and to return the current class instance.
For example, if you have a class attribute name
and a parameter name
in a method, this.name
refers to the class attribute while name
refers to the parameter.
Ideal answers should include explanations and examples of how 'this' helps in distinguishing between instance variables and method parameters, and how it can be used to call other constructors in constructor chaining.
10 Core Java questions related to OOP principles
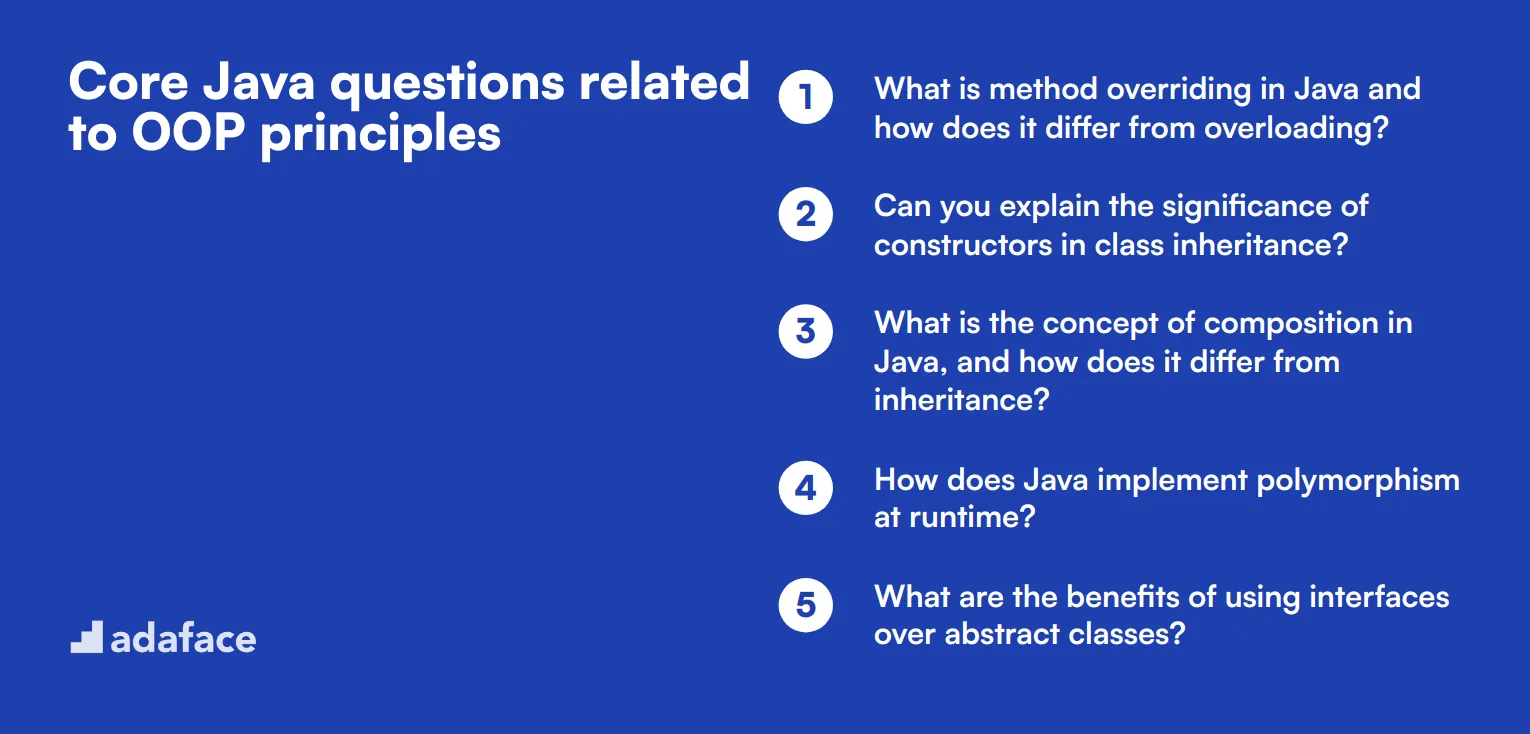
To assess whether candidates possess a solid grasp of object-oriented programming principles in Java, consider asking these targeted questions. This list can help you evaluate their understanding of essential OOP concepts, which are crucial for roles such as a Java Developer.
