Interviewing can be a daunting task, especially when it comes to assessing technical skills like C programming. Asking the right questions can help you identify candidates who possess the necessary skills and problem-solving abilities.
In this blog post, we will provide you with a comprehensive set of questions tailored to different levels of expertise in C programming. Whether you are interviewing junior developers or mid-tier professionals, this guide will help you evaluate their capabilities effectively.
Using this list, you can streamline your interview process and make informed hiring decisions. For a more in-depth evaluation, consider using our C Programming online test before conducting interviews.
Table of contents
10 common C Programming interview questions to ask your applicants
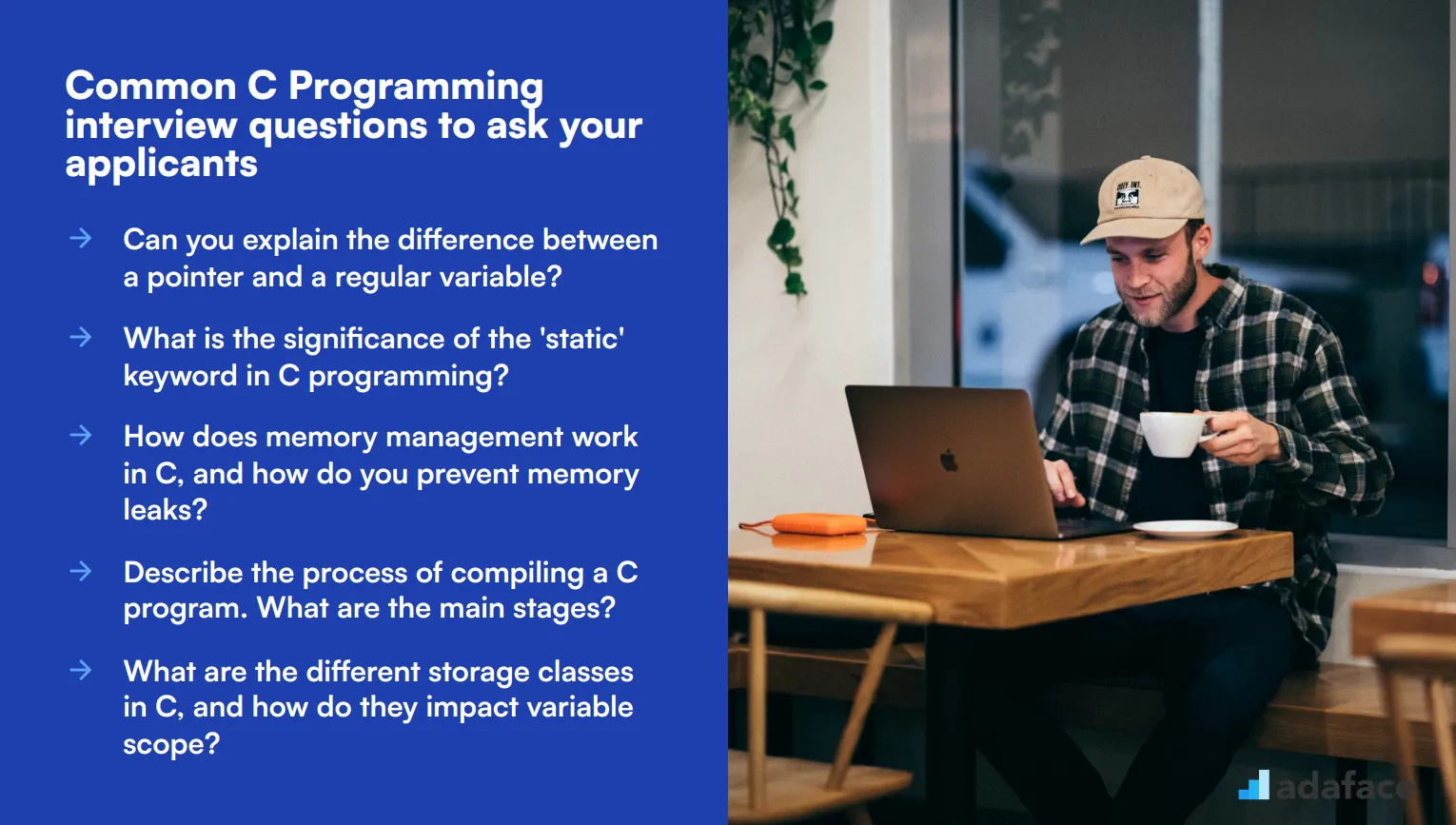
To identify whether your candidates possess essential C programming skills, consider incorporating these interview questions into your hiring process. They can help you gauge both basic knowledge and practical application, ensuring that you find the right fit for your team. For more insights, check out our C developer job description.
- Can you explain the difference between a pointer and a regular variable?
- What is the significance of the 'static' keyword in C programming?
- How does memory management work in C, and how do you prevent memory leaks?
- Describe the process of compiling a C program. What are the main stages?
- What are the different storage classes in C, and how do they impact variable scope?
- Can you illustrate how to use a structure in C and why it is useful?
- How do you handle errors in C? Can you provide an example of error handling?
- What is the difference between 'malloc' and 'calloc' in C?
- Explain the concept of recursion with a simple example in C.
- How do you create a multi-file project in C, and what are the benefits?
