Hiring the right C# developer can be a challenge, especially when you're not sure what questions to ask during the interview. A well-structured interview process helps you assess candidates' skills and find the perfect fit for your team.
This blog post provides a comprehensive list of C# interview questions tailored for different experience levels. We cover top questions for general interviews, junior developers, mid-tier developers, OOP concepts, and technical definitions.
By using these questions, you'll gain valuable insights into candidates' C# proficiency and problem-solving abilities. Consider pairing these interview questions with a C# skills assessment for a more thorough evaluation of potential hires.
Table of contents
Top 8 C# questions to ask in interviews
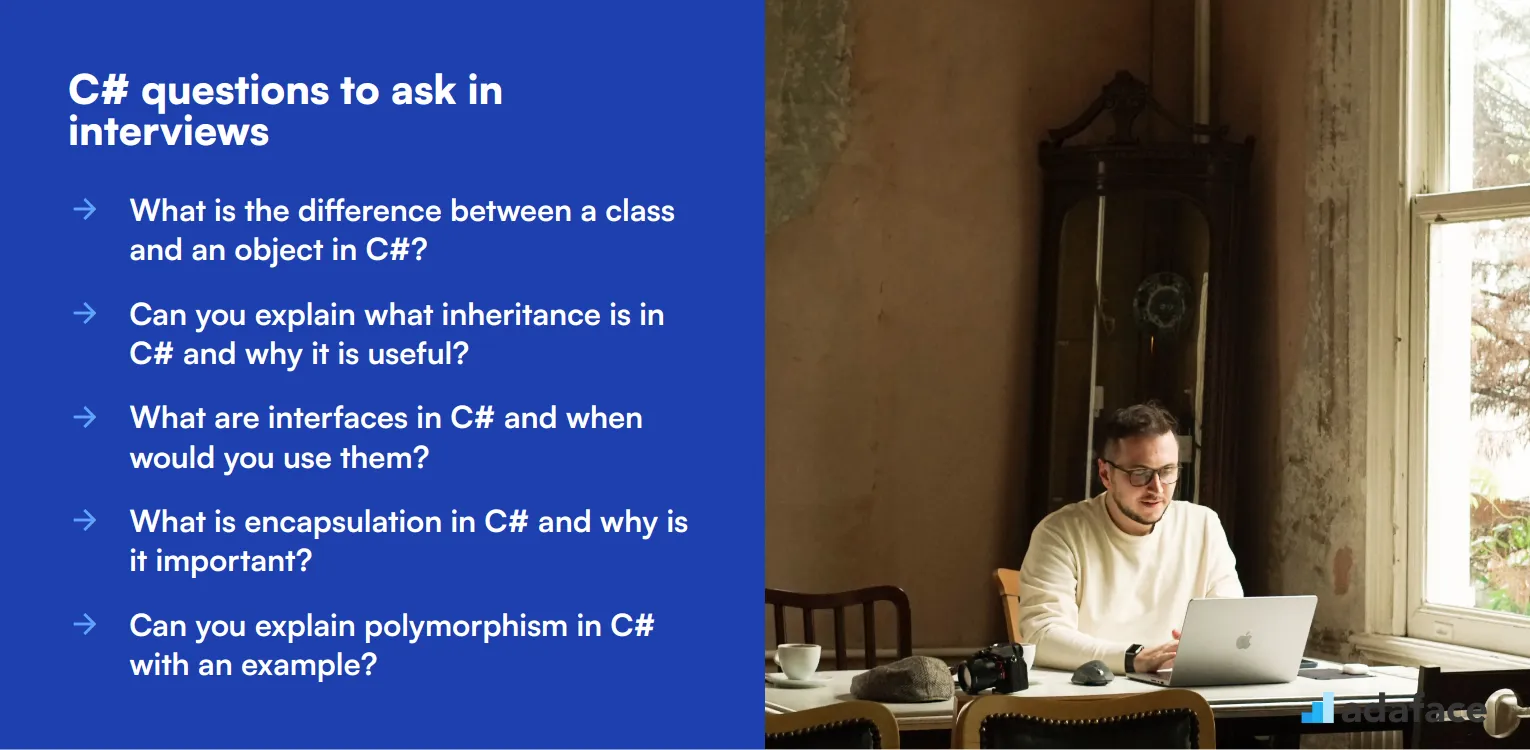
To gauge whether your candidates possess the right skills and understanding of C#, consider using these top interview questions. This list will help you identify strong candidates who can effectively contribute to your team and handle complex tasks.
1. What is the difference between a class and an object in C#?
A class in C# is a blueprint or template that defines the properties and behaviors of objects. It encapsulates data for the object and methods to manipulate that data.
An object, on the other hand, is an instance of a class. When a class is instantiated, it creates an object that adheres to the structure defined by the class. For example, if you have a class named 'Car', an object of this class could be 'myCar' with specific attributes like color and model.
Look for candidates who can clearly articulate the relationship between classes and objects, as well as provide practical examples. Follow up by asking them to discuss scenarios where they have used classes and objects in their past projects.
2. Can you explain what inheritance is in C# and why it is useful?
Inheritance in C# is a mechanism where one class (the child or derived class) inherits the attributes and methods of another class (the parent or base class). This allows for hierarchical classification and code reusability.
It is useful because it promotes code reuse, reduces redundancy, and makes the system easier to maintain. For instance, if you have a base class 'Animal' with properties like 'Name' and methods like 'Move', a derived class 'Bird' can inherit these properties and methods without having to rewrite them and can add new features like 'Fly'.
An ideal candidate should demonstrate an understanding of how inheritance can simplify code management and provide examples from their experience. Follow up by asking how they handle scenarios where inheritance might complicate the design.
3. What are interfaces in C# and when would you use them?
Interfaces in C# define a contract that classes can implement. They contain definitions for a group of related functionalities that a class must provide. However, interfaces do not provide implementation; they only specify what methods or properties a class must implement.
You would use interfaces to achieve loose coupling and enhance testability in your code. For instance, if you have an interface 'IDatabase' with methods like 'Save' and 'Load', different classes can implement this interface to provide specific implementations for these methods, such as 'SQLDatabase' or 'NoSQLDatabase'.
Look for candidates who understand the importance of interfaces in promoting cleaner, more modular code. Ask them to discuss scenarios where they have used interfaces to facilitate code maintenance and testing.
4. What is encapsulation in C# and why is it important?
Encapsulation is one of the fundamental principles of object-oriented programming (OOP). It involves bundling the data (variables) and methods (functions) that operate on the data into a single unit or class. Additionally, it restricts direct access to some of an object's components, which can prevent the accidental modification of data.
Encapsulation is important because it helps to protect the internal state of an object from unintended interference and misuse. It makes the code easier to understand and maintain by providing a clear separation of concerns. For instance, using private fields and public methods ensures that access to the data is controlled and validated.
