Hiring the right Ajax developers can be a challenge for recruiters and hiring managers. This comprehensive list of Ajax interview questions will help you evaluate candidates' skills and knowledge across different experience levels.
We've compiled 69 Ajax interview questions, ranging from general concepts to advanced topics, including XHR and callback functions. These questions are organized by difficulty level, making it easy to assess junior, mid-tier, and senior developers.
By using these questions, you can effectively gauge candidates' Ajax expertise and make informed hiring decisions. Consider pairing these interview questions with a JavaScript skills test to get a well-rounded view of candidates' abilities.
Table of contents
7 general Ajax interview questions and answers to assess applicants
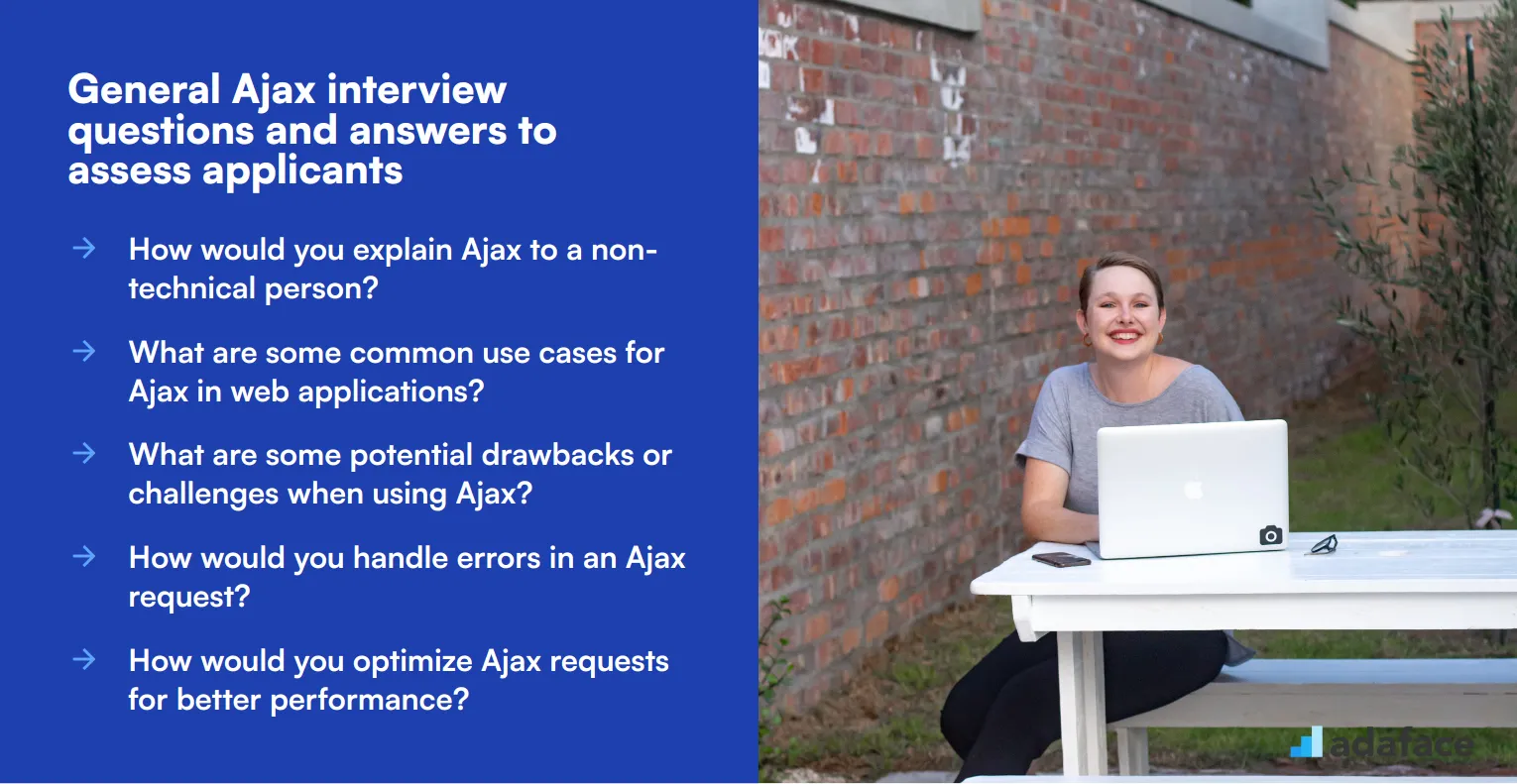
Ready to put your Ajax interview skills to the test? These seven general questions will help you assess candidates' understanding of Ajax fundamentals and best practices. Use this list to gauge applicants' knowledge and problem-solving abilities in a real-world context. Remember, the goal is to spark meaningful discussions, not just hear textbook answers!
1. How would you explain Ajax to a non-technical person?
A strong candidate should be able to simplify Ajax concepts for a layperson. They might compare it to ordering food at a restaurant:
- Ajax is like a waiter who takes your order and brings your food without closing the entire restaurant.
- It allows web pages to update small parts of content without reloading the whole page, making websites feel faster and more responsive.
Look for answers that use relatable analogies and focus on the user experience benefits of Ajax. The best responses will highlight improved interactivity and efficiency without delving into technical jargon.
2. What are some common use cases for Ajax in web applications?
Candidates should demonstrate knowledge of practical Ajax applications. Strong answers might include:
- Form submissions without page reloads
- Live search suggestions as users type
- Infinite scrolling on social media feeds
- Real-time chat applications
- Updating shopping carts without refreshing the page
Look for responses that show an understanding of how Ajax enhances user experience and improves website performance. The best candidates will provide specific examples and explain why Ajax is beneficial in each scenario.
3. What are some potential drawbacks or challenges when using Ajax?
This question assesses a candidate's critical thinking and awareness of Ajax limitations. Good answers might include:
- Browser history and bookmarking issues
- Search engine optimization challenges
- Increased complexity in error handling
- Potential for overuse, leading to performance issues
- Accessibility concerns for screen readers
Strong candidates will not only list drawbacks but also suggest potential solutions or workarounds. This demonstrates a problem-solving approach and a balanced understanding of Ajax technology.
4. How would you handle errors in an Ajax request?
This question evaluates a candidate's error handling strategies. A comprehensive answer might include:
- Implementing try-catch blocks to capture and log errors
- Using the error callback function to handle specific HTTP status codes
- Displaying user-friendly error messages on the frontend
- Implementing retry mechanisms for transient errors
- Logging errors on the server-side for debugging
Look for answers that demonstrate a systematic approach to error handling. The best candidates will discuss both client-side and server-side error management, showing a holistic understanding of Ajax communication.
5. How would you optimize Ajax requests for better performance?
This question assesses a candidate's knowledge of performance optimization. Strong answers might include:
- Minimizing the amount of data transferred by using JSON instead of XML
- Implementing caching strategies to reduce server requests
- Using compression techniques like GZIP
- Batching multiple requests into a single call where possible
- Implementing debouncing or throttling for frequently triggered requests
Look for candidates who understand the balance between frontend and backend optimizations. The best responses will show awareness of both network performance and user experience considerations.
