Coding interviews are a critical step in hiring talented developers for your team. Asking the right questions helps you assess a candidate's technical skills and problem-solving abilities effectively.
This blog post provides a comprehensive list of coding interview questions for various experience levels, from junior to senior developers. We cover general coding questions, algorithm and data structure problems, and situational scenarios to help you evaluate candidates thoroughly.
By using these questions, you'll be able to identify top talent and make informed hiring decisions. Consider using a coding assessment before the interview to streamline your selection process and focus on the most promising candidates.
Table of contents
7 general Coding interview questions and answers
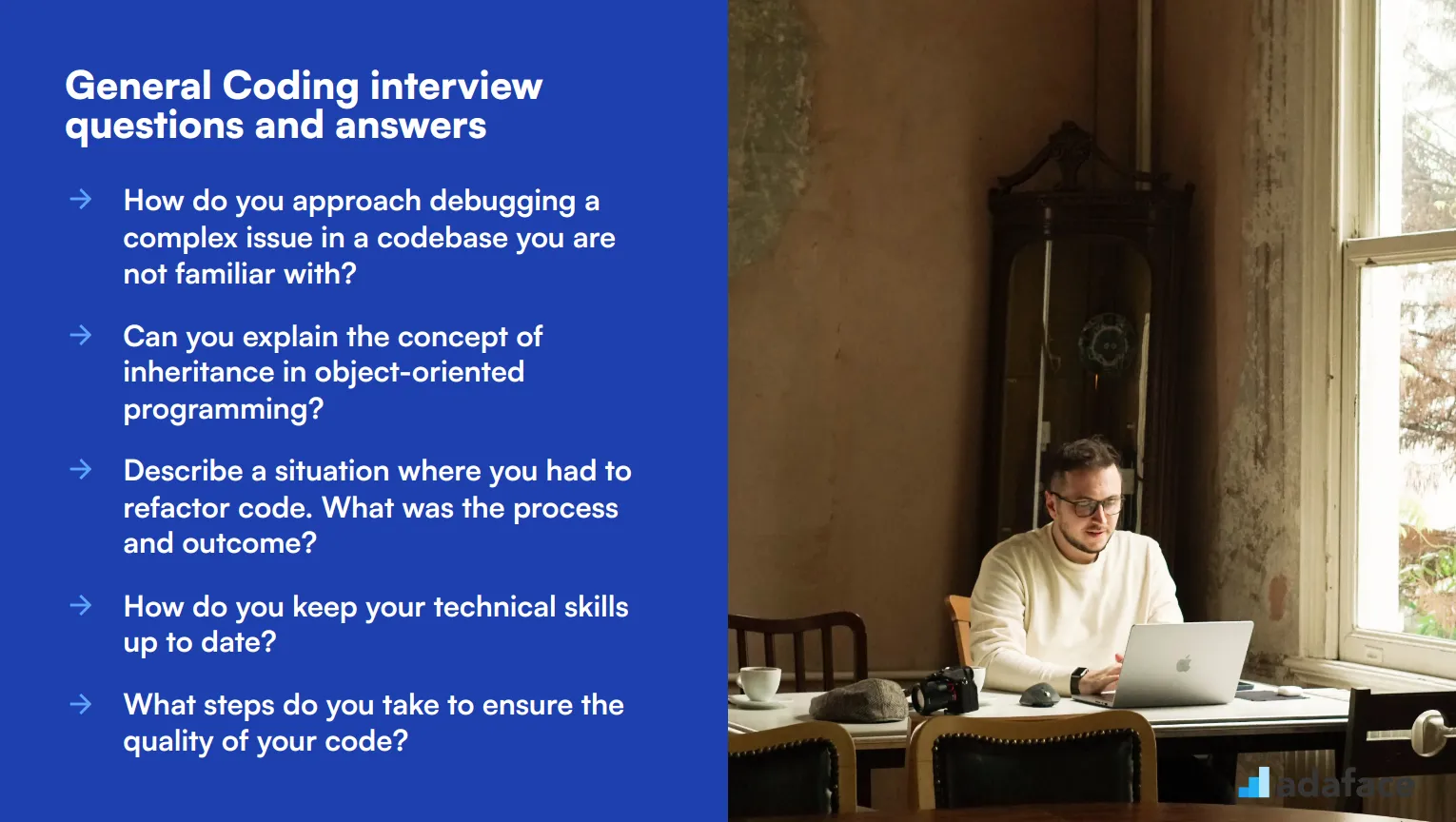
To ensure you hire the best coding talent, utilize these general coding interview questions. They are designed to help you understand the candidate's problem-solving abilities, logical thinking, and grasp of fundamental programming concepts.
1. How do you approach debugging a complex issue in a codebase you are not familiar with?
When faced with debugging a complex issue in an unfamiliar codebase, the first step is to reproduce the issue consistently. This helps understand the exact conditions under which the problem occurs.
Next, I would read through the relevant parts of the code, adding log statements or using a debugger to trace the execution flow and understand where things might be going wrong. I also review any documentation or comments provided within the code to gain context.
An ideal candidate should demonstrate a structured approach to problem-solving and an ability to stay calm under pressure. Look for responses that emphasize methodical investigation, effective use of debugging tools, and clear communication.
2. Can you explain the concept of inheritance in object-oriented programming?
Inheritance is a fundamental concept in object-oriented programming that allows a class to inherit properties and methods from another class. The class that inherits is called the child or subclass, and the class being inherited from is called the parent or superclass.
This mechanism promotes code reusability and establishes a natural hierarchy between classes. For instance, if you have a class Animal
with basic attributes and methods, a Dog
class can inherit from Animal
, gaining its properties while adding unique features specific to dogs.
Candidates should clearly explain the purpose of inheritance and how it helps in creating a well-structured codebase. Strong answers will include practical examples and might link to real-world scenarios.
3. Describe a situation where you had to refactor code. What was the process and outcome?
In a previous project, we encountered a module that had become difficult to maintain due to its complexity. I initiated a refactoring process by first identifying the code smells and understanding the functionality that needed improvement.
I then broke down the refactoring into smaller, manageable tasks, ensuring that I had comprehensive tests in place to verify the changes did not break existing functionality. The refactored code was more modular, readable, and easier to maintain, leading to fewer bugs and faster development cycles.
Look for candidates who emphasize a systematic approach to refactoring, the importance of testing, and the positive outcomes of their efforts. Their answer should reflect a balance between improving code quality and maintaining functionality.
4. How do you keep your technical skills up to date?
To keep my technical skills current, I regularly engage with online learning platforms, attend industry conferences, and participate in coding challenges. These activities help me stay informed about the latest tools, technologies, and best practices.
I also contribute to open-source projects and follow influential developers on social media to gain insights into emerging trends and real-world applications of new technologies.
A strong candidate should demonstrate a proactive approach to continuous learning and professional development. Look for specific examples of how they have applied new skills or knowledge to their work.
5. What steps do you take to ensure the quality of your code?
To ensure code quality, I follow best practices such as writing clean, readable code, adhering to coding standards, and conducting thorough code reviews with peers. Unit testing is a crucial part of my workflow to catch issues early.
I also use static analysis tools to identify potential problems and regularly refactor code to improve its structure and maintainability. Documentation is essential to ensure that others can understand and use the code effectively.
Ideal responses should highlight the importance of maintaining high coding standards, collaborative reviews, and the use of automated tools to enforce code quality. Candidates should provide examples of how these practices have benefited their previous projects.
6. Can you explain the difference between a synchronous and an asynchronous operation?
In a synchronous operation, tasks are performed one after another, with each task waiting for the previous one to complete before proceeding. This can lead to delays if a task takes a long time to finish.
Asynchronous operations allow tasks to be executed concurrently, without waiting for each one to complete before starting the next. This improves efficiency and responsiveness, especially in I/O operations or user interfaces.
Candidates should clearly articulate the differences and provide examples of when each type of operation is appropriate. Look for their understanding of the impact on performance and user experience.
7. How do you handle merge conflicts in a version control system?
When handling merge conflicts, my first step is to understand the changes that have caused the conflict. I review the conflicting changes in both branches to identify the best way to resolve them.
I then carefully merge the changes, ensuring that the final code retains the desired functionality without introducing new issues. After resolving the conflicts, I run tests to verify that everything works as expected before committing the changes.