Strong candidates should articulate the benefits of encapsulation and provide examples of how they have used it in their projects. Follow up by discussing the balance between too much and too little encapsulation.
5. Can you explain polymorphism in C# with an example?
Polymorphism is an OOP concept where methods or objects can take on multiple forms. In C#, this can be achieved through method overriding and method overloading. Polymorphism allows for the implementation of methods that behave differently based on the object that invokes them.
For example, consider a base class 'Shape' with a method 'Draw()'. A derived class 'Circle' and another derived class 'Square' can both override the 'Draw()' method to provide their specific implementations. When you call the 'Draw()' method on an object of type 'Shape', the correct method (either 'Circle's Draw()' or 'Square's Draw()') will be executed based on the actual object type.
An ideal candidate should be able to explain both types of polymorphism (overriding and overloading) and provide practical examples from their experience. Ask them to discuss how they ensure that polymorphism is implemented effectively without leading to complex and hard-to-maintain code.
6. What do you understand by the term 'delegate' in C#?
A delegate in C# is a type that represents references to methods with a particular parameter list and return type. Delegates are used to pass methods as arguments to other methods, enabling callback functionality and event handling.
Delegates are especially useful in designing extensible and flexible applications, such as implementing event handling in GUI applications. For example, you can define a delegate for a method that processes a string and then allow different methods to be assigned to this delegate, making your code more modular and reusable.
Look for candidates who can explain the concept clearly and provide examples from their work. Follow up by asking them how they use delegates in event-driven programming and the benefits they have observed.
7. Could you explain the concept of garbage collection in C#?
Garbage collection in C# is an automated process that frees up memory occupied by objects that are no longer in use. The .NET runtime's garbage collector periodically scans for such objects and reclaims the memory, thus helping in efficient memory management.
Garbage collection is important because it helps to prevent memory leaks and ensures that the application does not consume more memory than necessary. It simplifies the developer's job by handling memory deallocation automatically, reducing the risk of memory-related issues.
Candidates should demonstrate an understanding of how garbage collection works and its advantages. Ask them to discuss scenarios where they had to optimize memory usage and how they monitored and managed memory in their applications.
8. What is exception handling in C# and how do you implement it?
Exception handling in C# is a mechanism to handle runtime errors, ensuring that the normal flow of the application is maintained. It is implemented using try, catch, finally, and throw keywords.
The 'try' block contains the code that might throw an exception, 'catch' blocks handle specific exceptions, and the 'finally' block contains code that runs regardless of whether an exception was thrown or not, often used for cleanup. The 'throw' keyword is used to throw an exception explicitly.
Ideal candidates should explain the importance of robust exception handling and provide examples of how they have used it to manage errors gracefully in their applications. Follow up by asking how they ensure that exception handling does not obscure the root cause of issues.
20 C# interview questions to ask junior developers
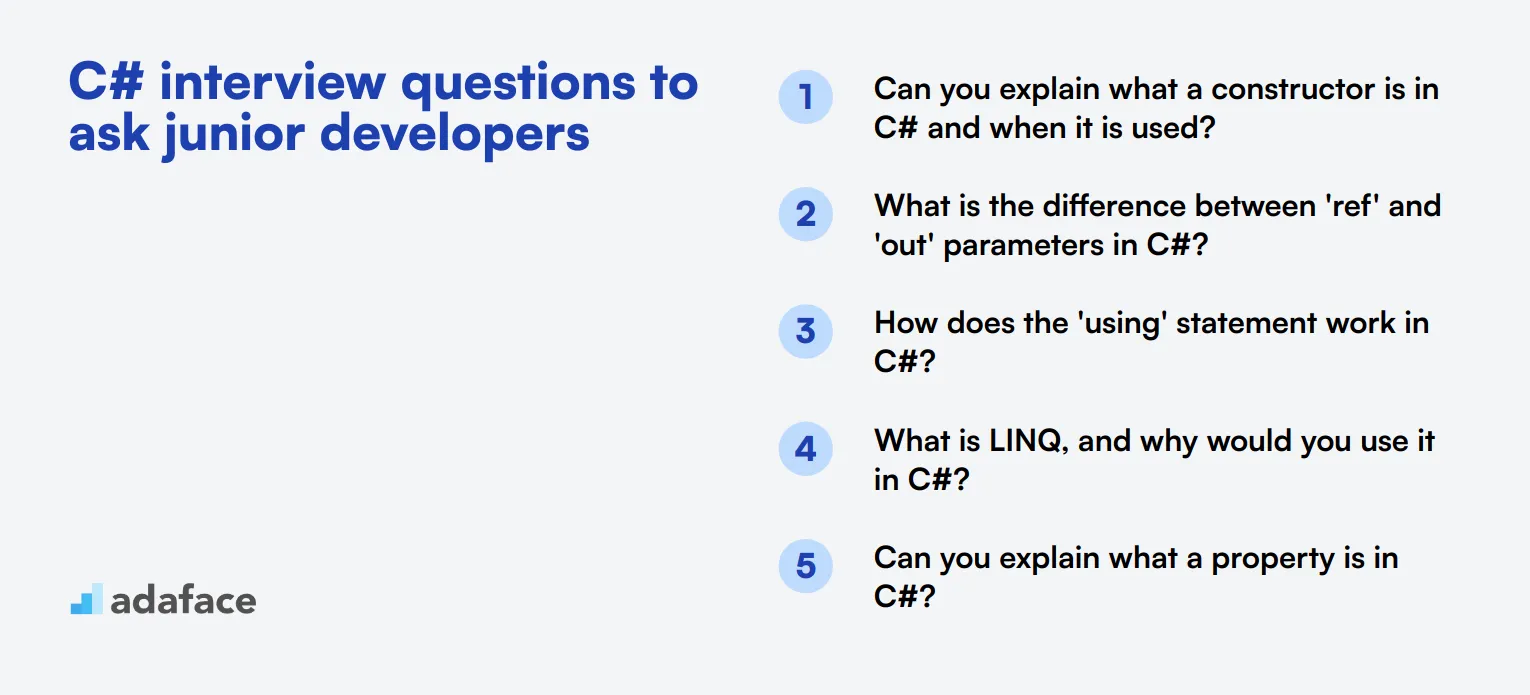
To assess whether junior developers possess the foundational knowledge necessary for a C# role, consider using this list of targeted questions. These inquiries will help you gauge their understanding of essential concepts and practices in C#. For more detailed guidance on what to look for, check out the job descriptions.
- Can you explain what a constructor is in C# and when it is used?
- What is the difference between 'ref' and 'out' parameters in C#?
- How does the 'using' statement work in C#?
- What is LINQ, and why would you use it in C#?
- Can you explain what a property is in C#?