6. How would you ensure accessibility in an Ajax-heavy application?
This question evaluates a candidate's awareness of web accessibility. Good answers might include:
- Using ARIA attributes to indicate dynamic content changes
- Ensuring keyboard navigation for Ajax-loaded content
- Providing visual feedback for loading states
- Using semantic HTML in dynamically loaded content
- Testing with screen readers and other assistive technologies
Strong candidates will demonstrate an understanding of the unique challenges Ajax poses for accessibility and show knowledge of best practices to address these issues. Look for responses that prioritize inclusive design principles.
7. How would you debug an Ajax request that's not working as expected?
This question assesses a candidate's troubleshooting skills. A comprehensive answer might include:
- Using browser developer tools to inspect network requests
- Checking for JavaScript console errors
- Verifying the correct URL and parameters in the Ajax call
- Testing the API endpoint separately using tools like Postman
- Adding logging or breakpoints in the Ajax callbacks
Look for candidates who demonstrate a systematic approach to debugging. The best answers will show familiarity with various debugging tools and techniques, indicating strong problem-solving skills in a real-world development environment.
20 Ajax interview questions to ask junior developers
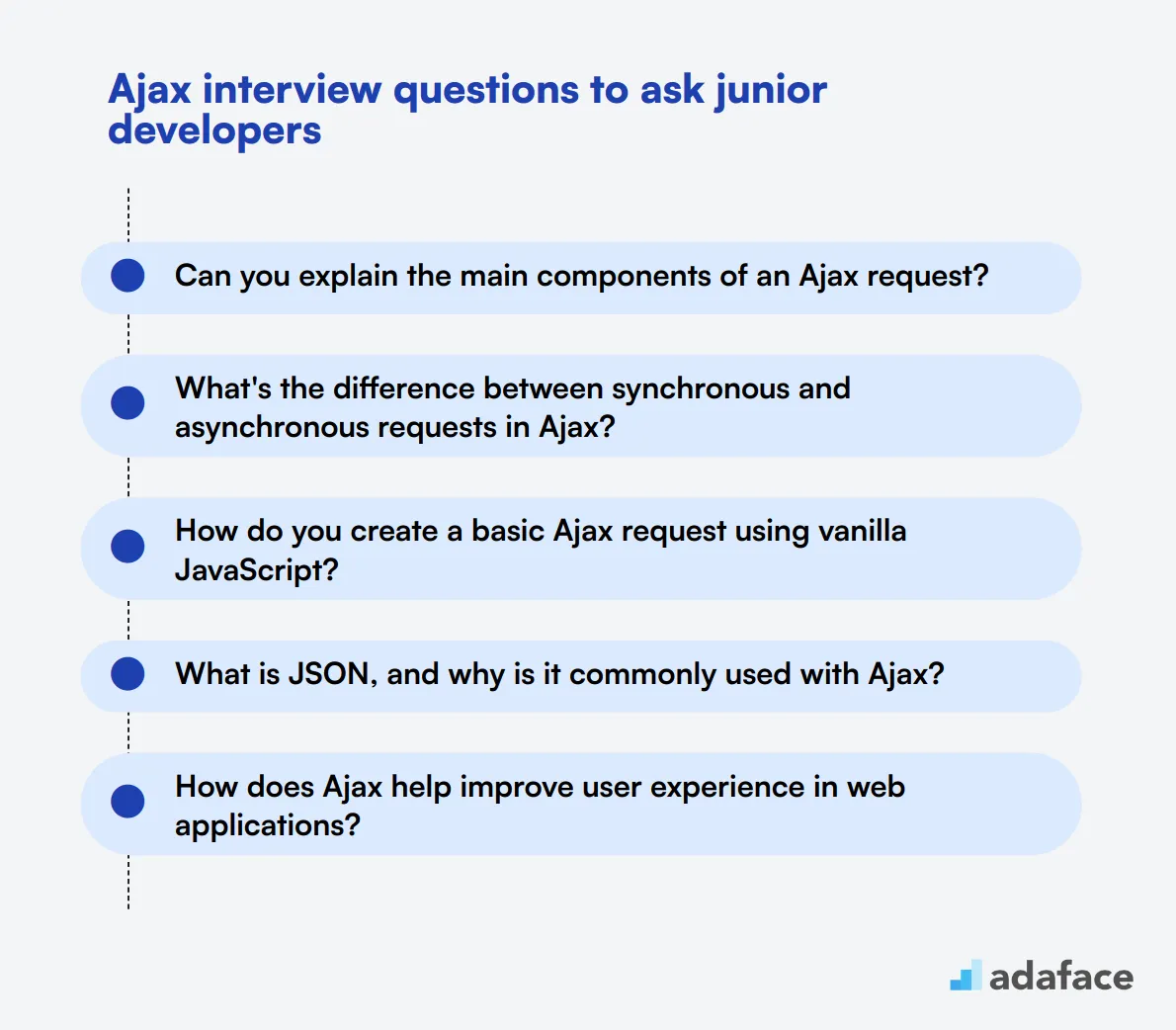
To assess a junior JavaScript developer's understanding of Ajax, use these 20 interview questions. They cover fundamental concepts and practical applications, helping you gauge candidates' readiness for Ajax-related tasks in web development.
- Can you explain the main components of an Ajax request?
- What's the difference between synchronous and asynchronous requests in Ajax?
- How do you create a basic Ajax request using vanilla JavaScript?
- What is JSON, and why is it commonly used with Ajax?
- How does Ajax help improve user experience in web applications?
- Can you explain the same-origin policy and its relevance to Ajax?
- What are the key differences between GET and POST methods in Ajax requests?
- How would you handle cross-domain Ajax requests?
- What is JSONP, and when would you use it?
- Can you describe the role of the XMLHttpRequest object in Ajax?
- How do you parse the response from an Ajax request?
- What are some security considerations when working with Ajax?
- How does Ajax interact with browser history and bookmarking?
- Can you explain the concept of Ajax polling?
- What are some alternatives to Ajax for real-time web applications?
- How would you implement form submission using Ajax?
- What is the purpose of the readyState property in an XMLHttpRequest object?
- How do you handle timeouts in Ajax requests?
- Can you explain the concept of Ajax caching?
- What are some best practices for organizing Ajax code in larger applications?