8 C Programming interview questions and answers to evaluate junior developers
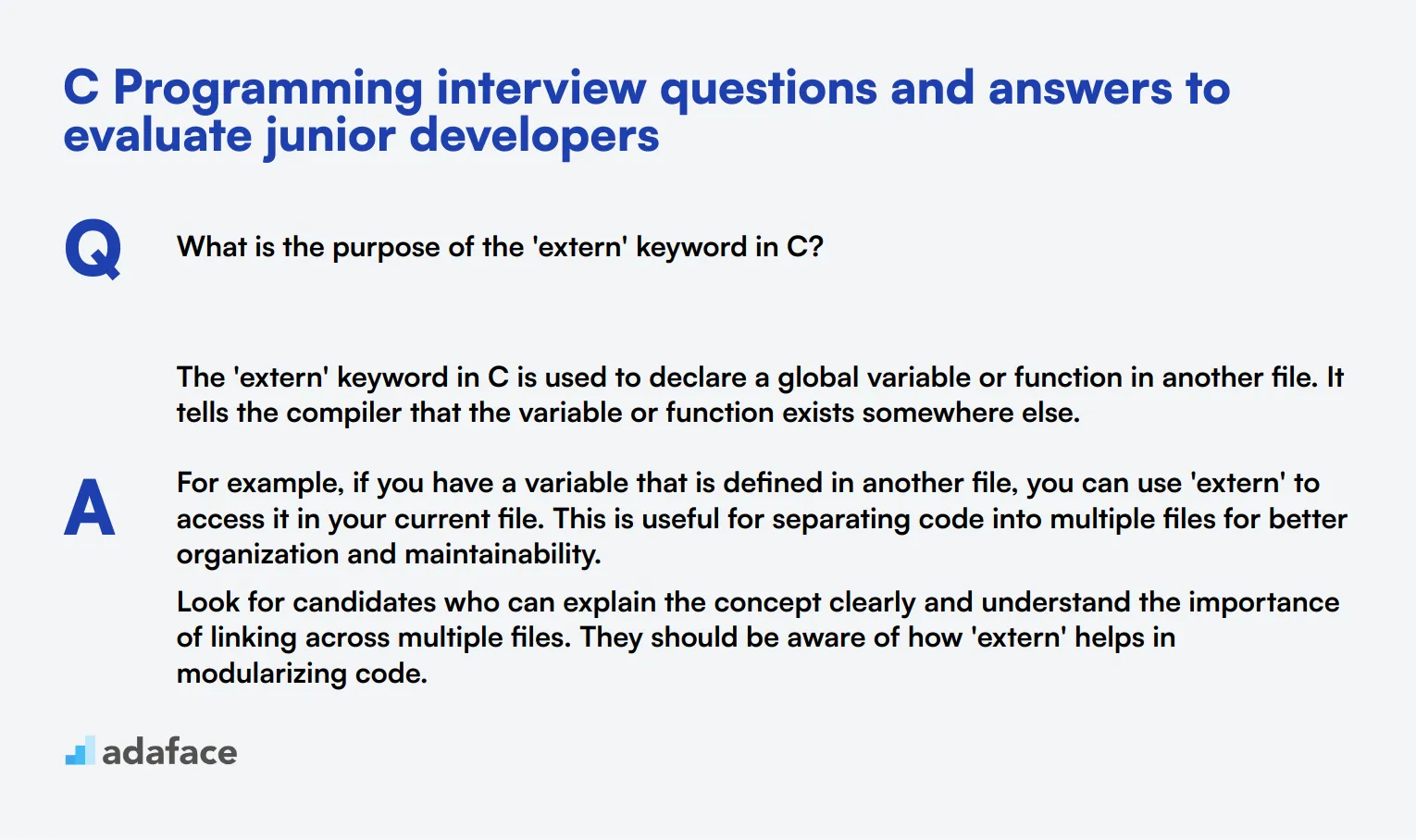
To evaluate junior developers' understanding of C programming, use these questions during interviews. They help gauge core competencies and problem-solving abilities without getting overly technical.
1. What is the purpose of the 'extern' keyword in C?
The 'extern' keyword in C is used to declare a global variable or function in another file. It tells the compiler that the variable or function exists somewhere else.
For example, if you have a variable that is defined in another file, you can use 'extern' to access it in your current file. This is useful for separating code into multiple files for better organization and maintainability.
Look for candidates who can explain the concept clearly and understand the importance of linking across multiple files. They should be aware of how 'extern' helps in modularizing code.
2. How does the 'const' keyword affect a variable in C?
The 'const' keyword in C is used to declare variables whose values cannot be changed after initialization. It makes the variable read-only.
For instance, if you declare a variable as 'const int x = 10;', any attempt to modify 'x' later in the code will result in a compilation error.
An ideal candidate should demonstrate an understanding of how 'const' helps in creating safer and more predictable code by preventing accidental changes to critical variables.
3. Can you explain what a 'dangling pointer' is?
A 'dangling pointer' is a pointer that points to a memory location that has already been freed or deallocated. Accessing a dangling pointer can lead to undefined behavior, crashes, or data corruption.
This usually occurs when memory is dynamically allocated and then freed, but the pointer is not set to NULL afterwards. Using the pointer after freeing the memory can be problematic.
Look for candidates who can explain the concept clearly and discuss preventive measures, such as setting pointers to NULL after freeing memory to avoid these issues.
4. What is the difference between 'break' and 'continue' statements in C?
The 'break' statement in C is used to exit from a loop or switch statement immediately. It terminates the loop or switch and transfers control to the statement following the loop or switch.
On the other hand, the 'continue' statement skips the remaining code inside the loop for the current iteration and moves to the next iteration of the loop.
Candidates should be able to clearly differentiate between 'break' and 'continue' and provide examples of scenarios where each would be appropriately used. Look for a clear understanding of loop control mechanisms.
5. How do you define a macro in C and what are its uses?
A macro in C is defined using the '#define' directive. Macros are used to create symbolic constants or to define small functions that are expanded inline, improving code readability and maintainability.
For example, you can define a macro for a constant value: #define PI 3.14
. You can also define a macro for a function-like operation: #define SQUARE(x) ((x) * (x))
.
Look for candidates who understand the benefits and potential pitfalls of using macros, such as the lack of type checking and possible side effects due to macro expansion.
6. What is the role of the 'volatile' keyword in C?
The 'volatile' keyword in C is used to inform the compiler that a variable's value may change at any time, without any action being taken by the code the compiler finds nearby. This prevents the compiler from optimizing the code in ways that assume the value cannot change unexpectedly.
This is particularly useful for variables that are accessed by multiple threads or hardware devices, such as memory-mapped I/O registers.
Candidates should demonstrate an understanding of when and why to use 'volatile', such as in embedded systems or multithreading scenarios, to ensure correct program behavior.