- What are value types and reference types in C#?
- How do you create an array in C#, and what are its limitations?
- What is the purpose of the 'static' keyword in C#?
- Can you explain the concept of a namespace in C#?
- What is the role of the 'this' keyword in C#?
- How do you handle events in C#?
- What is the difference between an abstract class and an interface?
- Can you describe what a collection is in C# and name a few types?
- What is the purpose of the 'async' and 'await' keywords in C#?
- Can you explain what dependency injection is and how it’s used in C#?
- What are the different types of exceptions in C#?
- How do you implement a simple method overloading in C#?
- What is unit testing, and how does it relate to C# development?
- Can you explain what nullable types are in C#?
- What is the purpose of the 'lock' statement in C#?
10 intermediate C# interview questions and answers to ask mid-tier developers
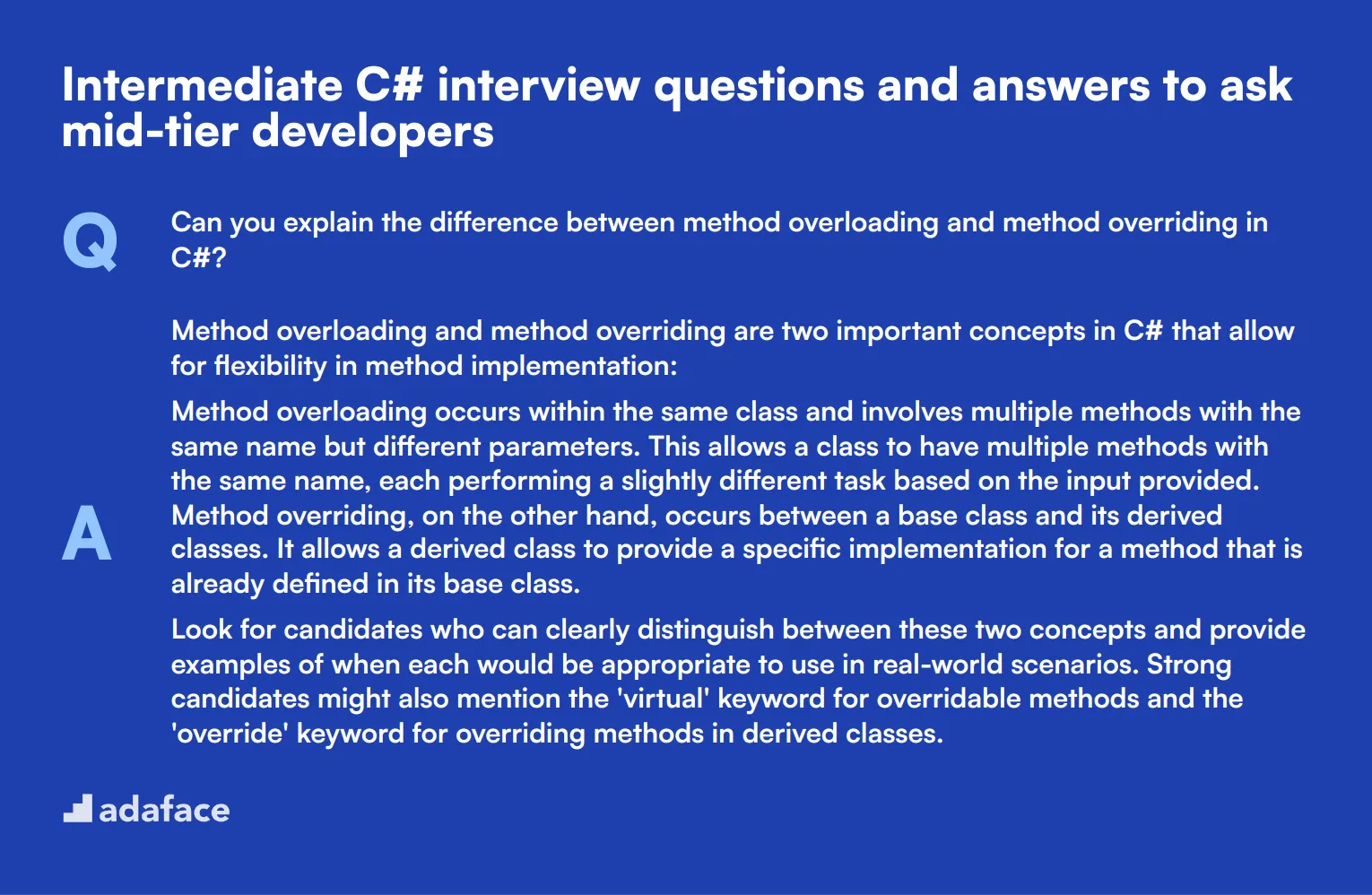
Ready to level up your C# interviews? These 10 intermediate questions are perfect for assessing mid-tier developers. They'll help you gauge a candidate's deeper understanding of C# concepts and their ability to apply them in real-world scenarios. Use these questions to spark meaningful discussions and uncover the true potential of your C# developer candidates.
1. Can you explain the difference between method overloading and method overriding in C#?
Method overloading and method overriding are two important concepts in C# that allow for flexibility in method implementation:
- Method overloading occurs within the same class and involves multiple methods with the same name but different parameters. This allows a class to have multiple methods with the same name, each performing a slightly different task based on the input provided.
- Method overriding, on the other hand, occurs between a base class and its derived classes. It allows a derived class to provide a specific implementation for a method that is already defined in its base class.
Look for candidates who can clearly distinguish between these two concepts and provide examples of when each would be appropriate to use in real-world scenarios. Strong candidates might also mention the 'virtual' keyword for overridable methods and the 'override' keyword for overriding methods in derived classes.
2. What is the difference between 'const' and 'readonly' keywords in C#?
The 'const' and 'readonly' keywords in C# are both used to create variables whose values cannot be modified, but they have some key differences:
- Const: Variables declared as const must be initialized at the time of declaration. Their values are determined at compile-time and cannot be changed. Const is typically used for values that are truly constant, like mathematical constants.
- Readonly: Variables declared as readonly can be initialized either at the time of declaration or in the constructor of the class. Their values are determined at runtime and can be different for different instances of a class. Readonly is often used for values that are constant for an object throughout its lifetime but may be different for different objects.
A strong candidate should be able to explain these differences clearly and provide examples of when to use each. They might also mention that const is implicitly static, while readonly can be instance-specific.
3. How does the 'yield' keyword work in C# and when would you use it?
The 'yield' keyword in C# is used to define an iterator method, which returns an enumerable object. It's a powerful feature for creating sequences without the need to create temporary collections. Here's how it works:
- When a method uses 'yield return', it returns each element one at a time, and only when requested.
- The state of the method is maintained between calls, allowing it to resume where it left off.