10 intermediate Ajax interview questions and answers to ask mid-tier developers
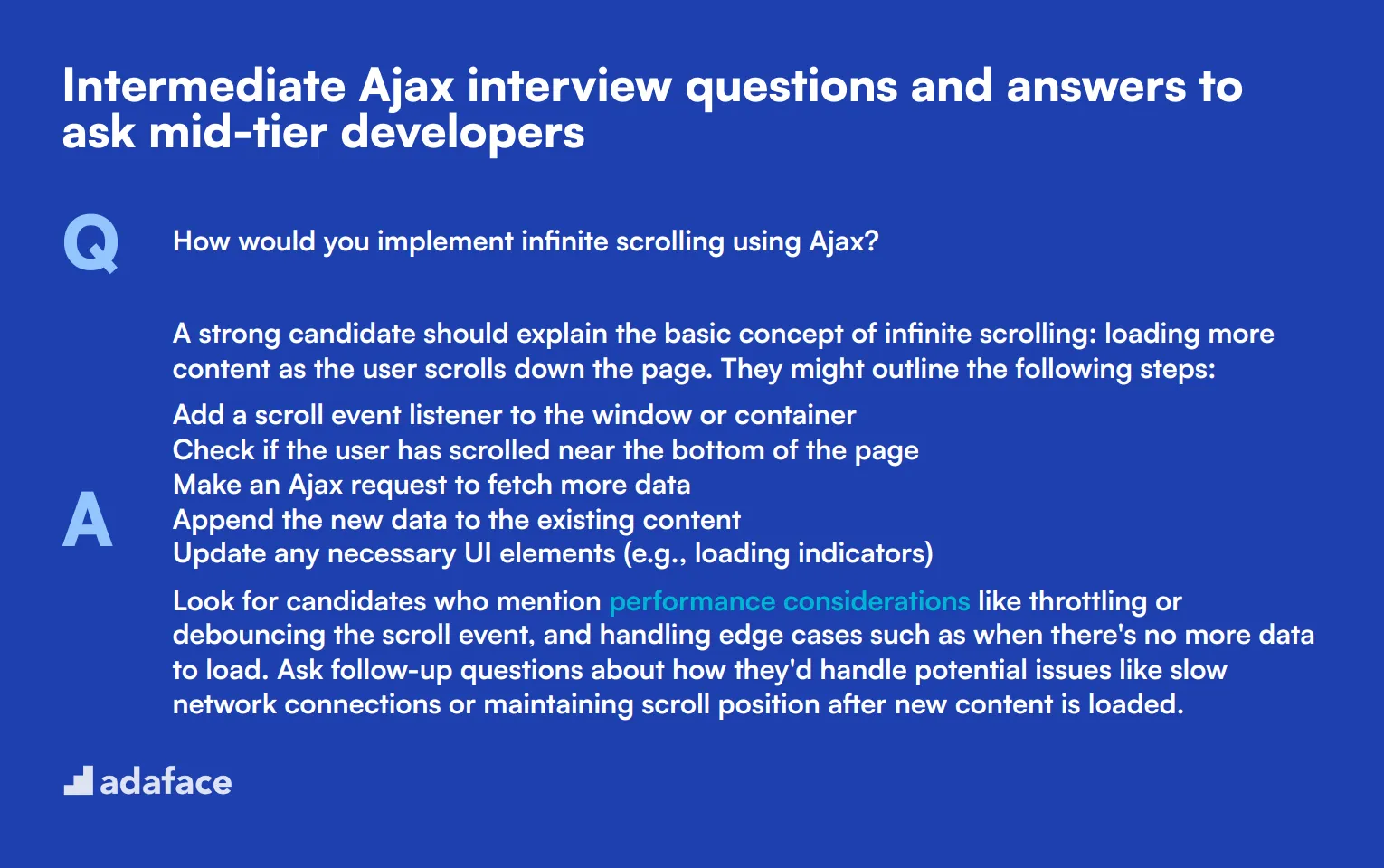
Ready to level up your Ajax interview game? These 10 intermediate questions are perfect for assessing mid-tier developers. They'll help you gauge a candidate's practical knowledge and problem-solving skills without diving too deep into technical jargon. Use these questions to spark insightful discussions and get a clearer picture of your potential hire's Ajax expertise.
1. How would you implement infinite scrolling using Ajax?
A strong candidate should explain the basic concept of infinite scrolling: loading more content as the user scrolls down the page. They might outline the following steps:
- Add a scroll event listener to the window or container
- Check if the user has scrolled near the bottom of the page
- Make an Ajax request to fetch more data
- Append the new data to the existing content
- Update any necessary UI elements (e.g., loading indicators)
Look for candidates who mention performance considerations like throttling or debouncing the scroll event, and handling edge cases such as when there's no more data to load. Ask follow-up questions about how they'd handle potential issues like slow network connections or maintaining scroll position after new content is loaded.
2. Explain the concept of Ajax long polling and when you might use it.
Ajax long polling is a technique where the client makes an Ajax request to the server, and the server holds the connection open until new data is available or a timeout occurs. If the server doesn't have new data, it sends an empty response, and the client immediately makes a new request.
Candidates might mention use cases such as:
• Real-time chat applications • Live sports updates • Stock market tickers • Collaborative editing tools
Look for answers that discuss the pros and cons of long polling compared to other real-time techniques like WebSockets. Strong candidates might also mention strategies for handling timeouts and reconnecting, as well as potential server-side considerations for managing many open connections.
3. How would you implement a progress bar for file uploads using Ajax?
A good answer should outline the following steps:
- Use the FormData API to create a form data object
- Create an XMLHttpRequest object
- Set up event listeners for 'progress', 'load', and 'error' events
- In the progress event handler, calculate the percentage of the upload completed
- Update the progress bar UI based on this percentage
- Handle the completion or any errors in the respective event handlers
Look for candidates who mention using the xhr.upload.onprogress
event specifically for upload progress. They should also discuss potential challenges like handling large files, providing a smooth user experience, and fallback options for browsers that don't support the progress event.
4. What strategies would you use to prevent Ajax request flooding?
Candidates should discuss methods to limit the number of Ajax requests sent in a short period. Some strategies they might mention include:
• Debouncing: Delaying the execution of a function until after a certain amount of time has passed • Throttling: Limiting the rate at which a function can fire • Caching: Storing and reusing previous request results to avoid unnecessary calls • Request queuing: Managing a queue of requests and processing them sequentially • Using a 'cooldown' period between requests
Look for answers that demonstrate an understanding of the impact of frequent requests on both client and server performance. Strong candidates might also mention implementing rate limiting on the server side as an additional safeguard.
5. How would you handle session timeouts in an Ajax-heavy application?
A good answer should cover both detection and handling of session timeouts:
- Detection methods: • Checking server responses for session status • Using a client-side timer to estimate session expiration • Implementing a heartbeat mechanism to periodically check session status
- Handling timeouts: • Displaying a warning message to the user • Offering an option to extend the session without losing work • Automatically saving user progress • Gracefully logging out and redirecting to a login page
Look for candidates who consider user experience in their approach, such as providing ample warning before a timeout occurs. They should also mention security considerations, like ensuring sensitive data is not cached or exposed after a timeout.
6. Explain the concept of Ajax request batching and when you might use it.
Ajax request batching is the practice of combining multiple Ajax requests into a single request to reduce overhead and improve performance. Candidates should explain that this is particularly useful when an application needs to make many small, related requests.
Use cases might include:
• Fetching multiple user profiles • Updating several database records at once • Retrieving various pieces of data for a dashboard
Look for answers that discuss the trade-offs of batching, such as reduced number of HTTP requests versus potentially increased complexity in request handling. Strong candidates might also mention considerations like error handling for batched requests and how to balance batching with real-time data needs.
7. How would you implement a search-as-you-type feature using Ajax?
A solid answer should outline the following steps:
- Add an input event listener to the search field
- Implement debouncing to avoid excessive requests
- Make an Ajax request with the current input value
- Update the UI with search results
- Handle empty results and error states
Look for candidates who mention usability considerations like minimum character limits before searching, highlighting matched terms, and keyboard navigation for results. They should also discuss performance optimizations such as caching results and cancelling pending requests when new ones are made.