7. How do you perform file operations in C?
File operations in C are performed using the standard library functions such as fopen
, fclose
, fread
, fwrite
, fgets
, and fprintf
. These functions allow you to open, read, write, and close files.
For example, fopen
is used to open a file, specifying the filename and the mode (read, write, append, etc.). After performing the necessary operations, fclose
is used to close the file.
Look for candidates who can explain the basic file operations and understand the importance of proper file handling, such as checking for successful file opening and closing files to avoid resource leaks.
8. Can you explain what a segmentation fault is and how you might troubleshoot it?
A segmentation fault is an error that occurs when a program tries to access a memory location that it's not allowed to access. This can happen due to various reasons such as dereferencing a null or invalid pointer, buffer overflows, or accessing memory that has been freed.
Troubleshooting a segmentation fault typically involves using debugging tools like gdb
to identify where the fault occurs. Checking pointer validity, ensuring proper memory allocation and deallocation, and using tools like Valgrind for memory analysis can help identify the root cause.
Candidates should demonstrate an understanding of common causes of segmentation faults and discuss practical debugging techniques. They should also emphasize the importance of careful memory management practices.
15 intermediate C Programming interview questions and answers to ask mid-tier developers.
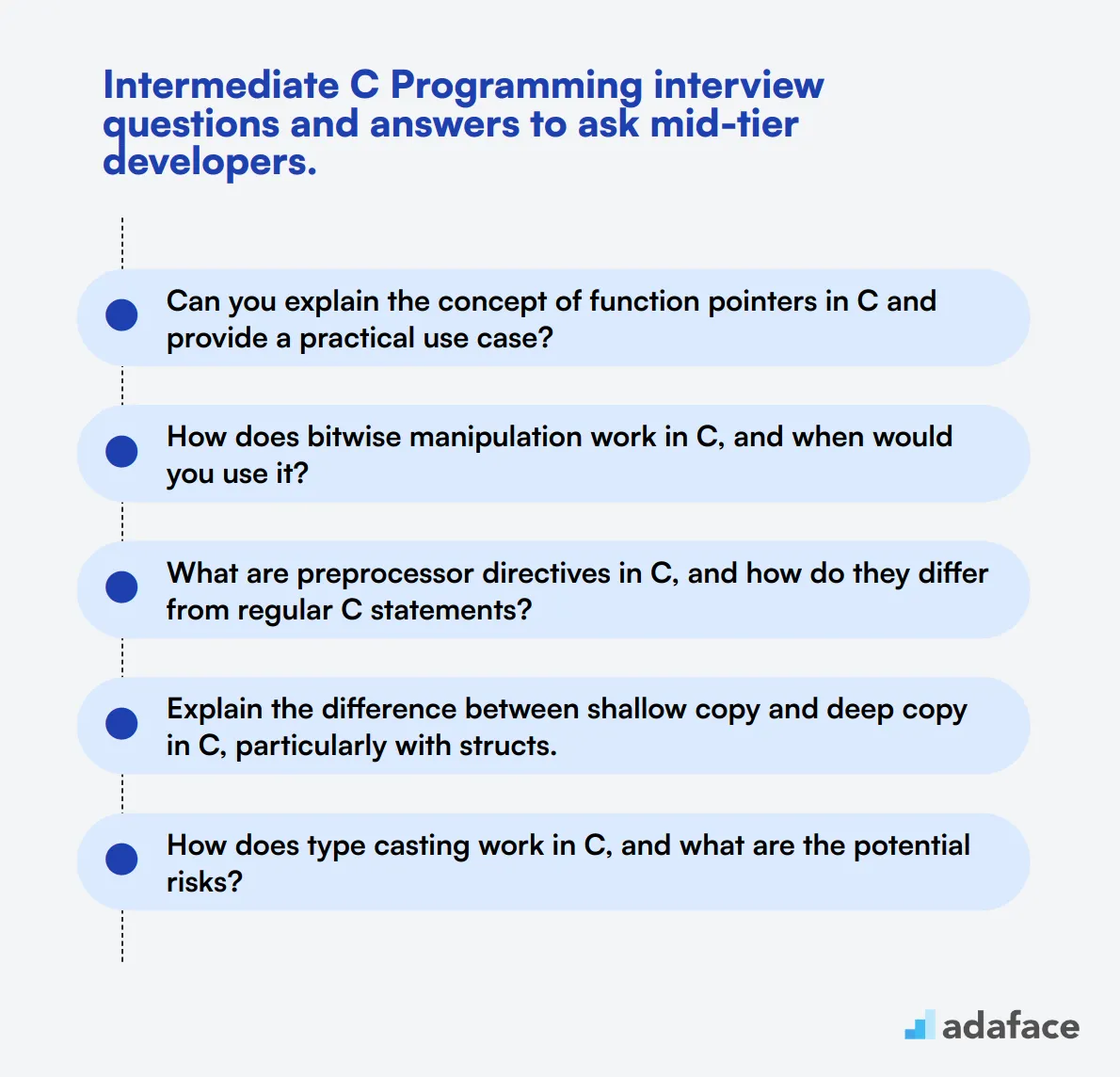
To assess the proficiency of mid-tier C developers, use these 15 intermediate C programming interview questions. These questions are designed to evaluate a candidate's deeper understanding of C concepts and their ability to apply them in real-world scenarios.
- Can you explain the concept of function pointers in C and provide a practical use case?
- How does bitwise manipulation work in C, and when would you use it?
- What are preprocessor directives in C, and how do they differ from regular C statements?
- Explain the difference between shallow copy and deep copy in C, particularly with structs.
- How does type casting work in C, and what are the potential risks?
- What is the purpose of the 'union' keyword in C, and how does it differ from a struct?
- Can you describe how to implement a linked list in C?
- What is the significance of the 'restrict' keyword in C99?
- How do you handle command-line arguments in a C program?
- Explain the concept of function inlining in C and its potential benefits.
- What are variadic functions in C, and how are they implemented?
- How do you use function pointers to implement callbacks in C?