- It's memory-efficient as it doesn't require the entire sequence to be in memory at once.
Look for candidates who can explain scenarios where 'yield' is beneficial, such as working with large data sets or infinite sequences. They should understand that it's useful for lazy evaluation and can improve performance in certain situations. A strong candidate might also mention the difference between 'yield return' and 'yield break'.
4. What are extension methods in C# and how do they work?
Extension methods in C# allow developers to add new methods to existing types without modifying the original type. They're a powerful feature for extending functionality, especially when working with types you can't modify directly. Here's how they work:
- Extension methods are defined as static methods in static classes.
- They use the 'this' keyword before the first parameter to specify the type being extended.
- Once defined, they can be called as if they were instance methods of the extended type.
When evaluating responses, look for candidates who can explain the syntax for creating extension methods and discuss their advantages and limitations. Strong candidates might mention that extension methods don't break encapsulation, as they can't access private members of the extended type. They might also discuss scenarios where extension methods are particularly useful, such as adding functionality to sealed classes or interfaces.
5. Can you explain what a finalizer is in C# and when you would use one?
A finalizer (also known as a destructor in C++) is a special method in a class that is called by the garbage collector before an object is destroyed. It's used to perform any necessary final clean-up when a class instance is being collected by the garbage collector. Key points about finalizers include:
- They are defined using the class name preceded by a tilde (~).
- They cannot be called directly and have no parameters or access modifiers.
- They are used to release unmanaged resources that the class may be holding.
Look for candidates who understand that finalizers should be used sparingly, as they can impact performance and delay garbage collection. Strong candidates might mention that it's generally better to implement the IDisposable interface for deterministic cleanup of resources. They should also be aware that finalizers run on a separate thread and that there's no guarantee when (or if) a finalizer will be called.
6. What is the difference between 'is' and 'as' operators in C#?
The 'is' and 'as' operators in C# are both used for type checking and casting, but they serve different purposes:
- The 'is' operator: Used to check if an object is compatible with a given type. It returns a boolean value (true or false) and does not perform any conversion.
- The 'as' operator: Used to perform conversions between compatible reference types or nullable types. It returns null if the conversion is not possible, rather than throwing an exception.
A strong candidate should be able to explain when to use each operator. They might mention that 'is' is often used in conditional statements, while 'as' is useful when you want to attempt a conversion without risking an exception. Look for candidates who can discuss the performance implications and safety considerations of each operator. They might also mention pattern matching with 'is' in more recent versions of C#.
7. How do you implement a custom exception class in C#?
Implementing a custom exception class in C# allows developers to create specific exceptions for their application's needs. Here's how it's typically done:
- Create a new class that inherits from the Exception class (or a more specific exception class if appropriate).
- Implement constructors that call the base class constructors.
- Override properties like Message or add custom properties to provide more context about the exception.
When evaluating responses, look for candidates who understand the importance of following .NET naming conventions (e.g., ending the class name with 'Exception'). Strong candidates might discuss serialization considerations, the use of custom error codes, or how to properly document custom exceptions. They should also be able to explain scenarios where custom exceptions are beneficial, such as providing more meaningful error information in complex business logic.
8. What are anonymous types in C# and when would you use them?
Anonymous types in C# allow you to create objects without explicitly defining a type. They are typically used for short-lived objects where creating a named type would be unnecessary. Key features of anonymous types include:
- They are created using the 'new' keyword followed by curly braces containing property definitions.
- Properties are read-only and inferred from the initialization expressions.
- They are particularly useful in LINQ queries for projecting results.
Look for candidates who can explain the syntax for creating anonymous types and discuss their limitations (e.g., they can't be used as method return types or parameters). Strong candidates might mention that anonymous types are immutable and that their scope is limited to the method where they are defined. They should also be able to provide examples of when anonymous types are particularly useful, such as in data transformation scenarios or when working with temporary data structures.
9. Can you explain the concept of covariance and contravariance in C#?
Covariance and contravariance are advanced concepts in C# that deal with the compatibility of generic types. They allow for more flexible use of generic interfaces and delegates:
- Covariance: Allows you to use a more derived type than originally specified. It's declared using the 'out' keyword and typically used with return types.
- Contravariance: Allows you to use a more general (less derived) type than originally specified. It's declared using the 'in' keyword and typically used with parameter types.
When evaluating responses, look for candidates who can provide concrete examples of where these concepts are useful, such as in collections or delegate scenarios. Strong candidates might discuss how covariance and contravariance relate to the Liskov Substitution Principle or explain the limitations (e.g., they only work with reference types). They should also be able to explain the benefits in terms of code flexibility and reusability.
10. What is the difference between 'IEnumerable' and 'IQueryable' in C#?
IEnumerable and IQueryable are both interfaces used for working with collections of data, but they have some key differences:
- IEnumerable: Represents a read-only collection of elements that can be enumerated. It operates on in-memory data and executes queries on the client side.
- IQueryable: Extends IEnumerable and represents a queryable collection of elements. It can translate queries into a format that can be understood by the data source, allowing for server-side execution of queries.
Look for candidates who can explain that IQueryable is typically used for remote data sources (like databases) to allow for more efficient querying. Strong candidates might discuss the performance implications of each interface, explaining that IQueryable can be more efficient for large datasets as it allows filtering to occur at the data source. They should also be able to provide examples of when to use each interface and discuss how they relate to LINQ operations.