8. What approaches would you use to debug a complex Ajax-based single-page application?
Strong candidates should mention a variety of debugging techniques:
• Using browser developer tools to inspect network requests and responses • Implementing logging on both client and server sides • Using breakpoints and step-through debugging • Leveraging browser extensions for additional debugging capabilities • Implementing error tracking and reporting systems
Look for answers that demonstrate a systematic approach to isolating and identifying issues. Candidates should also mention the importance of reproducing bugs consistently and considering different environments (e.g., various browsers, devices) in their debugging process.
9. How would you optimize Ajax performance in a large-scale web application?
Candidates should discuss multiple optimization strategies, such as:
• Minimizing request payload size (e.g., using compression, sending only necessary data) • Implementing efficient caching strategies (browser caching, local storage) • Using Content Delivery Networks (CDNs) for static assets • Optimizing server-side processing and database queries • Implementing pagination or infinite scrolling for large data sets • Using Ajax request batching where appropriate
Look for answers that consider both front-end and back-end optimizations. Strong candidates might also mention tools for performance profiling and the importance of setting and monitoring performance metrics.
10. Explain how you would implement offline support in an Ajax-based application.
A comprehensive answer should cover the following aspects:
- Using the Service Worker API to cache resources and intercept network requests
- Implementing local storage or IndexedDB to store data offline
- Syncing local changes with the server when the connection is restored
- Providing clear UI indicators for online/offline status
- Handling conflicts that may arise during sync
Look for candidates who discuss the challenges of offline support, such as managing data consistency and handling large datasets. They should also mention progressive enhancement and graceful degradation strategies to ensure the application remains functional for users who don't support offline features.
10 advanced Ajax interview questions to ask senior developers
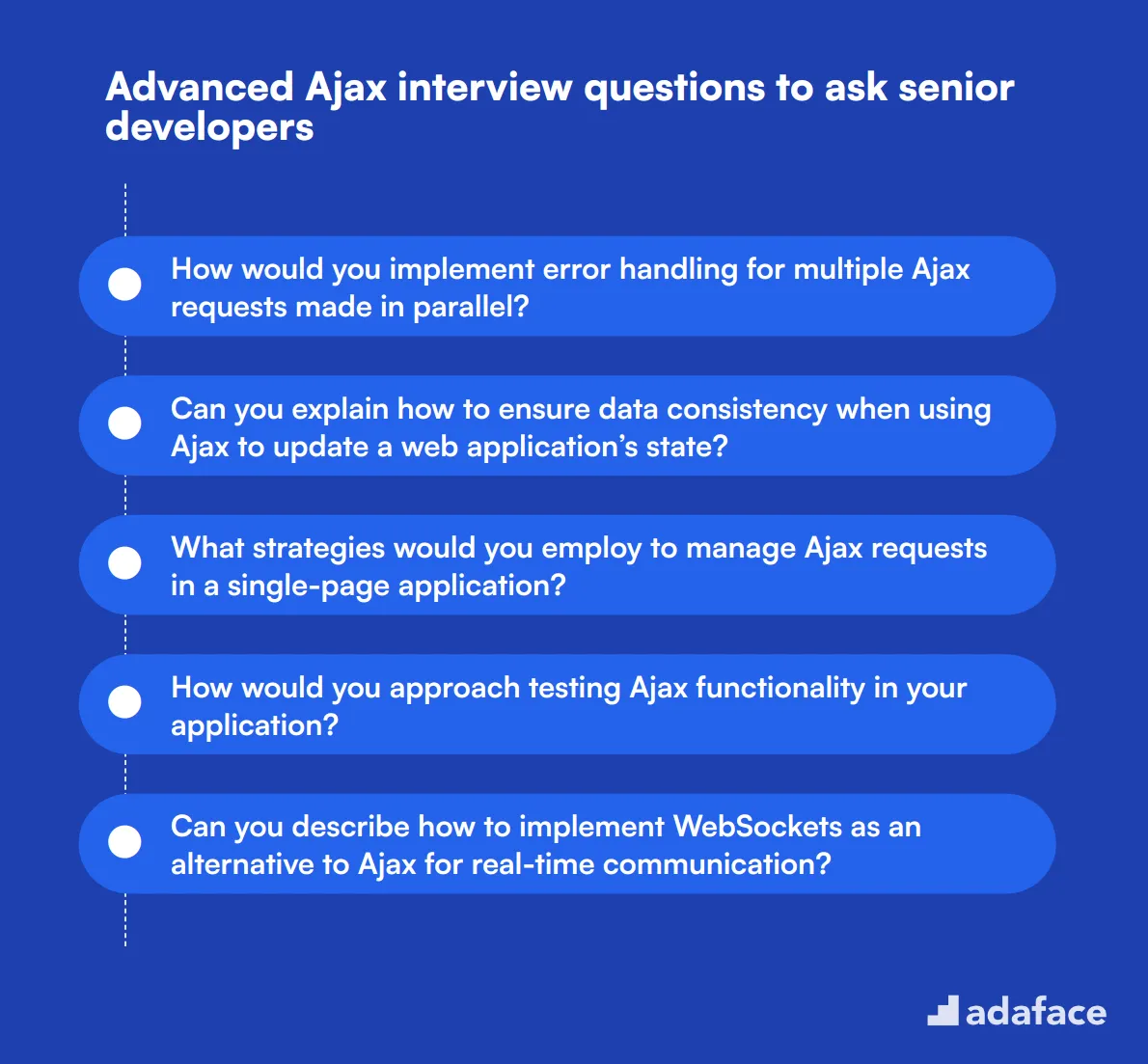
To gauge the advanced skills of candidates working with Ajax, consider using this list of interview questions. These inquiries can help you assess their understanding of complex functionalities, ensuring they have the right expertise for your team’s needs, especially when hiring for roles like a JavaScript developer.
- How would you implement error handling for multiple Ajax requests made in parallel?
- Can you explain how to ensure data consistency when using Ajax to update a web application’s state?
- What strategies would you employ to manage Ajax requests in a single-page application?
- How would you approach testing Ajax functionality in your application?
- Can you describe how to implement WebSockets as an alternative to Ajax for real-time communication?
- What are some techniques you would use to throttle or debounce Ajax calls?
- How would you manage user authentication in an Ajax-heavy application?
- Can you explain the importance of the Content-Type header in Ajax requests?
- What methods would you use to monitor the performance of Ajax requests in production?
- How would you handle loading states in a user interface during an Ajax request?