- What is the purpose of the 'volatile' keyword in C, especially in embedded systems?
- Can you explain how to create and use a circular buffer in C?
- What are the differences between stack and heap memory allocation in C?
7 C Programming interview questions and answers related to memory management
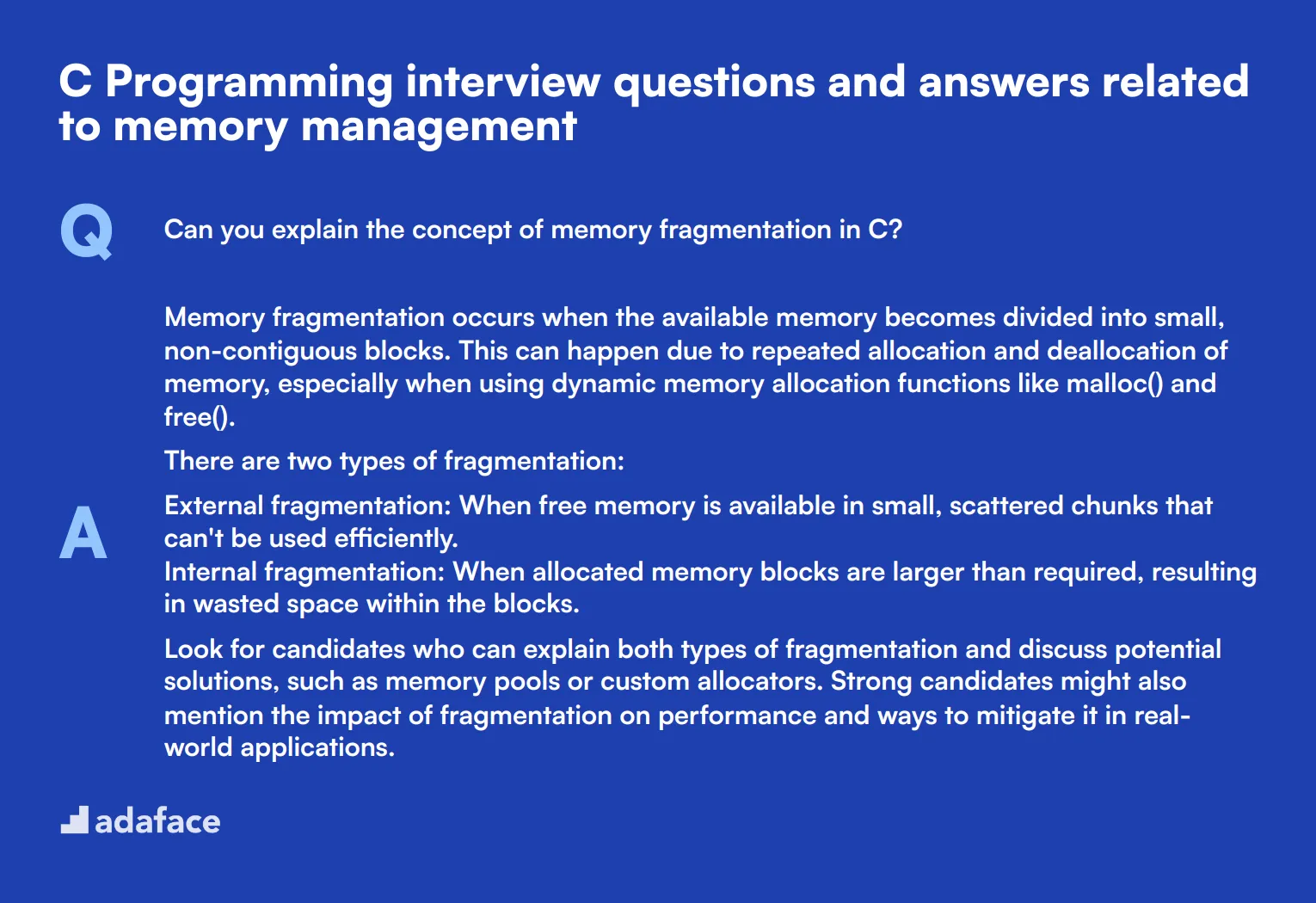
When it comes to hiring C programmers, memory management skills are crucial. These 7 interview questions will help you assess candidates' understanding of this fundamental aspect of C programming. Use them to gauge how well potential hires can handle the nitty-gritty of memory allocation and deallocation, ensuring you find the right software developer for your team.
1. Can you explain the concept of memory fragmentation in C?
Memory fragmentation occurs when the available memory becomes divided into small, non-contiguous blocks. This can happen due to repeated allocation and deallocation of memory, especially when using dynamic memory allocation functions like malloc() and free().
There are two types of fragmentation:
- External fragmentation: When free memory is available in small, scattered chunks that can't be used efficiently.
- Internal fragmentation: When allocated memory blocks are larger than required, resulting in wasted space within the blocks.
Look for candidates who can explain both types of fragmentation and discuss potential solutions, such as memory pools or custom allocators. Strong candidates might also mention the impact of fragmentation on performance and ways to mitigate it in real-world applications.
2. How would you detect a memory leak in a C program?
Detecting memory leaks in C programs involves several techniques:
- Use memory profiling tools like Valgrind or AddressSanitizer
- Implement custom memory allocation wrappers to track allocations
- Manually review code for proper deallocation of resources
- Use static analysis tools to identify potential leak points
Candidates should emphasize the importance of systematic testing and the use of specialized tools. They might also mention the significance of good coding practices, such as consistent use of free() for every malloc() call.
Pay attention to candidates who discuss the challenges of detecting memory leaks in complex programs and the importance of ongoing monitoring in production environments. Strong answers might also touch on strategies for preventing memory leaks in the first place.
3. What's the difference between stack and heap memory allocation in C?
Stack memory allocation:
- Automatic and managed by the compiler
- Faster allocation and deallocation
- Limited in size (depends on the system)
- Used for local variables and function calls
Heap memory allocation:
- Manual allocation and deallocation by the programmer
- Slower than stack allocation
- Larger available memory space
- Used for dynamic memory allocation (malloc, calloc, etc.)