A strong candidate will demonstrate a methodical approach to resolving conflicts and emphasize the importance of thorough testing. They should also discuss strategies for preventing conflicts, such as frequent integrations and clear communication within the team.
21 Coding interview questions to ask junior developers
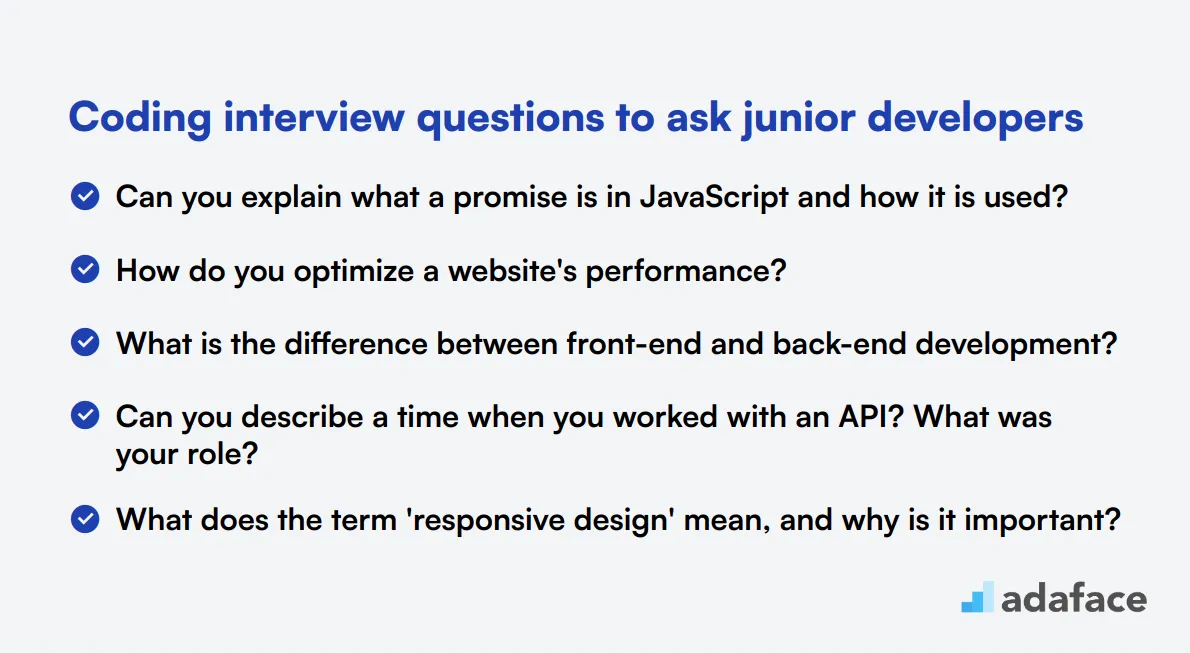
To effectively assess whether junior developers possess the necessary skills for your team, consider utilizing this list of coding interview questions. Tailored for various roles, these questions can help you gauge their technical understanding and problem-solving abilities during the interview process. For specific role requirements, check out our detailed job descriptions.
- Can you explain what a promise is in JavaScript and how it is used?
- How do you optimize a website's performance?
- What is the difference between front-end and back-end development?
- Can you describe a time when you worked with an API? What was your role?
- What does the term 'responsive design' mean, and why is it important?
- How would you handle an unexpected error in your code during a live deployment?
- What is the role of a package manager in your development process?
- Can you explain the concept of RESTful services?
- Describe how you would approach learning a new programming language.
- What tools or techniques do you use for version control?
- How do you prioritize tasks when working on multiple projects?
- What is your understanding of unit testing, and why is it important?
- Can you explain what a code review is and why it matters?
- How would you approach working in a team with varying skill levels?
- What steps would you take to troubleshoot a performance issue in a web application?
- Can you share your experience with any frameworks or libraries you have used?
- What do you understand by the term 'technical debt'?
- How do you ensure your code is understandable by others?
- Can you explain the difference between a class and an object?
- What is your experience with database management systems?
- How would you explain a complex technical concept to someone without a technical background?
10 intermediate Coding interview questions and answers to ask mid-tier developers
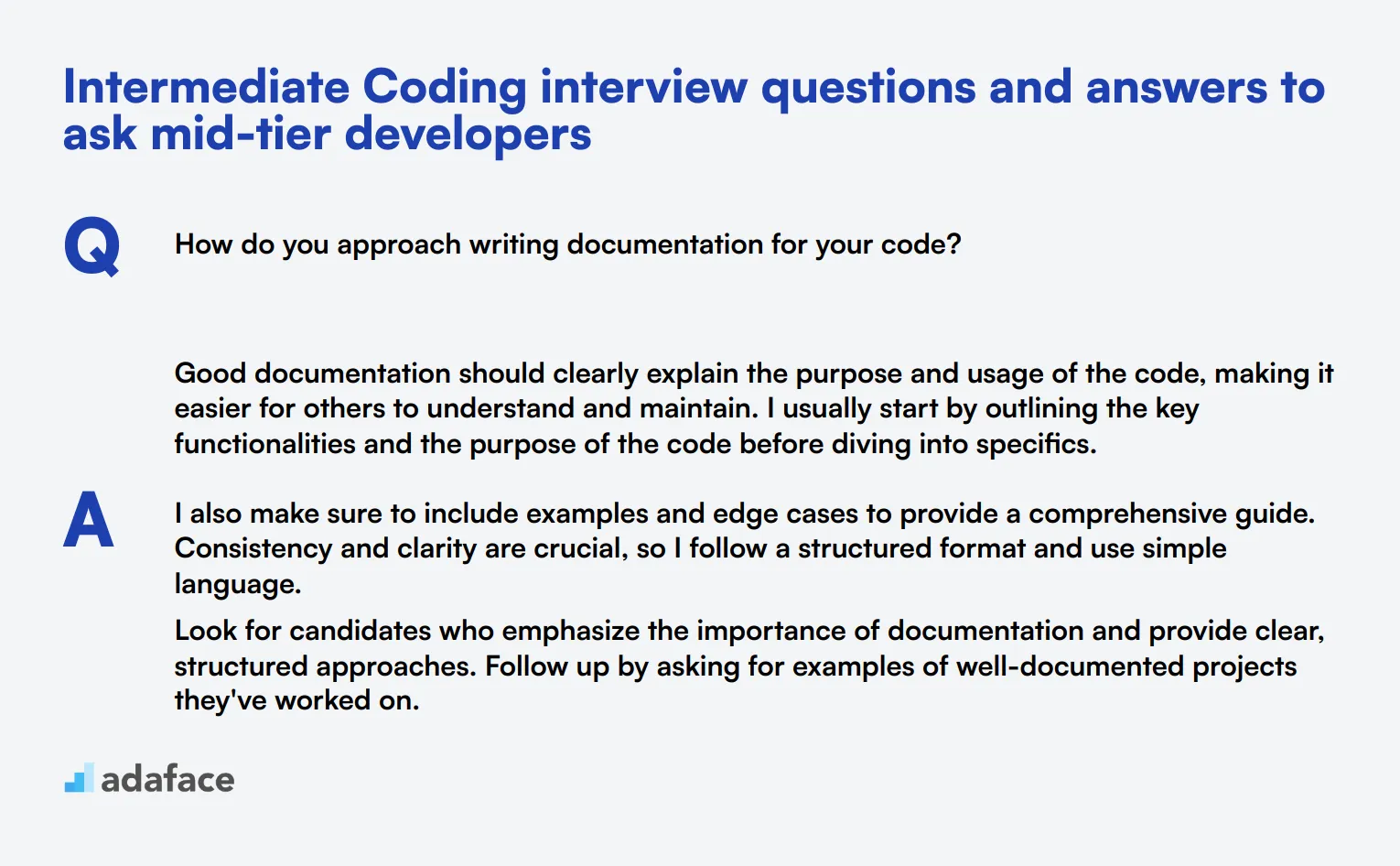
For hiring managers looking to assess the core competencies of mid-tier developers, our list of intermediate coding interview questions is your best bet. These questions are designed to evaluate problem-solving skills, familiarity with software development processes, and the ability to think critically. Utilize these questions to identify candidates who can seamlessly integrate into your development team and tackle complex projects with ease.
1. How do you approach writing documentation for your code?
Good documentation should clearly explain the purpose and usage of the code, making it easier for others to understand and maintain. I usually start by outlining the key functionalities and the purpose of the code before diving into specifics.