8 Ajax interview questions and answers related to XHR
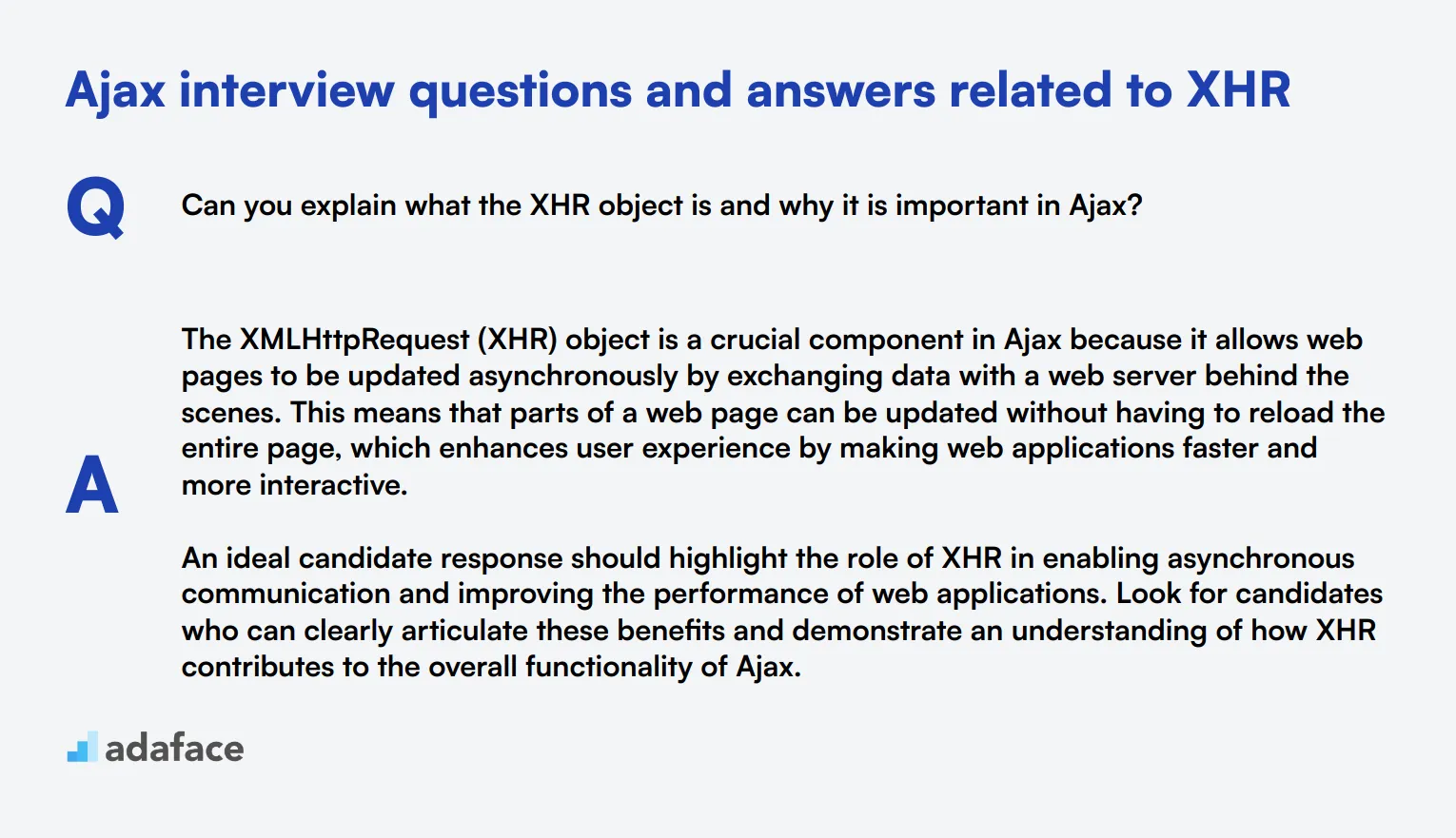
To determine whether your candidates have a strong understanding of XHR in Ajax, ask them some of these essential interview questions. These questions will help you gauge their knowledge and experience, ensuring they can handle the complexities of Ajax requests effectively.
1. Can you explain what the XHR object is and why it is important in Ajax?
The XMLHttpRequest (XHR) object is a crucial component in Ajax because it allows web pages to be updated asynchronously by exchanging data with a web server behind the scenes. This means that parts of a web page can be updated without having to reload the entire page, which enhances user experience by making web applications faster and more interactive.
An ideal candidate response should highlight the role of XHR in enabling asynchronous communication and improving the performance of web applications. Look for candidates who can clearly articulate these benefits and demonstrate an understanding of how XHR contributes to the overall functionality of Ajax.
2. How do you initiate an XHR request and what are the key steps involved?
To initiate an XHR request, you generally follow these steps: create a new instance of the XMLHttpRequest object, configure it with the desired HTTP method and URL, set any required request headers, define a callback function to handle the response, and finally, send the request.
Candidates should describe these steps in a clear and logical sequence. They should mention the importance of configuring the request correctly and handling the server's response appropriately. Look for candidates who can confidently explain each step and understand the purpose of each action in the process.
3. What are the common HTTP methods used with XHR, and when would you use each?
The two most common HTTP methods used with XHR are GET and POST. The GET method is typically used to retrieve data from a server, as it appends the request parameters to the URL, making it suitable for simple requests. The POST method, on the other hand, is used to send data to the server, especially when dealing with large amounts of data or more complex operations, as it sends the data in the body of the request.
An ideal response should explain the primary use cases for GET and POST methods in the context of XHR. Candidates should demonstrate their understanding of when to use each method based on the nature of the data being transmitted and the requirements of the task at hand.
4. How can you handle different response types in an XHR request?
In an XHR request, you can handle different response types by specifying the responseType
property of the XMLHttpRequest object. Common response types include 'text' for plain text, 'json' for JSON data, 'document' for XML documents, and 'blob' for binary data. Setting the appropriate response type ensures that the data is automatically parsed and available in the desired format.
Candidates should mention the importance of setting the responseType
property correctly to avoid data parsing issues. Look for answers that demonstrate a clear understanding of different response types and their use cases, as well as the ability to handle various data formats effectively.
5. What is the purpose of the `readyState` property in an XHR object?
The readyState
property of an XHR object provides the current state of the XMLHttpRequest. It can have values ranging from 0 to 4, where 0 indicates the request is not initialized, and 4 indicates the request is complete, and the response is ready. Monitoring the readyState
property helps in determining the progress of the request and taking appropriate actions at each stage.
A strong candidate should be able to explain the different values of the readyState
property and their significance. They should also emphasize the importance of checking the readyState
in conjunction with the status
property to ensure that the request has succeeded before processing the response.
6. How would you handle timeouts in an XHR request?
To handle timeouts in an XHR request, you can set the timeout
property of the XMLHttpRequest object to specify the maximum time (in milliseconds) the request should take. If the request exceeds this time, the ontimeout
event handler is triggered, allowing you to manage the timeout scenario, such as retrying the request or providing feedback to the user.
Candidates should discuss the importance of setting appropriate timeout values based on the expected response time from the server. They should also mention strategies for handling timeouts, like retry mechanisms or user notifications. Look for responses that show a proactive approach to managing network-related issues.
7. What are some common security considerations when using XHR in Ajax?
When using XHR in Ajax, common security considerations include protecting against cross-site scripting (XSS) attacks, ensuring that sensitive data is transmitted over secure protocols (HTTPS), and implementing proper authentication and authorization mechanisms. Additionally, attention should be given to handling cross-origin requests securely by using CORS (Cross-Origin Resource Sharing) headers.
An ideal candidate should demonstrate awareness of these security issues and suggest practical measures to mitigate them. Look for candidates who can provide examples of securing XHR requests and discuss best practices for maintaining the security of Ajax-based applications.
8. How can you monitor the performance of XHR requests in production?
To monitor the performance of XHR requests in production, you can use browser developer tools to analyze network activity, measure request and response times, and identify performance bottlenecks. Additionally, integrating performance monitoring tools and services can provide real-time insights and alerts on the performance of Ajax requests.
Candidates should mention the importance of continuous monitoring and performance optimization. Look for responses that demonstrate knowledge of specific tools and techniques for tracking and improving the performance of XHR requests in a live environment.