Look for candidates who can clearly differentiate between the two and explain their appropriate use cases. Strong answers might include discussions on memory layout, potential issues like stack overflow or heap fragmentation, and best practices for efficient memory management in C programming.
4. How does the C memory model handle multithreading?
The C memory model doesn't inherently provide built-in support for multithreading. However, it forms the foundation upon which threading libraries like POSIX threads (pthreads) are built. Key points to consider:
- Each thread typically has its own stack
- Heap memory is shared between threads
- Race conditions can occur when multiple threads access shared resources
- Synchronization primitives (mutexes, semaphores) are necessary for thread-safe operations
Candidates should demonstrate an understanding of the challenges posed by multithreading in C, such as data races and the need for proper synchronization. They might discuss the use of volatile for shared variables and the importance of atomic operations.
Strong answers could include mentions of memory ordering, the happens-before relationship, and how modern C standards (C11 and later) introduced better support for concurrency with features like the atomic library.
5. What strategies would you use to minimize memory usage in a C program?
To minimize memory usage in C programs, several strategies can be employed:
- Use appropriate data types (e.g., short instead of int when possible)
- Implement efficient algorithms and data structures
- Reuse memory instead of frequent allocation/deallocation
- Use memory pools for frequent small allocations
- Employ bit fields for compact storage of boolean flags
- Free unused memory promptly
- Consider memory-mapped files for large datasets
Look for candidates who can provide a range of techniques and explain their trade-offs. They should demonstrate an understanding of both micro-optimization (like using smaller data types) and macro-level strategies (like algorithm choice).
Strong candidates might also discuss the importance of profiling and measurement in identifying memory bottlenecks, as well as the balance between memory usage and performance considerations.
6. Explain the concept of memory alignment in C and why it matters.
Memory alignment refers to the way data is arranged and accessed in memory. In C, different data types have different alignment requirements, typically based on their size. Proper alignment ensures efficient memory access and can significantly impact performance.
Key points about memory alignment:
- Aligned data can be accessed faster by the CPU
- Misaligned access can cause performance penalties or even crashes on some architectures
- Structures may include padding to ensure proper alignment of members
- The 'sizeof' operator takes alignment into account
Look for candidates who understand the performance implications of alignment and can discuss how it affects structure packing and memory layout. Strong answers might include mentions of alignment specifiers, the impact of alignment on cache performance, and strategies for dealing with alignment issues in cross-platform code.
7. How would you implement a custom memory allocator in C?
Implementing a custom memory allocator involves several steps:
- Request a large block of memory from the operating system (e.g., using sbrk() or mmap())
- Divide this block into smaller chunks for allocation
- Implement functions for allocation (similar to malloc()) and deallocation (similar to free())
- Maintain metadata about free and allocated blocks
- Implement strategies for coalescing free blocks and handling fragmentation
Candidates should discuss the trade-offs involved in different allocation strategies (e.g., first-fit, best-fit, segregated lists) and how to handle issues like fragmentation and thread-safety.
Strong answers might include discussions on performance considerations, such as minimizing system calls and optimizing for common allocation sizes. Look for candidates who can explain why custom allocators might be beneficial in certain scenarios and how they would benchmark and profile their implementation.
12 C Programming interview questions about data structures
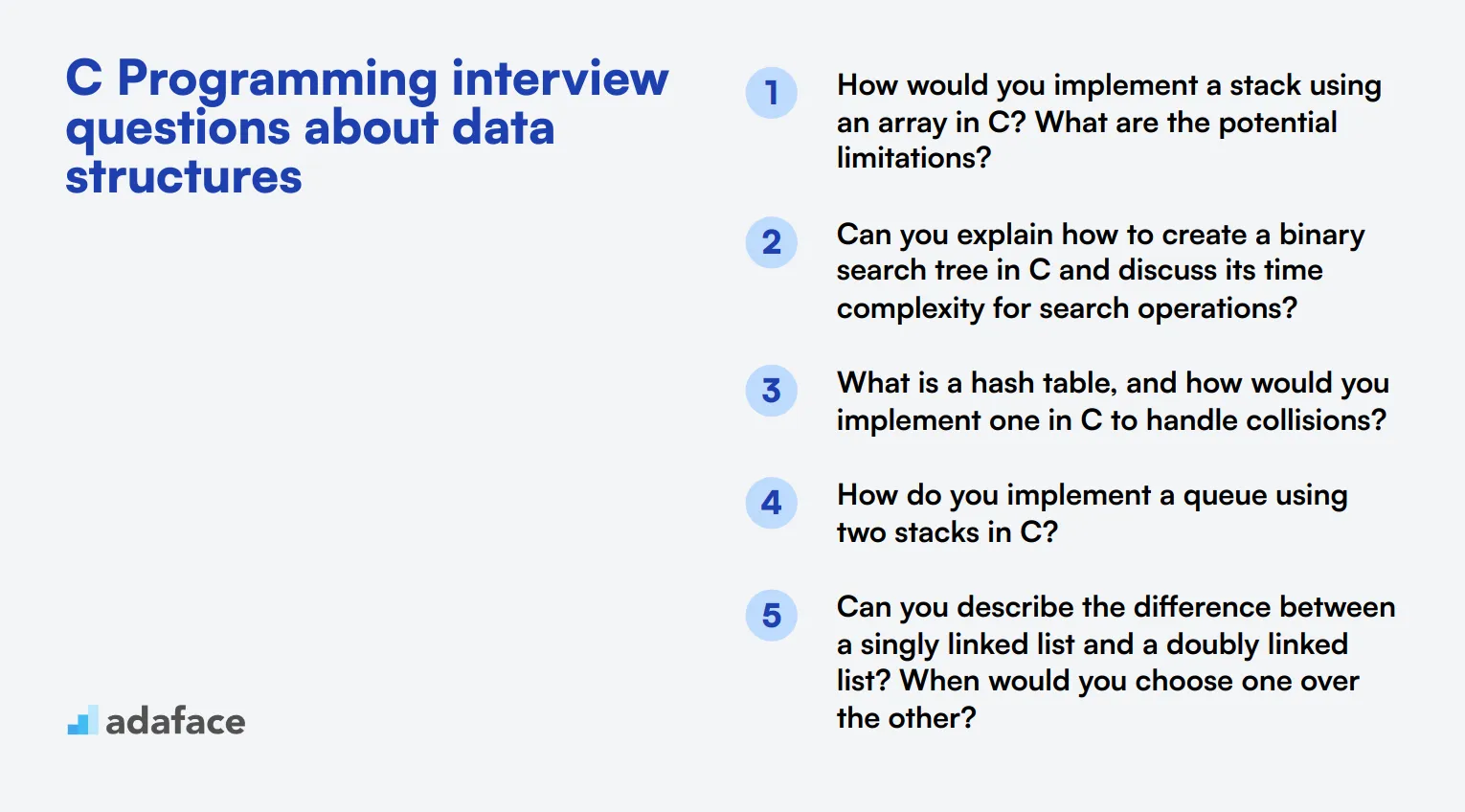
To assess a candidate's proficiency in C programming data structures, consider using these 12 interview questions. They are designed to evaluate a software developer's understanding of fundamental data structures and their implementation in C. Use these questions to gauge the depth of knowledge and problem-solving skills of potential hires.