I also make sure to include examples and edge cases to provide a comprehensive guide. Consistency and clarity are crucial, so I follow a structured format and use simple language.
Look for candidates who emphasize the importance of documentation and provide clear, structured approaches. Follow up by asking for examples of well-documented projects they've worked on.
2. Can you describe a challenging project you worked on and how you overcame the difficulties?
One challenging project I worked on involved integrating a third-party service with our existing system. The main difficulty was dealing with inconsistent data formats and unreliable API responses.
I tackled this by creating a robust error-handling mechanism and implementing data validation checks. I also maintained open communication with the third-party provider to address issues quickly.
An ideal candidate should demonstrate problem-solving skills and the ability to stay calm under pressure. Look for clear, actionable steps they took to resolve issues and the outcome of their efforts.
3. What is your approach to learning and integrating new technologies into your workflow?
I believe in continuous learning and staying updated with the latest trends. When a new technology catches my interest, I start by reading official documentation and going through tutorials.
Next, I try to implement a small project or feature using the new technology to get hands-on experience. This helps me understand its strengths and limitations.
Recruiters should look for candidates who are proactive in learning and can provide examples of new technologies they have successfully integrated into their workflow. Follow up on how they evaluate whether a new technology is worth adopting.
4. How do you handle tight deadlines while maintaining code quality?
Maintaining code quality under tight deadlines requires effective time management and prioritization. I start by breaking down the project into smaller tasks and identifying the most critical components.
I also make use of code reviews and automated testing to catch potential issues early. Communication with the team is key to ensure everyone is on the same page and working efficiently.
Look for candidates who can balance speed and quality. They should provide examples of past projects where they successfully met tight deadlines without compromising on code quality.
5. Can you explain the concept of continuous integration and its benefits?
Continuous Integration (CI) is a development practice where developers integrate code into a shared repository frequently. Each integration is verified by an automated build and tests, allowing teams to detect issues early.
The benefits of CI include faster detection of defects, improved software quality, and quicker feedback loops. It also helps in maintaining a consistent coding standard across the team.
An ideal answer will show a clear understanding of CI and its practical benefits. Follow up by asking about the candidate's experience with CI tools and how they have implemented CI in past projects.
6. How do you approach optimizing the performance of an application?
Optimizing application performance starts with identifying bottlenecks. I use profiling tools to analyze the performance and pinpoint areas that need improvement, such as slow database queries or inefficient algorithms.
Once identified, I prioritize optimizations based on their impact and feasibility. This may involve code refactoring, database indexing, or implementing caching mechanisms.
Candidates should demonstrate a methodical approach to optimization. Look for their ability to use profiling tools and their understanding of different optimization techniques.
7. What strategies do you use to ensure your code is modular and reusable?
To ensure code modularity and reusability, I follow the principles of SOLID and DRY (Don't Repeat Yourself). I break down complex functionalities into smaller, self-contained modules that can be easily tested and reused.
I also use design patterns and refactor code to eliminate redundancy. Writing unit tests for each module ensures that they work as expected independently.
Look for candidates who can articulate their approach to writing modular and reusable code. Follow up by asking for examples of how they have implemented these principles in past projects.
8. How do you handle and prioritize bug fixes in a project?
Handling and prioritizing bug fixes involves assessing the severity and impact of each bug. Critical bugs that affect the functionality of the application or user experience are addressed first.
I maintain a bug tracker to document and prioritize issues, and I communicate with the team to ensure everyone is aware of the current priorities. Regular updates and testing are crucial to ensure fixes do not introduce new issues.
An ideal candidate should demonstrate a structured approach to bug fixing and effective communication skills. Follow up by asking about their experience with specific bug tracking tools.
9. Can you describe your experience with agile methodologies?
Agile methodologies focus on iterative development, collaboration, and customer feedback. I have experience working in agile teams where we used Scrum or Kanban to manage our workflows.
In these teams, we held regular stand-ups, sprint planning, and retrospectives to ensure continuous improvement. Agile practices helped us adapt to changing requirements and deliver value consistently.
Look for candidates who understand the core principles of agile methodologies and can provide specific examples of how they have applied these practices in their projects.
10. How do you ensure effective communication within your development team?
Effective communication within a development team involves regular meetings, clear documentation, and the use of collaboration tools. I encourage open communication and feedback to ensure everyone is on the same page.
We use tools like Slack for instant messaging, Jira for task tracking, and Confluence for documentation. Regular code reviews and pair programming sessions also help in maintaining transparency and knowledge sharing.
Candidates should highlight their experience with communication tools and practices. Look for examples of how they have fostered a collaborative environment in their previous teams.
15 advanced Coding interview questions to ask senior developers
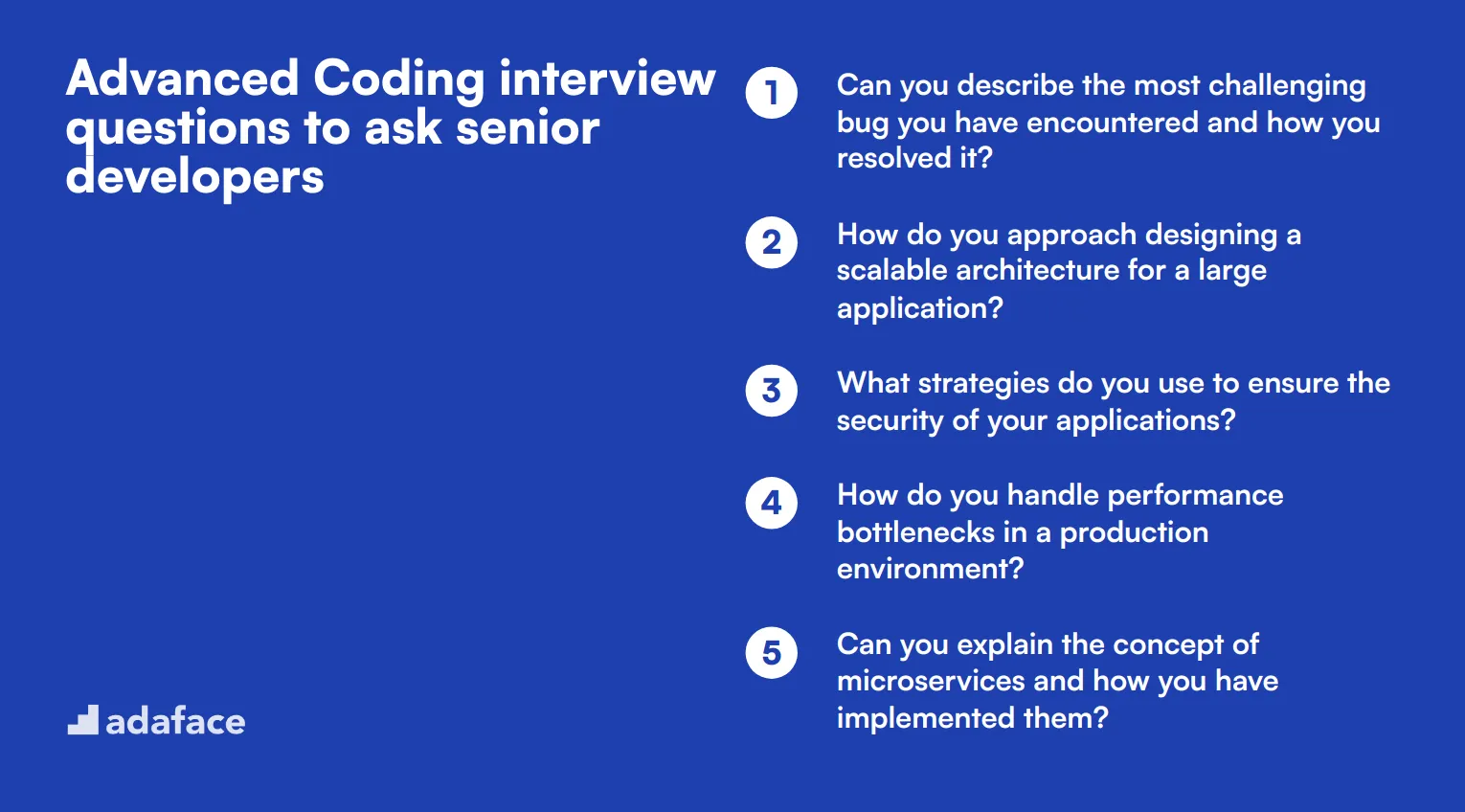
To effectively evaluate the expertise of senior developers, using a targeted list of advanced coding interview questions is crucial. These questions help identify candidates who possess the deep technical knowledge and problem-solving skills necessary for senior roles. For additional insights on what to look for in a developer, you might want to check out this JavaScript developer job description.