9 Ajax interview questions and answers related to callback functions
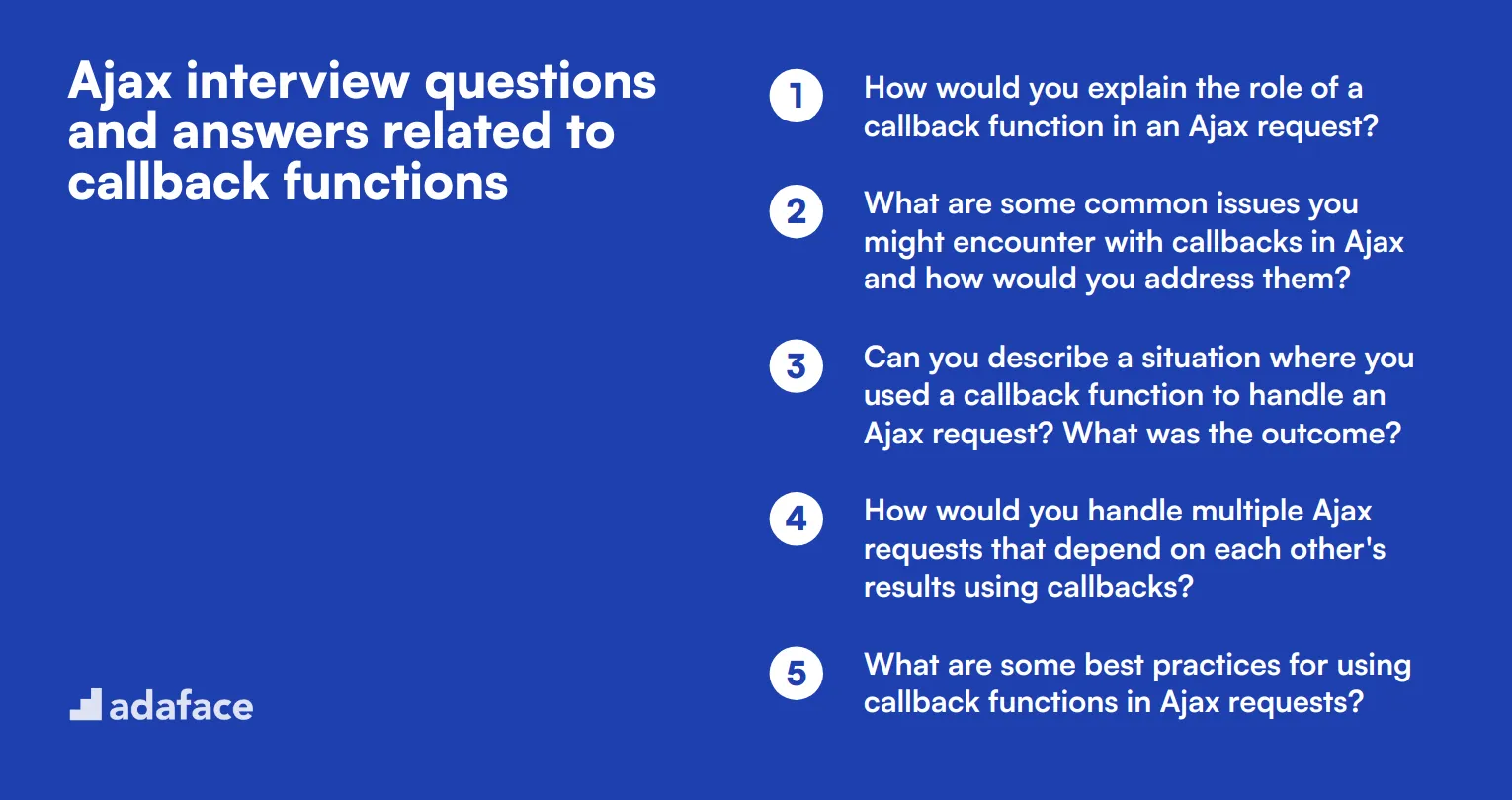
To gauge a candidate's understanding of callback functions within Ajax, these questions are perfect for your next interview. Use this list to pinpoint applicants who can effectively handle asynchronous operations and improve user experiences.
1. How would you explain the role of a callback function in an Ajax request?
A callback function in an Ajax request is a function that gets called once the server response is received. It allows for the processing of the server response after the request is completed, ensuring that the application can handle data dynamically without reloading the entire page.
Look for candidates who can explain this concept clearly and provide examples of how callbacks help in managing asynchronous operations in web applications.
2. What are some common issues you might encounter with callbacks in Ajax and how would you address them?
Common issues with callbacks in Ajax include callback hell, where nested callbacks become difficult to manage, and error handling within callbacks. Callback hell can be mitigated by using promises or async/await patterns to make the code more readable and manageable.
Candidates should demonstrate an understanding of these issues and propose practical solutions. Look for an explanation of how to handle nested callbacks and manage errors effectively.
3. Can you describe a situation where you used a callback function to handle an Ajax request? What was the outcome?
A good example might involve using a callback to update a user interface element after fetching data from a server. For instance, updating a list of items without reloading the page, thereby providing a seamless user experience.
Ideal candidates should provide a specific example, demonstrating their practical experience with Ajax and callback functions. They should also discuss the positive impact on user experience.
4. How would you handle multiple Ajax requests that depend on each other's results using callbacks?
Handling multiple dependent Ajax requests with callbacks usually involves nesting callbacks, where the callback of one request triggers another Ajax request. This ensures that each request waits for the previous one to complete before proceeding.
Look for candidates who suggest alternatives to nesting, such as using promises or async/await, to avoid callback hell and make the code more maintainable.
5. What are some best practices for using callback functions in Ajax requests?
Some best practices include keeping callbacks simple and focused, handling errors appropriately within callbacks, and avoiding deeply nested callbacks by using promises or async/await. It's also important to ensure that the code remains readable and maintainable.
Strong candidates should be able to list these practices and explain their importance. They might also provide examples of how these practices improve code quality and maintainability.
6. How would you implement a retry mechanism for a failed Ajax request using callbacks?
A retry mechanism can be implemented by including logic within the callback to check for a failure response and then re-invoking the Ajax request a specified number of times before giving up. This ensures robustness in the face of transient network issues.
Candidates should describe the logic clearly and mention the importance of setting a limit on retries to avoid infinite loops. This showcases their ability to handle failures gracefully.
7. Can you explain how to manage the execution order of multiple callback functions in Ajax?
Managing the execution order can be achieved by chaining callbacks or using techniques like promises or async/await to ensure that each callback waits for the previous one to complete. This control flow management is crucial in scenarios where the execution order impacts the application's functionality.
Look for explanations that include practical examples or scenarios. Candidates should highlight how managing execution order improves reliability and predictability in web applications.
8. What strategies can you use to avoid callback hell in Ajax-heavy applications?
To avoid callback hell, developers can use promises or async/await to flatten the code structure and make it more readable. Another strategy is to modularize the code by breaking down large callbacks into smaller, reusable functions.
Ideal candidates will not only mention these strategies but also provide insights into how they have applied them in real-world projects. This demonstrates their practical knowledge and experience.
9. How would you handle error propagation in a series of nested callbacks in Ajax?
Handling error propagation involves catching errors at each level of the callback chain and passing the error information up to a central error handler. This ensures that errors are not swallowed and can be dealt with appropriately at a higher level in the application.