12 C# interview questions about OOPs
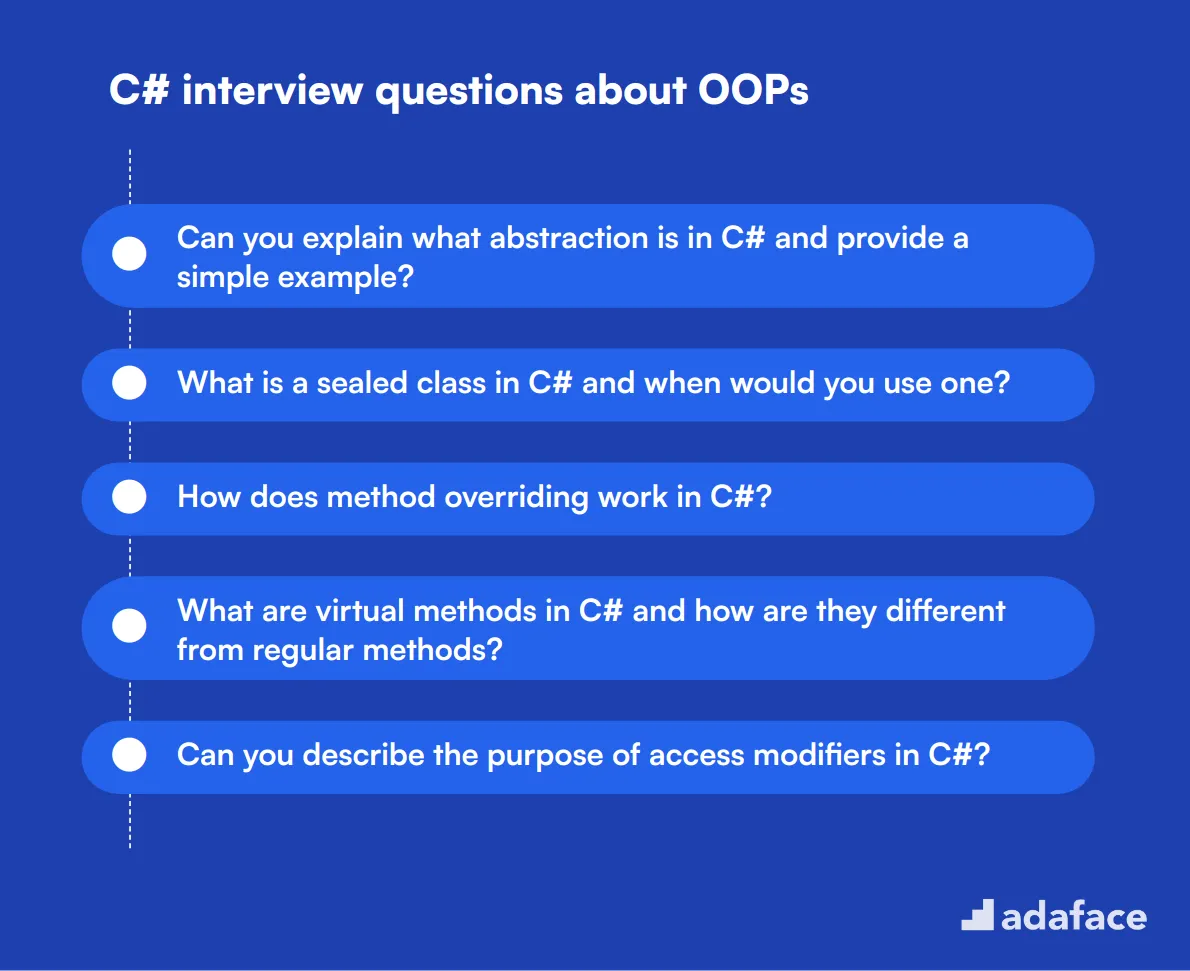
To assess whether your applicants have a solid understanding of Object-Oriented Programming principles in C#, use these 12 targeted interview questions. These questions are designed to help you evaluate their practical knowledge and ability to implement OOP concepts effectively in real-world scenarios. For more detailed role descriptions, you might find this C# Developer Job Description helpful.
- Can you explain what abstraction is in C# and provide a simple example?
- What is a sealed class in C# and when would you use one?
- How does method overriding work in C#?
- What are virtual methods in C# and how are they different from regular methods?
- Can you describe the purpose of access modifiers in C#?
- What is a nested class in C# and when might you use it?
- How do you implement multiple inheritance in C#?
- What is the difference between a method and a property in C#?
- How does the 'override' keyword work in C#?
- Can you explain the concept of 'early binding' and 'late binding' in C#?
- What is the role of an abstract class in C# and how is it different from an interface?
- Can you give an example of how to use the 'base' keyword in C#?
5 C# interview questions and answers related to technical definitions
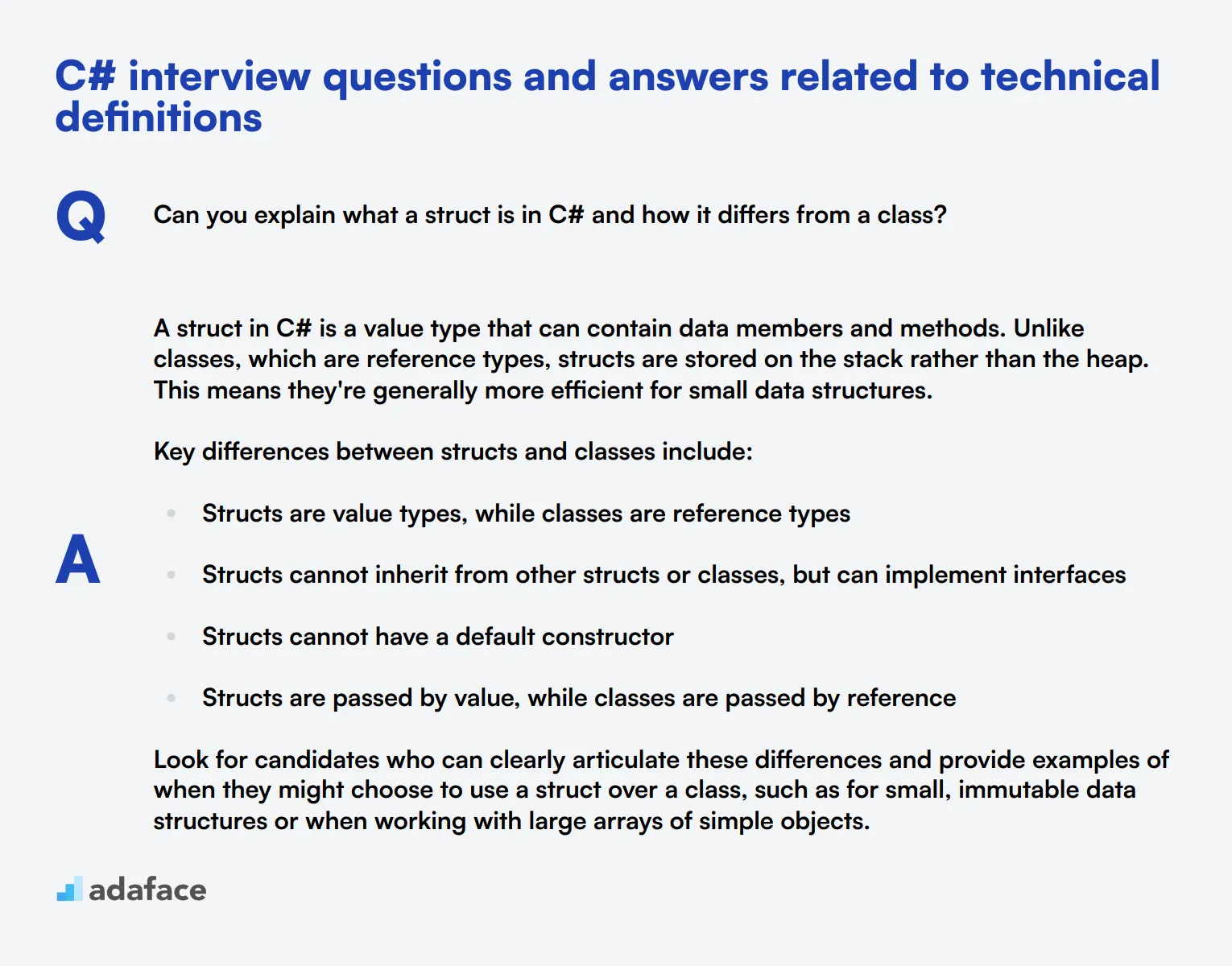
To gauge a candidate's understanding of C# fundamentals and their ability to articulate technical concepts, consider using these interview questions about technical definitions. These questions are designed to help you assess a candidate's theoretical knowledge and their potential fit for C# developer roles. Remember, the goal is not just to hear textbook definitions, but to understand how well the candidate can explain complex ideas in simple terms.