- What is method overriding in Java and how does it differ from overloading?
- Can you explain the significance of constructors in class inheritance?
- What is the concept of composition in Java, and how does it differ from inheritance?
- How does Java implement polymorphism at runtime?
- What are the benefits of using interfaces over abstract classes?
- Can you explain the concept of the 'super' keyword in Java?
- What is the role of an interface in achieving multiple inheritance in Java?
- How can you achieve abstraction using interfaces in Java?
- Can you discuss the differences between public, protected, and private access modifiers in Java?
- What is the importance of encapsulation and how can it be achieved in Java?
10 situational Core Java interview questions for hiring top developers
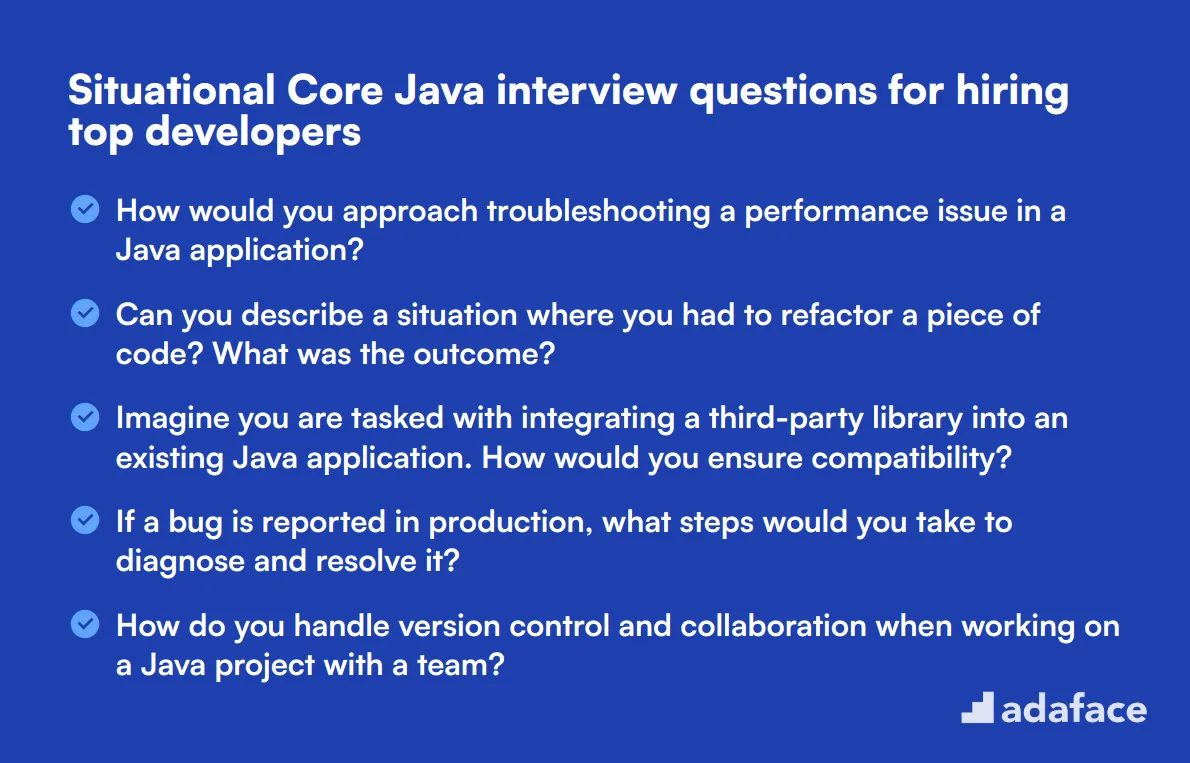
To ensure you hire the best Java developers, use these situational questions to gauge their problem-solving skills and practical knowledge. These questions are designed to reveal how candidates think critically and apply their Java expertise in real-world scenarios, making them ideal for your next interview. For detailed insights into the qualifications you seek, check out our Java Developer Job Description.