- How would you implement a stack using an array in C? What are the potential limitations?
- Can you explain how to create a binary search tree in C and discuss its time complexity for search operations?
- What is a hash table, and how would you implement one in C to handle collisions?
- How do you implement a queue using two stacks in C?
- Can you describe the difference between a singly linked list and a doubly linked list? When would you choose one over the other?
- How would you implement a priority queue in C? What data structure would you use?
- Explain how you would use a trie (prefix tree) in C for efficient string searching.
- Can you describe how to implement a graph using an adjacency list in C?
- How would you design a circular buffer in C, and what are its applications?
- Can you explain the concept of a heap data structure and how to implement a min-heap in C?
- How would you implement a sparse matrix in C to efficiently store and operate on mostly zero elements?
- Can you describe how to implement a skip list in C and its advantages over other data structures?
Which C Programming skills should you evaluate during the interview phase?
Assessing every aspect of a candidate's capabilities in a single interview is virtually impossible. However, for C Programming, there are core skills that significantly indicate a candidate's proficiency and potential to contribute effectively. Here's a rundown of the essential skills you should evaluate during the interview phase.
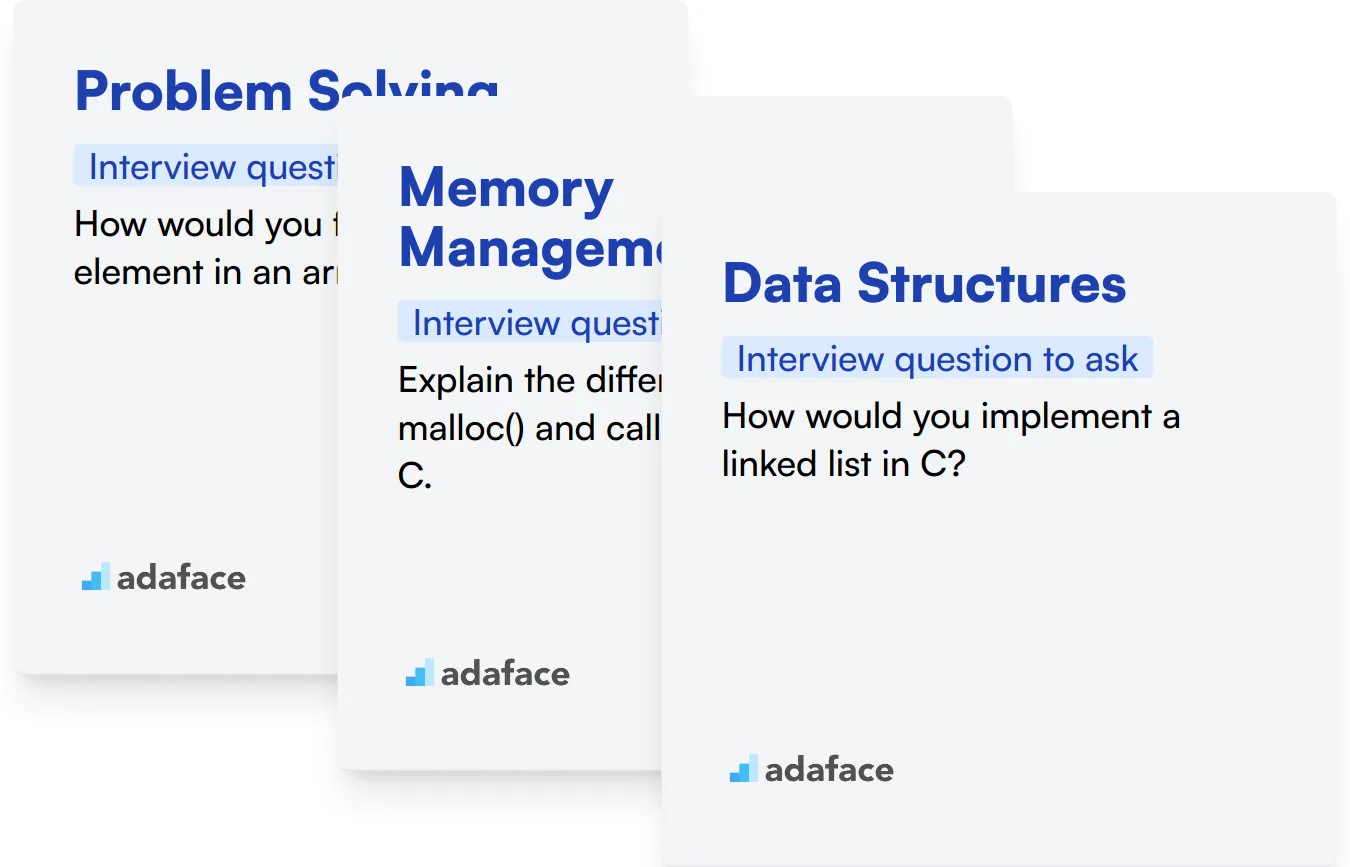
Problem Solving
Problem-solving is at the heart of C Programming. It involves understanding complex issues, breaking them down into manageable parts, and crafting efficient solutions. A strong problem-solver can handle real-world challenges effectively.