1. Can you explain what a struct is in C# and how it differs from a class?
A struct in C# is a value type that can contain data members and methods. Unlike classes, which are reference types, structs are stored on the stack rather than the heap. This means they're generally more efficient for small data structures.
Key differences between structs and classes include:
- Structs are value types, while classes are reference types
- Structs cannot inherit from other structs or classes, but can implement interfaces
- Structs cannot have a default constructor
- Structs are passed by value, while classes are passed by reference
Look for candidates who can clearly articulate these differences and provide examples of when they might choose to use a struct over a class, such as for small, immutable data structures or when working with large arrays of simple objects.
2. What is the difference between 'break' and 'continue' statements in C#?
The 'break' and 'continue' statements in C# are used to control the flow of loops, but they serve different purposes:
- The 'break' statement is used to exit a loop immediately, skipping any remaining iterations.
- The 'continue' statement skips the rest of the current iteration and moves to the next iteration of the loop.
A strong candidate should be able to provide examples of when each would be used. For instance, 'break' might be used when searching an array and finding a desired element, while 'continue' could be used to skip processing certain elements that don't meet specific criteria. Listen for explanations that demonstrate a clear understanding of loop control and efficiency in software development.
3. Can you explain what a generic type is in C# and provide an example of when you might use one?
A generic type in C# is a class, structure, interface, or method that can work with different data types while providing type safety. Generics allow you to write flexible, reusable code that can operate on objects of various types without sacrificing performance or type safety.
An example of when you might use a generic type is when creating a collection class that needs to work with different data types. For instance, a List
Look for candidates who can explain the benefits of generics, such as increased code reusability, type safety, and performance. They should also be able to provide examples of built-in generic types (like List
4. What is the purpose of the 'sealed' keyword in C#?
The 'sealed' keyword in C# is used to prevent inheritance of a class or to prevent overriding of a method or property. When applied to a class, it means that the class cannot be used as a base class for any other class. When applied to a method or property, it prevents derived classes from overriding that specific member.
The main purposes of using the 'sealed' keyword are:
- To improve performance, as the compiler can make certain optimizations
- To prevent unintended or unauthorized extension of a class or method
- To ensure that the behavior of a class or method remains consistent across all uses
A strong candidate should be able to discuss scenarios where using 'sealed' is beneficial, such as in security-sensitive code or when designing a class that shouldn't be inherited from. They might also mention potential drawbacks, like reduced flexibility in future code extensions.
5. Can you explain what an indexer is in C# and when you might use one?
An indexer in C# is a special type of property that allows a class or struct to be accessed like an array. It provides a way to access elements of an object using array-like syntax, even when the object doesn't explicitly store its data in an array.
Indexers are useful when:
- You want to provide array-like access to a class that encapsulates a list or collection
- You're creating a class that represents a logical collection or a database-like entity
- You want to provide easy, intuitive access to internal data structures
Look for candidates who can provide a simple example of an indexer implementation and explain its syntax. They should also be able to discuss the benefits of using indexers, such as providing a more natural and intuitive interface for certain types of objects. Strong candidates might also mention that indexers can be overloaded to accept different types or numbers of parameters.
Which C# skills should you evaluate during the interview phase?
While it's impossible to gauge every aspect of a candidate's capabilities in a single interview, focusing on key skills can significantly streamline the evaluation process. For C# developers, certain core skills are critical to assess, ensuring they align with the job's technical demands.
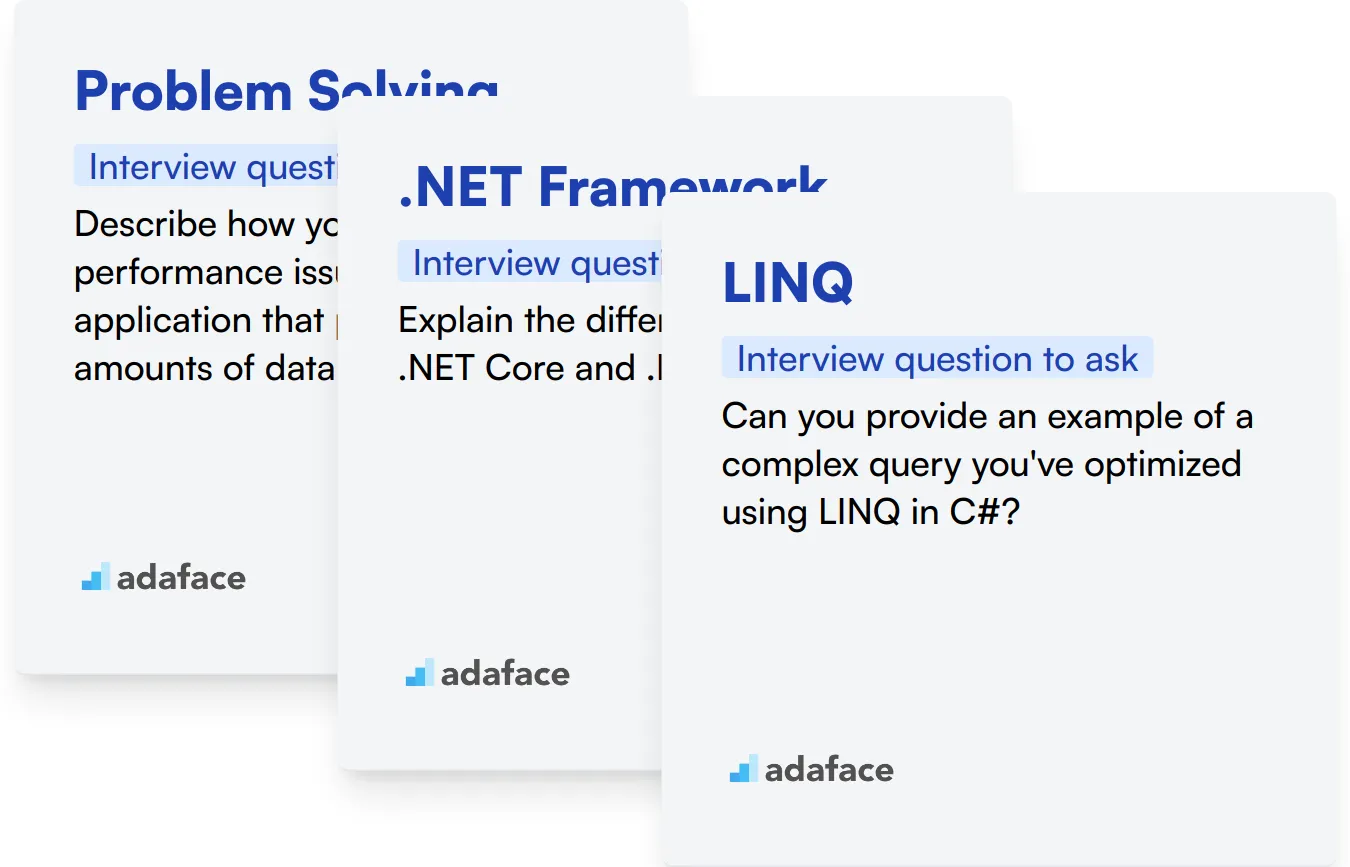
Problem Solving
Problem-solving skills are essential for C# developers as they enable them to effectively tackle bugs, design solutions, and optimize code. A developer's ability to think logically and solve problems directly affects their efficiency in writing clean, effective C# code.