- How would you approach troubleshooting a performance issue in a Java application?
- Can you describe a situation where you had to refactor a piece of code? What was the outcome?
- Imagine you are tasked with integrating a third-party library into an existing Java application. How would you ensure compatibility?
- If a bug is reported in production, what steps would you take to diagnose and resolve it?
- How do you handle version control and collaboration when working on a Java project with a team?
- Describe a challenging problem you faced in a Java project and how you resolved it.
- How would you implement logging in a Java application to ensure you can track issues effectively?
- If you had to design a RESTful web service in Java, what considerations would you take into account for security?
- How would you manage dependencies in a Java application to ensure smooth builds and deployments?
- Can you explain how you would approach writing unit tests for a Java method?
Which Core Java skills should you evaluate during the interview phase?
In the realm of Core Java interviews, it is unrealistic to assess every skill and trait of a candidate in a single session. However, focusing on key Core Java skills can provide insight into a candidate's capability and fit within your team. Evaluating these essential skills can help you make informed hiring decisions.
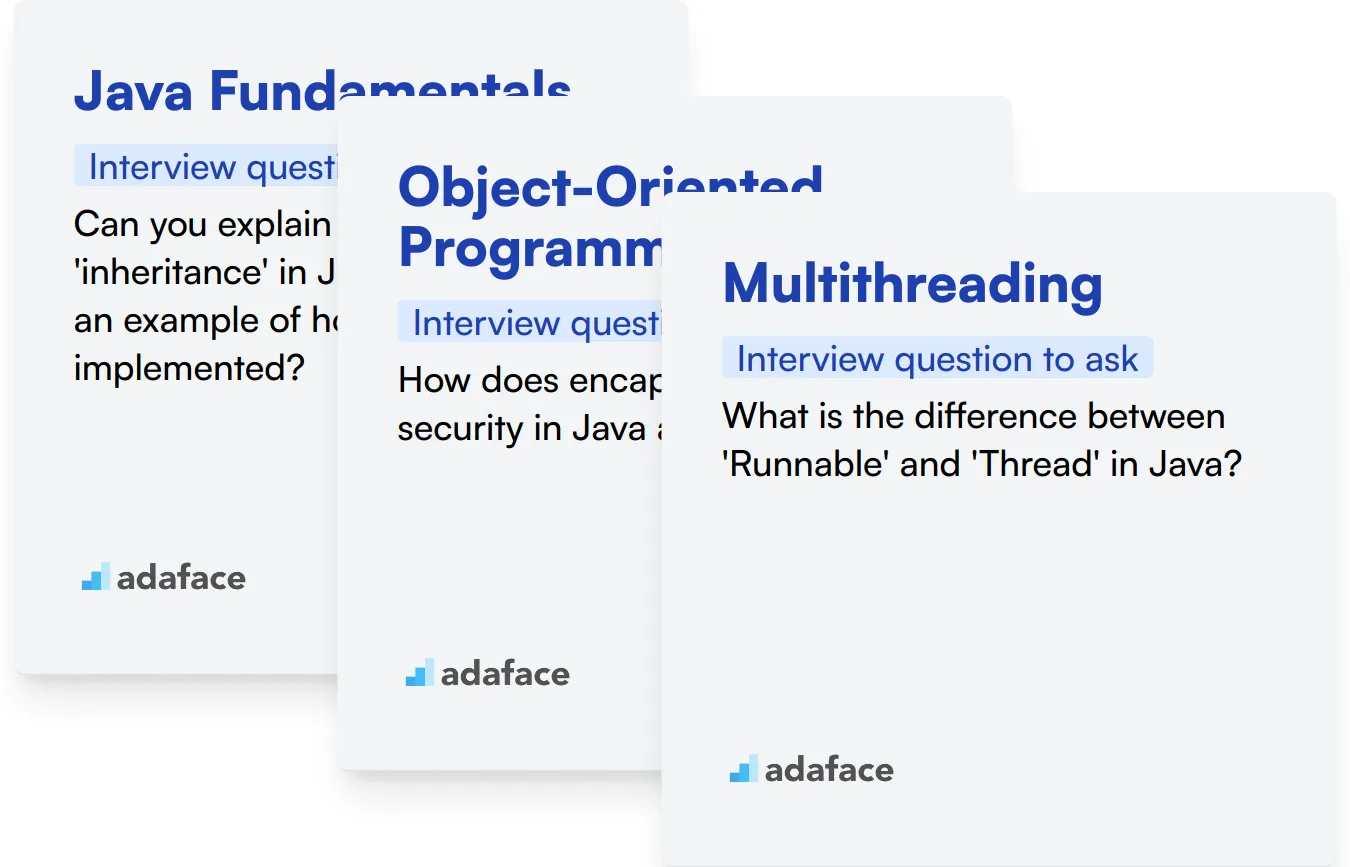
Java Fundamentals
You can use an assessment test that includes relevant MCQs on Java fundamentals to filter out candidates who possess this skill. For example, consider utilizing the Java online test in your evaluation process.
Additionally, you can ask targeted interview questions to assess a candidate's grasp of Java fundamentals. A well-structured question can reveal their depth of understanding.
Can you explain the concept of 'inheritance' in Java and provide an example of how it can be implemented?
When asking this question, look for a candidate's ability to articulate the definition clearly and their understanding of how inheritance promotes code reusability. Pay attention to their example; an effective candidate should be able to implement a basic inheritance structure showcasing parent-child class relationships.
Object-Oriented Programming (OOP)
To filter candidates based on their OOP knowledge, consider administering an assessment test that includes MCQs on these concepts. Use the Object Oriented Programming test from our library for an efficient evaluation.