- Can you describe the most challenging bug you have encountered and how you resolved it?
- How do you approach designing a scalable architecture for a large application?
- What strategies do you use to ensure the security of your applications?
- How do you handle performance bottlenecks in a production environment?
- Can you explain the concept of microservices and how you have implemented them?
- How do you manage database migrations in a continuous deployment pipeline?
- What is your experience with containerization technologies like Docker and Kubernetes?
- How would you architect a system to handle real-time data streaming?
- Can you describe a time when you had to balance technical debt with the need for new features?
- How do you ensure your application can handle high availability and disaster recovery?
- What practices do you follow for code reviews and ensuring code quality in your team?
- Can you explain the principles of SOLID and how they guide your software design?
- How do you approach optimizing SQL queries and database performance?
- What experience do you have with cloud platforms, and how have you utilized them in your projects?
- How do you mentor junior developers and encourage their growth within a team?
9 Coding interview questions and answers related to algorithms
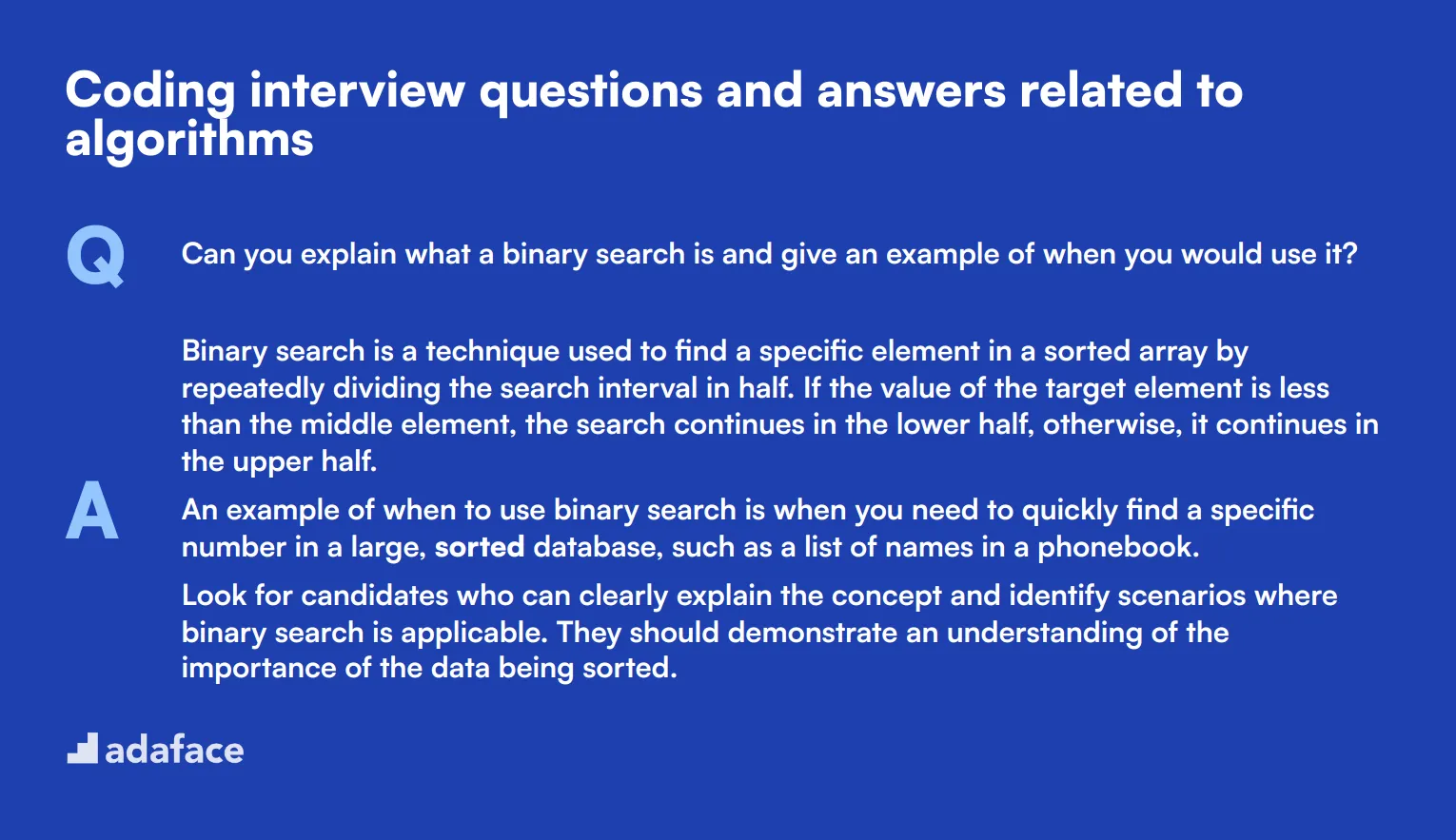
To determine whether your candidates have a solid grasp of algorithmic principles and problem-solving skills, ask them some of these 9 coding interview questions. These questions will help you gauge their ability to think logically and apply algorithmic concepts effectively during real-world scenarios.
1. Can you explain what a binary search is and give an example of when you would use it?
Binary search is a technique used to find a specific element in a sorted array by repeatedly dividing the search interval in half. If the value of the target element is less than the middle element, the search continues in the lower half, otherwise, it continues in the upper half.
An example of when to use binary search is when you need to quickly find a specific number in a large, sorted database, such as a list of names in a phonebook.
Look for candidates who can clearly explain the concept and identify scenarios where binary search is applicable. They should demonstrate an understanding of the importance of the data being sorted.
2. What is a hash table, and how does it work?
A hash table is a data structure that stores items in an associative manner. It uses a hash function to compute an index into an array of buckets or slots from which the desired value can be found.
Hash tables are particularly efficient for lookups, insertions, and deletions, with average time complexities of O(1). They are used in various applications, such as implementing associative arrays and database indexing.
A strong candidate should be able to explain how hash tables handle collisions and why they're useful for efficient data retrieval.
3. How would you approach finding the shortest path in a graph?
To find the shortest path in a graph, one common approach is to use Dijkstra's algorithm. This algorithm works by maintaining a set of visited nodes and updating the shortest known distance to each node from the start node.
Another method is the A* algorithm, which uses heuristics to guide the search for the shortest path more efficiently.
Ideal candidates should demonstrate knowledge of these algorithms and discuss when each might be used. They should also mention the importance of understanding the graph's properties, such as whether it has weighted edges or negative weights.
4. Can you explain what dynamic programming is and provide a scenario where it would be beneficial?
Dynamic programming is a method for solving complex problems by breaking them down into simpler subproblems, solving each subproblem just once, and storing their solutions.
A scenario where dynamic programming is beneficial is in solving the problem of finding the longest increasing subsequence in an array.
Candidates should be able to explain the trade-offs of using dynamic programming, such as the space-time complexity, and provide clear examples of problems that it can efficiently solve.
5. What is recursion, and can you give an example of a problem that can be solved using it?
Recursion is a technique where a function calls itself in order to solve smaller instances of the same problem. It is often used to solve problems that can be broken down into simpler, repetitive subproblems.
An example of a problem that can be solved using recursion is calculating the factorial of a number, where the factorial function calls itself with decremented values until it reaches the base case.
Look for candidates who can clearly explain recursion and identify its base cases and recursive calls. They should understand the potential pitfalls, such as stack overflow, and how to mitigate them.
6. How would you describe a linked list, and what are its advantages over arrays?
A linked list is a linear data structure where each element, called a node, contains a data part and a reference (or link) to the next node in the sequence.
The advantages of linked lists over arrays include dynamic size and ease of insertion/deletion operations, as these operations do not require shifting elements as in arrays.
Candidates should be able to explain the trade-offs, such as memory overhead due to additional pointers and the increased complexity of accessing elements.
7. What is a stack, and how is it different from a queue?
A stack is a data structure that follows the Last In, First Out (LIFO) principle, where the last element added is the first one to be removed. Common operations are push (add) and pop (remove).