An assessment test with relevant MCQs can help filter out candidates with excellent problem-solving skills. For example, you can use our C Programming Test to gauge their abilities.
To further assess this skill, ask targeted interview questions that require the candidate to solve a problem on the spot.
How would you find the largest element in an array using C?
When asking this question, look for a clear understanding of array manipulation, loop structures, and conditionals. The candidate should efficiently iterate through the array and compare elements to find the largest one.
Memory Management
Memory management is crucial in C Programming due to its low-level operations. Effective memory usage prevents leaks and errors, ensuring programs run smoothly and efficiently.
You can use assessment tests with MCQs related to memory management. Consider our C Programming Test which includes questions on dynamic memory allocation and deallocation.
Ask specific interview questions to judge the candidate's expertise in handling memory operations.
Explain the difference between malloc() and calloc() functions in C.
Look for an explanation that covers memory allocation differences, initialization behavior, and their appropriate use cases. The candidate should demonstrate a deep understanding of how these functions are utilized in real-world applications.
Data Structures
Understanding data structures is fundamental for any C programmer. It helps in organizing and storing data efficiently, which is critical for performance optimization and effective problem-solving.
Use an assessment test that includes MCQs on data structures to filter candidates. Our Data Structures Test is an excellent tool for this purpose.
You can ask direct questions about their knowledge and implementation of data structures.
How would you implement a linked list in C?
Evaluate their response based on their understanding of pointers, struct usage, and dynamic memory management. The candidate should be able to explain the creation, insertion, deletion, and traversal operations of a linked list.
Essential Tips for Leveraging C Programming Interview Questions
Before you begin applying the knowledge from this guide, here are a few crucial tips to enhance your interviewing process with C Programming questions.
1. Incorporate Skills Tests to Streamline Candidate Evaluation
Prioritize skills testing as a pre-interview filter to efficiently assess candidate abilities in C programming. This approach allows you to gauge technical proficiency before committing to a full interview.
For evaluating C programming skills, consider using Adaface's C Online Test and Embedded C Online Test. These tests provide insight into a candidate's practical and theoretical knowledge, ensuring that only the most competent applicants progress to the interview stage.
By implementing these tests, you can focus your interviews on deeper topics rather than basic programming capabilities. This streamlined process not only saves time but also enhances the effectiveness of your candidate evaluation.
2. Tailor Your Question Set to Maximize Interview Efficiency
Time is limited during interviews, so it's important to select a balanced and relevant set of questions that cover crucial aspects of C programming. This ensures a comprehensive assessment of each candidate's capabilities.
Besides core programming questions, consider incorporating queries from related areas such as data structures or Linux commands, depending on the role. Including questions on soft skills like communication can also provide insights into a candidate's fit for your team's culture.
Creating a concise yet diverse question set allows you to get a holistic view of candidates, helping you make informed hiring decisions.
3. Utilize Follow-up Questions to Uncover Deeper Insights
Using predefined interview questions is just the beginning. To truly understand a candidate's depth and suitability for the role, follow-up questions are key. They help reveal more than just surface-level knowledge.
For example, if a candidate discusses memory management techniques in C, follow up with, 'Can you explain a situation where manual memory management led to efficiency gains in a project?' This allows you to assess practical application skills and problem-solving abilities, critical for the role.
Hire skilled C programmers with targeted interview questions and assessments
Looking to hire someone with C programming skills? Make sure to test their abilities accurately. The most effective way to do this is by using skill tests. Consider using a C online test or data structures online test to evaluate candidates' proficiency.
After using these tests to shortlist the best applicants, you can invite them for interviews. To streamline your hiring process and find top C programming talent, explore our coding tests or sign up to access our full range of assessment tools.
C Online Test
Download C Programming interview questions template in multiple formats
C Programming Interview Questions FAQs
Common questions include those on basic syntax, simple loops, conditionals, and data types in C.
Ask questions related to dynamic memory allocation, pointers, memory leaks, and functions like malloc and free.
You can ask about pointers, structures, linked lists, file handling, and more complex algorithms.
Understanding data structures is crucial for effective problem-solving and optimizing code performance.
Ask about code readability, commenting, error handling, and adherence to coding standards.
Focus on basic programming concepts, simple problem-solving, and understanding of fundamental data structures.
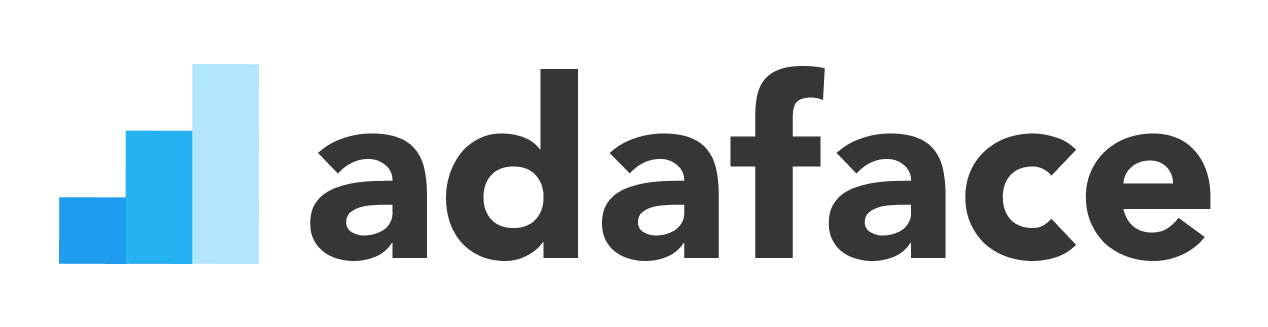
40 min skill tests.