Another effective approach is to pose targeted interview questions regarding OOP principles. This can help you gauge their practical understanding.
How does encapsulation improve security in Java applications?
When asking this question, observe how well the candidate explains the benefits of encapsulation. A strong response should include details about access modifiers and data hiding, demonstrating an understanding of how these concepts contribute to application security.
Multithreading
You can assess multithreading skills through a tailored assessment test that includes MCQs relevant to this area. Unfortunately, there is currently no specific multithreading test available in our library, so you may need to create custom questions.
Consider asking specific interview questions related to multithreading to further gauge a candidate's expertise.
What is the difference between 'Runnable' and 'Thread' in Java?
Pay attention to the candidate's explanation of the differences between these two approaches. A strong candidate should discuss the advantages and scenarios under which one might be preferred over the other, demonstrating a solid grasp of multithreading concepts.
Leverage Core Java Skills Tests and Interview Questions for Top Hiring Results
When aiming to hire professionals with Core Java skills, it's important to verify these skills accurately before making a hiring decision.
The best tactic to assess these competencies is through targeted skills tests. Consider incorporating tests such as Java Online Test, Java Spring Test, or Java Android Test from Adaface to ensure candidates meet your requirements.
After applying these tests, you can efficiently shortlist the top candidates. This selection streamlines the process, allowing you to focus on interviewing the best of the bunch.
To proceed, I suggest visiting our Signup Page to start using Adaface for your hiring needs. For more details on what we offer, explore our Online Assessment Platform.
Java Online Test
Download Core Java interview questions template in multiple formats
Core Java Interview Questions FAQs
You should ask a mix of common, junior-level, senior-level, OOP-related, and situational questions to assess a candidate's Java skills comprehensively.
The number of questions depends on the interview duration, but aim for a mix of 10-15 questions covering different aspects of Core Java.