A queue, on the other hand, follows the First In, First Out (FIFO) principle, where the first element added is the first one to be removed. Common operations are enqueue (add) and dequeue (remove).
Ideal candidates should demonstrate an understanding of these principles and provide practical examples of when to use each structure, such as using a stack for undo functionality and a queue for task scheduling.
8. Can you explain the concept of sorting algorithms and why they are important?
Sorting algorithms are methods for arranging elements in a list or array in a specific order, typically ascending or descending. Common sorting algorithms include bubble sort, quick sort, merge sort, and insertion sort.
Sorting is important because it optimizes the efficiency of other operations, such as searching and merging datasets.
Candidates should be able to compare different sorting algorithms based on their time and space complexities and provide examples of when each algorithm might be the most appropriate choice.
9. How would you handle a situation where you need to merge two sorted arrays?
To merge two sorted arrays, you can use a two-pointer technique. Start by comparing the first elements of both arrays, placing the smaller element into a new array, and incrementing the pointer of the array from which the element was taken. Repeat this process until all elements are merged.
This approach ensures that the merged array remains sorted and operates in O(n) time complexity.
Look for candidates who can clearly articulate this process and discuss edge cases, such as arrays of different lengths or empty arrays, and how they would handle them.
8 Coding interview questions and answers related to data structures
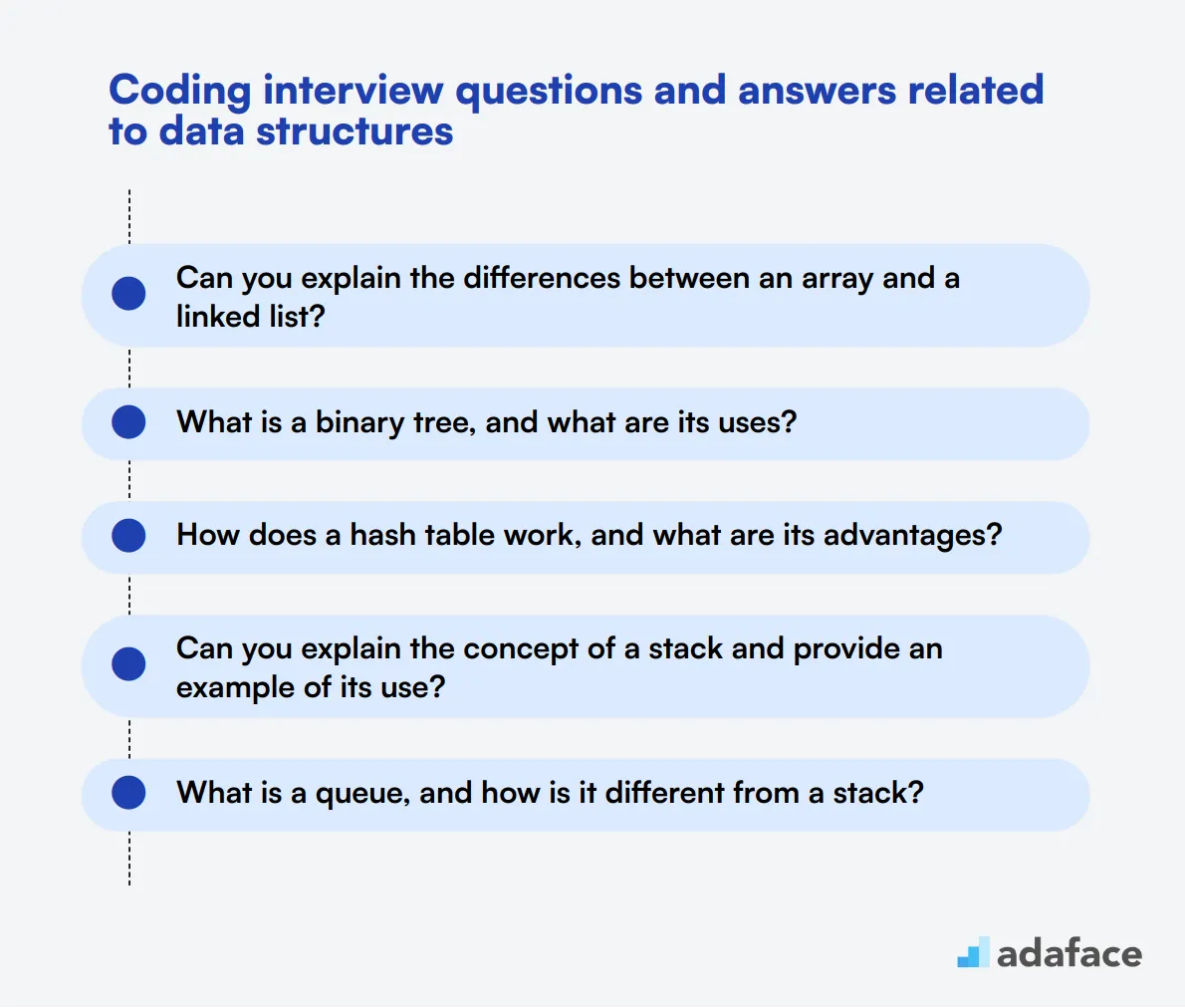
To determine whether your candidates have a solid understanding of essential data structures, consider asking them these 8 insightful interview questions. These questions will help you gauge their problem-solving abilities and their practical knowledge, ensuring they can handle real-world challenges in your projects.
1. Can you explain the differences between an array and a linked list?
An array is a collection of elements, each identified by an array index or key. Arrays have a fixed size, meaning the number of elements an array can hold is predetermined. They allow direct access to any element using its index, which makes access time very fast.
On the other hand, a linked list is a collection of nodes, where each node contains a value and a reference to the next node in the sequence. Linked lists do not have a fixed size and can grow or shrink dynamically. However, accessing an element in a linked list requires traversing the list from the head node to the desired node, which can be slower.
Look for candidates who can clearly explain the trade-offs between arrays and linked lists, such as memory usage, ease of insertion/deletion, and access time.
2. What is a binary tree, and what are its uses?
A binary tree is a hierarchical data structure in which each node has at most two children, referred to as the left child and the right child. This structure is particularly useful for organizing data in a way that allows for efficient searching, insertion, and deletion.
Binary trees are used in various applications, such as in the implementation of binary search trees (BSTs) for fast lookup, insertion, and deletion. They are also used in heap data structures, which are useful for priority queue implementations, and in parsing expressions in compilers.
An ideal response should outline these uses and demonstrate an understanding of how binary trees can improve data handling efficiency. Follow up by asking for examples of when they have used binary trees in their previous projects.
3. How does a hash table work, and what are its advantages?
A hash table is a data structure that stores key-value pairs. It uses a hash function to compute an index into an array of buckets or slots, from which the desired value can be found. This makes data retrieval very fast, typically O(1) time complexity.
The main advantages of hash tables are their efficiency in search, insert, and delete operations. They are widely used in situations where fast data retrieval is critical, such as in databases and caches.
Listen for a candidate's ability to explain the concept of hashing and how it helps in reducing search time. They should also understand the potential issues like hash collisions and ways to handle them, such as chaining or open addressing.
4. Can you explain the concept of a stack and provide an example of its use?
A stack is a linear data structure that follows a Last In, First Out (LIFO) order. This means that the last element added to the stack will be the first one to be removed. Basic stack operations include push (adding an element), pop (removing the top element), and peek (retrieving the top element without removing it).
Stacks are used in various applications, such as in function call management in recursive programming, where each function call is pushed onto the stack and popped off when the function returns. They are also useful in undo mechanisms in text editors, where the most recent action is undone first.
Candidates should be able to clearly articulate the LIFO principle and provide practical examples of where they have used stacks in their projects. Consider probing their understanding of stack-related issues, such as stack overflow.
5. What is a queue, and how is it different from a stack?
A queue is a linear data structure that follows a First In, First Out (FIFO) order. This means that the first element added to the queue will be the first one to be removed. Basic queue operations include enqueue (adding an element to the rear), dequeue (removing the front element), and peek (retrieving the front element without removing it).
Unlike a stack, where elements are added and removed from the same end, a queue adds elements at the rear and removes them from the front. Queues are commonly used in scenarios like task scheduling, where tasks are processed in the order they arrive, and in breadth-first search algorithms.
A strong candidate will be able to explain the FIFO principle and provide examples of practical applications of queues. Watch for their ability to differentiate between stack and queue operations and the contexts in which each is used.