No trick questions.
Accurate shortlisting.
We make it easy for you to find the best candidates in your pipeline with a 40 min skills test.
Try for freeRelated posts
Free resources
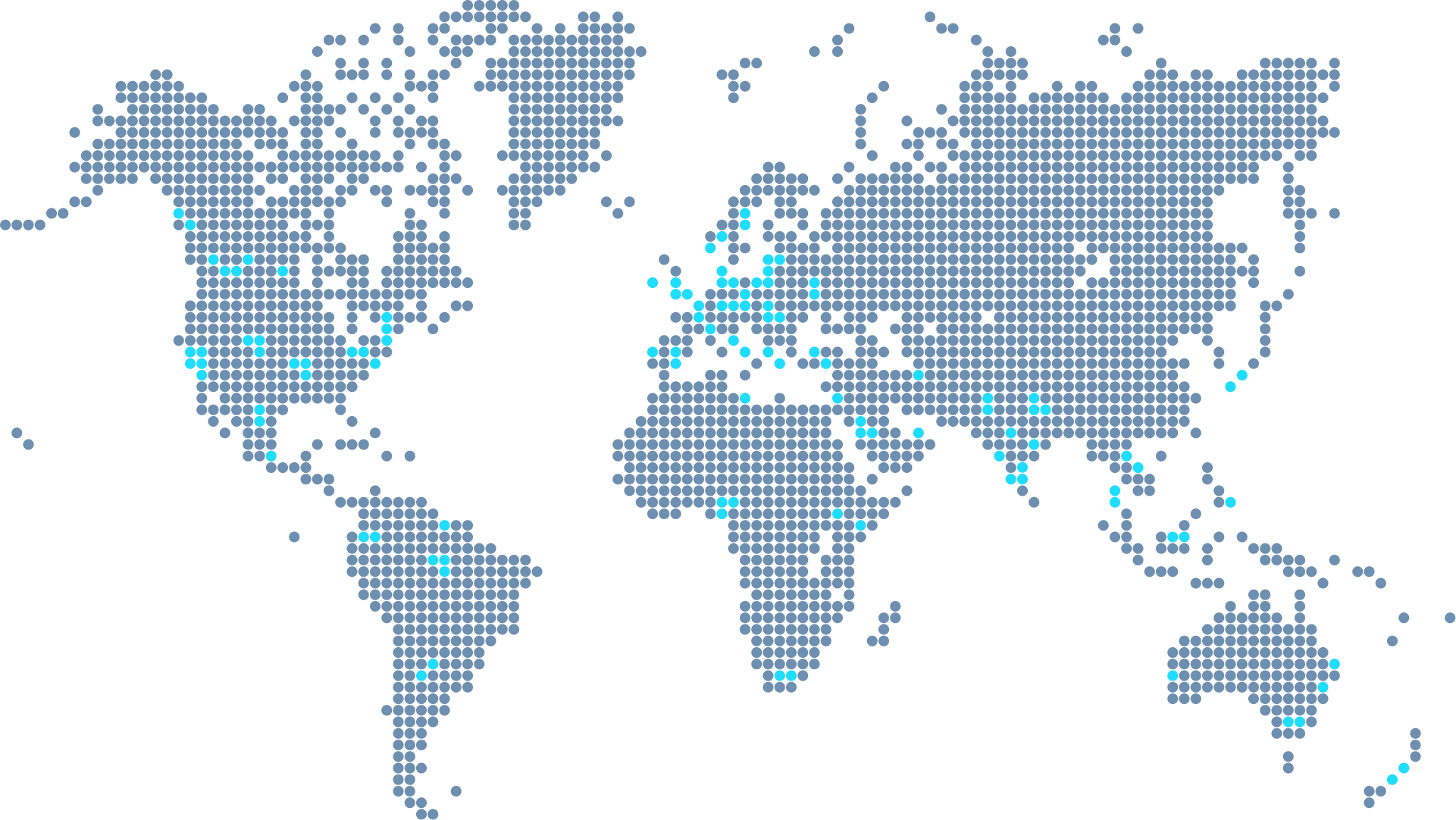
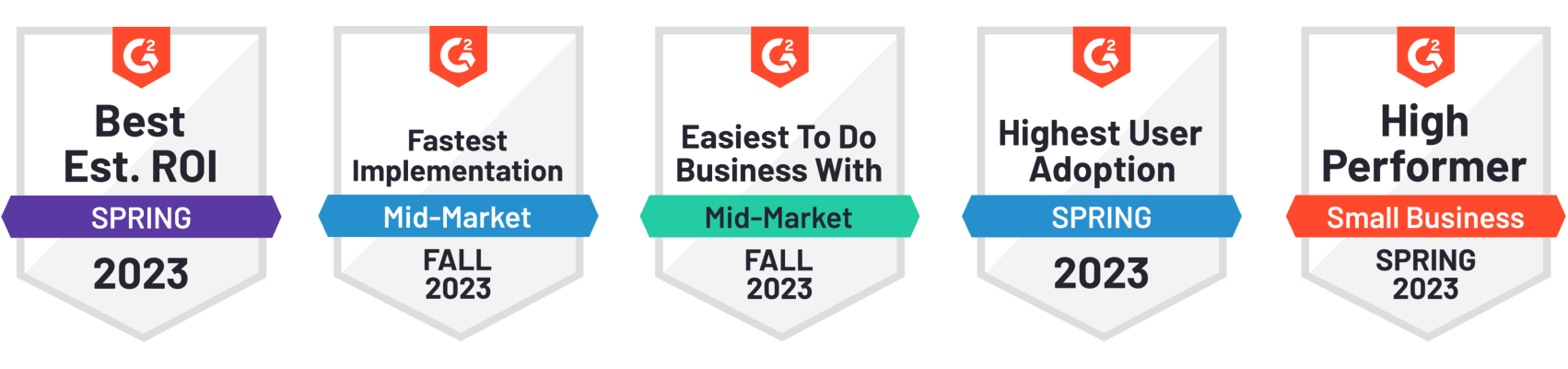