To assess problem-solving skills, consider using a targeted assessment test that includes relevant MCQs. This approach helps filter candidates before the interview stage. For C# related problem-solving skills, you can use the C# Online Test.
For a more direct assessment during the interview, ask questions that require candidates to demonstrate their problem-solving approach. Here's an example:
Describe how you would solve a performance issue in a C# application that processes large amounts of data.
Look for detailed explanations that include specific methods or tools they would use, such as profiling tools or specific C# features like async and await. The clarity of their process and the practicality of their solutions are key indicators of strong problem-solving skills.
.NET Framework
Understanding the .NET framework is fundamental for any C# developer, as it provides the necessary runtime and library support for all C# applications. This knowledge is critical for efficiently building and deploying robust applications.
To accurately gauge a candidate's proficiency with the .NET framework, consider incorporating an MCQ test into your screening process. For a comprehensive assessment, the .NET Online Test is an excellent option.
During the interview, it's also useful to ask specific questions about the .NET framework to understand their practical experience. Consider the following question:
Explain the difference between .NET Core and .NET Framework.
The candidate's answer should highlight understanding of the architectures and appropriate use cases for each version, demonstrating their in-depth knowledge of the platform's ecosystem.
LINQ
LINQ (Language Integrated Query) is a powerful feature in C# that allows developers to handle data in a more readable and concise manner. Proficiency in LINQ is indicative of a developer's ability to write efficient, maintainable code.
To pre-assess this skill, consider using a LINQ-specific assessment composed of MCQs. The LINQ Online Test available in our library is tailored for such evaluations.
To dive deeper into their LINQ skills during the interview, ask the following question:
Can you provide an example of a complex query you've optimized using LINQ in C#?
Evaluate the complexity of the example provided and how effectively the candidate uses LINQ to improve data handling and performance. Effective use of LINQ can significantly reduce data processing times and complexity.
Optimizing Your C# Interview Strategy: Three Essential Tips
Before you start implementing the insights from this post, let's explore some strategies to enhance your C# interview techniques effectively.
1. Incorporate C# Skills Tests Early in the Process
Integrating skills assessments early in the candidate evaluation process can significantly streamline your hiring. By identifying candidates who possess the necessary skills before the interview, you ensure that your shortlisted candidates are not only qualified but are likely to perform well in their roles.
Consider leveraging C# specific assessments like the C# Online Test and C# .NET SQL Test to evaluate the technical competencies of your candidates effectively. These tests are tailored to verify the programming prowess and problem-solving abilities crucial for roles involving C#.
Employing these tests efficiently filters out underqualified applicants and enhances the quality of your interview discussions. This approach allows you to focus on more in-depth topics during the interview, ensuring a thorough evaluation of each candidate.
2. Strategically Select and Compile Interview Questions
With limited time during interviews, selecting the right questions is key to evaluating the critical skills needed for the role. It's important to balance the number of questions to cover breadth without sacrificing the depth of each query.
In addition to technical questions, consider integrating queries that assess soft skills and cultural fit, which are just as indicative of a candidate's potential success. Explore related sets of questions, such as ASP.NET MVC or Entity Framework related questions, which complement C# evaluations.
This method ensures a holistic view of the candidate's capabilities and fit with your team, allowing for a more precise assessment of their potential impact in the role.
3. Emphasize the Importance of Follow-Up Questions
Relying solely on initial answers can be misleading; follow-up questions can unearth deeper insights and genuine skill levels. They help clarify doubts and reveal how candidates handle spontaneous problem-solving and in-depth discussions.
For example, if a candidate mentions experience with 'LINQ queries' during the interview, a good follow-up question might be, 'Can you describe a complex query you optimized using LINQ, and what the performance gains were?' This not only checks for technical knowledge but also insight into their problem-solving approach.
Utilize C# interview questions and skills tests to hire talented developers
If you are looking to hire someone with C# skills, ensuring that candidates possess the required expertise is critical. The most reliable way to evaluate these skills is to use specialized skill tests. Check out our assessments like C# Online Test and C# .NET SQL Test.
Once you use these tests, you can shortlist the best applicants and invite them for interviews. To proceed, you can sign up here or explore our full range of assessments on our test library.
C# .NET Junior Developer Test
Download C# interview questions template in multiple formats
C# Interview Questions FAQs
Ask a mix of questions covering basics, OOP concepts, technical definitions, and experience-level specific topics to gauge the candidate's overall C# knowledge and skills.
Use junior-level questions for entry-level positions, intermediate questions for mid-tier roles, and advanced questions for senior developer positions to match the job requirements.
Yes, including practical coding exercises can help assess a candidate's problem-solving skills and their ability to apply C# concepts in real-world scenarios.
Look for clear explanations, correct terminology, and the ability to provide examples. Consider their problem-solving approach and how they apply C# concepts to different scenarios.