6. Can you describe what a graph is and provide a real-world example of its use?
A graph is a data structure consisting of nodes, also known as vertices, and edges that connect pairs of nodes. Graphs can be directed or undirected, weighted or unweighted. They are used to represent networks of various kinds, such as social networks, computer networks, and transportation networks.
A real-world example of a graph is a city's road map, where intersections are represented as nodes and roads as edges. This structure allows for efficient pathfinding algorithms, such as finding the shortest path between two locations.
Look for candidates who can clearly explain the basic components of a graph and provide relevant examples. Their understanding of different types of graphs and their applications will be crucial in assessing their practical knowledge.
7. What is the significance of sorting algorithms, and can you name a few common ones?
Sorting algorithms are essential because they arrange data in a specific order, making it easier to search, analyze, and visualize. Efficient sorting is crucial for optimizing the performance of other algorithms that require sorted data.
Some common sorting algorithms include bubble sort, selection sort, insertion sort, quicksort, mergesort, and heapsort. Each algorithm has its strengths and weaknesses, and the choice of algorithm can depend on the size and nature of the data.
Candidates should demonstrate an understanding of why sorting is important and should be familiar with various sorting algorithms and their complexities. Consider asking them to compare the performance of different algorithms under various conditions.
8. How would you approach finding the shortest path in a graph?
To find the shortest path in a graph, one can use algorithms like Dijkstra's algorithm or the A* algorithm. Dijkstra's algorithm is commonly used for graphs with non-negative weights and works by exploring the shortest path to each node step-by-step. The A* algorithm, on the other hand, is a more advanced version that uses heuristics to optimize the search.
The choice of algorithm depends on the specific requirements, such as the nature of the graph and the type of weights it contains. For example, the Bellman-Ford algorithm is suitable for graphs with negative weights.
Look for candidates who can explain these algorithms and their use cases. They should also demonstrate an understanding of the trade-offs involved in choosing one algorithm over another.
8 situational Coding interview questions with answers for hiring top developers
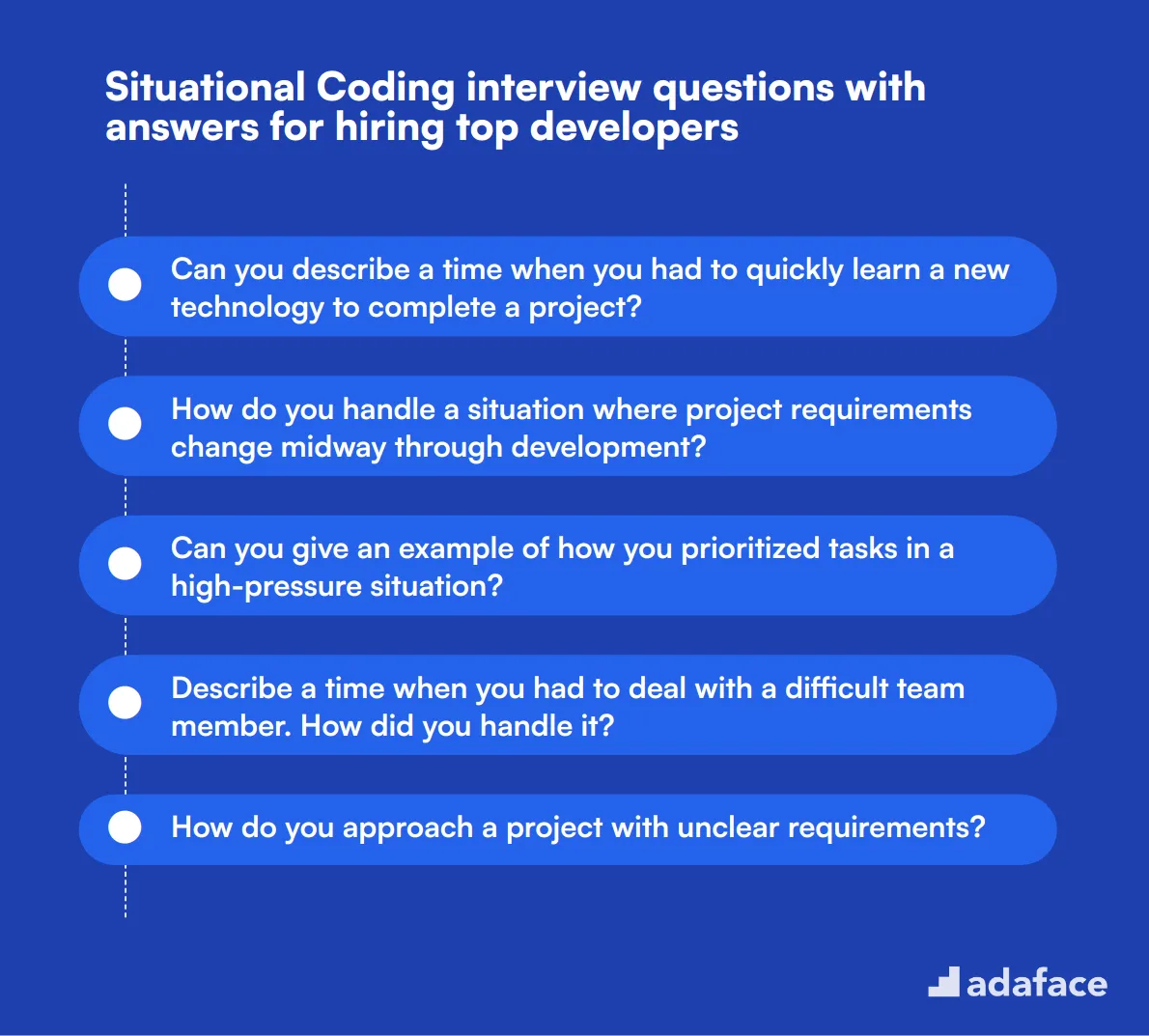
To hire top developers, you need to understand how they handle real-world coding challenges. These situational coding interview questions will help you assess their problem-solving skills, adaptability, and ability to thrive in your team. Use these questions during your interviews to identify candidates who can excel in your development environment.
1. Can you describe a time when you had to quickly learn a new technology to complete a project?
In this scenario, I had to pick up a new front-end framework to meet a tight deadline. I started by identifying the key features and components we needed for the project. Then, I went through the official documentation, online tutorials, and example projects to gain a working knowledge.
I also joined community forums and sought help from colleagues with experience in the framework. Within a week, I was able to get up to speed and contribute effectively to the project. As a result, we delivered the project on time and received positive feedback from the client.
Look for candidates who demonstrate initiative, resourcefulness, and the ability to quickly adapt to new technologies. Follow up by asking what resources they find most helpful when learning new tech and how they overcome initial learning hurdles.
2. How do you handle a situation where project requirements change midway through development?
When project requirements change, the first step is to understand the new requirements thoroughly. I usually arrange a meeting with the stakeholders to discuss the changes and their impact on the project. Once I have a clear understanding, I reassess the project timeline and resources.
I then communicate the changes to the team, updating our project plan and redistributing tasks as needed. Throughout this process, I ensure constant communication with stakeholders to manage expectations and avoid any surprises.
Ideal candidates should show strong communication skills and flexibility. They should be able to describe how they manage scope changes without compromising on quality or deadlines. Look for their ability to keep the team motivated during such shifts.
3. Can you give an example of how you prioritized tasks in a high-pressure situation?
In a high-pressure situation, I start by listing all tasks and categorizing them based on urgency and importance. I use techniques like the Eisenhower Matrix to identify which tasks need immediate attention and which can wait.
Once prioritized, I focus on completing high-impact tasks first. I also communicate with my team to ensure everyone is aligned on priorities and deadlines. Regular check-ins help us stay on track and make adjustments as needed.
Candidates should demonstrate a methodical approach to prioritizing tasks. Look for their ability to stay calm under pressure and their communication skills in ensuring everyone is on the same page. You might want to ask about specific tools or methods they use for task management.
4. Describe a time when you had to deal with a difficult team member. How did you handle it?
I once worked with a team member who was consistently missing deadlines and affecting the team's progress. I decided to address the issue directly by having a one-on-one conversation to understand the root cause of their performance issues.
During our discussion, I learned that they were struggling with personal issues and were overwhelmed with their workload. We agreed on a more manageable set of tasks and provided additional support where needed. This improved their performance and team dynamics.