Candidates should discuss the importance of error handling and provide examples of how they have implemented robust error propagation mechanisms in their projects. This indicates their ability to write resilient code.
Which Ajax skills should you evaluate during the interview phase?
Assessing a candidate's Ajax skills in a single interview can be challenging. However, there are core skills that are crucial to evaluate to ensure the candidate has the right abilities.
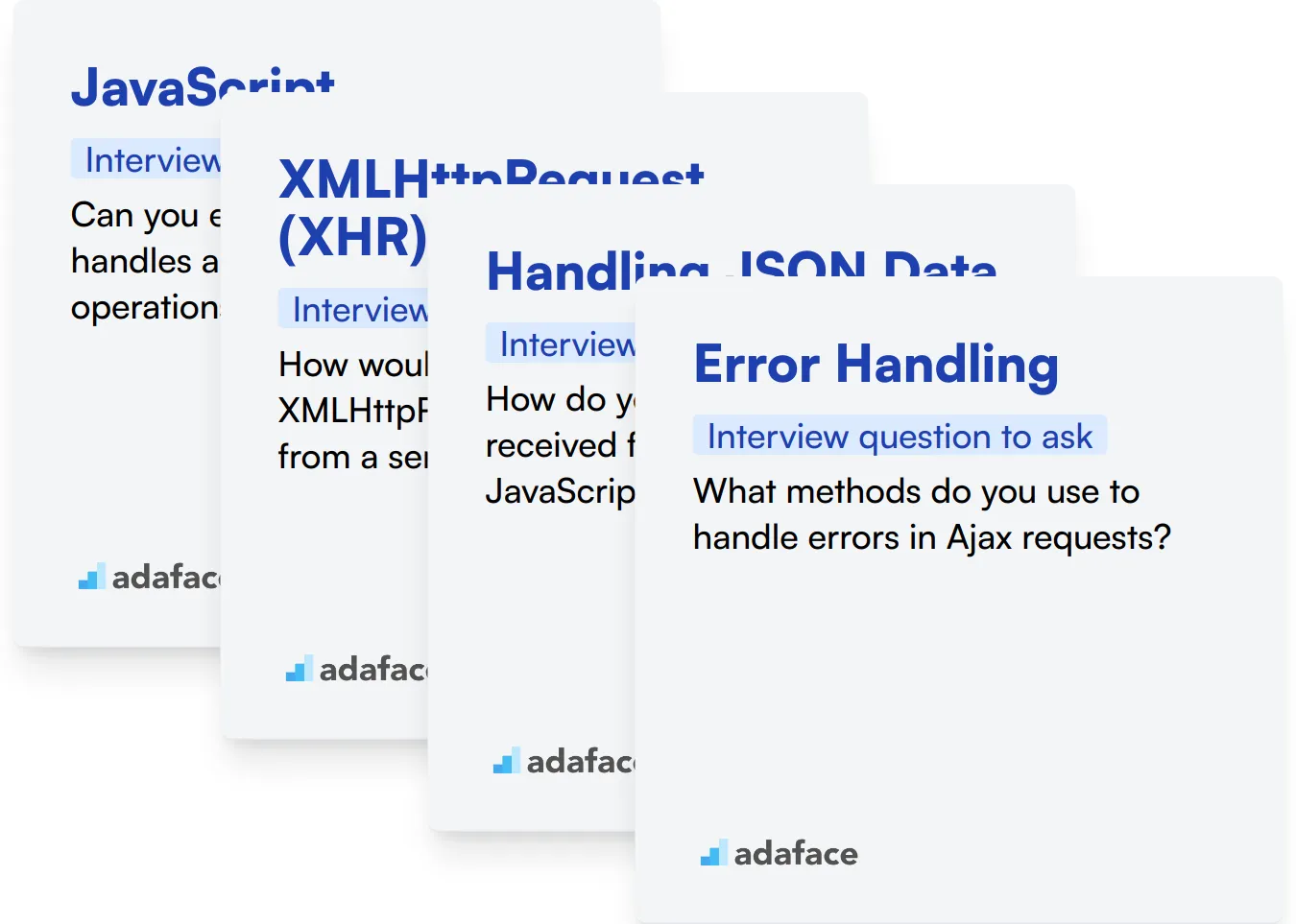
JavaScript
JavaScript is the backbone of Ajax. It’s necessary for dynamically updating web pages and handling asynchronous requests.
You can use an assessment test that includes relevant MCQs to filter out JavaScript skills. The JavaScript test in our library can be beneficial.
To further evaluate, ask targeted JavaScript questions during the interview to judge the candidate’s competency.
Can you explain how JavaScript handles asynchronous operations?
Look for an understanding of callback functions, promises, and async/await syntax.
XMLHttpRequest (XHR)
Understanding XHR is essential for executing Ajax calls, as it allows the browser to communicate with the server without refreshing the page.
Ask targeted questions about XHR to gauge the candidate’s familiarity with its methods and properties.
How would you use XMLHttpRequest to fetch data from a server?
Check if the candidate knows about methods like open(), send(), and handling response with onreadystatechange.
Handling JSON Data
JSON is often used with Ajax to exchange data between the client and server. Proficiency in handling JSON is critical for effective Ajax implementations.
You can use an assessment test to evaluate JSON skills. The JSON test in our library includes relevant questions.
Ask questions about manipulating JSON data to assess the candidate’s ability.
How do you parse JSON data received from an Ajax request in JavaScript?
Look for knowledge on JSON.parse() for converting JSON strings into JavaScript objects.
Error Handling
Robust error handling ensures that applications gracefully recover from failed Ajax requests, providing a better user experience.
Evaluate their understanding by asking about error handling strategies in Ajax.
What methods do you use to handle errors in Ajax requests?
Check if they mention try-catch blocks, handling HTTP status codes, and displaying user-friendly error messages.
Streamline Your Hiring with Ajax Skills Tests and Targeted Interview Questions
If you're aiming to hire professionals with Ajax skills, it's important to ensure they genuinely possess the necessary capabilities. Accurate assessment of these skills is the first step.
The most effective way to evaluate Ajax capabilities is through specialized skills tests. Consider using tests like our JavaScript Online Test or JavaScript Node React Test to gauge their proficiency.
After administering these tests, you can efficiently shortlist candidates who meet your criteria. This process allows you to save time by only interviewing the most qualified applicants.
For the next steps in your hiring process, invite your top candidates to interviews. Get started by signing up on our Dashboard or explore more about our platform on the Online Assessment Platform page.
JavaScript Online Test
Download Ajax interview questions template in multiple formats
Ajax Interview Questions FAQs
The article covers general, junior, intermediate, advanced, XHR-related, and callback function-related Ajax interview questions.
There are 20 Ajax interview questions specifically tailored for junior developers.
Yes, the article includes questions for junior, intermediate, and senior developers, as well as general Ajax questions.
Yes, answers are provided for many of the questions, particularly in the general, intermediate, XHR-related, and callback function sections.
These questions can help assess candidates' Ajax knowledge and skills, allowing interviewers to make informed hiring decisions based on technical proficiency.