A balance of both is ideal. Theoretical questions assess knowledge, while practical ones evaluate problem-solving skills and real-world application.
Ask questions specifically related to OOP concepts and principles in Java, such as inheritance, polymorphism, encapsulation, and abstraction.
Yes, situational questions help assess how candidates apply their Java knowledge to solve real-world problems and handle typical development scenarios.
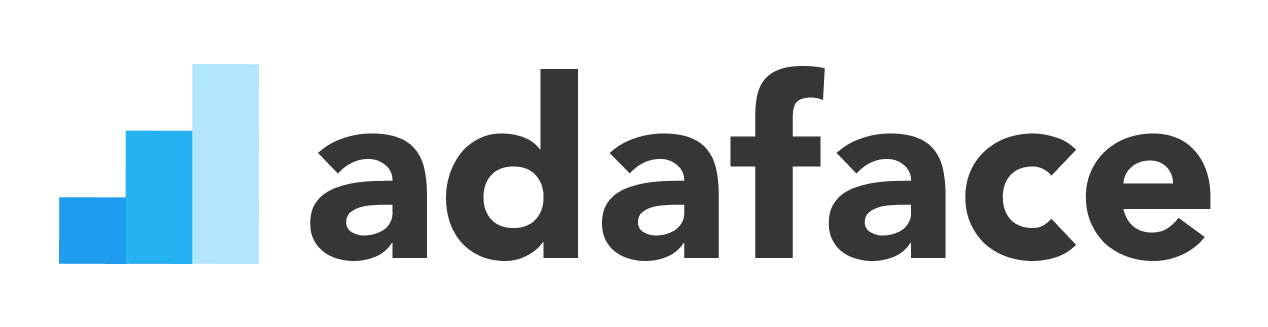
40 min skill tests.
No trick questions.
Accurate shortlisting.
We make it easy for you to find the best candidates in your pipeline with a 40 min skills test.
Try for freeRelated posts
Free resources
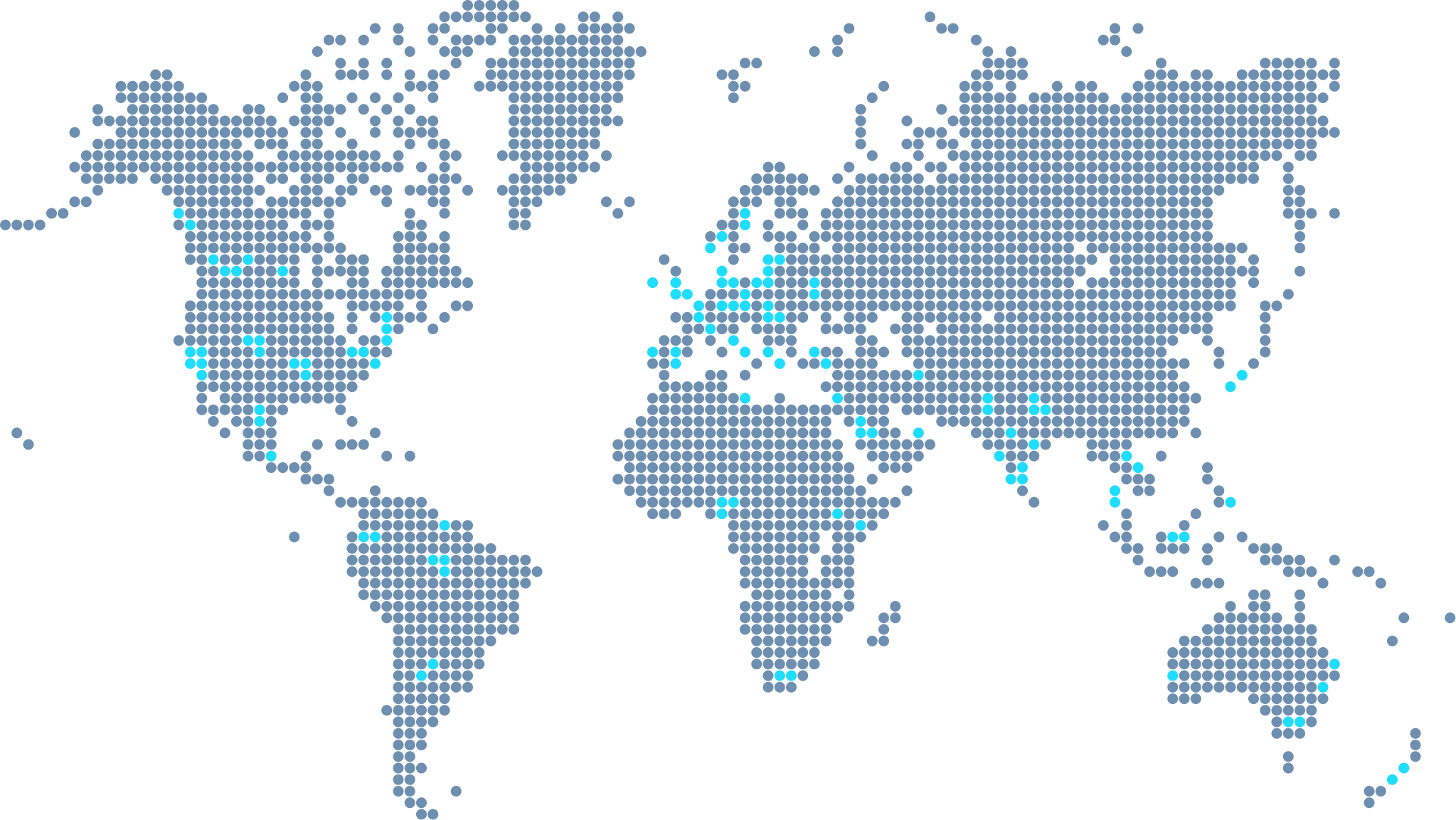
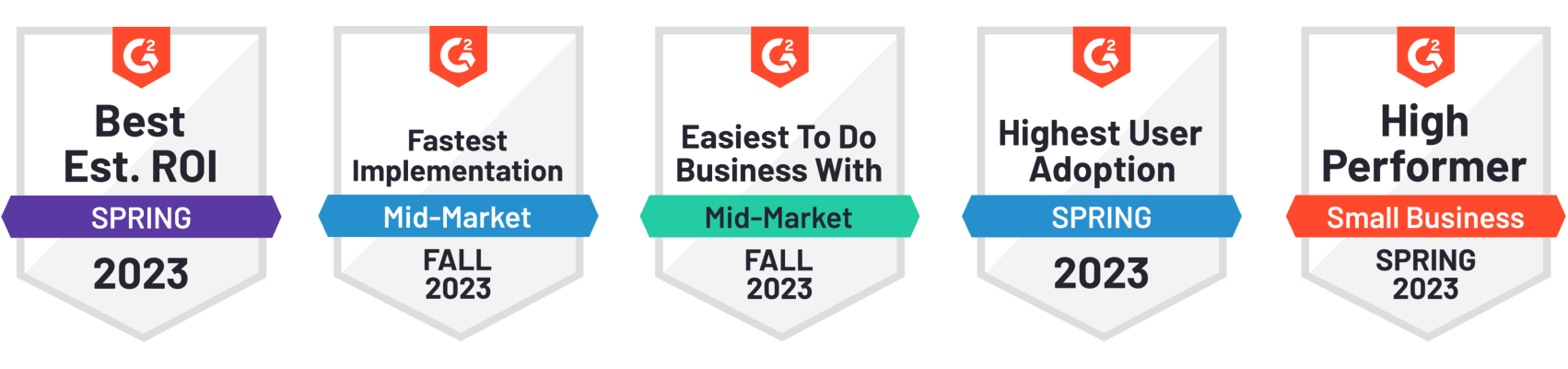