Look for candidates who handle conflicts professionally and empathetically. They should be able to describe how they turned a challenging situation around and maintained a positive team environment. Follow up by asking how they prevent such issues from recurring.
5. How do you approach a project with unclear requirements?
When faced with unclear requirements, my first step is to seek clarity from stakeholders. I arrange meetings or workshops to gather detailed information and align on project goals. Creating user stories and use cases can also help in understanding the requirements better.
I document everything meticulously and keep a record of all communications. This helps in resolving any discrepancies later. Once I have enough clarity, I proceed with a phased approach, ensuring frequent check-ins with stakeholders to validate our progress.
Candidates should demonstrate proactive communication and a structured approach to handling ambiguity. Look for their ability to gather and document requirements effectively. You might also want to ask about their techniques for verifying and validating requirements throughout the project lifecycle.
6. Can you share an experience where you had to work on a project with limited resources?
In a project with limited resources, prioritization and efficient resource management are key. I started by identifying the must-have features and focusing our efforts on delivering those first. I also looked for ways to automate repetitive tasks to save time.
We made use of open-source tools and libraries to cut down costs and leveraged the team's diverse skills to fill in any gaps. Regular progress reviews ensured we stayed on track and adjusted our approach as needed to meet our goals.
Ideal candidates should show creativity and problem-solving skills. Look for their ability to make the best use of available resources and deliver quality results despite constraints. Follow up by asking how they ensure resource constraints do not compromise the project's outcomes.
7. How do you ensure that a project stays on schedule?
To keep a project on schedule, I start with a detailed project plan that includes milestones, deadlines, and responsibilities. I use project management tools to track progress and set up regular status meetings to review where we stand.
I also anticipate potential risks and have contingency plans in place. If any delays occur, I communicate them promptly with stakeholders and adjust our plan accordingly to get back on track.
Candidates should demonstrate strong organizational skills and proactive risk management. Look for their ability to use project management tools effectively and their communication strategies to keep everyone aligned on timelines.
8. Describe a time when you had to mentor a junior developer. How did you approach it?
I once mentored a junior developer who was struggling with understanding our codebase. I started by conducting regular one-on-one sessions to explain complex concepts and provide hands-on guidance. We pair-programmed on tasks to help them understand our coding standards and processes.
I also encouraged them to ask questions and provided feedback on their code. Over time, they became more confident and started contributing effectively to the team. This experience not only helped them grow but also improved our team's overall productivity.
Look for candidates who show patience, clear communication, and a structured approach to mentoring. They should be able to describe how they personalized their mentorship to meet the junior developer's needs and fostered a supportive learning environment. Follow up by asking about specific mentoring techniques they found most effective.
Which Coding skills should you evaluate during the interview phase?
While it's challenging to assess every aspect of a candidate's abilities in a single interview, focusing on core coding skills is crucial. These fundamental competencies provide insight into a developer's potential and fit for the role.
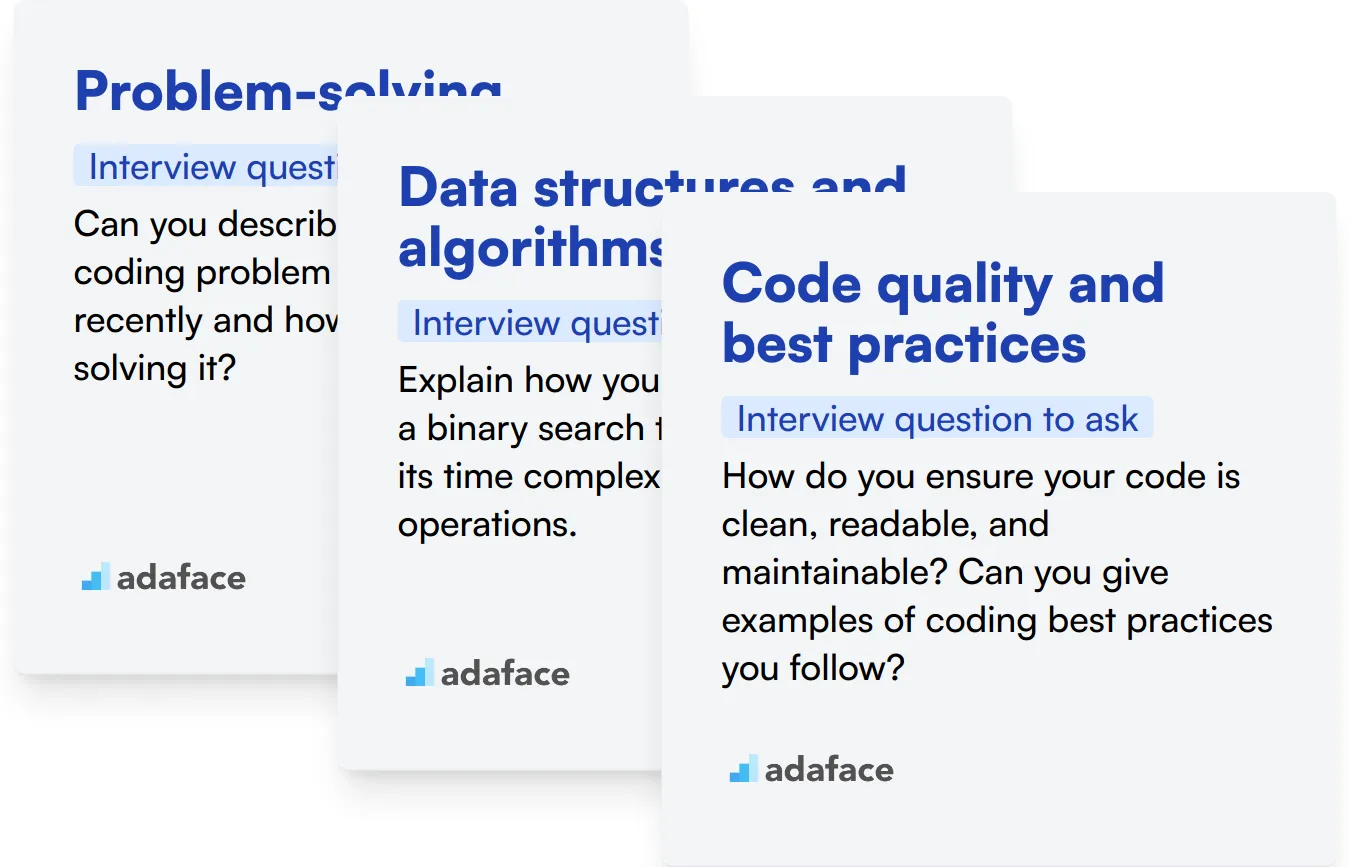
Problem-solving
Problem-solving is at the heart of coding. It reflects a developer's ability to break down complex issues and devise effective solutions.
To evaluate problem-solving skills, consider using an assessment test with relevant MCQs. This can help filter candidates based on their analytical thinking abilities.
During the interview, ask targeted questions to gauge this skill. Here's an example:
Can you describe a challenging coding problem you've faced recently and how you approached solving it?
Look for candidates who can articulate their thought process, explain their problem-solving strategy, and discuss any obstacles they encountered and overcame.
Data structures and algorithms
Knowledge of data structures and algorithms is fundamental for efficient coding. It enables developers to choose the right tools for specific tasks and optimize performance.
Consider using a data structures online test to assess candidates' understanding of these concepts efficiently.
To evaluate this skill during the interview, you might ask:
Explain how you would implement a binary search tree and discuss its time complexity for different operations.
Pay attention to the candidate's understanding of the data structure, their ability to explain the implementation, and their grasp of time complexity concepts.
Code quality and best practices
Writing clean, maintainable code is essential for long-term project success. It shows a developer's attention to detail and commitment to professional standards.
You can use a coding test that evaluates code quality alongside functionality to assess this skill.
During the interview, consider asking:
How do you ensure your code is clean, readable, and maintainable? Can you give examples of coding best practices you follow?
Look for answers that demonstrate awareness of coding standards, commenting practices, modular design, and principles like DRY (Don't Repeat Yourself).
Hire top talent with coding skills tests and the right interview questions
When you're looking to hire someone with coding skills, it’s important to ensure they possess the necessary abilities. Accurate assessment is key to identifying candidates who can truly excel in their roles.
The most effective way to verify these skills is by using coding skills tests. Consider using our Software Engineering Online Test to gauge candidates' expertise accurately.