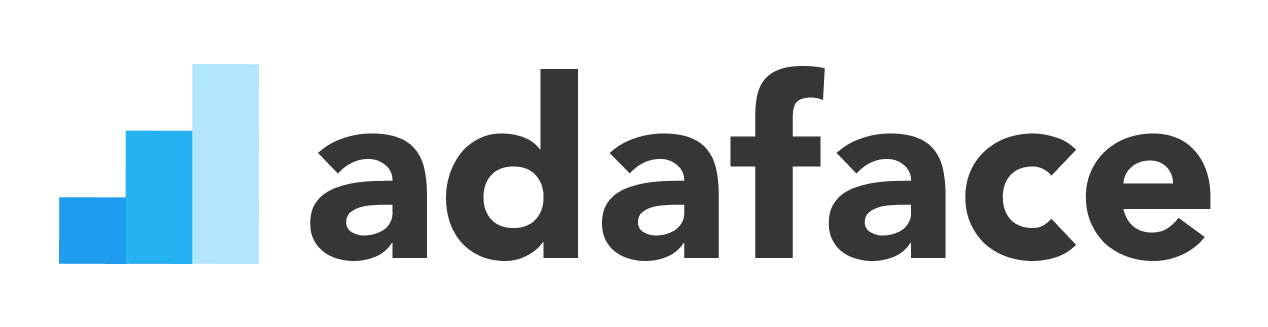
40 min skill tests.
No trick questions.
Accurate shortlisting.
We make it easy for you to find the best candidates in your pipeline with a 40 min skills test.
Try for freeRelated posts
Free resources
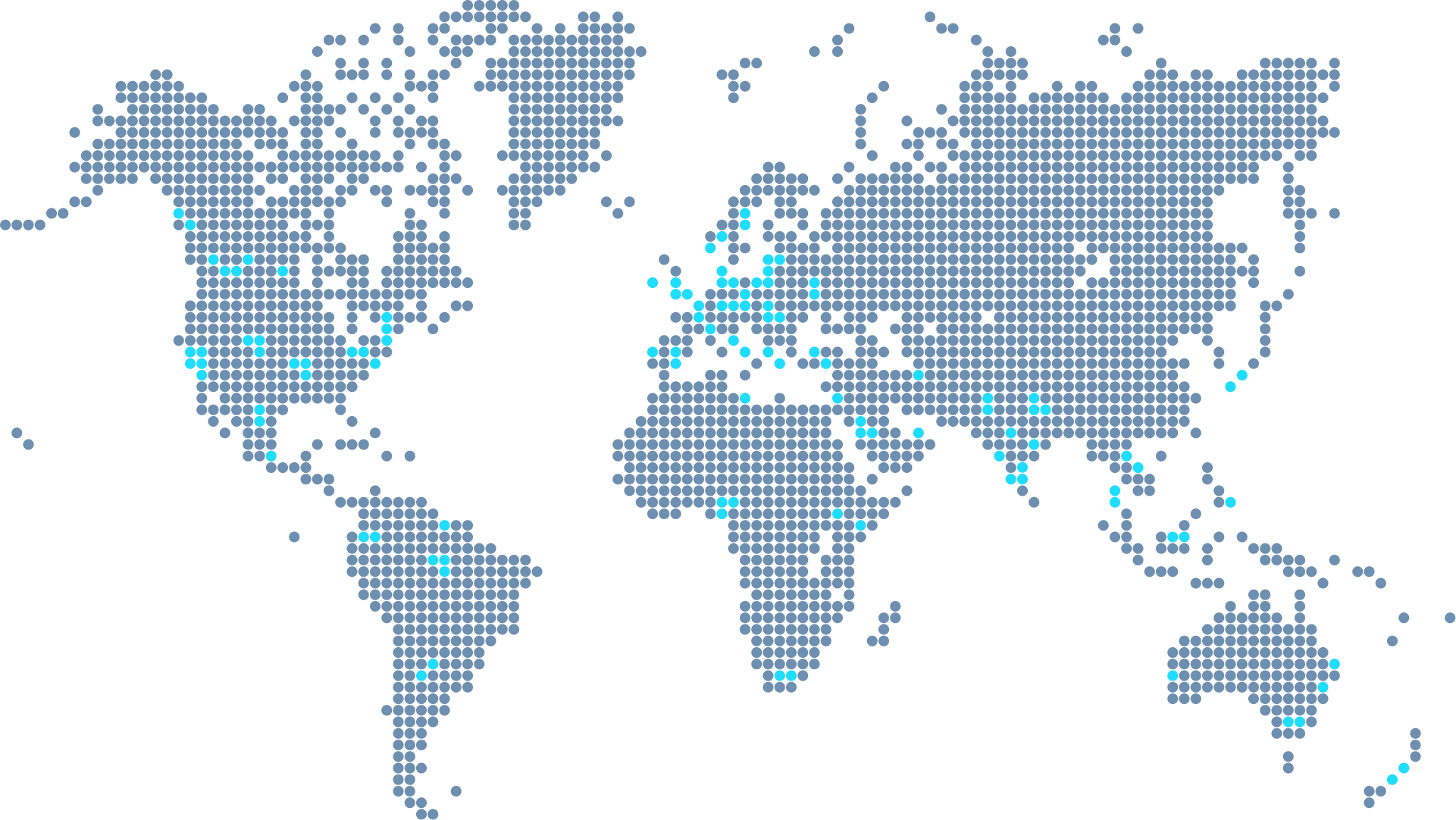
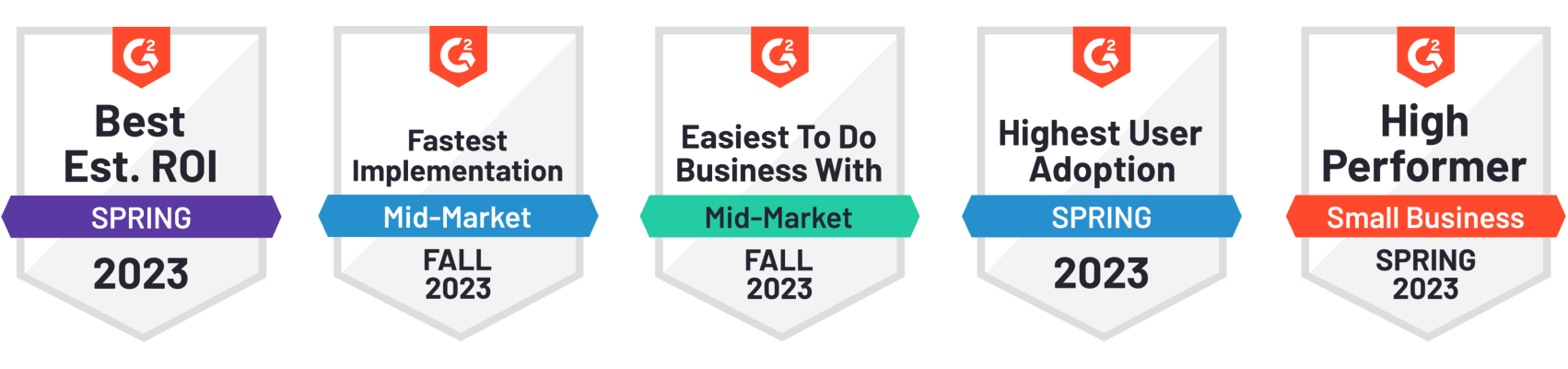