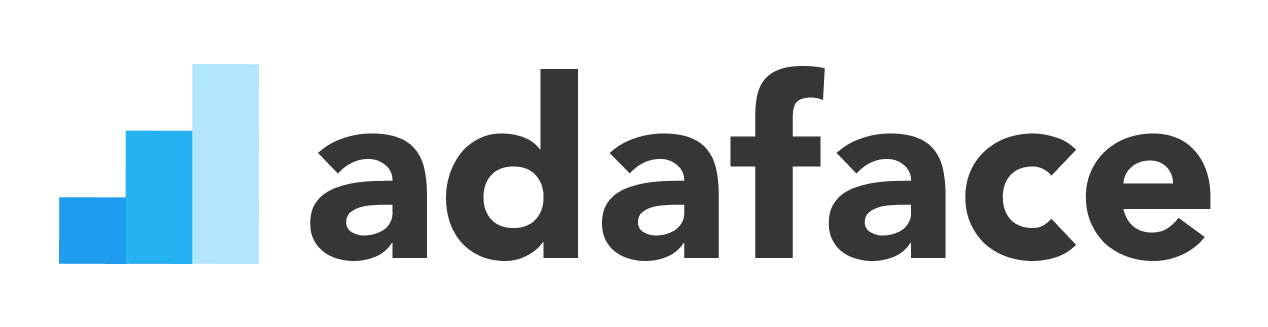
40 min skill tests.
No trick questions.
Accurate shortlisting.
We make it easy for you to find the best candidates in your pipeline with a 40 min skills test.
Try for freeRelated posts
Free resources
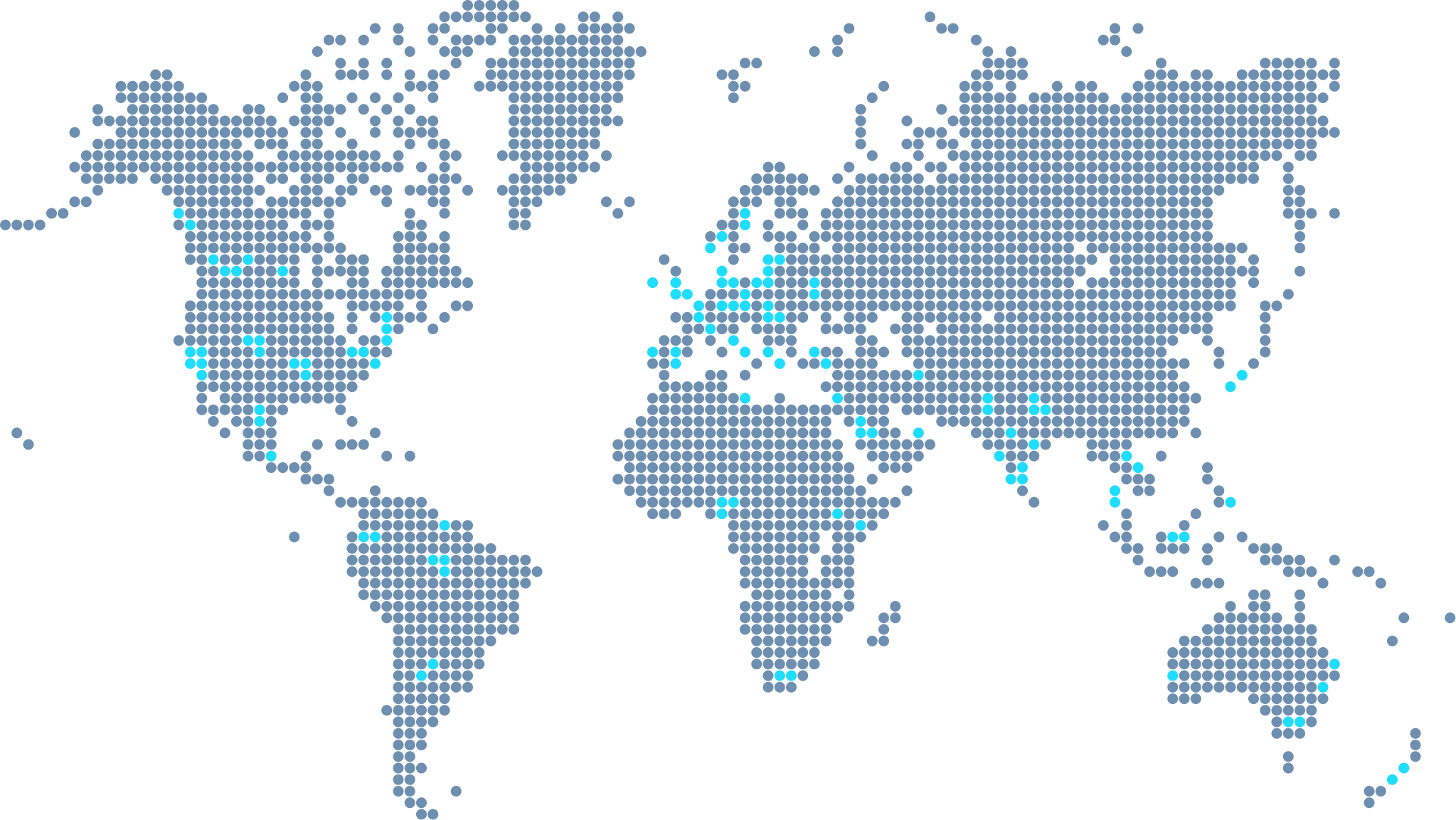
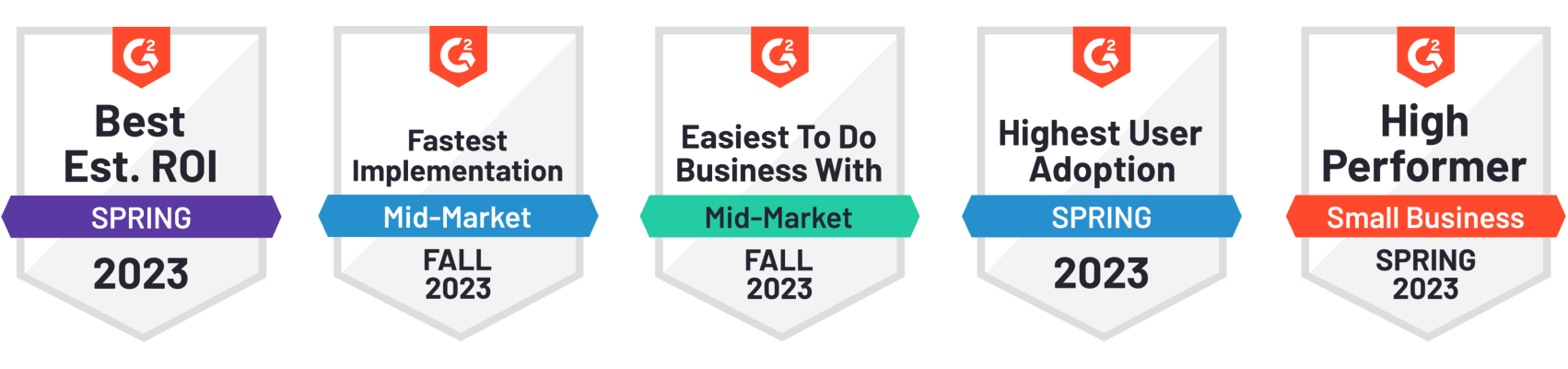