After administering these tests, you can efficiently shortlist the best applicants and invite them for interviews. This approach allows you to focus on candidates who demonstrate genuine coding proficiency.
To get started, visit our test library and explore our range of assessments. Sign up today to streamline your hiring process and secure top coding talent for your team.
Computer Programmer Coding Aptitude Test
Download Coding interview questions template in multiple formats
Coding Interview Questions FAQs
Focus on basic concepts, problem-solving abilities, and foundational understanding of programming languages.
Ask questions related to common algorithms and evaluate their approach to solving algorithmic problems.
Senior developers should be asked advanced questions that test their deep understanding, architecture skills, and decision-making abilities.
These questions help gauge how candidates react to real-world scenarios and their problem-solving capabilities in dynamic situations.
Use coding skills tests to screen candidates before interviews, ensuring they possess the basic technical skills required for the role.
Evaluate their problem-solving process, code efficiency, and ability to communicate their thought process clearly.
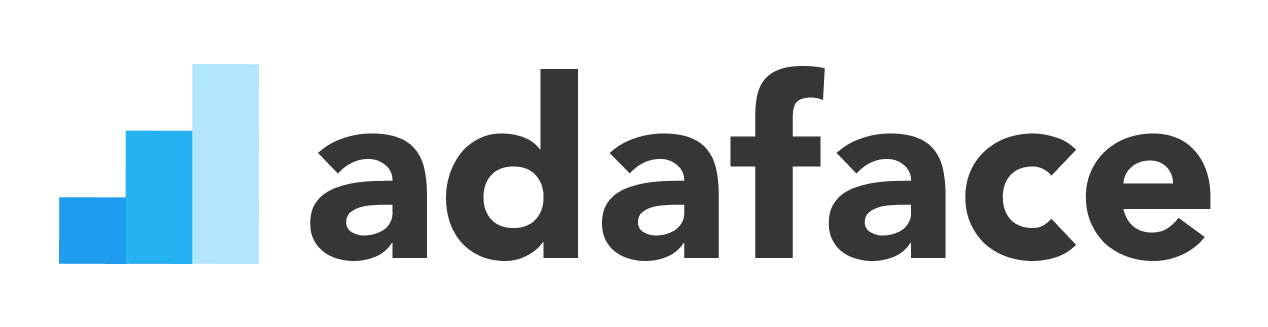
40 min skill tests.
No trick questions.
Accurate shortlisting.
We make it easy for you to find the best candidates in your pipeline with a 40 min skills test.
Try for freeRelated posts
Free resources
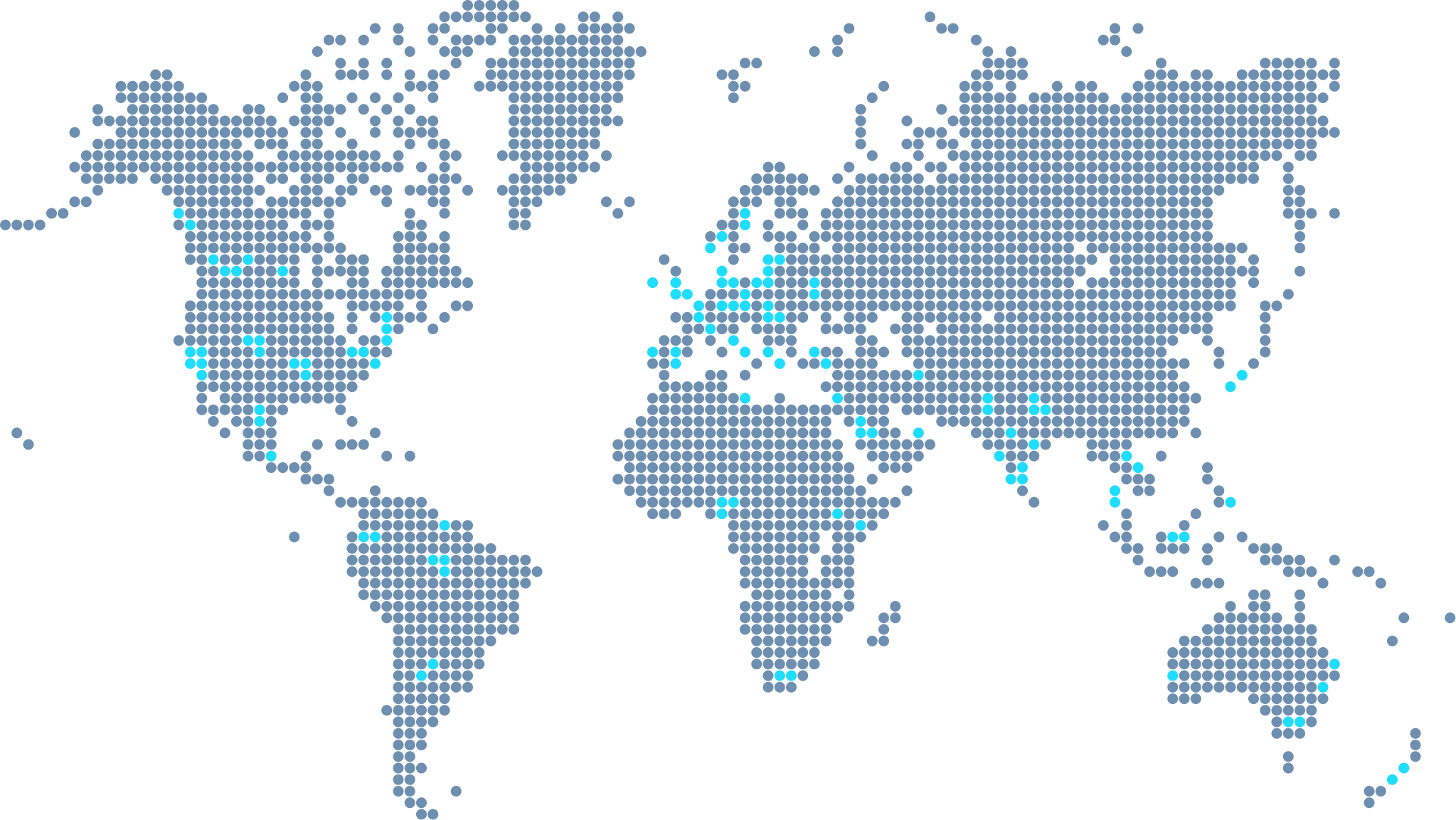
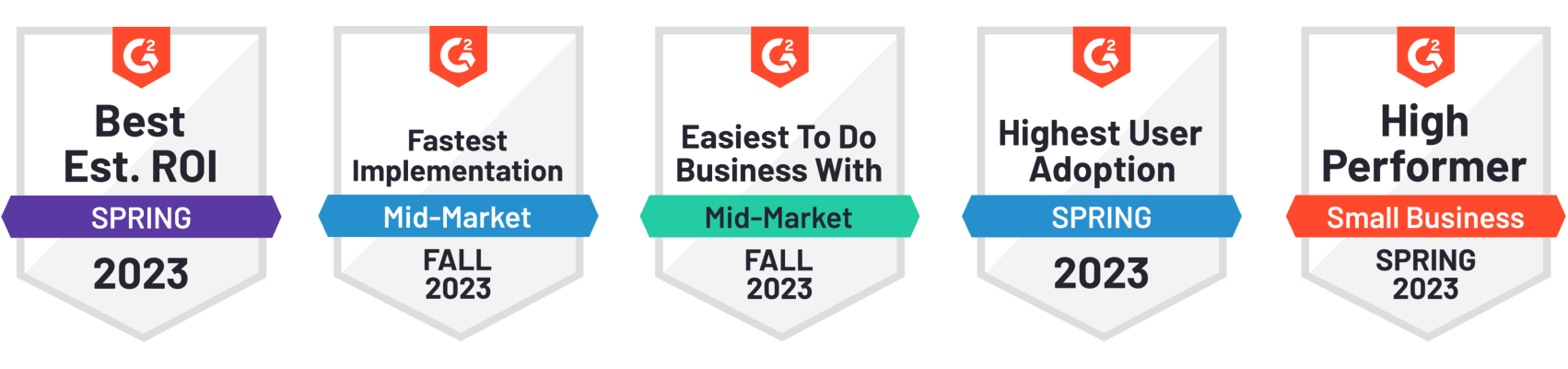